
- •Chapter 1. Introduction
- •How to Develop A Program
- •What is an Assembler?
- •Modular Programming
- •Modular Program Development Process
- •Segments, Modules, and Programs
- •Translate and Link Process
- •Filename Extensions
- •Program Template File
- •Chapter 2. Architecture Overview
- •Memory Classes and Memory Layout
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •CPU Registers
- •CPU Registers of the 8051 Variants
- •CPU Registers of the Intel/Atmel WM 251
- •Program Status Word (PSW)
- •Instruction Sets
- •Opcode Map
- •8051 Instructions
- •Additional 251 Instructions
- •Additional 80C51MX Instructions via Prefix A5
- •Chapter 3. Writing Assembly Programs
- •Assembly Statements
- •Directives
- •Controls
- •Instructions
- •Comments
- •Symbols
- •Symbol Names
- •Labels
- •Operands
- •Special Assembler Symbols
- •Immediate Data
- •Memory Access
- •Program Addresses
- •Expressions and Operators
- •Numbers
- •Characters
- •Character Strings
- •Location Counter
- •Operators
- •Expressions
- •Chapter 4. Assembler Directives
- •Introduction
- •Segment Directives
- •Location Counter
- •Generic Segments
- •Stack Segment
- •Absolute Segments
- •Default Segment
- •SEGMENT
- •RSEG
- •BSEG, CSEG, DSEG, ISEG, XSEG
- •Symbol Definition
- •CODE, DATA, IDATA, XDATA
- •esfr, sfr, sfr16, sbit
- •LIT (AX51 & A251 only)
- •Memory Initialization
- •DD (AX51 & A251 only)
- •Reserving Memory
- •DBIT
- •DSW (AX51 & A251 only)
- •DSD (AX51 & A251 only)
- •Procedure Declaration (AX51 & A251 only)
- •PROC / ENDP (AX51 & A251 only)
- •LABEL (AX51 and A251 only)
- •Program Linkage
- •PUBLIC
- •EXTRN / EXTERN
- •NAME
- •Address Control
- •EVEN (AX51 and A251 only)
- •USING
- •Other Directives
- •_ _ERROR_ _
- •Chapter 5. Assembler Macros
- •Standard Macro Directives
- •Defining a Macro
- •Parameters
- •Labels
- •Repeating Blocks
- •REPT
- •IRPC
- •Nested Definitions
- •Nested Repeating Blocks
- •Recursive Macros
- •Operators
- •NUL Operator
- •& Operator
- •< and > Operators
- •% Operator
- •;; Operator
- •! Operator
- •Invoking a Macro
- •C Macros
- •C Macro Preprocessor Directives
- •Stringize Operator
- •Predefined C Macro Constants
- •Examples with C Macros
- •C Preprocessor Side Effects
- •Chapter 6. Macro Processing Language
- •Overview
- •Creating and Calling MPL Macros
- •Creating Parameterless Macros
- •MPL Macros with Parameters
- •Local Symbols List
- •Macro Processor Language Functions
- •Comment Function
- •Escape Function
- •Bracket Function
- •METACHAR Function
- •Numbers and Expressions
- •Numbers
- •Character Strings
- •SET Function
- •EVAL Function
- •Logical Expressions and String Comparison
- •Conditional MPL Processing
- •IF Function
- •WHILE Function
- •REPEAT Function
- •EXIT Function
- •String Manipulation Functions
- •LEN Function
- •SUBSTR Function
- •MATCH Function
- •Console I/O Functions
- •Advanced Macro Processing
- •Literal Delimiters
- •Blank Delimiters
- •Identifier Delimiters
- •Literal and Normal Mode
- •MACRO Errors
- •Chapter 7. Invocation and Controls
- •Environment Settings
- •Running Ax51
- •ERRORLEVEL
- •Output Files
- •Assembler Controls
- •Controls for Conditional Assembly
- •Conditional Assembly Controls
- •Chapter 8. Error Messages
- •Fatal Errors
- •Non–Fatal Errors
- •Chapter 9. Linker/Locator
- •Overview
- •Combining Program Modules
- •Segment Naming Conventions
- •Combining Segments
- •Locating Segments
- •Overlaying Data Memory
- •Resolving External References
- •Absolute Address Calculation
- •Generating an Absolute Object File
- •Generating a Listing File
- •Bank Switching
- •Using RTX51, RTX251, and RTX51 Tiny
- •Linking Programs
- •Command Line Examples
- •Control Linker Input with µVision2
- •ERRORLEVEL
- •Output File
- •Linker/Locater Controls
- •Locating Programs to Physical Memory
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •Data Overlaying
- •Program and Data Segments of Functions
- •Using the Overlay Control
- •Tips and Tricks for Program Locating
- •Locate Segments with Wildcards
- •Special ROM Handling (LX51 & L251 only)
- •Bank Switching
- •Common Code Area
- •Code Bank Areas
- •Bank Switching Configuration
- •Configuration Examples
- •Control Summary
- •Listing File Controls
- •Output File Controls
- •Segment and Memory Location Controls
- •High-Level Language Controls
- •Error Messages
- •Warnings
- •Non-Fatal Errors
- •Fatal Errors
- •Exceptions
- •Chapter 10. Library Manager
- •Using LIBx51
- •Interactive Mode
- •Create Library within µVision2
- •Command Summary
- •Creating a Library
- •Adding or Replacing Object Modules
- •Removing Object Modules
- •Extracting Object Modules
- •Listing Library Contents
- •Error Messages
- •Fatal Errors
- •Errors
- •Chapter 11. Object-Hex Converter
- •Using OHx51
- •OHx51 Command Line Examples
- •Creating HEX Files for Banked Applications
- •OHx51 Error Messages
- •Using OC51
- •OC51 Error Messages
- •Intel HEX File Format
- •Record Format
- •Data Record
- •Extended 8086 Segment Record
- •Extended Linear Address Record
- •Example Intel HEX File
- •Appendix A. Application Examples
- •ASM – Assembler Example
- •Using A51 and BL51
- •Using AX51 and LX51
- •Using A251 and L251
- •CSAMPLE – C Compiler Example
- •Using C51 and BL51
- •Using C51 and LX51
- •Using C251 and L251
- •BANK_EX1 – Code Banking with C51
- •Using C51 and BL51
- •Using C51 and LX51
- •BANK_EX2 – Banking with Constants
- •Using C51 and BL51
- •Using C51 and LX51
- •Using BL51
- •Using C51 and LX51
- •Philips 80C51MX – Assembler Example
- •Philips 80C51MX – C Compiler Example
- •Appendix B. Reserved Symbols
- •Appendix C. Listing File Format
- •Assembler Listing File Format
- •Listing File Heading
- •Source Listing
- •Macro / Include File / Save Stack Format
- •Symbol Table
- •Listing File Trailer
- •Appendix D. Assembler Differences
- •Differences Between A51 and A251/AX51
- •Differences between A51 and ASM51
- •Differences between A251/AX51 & ASM51
- •Glossary
- •Index
140 |
Chapter 5. Assembler Macros |
|
|
Defining a Macro
Macros must be defined in the program before they can be used. A macro definition begins with the MACRO directive which declares the name of the macro as well as the formal parameters. The macro definition must be terminated with the ENDM directive. The text between the MACRO and ENDM directives is called the macro body.
Example
WAIT |
MACRO |
X |
; macro definition |
|
REPT |
X |
; generate X NOP instructions |
|
NOP |
|
|
|
ENDM |
|
; end REPT |
|
ENDM |
|
; end MACRO |
|
|
In this example, |
WAIT is the name of the macro and X is the only formal |
|||
|
|
parameter. |
|
|
|
|
|
|
In addition to the ENDM directive, the EXITM directive can be used to |
||||
|
|
immediately terminate a macro expansion. When an EXITM directive is |
||||
|
|
detected, the macro processor stops expanding the current macro and resumes |
||||
|
|
processing after the next ENDM directive. The EXITM directive is useful in |
||||
5 |
||||||
|
Exampleconditional statements. |
|
|
|||
|
|
|
|
|
|
|
|
|
WAIT |
MACRO |
X |
; macro definition |
|
|
|
|
IF NUL X |
|
; make sure X has a value |
|
|
|
|
EXITM |
|
; if not then exit |
|
|
|
|
ENDIF |
|
|
|
|
|
|
REPT |
X |
; generate X NOP instructions |
|
|
|
|
NOP |
|
|
|
|
|
|
ENDM |
|
; end REPT |
|
|
|
|
ENDM |
|
; end MACRO |
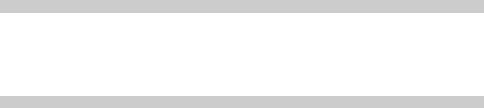
Keil Software — A51/AX51/A251 Macro Assembler and Utilities |
141 |
|
|
Parameters
Up to 16 parameters can be passed to a macro in the invocation line. Formal parameter names must be defined using the MACRO directive.
Example
MNAME MACRO P1,P2,P3,P4,P5,P6,P7,P8,P9,P10,P11,P12,P13,P14,P15,P16
defines a macro with 16 parameters. Parameters must be separated by commas in both the macro definition and invocation. The invocation line for the above macro would appear as follows:
MNAME A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P
where A, B, C, … O, P are parameters that correspond to the format parameter
names P1, P2, P3, … P15, P16.
Null parameters can be passed to a macro. Null parameters have the value NULL and can be tested for using the NUL operator described later in this chapter. If a parameter is omitted from the parameter list in the macro invocation, that parameter is assigned a value of NULL.
Example |
|
|
5 |
|
|
|
|
MNAME A,,C,,E,,G,,I,,K,,M,,O, |
|
|
|
P2, P4, P6, P8, P10, P12, P14, and |
P16 will all be assigned the value NULL |
|
|
|
when the macro is invoked. You should note that there are no spaces between the comma separators in the above invocation line. A space has an ASCII value of 20h and is not equivalent to a NULL.
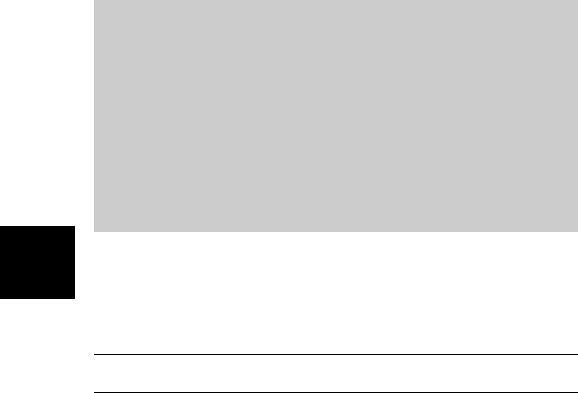
142 |
Chapter 5. Assembler Macros |
|
|
Labels
You can use labels within a macro definition. By default, labels used in a macro are global and if the macro is used more than once in a module, Ax51 will generate an error.
Example
LOC OBJ |
LINE |
SOURCE |
|
|
|
1 |
GLABEL |
MACRO |
|
|
2 |
LOOP: |
NOP |
|
|
3 |
|
JMP |
LOOP |
|
4 |
|
ENDM |
|
|
5 |
|
|
|
|
6 |
|
|
|
|
7 |
|
GLABEL |
|
0000 00 |
8+1 |
LOOP: |
NOP |
|
0001 80FD |
9+1 |
|
JMP |
LOOP |
|
10 |
|
GLABEL |
|
|
11+1 |
LOOP: |
NOP |
|
***_________________________^
***ERROR #9, LINE #11, ATTEMPT TO DEFINE AN ALREADY DEFINED LABEL
|
0003 80FB |
12+1 |
JMP |
LOOP |
|
|
13 |
|
|
|
|
14 |
|
|
|
|
15 |
END |
|
5 |
Labels used in a macro should be local labels. Local labels are visible only |
|||
within the macro and will not generate errors if the macro is used multiple times |
in one source file. You can define a label (or any symbol) used in a macro to be local with the LOCAL directive. Up to 16 local symbols may be defined using the LOCAL directive.
NOTE
LOCAL must be in the next line after the MACRO definition.

Keil Software — A51/AX51/A251 Macro Assembler and Utilities |
143 |
|
|
Example
CLRMEM |
MACRO |
ADDR, LEN |
|
LOCAL |
LOOP |
|
MOV |
R7, #LEN |
|
MOV |
R0, #ADDR |
|
MOV |
A, #0 |
LOOP: |
MOV |
@R0, A |
|
INC |
R0 |
|
DJNZ |
R7, LOOP |
|
ENDM |
|
In this example, the label LOOP |
is local because it is defined with the LOCAL |
directive. Any symbol that is not defined using the LOCAL directive will be a global symbol.
Ax51 generates an internal symbol for local symbols defined in a macro. The internal symbol has the form ??0000 and is incremented each time the macro is invoked. Therefore, local labels used in a macro are unique and will not generate errors.
5