
- •Contents
- •Introduction
- •About These Application Notes
- •What Is a Cell?
- •Cell Interconnect Delays
- •What Is Delay Back Annotation?
- •What Is Delay Calculation?
- •Prerequisites and Related Reading
- •Creating a Back Annotator
- •The Programming Language Interface (PLI) Mechanism
- •PLI Access Routines
- •Access Routine Families
- •Required UTILITY Routines
- •Access to Timing Information
- •Effect of Source Protection
- •Where to Look for More Information
- •Back Annotating Delays
- •Examples Used in This Chapter
- •Retrieving a Handle to the Object Associated with the Delay
- •Retrieving a Handle to a Net
- •Retrieving a Handle to a Module Path Delay
- •Retrieving a Handle to a Timing Check
- •Annotating the Delay
- •Determining How and Where to Place Delays
- •Annotating to Cells with Path Delays
- •Annotating to Cells with Lumped or Distributed Delays
- •Libraries with Mixed Cell Timing Descriptions
- •Annotating to Timing Checks
- •Calculating Delays
- •Examples Used in This Chapter
- •Scanning Cell Instances Within the Design
- •Determining the Scope of the Delay Calculation
- •Scan Methodology
- •Scanning Objects Within the Cell Instance
- •Relationship to Modeling Methodology
- •Scanning Path Delays
- •Scanning Cell Output Ports
- •Libraries with Mixed Cell Timing Descriptions
- •Types of Data Needed
- •Coding Data into Cell Descriptions (Attributes)
- •Retrieving Cell I/O Load Factors
- •Load Due to Interconnect Wire
- •Calculating and Annotating the Delays
- •Calculating the Delays
- •Annotating the Delays
- •Example Listings
- •Delay Back Annotators
- •Cell Output Nets to Lumped or Distributed Delay Cells
- •Interconnect Nets to Lumped or Distributed Delay Cells
- •Cell Output Nets to Path Delay Cells
- •Interconnect Nets to Path Delay Cells
- •Delay Calculators
- •Creating and Extracting Data From a Hash Table
- •Random Cell Scan and Cell Output Nets
- •Random Cell Scan and Cell Interconnect Nets
- •Delay Calculation Driven by Cell Output Nets
- •Delay Calculation Driven by a Cell Interconnect Nets
- •Index

Back Annotation and Delay Calculation
Calculating Delays
Libraries with Mixed Cell Timing Descriptions
If you are calculating delays for a description that contains cells with path delays and distributed delays, you must use some mechanism to determine which objects to scan on a cell-by-cell basis. Following are some of the methods you can use to accomplish this:
■scan method—Use acc_next_modpath to check for path delays within a cell, scanning cell outputs if none are found.
■attribute method—Declare a specparam in all cell descriptions that indicate the type of delay methodology used within the cell, and retrieve this specparam value using acc_fetch_attribute during delay calculation.
■table lookup method—Use acc_fetch_defname to retrieve the cell -type name during delay calculation and use this name in conjunction with an external (file) or internal (data structure) table lookup mechanism to determine which objects to scan.
Once you have determined the delay type for a particular cell instance, use the methods previously described in this section to scan the path delays or output ports within the instance.
Providing and Retrieving Delay
Calculation Data
Types of Data Needed
Typically, several pieces of data are needed to calculate delays. Some of this information, such as cell I/O loading factors and cell output drive factors, is the same for all instances of a given cell type. Other data, such as the loading capacitance due to interconnect wire, may be different for every cell instance in the design. This section describes methods of providing and retrieving both classes of data. Note that, although the examples in this section use data for a specific delay calculation formula to illustrate these methods, delay calculation data can always be placed into one of these two classes and thus handled with the generalized approaches described here.
Coding Data into Cell Descriptions (Attributes)
In most delay calculation processes, a subset of the data is constant across all instances of a given cell type. Typical pieces of data that fall into this category are as follows:
■cell I/O load factors
■cell output drive factors
October 2000 |
56 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
■delay scaling constants
Usually, each piece of data is associated with a particular object in the cell, such as a port, net or path delay. By using a special specparam naming convention in conjunction with the access routine acc_fetch_attribute, you can code this data directly into the Verilog-XL
HDL description of the cell and retrieve it during delay calculation. The pieces of data that you provide in the cell description are known as cell attributes.
To attach an attribute to an object within a cell, define a specparam in the cell’s specify block. This specparam must contain the name of the object as part of its full name using the convention described in Figure 4-12 on page 57 and Figure 4-13 on page 57 .
Figure 4-12 Specparam attribute naming conventions
Object |
Specparam naming convention |
|
|
module path delay |
<attribute_string><source><delim><dest> |
other |
<attribute_string><object_name> |
|
|
Figure 4-13 Definition of terms used in Figure 4-12 on page 57
Term |
Definition |
|
|
<attribute_string> |
User-defined string unique to the attribute |
<source> |
First path source net name |
<delim> |
Delimiter string set by acc_configure |
<dest> |
First path destination net name |
<object_name> |
Name of object associated with attribute |
|
|
Figure 4-2 on page 45 , repeated next as Figure 4-14 on page 58 , shows an example VerilogXL HDL cell module description with path delay timing that contains several attributes coded as specparams. The attributes are associated with path delays and nets within the module.
October 2000 |
57 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-14 Cell module with path delay timing and attributes coded as specparams
// D flip-flop path delay timing model ‘celldefine
module dflop(q,qb,clk,d); input clk, d;
output q, qb;‘
dff g1(q,clk,d); not g2(qb,q);
specify
//specparam attributes specparam FanoutLoad$clk = 1,
FanoutLoad$d = 2, FanoutLoad$q = 25, FanoutLoad$qb = 28, RiseStrength$clk$q = 5, FallStrength$clk$q = 4, RiseStrength$clk$qb = 9, FallStrength$clk$qb = 6;
//path delays
(clk *> q) = 102; (clk *> qb) = 156;
// timing checks $setup(d,edge[01,x1] clk,9); $hold(posedge clk,d,6);
endspecify endmodule ‘endcelldefine
Load factors attached to nets
Drive strengths attached to path delays
Figure 4-15 on page 59 shows an example Verilog-XL HDL cell module description with lumped (primitive output) delay timing that contains several attributes coded as specparams.
In this case, all of the attributes are associated with nets within the module.
October 2000 |
58 |
Product Version 3.2 |
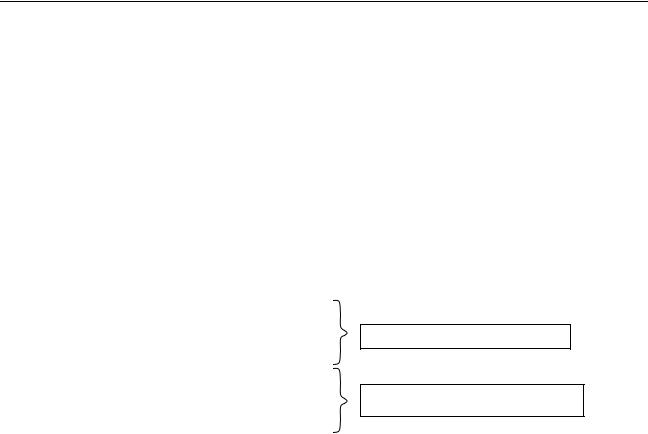
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-15 Cell module with lumped (primitive output) delay timing and attributes coded as specparams
// D flip-flop lumped delay timing model ‘celldefine
module dflop(q,qb,clk,d); input clk, d;
output q, qb;
dff g1(qi,clk,d); buf #102 g2(q,qi); not #156 g3(qb,qi);
specify
// specparam attributes specparam FanoutLoad$clk = 1,
FanoutLoad$d = 2,
FanoutLoad$q = 25,
FanoutLoad$qb = 28,
RiseStrength$q = 5,
FallStrength$q = 4,
RiseStrength$qb = 9,
FallStrength$qb = 6;
endspecify endmodule ‘endcelldefine
Load factors attached to nets
Drive strengths attached to nets
To retrieve specparam attributes from within your delay calculation facility, call acc_fetch_attribute with a handle to the object and the appropriate <attribute_string>. Figure 4-16 on page 59 shows a C-language routine fragment that retrieves attribute values that are attached to path delays with the attribute string RiseStrength$, as in the example design of Figure 4-2 on page 45 , Figure 4-3 on page 46 and Figure 4-4 on page 46 . Note that no call to acc_configure is needed because the attribute specparams use the default path delimiter string $.
Figure 4-16 Retrieving attribute information with acc_fetch_attribute
static void dl_calc_path_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle mod, path; handle pathout_net; int fanout_count;
October 2000 |
59 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
double fanout_load, wire_load;
double rise_strength, fall_strength, rise, fall; /**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
. . .
/*************************************************/ /*** Determine attributes associated with path ***/
/*************************************************/ rise_strength = acc_fetch_attribute(path, "RiseStrength$"); fall_strength = acc_fetch_attribute(path, "FallStrength$");
. . .
}
}
}
If acc_fetch_attribute does not find the attribute associated with the indicated object, it will look for a specparam attribute with a name identical to <attribute_string>. This provides a convenient way to describe an attribute that is associated with several objects in a cell. Figure 4-17 on page 61 shows an example cell description containing an attribute value described with the specparam FanoutLoad$ that is not attached to any specific object. The routine acc_fetch_attribute will return this value for the attribute when called with a handle to any object in the cell except input sel and output y. These two objects have their own explicitly associated attribute values.
October 2000 |
60 |
Product Version 3.2 |