
Beginning Visual Basic 2005 (2006)
.pdf
Chapter 16
Using the DataBindings property of the controls on you form, you execute the Clear method of the ControlBindingsCollection class to remove the bindings from them. Notice that the controls that you bound are all the text boxes on your form that will contain data from the DataView object:
Private Sub BindFields()
‘ Clear any previous bindings to the DataView object...
txtLastName.DataBindings.Clear()
txtFirstName.DataBindings.Clear()
txtBookTitle.DataBindings.Clear()
txtPrice.DataBindings.Clear()
After you clear the previous bindings, you can set the new bindings back to the same data source, our DataView object. You do this by executing the Add method of the ControlBindingsCollection object returned by the DataBindings property. As described earlier, the Add method has three arguments, which are shown in the code that follows:
The first argument is propertyname and specifies the property of the control to be bound. Since you want to bind your data to the Text property of the text boxes, you have specified “Text” for this argument.
The next argument is the datasource argument and specifies the data source to be bound. Remember that this can be any valid object, such as a DataSet, DataView, or DataTable, that contains data. In this case, you are using a DataView object.
The last argument specifies the datamember. This is the data field in the data source that contains the data to be bound to this control. Notice that you have specified the various column names from your SELECT statement that you executed in the previous procedure.
‘Add new bindings to the DataView object...
txtLastName.DataBindings.Add(“Text”, objDataView, “au_lname”) txtFirstName.DataBindings.Add(“Text”, objDataView, “au_fname”) txtBookTitle.DataBindings.Add(“Text”, objDataView, “title”) txtPrice.DataBindings.Add(“Text”, objDataView, “price”)
The last thing you did in this procedure was set a message in the status bar using the Text property of
ToolStripStatusLabel1:
‘ Display a ready status...
ToolStripStatusLabel1.Text = “Ready” End Sub
How It Works: ShowPosition
The CurrencyManager object keeps track of the current record position within the DataView object.
The price column in the titles table in pubs is defined as a Currency data type. Therefore, if a book is priced at 40.00 dollars, the number that you get is 40; the decimal portion is dropped. The ShowPosition procedure seems like a good place to format the data in the txtPrice text box, because this procedure is called whenever you move to a new record:
Private Sub ShowPosition()
‘Always format the number in the txtPrice field to include cents Try
526
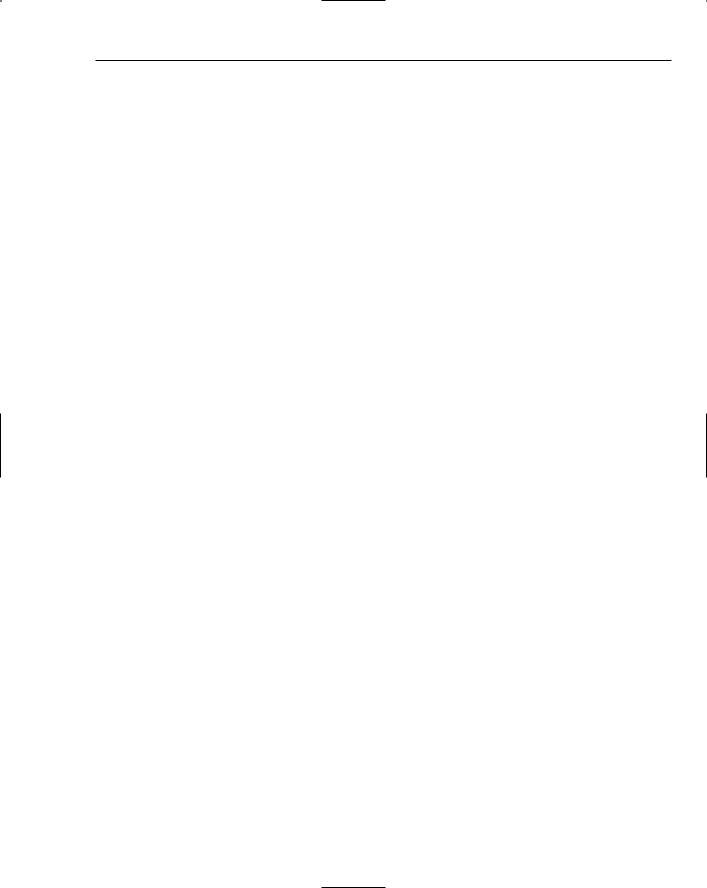
Database Programming with SQL Server and ADO.NET
txtPrice.Text = Format(CType(txtPrice.Text, Decimal), “##0.00”) Catch e As System.Exception
txtPrice.Text = “0”
txtPrice.Text = Format(CType(txtPrice.Text, Decimal), “##0.00”) End Try
‘ Display the current position and the number of records txtRecordPosition.Text = objCurrencyManager.Position + 1 & _
“ of “ & objCurrencyManager.Count()
End Sub
This part of the function is enclosed in a Try . . . Catch block in case the txtPrice is empty. If txt Price is empty, the Format function throws a handled exception, and the exception handler defaults the price to 0. The second line of code in this procedure uses the Format function to format the price in the txtPrice text box. This function accepts the numeric data to be formatted as the first argument and a format string as the second argument. For the format function to work correctly, you need to convert the string value in the txtPrice field to a decimal value using the CType function.
The last line of code displays the current record position and the total number of records that you have. Using the Position property of the CurrencyManager object, you can determine which record you are on. The Position property uses a zero-based index, so the first record is always 0. Therefore, you specified the Position property plus 1 to display the true number.
The CurrencyManager class’s Count property returns the actual number of items in the list, and you are using this property to display the total number of records in the DataView object.
How It Works: Form_Load
Now that you’ve looked at the code for the main procedures, you need to go back and look at your initialization code.
You have a combo box on your form that will be used when sorting or searching for data. This combo box needs be populated with data representing the columns in the DataView object. You specify the Add method of the Items property of the combo box to add items to it. Here you are specifying text that represents the columns in the DataView object in the same order that they appear in the DataView object:
‘Add any initialization after the InitializeComponent() call
‘ Add items to the combo box...
cboField.Items.Add(“Last Name”) cboField.Items.Add(“First Name”) cboField.Items.Add(“Book Title”) cboField.Items.Add(“Price”)
After you have loaded all of the items into your combo box, you want to select the first item. You do this by setting the SelectedIndex property to 0. The SelectedIndex property is zero-based, so the first item in the list is item 0.
‘ Make the first item selected...
cboField.SelectedIndex = 0
527

Chapter 16
Next, you call the FillDataSetAndView procedure to retrieve the data, and then call the BindFields procedure to bind the controls on your form to your DataView object. Finally, you call the ShowPosition procedure to display the current record position and the total number of records contained in your
DataView object:
‘Fill the DataSet and bind the fields...
FillDataSetAndView()
BindFields()
‘Show the current record position...
ShowPosition()
How It Works: Navigation Buttons
The procedure for the btnMoveFirst button causes the first record in the DataView object to be displayed. This is accomplished using the Position property of the CurrencyManager object. Here you set the Position property to 0, indicating that the CurrencyManager should move to the first record:
‘ Set the record position to the first record...
objCurrencyManager.Position = 0
Because your controls are bound to the DataView object, they will always stay in sync with the current record in the DataView object and display the appropriate data.
After you reposition the current record, you need to call the ShowPosition procedure to update the display of the current record on your form:
‘ Show the current record position...
ShowPosition()
Next, you add the code for the btnMovePrevious button. You move to the prior record by subtracting 1 from the Position property. The CurrencyManager object will automatically detect and handle the beginning position of the DataView object. It will not let you move to a position prior to the first record; it will just quietly keep its position at 0:
‘ Move to the previous record...
objCurrencyManager.Position -= 1
Again, after you have repositioned the current record being displayed, you need to call the ShowPosition procedure to display the current position on the form.
In the btnMoveNext procedure, you want to increment the Position property by 1. Again, the Currency Manager will automatically detect the last record in the DataView object and will not let you move past it:
‘ Move to the next record...
objCurrencyManager.Position += 1
You call the ShowPosition procedure to display the current record position.
When the btnMoveLast procedure is called, you want to move to the last record in the DataView object. You do accomplish this by setting the Position property equal to the Count property minus one. Then you call the ShowPosition procedure to display the current record:
528

Database Programming with SQL Server and ADO.NET
‘Set the record position to the last record...
objCurrencyManager.Position = objCurrencyManager.Count - 1
‘Show the current record position...
ShowPosition()
Now that you have built the navigation, you move on to add sorting functionality to this project in the next Try It Out.
Try It Out |
Including Sorting Functionality |
1.Double-click the Perform Sort button on the form in design mode to have the empty procedure added to the form class, or select the button in the Class Name combo box and then select the Click event in the Method Name combo box. Insert the following highlighted code in the btnPerformSort_Click event procedure:
Private Sub btnPerformSort_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnPerformSort.Click
‘Determine the appropriate item selected and set the
‘Sort property of the DataView object...
Select Case cboField.SelectedIndex Case 0 ‘Last Name
objDataView.Sort = “au_lname” Case 1 ‘First Name
objDataView.Sort = “au_fname” Case 2 ‘Book Title
objDataView.Sort = “title”
Case 3 ‘Price
objDataView.Sort = “price”
End Select
‘Call the click event for the MoveFirst button...
btnMoveFirst_Click(Nothing, Nothing)
‘Display a message that the records have been sorted...
ToolStripStatusLabel1.Text = “Records Sorted”
End Sub
2.Test out this newest functionality that you have added to your project by running it; click the Start button to compile and run it. Select a column to sort and then click the Perform Sort button. You should see the data sorted by the column that you have chosen. Figure 16-8 shows the data sorted by book price:
How It Works
The first thing you do in this procedure is determine which field you should sort on. This information is contained in the cboField combo box.
‘Determine the appropriate item selected and set the
‘Sort property of the DataView object...
Select Case cboField.SelectedIndex Case 0 ‘Last Name
objDataView.Sort = “au_lname” Case 1 ‘First Name
529

Chapter 16
objDataView.Sort = “au_fname” Case 2 ‘Book Title
objDataView.Sort = “title” Case 3 ‘Price
objDataView.Sort = “price” End Select
Figure 16-8
Using a Select Case statement to examine the SelectedIndex property of the combo box, you can determine which field the user has chosen. After you have determined the correct entry in the combo box, you can set the Sort property of the DataView object using the column name of the column that you want sorted. After the Sort property has been set, the data will be sorted.
After the data has been sorted, you want to move to the first record, and there are a couple of ways you can do this. You could set the Position property of the CurrencyManager object and then call the ShowPosition procedure, or you can simply call the btnMoveFirst_Click procedure, passing it Nothing for both arguments. This is the procedure that would be executed had you actually clicked the Move First button on the form.
The btnMoveFirst_Click procedure has two arguments: ByVal sender As Object and ByVal e As System.EventArgs. Since these arguments are required (even though they’re not actually used in the procedure), you need to pass something to them, so you pass the Nothing keyword. The Nothing keyword is used to disassociate an object variable from an object. Thus by using the Nothing keyword, you satisfy the requirement of passing an argument to the procedure, but have not passed any actual value:
‘ Call the click event for the MoveFirst button...
btnMoveFirst_Click(Nothing, Nothing)
After the first record has been displayed, you want to display a message in the status bar indicating that the records have been sorted. You did this by setting the Text property of the status bar as you have done before.
530

Database Programming with SQL Server and ADO.NET
Note that another way would have been to have had a procedure called MoveFirst, and to have called that from here and from the btnMoveFirst_Click procedure. Some developers would opt for this instead of having to pass Nothing to a procedure.
In the next Try It Out, you take a look at what’s involved in searching for a record.
Try It Out |
Including Searching Functionality |
1.Double-click the Perform Search button or select the button in the Class Name combo box and then select the Click event in the Method Name combo box, and add the following highlighted code to the btnPerformSearch_Click event procedure:
Private Sub btnPerformSearch_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnPerformSearch.Click
‘Declare local variables...
Dim intPosition As Integer
‘Determine the appropriate item selected and set the
‘Sort property of the DataView object...
Select Case cboField.SelectedIndex Case 0 ‘Last Name
objDataView.Sort = “au_lname” Case 1 ‘First Name
objDataView.Sort = “au_fname” Case 2 ‘Book Title
objDataView.Sort = “title” Case 3 ‘Price
objDataView.Sort = “price” End Select
‘ If the search field is not price then...
If cboField.SelectedIndex < 3 Then
‘ Find the last name, first name, or title...
intPosition = objDataView.Find(txtSearchCriteria.Text)
Else
‘ otherwise find the price...
intPosition = objDataView.Find(CType(txtSearchCriteria.Text, Decimal)) End If
If intPosition = -1 Then
‘ Display a message that the record was not found...
ToolStripStatusLabel1.Text = “Record Not Found”
Else
‘Otherwise display a message that the record was
‘found and reposition the CurrencyManager to that
‘record...
ToolStripStatusLabel1.Text = “Record Found” objCurrencyManager.Position = intPosition
End If
‘ Show the current record position...
ShowPosition() End Sub
531

Chapter 16
2.Test the searching functionality that you added. Run the project and select a field in the Field combo box that you want to search on, and then enter the search criteria in the Search Criteria textbox. Finally, click the Perform Search button.
If a match is found, you see the first matched record displayed, along with a message in the status bar indicating that the record was found, as shown in Figure 16-9. If no record was found, you see a message in the status bar indicating the record was not found.
Figure 16-9
How It Works
This is a little more involved than previous Try It Outs, because there are multiple conditions that you must test for and handle, such as a record that was not found. The first thing that you do in this procedure is to declare a variable that will receive the record position of the record that has been found or not found.
‘ Declare local variables...
Dim intPosition As Integer
Next, you sort the data based on the column used in the search. The Find method will search for data in the sort key. Therefore, by setting the Sort property, the column that is sorted on becomes the sort key in the DataView object. You use a Select Case statement, just as you did in the previous procedure:
‘Determine the appropriate item selected and set the
‘Sort property of the DataView object...
Select Case cboField.SelectedIndex Case 0 ‘Last Name
objDataView.Sort = “au_lname” Case 1 ‘First Name
objDataView.Sort = “au_fname” Case 2 ‘Book Title
objDataView.Sort = “title” Case 3 ‘Price
objDataView.Sort = “price” End Select
532
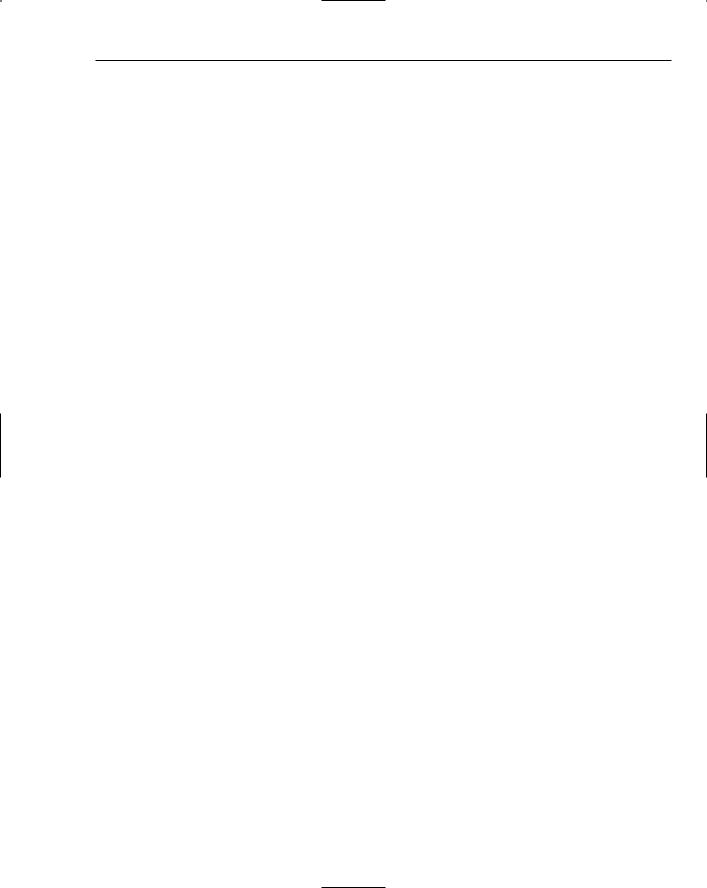
Database Programming with SQL Server and ADO.NET
The columns for the authors’ first and last names, as well as the column for the book titles, all contain text data. However, the column for the book price contains data that is in a currency format. Therefore, you need to determine which column you are searching on, and if that column is the price column, you need to format the data in the txtSearchCriteria text box to a decimal value.
Again, you use the SelectedIndex property of the cboField combo box to determine which item has been selected. If the SelectedIndex property is less than 3, you know that you want to search on a column that contains text data.
You then set the intPosition variable to the results returned by the Find method of the DataView object. The Find method accepts the data to search for as the only argument. Here you pass it the data contained in the Text property of the txtSearchCriteria text box.
If the SelectedIndex equals 3, you are searching for a book with a specific price, and this requires you to convert the value contained in the txtSearchCriteria text box to a decimal value. The CType function accepts an expression and the data type that you want to convert that expression to and returns a value, in this case a decimal value. This value is then used as the search criterion by the Find method.
‘ If the search field is not price then...
If cboField.SelectedIndex < 3 Then
‘ Find the last name, first name or title...
intPosition = objDataView.Find(txtSearchCriteria.Text)
Else
‘ otherwise find the price...
intPosition = objDataView.Find(CType(txtSearchCriteria.Text, Decimal))
End If
After you have executed the Find method of the DataView object, you need to check the value contained in the intPosition variable. If this variable contains a value of –1, no match was found. Any value other than –1 points to the record position of the record that contains the data. So, if the value in this variable is –1, you want to display a message in the status bar that says that no record was found. If the value is greater than –1, you want to display a message that the record was found and position the DataView object to that record using the Position property of the CurrencyManager object:
ToolStripStatusLabel1.Text = “Record Found” objCurrencyManager.Position = intPosition
It is worth noting that the Find method of the DataView object performs a search looking for an exact match of characters. There is no “wildcard” search method here, so you must enter the entire text string that you want to search for. The case, however, does not matter, so the name Ann is the same as ann, and you do not need to be concerned with entering proper case when you enter your search criteria.
The last thing that you want to do in this procedure is to show the current record position, which you do by calling the ShowPosition procedure.
Now all that is left is to add the functionality to add, update, and delete records. Take a look at what is required to add a record first.
533

Chapter 16
Try It Out |
Adding Records |
1.First, you need to add just two lines of code to the btnNew_Click procedure:
Private Sub btnNew_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnNew.Click ‘ Clear the book title and price fields...
txtBookTitle.Text = “” txtPrice.Text = “”
End Sub
2.The next procedure that you want to add code to is the btnAdd_Click procedure. This procedure is responsible for adding a new record. This procedure has the largest amount of code by far of any of the procedures you have coded or will code in this project. The reason for this is the relationship of book titles to authors and the primary key used for book titles:
Private Sub btnAdd_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnAdd.Click ‘ Declare local variables and objects...
Dim intPosition As Integer, intMaxID As Integer Dim strID As String
Dim objCommand As SqlCommand = New SqlCommand()
‘Save the current record position...
intPosition = objCurrencyManager.Position
‘Create a new SqlCommand object...
Dim maxIdCommand As SqlCommand = New SqlCommand _ (“SELECT MAX(title_id) AS MaxID “ & _
“FROM titles WHERE title_id LIKE ‘DM%’”, objConnection)
‘Open the connection, execute the command objConnection.Open()
Dim maxId As Object = maxIdCommand.ExecuteScalar()
‘If the MaxID column is null...
If maxId Is DBNull.Value Then
‘ Set a default value of 1000...
intMaxID = 1000
Else
‘otherwise set the strID variable to the value in MaxID...
strID = CType(maxId, String)
‘Get the integer part of the string...
intMaxID = CType(strID.Remove(0, 2), Integer) ‘ Increment the value...
intMaxID += 1 End If
‘Finally, set the new ID...
strID = “DM” & intMaxID.ToString
‘Set the SqlCommand object properties...
objCommand.Connection = objConnection objCommand.CommandText = “INSERT INTO titles “ & _
“(title_id, title, type, price, pubdate) “ & _
534

Database Programming with SQL Server and ADO.NET
“VALUES(@title_id,@title,@type,@price,@pubdate);” & _
“INSERT INTO titleauthor (au_id, title_id) VALUES(@au_id,@title_id)”
‘Add parameters for the placeholders in the SQL in the
‘CommandText property...
‘Parameter for the title_id column...
objCommand.Parameters.AddWithValue (“@title_id”, strID)
‘Parameter for the title column...
objCommand.Parameters.AddWithValue (“@title”, txtBookTitle.Text)
‘Parameter for the type column objCommand.Parameters.AddWithValue (“@type”, “Demo”)
‘Parameter for the price column...
objCommand.Parameters.AddWithValue (“@price”, txtPrice.Text).DbType _
=DbType.Currency
‘Parameter for the pubdate column objCommand.Parameters.AddWithValue (“@pubdate”, Date.Now)
‘Parameter for the au_id column...
objCommand.Parameters.AddWithValue _
(“@au_id”, BindingContext(objDataView).Current(“au_id”))
‘Execute the SqlCommand object to insert the new data...
Try
objCommand.ExecuteNonQuery() Catch SqlExceptionErr As SqlException
MessageBox.Show(SqlExceptionErr.Message) End Try
‘Close the connection...
objConnection.Close()
‘Fill the dataset and bind the fields...
FillDataSetAndView()
BindFields()
‘Set the record position to the one that you saved...
objCurrencyManager.Position = intPosition
‘Show the current record position...
ShowPosition()
‘ Display a message that the record was added...
ToolStripStatusLabel1.Text = “Record Added” End Sub
3.Run your project. Find an author that you want to add a new title for and then click the New button. The Book Title and Price fields will be cleared, and you are ready to enter new data to be added as shown in Figure 16-10. Take note of the number of records in the DataView (26 in Figure 16-10).
535