
- •C and Objective-C
- •How this book works
- •How the life of a programmer works
- •Installing Apple’s developer tools
- •Getting started with Xcode
- •Where do I start writing code?
- •How do I run my program?
- •So what is a program?
- •Don’t stop
- •Types
- •A program with variables
- •Challenge
- •Boolean variables
- •When should I use a function?
- •How do I write and use a function?
- •How functions work together
- •Local variables, frames, and the stack
- •Recursion
- •Looking at the frames in the debugger
- •return
- •Global and static variables
- •Challenge
- •printf()
- •Integer operations
- •Integer division
- •Operator shorthand
- •Floating-point numbers
- •Tokens for displaying floating-point numbers
- •The while loop
- •The for loop
- •break
- •continue
- •The do-while loop
- •Challenge
- •Getting addresses
- •Storing addresses in pointers
- •Getting the data at an address
- •How many bytes?
- •NULL
- •Stylish pointer declarations
- •Challenges
- •Writing pass-by-reference functions
- •Avoid dereferencing NULL
- •Creating and using your first object
- •Message anatomy
- •Objects in memory
- •Challenge
- •Nesting message sends
- •Multiple arguments
- •Sending messages to nil
- •Challenge
- •Challenge
- •NSMutableArray
- •Reference pages
- •Quick Help
- •Other options and resources
- •Accessor methods
- •Dot notation
- •Properties
- •self
- •Multiple files
- •Challenge
- •Overriding methods
- •super
- •Challenge
- •Object ownership and ARC
- •Creating the Asset class
- •Adding a to-many relationship to Employee
- •Challenge
- •Retain cycles
- •Weak references
- •Zeroing of weak references
- •For the More Curious: Manual reference counting and ARC History
- •Retain count rules
- •NSArray/NSMutableArray
- •Immutable objects
- •Sorting
- •Filtering
- •NSSet/NSMutableSet
- •NSDictionary/NSMutableDictionary
- •Preprocessor directives
- •#include and #import
- •#define
- •Global variables
- •enum
- •#define vs global variables
- •Writing an NSString to a file
- •Reading files with NSString
- •Writing an NSData object to a file
- •Reading an NSData from a file
- •Target-action
- •Helper objects
- •Notifications
- •Which to use?
- •Callbacks and object ownership
- •Challenge
- •Getting started with iTahDoodle
- •BNRAppDelegate
- •Adding a C helper function
- •Objects in iTahDoodle
- •Model-View-Controller
- •The application delegate
- •Setting up views
- •Running on the iOS simulator
- •Wiring up the table view
- •Adding new tasks
- •Saving task data
- •For the More Curious: What about main()?
- •Edit BNRDocument.h
- •A look at Interface Builder
- •Edit BNRDocument.xib
- •Making connections
- •Revisiting MVC
- •Edit BNRDocument.m
- •Writing init methods
- •A basic init method
- •Using accessors
- •init methods that take arguments
- •Deadly init methods
- •Property attributes
- •Mutability
- •Lifetime specifiers
- •copy
- •More about copying
- •Advice on atomic vs. nonatomic
- •Key-value coding
- •Non-object types
- •Defining blocks
- •Using blocks
- •Declaring a block variable
- •Assigning a block
- •Passing in a block
- •typedef
- •Return values
- •Memory management
- •The block-based future
- •Challenges
- •Anonymous block
- •NSNotificationCenter
- •Bitwise-OR
- •Bitwise-AND
- •Other bitwise operators
- •Exclusive OR
- •Complement
- •Left-shift
- •Right-shift
- •Using enum to define bit masks
- •More bytes
- •Challenge
- •char
- •char *
- •String literals
- •Converting to and from NSString
- •Next Steps
- •Index
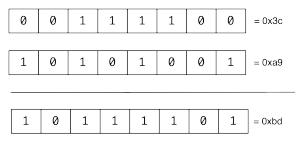
Chapter 33 |
Bitwise Operations |
|
|
|
|
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||||
$ hexdump myfile.txt |
|
|
|
|
|
|
|
|
|
|
|
|
||||
0000000 |
3c |
3f |
78 |
6d |
6c |
20 |
76 |
65 |
72 |
73 |
69 |
6f |
6e |
3d |
22 |
31 |
0000010 |
2e |
30 |
22 |
3f |
3e |
0a |
3c |
62 |
6f |
6f |
6b |
20 |
78 |
6d |
6c |
6e |
0000020 |
73 |
3d |
22 |
68 |
74 |
74 |
70 |
3a |
2f |
2f |
64 |
6f |
63 |
62 |
6f |
6f |
0000030 |
6b |
2e |
6f |
72 |
67 |
2f |
6e |
73 |
2f |
64 |
6f |
63 |
62 |
6f |
6f |
6b |
0000040 |
22 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
0000041 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
The first column is the offset (in hex) from the beginning of the file of the byte listed in the second column. Each two digit number represents one byte.
Bitwise-OR
If you have two bytes, you can bitwise-OR them together to create a third byte. A bit on the third byte will be 1 if at least one of the corresponding bits in the first two bytes is 1.
Figure 33.3 Two bytes bitwise-ORed together
This is done with the | operator. To try your hand at manipulating bits, create a new project: a C Command Line Tool (not Foundation) named bitwize.
Edit main.c:
#include <stdio.h>
int main (int argc, const char * argv[])
{
unsigned char a = 0x3c; unsigned char b = 0xa9; unsigned char c = a | b;
printf("Hex: %x | %x = %x\n", a, b, c); printf("Decimal: %d | %d = %d\n", a, b, c);
return 0;
}
When you run this program, you will see the two bytes bitwise-ORed together:
Hex: 3c | a9 = bd
Decimal: 60 | 169 = 189
What’s this good for? In Objective-C, we often use an integer to specify a certain setting. An integer is always a sequence of bits, and each bit is used to represent one aspect of the setting that can be turned
240

Bitwise-AND
on or off. We create this integer (also known as a bit mask) by picking and choosing from a set of constants. These constants are integers, too, and each constant specifies a single aspect of the setting by having only one of its bits turned on. You can bitwise-OR together the constants that represent the particular aspects you want. The result is the exact setting you’re looking for.
Let’s look at an example. iOS comes with a class called NSDataDetector. Instances of NSDataDetector go through text and look for common patterns like dates or URLs. The patterns an instance will look for is determined by the bitwise-OR result of a set of integer constants.
NSDataDetector.h defines these constants: NSTextCheckingTypeDate, NSTextCheckingTypeAddress, NSTextCheckingTypeLink, NSTextCheckingTypePhoneNumber, and
NSTextCheckingTypeTransitInformation. When you create an instance of NSDataDetector, you tell it what to search for. For example, if you wanted it to search for phone numbers and dates, you would do this:
NSError *e;
NSDataDetector *d = [NSDataDetector dataDetectorWithTypes: NSTextCheckingTypePhoneNumber|NSTextCheckingTypeDate
error:&e];
Notice the bitwise-OR operator. You’ll see this pattern a lot in Cocoa and iOS programming, and now you’ll know what’s going on behind the scenes.
Bitwise-AND
You can also bitwise-AND two bytes together to create a third. In this case, a bit on the third byte is 1 if the corresponding bits in the first two bytes are both 1.
Figure 33.4 Two bytes bitwise-ANDed together
This is done with the & operator. Add the following lines to main.c:
#include <stdio.h>
int main (int argc, const char * argv[])
{
unsigned char a = 0x3c; unsigned char b = 0xa9; unsigned char c = a | b;
printf("Hex: %x | %x = %x\n", a, b, c); printf("Decimal: %d | %d = %d\n", a, b, c);
241