
- •Chapter 1. Introduction
- •How to Develop A Program
- •What is an Assembler?
- •Modular Programming
- •Modular Program Development Process
- •Segments, Modules, and Programs
- •Translate and Link Process
- •Filename Extensions
- •Program Template File
- •Chapter 2. Architecture Overview
- •Memory Classes and Memory Layout
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •CPU Registers
- •CPU Registers of the 8051 Variants
- •CPU Registers of the Intel/Atmel WM 251
- •Program Status Word (PSW)
- •Instruction Sets
- •Opcode Map
- •8051 Instructions
- •Additional 251 Instructions
- •Additional 80C51MX Instructions via Prefix A5
- •Chapter 3. Writing Assembly Programs
- •Assembly Statements
- •Directives
- •Controls
- •Instructions
- •Comments
- •Symbols
- •Symbol Names
- •Labels
- •Operands
- •Special Assembler Symbols
- •Immediate Data
- •Memory Access
- •Program Addresses
- •Expressions and Operators
- •Numbers
- •Characters
- •Character Strings
- •Location Counter
- •Operators
- •Expressions
- •Chapter 4. Assembler Directives
- •Introduction
- •Segment Directives
- •Location Counter
- •Generic Segments
- •Stack Segment
- •Absolute Segments
- •Default Segment
- •SEGMENT
- •RSEG
- •BSEG, CSEG, DSEG, ISEG, XSEG
- •Symbol Definition
- •CODE, DATA, IDATA, XDATA
- •esfr, sfr, sfr16, sbit
- •LIT (AX51 & A251 only)
- •Memory Initialization
- •DD (AX51 & A251 only)
- •Reserving Memory
- •DBIT
- •DSW (AX51 & A251 only)
- •DSD (AX51 & A251 only)
- •Procedure Declaration (AX51 & A251 only)
- •PROC / ENDP (AX51 & A251 only)
- •LABEL (AX51 and A251 only)
- •Program Linkage
- •PUBLIC
- •EXTRN / EXTERN
- •NAME
- •Address Control
- •EVEN (AX51 and A251 only)
- •USING
- •Other Directives
- •_ _ERROR_ _
- •Chapter 5. Assembler Macros
- •Standard Macro Directives
- •Defining a Macro
- •Parameters
- •Labels
- •Repeating Blocks
- •REPT
- •IRPC
- •Nested Definitions
- •Nested Repeating Blocks
- •Recursive Macros
- •Operators
- •NUL Operator
- •& Operator
- •< and > Operators
- •% Operator
- •;; Operator
- •! Operator
- •Invoking a Macro
- •C Macros
- •C Macro Preprocessor Directives
- •Stringize Operator
- •Predefined C Macro Constants
- •Examples with C Macros
- •C Preprocessor Side Effects
- •Chapter 6. Macro Processing Language
- •Overview
- •Creating and Calling MPL Macros
- •Creating Parameterless Macros
- •MPL Macros with Parameters
- •Local Symbols List
- •Macro Processor Language Functions
- •Comment Function
- •Escape Function
- •Bracket Function
- •METACHAR Function
- •Numbers and Expressions
- •Numbers
- •Character Strings
- •SET Function
- •EVAL Function
- •Logical Expressions and String Comparison
- •Conditional MPL Processing
- •IF Function
- •WHILE Function
- •REPEAT Function
- •EXIT Function
- •String Manipulation Functions
- •LEN Function
- •SUBSTR Function
- •MATCH Function
- •Console I/O Functions
- •Advanced Macro Processing
- •Literal Delimiters
- •Blank Delimiters
- •Identifier Delimiters
- •Literal and Normal Mode
- •MACRO Errors
- •Chapter 7. Invocation and Controls
- •Environment Settings
- •Running Ax51
- •ERRORLEVEL
- •Output Files
- •Assembler Controls
- •Controls for Conditional Assembly
- •Conditional Assembly Controls
- •Chapter 8. Error Messages
- •Fatal Errors
- •Non–Fatal Errors
- •Chapter 9. Linker/Locator
- •Overview
- •Combining Program Modules
- •Segment Naming Conventions
- •Combining Segments
- •Locating Segments
- •Overlaying Data Memory
- •Resolving External References
- •Absolute Address Calculation
- •Generating an Absolute Object File
- •Generating a Listing File
- •Bank Switching
- •Using RTX51, RTX251, and RTX51 Tiny
- •Linking Programs
- •Command Line Examples
- •Control Linker Input with µVision2
- •ERRORLEVEL
- •Output File
- •Linker/Locater Controls
- •Locating Programs to Physical Memory
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •Data Overlaying
- •Program and Data Segments of Functions
- •Using the Overlay Control
- •Tips and Tricks for Program Locating
- •Locate Segments with Wildcards
- •Special ROM Handling (LX51 & L251 only)
- •Bank Switching
- •Common Code Area
- •Code Bank Areas
- •Bank Switching Configuration
- •Configuration Examples
- •Control Summary
- •Listing File Controls
- •Output File Controls
- •Segment and Memory Location Controls
- •High-Level Language Controls
- •Error Messages
- •Warnings
- •Non-Fatal Errors
- •Fatal Errors
- •Exceptions
- •Chapter 10. Library Manager
- •Using LIBx51
- •Interactive Mode
- •Create Library within µVision2
- •Command Summary
- •Creating a Library
- •Adding or Replacing Object Modules
- •Removing Object Modules
- •Extracting Object Modules
- •Listing Library Contents
- •Error Messages
- •Fatal Errors
- •Errors
- •Chapter 11. Object-Hex Converter
- •Using OHx51
- •OHx51 Command Line Examples
- •Creating HEX Files for Banked Applications
- •OHx51 Error Messages
- •Using OC51
- •OC51 Error Messages
- •Intel HEX File Format
- •Record Format
- •Data Record
- •Extended 8086 Segment Record
- •Extended Linear Address Record
- •Example Intel HEX File
- •Appendix A. Application Examples
- •ASM – Assembler Example
- •Using A51 and BL51
- •Using AX51 and LX51
- •Using A251 and L251
- •CSAMPLE – C Compiler Example
- •Using C51 and BL51
- •Using C51 and LX51
- •Using C251 and L251
- •BANK_EX1 – Code Banking with C51
- •Using C51 and BL51
- •Using C51 and LX51
- •BANK_EX2 – Banking with Constants
- •Using C51 and BL51
- •Using C51 and LX51
- •Using BL51
- •Using C51 and LX51
- •Philips 80C51MX – Assembler Example
- •Philips 80C51MX – C Compiler Example
- •Appendix B. Reserved Symbols
- •Appendix C. Listing File Format
- •Assembler Listing File Format
- •Listing File Heading
- •Source Listing
- •Macro / Include File / Save Stack Format
- •Symbol Table
- •Listing File Trailer
- •Appendix D. Assembler Differences
- •Differences Between A51 and A251/AX51
- •Differences between A51 and ASM51
- •Differences between A251/AX51 & ASM51
- •Glossary
- •Index
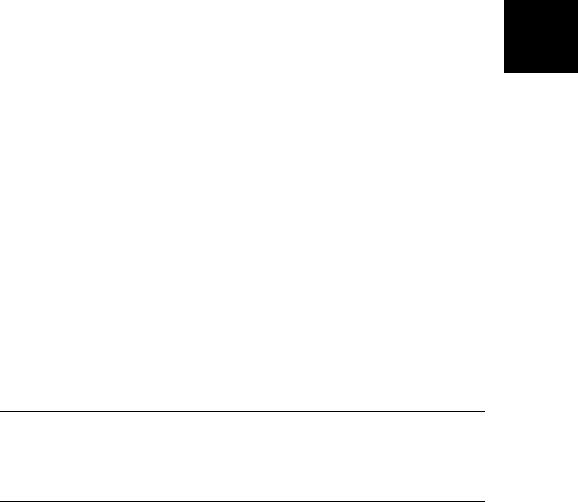
Keil Software — A51/AX51/A251 Macro Assembler and Utilities |
257 |
|
|
“Tips and Tricks for Program Locating” on page 289 shows you several additional features of the Lx51 linker/locater. These features allow you to create
in-system programmable applications, to determine the addresses of segments, to 9 use the C251 memory class NCONST without ROM in segment 0, or to locate
several segments within a 2KB block.
“Bank Switching” on page 293 describes what bank switching is and how it is implemented by the Lx51 linker/locator. This chapter also shows how to make applications that are larger than 64 KBytes work with code banking.
“Control Summary” on page 305 lists the command-line controls by category and provides you with descriptions of each, along with examples.
“Error Messages” on page 360 lists the errors that you may encounter when you use the Lx51 linker/locator.
Overview
The Lx51 linker/locator takes the object files and library files you specify and generates a absolute object file. Absolute object files can be loaded into debugging tools or may be converted into Intel HEX files for PROM programming by OHx51 Object-Hex Converter.
NOTE
Banked object files generated by the BL51 linker/locater must be converted by the OC51 Banked Object File Converter into absolute object files (one for each bank) before they can be converted into Intel HEX files by the OH51 Object-Hex Converter.
While processing object and library files, the Lx51 linker/locator performs the following operations.

258 Chapter 9. Linker/Locator
|
Combining Program Modules |
|
9 |
||
The object modules that the Lx51 linker/locator combines are processed in the |
||
order in which they are specified on the command line. The Lx51 linker/locator |
||
|
processes the contents of object modules created with the Ax51 assembler or the |
|
|
Cx51 compiler. Library files, however, contain a number of different object |
|
|
modules; and, only the object modules in the library file that specifically resolve |
|
|
external references are processed by the Lx51 linker/locator. |
Segment Naming Conventions
Objects generated by the Cx51 and Intel PL/M-51 compilers are stored in segments, which are units of code or data memory. A segment may be relocatable or may be absolute. Each relocatable segment has a type and a name. This section describes the conventions used for naming these segments.
Segment names include a module_name. The module_name is the name of the source file in which the object is declared and excludes the drive letter, path specification, and file extension. In order to accommodate a wide variety of existing software and hardware tools, all segment names are converted and stored in uppercase.
Each segment name has a prefix (or in case of PL/M-51 a postfix) that corresponds to the memory type used for the segment. The prefix is enclosed in question marks (?). The following is a list of the standard segment name prefixes.
Segment Prefix |
Memory Class |
Description |
?PR? |
CODE |
Executable program code |
?CO? |
CONST |
Constant data in program memory |
?ED? |
EDATA |
EDATA memory for near variables |
?FD? |
HDATA |
HDATA memory for far variables |
?XD? |
XDATA |
XDATA memory |
?DT? |
DATA |
DATA memory |
?ID? |
IDATA |
IDATA memory |
?BI? |
BIT |
Bit data in internal data memory |
?BA? |
DATA |
Bit-addressable data in internal data memory |
?PD? |
XDATA |
Paged data in XDATA memory |
For detailed information about the segment naming conventions refer to the Cx51 Compiler User’s Guide.

Keil Software — A51/AX51/A251 Macro Assembler and Utilities |
259 |
|
|
Combining Segments
A segment is a code or data block that is created by the compiler or assembler |
9 |
|
from your source code. There are two basic types of segments: absolute and |
||
relocatable. Absolute segments reside in a fixed memory location. They cannot |
|
|
be moved by the linker. Absolute segments do not have a segment name and will |
|
|
not be combined with other segments. Relocatable segments have a name and a |
|
|
type (as well as other attributes shown in the table below). Relocatable segments |
|
|
with the same name but from different object modules are considered parts of the |
|
|
same segment and are called partial segments. The linker/locator combines these |
|
|
partial relocatable segments. |
|
|
The following table lists the segment attributes that are used to determine how to |
|
|
link, combine, and locate code or data in the segment. |
|
|
|
|
|
Attribute |
Description |
|
Name |
Each relocatable segment has a name that is used when combining |
|
|
relocatable segments from different program modules. Absolute |
|
|
segments do not have names. |
|
Memory Class |
The memory class identifies the address space to which the segment |
|
|
belongs. For BL51 the type can be CODE, XDATA, DATA, IDATA, or |
|
|
BIT. LX51 and L251 support in addition CONST, EBIT, ECONST, |
|
|
EDATA, HDATA, HCODE, HCONST, and user-define memory classes. |
|
Relocation Type |
The relocation type specifies the relocation operations that can be |
|
|
performed by the linker/locator. Valid relocation types are AT address, |
|
|
BITADDRESSABLE, INBLOCK, INPAGE, INSEG, OFFS offset, and |
|
|
OVERLAYABLE. |
|
Alignment Type |
The alignment type specifies the alignment operations that can be |
|
|
performed by the linker/locator. Valid alignment types are BIT, BYTE, |
|
|
WORD, DWORD, PAGE, BLOCK, and SEG. |
|
Length |
The length attribute specifies the length of the segment. |
|
Base Address |
The base address specifies the first assigned address of the segment. |
|
|
For absolute segments, the address is assigned by the assembler. For |
|
|
relocatable segments, the address is assigned by the linker/locator. |
|
|
|
|
While processing your program modules, the linker/locator produces a table or map of all segments. The table contains name, type, location method, length, and base address of each segment. This table aids in combining partial relocatable segments. All partial segments having the same name are combined by the linker/locator into one single relocatable segment.
|
260 |
Chapter 9. Linker/Locator |
|
|
|
|
The linker/locator uses the following rules when combining partial segments. |
|
9 |
|
All partial segments that share a common name must have the same memory |
|
||
|
|
class. An error occurs if the types do not correspond. |
|
|
The length of the combined segments must not exceed the length of the |
|
|
physical memory area. |
|
|
The location method for each of the combined partial segments must |
|
|
correspond. |
|
Absolute segments are not combined with other absolute segments, they are |
|
|
copied directly to the output file. |
|
|
Locating Segments |
|
|
After the linker/locator combines partial segments it must determine a physical |
|
|
address for them. The linker/locator processes each memory class separately. |
|
|
Refer to “Memory Classes and Memory Layout” on page 27 for a discussion of |
|
|
the different memory class and the physical address ranges. |
|
|
After the linker/locator combines partial segments, it must determine a physical |
|
|
address for them. The linker/locator places different segments in each of these |
|
|
memory areas. The memory is allocated in the following order: |
|
|
1. |
Register Banks and segments with an absolute address. |
|
2. |
Segments specified in Lx51 segment allocation controls. |
|
3. |
Segments with the relocation type BITADDRESSABLE and other BIT |
|
|
segments. |
|
4. |
All other segments with the memory class DATA. |
|
5. |
Segments with the memory class IDATA, EDATA and NCONST. |
|
6. |
Segments with the memory class XDATA. |
|
7. |
Segments with the memory class CODE and the relocation type INBLOCK. |
|
8. |
Other Segments with the memory class CODE and CONST. |
|
9. |
Segments with the memory classes ECODE, HCONST, and HDATA. |