
- •Chapter 1. Introduction
- •Support for all 8051 Variants
- •Books About the C Language
- •Chapter 2. Compiling with the Cx51 Compiler
- •Environment Variables
- •Running Cx51 from the Command Prompt
- •ERRORLEVEL
- •Cx51 Output Files
- •Control Directives
- •Directive Categories
- •Reference
- •Chapter 3. Language Extensions
- •Keywords
- •Memory Areas
- •Program Memory
- •Internal Data Memory
- •External Data Memory
- •Far Memory
- •Special Function Register Memory
- •Memory Models
- •Small Model
- •Compact Model
- •Large Model
- •Memory Types
- •Explicitly Declared Memory Types
- •Implicit Memory Types
- •Data Types
- •Bit Types
- •Special Function Registers
- •sbit
- •Absolute Variable Location
- •Pointers
- •Generic Pointers
- •Pointer Conversions
- •Abstract Pointers
- •Function Declarations
- •Function Parameters and the Stack
- •Passing Parameters in Registers
- •Function Return Values
- •Specifying the Memory Model for a Function
- •Specifying the Register Bank for a Function
- •Register Bank Access
- •Interrupt Functions
- •Reentrant Functions
- •Chapter 4. Preprocessor
- •Directives
- •Stringize Operator
- •Predefined Macro Constants
- •Chapter 5. 8051 Derivatives
- •Analog Devices MicroConverter B2 Series
- •Atmel 89x8252 and Variants
- •Dallas 80C320, 420, 520, and 530
- •Arithmetic Accelerator
- •Data Pointers
- •Library Routines
- •Philips 8xC750, 8xC751, and 8xC752
- •Philips 80C51MX Architecture
- •Philips and Atmel WM Dual DPTR
- •Customization Files
- •STARTUP.A51
- •INIT.A51
- •XBANKING.A51
- •Basic I/O Functions
- •Memory Allocation Functions
- •Optimizer
- •General Optimizations
- •Options for Code Generation
- •Segment Naming Conventions
- •Data Objects
- •Program Objects
- •Interfacing C Programs to Assembler
- •Function Parameters
- •Parameter Passing in Registers
- •Parameter Passing in Fixed Memory Locations
- •Function Return Values
- •Using the SRC Directive
- •Register Usage
- •Overlaying Segments
- •Example Routines
- •Small Model Example
- •Compact Model Example
- •Large Model Example
- •Data Storage Formats
- •Bit Variables
- •Signed and Unsigned Long Integers
- •Generic and Far Pointers
- •Floating-point Numbers
- •Accessing Absolute Memory Locations
- •Absolute Memory Access Macros
- •Linker Location Controls
- •The _at_ Keyword
- •Debugging
- •Chapter 7. Error Messages
- •Fatal Errors
- •Actions
- •Errors
- •Syntax and Semantic Errors
- •Warnings
- •Chapter 8. Library Reference
- •Intrinsic Routines
- •Library Files
- •Standard Types
- •va_list
- •Absolute Memory Access Macros
- •CBYTE
- •CWORD
- •DBYTE
- •DWORD
- •FARRAY, FCARRAY
- •FVAR, FCVAR,
- •PBYTE
- •PWORD
- •XBYTE
- •XWORD
- •Routines by Category
- •Buffer Manipulation
- •Character Conversion and Classification
- •Data Conversion
- •Math Routines
- •Memory Allocation Routines
- •Stream Input and Output Routines
- •String Manipulation Routines
- •Miscellaneous Routines
- •Include Files
- •8051 Special Function Register Include Files
- •ABSACC.H
- •ASSERT.H
- •CTYPE.H
- •INTRINS.H
- •MATH.H
- •SETJMP.H
- •STDARG.H
- •STDDEF.H
- •STDIO.H
- •STDLIB.H
- •STRING.H
- •Reference
- •Compiler-related Differences
- •Library-related Differences
- •Appendix B. Version Differences
- •Version 6.0 Differences
- •Version 5 Differences
- •Version 4 Differences
- •Version 3.4 Differences
- •Version 3.2 Differences
- •Version 3.0 Differences
- •Version 2 Differences
- •Appendix C. Writing Optimum Code
- •Memory Model
- •Variable Location
- •Variable Size
- •Unsigned Types
- •Local Variables
- •Other Sources
- •Appendix D. Compiler Limits
- •Appendix E. Byte Ordering
- •Recursive Code Reference Error
- •Problems Using the printf Routines
- •Uncalled Functions
- •Using Monitor-51
- •Trouble with the bdata Memory Type
- •Function Pointers
- •Glossary
- •Index

C51 Compiler |
223 |
|
|
Memory Allocation Routines
Routine |
Attributes |
Description |
calloc |
|
Allocates storage for an array from the memory pool. |
free |
|
Frees a memory block that was allocated using calloc, |
|
|
malloc, or realloc. |
init_mempool |
|
Initializes the memory location and size of the memory |
|
|
pool. |
malloc |
|
Allocates a block from the memory pool. |
realloc |
|
Reallocates a block from the memory pool. |
The memory allocation functions provide you with a means to specify, allocate, and free blocks of memory from a memory pool. All memory allocation functions are implemented as functions and are prototyped in the STDLIB.H include file.
Before using any of these functions to allocate memory, you must first specify, using the init_mempool routine, the location and size of a memory pool from which subsequent memory requests are satisfied.
The calloc and malloc routines allocate blocks of memory from the pool. The calloc routine allocates an array with a specified number of elements of a given size and initializes the array to 0. The malloc routine allocates a specified number of bytes.
The realloc routine changes the size of an allocated block, while the free routine returns a previously allocated memory block to the memory pool.
8

224 Chapter 8. Library Reference
Stream Input and Output Routines
Routine |
Attributes |
Description |
getchar |
reentrant |
Reads and echoes a character using the _getkey and |
|
|
putchar routines. |
_getkey |
|
Reads a character using the 8051 serial interface. |
gets |
|
Reads and echoes a character string using the getchar |
|
|
routine. |
printf / printf517 |
|
Writes formatted data using the putchar routine. |
putchar |
|
Writes a character using the 8051 serial interface. |
puts |
reentrant |
Writes a character string and newline (‘\n’) character |
|
|
using the putchar routine. |
scanf / scanf517 |
|
Reads formatted data using the getchar routine. |
sprintf / sprintf517 |
|
Writes formatted data to a string. |
sscanf / sscanf517 |
|
Reads formatted data from a string. |
ungetchar |
|
Places a character back into the getchar input buffer. |
vprintf |
|
Writes formatted data using the putchar function. |
vsprintf |
|
Writes formatted data to a string. |
The stream input and output routines allow you to read and write data to and from the 8051 serial interface or a user-defined I/O interface. The default _getkey and putchar functions found in the Cx51 library read and write characters using the 8051 serial interface. You can find the source for these functions in the LIB directory. You may modify these source files and substitute them for the library routines. When this is done, other stream functions then perform input and output using the new _getkey and putchar routines.
If you want to use the existing _getkey and putchar functions, you must first initialize the 8051 serial port. If the serial port is not properly initialized, the default stream functions do not function. Initializing the serial port requires manipulating special function registers SFRs of the 8051. The include file REG51.H contains definitions for the required SFRs.
8

C51 Compiler |
225 |
|
|
The following example code must be executed immediately after reset, before any stream functions are invoked.
. |
|
. |
|
. |
|
#include <reg51.h> |
|
. |
|
. |
|
. |
|
SCON = 0x50; |
/* Setup serial port control register */ |
|
/* Mode 1: 8-bit uart var. baud rate */ |
|
/* REN: enable receiver */ |
PCON &= 0x7F; |
/* Clear SMOD bit in power ctrl reg */ |
|
/* This bit doubles the baud rate */ |
TMOD &= 0xCF |
/* Setup timer/counter mode register */ |
|
/* Clear M1 and M0 for timer 1 */ |
TMOD |= 0x20; |
/* Set M1 for 8-bit autoreload timer */ |
TH1 = 0xFD; |
/* Set autoreload value for timer 1 */ |
|
/* 9600 baud with 11.0592 MHz xtal */ |
TR1 = 1; |
/* Start timer 1 */ |
TI = 1; |
/* Set TI to indicate ready to xmit */ |
. |
|
. |
|
. |
|
The stream routines treat input and output as streams of individual characters. There are routines that process characters as well as functions that process strings. Choose the routines that best suit your requirements.
All of these routines are implemented as functions. Most are prototyped in the
STDIO.H include file. The printf517, scanf517, sprintf517, and sscanf517 functions are prototyped in the 80C517.H include file.
8
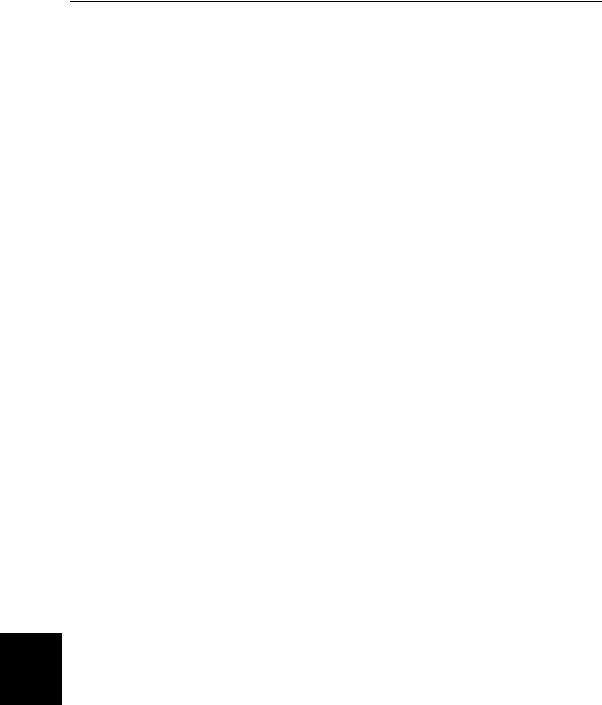
226 Chapter 8. Library Reference
String Manipulation Routines
|
Routine |
Attributes |
Description |
|
|
|
strcat |
|
Concatenates two strings. |
|
|
|
strchr |
reentrant |
Returns a pointer to the first occurrence of a specified |
|
|
|
|
|
|
character in a string. |
|
|
strcmp |
reentrant |
Compares two strings. |
|
|
|
strcpy |
reentrant |
Copies one string to another. |
|
|
|
strcspn |
|
Returns the index of the first character in a string that |
|
|
|
|
|
|
matches any character in a second string. |
|
|
strlen |
reentrant |
Returns the length of a string. |
|
|
|
strncat |
|
Concatenates up to a specified number of characters from |
|
|
|
|
|
|
one string to another. |
|
|
strncmp |
|
Compares two strings up to a specified number of |
|
|
|
|
|
|
characters. |
|
|
strncpy |
|
Copies up to a specified number of characters from one |
|
|
|
|
|
|
string to another. |
|
|
strpbrk |
|
Returns a pointer to the first character in a string that |
|
|
|
|
|
|
matches any character in a second string. |
|
|
strpos |
Reentrant |
Returns the index of the first occurrence of a specified |
|
|
|
|
|
|
character in a string. |
|
|
strrchr |
Reentrant |
Returns a pointer to the last occurrence of a specified |
|
|
|
|
|
|
character in a string. |
|
|
strrpbrk |
|
Returns a pointer to the last character in a string that |
|
|
|
|
|
|
matches any character in a second string. |
|
|
strrpos |
Reentrant |
Returns the index of the last occurrence of a specified |
|
|
|
|
|
|
character in a string. |
|
|
strspn |
|
Returns the index of the first character in a string that |
|
|
|
|
|
|
does not match any character in a second string. |
|
|
strstr |
|
Returns a pointer in a string that is identical to a second |
|
|
|
|
|
|
sub-string. |
|
|
The string routines are implemented as functions and are prototyped in the |
|
|||
|
STRING.H include file. They perform the following operations: |
|
|||
|
|
Copying strings |
|
|
|
|
Appending one string to the end of another |
|
|||
8 |
|
Comparing two strings |
|
|
|
|
Locating one or more characters from a specified set in a string |
|
|||
|
|
All string functions operate on null-terminated character strings. To work on non-terminated strings, use the buffer manipulation routines described earlier in this section.