
AhmadLang / Java, How To Program, 2004
.pdf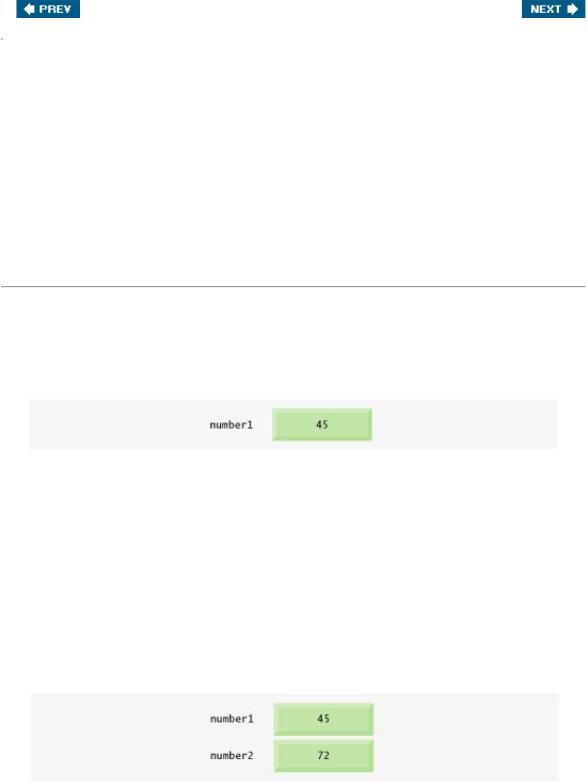
[Page 51 (continued)]
2.6. Memory Concepts
Variable names such as number1, number2 and sum actually correspond to locations in the computer's memory. Every variable has a name, a type, a size and a value.
In the addition program of Fig. 2.7, when the statement (line 18)
number1 = input.nextInt(); // read first number from user
executes, the number typed by the user is placed into a memory location to which the name number1 has been assigned by the compiler. Suppose that the user enters 45. The computer places that integer value into location number1, as shown in Fig. 2.8. Whenever a value is placed in a memory location, the value replaces the previous value in that location. The previous value is lost.
[Page 52]
Figure 2.8. Memory location showing the name and value of variable number1.
[View full size image]
When the statement (line 21)
number2 = input.nextInt(); // read second number from user
executes, suppose that the user enters 72. The computer places that integer value into location number2. The memory now appears as shown in Fig. 2.9.
Figure 2.9. Memory locations after storing values for number1 and number2.
[View full size image]
After the program of Fig. 2.7 obtains values for number1 and number2, it adds the values and places the sum into variable sum. The statement (line 23)

sum = number1 + number2; // add numbers
performs the addition, then replaces sum's previous value. After sum has been calculated, memory appears as shown in Fig. 2.10. Note that the values of number1 and number2 appear exactly as they did before they were used in the calculation of sum. These values were used, but not destroyed, as the computer performed the calculation. Thus, when a valueis read from a memory location, the process is nondestructive.
Figure 2.10. Memory locations after calculating and storing the sum of number1 and number2.
[View full size image]

[Page 52 (continued)]
2.7. Arithmetic
Most programs perform arithmetic calculations. The arithmetic operators are summarized in Fig. 2.11. Note the use of various special symbols not used in algebra. The asterisk (*) indicates multiplication, and the percent sign (%) is the remainder operator (called-modulus in some languages), which we will discuss shortly. The arithmetic operators in Fig. 2.11 are binary operators because they each operate on two operands. For example, the expression f + 7 contains the binary operator + and the two operands f and 7.
[Page 53]
Figure 2.11. Arithmetic operators.
|
Arithmetic |
|
|
|
|
Java operation |
operator |
Algebraic expression |
Java expression |
||
|
|
|
|
|
|
Addition |
+ |
f+7 |
f |
+ |
7 |
Subtraction |
- |
pc |
p |
- |
c |
Multiplication |
* |
bm |
b |
* |
m |
Division |
/ |
|
x |
/ |
y |
Remainder |
% |
r mod s |
r |
% |
s |
|
|
|
|
|
|
Integer division yields an integer quotientfor example, the expression 7 / 4 evaluates to 1, and the expression 17 / 5 evaluates to 3. Any fractional part in integer division is simply discarded (i.e., truncated)no rounding occurs. Java provides the remainder operator, %, which yields the remainder after division. The expression x % y yields the remainder after x is divided by y. Thus, 7 % 4 yields 3, and 17 % 5 yields 2. This operator is most commonly used with integer operands, but can also be used with other arithmetic types. In this chapter's exercises and in later chapters, we consider several interesting applications of the remainder operator, such as determining whether one number is a multiple of another.
Arithmetic expressions in Java must be written in straight-line form to facilitate entering programs into the computer. Thus, expressions such as "a divided by b" must be written as a / b, so that all constants, variables and operators appear in a straight line. The following algebraic notation is generally not acceptable to compilers:
Parentheses are used to group terms in Java expressions in the same manner as in algebraic expressions. For example, to multiply a times the quantity b + c, we write
If an expression contains nested parentheses, such as

the expression in the innermost set of parentheses (a + b in this case) is evaluated first.
Java applies the operators in arithmetic expressions in a precise sequence determined by the following rules of operator precedence, which are generally the same as those followed in algebra (Fig. 2.12):
1.Multiplication, division and remainder operations are applied first. If an expression contains several such operations, the operators are applied from left to right. Multiplication, division and remainder operators have the same level of precedence.
2.Addition and subtraction operations are applied next. If an expression contains several such operations, the operators are applied from left to right. Addition and subtraction operators have the same level of precedence.
|
|
[Page 54] |
|
Figure 2.12. Precedence of arithmetic operators. |
|||
Operator(s) |
Operation(s) |
Order of evaluation (precedence) |
|
|
|
|
|
* |
Multiplication |
Evaluated first. If there are several operators of this |
|
/ |
Division |
type, they are evaluated from left to right. |
|
% |
|||
Remainder |
|
||
|
|
||
+ |
Addition |
Evaluated next. If there are several operators of this |
|
- |
Subtraction |
type, they are evaluated from left to right. |
|
|
|||
|
|
|
These rules enable Java to apply operators in the correct order. When we say that operators are applied from left to right, we are referring to their associativity. You will see that some operators associate from right to left. Figure 2.12 summarizes these rules of operator precedence. The table will be expanded as additional Java operators are introduced. A complete precedence chart is included in Appendix A.
Now let us consider several expressions in light of the rules of operator precedence. Each example lists an algebraic expression and its Java equivalent. The following is an example of an arithmetic mean (average) of five terms:
The parentheses are required because division has higher precedence than addition. The entire quantity
(a + b + c |
+ |
d |
+ e) is to be divided by 5. If the parentheses are erroneously omitted, we obtain a + b |
+ c + d + |
e |
/ |
5, which evaluates as |
The following is an example of the equation of a straight line:
No parentheses are required. The multiplication operator is applied first because multiplication has a higher precedence than addition. The assignment occurs last because it has a lower precedence than
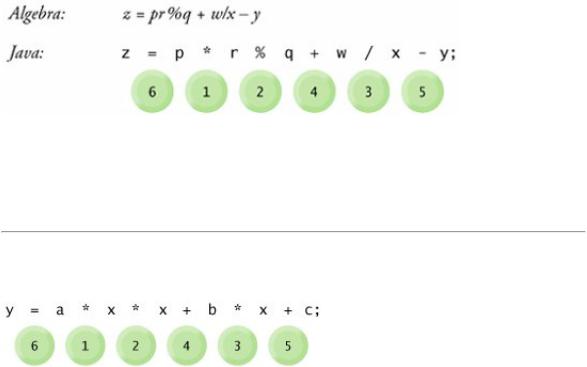
multiplication or addition.
The following example contains remainder (%), multiplication, division, addition and subtraction operations:
The circled numbers under the statement indicate the order in which Java applies the operators. The multiplication, remainder and division operations are evaluated first in left-to-right order (i.e., they associate from left to right), because they have higher precedence than addition and subtraction. The addition and subtraction operations are evaluated next. These operations are also applied from left to right.
[Page 55]
To develop a better understanding of the rules of operator precedence, consider the evaluation of a second-degree polynomial (y = ax2 + bx + c):
The circled numbers indicate the order in which Java applies the operators. The multiplication operations are evaluated first in left-to-right order (i.e., they associate from left to right), because they have higher precedence than addition. The addition operations are evaluated next and are applied from left to
right. There is no arithmetic operator for exponentiation in Java, so x2 is represented as x * x. Section 5.4 shows an alternative for performing exponentiation in Java.
Suppose that a, b, c and x in the preceding second-degree polynomial are initialized (given values) as follows: a = 2, b = 3, c = 7 and x = 5. Figure 2.13 illustrates the order in which the operators are applied.
Figure 2.13. Order in which a second-degree polynomial is evaluated.
[View full size image]
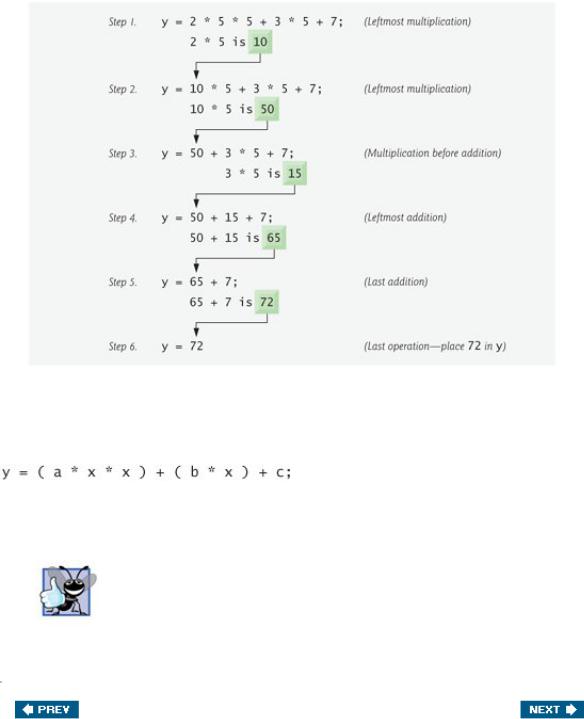
As in algebra, it is acceptable to place unnecessary parentheses in an expression to make the expression clearer. These are called redundant parentheses. For example, the preceding assignment statement might be parenthesized as follows:
Good Programming Practice 2.14
Using parentheses for complex arithmetic expressions, even when the parentheses are not necessary, can make the arithmetic expressions easier to read.

[Page 56]
2.8. Decision Making: Equality and Relational Operators
A condition is an expression that can be either true or false. This section introduces a simple version of Java's if statement that allows a program to make a decision based on the value of a condition. For example, the condition "grade is greater than or equal to 60" determines whether a student passed a test. If the condition in an if statement is true, the body of the if statement executes. If the condition is false, the body does not execute. We will see an example shortly.
Conditions in if statements can be formed by using the equality operators (== and !=) and relational operators (>, <, >= and <=) summarized in Fig. 2.14. Both equality operators have the same level of precedence, which is lower than that of the relational operators. The equality operators associate from left to right. The relational operators all have the same level of precedence and also associate from left to right.
Figure 2.14. Equality and relational operators.
Standard |
|
|
|
algebraic |
|
|
|
equality or |
|
|
|
relational |
Java equality or |
Sample Java |
Meaning of Java |
operator |
relational operator |
condition |
condition |
|
|
|
|
Equality |
|
|
|
operators |
|
|
|
= |
== |
x == y |
x is equal to y |
|
!= |
x != y |
x is not equal to y |
Relational |
|
|
|
operators |
|
|
|
> |
> |
x > y |
x is greater than y |
< |
< |
x < y |
x is less than y |
|
>= |
x >= y |
x is greater than or |
|
|
|
equal to y |
|
<= |
x <= y |
x is less than or |
|
|
|
equal to y |
|
|
|
|
The application of Fig. 2.15 uses six if statements to compare two integers input by the user. If the condition in any of these if statements is true, the assignment statement associated with that if statement executes. The program uses a Scanner to input the two integers from the user and store them in variables number1 and number2. Then the program compares the numbers and displays the results of the comparisons that are true.
Figure 2.15. Equality and relational operators.
(This item is displayed on pages 57 - 58 in the print version)
1 |
// Fig. 2.15: Comparison.java |
|
2 |
// Compare integers using if statements, relational operators |
|
3 |
// and |
equality operators. |
4 |
import |
java.util.Scanner; // program uses class Scanner |
5 |
|
|
6 |
public |
class Comparison |
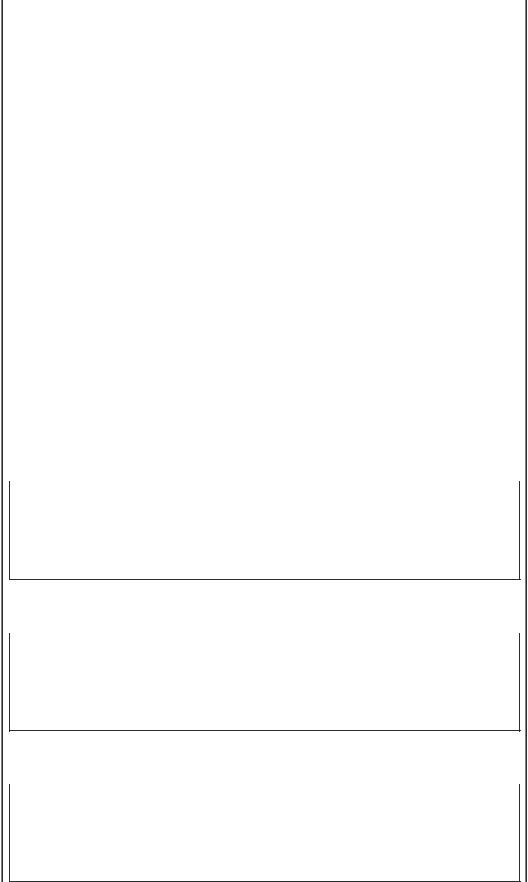
7{
8// main method begins execution of Java application
9public static void main( String args[] )
10{
11// create Scanner to obtain input from command window
12Scanner input = new Scanner( System.in );
13
14int number1; // first number to compare
15int number2; // second number to compare
17System.out.print( "Enter first integer: " ); // prompt
18number1 = input.nextInt(); // read first number from user
20System.out.print( "Enter second integer: " ); // prompt
21number2 = input.nextInt(); // read second number from user
23if ( number1 == number2 )
24 |
System.out.printf( "%d == %d\n", number1, number2 ); |
25 |
|
26if ( number1 != number2 )
27System.out.printf( "%d != %d\n", number1, number2 );
29if ( number1 < number2 )
30 |
System.out.printf( "%d < %d\n", number1, number2 ); |
31 |
|
32if ( number1 > number2 )
33System.out.printf( "%d > %d\n", number1, number2 );
35if ( number1 <= number2 )
36 |
System.out.printf( "%d <= %d\n", number1, number2 ); |
37 |
|
38if ( number1 >= number2 )
39System.out.printf( "%d >= %d\n", number1, number2 );
41} // end method main
43} // end class Comparison
Enter first integer: 777 Enter second integer: 777 777 == 777 777 <= 777 777 >= 777
Enter first integer: 1000 Enter second integer: 2000 1000 != 2000 1000 < 2000 1000 <= 2000
Enter first integer: 2000 Enter second integer: 1000 2000 != 1000 2000 > 1000 2000 >= 1000
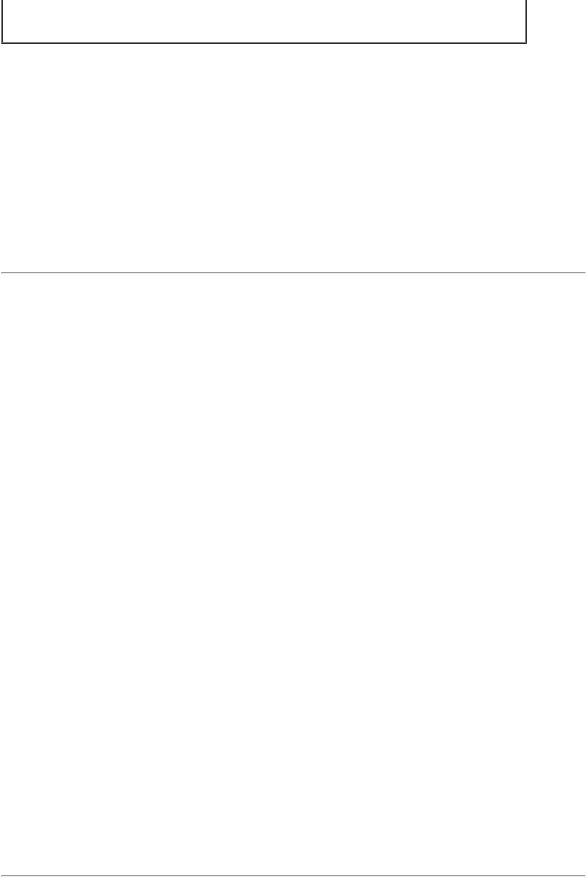
The declaration of class Comparison begins at line 6
public class Comparison
The class's main method (lines 941) begins the execution of the program.
Line 12
Scanner input = new Scanner( System.in );
declares Scanner variable input and assigns it a Scanner that inputs data from the standard input (i.e., the keyboard).
[Page 58]
Lines 1415
int number1; // first number to compare int number2; // second number to compare
declare the int variables used to store the values input from the user.
Lines 1718
System.out.print( "Enter first integer: " ); // prompt number1 = input.nextInt(); // read first number from user
prompt the user to enter the first integer and input the value, respectively. The input value is stored in variable number1.
Lines 2021
System.out.print( "Enter second integer: " ); // prompt number2 = input.nextInt(); // read second number from user
prompt the user to enter the second integer and input the value, respectively. The input value is stored in variable number2.
Lines 2324
if ( number1 == number2 )
System.out.printf( "%d == %d\n", number1, number2 );
declare an if statement that compares the values of the variables number1 and number2 to determine whether they are equal. An if statement always begins with keyword if, followed by a condition in parentheses. An if statement expects one statement in its body. The indentation of the body statement shown here is not required, but it improves the program's readability by emphasizing that the statement in line 24 is part of the if statement that begins on line 23. Line 24 executes only if the numbers stored in variables number1 and number2 are equal (i.e., the condition is true). The if statements at lines 2627, 2930, 3233, 3536 and 3839 compare number1 and number2 with the operators !=, <, >, <= and >=, respectively. If the condition in any of the if statements is true, the corresponding body statement executes.

[Page 59]
Common Programming Error 2.9
Forgetting the left and/or right parentheses for the condition in an if statement is a syntax errorthe parentheses are required.
Common Programming Error 2.10
Confusing the equality operator, ==, with the assignment operator, =, can cause a logic error or a syntax error. The equality operator should be read as "is equal to," and the assignment operator should be read as "gets" or "gets the value of." To avoid confusion, some people read the equality operator as "double equals" or "equals equals."
Common Programming Error 2.11
It is a syntax error if the operators ==, !=, >= and <= contain spaces between their symbols, as in = =, ! =, > = and < =, respectively.
Common Programming Error 2.12
Reversing the operators !=, >= and <=, as in =!, => and =<, is a syntax error.
Good Programming Practice 2.15
Indent an if statement's body to make it stand out and to enhance program readability.