
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference


PUBLISHED BY
Microsoft Press
A Division of Microsoft Corporation
One Microsoft Way
Redmond, Washington 98052-6399
Copyright © 2008 by John Sharp
All rights reserved. No part of the contents of this book may be reproduced or transmitted in any form or by any means without the written permission of the publisher.
Library of Congress Control Number: 2007939305
Printed and bound in the United States of America.
1 2 3 4 5 6 7 8 9 QWT 2 1 0 9 8 7
Distributed in Canada by H.B. Fenn and Company Ltd.
A CIP catalogue record for this book is available from the British Library.
Microsoft Press books are available through booksellers and distributors worldwide. For further information about international editions, contact your local Microsoft Corporation office or contact Microsoft Press International directly at fax (425) 936-7329. Visit our Web site at www.microsoft.com/mspress. Send comments to mspinput@microsoft.com.
Microsoft, Microsoft Press, MSDN, SQL Server, Excel, Intellisense, Internet Explorer, Jscript, Silverlight, Visual Basic, Visual C#, Visual Studio, Win32, Windows, Windows Server, and Windows Vista are either registered trademarks or trademarks of Microsoft Corporation in the United States and/or other countries. Other product and company names mentioned herein may be the trademarks of their respective owners.
The example companies, organizations, products, domain names, e-mail addresses, logos, people, places, and events depicted herein are fictitious. No association with any real company, organization, product, domain name, e-mail address, logo, person, place, or event is intended or should be inferred.
7KLV ERRN H[SUHVVHV WKH DXWKRU¶V YLHZV DQG RSLQLRQV 7KH LQIRUPDWLRQ FRQWDLQHG LQ WKLV ERRN LV SURYLGHG without any express, statutory, or implied warranties. Neither the authors, Microsoft Corporation, nor its resellers, or distributors will be held liable for any damages caused or alleged to be caused either directly or indirectly by this book.
Acquisitions Editor: Ben Ryan
Developmental and Project Editor: Lynn Finnel Editorial Production: Waypoint Press
Technical Reviewer: Kurt Meyer; Technical Review services provided by Content Master, a member of CM Group, Ltd.
Body Part No. X14-22686

Contents at a Glance
Part I Introducing Microsoft Visual C# and
Microsoft Visual Studio 2008
1 Welcome to C# . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3 2 Working with Variables, Operators, and Expressions . . . . . . . . . 29 3 Writing Methods and Applying Scope . . . . . . . . . . . . . . . . . . . . . 49 4 Using Decision Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67 5 Using Compound Assignment and Iteration Statements . . . . . . 85 6 Managing Errors and Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . 103
Part II Understanding the C# Language |
|
|
7 |
Creating and Managing Classes and Objects . . . . . . . . . . . . . . . |
123 |
8 |
Understanding Values and References . . . . . . . . . . . . . . . . . . . . |
145 |
9 |
Creating Value Types with Enumerations and Structures . . . . . |
167 |
10 |
Using Arrays and Collections. . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
185 |
11 |
Understanding Parameter Arrays . . . . . . . . . . . . . . . . . . . . . . . . . |
207 |
12 |
Working with Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
217 |
13 |
Creating Interfaces and Defining Abstract Classes . . . . . . . . . . |
239 |
14 |
Using Garbage Collection and Resource Management. . . . . . . |
257 |
Part III |
Creating Components |
|
15 |
Implementing Properties to Access Fields . . . . . . . . . . . . . . . . . |
275 |
16 |
Using Indexers. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
295 |
17 |
Interrupting Program Flow and Handling Events . . . . . . . . . . . |
311 |
18 |
Introducing Generics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
333 |
19 |
Enumerating Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
355 |
20 |
Querying In-Memory Data by Using Query Expressions . . . . . |
371 |
21 |
Operator Overloading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
395 |
iii
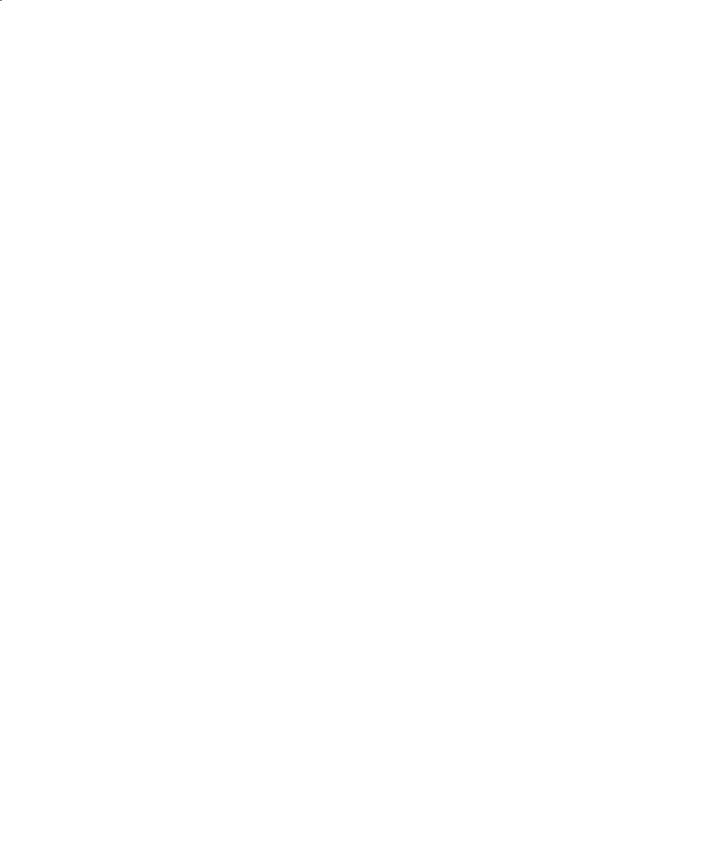
iv Contents at a Glance
Part IV Working with Windows Applications
22 |
Introducing Windows Presentation Foundation . . . . . . . . . . . . |
415 |
23 |
Working with Menus and Dialog Boxes . . . . . . . . . . . . . . . . . . . |
451 |
24 |
Performing Validation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
473 |
Part V |
Managing Data |
|
25 |
Querying Information in a Database . . . . . . . . . . . . . . . . . . . . . . |
499 |
26 |
Displaying and Editing Data by Using Data Binding . . . . . . . . . |
529 |
Part VI |
Building Web Applications |
|
27 |
Introducing ASP.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
559 |
28 |
Understanding Web Forms Validation Controls. . . . . . . . . . . . . |
587 |
29 |
Protecting a Web Site and Accessing Data with |
|
|
Web Forms. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
597 |
30 |
Creating and Using a Web Service . . . . . . . . . . . . . . . . . . . . . . . . |
623 |
|
Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
645 |

Table of Contents
Acknowledgments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xvii Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .xix
Part I Introducing Microsoft Visual C# and
Microsoft Visual Studio 2008
1 Welcome to C# . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
Beginning Programming with the Visual Studio 2008 Environment. . . . . . . . . . 3 Writing Your First Program. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8 Using Namespaces. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14 Creating a Graphical Application. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17 Chapter 1 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
2 Working with Variables, Operators, and Expressions . . . . . . . . . 29
Understanding Statements. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29 Using Identifiers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30 Identifying Keywords. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30 Using Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31 Naming Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32 Declaring Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32 Working with Primitive Data Types. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33 Displaying Primitive Data Type Values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34 Using Arithmetic Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38 Operators and Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 39 Examining Arithmetic Operators. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40 Controlling Precedence . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43 Using Associativity to Evaluate Expressions . . . . . . . . . . . . . . . . . . . . . . . . 44 Associativity and the Assignment Operator . . . . . . . . . . . . . . . . . . . . . . . . 45
What do you think of this book? We want to hear from you!
Microsoft is interested in hearing your feedback so we can continually improve our books and learning resources for you. To participate in a brief online survey, please visit:
www.microsoft.com/learning/booksurvey
v
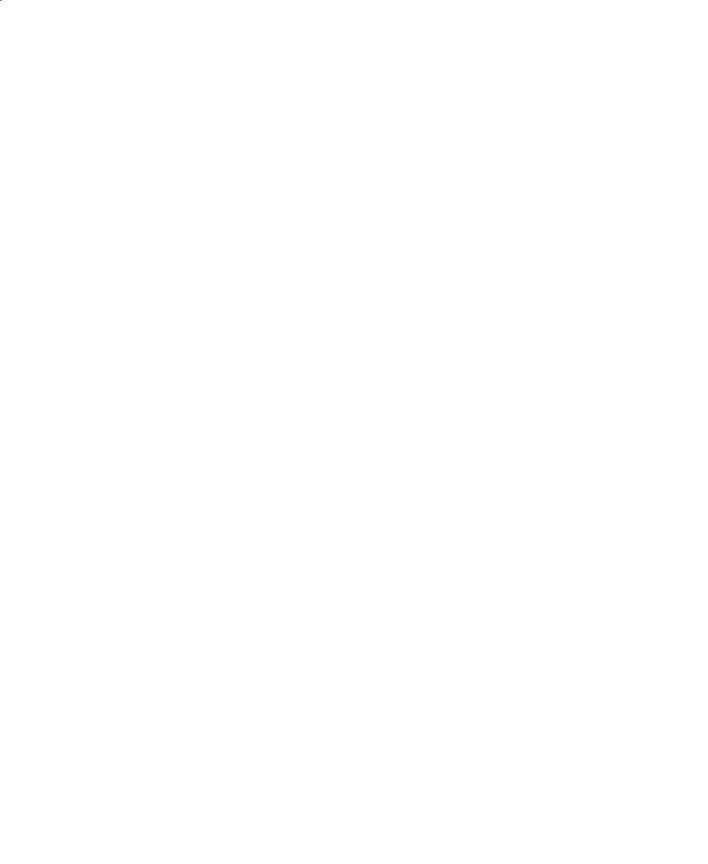
vi |
Table of Contents |
|
|
Incrementing and Decrementing Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
45 |
|
Prefix and Postfix . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
46 |
|
Declaring Implicitly Typed Local Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
47 |
|
Chapter 2 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
48 |
3 Writing Methods and Applying Scope . . . . . . . . . . . . . . . . . . . . . 49
Declaring Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49 Specifying the Method Declaration Syntax. . . . . . . . . . . . . . . . . . . . . . . . . 50 Writing return Statements. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51 Calling Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53 Specifying the Method Call Syntax. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
Applying Scope . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56 Defining Local Scope. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56 Defining Class Scope . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56 Overloading Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57 Writing Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58 Chapter 3 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
4 Using Decision Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67
Declaring Boolean Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67 Using Boolean Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 68 Understanding Equality and Relational Operators . . . . . . . . . . . . . . . . . . 68 Understanding Conditional Logical Operators. . . . . . . . . . . . . . . . . . . . . . 69 Summarizing Operator Precedence and Associativity . . . . . . . . . . . . . . . 70 Using if Statements to Make Decisions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 71 Understanding if Statement Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 71 Using Blocks to Group Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73 Cascading if Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73 Using switch Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 78 Understanding switch Statement Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . 79 Following the switch Statement Rules. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 80 Chapter 4 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84
5 Using Compound Assignment and Iteration Statements . . . . . . 85
Using Compound Assignment Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 85 Writing while Statements. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87 Writing for Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 91 Understanding for Statement Scope . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 92
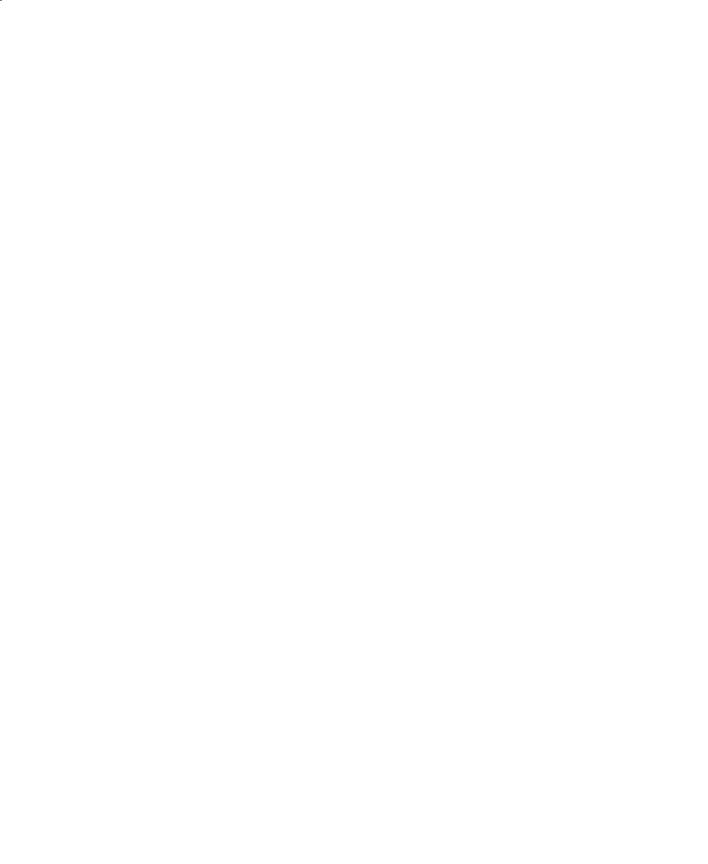
Table of Contents |
vii |
Writing do Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93 Chapter 5 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
6 Managing Errors and Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . 103
Coping with Errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 103 Trying Code and Catching Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 104 Handling an Exception . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105 Using Multiple catch Handlers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106 Catching Multiple Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106 Using Checked and Unchecked Integer Arithmetic . . . . . . . . . . . . . . . . . . . . . . 111 Writing Checked Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 112 Writing Checked Expressions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113 Throwing Exceptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 114 Using a finally Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118 Chapter 6 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 120
Part II Understanding the C# Language
7 Creating and Managing Classes and Objects . . . . . . . . . . . . . . . 123
Understanding Classification . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123 The Purpose of Encapsulation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124 Defining and Using a Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124 Controlling Accessibility . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126 Working with Constructors. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 127
Overloading Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 128 Understanding static Methods and Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 136 Creating a Shared Field. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137 Creating a static Field by Using the const Keyword . . . . . . . . . . . . . . . . 137 Chapter 7 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 142
8 Understanding Values and References . . . . . . . . . . . . . . . . . . . . 145
Copying Value Type Variables and Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145 Understanding Null Values and Nullable Types . . . . . . . . . . . . . . . . . . . . . . . . . 150 Using Nullable Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151 Understanding the Properties of Nullable Types . . . . . . . . . . . . . . . . . . . 152 Using ref and out Parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 152 Creating ref Parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153 Creating out Parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 154

viii Table of Contents
How Computer Memory Is Organized . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156 Using the Stack and the Heap . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 157 The System.Object Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
Boxing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159 Unboxing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159 Casting Data Safely . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161 The is Operator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161 The as Operator. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 162
Chapter 8 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 164
9 Creating Value Types with Enumerations and Structures . . . . . 167
Working with Enumerations. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167 Declaring an Enumeration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167 Using an Enumeration. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168 Choosing Enumeration Literal Values. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169 Choosing an Enumeration’s Underlying Type . . . . . . . . . . . . . . . . . . . . . . 170
Working with Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 172 Declaring a Structure. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174 Understanding Structure and Class Differences. . . . . . . . . . . . . . . . . . . . 175 Declaring Structure Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 176 Understanding Structure Initialization . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177 Copying Structure Variables. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179
Chapter 9 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183
10 Using Arrays and Collections. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185
What Is an Array? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185
Declaring Array Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185
Creating an Array Instance. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186
Initializing Array Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 187
Creating an Implicitly Typed Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 188
Accessing an Individual Array Element . . . . . . . . . . . . . . . . . . . . . . . . . . . 189
Iterating Through an Array. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 190
Copying Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 191
What Are Collection Classes? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 192
The ArrayList Collection Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 194
The Queue Collection Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 196
The Stack Collection Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197
The Hashtable Collection Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198
The SortedList Collection Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 199

Table of Contents |
ix |
Using Collection Initializers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200 Comparing Arrays and Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200 Using Collection Classes to Play Cards. . . . . . . . . . . . . . . . . . . . . . . . . . . . 201 Chapter 10 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 206
11 Understanding Parameter Arrays . . . . . . . . . . . . . . . . . . . . . . . . . 207
Using Array Arguments. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 208
Declaring a params Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 209
Using params object[ ] . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .211
Using a params Array. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 212
Chapter 11 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 215
12 Working with Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217
What Is Inheritance? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217 Using Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 218 Base Classes and Derived Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 218 Calling Base Class Constructors. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 220 Assigning Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 221 Declaring new Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 222 Declaring Virtual Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 224 Declaring override Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 225 Understanding protected Access . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 227 Understanding Extension Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 233 Chapter 12 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 237
13 Creating Interfaces and Defining Abstract Classes . . . . . . . . . . 239
Understanding Interfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 239 Interface Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240 Interface Restrictions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 241 Implementing an Interface. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 241 Referencing a Class Through Its Interface . . . . . . . . . . . . . . . . . . . . . . . . . 243 Working with Multiple Interfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 244 Abstract Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 244 Abstract Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 245 Sealed Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246 Sealed Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 246 Implementing an Extensible Framework . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247 Summarizing Keyword Combinations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 255 Chapter 13 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 256
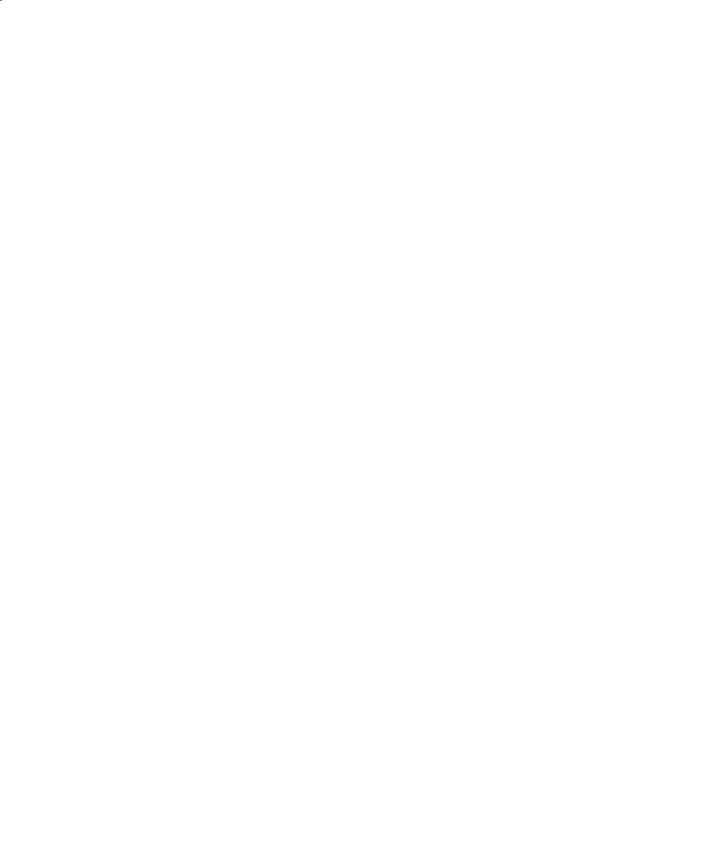
x |
Table of Contents |
|
|
14 Using Garbage Collection and Resource Management. . . . . . . |
257 |
|
The Life and Times of an Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 257 |
|
Writing Destructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 258 |
|
Why Use the Garbage Collector? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 260 |
|
How Does the Garbage Collector Work?. . . . . . . . . . . . . . . . . . . . . . . . . |
. 261 |
|
Recommendations. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 262 |
|
Resource Management . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 262 |
|
Disposal Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 263 |
|
Exception-Safe Disposal . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 263 |
|
The using Statement. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 264 |
|
Calling the Dispose Method from a Destructor. . . . . . . . . . . . . . . . . . . |
. 266 |
|
Making Code Exception-Safe. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 267 |
|
Chapter 14 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 270 |
Part III Creating Components
15 Implementing Properties to Access Fields . . . . . . . . . . . . . . . . . 275
Implementing Encapsulation by Using Methods . . . . . . . . . . . . . . . . . . . . . . . . 276 What Are Properties?. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 278 Using Properties. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 279 Read-Only Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 280 Write-Only Properties. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 280 Property Accessibility . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 281 Understanding the Property Restrictions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 282 Declaring Interface Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 284 Using Properties in a Windows Application . . . . . . . . . . . . . . . . . . . . . . . 285 Generating Automatic Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 287 Initializing Objects by Using Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 288 Chapter 15 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 292
16 Using Indexers. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 295
What Is an Indexer? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 295
An Example That Doesn’t Use Indexers . . . . . . . . . . . . . . . . . . . . . . . . . . . 295
The Same Example Using Indexers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 297
Understanding Indexer Accessors. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 299
Comparing Indexers and Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 300
Indexers in Interfaces. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 302
Using Indexers in a Windows Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 303
Chapter 16 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 308
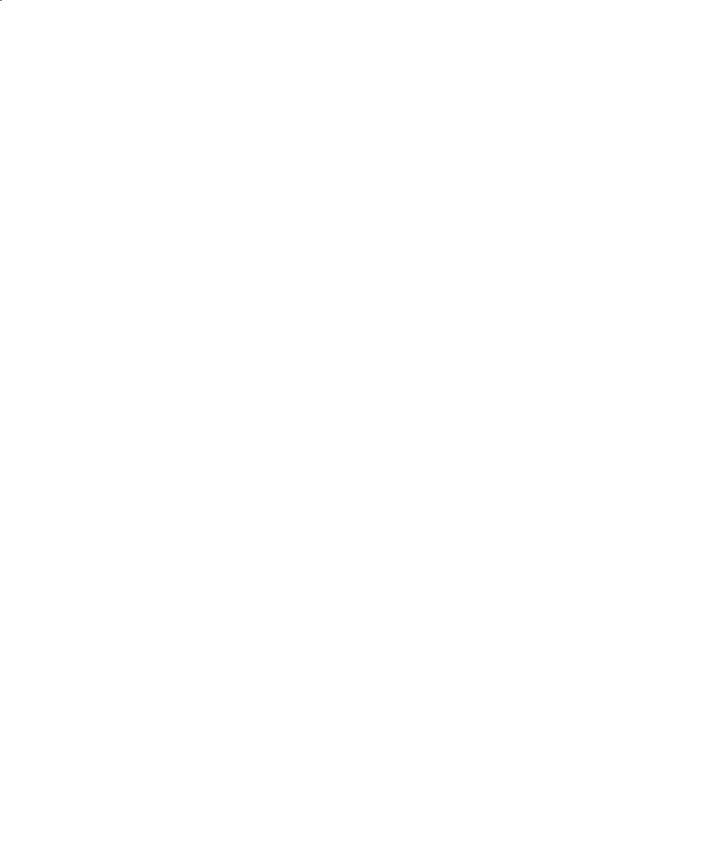
Table of Contents |
xi |
17 Interrupting Program Flow and Handling Events . . . . . . . . . . . 311
Declaring and Using Delegates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 311 The Automated Factory Scenario . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 312 Implementing the Factory Without Using Delegates . . . . . . . . . . . . . . . 312 Implementing the Factory by Using a Delegate. . . . . . . . . . . . . . . . . . . . 313 Using Delegates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 316
Lambda Expressions and Delegates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 319 Creating a Method Adapter. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 319 Using a Lambda Expression as an Adapter . . . . . . . . . . . . . . . . . . . . . . . . 320 The Form of Lambda Expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 321
Enabling Notifications with Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 323 Declaring an Event. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 323 Subscribing to an Event . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 324 Unsubscribing from an Event . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 324 Raising an Event . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 325 Understanding WPF User Interface Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 325 Using Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 327 Chapter 17 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 329
18 Introducing Generics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333
The Problem with objects. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333 The Generics Solution . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 335 Generics vs. Generalized Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 337 Generics and Constraints . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 338 Creating a Generic Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 338 The Theory of Binary Trees. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 338 Building a Binary Tree Class by Using Generics . . . . . . . . . . . . . . . . . . . . 341 Creating a Generic Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 350 Defining a Generic Method to Build a Binary Tree . . . . . . . . . . . . . . . . . 351 Chapter 18 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 354
19 Enumerating Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 355
Enumerating the Elements in a Collection. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 355 Manually Implementing an Enumerator . . . . . . . . . . . . . . . . . . . . . . . . . . 357 Implementing the IEnumerable Interface . . . . . . . . . . . . . . . . . . . . . . . . 361 Implementing an Enumerator by Using an Iterator . . . . . . . . . . . . . . . . . . . . . . 363 A Simple Iterator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 364
Defining an Enumerator for the Tree<TItem> Class by
Using an Iterator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 366 Chapter 19 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 368

xii |
Table of Contents |
|
|
20 Querying In-Memory Data by Using Query Expressions . . . . . |
371 |
|
What Is Language Integrated Query (LINQ)? . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 371 |
|
Using LINQ in a C# Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 372 |
|
Selecting Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 374 |
|
Filtering Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 377 |
|
Ordering, Grouping, and Aggregating Data. . . . . . . . . . . . . . . . . . . . . . |
. 377 |
|
Joining Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 380 |
|
Using Query Operators. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 381 |
|
Querying Data in Tree<TItem> Objects . . . . . . . . . . . . . . . . . . . . . . . . . |
. 383 |
|
LINQ and Deferred Evaluation. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 389 |
|
Chapter 20 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . |
. 392 |
21 Operator Overloading . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 395
Understanding Operators. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 395
Operator Constraints. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 396
Overloaded Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 396
Creating Symmetric Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 398
Understanding Compound Assignment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 400
Declaring Increment and Decrement Operators . . . . . . . . . . . . . . . . . . . . . . . . 401
Defining Operator Pairs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 403
Implementing an Operator. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 404
Understanding Conversion Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 406
Providing Built-In Conversions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 406
Implementing User-Defined Conversion Operators . . . . . . . . . . . . . . . . 407
Creating Symmetric Operators, Revisited . . . . . . . . . . . . . . . . . . . . . . . . . 408
Adding an Implicit Conversion Operator. . . . . . . . . . . . . . . . . . . . . . . . . . 409
Chapter 21 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 411
Part IV Working with Windows Applications
22 Introducing Windows Presentation Foundation . . . . . . . . . . . . 415
Creating a WPF Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 415 Creating a Windows Presentation Foundation Application . . . . . . . . . . 416 Adding Controls to the Form . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 430 Using WPF Controls. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 430 Changing Properties Dynamically. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 439 Handling Events in a WPF Form . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 443 Processing Events in Windows Forms. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 443 Chapter 22 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 449
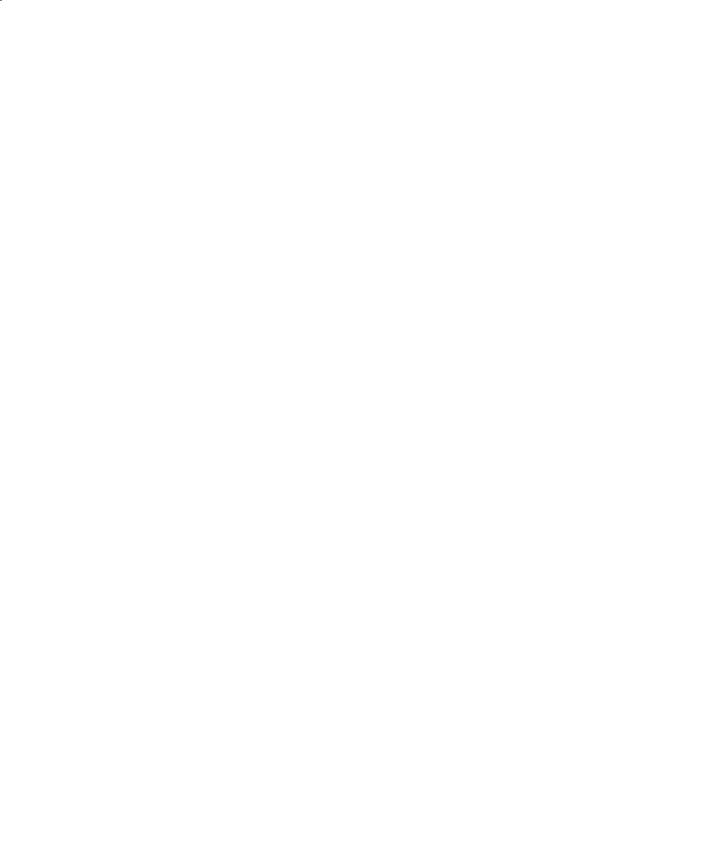
Table of Contents |
xiii |
23 Working with Menus and Dialog Boxes . . . . . . . . . . . . . . . . . . . 451
Menu Guidelines and Style. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 451 Menus and Menu Events. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 452 Creating a Menu . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 452 Handling Menu Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 458 Shortcut Menus . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 464 Creating Shortcut Menus . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 464 Windows Common Dialog Boxes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 468 Using the SaveFileDialog Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 468 Chapter 23 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 471
24 Performing Validation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 473
Validating Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 473
Strategies for Validating User Input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 473
An Example—Customer Information Maintenance. . . . . . . . . . . . . . . . . . . . . . 474
Performing Validation by Using Data Binding . . . . . . . . . . . . . . . . . . . . . 475
Changing the Point at Which Validation Occurs . . . . . . . . . . . . . . . . . . . 491
Chapter 24 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 495
Part V Managing Data
25 Querying Information in a Database . . . . . . . . . . . . . . . . . . . . . . 499
Querying a Database by Using ADO.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 499 The Northwind Database . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 500 Creating the Database . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 500 Using ADO.NET to Query Order Information . . . . . . . . . . . . . . . . . . . . . . 503 Querying a Database by Using DLINQ. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 512 Defining an Entity Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 512 Creating and Running a DLINQ Query. . . . . . . . . . . . . . . . . . . . . . . . . . . . 514 Deferred and Immediate Fetching . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 516 Joining Tables and Creating Relationships . . . . . . . . . . . . . . . . . . . . . . . . 517 Deferred and Immediate Fetching Revisited. . . . . . . . . . . . . . . . . . . . . . . 521 Defining a Custom DataContext Class . . . . . . . . . . . . . . . . . . . . . . . . . . . 522 Using DLINQ to Query Order Information . . . . . . . . . . . . . . . . . . . . . . . . 523 Chapter 25 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 527
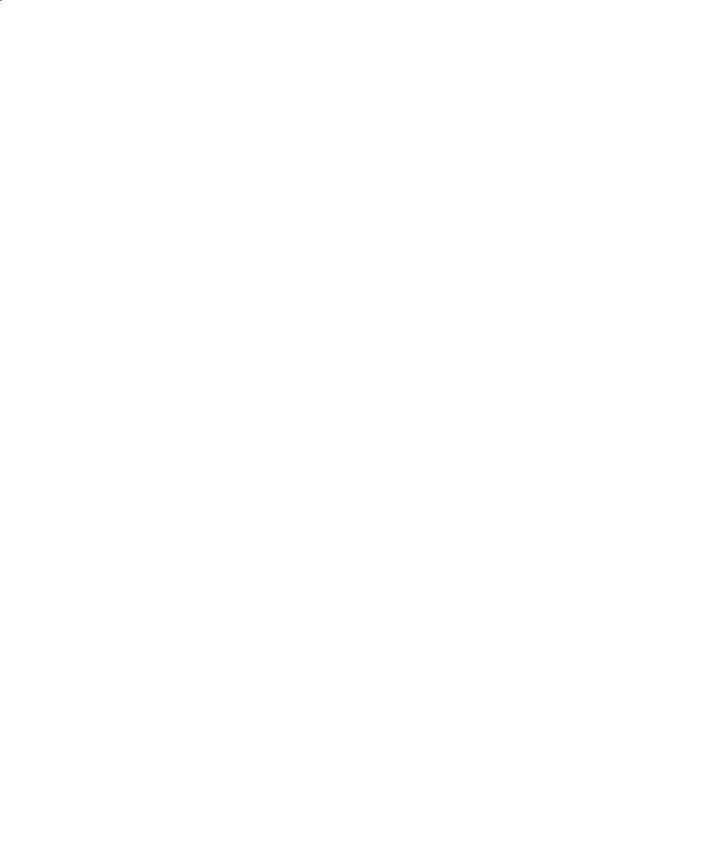
xiv Table of Contents
26 Displaying and Editing Data by Using Data Binding . . . . . . . . . 529
Using Data Binding with DLINQ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 529 Using DLINQ to Modify Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 544 Updating Existing Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 544 Handling Conflicting Updates . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 545 Adding and Deleting Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 548 Chapter 26 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 556
Part VI Building Web Applications
27 Introducing ASP.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 559
Understanding the Internet as an Infrastructure . . . . . . . . . . . . . . . . . . . . . . . . 560 Understanding Web Server Requests and Responses . . . . . . . . . . . . . . . 560 Managing State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 561 Understanding ASP.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 561 Creating Web Applications with ASP.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 563 Building an ASP.NET Application. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 564 Understanding Server Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 575 Creating and Using a Theme . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 582 Chapter 27 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 586
28 Understanding Web Forms Validation Controls. . . . . . . . . . . . . 587
Comparing Server and Client Validations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 587
Validating Data at the Web Server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 588
Validating Data in the Web Browser. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 588
Implementing Client Validation. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 589
Chapter 28 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 596
29 Protecting a Web Site and Accessing Data with
Web Forms. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 597
Managing Security. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 597 Understanding Forms-Based Security . . . . . . . . . . . . . . . . . . . . . . . . . . . . 598 Implementing Forms-Based Security . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 598 Querying and Displaying Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 605 Understanding the Web Forms GridView Control . . . . . . . . . . . . . . . . . 605 Displaying Customer and Order History Information . . . . . . . . . . . . . . . 606 Paging Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 611

Table of Contents |
xv |
Editing Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 612
Updating Rows Through a GridView Control . . . . . . . . . . . . . . . . . . . . . 612
Navigating Between Forms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 614
Chapter 29 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 621
30 Creating and Using a Web Service . . . . . . . . . . . . . . . . . . . . . . . . 623
What Is a Web Service? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 623 The Role of SOAP. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 624 What Is the Web Services Description Language? . . . . . . . . . . . . . . . . . . 625 Nonfunctional Requirements of Web Services . . . . . . . . . . . . . . . . . . . . . 625 The Role of Windows Communication Foundation . . . . . . . . . . . . . . . . . 627
Building a Web Service . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 627 Creating the ProductsService Web Service . . . . . . . . . . . . . . . . . . . . . . . . 628 Web Services, Clients, and Proxies . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 637 Talking SOAP: The Difficult Way . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 637 Talking SOAP: The Easy Way . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 637 Consuming the ProductsService Web Service . . . . . . . . . . . . . . . . . . . . . 638 Chapter 30 Quick Reference. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .644
Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 645
What do you think of this book? We want to hear from you!
Microsoft is interested in hearing your feedback so we can continually improve our books and learning resources for you. To participate in a brief online survey, please visit:
www.microsoft.com/learning/booksurvey
