
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference
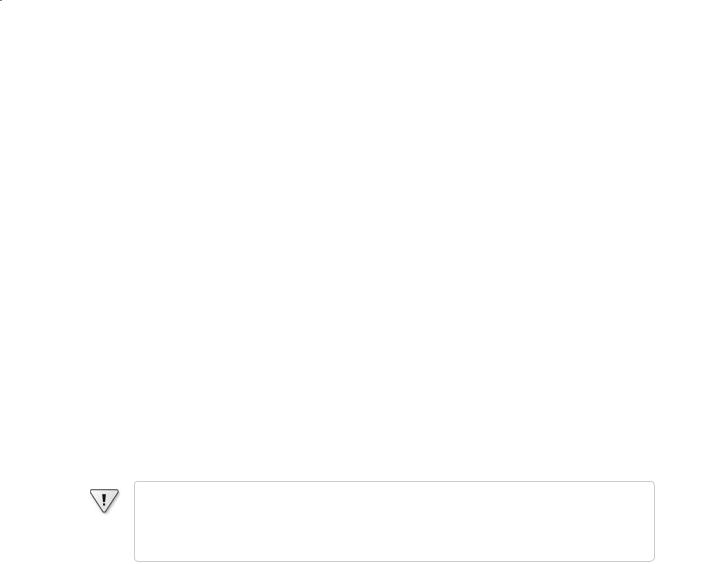
Chapter 17 Interrupting Program Flow and Handling Events |
325 |
Raising an Event
An event can be raised, just like a delegate, by calling it like a method. When you raise
an event, all the attached delegates are called in sequence. For example, here’s the
TemperatureMonitor class with a private Notify method that raises the MachineOverheating event:
class TemperatureMonitor
{
public delegate void StopMachineryDelegate;
public event StopMachineryDelegate MachineOverheating;
...
private void Notify()
{
if (this.MachineOverheating != null)
{
this.MachineOverheating();
}
}
...
}
This is a common idiom. The null check is necessary because an event field is implicitly null
and only becomes non-null when a method subscribes to it by using the += operator. If you try to raise a null event, you will get a NullReferenceException. If the delegate defining the
event expects any parameters, the appropriate arguments must be provided when you raise the event. You will see some examples of this later.
Important Events have a very useful built-in security feature. A public event (such as MachineOverheating) can be raised only by methods in the class that defines it (the TemperatureMonitor class). Any attempt to raise the method outside the class results in a
compiler error.
Understanding WPF User Interface Events
As mentioned earlier, the .NET Framework classes and controls used for building graphi-
cal user interfaces (GUIs) employ events extensively. You’ll see and use GUI events on many occasions in the second half of this book. For example, the WPF Button class derives from the ButtonBase class, inheriting a public event called Click of type RoutedEventHandler. The RoutedEventHandler delegate expects two parameters: a reference to the object that caused the event to be raised and a RoutedEventArgs object that contains additional information
about the event:
public delegate void RoutedEventHandler(Object sender, RoutedEventArgs e);

326 Part III Creating Components
The Button class looks like this:
public class ButtonBase: ...
{
public event RoutedEventHandler Click;
...
}
public class Button: ButtonBase
{
...
}
The Button class automatically raises the Click event when you click the button on-screen. (How this actually happens is beyond the scope of this book.) This arrangement makes it easy to create a delegate for a chosen method and attach that delegate to the required event. The
following example shows the code for a WPF form that contains a button called okay and the code to connect the Click event of the okay button to the okayClick method:
public partial class Example : System.Windows.Window, System.Windows.Markup. IComponentConnector
{
internal System.Windows.Controls.Button okay;
...
void System.Windows.Markup.IComponentConnector.Connect(...)
{
...
this.okay.Click += new System.Windows.RoutedEventHandler(this.okayClick);
...
}
...
}
This code is usually hidden from you. When you use the Design View window in Visual Studio 2008 and set the Click property of the okay button to okayClick in the Extensible Application
Markup Language (XAML) description of the form, Visual Studio 2008 generates this code
for you. All you have to do is write your application logic in the event handling method, okayClick, in the part of the code that you do have access to, in the Example.xaml.cs file in
this case:
public partial class Example : System.Windows.Window
{
...
private void okayClick(object sender, RoutedEventArgs args)
{
// your code to handle the Click event
}
}
The events that the various GUI controls generate always follow the same pattern. The events are of a delegate type whose signature has a void return type and two arguments. The first
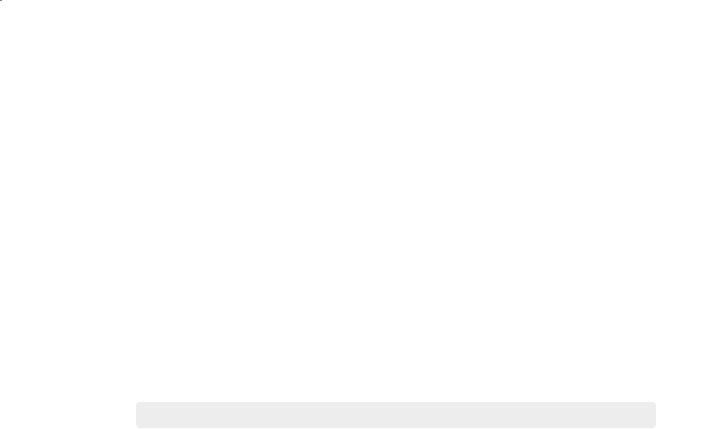
Chapter 17 Interrupting Program Flow and Handling Events |
327 |
argument is always the sender (the source) of the event, and the second argument is always an EventArgs argument (or a class derived from EventArgs).
With the sender argument, you can reuse a single method for multiple events. The del-
egated method can examine the sender argument and respond accordingly. For example, you can use the same method to subscribe to the Click event for two buttons (you add the
same method to two different events). When the event is raised, the code in the method can examine the sender argument to ascertain which button was clicked.
You learn more about how to handle events for WPF controls in Chapter 22, “Introducing Windows Presentation Foundation.”
Using Events
In the following exercise, you will use events to simplify the program you completed in the first exercise. You will add an event field to the Ticker class and delete its Add and Remove methods. You will then modify the Clock.Start and Clock.Stop methods to subscribe to the event. You will also examine the Timer object, used by the Ticker class to obtain a pulse once
each second.
Rework the digital clock application
1.Return to the Visual Studio 2008 window displaying the Delegates project.
2.Display the Ticker.cs file in the Code and Text Editor window.
This file contains the declaration of the Tick delegate type in the Ticker class:
public delegate void Tick(int hh, int mm, int ss);
3.Add a public event called tick of type Tick to the Ticker class, as shown in bold type in the following code:
class Ticker
{
public delegate void Tick(int hh, int mm, int ss); public event Tick tick;
...
}
4.Comment out the following delegate variable tickers near the bottom of the Ticker class definition because it is now obsolete:
// private Tick tickers;
5.Comment out the Add and Remove methods from the Ticker class.
The add and remove functionality is automatically provided by the += and –= operators of the event object.

328Part III Creating Components
6.Locate the Ticker.Notify method. This method previously invoked an instance of the Tick delegate called tickers. Modify it so that it calls the tick event instead. Don’t forget to check whether tick is null before calling the event.
The Notify method should look like this:
private void Notify(int hours, int minutes, int seconds)
{
if (this.tick != null) this.tick(hours, minutes, seconds);
}
Notice that the Tick delegate specifies parameters, so the statement that raises the tick event must specify arguments for each of these parameters.
7. Examine the definition of the ticking variable at the end of the class:
private DispatcherTimer ticking = new DispatcherTimer();
The DispatcherTimer class can be programmed to raise an event repeatedly at a specified interval.
8. Examine the constructor for the Ticker class:
public Ticker()
{
this.ticking.Tick += new EventHandler(this.OnTimedEvent); this.ticking.Interval = new TimeSpan(0, 0, 1); // 1 second this.ticking.Start();
}
The DispatcherTimer class exposes the Tick event, which can be raised at regular intervals according to the value of the Interval property. Setting Interval to the
TimeSpan shown causes the Tick event to be raised once a second. The timer starts when you invoke the Start method. Methods that subscribe to the Tick event must match the signature of the EventHandler delegate. The EventHandler delegate has the same signature as the RoutedEventHandler delegate described earlier. The Ticker constructor creates an instance of this delegate referring to the OnTimedEvent method and
subscribes to the Tick event.
The OnTimedEvent method in the Ticker class obtains the current time by examining the static DateTime.Now property. The DateTime structure is part of the .NET Framework class library. The Now property returns a DateTime structure. This structure has several fields, including those used by the OnTimedEvent method shown in the following code and called Hour, Minute, and Second. The OnTimedEvent method uses this information in turn to raise the tick event through the Notify method:
private void OnTimedEvent(object source, EventArgs args)
{
DateTime now = DateTime.Now; int hh = now.Hour;
int mm = now.Minutes;