
- •Contents
- •Introduction
- •About These Application Notes
- •What Is a Cell?
- •Cell Interconnect Delays
- •What Is Delay Back Annotation?
- •What Is Delay Calculation?
- •Prerequisites and Related Reading
- •Creating a Back Annotator
- •The Programming Language Interface (PLI) Mechanism
- •PLI Access Routines
- •Access Routine Families
- •Required UTILITY Routines
- •Access to Timing Information
- •Effect of Source Protection
- •Where to Look for More Information
- •Back Annotating Delays
- •Examples Used in This Chapter
- •Retrieving a Handle to the Object Associated with the Delay
- •Retrieving a Handle to a Net
- •Retrieving a Handle to a Module Path Delay
- •Retrieving a Handle to a Timing Check
- •Annotating the Delay
- •Determining How and Where to Place Delays
- •Annotating to Cells with Path Delays
- •Annotating to Cells with Lumped or Distributed Delays
- •Libraries with Mixed Cell Timing Descriptions
- •Annotating to Timing Checks
- •Calculating Delays
- •Examples Used in This Chapter
- •Scanning Cell Instances Within the Design
- •Determining the Scope of the Delay Calculation
- •Scan Methodology
- •Scanning Objects Within the Cell Instance
- •Relationship to Modeling Methodology
- •Scanning Path Delays
- •Scanning Cell Output Ports
- •Libraries with Mixed Cell Timing Descriptions
- •Types of Data Needed
- •Coding Data into Cell Descriptions (Attributes)
- •Retrieving Cell I/O Load Factors
- •Load Due to Interconnect Wire
- •Calculating and Annotating the Delays
- •Calculating the Delays
- •Annotating the Delays
- •Example Listings
- •Delay Back Annotators
- •Cell Output Nets to Lumped or Distributed Delay Cells
- •Interconnect Nets to Lumped or Distributed Delay Cells
- •Cell Output Nets to Path Delay Cells
- •Interconnect Nets to Path Delay Cells
- •Delay Calculators
- •Creating and Extracting Data From a Hash Table
- •Random Cell Scan and Cell Output Nets
- •Random Cell Scan and Cell Interconnect Nets
- •Delay Calculation Driven by Cell Output Nets
- •Delay Calculation Driven by a Cell Interconnect Nets
- •Index

Back Annotation and Delay Calculation
Calculating Delays
handle net;
{
if (cellout_count >= MAX_CELLOUT_HANDLES - 1)
{
io_printf("Error in dl_net_insert : cell handle overflow.%s\n", "Delay calculation may contain errors");
return;
} |
|
|
cellout_array[cellout_count++] = net; |
// store net in array |
|
} |
|
|
static bool dl_net_done(net) |
|
|
handle net; |
|
|
{ |
|
|
int i; |
|
|
if (cellout_count > 0) |
|
|
{ |
|
|
for (i = 0; i < cellout_count; i++) |
// search for net |
|
{ |
|
|
if (cellout_array[i] == net) |
|
|
return(TRUE); |
// return TRUE if net is found |
|
} |
|
|
} |
|
|
return(FALSE); |
//returns FALSE otherwise |
|
} |
|
|
Calculating and Annotating the Delays
Calculating the Delays
Use the data retrieved from the Veritool data structure and from external sources to calculate delays using the delay calculation formula specified for the target cell library. Because the access routines that place delays into the Veritool data structure expect the numbers to be passed in double precision floating point arguments, you must calculate the delays in this format.
Figure 4-35 on page 85 shows a C-language routine fragment that calculates delays for the example design shown in Figure 4-2 on page 45 , Figure 4-3 on page 46 and Figure 4-4 on page 46 . Interconnect delays for the associated cell library are calculated by multiplying the sum of the cell I/O loading capacitance plus the interconnect wire capacitance by a strength factor.
Figure 4-35 Calculating delays using retrieved data
static void dl_calc_path_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
October 2000 |
85 |
Product Version 3.2 |
|
Back Annotation and Delay Calculation |
|
Calculating Delays |
|
|
handle |
mod, path; |
handle |
pathout_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
. . .
/***************************************/ /*** Calculate load dependent delays ***/
/***************************************/
rise = rise_strength * (fanout_load + wire_load); fall = fall_strength * (fanout_load + wire_load);
. . .
}
}
}
Annotating the Delays
Determining where to place the delays
The modeling methodology used in the target Verilog-XL HDL cell library determines the types of structures to which the calculated delays are annotated. This dependency results from the fact that the two methods available for describing delays in the Verilog-XL HDL— primitive output delays and module path delays—are meant to be used separately. In general, you cannot use both constructs to describe delays within the same module. Therefore, for cells described with module path (pin-to-pin) delay timing, place the delays on the appropriate path delays within the cell instance. For cells described with lumped or distributed (primitive output) delay timing, place the delays on primitive outputs within the cell instance.
See “Libraries with Mixed Cell Timing Descriptions” on page 56 for information on calculating delays for libraries with mixed (path delay and distributed delay) cell timing descriptions.
Note that the examples presented in this chapter assume that the calculated delays represent incremental (interconnect only) delays. Therefore, the example routines use acc_append_delays to add the delays to those already present in the data structure. The resulting delay is the sum of the delay that appeared in the Verilog-XL HDL source description
October 2000 |
86 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
of the cell plus the calculated delay. If the delays that you calculate represent total delays, your delay calculation C-language routines must use acc_replace_delays to replace the delays in the data structure.
Annotating to cells with path delays
To annotate the calculated delays to a cell instance described with path delay timing, call the delay annotation access routine with a handle to the appropriate path delay. If, as described in “Scanning Path Delays” on page 52 you use a loop to scan the path delays in each cell instance, place this call within the loop.
Figure 4-36 on page 87 shows a C-language routine fragment that uses this method to calculate and annotate delays. This routine corresponds to the design in Figure 4-2 on page 45 , Figure 4-3 on page 46 and Figure 4-4 on page 46 . The routine scans the path delays in each cell instance, calculates the rise and fall interconnect delays (based on the current path output net), and adds these delays to the current path delay. Note that acc_configure has been called prior to calling this routine to configure acc_append_delays to accept rise and fall delays.
Figure 4-36 Annotating delays to path delays
static void dl_calc_path_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, path; |
handle |
pathout_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
. . .
/***************************************/ /*** Calculate load dependent delays ***/
/***************************************/
rise = rise_strength * (fanout_load + wire_load); fall = fall_strength * (fanout_load + wire_load);
/*****************************************/
October 2000 |
87 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
/*** Append delays to the driving path ***/
/*****************************************/ acc_append_delays(path, rise, fall);
. . .
}
}
}
Modifying path pulse control values
The pulse control values associated with path delays are not affected when the path delay is modified. You must modify these values explicitly. You can usually accomplish this modification within the same code that modifies the path delay. Figure 4-37 on page 88 shows an expanded version of the C-language routine fragment shown in Figure 4-36 on page 87 that resets the path pulse control values using acc_set_pulsere so that all pulses shorter than the new (combined) delay are rejected.
Figure 4-37 Setting path pulse control values using percentages passed to acc_set_pulsere
static void dl_calc_path_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, path; |
handle |
pathout_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
. . .
/*****************************************/ /*** Append delays to the driving path ***/
/*****************************************/ acc_append_delays(path, rise, fall); /**********************************************/ /*** Set pulse control to 100% x-generation ***/
/*** for new delay ***/
/**********************************************/ acc_set_pulsere(path,0.0,1.0);
. . .
}
October 2000 |
88 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
}
}
Use acc_append_pulsere, acc_replace_pulsere and acc_fetch_pulsere to manipulate path pulse control values in more complex ways. These routines allow you to access and modify the time values that delimit the pulse control regions directly, using actual delay values instead of percentages.
Annotating to cells with lumped or distributed (primitive output) delays
To annotate the calculated delays to a cell instance described with lumped or distributed (primitive output) delay timing, place the delays on the outputs of all primitive drivers (within the instance) that drive the appropriate cell output net. If, as described in “Scanning Cell Output Ports” on page 54 , you use a loop to scan the output ports of each cell instance, scan the drivers within this loop.
Figure 4-38 on page 90 shows a C-language routine fragment that uses this method to calculate and annotate delays. This routine corresponds to the design shown in Figure 4-1 on page 44 and Figure 4-4 on page 46 . This routine performs the following functions:
■scans the output ports of each cell instance
■calculates the rise and fall interconnect delays based on the cell output net that feeds the current cell output port
■adds these delays to the primitives (within the instance) that drive this net
The routine checks the value returned by acc_handle_parent for each primitive driver to ensure that it updates drivers only in the current cell instance.
October 2000 |
89 |
Product Version 3.2 |
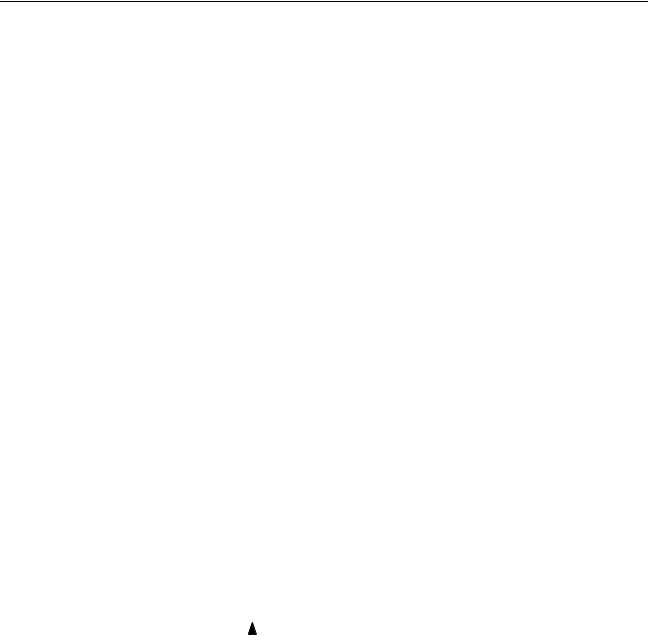
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-38 Annotating delays to primitive outputs
static void dl_calc_prim_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, term, prim, driver; |
handle |
portout, portout_net, portout_term; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/****************************************************/ /*** Process each output port in this cell module ***/
/****************************************************/ portout = null;
while (portout = acc_next_portout(mod,portout))
{ |
|
|
|
Scan all output |
|
. . . |
|
|
ports on cell |
|
|
|
|
|
|
continued . . . |
|||
|
|
|
October 2000 |
90 |
Product Version 3.2 |
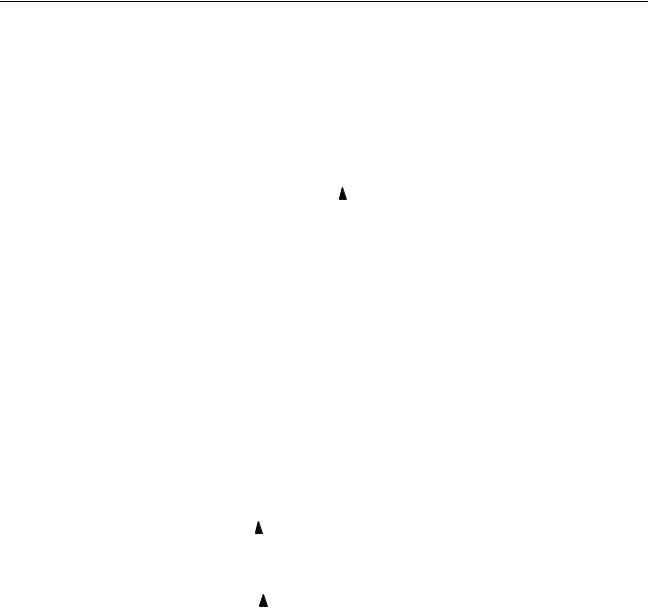
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-38 Annotating delays to primitive outputs, continued
/***********************************************/ /*** Process each net connected to this port ***/
/***********************************************/ portout_net = null;
while (portout_net = acc_next_loconn(portout,portout_net))
{ |
|
|
Scan all nets |
|
|
||
|
. . . |
|
connected to port |
|
|
||
|
|
|
|
|
/***************************************/ |
|
|
|
/*** Calculate load-dependent delays ***/ |
||
|
/***************************************/ |
|
|
|
rise = rise_strength * (fanout_load + wire_load); |
||
|
fall = fall_strength * (fanout_load + wire_load); |
/*************************************************/ /*** Scan the primitives which drive this port ***/
/*************************************************/ driver = null;
while (driver = acc_next_driver(portout_net,driver))
{ |
|
|
|
|
Scan all drivers on net |
|
|
|
|
||
|
|
|
|
||
prim = acc_handle_parent(driver); |
|
|
|||
|
|
||||
if (acc_handle_parent(prim) != mod) |
|
|
|||
continue; |
|
|
|
|
|
|
|
Skip if not in same instance |
|||
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
continued . . . |
October 2000 |
91 |
Product Version 3.2 |
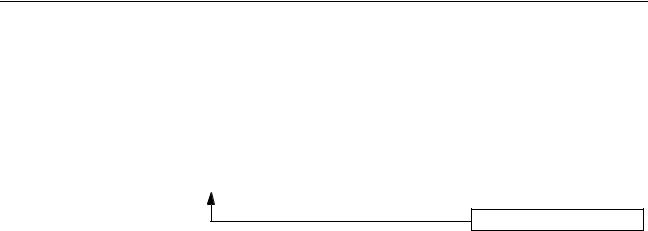
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-38 Annotating delays to primitive outputs, continued
/*******************************************/ /*** Add calculated delays to the delays ***/
/*** on the driving primitive ***/
/*******************************************/ acc_append_delays(prim, rise, fall);
. . .
Add delays to primitive
}
. . .
}
}
}
}
Use the access routines to move farther back into the cell if more complex distribution techniques are required.
October 2000 |
92 |
Product Version 3.2 |