
- •Chapter 1. Introduction
- •How to Develop A Program
- •What is an Assembler?
- •Modular Programming
- •Modular Program Development Process
- •Segments, Modules, and Programs
- •Translate and Link Process
- •Filename Extensions
- •Program Template File
- •Chapter 2. Architecture Overview
- •Memory Classes and Memory Layout
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •CPU Registers
- •CPU Registers of the 8051 Variants
- •CPU Registers of the Intel/Atmel WM 251
- •Program Status Word (PSW)
- •Instruction Sets
- •Opcode Map
- •8051 Instructions
- •Additional 251 Instructions
- •Additional 80C51MX Instructions via Prefix A5
- •Chapter 3. Writing Assembly Programs
- •Assembly Statements
- •Directives
- •Controls
- •Instructions
- •Comments
- •Symbols
- •Symbol Names
- •Labels
- •Operands
- •Special Assembler Symbols
- •Immediate Data
- •Memory Access
- •Program Addresses
- •Expressions and Operators
- •Numbers
- •Characters
- •Character Strings
- •Location Counter
- •Operators
- •Expressions
- •Chapter 4. Assembler Directives
- •Introduction
- •Segment Directives
- •Location Counter
- •Generic Segments
- •Stack Segment
- •Absolute Segments
- •Default Segment
- •SEGMENT
- •RSEG
- •BSEG, CSEG, DSEG, ISEG, XSEG
- •Symbol Definition
- •CODE, DATA, IDATA, XDATA
- •esfr, sfr, sfr16, sbit
- •LIT (AX51 & A251 only)
- •Memory Initialization
- •DD (AX51 & A251 only)
- •Reserving Memory
- •DBIT
- •DSW (AX51 & A251 only)
- •DSD (AX51 & A251 only)
- •Procedure Declaration (AX51 & A251 only)
- •PROC / ENDP (AX51 & A251 only)
- •LABEL (AX51 and A251 only)
- •Program Linkage
- •PUBLIC
- •EXTRN / EXTERN
- •NAME
- •Address Control
- •EVEN (AX51 and A251 only)
- •USING
- •Other Directives
- •_ _ERROR_ _
- •Chapter 5. Assembler Macros
- •Standard Macro Directives
- •Defining a Macro
- •Parameters
- •Labels
- •Repeating Blocks
- •REPT
- •IRPC
- •Nested Definitions
- •Nested Repeating Blocks
- •Recursive Macros
- •Operators
- •NUL Operator
- •& Operator
- •< and > Operators
- •% Operator
- •;; Operator
- •! Operator
- •Invoking a Macro
- •C Macros
- •C Macro Preprocessor Directives
- •Stringize Operator
- •Predefined C Macro Constants
- •Examples with C Macros
- •C Preprocessor Side Effects
- •Chapter 6. Macro Processing Language
- •Overview
- •Creating and Calling MPL Macros
- •Creating Parameterless Macros
- •MPL Macros with Parameters
- •Local Symbols List
- •Macro Processor Language Functions
- •Comment Function
- •Escape Function
- •Bracket Function
- •METACHAR Function
- •Numbers and Expressions
- •Numbers
- •Character Strings
- •SET Function
- •EVAL Function
- •Logical Expressions and String Comparison
- •Conditional MPL Processing
- •IF Function
- •WHILE Function
- •REPEAT Function
- •EXIT Function
- •String Manipulation Functions
- •LEN Function
- •SUBSTR Function
- •MATCH Function
- •Console I/O Functions
- •Advanced Macro Processing
- •Literal Delimiters
- •Blank Delimiters
- •Identifier Delimiters
- •Literal and Normal Mode
- •MACRO Errors
- •Chapter 7. Invocation and Controls
- •Environment Settings
- •Running Ax51
- •ERRORLEVEL
- •Output Files
- •Assembler Controls
- •Controls for Conditional Assembly
- •Conditional Assembly Controls
- •Chapter 8. Error Messages
- •Fatal Errors
- •Non–Fatal Errors
- •Chapter 9. Linker/Locator
- •Overview
- •Combining Program Modules
- •Segment Naming Conventions
- •Combining Segments
- •Locating Segments
- •Overlaying Data Memory
- •Resolving External References
- •Absolute Address Calculation
- •Generating an Absolute Object File
- •Generating a Listing File
- •Bank Switching
- •Using RTX51, RTX251, and RTX51 Tiny
- •Linking Programs
- •Command Line Examples
- •Control Linker Input with µVision2
- •ERRORLEVEL
- •Output File
- •Linker/Locater Controls
- •Locating Programs to Physical Memory
- •Classic 8051
- •Extended 8051 Variants
- •Philips 80C51MX
- •Intel/Atmel WM 251
- •Data Overlaying
- •Program and Data Segments of Functions
- •Using the Overlay Control
- •Tips and Tricks for Program Locating
- •Locate Segments with Wildcards
- •Special ROM Handling (LX51 & L251 only)
- •Bank Switching
- •Common Code Area
- •Code Bank Areas
- •Bank Switching Configuration
- •Configuration Examples
- •Control Summary
- •Listing File Controls
- •Output File Controls
- •Segment and Memory Location Controls
- •High-Level Language Controls
- •Error Messages
- •Warnings
- •Non-Fatal Errors
- •Fatal Errors
- •Exceptions
- •Chapter 10. Library Manager
- •Using LIBx51
- •Interactive Mode
- •Create Library within µVision2
- •Command Summary
- •Creating a Library
- •Adding or Replacing Object Modules
- •Removing Object Modules
- •Extracting Object Modules
- •Listing Library Contents
- •Error Messages
- •Fatal Errors
- •Errors
- •Chapter 11. Object-Hex Converter
- •Using OHx51
- •OHx51 Command Line Examples
- •Creating HEX Files for Banked Applications
- •OHx51 Error Messages
- •Using OC51
- •OC51 Error Messages
- •Intel HEX File Format
- •Record Format
- •Data Record
- •Extended 8086 Segment Record
- •Extended Linear Address Record
- •Example Intel HEX File
- •Appendix A. Application Examples
- •ASM – Assembler Example
- •Using A51 and BL51
- •Using AX51 and LX51
- •Using A251 and L251
- •CSAMPLE – C Compiler Example
- •Using C51 and BL51
- •Using C51 and LX51
- •Using C251 and L251
- •BANK_EX1 – Code Banking with C51
- •Using C51 and BL51
- •Using C51 and LX51
- •BANK_EX2 – Banking with Constants
- •Using C51 and BL51
- •Using C51 and LX51
- •Using BL51
- •Using C51 and LX51
- •Philips 80C51MX – Assembler Example
- •Philips 80C51MX – C Compiler Example
- •Appendix B. Reserved Symbols
- •Appendix C. Listing File Format
- •Assembler Listing File Format
- •Listing File Heading
- •Source Listing
- •Macro / Include File / Save Stack Format
- •Symbol Table
- •Listing File Trailer
- •Appendix D. Assembler Differences
- •Differences Between A51 and A251/AX51
- •Differences between A51 and ASM51
- •Differences between A251/AX51 & ASM51
- •Glossary
- •Index
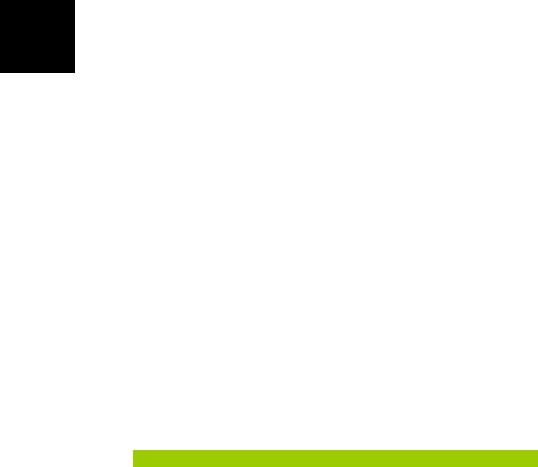
130 |
Chapter 4. Assembler Directives |
|
|
Program Linkage
Program linkage directives allow the separately assembled modules to communicate by permitting inter-module references and the naming of modules.
PUBLIC
The PUBLIC directive lists symbols that may be used in other object modules. The PUBLIC directive makes the specified symbols available in the generated object module. This, in effect, publicizes the names of these symbols. The PUBLIC directive has the following format:
|
PUBLIC symbol |
, symbol … |
4 |
where |
|
symbol |
must be a symbol that was defined somewhere within the |
|
|
source file. Forward references to symbol names are |
permitted. All symbol names, with the exception of register symbols and segment symbols, may be specified with the PUBLIC directive. Multiple symbols must be separated with a comma (,).
If you want to use public symbols in other source files, the EXTRN or EXTERN directive must be used to specify that the symbols are declared in another object module.
Example
PUBLIC |
PUT_CRLF, PUT_STRING, PUT_EOS |
PUBLIC |
ASCBIN, BINASC |
PUBLIC |
GETTOKEN, GETNUMBER |
Shaded directives and options are available only in AX51 and A251.
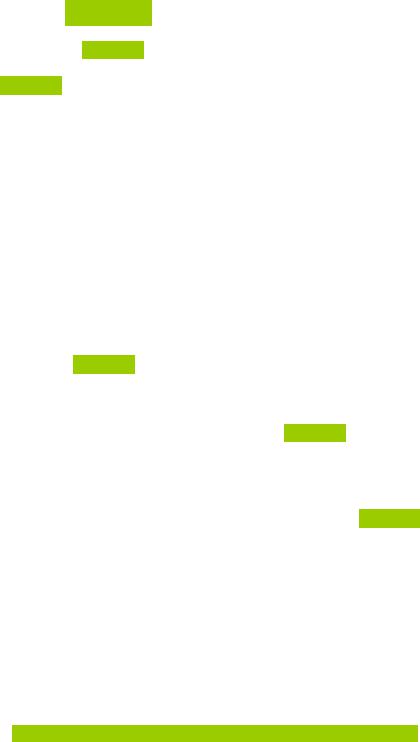
Keil Software — A51/AX51/A251 Macro Assembler and Utilities |
131 |
|
|
EXTRN / EXTERN
The EXTRN and EXTERN directives list symbols (referenced by the source module) that are actually declared in other modules. The format for the EXTRN and EXTERN directives is as follows:
EXTRN |
class |
: type |
|
(symbol |
, |
symbol |
… ) |
|
|
|||||||||||||
EXTERN |
class |
: type |
(symbol |
, |
symbol |
… ) |
|
|
|
|
|
|
|
|
|
|||||||
where |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
class |
|
is the memory class where the symbol has been defined and |
|
|||||||||||||||||||
|
|
may be one of the following: BIT, CODE, |
CONST |
, |
|
|
||||||||||||||||
|
|
DATA, |
EBIT |
, |
ECONST |
, |
EDATA |
, |
ECODE |
|
, |
HDATA |
, |
|
||||||||
|
|
HCONST |
|
, IDATA, XDATA, or NUMBER (to specify a |
|
|||||||||||||||||
|
|
typeless |
symbol). |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
||||||||||||||||||
type |
|
is the symbol type of the external symbol and may be one of |
|
|||||||||||||||||||
|
4 |
|||||||||||||||||||||
|
|
the following: BYTE, WORD, DWORD, NEAR, FAR. |
|
|||||||||||||||||||
symbol |
|
is an external symbol name. |
|
|
|
|
|
|
|
|
|
|
||||||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
The EXTRN or EXTERN directive may appear anywhere in the source program. Multiple symbols may be separated and included in parentheses following the class and type information.
Symbol names that are specified with the EXTRN / EXTERN directive must have been specified as public symbols with the PUBLIC directive in the source file in which they were declared.
The Linker/Locator resolves all external symbols at link time and verifies that the symbol class and symbol types (specified with the EXTRN / EXTERN and PUBLIC directives) match. Symbols with the class NUMBER match every memory class.
Examples
EXTRN |
CODE (PUT_CRLF), DATA (BUFFER) |
|||
EXTERN |
CODE (BINASC, ASCBIN) |
|||
EXTRN |
NUMBER (TABLE_SIZE) |
|||
EXTERN |
CODE:FAR (main) |
|
|
|
EXTRN |
EDATA:BYTE (VALUE, COUNT) |
|
||
EXTRN |
NCONST:DWORD (LIMIT) |
|
Shaded directives and options are available only in AX51 and A251.
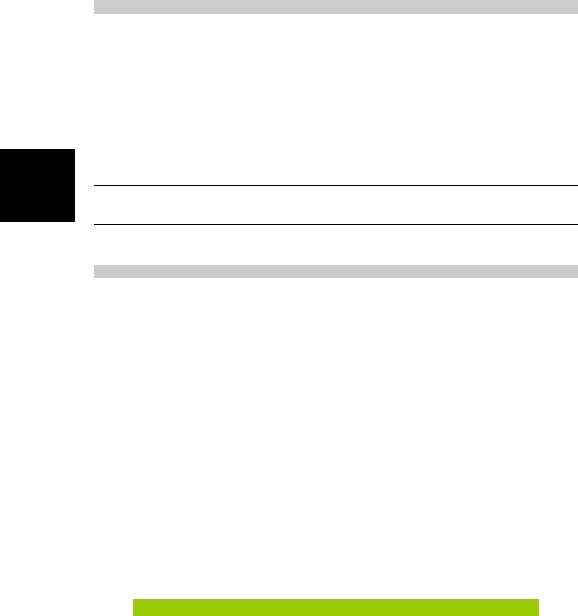
132 |
Chapter 4. Assembler Directives |
|
|
NAME
The NAME directive specifies the name to use for the object module generated for the current program. The filename for the object file is not the object module name. The object module name is embedded within the object file. The format for the NAME directive is as follows:
NAME modulename
where
modulename is the name to use for the object module and can be up to 40 characters long. The modulename must adhere to the rules for symbol names.
If a NAME directive is not present in the source program, the object module name will be the basename of the source file without the extension.
4 NOTE
Only one NAME directive may be specified in a source file.
Example
NAME PARSERMODULE
Shaded directives and options are available only in AX51 and A251.