
- •C and Objective-C
- •How this book works
- •How the life of a programmer works
- •Installing Apple’s developer tools
- •Getting started with Xcode
- •Where do I start writing code?
- •How do I run my program?
- •So what is a program?
- •Don’t stop
- •Types
- •A program with variables
- •Challenge
- •Boolean variables
- •When should I use a function?
- •How do I write and use a function?
- •How functions work together
- •Local variables, frames, and the stack
- •Recursion
- •Looking at the frames in the debugger
- •return
- •Global and static variables
- •Challenge
- •printf()
- •Integer operations
- •Integer division
- •Operator shorthand
- •Floating-point numbers
- •Tokens for displaying floating-point numbers
- •The while loop
- •The for loop
- •break
- •continue
- •The do-while loop
- •Challenge
- •Getting addresses
- •Storing addresses in pointers
- •Getting the data at an address
- •How many bytes?
- •NULL
- •Stylish pointer declarations
- •Challenges
- •Writing pass-by-reference functions
- •Avoid dereferencing NULL
- •Creating and using your first object
- •Message anatomy
- •Objects in memory
- •Challenge
- •Nesting message sends
- •Multiple arguments
- •Sending messages to nil
- •Challenge
- •Challenge
- •NSMutableArray
- •Reference pages
- •Quick Help
- •Other options and resources
- •Accessor methods
- •Dot notation
- •Properties
- •self
- •Multiple files
- •Challenge
- •Overriding methods
- •super
- •Challenge
- •Object ownership and ARC
- •Creating the Asset class
- •Adding a to-many relationship to Employee
- •Challenge
- •Retain cycles
- •Weak references
- •Zeroing of weak references
- •For the More Curious: Manual reference counting and ARC History
- •Retain count rules
- •NSArray/NSMutableArray
- •Immutable objects
- •Sorting
- •Filtering
- •NSSet/NSMutableSet
- •NSDictionary/NSMutableDictionary
- •Preprocessor directives
- •#include and #import
- •#define
- •Global variables
- •enum
- •#define vs global variables
- •Writing an NSString to a file
- •Reading files with NSString
- •Writing an NSData object to a file
- •Reading an NSData from a file
- •Target-action
- •Helper objects
- •Notifications
- •Which to use?
- •Callbacks and object ownership
- •Challenge
- •Getting started with iTahDoodle
- •BNRAppDelegate
- •Adding a C helper function
- •Objects in iTahDoodle
- •Model-View-Controller
- •The application delegate
- •Setting up views
- •Running on the iOS simulator
- •Wiring up the table view
- •Adding new tasks
- •Saving task data
- •For the More Curious: What about main()?
- •Edit BNRDocument.h
- •A look at Interface Builder
- •Edit BNRDocument.xib
- •Making connections
- •Revisiting MVC
- •Edit BNRDocument.m
- •Writing init methods
- •A basic init method
- •Using accessors
- •init methods that take arguments
- •Deadly init methods
- •Property attributes
- •Mutability
- •Lifetime specifiers
- •copy
- •More about copying
- •Advice on atomic vs. nonatomic
- •Key-value coding
- •Non-object types
- •Defining blocks
- •Using blocks
- •Declaring a block variable
- •Assigning a block
- •Passing in a block
- •typedef
- •Return values
- •Memory management
- •The block-based future
- •Challenges
- •Anonymous block
- •NSNotificationCenter
- •Bitwise-OR
- •Bitwise-AND
- •Other bitwise operators
- •Exclusive OR
- •Complement
- •Left-shift
- •Right-shift
- •Using enum to define bit masks
- •More bytes
- •Challenge
- •char
- •char *
- •String literals
- •Converting to and from NSString
- •Next Steps
- •Index

NSMutableArray
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[])
{
@autoreleasepool {
//Create three NSDate objects NSDate *now = [NSDate date];
NSDate *tomorrow = [now dateByAddingTimeInterval:24.0 * 60.0 * 60.0]; NSDate *yesterday = [now dateByAddingTimeInterval:-24.0 * 60.0 * 60.0];
//Create an array containing all three (nil terminates the list)
NSArray *dateList = [NSArray arrayWithObjects:now, tomorrow, yesterday, nil];
for (NSDate *d in dateList) { NSLog(@"Here is a date: %@", d);
}
}
return 0;
}
This type of loop is known as fast enumeration, and it is an extremely efficient way to walk through the items in an array. There is one restriction: you may not add to or remove from the array while enumerating over it.
NSMutableArray
Arrays come in two flavors:
•An instance of NSArray is created with a list of pointers. You can never add or remove a pointer from that array.
•An instance of NSMutableArray is similar to an instance of NSArray, but you can add and remove pointers. (NSMutableArray is a subclass of NSArray. More about subclasses in Chapter 18.)
Change your example to use an NSMutableArray instance and methods from the NSMutableArray class:
#import <Foundation/Foundation.h>
int main(int argc, const char * argv[])
{
@autoreleasepool {
//Create three NSDate objects NSDate *now = [NSDate date];
NSDate *tomorrow = [now dateByAddingTimeInterval:24.0 * 60.0 * 60.0]; NSDate *yesterday = [now dateByAddingTimeInterval:-24.0 * 60.0 * 60.0];
//Create an empty array
NSMutableArray *dateList = [NSMutableArray array];
// Add the dates to the array [dateList addObject:now]; [dateList addObject:tomorrow];
89

'""@-+ 5
! " # "
R#-: ; U" # " # IU&S
2A " D % &
2AD ['( Q['! %,
.
W " # "
R#-: ; L # IU&S
N6-T N # 0T 1 R#-; L # IU&S
.
-,
.
&& '
+ ''&! % ' N6%! L "@ ! ( " * ' ! 3K )G " !4 , ) & )' ) ) !
( ; + ' ! ) & + ' + & '' ' + @ G !
@ @
! " #$%
&
[ &
X ? %
2AA " A
I #2AA ) > N @ [' '
2A3;@8A V
23DD$,
B G ?
2A ? " I # A A B?A ['+ '$,
1 ?
2AA " % &
D G ' '
2AX I # N A [' ' 2A> / A$,
) K
*I 2A2 @ % &
2AD ['Q['! %,
.
.
.
-,
.
( & '' ' ! ( & 0 ) + ' ! B ' " &
34

%%
'' ' ) + ! , ; ' Q0 R ) G ' Q R+ " ! + & ' F !
B ' ) ) ' & +! ( ' ) ' 2 U!
2AA " I [' B>',
2AA " I [' ',
# / >$II 2AN A % &
2AD [' Z '%,
.
# / >$II 2AN % &
2AD [' '%,
.
# / >$II 2AN % &
2AD [' '%,
.
3
This page intentionally left blank
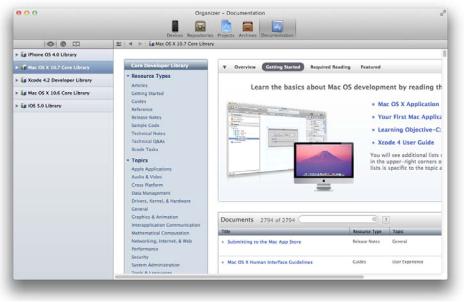
16
Developer Documentation
In the last chapter, I gave you some useful tidbits about the class NSArray. When you begin programming without my guidance (including taking on Challenges in this book), you will need to find such tidbits yourself. This is where Apple’s documentation comes in.
To see the documentation in Xcode, bring up the Organizer (click the button at the top right of the Xcode window) and choose the Documentation tab.
Figure 16.1 Docs in the Organizer
There are essentially five types of documentation that Apple provides:
•References: Every Objective-C class and every C function is tersely documented in these pages.
•Guides and Getting Starteds: Guides are less terse and group ideas conceptually. For example, there is an Error Handling Programming Guide that describes the myriad of ways that Mac and iOS developers can be informed that something has gone wrong.
93