
- •Preface
- •Introduction
- •SWIG resources
- •About this manual
- •Prerequisites
- •Organization of this manual
- •How to avoid reading the manual
- •Credits
- •What’s new?
- •Bug reports
- •SWIG is free
- •Introduction
- •What is SWIG?
- •Life before SWIG
- •Life after SWIG
- •The SWIG package
- •A SWIG example
- •The swig command
- •Building a Perl5 module
- •Building a Python module
- •Shortcuts
- •Documentation generation
- •Building libraries and modules
- •C syntax, but not a C compiler
- •Non-intrusive interface building
- •Hands off code generation
- •Event driven C programming
- •Automatic documentation generation
- •Summary
- •SWIG for Windows and Macintosh
- •SWIG on Windows 95/NT
- •SWIG on the Power Macintosh
- •Cross platform woes
- •How to survive this manual
- •Scripting Languages
- •The two language view of the world
- •How does a scripting language talk to C?
- •Wrapper functions
- •Variable linking
- •Constants
- •Structures and classes
- •Shadow classes
- •Building scripting language extensions
- •Static linking
- •Shared libraries and dynamic loading
- •Linking with shared libraries
- •SWIG Basics
- •Running SWIG
- •Input format
- •SWIG Output
- •Comments
- •C Preprocessor directives
- •SWIG Directives
- •Simple C functions, variables, and constants
- •Integers
- •Floating Point
- •Character Strings
- •Variables
- •Constants
- •Pointers and complex objects
- •Simple pointers
- •Run time pointer type checking
- •Derived types, structs, and classes
- •Typedef
- •Getting down to business
- •Passing complex datatypes by value
- •Return by value
- •Linking to complex variables
- •Arrays
- •Creating read-only variables
- •Renaming declarations
- •Overriding call by reference
- •Default/optional arguments
- •Pointers to functions
- •Typedef and structures
- •Character strings and structures
- •Array members
- •C constructors and destructors
- •Adding member functions to C structures
- •Nested structures
- •Other things to note about structures
- •C++ support
- •Supported C++ features
- •C++ example
- •Constructors and destructors
- •Member functions
- •Static members
- •Member data
- •Protection
- •Enums and constants
- •References
- •Inheritance
- •Templates
- •Renaming
- •Adding new methods
- •SWIG, C++, and the Legislation of Morality
- •The future of C++ and SWIG
- •Objective-C
- •Objective-C Example
- •Constructors and destructors
- •Instance methods
- •Class methods
- •Member data
- •Protection
- •Inheritance
- •Referring to other classes
- •Categories
- •Implementations and Protocols
- •Renaming
- •Adding new methods
- •Other issues
- •Conditional compilation
- •The #if directive
- •Code Insertion
- •The output of SWIG
- •Code blocks
- •Inlined code blocks
- •Initialization blocks
- •Wrapper code blocks
- •A general interface building strategy
- •Preparing a C program for SWIG
- •What to do with main()
- •Working with the C preprocessor
- •How to cope with C++
- •How to avoid creating the interface from hell
- •Multiple files and the SWIG library
- •The %include directive
- •The %extern directive
- •The %import directive
- •The SWIG library
- •Library example
- •Creating Library Files
- •tclsh.i
- •malloc.i
- •Static initialization of multiple modules
- •More about the SWIG library
- •Documentation System
- •Introduction
- •How it works
- •Choosing a documentation format
- •Function usage and argument names
- •Titles, sections, and subsections
- •Formatting
- •Default Formatting
- •Comment Formatting variables
- •Sorting
- •Comment placement and formatting
- •Tabs and other annoyances
- •Ignoring comments
- •C Information
- •Adding Additional Text
- •Disabling all documentation
- •An Example
- •ASCII Documentation
- •HTML Documentation
- •LaTeX Documentation
- •C++ Support
- •The Final Word?
- •Pointers, Constraints, and Typemaps
- •Introduction
- •The SWIG Pointer Library
- •Pointer Library Functions
- •A simple example
- •Creating arrays
- •Packing a data structure
- •Introduction to typemaps
- •The idea (in a nutshell)
- •Using some typemaps
- •Managing input and output parameters
- •Input Methods
- •Output Methods
- •Input/Output Methods
- •Using different names
- •Applying constraints to input values
- •Simple constraint example
- •Constraint methods
- •Applying constraints to new datatypes
- •Writing new typemaps
- •Motivations for using typemaps
- •Managing special data-types with helper functions
- •A Typemap Implementation
- •What is a typemap?
- •Creating a new typemap
- •Deleting a typemap
- •Copying a typemap
- •Typemap matching rules
- •Common typemap methods
- •Writing typemap code
- •Scope
- •Creating local variables
- •Special variables
- •Typemaps for handling arrays
- •Typemaps and the SWIG Library
- •Implementing constraints with typemaps
- •Typemap examples
- •How to break everything with a typemap
- •Typemaps and the future
- •Exception Handling
- •The %except directive
- •Handling exceptions in C code
- •Exception handling with longjmp()
- •Handling C++ exceptions
- •Using The SWIG exception library
- •Debugging and other interesting uses for %except
- •More Examples
- •SWIG and Perl5
- •Preliminaries
- •Running SWIG
- •Compiling a dynamic module
- •Building a dynamic module with MakeMaker
- •Building a static version of Perl
- •Compilation problems and compiling with C++
- •Building Perl Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •Modules, packages, and classes
- •Basic Perl interface
- •Functions
- •Global variables
- •Constants
- •Pointers
- •Structures and C++ classes
- •A simple Perl example
- •Graphs
- •Sample Perl Script
- •Accessing arrays and other strange objects
- •Implementing methods in Perl
- •Shadow classes
- •Getting serious
- •Wrapping C libraries and other packages
- •Building a Perl5 interface to MATLAB
- •The MATLAB engine interface
- •Wrapping the MATLAB matrix functions
- •Putting it all together
- •Graphical Web-Statistics in Perl5
- •Handling output values (the easy way)
- •Exception handling
- •Remapping datatypes with typemaps
- •A simple typemap example
- •Perl5 typemaps
- •Typemap variables
- •Name based type conversion
- •Converting a Perl5 array to a char **
- •Using typemaps to return values
- •Accessing array structure members
- •Turning Perl references into C pointers
- •Useful functions
- •Standard typemaps
- •Pointer handling
- •Return values
- •The gory details on shadow classes
- •Module and package names
- •What gets created?
- •Object Ownership
- •Nested Objects
- •Shadow Functions
- •Inheritance
- •Iterators
- •Where to go from here?
- •SWIG and Python
- •Preliminaries
- •Running SWIG
- •Compiling a dynamic module
- •Using your module
- •Compilation problems and compiling with C++
- •Building Python Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •The low-level Python/C interface
- •Modules
- •Functions
- •Variable Linking
- •Constants
- •Pointers
- •Structures
- •C++ Classes
- •Python shadow classes
- •A simple example
- •Why write shadow classes in Python?
- •Automated shadow class generation
- •Compiling modules with shadow classes
- •Where to go for more information
- •About the Examples
- •Solving a simple heat-equation
- •The C++ code
- •Making a quick and dirty Python module
- •Using our new module
- •Accessing array data
- •Use Python for control, C for performance
- •Getting even more serious about array access
- •Implementing special Python methods in C
- •Summary (so far)
- •Wrapping a C library
- •Preparing a module
- •Using the gd module
- •Building a simple 2D imaging class
- •A mathematical function plotter
- •Plotting an unstructured mesh
- •From C to SWIG to Python
- •Putting it all together
- •Merging modules
- •Using dynamic loading
- •Use static linking
- •Building large multi-module systems
- •A complete application
- •Exception handling
- •Remapping C datatypes with typemaps
- •What is a typemap?
- •Python typemaps
- •Typemap variables
- •Name based type conversion
- •Converting Python list to a char **
- •Using typemaps to return arguments
- •Mapping Python tuples into small arrays
- •Accessing array structure members
- •Useful Functions
- •Standard typemaps
- •Pointer handling
- •Implementing C callback functions in Python
- •Other odds and ends
- •Adding native Python functions to a SWIG module
- •The gory details of shadow classes
- •A simple shadow class
- •Module names
- •Two classes
- •The this pointer
- •Object ownership
- •Constructors and Destructors
- •Member data
- •Printing
- •Shadow Functions
- •Nested objects
- •Inheritance and shadow classes
- •Methods that return new objects
- •Performance concerns and hints
- •SWIG and Tcl
- •Preliminaries
- •Running SWIG
- •Additional SWIG options
- •Compiling a dynamic module (Unix)
- •Using a dynamic module
- •Static linking
- •Compilation problems
- •Using [incr Tcl] namespaces
- •Building Tcl/Tk Extensions under Windows 95/NT
- •Running SWIG from Developer Studio
- •Using NMAKE
- •Basic Tcl Interface
- •Functions
- •Global variables
- •Constants
- •Pointers
- •Structures
- •C++ Classes
- •The object oriented interface
- •Creating new objects
- •Invoking member functions
- •Deleting objects
- •Accessing member data
- •Changing member data
- •Relationship with pointers
- •About the examples
- •Binary trees in Tcl
- •Making a quick a dirty Tcl module
- •Building a C data structure in Tcl
- •Implementing methods in C
- •Building an object oriented C interface
- •Building C/C++ data structures with Tk
- •Accessing arrays
- •Building a simple OpenGL module
- •Wrapping gl.h
- •Wrapping glu.h
- •Wrapping the aux library
- •A few helper functions
- •An OpenGL package
- •Using the OpenGL module
- •Problems with the OpenGL interface
- •Exception handling
- •Typemaps
- •What is a typemap?
- •Tcl typemaps
- •Typemap variables
- •Name based type conversion
- •Converting a Tcl list to a char **
- •Remapping constants
- •Returning values in arguments
- •Mapping C structures into Tcl Lists
- •Useful functions
- •Standard typemaps
- •Pointer handling
- •Writing a main program and Tcl_AppInit()
- •Creating a new package initialization library
- •Combining Tcl/Tk Extensions
- •Limitations to this approach
- •Dynamic loading
- •Turning a SWIG module into a Tcl Package.
- •Building new kinds of Tcl interfaces (in Tcl)
- •Shadow classes
- •Extending the Tcl Netscape Plugin
- •Using the plugin
- •Tcl8.0 features
- •Advanced Topics
- •Creating multi-module packages
- •Runtime support (and potential problems)
- •Why doesn’t C++ inheritance work between modules?
- •The SWIG runtime library
- •A few dynamic loading gotchas
- •Dynamic Loading of C++ modules
- •Inside the SWIG type-checker
- •Type equivalence
- •Type casting
- •Why a name based approach?
- •Performance of the type-checker
- •Extending SWIG
- •Introduction
- •Prerequisites
- •SWIG Organization
- •The organization of this chapter
- •Compiling a SWIG extension
- •Required C++ compiler
- •Writing a main program
- •Compiling
- •SWIG output
- •The Language class (simple version)
- •A tour of SWIG datatypes
- •The DataType class
- •Function Parameters
- •The String Class
- •Hash Tables
- •The WrapperFunction class
- •Typemaps (from C)
- •The typemap C API.
- •What happens on typemap lookup?
- •How many typemaps are there?
- •File management
- •Naming Services
- •Code Generation Functions
- •Writing a Real Language Module
- •Command Line Options and Basic Initialization
- •Starting the parser
- •Emitting headers and support code
- •Setting a module name
- •Final Initialization
- •Cleanup
- •Creating Commands
- •Creating a Wrapper Function
- •Manipulating Global Variables
- •Constants
- •A Quick Intermission
- •Writing the default typemaps
- •The SWIG library and installation issues
- •C++ Processing
- •How C++ processing works
- •Language extensions
- •Hints
- •Documentation Processing
- •Documentation entries
- •Creating a usage string
- •Writing a new documentation module
- •Using a new documentation module
- •Where to go for more information
- •The Future of SWIG
- •Index
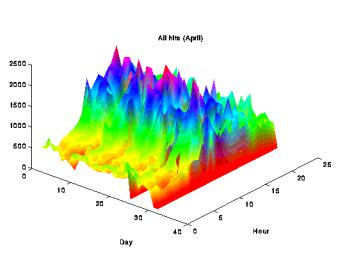
SWIG Users Guide |
SWIG and Perl5 |
142 |
|
|
|
Handling output values (the easy way)
Some C libraries rely on functions that return values in their arguments. For example :
void fadd(double a, double b, double *result) { *result = a+b;
}
By default, SWIG does not handle this case (leaving it up to you to figure out what to do about the result parameter). However, this problem is easily fixed using the typemaps.i library file (see the Typemaps chapter for more information). For example :
// SWIG interface file with output arguments %module example
%include typemaps.i |
// |
Load |
the typemaps librayr |
%apply double *OUTPUT {double *result}; |
// |
Make |
“result” an output parameter |
void fadd(double a, double b, double *result);
When used in a Perl script, the fadd function now takes 2 parameters and returns the result.
$a = fadd(3,4); |
# $a gets the value of double *result |
When multiple output arguments are involved, a Perl array of values will be returned instead. It is also possible to use Perl references as function values. This is done as shown :
// SWIG interface file with output arguments %module
%include typemaps.i
Version 1.1, June 24, 1997
SWIG Users Guide |
SWIG and Perl5 |
143 |
|
|
|
%apply double *REFERENCE {double *result}; |
// Make “result” a Perl reference |
|
void fadd(double a, double b, double *result); |
|
Now in a Perl script, the function works like this :
$c = 0.0; |
# |
Variable for holding the |
result |
fadd(3,4,\$c); |
# |
fadd() places the result |
in $c |
In addition to handling pointers as output values or references, two other methods are available. The INPUT method indicates that a pointer is an input value and the BOTH method indicates that a pointer is both an input and output value. These would be specified as follows :
%apply |
double |
*INPUT {double *in}; |
// |
double |
*in is an input value |
%apply |
double |
*BOTH {double *r}; |
// |
double |
*r is both an input/output value |
This processing of datatypes is built using SWIG’s typemap mechanism. The details of typemaps is described shortly although the typemaps.i library already includes a variety of useful typemaps ready for use.
Exception handling
The SWIG %except directive can be used to create a user-definable exception handler for converting exceptions in your C/C++ program into Perl exceptions. The chapter on exception handling contains more details, but suppose you have a C++ class like the following :
class RangeError {}; // Used for an exception
class DoubleArray { private:
int n; double *ptr;
public:
//Create a new array of fixed size DoubleArray(int size) {
ptr = new double[size]; n = size;
}
//Destroy an array
~DoubleArray() { delete ptr;
}
//Return the length of the array int length() {
return n;
}
//Get an item from the array and perform bounds checking. double getitem(int i) {
if ((i >= 0) && (i < n)) return ptr[i];
else
throw RangeError();
}
Version 1.1, June 24, 1997