
AhmadLang / Java, How To Program, 2004
.pdf
[Page 120 (continued)]
Answers to Self-Review Exercises
3.1 a) object. b) public. c) class. d) new. e) type, name. f) default package. g) instance variable. h) float, double. i) double-precision. j) nexTDouble. k) access modifier. l) void. m) nextLine. n) java.lang. o) import declaration. p) floating-point number. q) single-precision. r) %f. s) primitive, reference.
3.2 a) False. By convention, method names begin with a lowercase first letter and all subsequent words in the name begin with a capital first letter. b) True. c) True. d) True. e) False. A primitive-type variable cannot be used to invoke a methoda reference to an object is required to invoke the object's methods. f) False. Such variables are called local variables and can be used only in the method in which they are declared. g) True. h) False. Primitive-type instance variables are initialized by default. i) True. j) True. k) True. l) False. Such literals are of type double by default.
[Page 121]
3.3 A local variable is declared in the body of a method and can be used only from the point at which it is declared through the end of the method declaration. A field is declared in a class, but not in the body of any of the class's methods. Every object (instance) of a class has a separate copy of the class's fields. Also, fields are accessible to all methods of the class. (We will see an exception to this in Chapter 8, Classes and Objects: A Deeper Look.)
3.4 A parameter represents additional information that a method requires to perform its task. Each parameter required by a method is specified in the method's declaration. An argument is the actual value for a method parameter. When a method is called, the argument values are passed to the method so that it can perform its task.

[Page 121 (continued)]
Exercises
3.5What is the purpose of keyword new? Explain what happens when this keyword is used in an application.
3.6What is a default constructor? How are an object's instance variables initialized if a class has only a default constructor?
3.7Explain the purpose of an instance variable.
3.8Most classes need to be imported before they can be used in an application. Why is every application allowed to use classes System and String without first importing them?
3.9Explain how a program could use class Scanner without importing the class from package java.util.
3.10Explain why a class might provide a set method and a get method for an instance variable.
3.11Modify class GradeBook (Fig. 3.10) as follows:
a.Include a second String instance variable that represents the name of the course's instructor.
b.Provide a set method to change the instructor's name and a get method to retrieve it.
c.Modify the constructor to specify two parametersone for the course name and one for the instructor's name.
d.Modify method displayMessage such that it first outputs the welcome message and course name, then outputs "This course is presented by: " followed by the instructor's name.
Use your modified class in a test application that demonstrates the class's new capabilities.
3.12Modify class Account (Fig. 3.13) to provide a method called debit that withdraws money from an Account. Ensure that the debit amount does not exceed the Account's balance. If it does, the balance should be left unchanged and the method should print a message indicating "Debit amount exceeded account balance."
Modify class AccountTest (Fig. 3.14) to test method debit.
3.13Create a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as instance variablesa part number (type String), a part description (type String), a quantity of the item being purchased (type int) and a price per item (double). Your class should have a constructor that initializes the four instance variables. Provide a set and a get method for each instance variable. In addition, provide a method named getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by the price per item), then returns the amount as a double value. If the quantity is not positive, it should be set to 0. If the price per item is not positive, it should be set to 0.0. Write a test application named InvoiceTest that demonstrates class Invoice's capabilities.
3.14Create a class called Employee that includes three pieces of information as instance variablesa first name (type String), a last name (type String) and a monthly salary

(double). Your class should have a constructor that initializes the three instance variables. Provide a set and a get method for each instance variable. If the monthly salary is not positive, set it to 0.0. Write a test application named EmployeeTest that demonstrates class Employee's capabilities. Create two Employee objects and display each object's yearly salary. Then give each Employee a 10% raise and display each Employee's yearly salary again.
[Page 122]
3.15Create a class called Date that includes three pieces of information as instance variablesa month (type int), a day (type int) and a year (type int). Your class should have a constructor that initializes the three instance variables and assumes that the values provided are correct. Provide a set and a get method for each instance variable. Provide a method displayDate that displays the month, day and year separated by forward slashes (/). Write a test application named DateTest that demonstrates class Date's capabilities.

[Page 123]
Chapter 4. Control Statements: Part I
Let's all move one place on.
Lewis Carroll
The wheel is come full circle.
William Shakespeare
How many apples fell on Newton's head before he took the hint!
Robert Frost
All the evolution we know of proceeds from the vague to the definite.
Charles Sanders Peirce
OBJECTIVES
In this chapter you will learn:
To use basic problem-solving techniques.
To develop algorithms through the process of top-down, stepwise refinement.
To use the if and if...else selection statements to choose among alternative actions.
To use the while repetition statement to execute statements in a program repeatedly.
To use counter-controlled repetition and sentinel-controlled repetition.
To use the assignment, increment and decrement operators.
[Page 124]
Outline
4.1 Introduction
4.2 Algorithms
4.3 Pseudocode
4.4 Control Structures
4.5 if Single-Selection Statement
4.6 if...else Double-Selection Statement
4.7 while Repetition Statement
4.8 Formulating Algorithms: Counter-Controlled Repetition
4.9 Formulating Algorithms: Sentinel-Controlled Repetition
4.10 Formulating Algorithms: Nested Control Statements

4.11 Compound Assignment Operators
4.12 Increment and Decrement Operators
4.13 Primitive Types
4.14 (Optional) GUI and Graphics Case Study: Creating Simple Drawings
4.15 (Optional) Software Engineering Case Study: Identifying Class Attributes
4.16 Wrap-Up
Summary
Terminology
Self-Review Exercises
Answers to Self-Review Exercises
Exercises
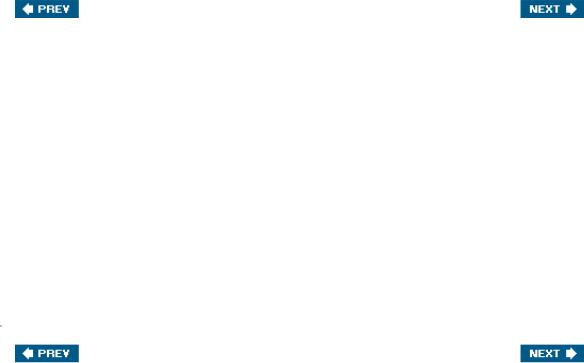
[Page 124 (continued)]
4.1. Introduction
Before writing a program to solve a problem, it is essential to have a thorough understanding of the problem and a carefully planned approach to solving it. When writing a program, it is also essential to understand the types of building blocks that are available and to employ proven program-construction techniques. In this chapter and in Chapter 5, Control Statements: Part 2, we discuss these issues in our presentation of the theory and principles of structured programming. The concepts presented here are crucial in building classes and manipulating objects.
In this chapter, we introduce Java's if, if...else and while statements, three of the building blocks that allow programmers to specify the logic required for methods to perform their tasks. We devote a portion of this chapter (and Chapters 5 and 7) to further developing the GradeBook class introduced in Chapter 3. In particular, we add a method to the GradeBook class that uses control statements to calculate the average of a set of student grades. Another example demonstrates additional ways to combine control statements to solve a similar problem. We introduce Java's compound assignment operators and explore Java's increment and decrement operators. These additional operators abbreviate and simplify many program statements. Finally, we present an overview of the primitive data types available to programmers.
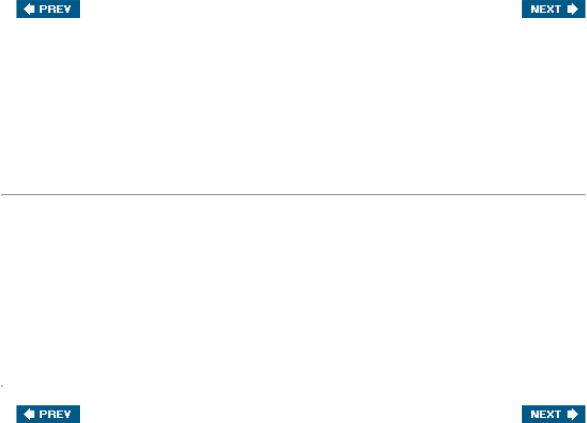
[Page 124 (continued)]
4.2. Algorithms
Any computing problem can be solved by executing a series of actions in a specific order. A procedure for solving a problem in terms of
1.the actions to execute and
2.the order in which these actions execute
[Page 125]
is called an algorithm. The following example demonstrates that correctly specifying the order in which the actions execute is important.
Consider the "rise-and-shine algorithm" followed by one executive for getting out of bed and going to work: (1) Get out of bed; (2) take off pajamas; (3) take a shower; (4) get dressed; (5) eat breakfast;
(6) carpool to work. This routine gets the executive to work well prepared to make critical decisions. Suppose that the same steps are performed in a slightly different order: (1) Get out of bed; (2) take off pajamas; (3) get dressed; (4) take a shower; (5) eat breakfast; (6) carpool to work. In this case, our executive shows up for work soaking wet.
Specifying the order in which statements (actions) execute in a program is called program control. This chapter investigates program control using Java's control statements.
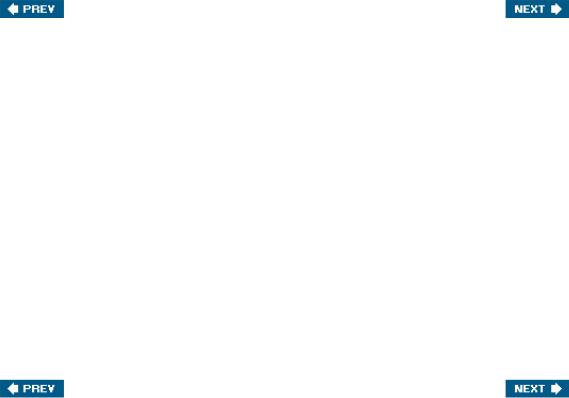
[Page 125 (continued)]
4.3. Pseudocode
Pseudocode is an informal language that helps programmers develop algorithms without having to worry about the strict details of Java language syntax. The pseudocode we present is particularly useful for developing algorithms that will be converted to structured portions of Java programs. Pseudocode is similar to everyday Englishit is convenient and user friendly, but it is not an actual computer programming language.
Pseudocode does not execute on computers. Rather, it helps the programmer "think out" a program before attempting to write it in a programming language, such as Java. This chapter provides several examples of how to use pseudocode to develop Java programs.
The style of pseudocode we present consists purely of characters, so programmers can type pseudocode conveniently, using any text-editor program. A carefully prepared pseudocode program can easily be converted to a corresponding Java program. In many cases, this simply requires replacing pseudocode statements with Java equivalents.
Pseudocode normally describes only statements representing the actions that occur after a programmer converts a program from pseudocode to Java and the program is run on a computer. Such actions might include input, output or a calculation. We typically do not include variable declarations in our pseudocode. However, some programmers choose to list variables and mention their purposes at the beginning of their pseudocode.
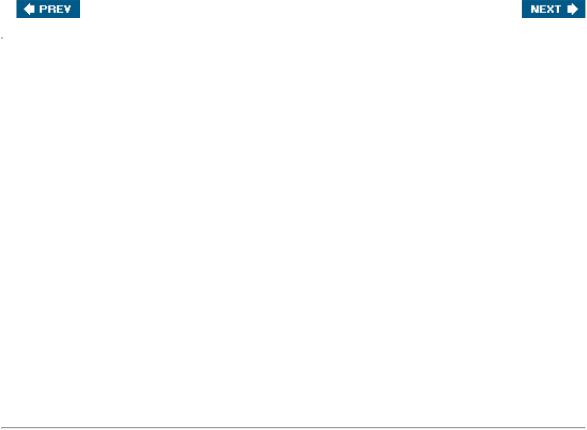
[Page 125 (continued)]
4.4. Control Structures
Normally, statements in a program are executed one after the other in the order in which they are written. This process is called sequential execution. Various Java statements, which we will soon discuss, enable the programmer to specify that the next statement to execute is not necessarily the next one in sequence. This is called transfer of control.
During the 1960s, it became clear that the indiscriminate use of transfers of control was the root of much difficulty experienced by software development groups. The blame was pointed at the goto statement (used in most programming languages of the time), which allows the programmer to specify a transfer of control to one of a very wide range of possible destinations in a program. The notion of so-called structured programming became almost synonymous with "goto elimination."
[Note: Java does not have a goto statement; however, the word goto is reserved by Java and should not be used as an identifier in programs.]
The research of Bohm and Jacopini[1] had demonstrated that programs could be written without any goto statements. The challenge of the era for programmers was to shift their styles to "goto-less programming." Not until the 1970s did programmers start taking structured programming seriously. The results were impressive. Software development groups reported shorter development times, more frequent on-time delivery of systems and more frequent within-budget completion of software projects. The key to these successes was that structured programs were clearer, easier to debug and modify, and more likely to be bug free in the first place.
[1] Bohm, C., and G. Jacopini, "Flow Diagrams, Turing Machines, and Languages with Only Two Formation Rules," Communications of the ACM, Vol. 9, No. 5, May 1966, pp. 336371.
[Page 126]
Bohm and Jacopini's work demonstrated that all programs could be written in terms of only three control structuresthe sequence structure, the selection structure and the repetition structure. The term "control structures" comes from the field of computer science. When we introduce Java's implementations of control structures, we will refer to them in the terminology of the Java Language Specification as "control statements."
Sequence Structure in Java
The sequence structure is built into Java. Unless directed otherwise, the computer executes Java statements one after the other in the order in which they are written, that is, in sequence. The activity diagram in Fig. 4.1 illustrates a typical sequence structure in which two calculations are performed in order. Java lets us have as many actions as we want in a sequence structure. As we will soon see, anywhere a single action may be placed, we may place several actions in sequence.
Figure 4.1. Sequence structure activity diagram.
(This item is displayed on page 127 in the print version)
[View full size image]

Activity diagrams are part of the UML. An activity diagram models the workflow (also called the activity) of a portion of a software system. Such workflows may include a portion of an algorithm, such as the sequence structure in Fig. 4.1. Activity diagrams are composed of special-purpose symbols, such as action-state symbols (rectangles with their left and right sides replaced with arcs curving outward), diamonds and small circles. These symbols are connected by transition arrows, which represent the flow of the activity, that is, the order in which the actions should occur
Like pseudocode, activity diagrams help programmers develop and represent algorithms, although many programmers prefer pseudocode. Activity diagrams clearly show how control structures operate.
Consider the activity diagram for the sequence structure in Fig. 4.1. It contains two action states that represent actions to perform. Each action state contains an action expressionfor example, "add grade to total" or "add 1 to counter"that specifies a particular action to perform. Other actions might include calculations or input/output operations. The arrows in the activity diagram represent transitions, which indicate the order in which the actions represented by the action states occur. The program that implements the activities illustrated by the diagram in Fig. 4.1 first adds grade to total, then adds 1 to
counter.
The solid circle located at the top of the activity diagram represents the activity's initial statethe beginning of the workflow before the program performs the modeled actions. The solid circle surrounded by a hollow circle that appears at the bottom of the diagram represents the final statethe end of the workflow after the program performs its actions.
Figure 4.1 also includes rectangles with the upper-right corners folded over. These are UML notes (like comments in Java)explanatory remarks that describe the purpose of symbols in the diagram. Figure 4.1 uses UML notes to show the Java code associated with each action state in the activity diagram. A dotted line connects each note with the element that the note describes. Activity diagrams normally do not show the Java code that implements the activity. We use notes for this purpose here to illustrate how the diagram relates to Java code. For more information on the UML, see our optional case study, which appears in the Software Engineering Case Study sections at the ends of Chapters 18 and 10, or visit www.uml.org.
[Page 127]
Selection Statements in Java
Java has three types of selection statements (discussed in this chapter and Chapter 5). The if statement either performs (selects) an action if a condition is true or skips the action, if the condition is false. The if...else statement performs an action if a condition is true and performs a different action if the condition is false. The switch statement (Chapter 5) performs one of many different actions, depending on the value of an expression.
The if statement is a single-selection statement because it selects or ignores a single action (or, as we will soon see, a single group of actions). The if...else statement is called a double-selection statement because it selects between two different actions (or groups of actions). The switch statement is called a multiple-selection statement because it selects among many different actions (or groups of actions).