
- •Preface
- •Introduction
- •SWIG resources
- •About this manual
- •Prerequisites
- •Organization of this manual
- •How to avoid reading the manual
- •Credits
- •What’s new?
- •Bug reports
- •SWIG is free
- •Introduction
- •What is SWIG?
- •Life before SWIG
- •Life after SWIG
- •The SWIG package
- •A SWIG example
- •The swig command
- •Building a Perl5 module
- •Building a Python module
- •Shortcuts
- •Documentation generation
- •Building libraries and modules
- •C syntax, but not a C compiler
- •Non-intrusive interface building
- •Hands off code generation
- •Event driven C programming
- •Automatic documentation generation
- •Summary
- •SWIG for Windows and Macintosh
- •SWIG on Windows 95/NT
- •SWIG on the Power Macintosh
- •Cross platform woes
- •How to survive this manual
- •Scripting Languages
- •The two language view of the world
- •How does a scripting language talk to C?
- •Wrapper functions
- •Variable linking
- •Constants
- •Structures and classes
- •Shadow classes
- •Building scripting language extensions
- •Static linking
- •Shared libraries and dynamic loading
- •Linking with shared libraries
- •SWIG Basics
- •Running SWIG
- •Input format
- •SWIG Output
- •Comments
- •C Preprocessor directives
- •SWIG Directives
- •Simple C functions, variables, and constants
- •Integers
- •Floating Point
- •Character Strings
- •Variables
- •Constants
- •Pointers and complex objects
- •Simple pointers
- •Run time pointer type checking
- •Derived types, structs, and classes
- •Typedef
- •Getting down to business
- •Passing complex datatypes by value
- •Return by value
- •Linking to complex variables
- •Arrays
- •Creating read-only variables
- •Renaming declarations
- •Overriding call by reference
- •Default/optional arguments
- •Pointers to functions
- •Typedef and structures
- •Character strings and structures
- •Array members
- •C constructors and destructors
- •Adding member functions to C structures
- •Nested structures
- •Other things to note about structures
- •C++ support
- •Supported C++ features
- •C++ example
- •Constructors and destructors
- •Member functions
- •Static members
- •Member data
- •Protection
- •Enums and constants
- •References
- •Inheritance
- •Templates
- •Renaming
- •Adding new methods
- •SWIG, C++, and the Legislation of Morality
- •The future of C++ and SWIG
- •Objective-C
- •Objective-C Example
- •Constructors and destructors
- •Instance methods
- •Class methods
- •Member data
- •Protection
- •Inheritance
- •Referring to other classes
- •Categories
- •Implementations and Protocols
- •Renaming
- •Adding new methods
- •Other issues
- •Conditional compilation
- •The #if directive
- •Code Insertion
- •The output of SWIG
- •Code blocks
- •Inlined code blocks
- •Initialization blocks
- •Wrapper code blocks
- •A general interface building strategy
- •Preparing a C program for SWIG
- •What to do with main()
- •Working with the C preprocessor
- •How to cope with C++
- •How to avoid creating the interface from hell
- •Multiple files and the SWIG library
- •The %include directive
- •The %extern directive
- •The %import directive
- •The SWIG library
- •Library example
- •Creating Library Files
- •tclsh.i
- •malloc.i
- •Static initialization of multiple modules
- •More about the SWIG library
- •Documentation System
- •Introduction
- •How it works
- •Choosing a documentation format
- •Function usage and argument names
- •Titles, sections, and subsections
- •Formatting
- •Default Formatting
- •Comment Formatting variables
- •Sorting
- •Comment placement and formatting
- •Tabs and other annoyances
- •Ignoring comments
- •C Information
- •Adding Additional Text
- •Disabling all documentation
- •An Example
- •ASCII Documentation
- •HTML Documentation
- •LaTeX Documentation
- •C++ Support
- •The Final Word?
- •Pointers, Constraints, and Typemaps
- •Introduction
- •The SWIG Pointer Library
- •Pointer Library Functions
- •A simple example
- •Creating arrays
- •Packing a data structure
- •Introduction to typemaps
- •The idea (in a nutshell)
- •Using some typemaps
- •Managing input and output parameters
- •Input Methods
- •Output Methods
- •Input/Output Methods
- •Using different names
- •Applying constraints to input values
- •Simple constraint example
- •Constraint methods
- •Applying constraints to new datatypes
- •Writing new typemaps
- •Motivations for using typemaps
- •Managing special data-types with helper functions
- •A Typemap Implementation
- •What is a typemap?
- •Creating a new typemap
- •Deleting a typemap
- •Copying a typemap
- •Typemap matching rules
- •Common typemap methods
- •Writing typemap code
- •Scope
- •Creating local variables
- •Special variables
- •Typemaps for handling arrays
- •Typemaps and the SWIG Library
- •Implementing constraints with typemaps
- •Typemap examples
- •How to break everything with a typemap
- •Typemaps and the future
- •Exception Handling
- •The %except directive
- •Handling exceptions in C code
- •Exception handling with longjmp()
- •Handling C++ exceptions
- •Using The SWIG exception library
- •Debugging and other interesting uses for %except
- •More Examples
- •SWIG and Perl5
- •Preliminaries
- •Running SWIG
- •Compiling a dynamic module
- •Building a dynamic module with MakeMaker
- •Building a static version of Perl
- •Compilation problems and compiling with C++
- •Building Perl Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •Modules, packages, and classes
- •Basic Perl interface
- •Functions
- •Global variables
- •Constants
- •Pointers
- •Structures and C++ classes
- •A simple Perl example
- •Graphs
- •Sample Perl Script
- •Accessing arrays and other strange objects
- •Implementing methods in Perl
- •Shadow classes
- •Getting serious
- •Wrapping C libraries and other packages
- •Building a Perl5 interface to MATLAB
- •The MATLAB engine interface
- •Wrapping the MATLAB matrix functions
- •Putting it all together
- •Graphical Web-Statistics in Perl5
- •Handling output values (the easy way)
- •Exception handling
- •Remapping datatypes with typemaps
- •A simple typemap example
- •Perl5 typemaps
- •Typemap variables
- •Name based type conversion
- •Converting a Perl5 array to a char **
- •Using typemaps to return values
- •Accessing array structure members
- •Turning Perl references into C pointers
- •Useful functions
- •Standard typemaps
- •Pointer handling
- •Return values
- •The gory details on shadow classes
- •Module and package names
- •What gets created?
- •Object Ownership
- •Nested Objects
- •Shadow Functions
- •Inheritance
- •Iterators
- •Where to go from here?
- •SWIG and Python
- •Preliminaries
- •Running SWIG
- •Compiling a dynamic module
- •Using your module
- •Compilation problems and compiling with C++
- •Building Python Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •The low-level Python/C interface
- •Modules
- •Functions
- •Variable Linking
- •Constants
- •Pointers
- •Structures
- •C++ Classes
- •Python shadow classes
- •A simple example
- •Why write shadow classes in Python?
- •Automated shadow class generation
- •Compiling modules with shadow classes
- •Where to go for more information
- •About the Examples
- •Solving a simple heat-equation
- •The C++ code
- •Making a quick and dirty Python module
- •Using our new module
- •Accessing array data
- •Use Python for control, C for performance
- •Getting even more serious about array access
- •Implementing special Python methods in C
- •Summary (so far)
- •Wrapping a C library
- •Preparing a module
- •Using the gd module
- •Building a simple 2D imaging class
- •A mathematical function plotter
- •Plotting an unstructured mesh
- •From C to SWIG to Python
- •Putting it all together
- •Merging modules
- •Using dynamic loading
- •Use static linking
- •Building large multi-module systems
- •A complete application
- •Exception handling
- •Remapping C datatypes with typemaps
- •What is a typemap?
- •Python typemaps
- •Typemap variables
- •Name based type conversion
- •Converting Python list to a char **
- •Using typemaps to return arguments
- •Mapping Python tuples into small arrays
- •Accessing array structure members
- •Useful Functions
- •Standard typemaps
- •Pointer handling
- •Implementing C callback functions in Python
- •Other odds and ends
- •Adding native Python functions to a SWIG module
- •The gory details of shadow classes
- •A simple shadow class
- •Module names
- •Two classes
- •The this pointer
- •Object ownership
- •Constructors and Destructors
- •Member data
- •Printing
- •Shadow Functions
- •Nested objects
- •Inheritance and shadow classes
- •Methods that return new objects
- •Performance concerns and hints
- •SWIG and Tcl
- •Preliminaries
- •Running SWIG
- •Additional SWIG options
- •Compiling a dynamic module (Unix)
- •Using a dynamic module
- •Static linking
- •Compilation problems
- •Using [incr Tcl] namespaces
- •Building Tcl/Tk Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •Basic Tcl Interface
- •Functions
- •Global variables
- •Constants
- •Pointers
- •Structures
- •C++ Classes
- •The object oriented interface
- •Creating new objects
- •Invoking member functions
- •Deleting objects
- •Accessing member data
- •Changing member data
- •Relationship with pointers
- •About the examples
- •Binary trees in Tcl
- •Making a quick a dirty Tcl module
- •Building a C data structure in Tcl
- •Implementing methods in C
- •Building an object oriented C interface
- •Building C/C++ data structures with Tk
- •Accessing arrays
- •Building a simple OpenGL module
- •Wrapping gl.h
- •Wrapping glu.h
- •Wrapping the aux library
- •A few helper functions
- •An OpenGL package
- •Using the OpenGL module
- •Problems with the OpenGL interface
- •Exception handling
- •Typemaps
- •What is a typemap?
- •Tcl typemaps
- •Typemap variables
- •Name based type conversion
- •Converting a Tcl list to a char **
- •Remapping constants
- •Returning values in arguments
- •Mapping C structures into Tcl Lists
- •Useful functions
- •Standard typemaps
- •Pointer handling
- •Writing a main program and Tcl_AppInit()
- •Creating a new package initialization library
- •Combining Tcl/Tk Extensions
- •Limitations to this approach
- •Dynamic loading
- •Turning a SWIG module into a Tcl Package.
- •Building new kinds of Tcl interfaces (in Tcl)
- •Shadow classes
- •Extending the Tcl Netscape Plugin
- •Using the plugin
- •Tcl8.0 features
- •Advanced Topics
- •Creating multi-module packages
- •Runtime support (and potential problems)
- •Why doesn’t C++ inheritance work between modules?
- •The SWIG runtime library
- •A few dynamic loading gotchas
- •Dynamic Loading of C++ modules
- •Inside the SWIG type-checker
- •Type equivalence
- •Type casting
- •Why a name based approach?
- •Performance of the type-checker
- •Extending SWIG
- •Introduction
- •Prerequisites
- •SWIG Organization
- •The organization of this chapter
- •Compiling a SWIG extension
- •Required C++ compiler
- •Writing a main program
- •Compiling
- •SWIG output
- •The Language class (simple version)
- •A tour of SWIG datatypes
- •The DataType class
- •Function Parameters
- •The String Class
- •Hash Tables
- •The WrapperFunction class
- •Typemaps (from C)
- •The typemap C API.
- •What happens on typemap lookup?
- •How many typemaps are there?
- •File management
- •Naming Services
- •Code Generation Functions
- •Writing a Real Language Module
- •Command Line Options and Basic Initialization
- •Starting the parser
- •Emitting headers and support code
- •Setting a module name
- •Final Initialization
- •Cleanup
- •Creating Commands
- •Creating a Wrapper Function
- •Manipulating Global Variables
- •Constants
- •A Quick Intermission
- •Writing the default typemaps
- •The SWIG library and installation issues
- •C++ Processing
- •How C++ processing works
- •Language extensions
- •Hints
- •Documentation Processing
- •Documentation entries
- •Creating a usage string
- •Writing a new documentation module
- •Using a new documentation module
- •Where to go for more information
- •The Future of SWIG
- •Index
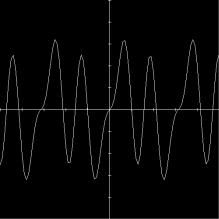
SWIG Users Guide |
SWIG and Python |
185 |
|
|
|
A mathematical function plotter
Here’s a simple class that can be used to plot mathematical functions :
# funcplot.py
from image import *
class PlotFunc(Image):
def __init__(self,func,xmin,ymin,xmax,ymax,width=500,height=500): Image.__init__(self,width,height,xmin,ymin,xmax,ymax)
self.func = func |
# The function being plotted |
self.npoints = 100 |
# Number of samples |
self.color = 1 |
|
def draw(self): |
|
self.clear(0) lastx = self.xmin
lasty = self.func(lastx)
dx = 1.0*(self.xmax-self.xmin)/self.npoints x = lastx+dx
for i in range(0,self.npoints): y = self.func(x)
self.line(lastx,lasty,x,y,self.color) lastx = x
lasty = y x = x + dx
self.axis(1)
Most of the functionality is implemented in our base image class so this is pretty simple. However, if we wanted to make a GIF image of a mathematical function, we could just do this :
>>>from funcplot import *
>>>import math
>>>p = PlotFunc(lambda x: 0.5*math.sin(x)+0.75*math.sin(2*x)-0.6*math.sin(3*x),
-10,-2,10,2)
>>> p.show(“plot.gif”)
Which would produce the following GIF image :
Version 1.1, June 24, 1997
SWIG Users Guide |
SWIG and Python |
186 |
|
|
|
Plotting an unstructured mesh
Of course, perhaps we want to plot something a little more complicated like a mesh. Recently, a colleague came to me with some unstructured mesh data contained in a pair of ASCII formatted files. These files contained a collection of points, and a list of connectivities defining a mesh on these points. Reading and plotting this data in Python turned out to be relatively easy using the following script and our image base class :
#plotmesh.py
#Plots an unstructured mesh stored as an ASCII file from image import *
import string
class PlotMesh(Image):
def __init__(self,filename,xmin,ymin,xmax,ymax,width=500,height=500): Image.__init__(self,width,height,xmin,ymin,xmax,ymax)
#read in a mesh file in pieces pts = []
#Read in data points
atoi = string.atoi atof = string.atof
f = open(filename+".pts","r") npoints = atoi(f.readline()) for i in range(0,npoints):
l = string.split(f.readline()) pts.append((atof(l[0]),atof(l[1])))
f.close()
# Read in mesh data
f = open(filename+".tris","r") ntris = string.atoi(f.readline()) tris = [ ]
for i in range(0,ntris):
l = string.split(f.readline()) tris.append((atoi(l[0])-1,atoi(l[1])-1,atoi(l[2])-1,atoi(l[3])))
f.close()
#Set up local attributes self.pts = pts self.npoints = npoints self.tris = tris self.ntris = ntris
#Draw mesh
def draw(self): self.clear(0); i = 0
while i < self.ntris:
tri = self.tris[i] pt1 = self.pts[tri[0]] pt2 = self.pts[tri[1]] pt3 = self.pts[tri[2]]
# Now draw the mesh self.triangle(pt1[0],pt1[1],pt2[0],pt2[1],pt3[0],pt3[1],tri[3]); i = i + 1
# Draw a triangle
def triangle(self,x1,y1,x2,y2,x3,y3,color):
Version 1.1, June 24, 1997

SWIG Users Guide |
SWIG and Python |
187 |
|
|
|
self.line(x1,y1,x2,y2,color)
self.line(x2,y2,x3,y3,color)
self.line(x3,y3,x1,y1,color)
This class simply reads the data into a few Python lists, has a drawing function for making a plot, and adds a special method for making triangles. Making a plot is now easy, just do this :
>>>from plotmesh.py import *
>>>mesh = PlotMesh("mesh",5,0,35,25)
>>>mesh.show(“mesh.gif”)
This produces the following GIF image :
When run interactively, we can also use simple commands to zoom in and move the image around. For example :
>>> mesh = PlotMesh(“mesh”,5,0,35,25)
>>> mesh.zoom(200) |
# Enlarge by 200% |
>>> mesh.left(50) |
# Move image half a screen to left |
>>> mesh.show() |
|
>>> |
|
While a Python-only implementation would be unsuitable for huge datasets, performance critical operations could be moved to C and used in conjunction with our Image base class.
From C to SWIG to Python
This example illustrates a number of things that are possible with SWIG and Python. First, it is usually relatively easy to build a Python interface to an existing C library. With a little extra work, it is possible to improve the interface by adding a few support functions such as our Point extensions. Finally, once in Python, it is possible to encapsulate C libraries in new kinds of Python objects and classes. We built a simple Image base class and used it to plot mathematical functions and unstructured 2D mesh data---two entirely different tasks, yet easily accomplished with a small amount of Python code. If we later decided to use a different C library such as OpenGL, we could wrap it in SWIG, change the Image base class appropriately , and use the function and mesh plotting examples without modification. I think this is pretty cool.
Version 1.1, June 24, 1997