
- •Contents
- •Introduction
- •About These Application Notes
- •What Is a Cell?
- •Cell Interconnect Delays
- •What Is Delay Back Annotation?
- •What Is Delay Calculation?
- •Prerequisites and Related Reading
- •Creating a Back Annotator
- •The Programming Language Interface (PLI) Mechanism
- •PLI Access Routines
- •Access Routine Families
- •Required UTILITY Routines
- •Access to Timing Information
- •Effect of Source Protection
- •Where to Look for More Information
- •Back Annotating Delays
- •Examples Used in This Chapter
- •Retrieving a Handle to the Object Associated with the Delay
- •Retrieving a Handle to a Net
- •Retrieving a Handle to a Module Path Delay
- •Retrieving a Handle to a Timing Check
- •Annotating the Delay
- •Determining How and Where to Place Delays
- •Annotating to Cells with Path Delays
- •Annotating to Cells with Lumped or Distributed Delays
- •Libraries with Mixed Cell Timing Descriptions
- •Annotating to Timing Checks
- •Calculating Delays
- •Examples Used in This Chapter
- •Scanning Cell Instances Within the Design
- •Determining the Scope of the Delay Calculation
- •Scan Methodology
- •Scanning Objects Within the Cell Instance
- •Relationship to Modeling Methodology
- •Scanning Path Delays
- •Scanning Cell Output Ports
- •Libraries with Mixed Cell Timing Descriptions
- •Types of Data Needed
- •Coding Data into Cell Descriptions (Attributes)
- •Retrieving Cell I/O Load Factors
- •Load Due to Interconnect Wire
- •Calculating and Annotating the Delays
- •Calculating the Delays
- •Annotating the Delays
- •Example Listings
- •Delay Back Annotators
- •Cell Output Nets to Lumped or Distributed Delay Cells
- •Interconnect Nets to Lumped or Distributed Delay Cells
- •Cell Output Nets to Path Delay Cells
- •Interconnect Nets to Path Delay Cells
- •Delay Calculators
- •Creating and Extracting Data From a Hash Table
- •Random Cell Scan and Cell Output Nets
- •Random Cell Scan and Cell Interconnect Nets
- •Delay Calculation Driven by Cell Output Nets
- •Delay Calculation Driven by a Cell Interconnect Nets
- •Index

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-17 Attaching attributes to multiple objects
// 2x1 multiplexer path delay timing model ‘celldefine
module mux21(y,i1,i2,sel); input i1, i2, sel;
output y;
bufif0 #56 g1(y,i1,sel); bufif1 #72 g2(y,i2,sel);
specify
// specparam attributes specparam FanoutLoad$sel = 2,
FanoutLoad$y = 15,
Load factor attached to inputs i1 and i2
FanoutLoad$ = 3, RiseStrength$y = 4, FallStrength$y = 3;
endspecify endmodule ‘endcelldefine
Note that you can use the routine acc_configure to control the value returned by acc_fetch_attribute when the indicated attribute is not found.
Retrieving Cell I/O Load Factors
A large portion of each cell interconnect delay typically results from the capacitive effects of cell I/O terminals on the cell output that drives them. The data related to these effects is usually provided in the form of a constant, or load factor, associated with each cell I/O terminal. This constant defines the incremental loading due to the terminal on any cell output that drives it. Because this data is the same for all cell instances of a given type, it may be coded and retrieved using the specparam attribute mechanism described earlier.
Figure 4-18 on page 62 shows a C-language routine, corresponding to the example designs in Figure 4-1 on page 44 through Figure 4-4 on page 46 , that retrieves the total loading factor on a net due to the cell I/O terminals to which it is connected. In this implementation, the individual load factors are described with the attribute string FanoutLoad$. This routine uses acc_next_cell_load (instead of the more general acc_next_load) to ensure that only one load is returned for each cell input or inout port driven by the net. The routine also uses acc_handle_parent when retrieving load factors due to other drivers on the net in order to skip drivers contained in the current cell instance.
October 2000 |
61 |
Product Version 3.2 |
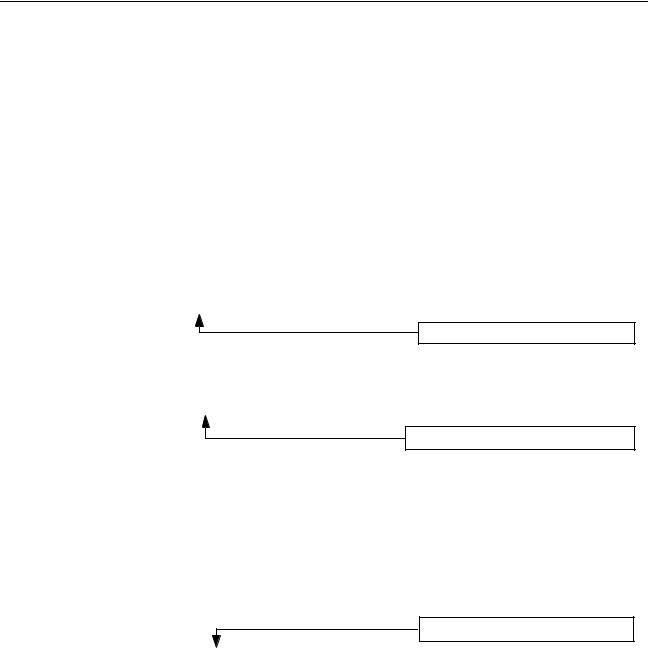
continued . . .
Product Version 3.2
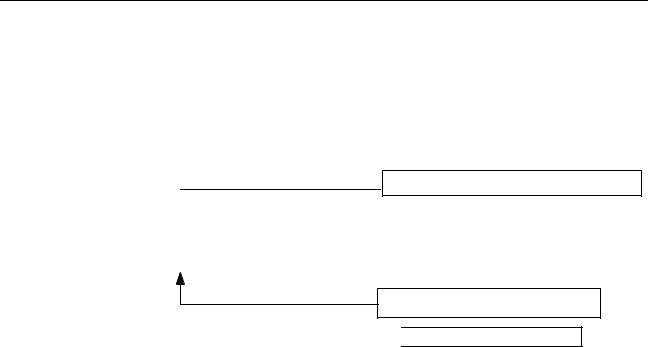
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-18 Retrieving cell I/O loading factors, continued
/* Don’t include load factor of driving terminal */ driver_prim = acc_handle_parent(driver); driver_mod = acc_handle_parent(driver_prim);
if (driver_mod == net_mod) continue;
/* Get net connected to this driver */ driver_net = acc_handle_conn(driver);
total_lf += acc_fetch_attribute(driver_net,"FanoutLoad$");
}
Retrieve and add load contribution
}
return (total_lf); Return total load on net
}
Load Due to Interconnect Wire
Pre-layout (estimated) vs. post-layout (actual)
Most delay calculation methodologies incorporate the effects of the interconnect metal used to connect cells together. The data related to these effects is usually provided in the form of a capacitance that is derived from the associated metal length. Since this data depends upon the interconnection of cells within the design, it varies for every cell instance. Thus, you cannot code it directly into your Verilog-XL HDL cell module descriptions. This section describes the following methods of incorporating this data into your delay calculation facility:
■estimating the capacitance based on the number of cell loads on the interconnect wire (pre-layout simulation)
■interpreting and retrieving the actual capacitance values from a list of interconnect nets and their associated capacitances (post-layout simulation)
Although the algorithms used to retrieve the data in these two scenarios are very different, you can write a generalized delay calculator that determines which method is being used and that takes the appropriate actions. To do this, use one of the following mechanisms to set the delay calculator into the proper mode when the Veritool is invoked:
■an argument passed to the delay calculation system task
October 2000 |
63 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
■a command-line option
Figure 4-19 on page 65 shows a C-language routine that uses an argument passed to the delay calculation system task to determine whether the interconnect wire capacitance is estimated based on the number of loads, or extracted from a capacitance back annotation file during delay calculation. The routine returns true if the interconnect wire capacitance should be estimated and false if the actual interconnect wire capacitance should be retrieved.
October 2000 |
64 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-19 Determining how to retrieve interconnect capacitance
static bool dl_process_estimate_arg()
{
int |
arg_type = tf_typep(2); |
char |
*arg = (char *)tf_getp(2); |
char |
net_filename[256]; |
Retrieve second system task argument
if (arg_type != tf_string)
{
io_printf("%s%s","Warning from delay calculation :",
" invalid second argument, will estimate wire capacitance\n");
/*** return true to indicate estimated capacitance ***/
return (true);
}
if ((arg == null) || (strcmp(arg, "estimate") == 0)) {
if (dl_verbose_flag)
Check for io_printf(" Wire capacitance will be estimated\n");estimate and
return true
/*** return true to indicate estimated capacitance ***/
return (true);
}
else
continued . . .
{
October 2000 |
65 |
Product Version 3.2 |
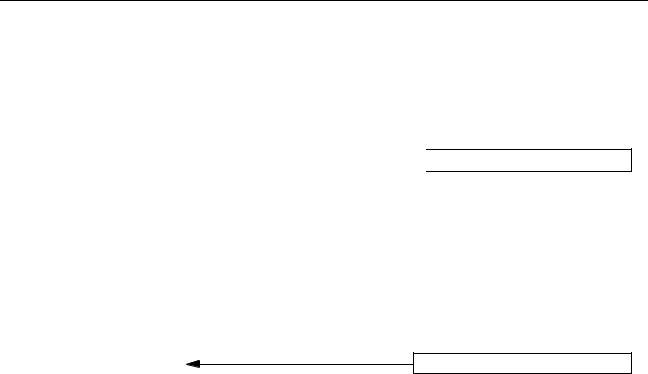
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-19 Determining how to retrieve interconnect capacitance, continued
if (dl_verbose_flag)
io_printf(" Wire capacitance file : \"%s\"\n", arg);
strcpy(net_filename, arg); Copy capacitance filename
if (!dl_make_backan_table(net_filename))
{
io_printf(" Wire capacitance will be estimated\n"); return(true);
}
/*** return false to indicate back annotated capacitance ***/
return (false);
}
}
Estimating interconnect wire capacitance
To estimate cell interconnect wire capacitance based on the number of cell loads on a wire, use acc_countwith a table lookup mechanism. Figure 4-20 on page 67 shows a C- language routine that accepts as input the number of cell loads on a net and returns a capacitance estimate based on this number. The capacitance estimation table, in Figure 4-20 on page 67 , is described with the array of double precision floating point numbers wirecap_est_table.
October 2000 |
66 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-20 Capacitance estimate table lookup routine |
|
|
|
|
||||||
/*** Wire capacitance estimation table ***/ |
|
|
|
|
||||||
int wirecap_est_table_size = 10; |
|
|
|
|
|
|
||||
double wirecap_est_table[] = |
|
|
|
|
|
|
|
|||
{1.2, 2.2, |
3.4, |
5.0, |
6.7, |
8.8, |
10.2, 12.7, |
15.3, |
18.0}; |
|
|
|
|
|
|||||||||
static double dl_estimate_capacitance(fanout_count) |
|
|
|
|||||||
|
|
|
|
|
|
|
|
|||
int fanout_count; |
|
|
|
|
Capacitance estimation table |
|||||
|
|
|
|
|
|
|
|
|
|
|
{
if (fanout_count > wirecap_est_table_size)
{
io_printf("Warning from dl_estimate_capacitance : ",
"excessive fanout\n");
fanout_count = wirecap_est_table_size;
}
Return estimated capacitance
return (wirecap_est_table[fanout_count-1]);
}
Figure 4-21 on page 67 shows a C routine fragment that uses acc_count to find the number of cell loads on a net and calls the routine shown in Figure 4-20 on page 67 to retrieve an estimate of the interconnect capacitance for this net. This routine uses acc_next_cell_load as an argument to acc_count (instead of the more general acc_next_load) so that only one load count is contributed by each cell input or inout port to which the net is connected.
Figure 4-21 Retrieving estimated interconnect wire capacitance based on load
static void dl_calc_path_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, path; |
handle |
pathout_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
October 2000 |
67 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
/*****************************************************/ /* Process each cell module instance in the scope */ /*****************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
/*********************/ /*** Count fanouts ***/
/*********************/
pathout_net = acc_handle_pathout(path);
fanout_count = acc_count(acc_next_cell_load,pathout_net);
. . .
/*********************************************************/ /* Determine estimated or backannotated wire capacitance */ /*********************************************************/ if (estimate_flag)
wire_load = dl_estimate_capacitance(fanout_count); else
/* call estimation routine */
wire_load = dl_backan_fetch(pathout_net);
. . .
}
)
Because capacitance estimation techniques vary in complexity from library to library, your mechanism may be somewhat different from the array indexing routine illustrated in Figure 4-20 on page 67 . For example, many libraries specify a simple linear relationship between the number of loads and the estimated wire capacitance. For this type of library, the estimation formula can be hard coded directly into the delay calculator; no table lookup mechanism is necessary. Other libraries, however, stipulate different capacitance estimation tables for different cell classes within the library. This type of library requires the use of multiple arrays and more complex cell recognition techniques in the associated delay calculator.
Retrieving interconnect wire capacitance from a file
To retrieve cell interconnect wire capacitance from a file, use standard C capabilities to read the file data into a data structure and extract it as you scan the design. One technique that provides an efficient data storage and retrieval mechanism involves the use of hash tables. A hash table is a data structure that contains information associated with a set of identifiers; the address at which a particular piece of data is stored is a mathematical function of the corresponding identifier. The use of relatively high-speed mathematical operations to derive the address directly minimizes the amount of computation time required to locate data,
October 2000 |
68 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
resulting in an efficient table lookup mechanism. A complete discussion of hash tables can be found in many programming applications manuals. Figure 4-22 on page 69 , Figure 4-23 on page 70 and Figure 4-24 on page 71 show C-language routines that read a capacitance back annotation file and use a simple hashing technique based on a handle to the identified object to derive the capacitance value storage location. The capacitance back annotation file must contain pairs of net names and capacitance values, as do the files in Figure 4-5 on page 47 and Figure 4-6 on page 47 .
Figure 4-22 Reading a file of cell interconnect wire capacitance values into a hash table
exfunc bool dl_make_backan_table(filename) char *filename;
{
FILE *net_file; double val; int num_lines; handle addr;
handle net_handle; char name[100]; int net_count; int i;
if ((net_file = fopen(filename,"r")) == 0)
{
io_printf("Warning: Back-annotate file \"%s\" not found.\n", filename);
return(false);
}
net_count = dl_get_numlines(filename);
/* allocate and initialize the hash table */ dl_init_backan_table(net_count);
for (i=0; i<net_count; i++)
{
/* read a net/capacitance value pair */
if (fscanf(net_file,"%s%lf\n",name,&val) == EOF)
{
io_printf("Warning: \"%s\", unexpected end of file.\n",filename);
break;
}
/* retrieve a handle to a net */ net_handle = acc_handle_object(name); if (net_handle == null)
io_printf("%s%s%s",
"Warning from file back annotation : net name \"", name,"\" not found\n");
else
/* place a net/capacitance in hash table */ dl_backan_insert(net_handle,val);
}
fflush(stdout); fclose(net_file);
October 2000 |
69 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
return(true);
}
Figure 4-23 Allocating and initializing the hash table
#define no_backan_val -1.0 int backan_table_size;
struct table
{
handle addr; double val;
} *backan_table;
Hash table data structure
static void dl_init_backan_table(net_count) int net_count;
{
int i;
Get hash table size
backan_table_size = dl_next_prime(net_count * 10); if (backan_table_size == 0)
{
io_printf("%s%s\n","Back-annotation error : ",
"required backan table too large; must enhance primes array"); return;
}
else
}
continued . . .
October 2000 |
70 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-23 Allocating and initializing the hash table, continued
backan_table = (struct table *)
malloc(backan_table_size * sizeof(struct table));
} Allocate hash table
for (i=0; i<backan_table_size; i++) backan_table[i].val = no_backan_val;
Initialize hash table
} |
|
|
|
|
|
|
int primes[] = { |
|
|
|
|
|
|
20011, 25013, |
30011, |
35023, 40009, 45007, |
50021 |
|
Array of prime |
|
|
|
|
|
|
|
|
80021, 100003, 150001, 200003, |
1000003, 5000011, |
|
numbers |
|||
|
|
|||||
0 |
|
|
|
|
|
|
}; |
|
|
|
|
|
|
exfunc int dl_next_prime(num) int num;
{
int i;
for (i=0; primes[i]!=0; i++) if (primes[i] > num)
return(primes[i]);
return(0); /* Exception value */
}
Figure 4-24 Inserting a value into the hash table
static void dl_backan_insert(addr,val) handle addr;
double val;
{
/* retrieve hash value */ int h = dl_hash(addr);
/* find empty backan table slot */
while (backan_table[h].val != no_backan_val)
Hash table size is the next largest prime number
October 2000 |
71 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
{
if (backan_table[h].addr == addr)
{
io_printf("Warning: value for net \"%s\" reassigned.\n", acc_fetch_fullname(addr));
break;
}
h = (h+1) % backan_table_size;
}
/* assign entry to backan table slot */ backan_table[h].val = val; backan_table[h].addr = addr;
}
static int dl_hash(addr) handle addr;
{
return(((int)addr) % backan_table_size);
}
Figure 4-25 on page 73 shows a C-language routine that accepts a net handle as input, retrieves the cell interconnect wire capacitance associated with the net from the hash table, and returns the data to the calling routine.
October 2000 |
72 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-25 Retrieving cell interconnect wire capacitance values from a hash table
exfunc double dl_backan_fetch(handle_val) handle handle_val;
{
int h = dl_hash(handle_val); int dup = 0;
Retrieve hash value
Find correct table entry
while (backan_table[h].addr != handle_val && dup <= backan_table_size && backan_table[h].val != no_backan_val)
{
dup++;
h = (h+1) % backan_table_size;
}
/* if every slot in the backan table was checked and the correct */ /* handle_val was not found, notify user of error */
if (dup > backan_table_size)
{
io_printf("Error: net %s not in back annotation file\n", acc_fetch_fullname(handle_val));
return(no_backan_val);
}
return(backan_table[h].val); |
|
Return net capacitance |
|
}
Figure 4-26 on page 73 shows a C-language routine fragment that scans the design and calls the routine shown in Figure 4-25 on page 73 with cell output net handles to retrieve the associated capacitance values, as needed.
Figure 4-26 Calling a routine with a net handle to retrieve capacitance values from a hash table
October 2000 |
73 |
Product Version 3.2 |
|
Back Annotation and Delay Calculation |
|
Calculating Delays |
|
|
static void dl_calc_path_delays(start_mod,estimate_flag) |
|
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, path; |
handle |
pathout_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/*********************************************/ /*** Process each path in this cell module ***/
/*********************************************/ path = null;
while (path = acc_next_modpath(mod,path))
{
. . .
/* retrieve handle to path output net */ pathout_net = acc_handle_pathout(path);
. . .
/**********************************************************/ /* Determine estimated or back-annotated wire capacitance */ /**********************************************************/ if (estimate_flag)
wire_load = dl_estimate_capacitance(fanout_count); else
/* retrieve capacitance value */
wire_load = dl_backan_fetch(pathout_net);
. . .
}
}
}
Retrieving the interconnect net associated with a cell output net
The handle used to retrieve the interconnect capacitance from the hash table must be for the exact same net named in the capacitance back annotation file in order for the routine to locate the data successfully. Even nets that are logically connected across module ports have unique handles. Therefore, if the capacitance back annotation file contains interconnect net names, you may need to retrieve a handle to the interconnect net associated with a given cell output net. If you have a handle to the output port fed by the cell output net (as in the delay calculator for lumped or distributed delay libraries in these application notes), you can accomplish this by calling acc_next_hiconn with the port handle.
Figure 4-27 on page 75 shows a C-language routine fragment that uses this method to retrieve a handle to the interconnect net that is associated with a given cell output net. This routine corresponds to the design shown in Figure 4-1 on page 44 and Figure 4-4 on page 46 . The routine passes the interconnect net handle to the routine shown in Figure 4-25 on
October 2000 |
74 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
page 73 to retrieve the appropriate capacitance value, assuming that the capacitance back annotation file contains interconnect net names.
Figure 4-27 Retrieving a handle to the interconnect net associated with a cell output net using a cell output port handle
static void dl_calc_prim_icn_delays(start_mod,estimate_flag)
handle |
start_mod; |
bool |
estimate_flag; |
{ |
|
handle |
mod, term, prim, driver; |
handle |
portout, portout_net, portout_term, interconnect_net; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/ mod = null;
while (mod = acc_next_cell(start_mod, mod))
{
/****************************************************/ /*** Process each output port in this cell module ***/
/****************************************************/ portout = null;
while (portout = acc_next_portout(mod,portout))
{
/***********************************************/ /*** Process each net connected to this port ***/
/***********************************************/ portout_net = null;
interconnect_net = null;
while (portout_net = acc_next_loconn(portout,portout_net))
{
/****************************************************/ /* If capacitance is backannotated, get interconnect */
/* |
net for this output net - break out at first |
*/ |
/* |
unconnected bit |
*/ |
/****************************************************/ if (!estimate_flag)
{
interconnect_net = acc_next_hiconn(portout,interconnect_net);
/* retrieve handle to interconnect net */ if (interconnect_net == null)
break;
}
. . .
. . .
/********************************************************/ /* Determine estimated or backannotated wire capacitance*/ /********************************************************/ if (estimate_flag)
wire_load = dl_estimate_capacitance(fanout_count); else
/* retrieve capacitance value */
wire_load = dl_backan_fetch(interconnect_net);
October 2000 |
75 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
. . .
}
}
}
}
If you do not have a handle to the output port that is fed by the cell output net (as in the delay calculator for path delay libraries presented in these application notes), you must scan the output ports of the cell and use acc_next_loconn to locate the port that is driven by the current cell output net. You can then call acc_next_hiconn to retrieve the associated interconnect net. Figure 4-28 on page 77 shows a C-language routine that accomplishes this task. Note that this routine assumes that only scalar nets are connected to cell ports.
October 2000 |
76 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-28 Retrieving a handle to the interconnect net associated with a cell output net when the cell output port is not known
static handle dl_handle_icn(mod,cell_output_net) handle mod, cell_output_net;
{
handle portout;
/********************************************************/ /** Scan output ports until the one fed by the net is **/ /** found, then return the hiconn net **/ /********************************************************/
Scan cell output ports portout = null;
while (portout = acc_next_portout(mod,portout))
{
if (acc_next_loconn(portout,null) == cell_output_net) return(acc_next_hiconn(portout,null));
} |
|
Check for correct port and |
|
||
|
|
return interconnect net |
|
|
/******************************************************/
/*** Print warning and return null if net not found ***/
/******************************************************/
io_printf("Warning from dl_handle_icn : output net \"%s\"
not found\n",acc_fetch_fullname(cell_output_net));
return(null);
}
Figure 4-29 on page 78 shows a C-language routine fragment that calls the routine in Figure 4-28 on page 77 to retrieve a handle to the interconnect net that is associated with a given path output net. This routine corresponds to the design in Figure 4-2 on page 45 , Figure 4-3 on page 46 and Figure 4-4 on page 46 . The handle is then passed to the routine in Figure 4-25 on page 73 to retrieve its associated capacitance value, assuming that the capacitance back annotation file contains interconnect net names.
October 2000 |
77 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-29 Retrieving the capacitance value associated with the interconnect net connected to a path output net
static void dl_calc_path_icn_delays(start_mod,estimate_flag)
handle |
start_mod; |
|
bool |
estimate_flag; |
|
{ |
|
|
handle |
mod, path; |
|
handle |
pathout_net, |
interconnect_net; |
int |
fanout_count; |
|
double |
fanout_load, |
wire_load; |
double |
rise_strength, fall_strength, rise, fall; |
/**********************************************************/ /*** Process each cell module instance within the scope ***/
/**********************************************************/
mod |
= null; |
|
while (mod = acc_next_cell(start_mod, mod)) |
|
|
{ |
|
|
/*********************************************/ |
|
|
/*** Process each path in this cell module ***/ |
|
|
/*********************************************/ |
|
|
path = null; |
|
|
while (path = acc_next_modpath(mod,path)) |
|
|
{ |
/*****************************/ |
|
|
|
|
|
/*** Get number of fanouts ***/ |
|
|
/*****************************/ |
|
|
pathout_net = acc_handle_pathout(path); |
|
|
fanout_count = acc_count(acc_next_cell_load,pathout_net); |
|
|
if (estimate_flag && (fanout_count == 0)) |
|
|
continue; |
|
|
if (!estimate_flag) |
|
|
/*retrieve handle to interconnect net */ |
|
|
interconnect_net = dl_handle_icn(mod,pathout_net); |
|
|
. . . |
|
|
/******************************************************/ |
|
|
/* Determine estimated or backannotated |
*/ |
|
/* wire capacitance |
*/ |
/******************************************************/ if (estimate_flag)
wire_load = dl_estimate_capacitance(fanout_count); else
/* retrieve capacitance value */
wire_load = dl_backan_fetch(interconnect_net);
. . .
}
}
}
Scanning the design using the capacitance back annotation file
The existence of a capacitance back annotation file presents an alternative (and potentially faster) method of scanning the design and performing delay calculation. With this method, use the net name contained in the file to retrieve a handle to the net within the design. Once you have retrieved this handle, perform the delay calculation for the net and all primitives or
October 2000 |
78 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
path delays connected to it, limiting the calculation to a single cell if cell output nets are identified in the file.
This file-driven algorithm replaces the methods, previously presented, that use acc_next_cell to scan the cell instances for a particular delay calculation. Because the
file-driven approach eliminates the table storage and lookup steps, the resulting delay calculator will usually be faster than a delay calculator that scans the design with acc_next_cell.
The viability of this method depends upon the structure and content of the capacitance back annotation file; it may not be possible or practical in all cases (for example, when only some of the cell output nets or interconnect nets are listed in the file, and the other nets use a default capacitance value).
Figure 4-30 on page 79 shows a C-language routine fragment that reads the example capacitance back annotation file shown in Figure 4-5 on page 47 , retrieves handles to the cell output nets named in the file, and calculates delays to be placed on the appropriate primitive drivers or paths within the cell.
Figure 4-30 Using the cell output net names in a capacitance back annotation file to scan the design
static void dl_calc_delays(prim_or_path,net_filename) int prim_or_path;
char *net_filename;
{
handle |
cellout_net; |
FILE |
*net_file; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
char |
cellout_name[256]; |
/*****************************************************/ /*** Read each net/capacitance pair and calculate ****/
/*** the delays for the net ***/
/*****************************************************/ if ((net_file = fopen(net_filename,"r")) == null)
{
io_printf("Warning: Back-annotate file \"%s\" not found. %s\n", net_filename,"No delays will be calculated.");
return;
}
/* read a net/capacitance value pair */
while (fscanf(net_file,"%s%lf\n",cellout_name,&wire_load) != EOF)
{
/* retrieve handle to cell output/net */
if ((cellout_net = acc_handle_object(cellout_name)) == null)
{
io_printf("Warning: Cell output net \"%s\" not found.\n", cellout_name);
October 2000 |
79 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
continue;
}
/*********************/ /*** Count fanouts ***/
/*********************/
fanout_count = acc_count(acc_next_cell_load,cellout_net);
. . .
/* calculate and annotate delays */ if (prim_or_path == dlPrim)
dl_ann_prim_delays(cellout_net,fanout_load,wire_load); else
dl_ann_path_delays(cellout_net,fanout_load,wire_load);
}
}
Figure 4-31 on page 80 and Figure 4-32 on page 81 show the C-language routines that are called by the routine shown in Figure 4-30 on page 79 to calculate and annotate the delays to the appropriate primitives or path delays. Note that the routine shown in Figure 4-32 on page 81 must scan all of the path delays in the module and identify those that feed the given cell output net.
Figure 4-31 Calculating interconnect delays for primitives that drive a cell output net
static void dl_ann_prim_delays(net,fanout_load,wire_load) handle net;
double fanout_load, wire_load;
{
double rise_strength, fall_strength; double rise, fall;
handle driver, prim, parent;
/****************************************************/ /*** Determine attributes associated with the net ***/
/****************************************************/ rise_strength = acc_fetch_attribute(net, "RiseStrength$"); fall_strength = acc_fetch_attribute(net, "FallStrength$");
/***************************************/ /*** Calculate load dependent delays ***/
/***************************************/
rise = rise_strength * (fanout_load + wire_load); fall = fall_strength * (fanout_load + wire_load);
/*******************************************************/ /*** Add the delays to all primitives in the current ***/
/*** module that drive this net ***/
/*******************************************************/ parent = acc_handle_parent(net);
driver = null;
while (driver = acc_next_driver(net,driver))
{
prim = acc_handle_parent(driver);
if (acc_handle_parent(prim) != parent) continue;
. . .
}
}
October 2000 |
80 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-32 Calculating interconnect delays for paths that feed a cell output net
static void dl_ann_path_delays(net,fanout_load,wire_load) handle net;
double fanout_load, wire_load;
{
handle parent, path;
double rise_strength, fall_strength, rise, fall;
/***********************************************************/ /* For each path that feeds the net, retrieve the strength */ /* attributes, calculate and annotate the delays */ /***********************************************************/ parent = acc_handle_parent(net);
path = null;
while (path = acc_next_modpath(parent,path))
{
if (acc_handle_pathout(path) != net) continue;
/*************************************************/ /*** Determine attributes associated with path ***/
/*************************************************/ rise_strength = acc_fetch_attribute(path, "RiseStrength$"); fall_strength = acc_fetch_attribute(path, "FallStrength$");
/***************************************/
/*** Calculate load dependent delays ***/
/***************************************/
rise = rise_strength * (fanout_load + wire_load); fall = fall_strength * (fanout_load + wire_load);
/*****************************************/
/*** Append delays to the driving path ***/
/*****************************************/
. . .
}
If the capacitance back annotation file contains interconnect net names instead of cell output net names, you must calculate delays for all cell instances that drive the appropriate net. To accomplish complete coverage, use acc_next_driver to retrieve a handle to a driver within each of these cell instances. Then, use acc_handle_conn to retrieve the appropriate cell output net.
If wired logic is present within the cells, you must use caution to ensure that you calculate delays only once for a given cell output net.
Figure 4-33 on page 82 shows a C-language routine fragment that reads the example capacitance back annotation file shown in Figure 4-6 on page 47 , retrieves handles to the interconnect nets named in the file, and uses the method described previously to scan all of the cell output nets connected to an interconnect net. This routine keeps a list of handles to the associated cell output nets as each interconnect net is processed and checks the list to ensure that it does not calculate delays for a particular cell output net more than once.
October 2000 |
81 |
Product Version 3.2 |
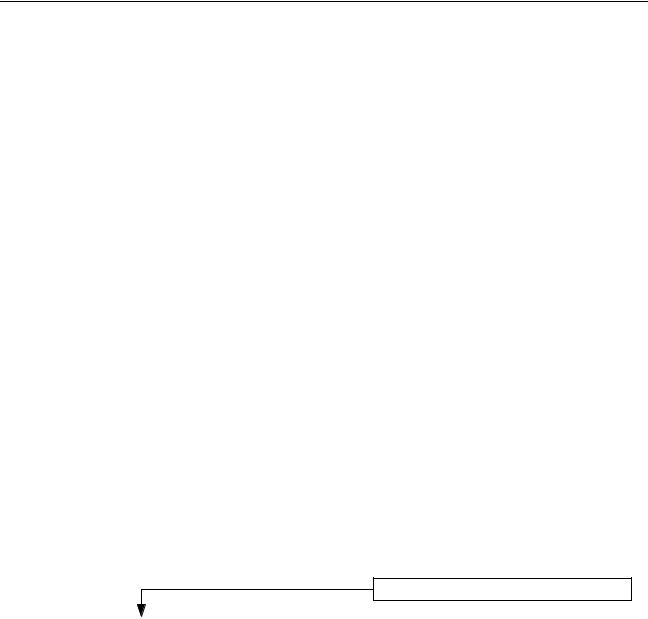
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-33 Using the interconnect net names in a capacitance backannotation file to scan the design
static void dl_calc_delays(prim_or_path,net_filename) int prim_or_path;
char *net_filename;
{
handle |
interconnect_net, cellout_net; |
handle |
driver; |
FILE |
*net_file; |
int |
fanout_count; |
double |
fanout_load, wire_load; |
char |
interconnect_name[256]; |
/***********************************************************/
/*** |
Read each net/capacitance pair and calculate |
***/ |
/*** |
the delays for the net |
***/ |
/***********************************************************/ if ((net_file = fopen(net_filename,"r")) == null)
{
io_printf("Warning: Back-annotate file \"%s\" not found.%s\n", net_filename,"No delays will be calculated.");
return;
Read a net/capacitance value pair
}
while (fscanf(net_file,"%s%lf\n",interconnect_name,&wire_load)
!= EOF)
continued . . .
October 2000 |
82 |
Product Version 3.2 |
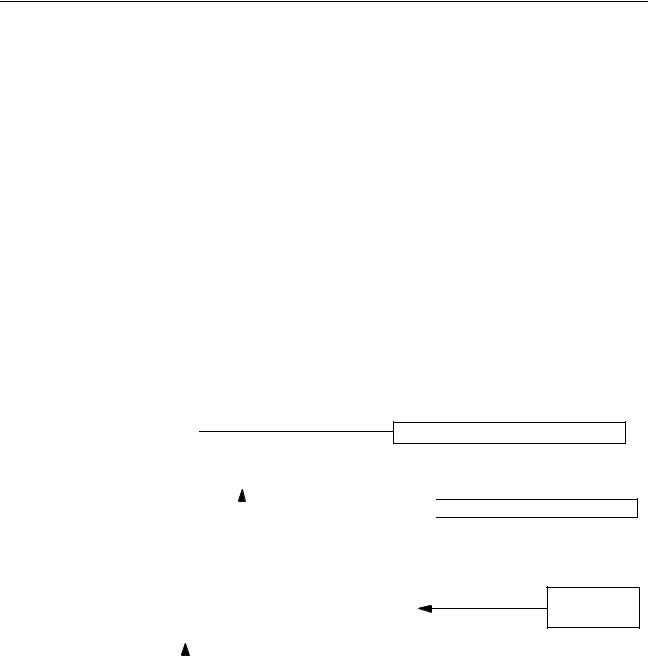
Back Annotation and Delay Calculation
Calculating Delays
Figure 4-33 Using the interconnect net names in a capacitance backannotation file to scan the design, continued
{
if ((interconnect_net = acc_handle_object(interconnect_name)) == null)
{
io_printf("Warning: Interconnect net \"%s\" not found.\n", interconnect_name);
continue;
}
. . .
/****************************************************/ /*** Scan all cells with ports that feed this net ***/
/****************************************************/
dl_net_init(); driver = null;
while (driver = acc_next_driver(interconnect_net,driver)) {
/**************************************/
/*** Skip if this net has been done ***/
/**************************************/
cellout_net = acc_handle_conn(driver);
if (dl_net_done(cellout_net))
Retrieve cell output net
continue; |
|
Skip if net has been done |
|
||
|
||
|
|
|
continued . . .
October 2000 |
83 |
Product Version 3.2 |

Back Annotation and Delay Calculation
Calculating Delays
Figure 4-33 Using the interconnect net names in a capacitance backannotation file to scan the design, continued
/*********************/ /*** Count fanouts ***/
*********************/
fanout_count = acc_count(acc_next_cell_load,cellout_net);
. . .
/*****************************************/ /*** Calculate and annotate the delays ***/
/*****************************************/ if (prim_or_path == dlPrim)
dl_ann_prim_delays(cellout_net,fanout_load,wire_load); else
dl_ann_path_delays(cellout_net,fanout_load,wire_load);
dl_net_insert(cellout_net);
} Store handle to net
. . .
}
}
Figure 4-34 on page 84 shows the C-language routines that store a cell output net handle, check for its existence in the list, and initialize the associated data structures.
Figure 4-34 Routines to store cell output net handles and check whether they have been processed
#define MAX_CELLOUT_HANDLES 256
int cellout_count; // net storage count and array handle cellout_array[MAX_CELLOUT_HANDLES];
static void dl_net_init() |
|
{ |
|
cellout_count = 0; |
// initialize count of stored nets |
} |
|
static void dl_net_insert(net) |
|
October 2000 |
84 |
Product Version 3.2 |