
- •Chapter 1. Introduction
- •Support for all 8051 Variants
- •Books About the C Language
- •Chapter 2. Compiling with the Cx51 Compiler
- •Environment Variables
- •Running Cx51 from the Command Prompt
- •ERRORLEVEL
- •Cx51 Output Files
- •Control Directives
- •Directive Categories
- •Reference
- •Chapter 3. Language Extensions
- •Keywords
- •Memory Areas
- •Program Memory
- •Internal Data Memory
- •External Data Memory
- •Far Memory
- •Special Function Register Memory
- •Memory Models
- •Small Model
- •Compact Model
- •Large Model
- •Memory Types
- •Explicitly Declared Memory Types
- •Implicit Memory Types
- •Data Types
- •Bit Types
- •Special Function Registers
- •sbit
- •Absolute Variable Location
- •Pointers
- •Generic Pointers
- •Pointer Conversions
- •Abstract Pointers
- •Function Declarations
- •Function Parameters and the Stack
- •Passing Parameters in Registers
- •Function Return Values
- •Specifying the Memory Model for a Function
- •Specifying the Register Bank for a Function
- •Register Bank Access
- •Interrupt Functions
- •Reentrant Functions
- •Chapter 4. Preprocessor
- •Directives
- •Stringize Operator
- •Predefined Macro Constants
- •Chapter 5. 8051 Derivatives
- •Analog Devices MicroConverter B2 Series
- •Atmel 89x8252 and Variants
- •Dallas 80C320, 420, 520, and 530
- •Arithmetic Accelerator
- •Data Pointers
- •Library Routines
- •Philips 8xC750, 8xC751, and 8xC752
- •Philips 80C51MX Architecture
- •Philips and Atmel WM Dual DPTR
- •Customization Files
- •STARTUP.A51
- •INIT.A51
- •XBANKING.A51
- •Basic I/O Functions
- •Memory Allocation Functions
- •Optimizer
- •General Optimizations
- •Options for Code Generation
- •Segment Naming Conventions
- •Data Objects
- •Program Objects
- •Interfacing C Programs to Assembler
- •Function Parameters
- •Parameter Passing in Registers
- •Parameter Passing in Fixed Memory Locations
- •Function Return Values
- •Using the SRC Directive
- •Register Usage
- •Overlaying Segments
- •Example Routines
- •Small Model Example
- •Compact Model Example
- •Large Model Example
- •Data Storage Formats
- •Bit Variables
- •Signed and Unsigned Long Integers
- •Generic and Far Pointers
- •Floating-point Numbers
- •Accessing Absolute Memory Locations
- •Absolute Memory Access Macros
- •Linker Location Controls
- •The _at_ Keyword
- •Debugging
- •Chapter 7. Error Messages
- •Fatal Errors
- •Actions
- •Errors
- •Syntax and Semantic Errors
- •Warnings
- •Chapter 8. Library Reference
- •Intrinsic Routines
- •Library Files
- •Standard Types
- •va_list
- •Absolute Memory Access Macros
- •CBYTE
- •CWORD
- •DBYTE
- •DWORD
- •FARRAY, FCARRAY
- •FVAR, FCVAR,
- •PBYTE
- •PWORD
- •XBYTE
- •XWORD
- •Routines by Category
- •Buffer Manipulation
- •Character Conversion and Classification
- •Data Conversion
- •Math Routines
- •Memory Allocation Routines
- •Stream Input and Output Routines
- •String Manipulation Routines
- •Miscellaneous Routines
- •Include Files
- •8051 Special Function Register Include Files
- •ABSACC.H
- •ASSERT.H
- •CTYPE.H
- •INTRINS.H
- •MATH.H
- •SETJMP.H
- •STDARG.H
- •STDDEF.H
- •STDIO.H
- •STDLIB.H
- •STRING.H
- •Reference
- •Compiler-related Differences
- •Library-related Differences
- •Appendix B. Version Differences
- •Version 6.0 Differences
- •Version 5 Differences
- •Version 4 Differences
- •Version 3.4 Differences
- •Version 3.2 Differences
- •Version 3.0 Differences
- •Version 2 Differences
- •Appendix C. Writing Optimum Code
- •Memory Model
- •Variable Location
- •Variable Size
- •Unsigned Types
- •Local Variables
- •Other Sources
- •Appendix D. Compiler Limits
- •Appendix E. Byte Ordering
- •Recursive Code Reference Error
- •Problems Using the printf Routines
- •Uncalled Functions
- •Using Monitor-51
- •Trouble with the bdata Memory Type
- •Function Pointers
- •Glossary
- •Index
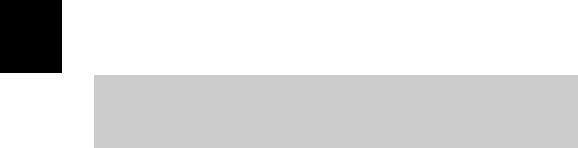
168 |
Chapter 6. Advanced Programming Techniques |
|
|
Register Usage
Assembler functions can change all register contents in the current selected register bank as well as the contents of the registers ACC, B, DPTR, and PSW. When invoking a C function from assembly, assume that these registers may be destroyed by the C function that is called.
Overlaying Segments
If the overlay process is executed during program linking and locating, it is important that each assembler subroutine have a unique program segment. This is necessary so that during the overlay process the references between the functions are calculated using the references of the individual segments. The data areas of the assembler subprograms may be included in the overlay analysis when the following points are observed:
All segment names must be created using the Cx51 compiler segment naming conventions.
Each assembler function with local variables must be assigned its own data segment. This data segment may be accessed by other functions only for passing parameters. Parameters must be passed in order.
Example Routines
6 |
The following program examples show you how to pass parameters to and from |
assembly routines. The following C functions are used in all of these examples: |
|
|
int function ( |
int v_a, |
/* passed in R6 |
& R7 */ |
char v_b, |
/* passed in R5 |
*/ |
bit v_c, |
/* passed in fixed memory location */ |
|
long v_d, |
/* passed in fixed memory location */ |
|
bit v_e); |
/* passed in fixed memory location */ |

Keil Software — Cx51 Compiler User’s Guide |
169 |
|
|
Small Model Example
In the small model, parameters passed in fixed memory locations are stored in internal data memory. The parameter passing segment for variables is located in the data area.
The following are two assembly code examples. The first shows how the example function is called from assembly. The second example displays the assembly code for the example function.
Calling a C function from assembly.
.
.
.
EXTRN CODE |
(_function) |
; Ext declarations for function names |
|
||
EXTRN DATA |
(?_function?BYTE) |
; Seg for local variables |
|
||
EXTRN BIT |
(?_function?BIT) |
; Seg for local bit variables |
|
||
. |
|
|
|
|
|
. |
|
|
|
|
|
. |
|
|
|
|
|
MOV |
R6,#HIGH intval |
|
; int |
a |
|
MOV |
R7,#LOW intval |
|
; int |
a |
|
MOV |
R7,#charconst |
|
; char b |
|
|
SETB |
?_function?BIT+0 |
|
; bit |
c |
|
MOV |
?_function?BYTE+3,longval+0 |
; long d |
|
||
MOV |
?_function?BYTE+4,longval+1 |
; long d |
|
||
MOV |
?_function?BYTE+5,longval+2 |
; long d |
|
||
MOV |
?_function?BYTE+6,longval+3 |
; long d |
|
||
MOV |
C,bitvalue |
|
|
|
|
MOV |
?_function?BIT+1,C |
|
; bit |
e |
|
LCALL |
_function |
|
|
|
|
MOV |
intresult+0,R6 |
|
; store int |
6 |
|
. |
intresult+1,R7 |
|
; retval |
||
MOV |
|
|
.
.

170 Chapter 6. Advanced Programming Techniques
Asssembly code for the example function:
|
|
NAME |
|
MODULE |
|
; Names of the program module |
||
|
|
?PR?FUNCTION?MODULE |
SEGMENT |
CODE |
; Seg for prg code in 'function' |
|||
|
|
?DT?FUNCTION?MODULE |
SEGMENT |
DATA OVERLAYABLE |
||||
|
|
|
|
|
|
|
; Seg for local vars in 'function' |
|
|
|
?BI?FUNCTION?MODULE |
SEGMENT |
BIT |
OVERLAYABLE |
|||
|
|
|
|
|
|
|
; Seg for local bit vars in 'function' |
|
|
|
PUBLIC |
|
_function, ?_function?BYTE, ?_function?BIT |
||||
|
|
|
|
|
|
|
; Public symbols for 'C' function call |
|
|
|
RSEG |
|
?PD?FUNCTION?MODULE |
; Segment for local variables |
|||
|
|
?_function?BYTE: |
|
|
; Start of parameter passing segment |
|||
|
|
v_a: |
DS |
2 |
|
|
; |
int variable: v_a |
|
|
v_b: |
DS |
1 |
|
|
; |
char variable: v_b |
|
|
v_d: |
DS |
4 |
|
|
; |
long variable: v_d |
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
; Additional local variables |
||
|
. |
|
|
|
|
|
|
|
|
|
RSEG |
|
?BI?FUNCTION?MODULE |
; Segment for local bit variables |
|||
|
|
?_function?BIT: |
|
|
; Start of parameter passing segment |
|||
|
|
v_c: |
DBIT |
1 |
|
|
; |
bit variable: v_c |
|
|
v_e: |
DBIT |
1 |
|
|
; |
bit variable: v_e |
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
; Additional local bit variables |
||
|
. |
|
|
|
|
|
|
|
|
|
RSEG |
|
?PR?FUNCTION?MODULE |
; Program segment |
|||
|
|
_function: |
MOV |
v_a,R6 |
|
; A function prolog and epilog is |
||
|
|
|
|
MOV |
v_a+1,R7 |
|
; not necessary. All variables can |
|
|
|
|
|
MOV |
v_b,R5 |
|
; immediately be accessed. |
|
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
|
|
|
|
|
|
|
MOV |
R6,#HIGH retval |
; Return value |
||
6 |
|
|
|
MOV |
R7,#LOW |
retval |
; int constant |
|
|
|
|
RET |
|
|
; Return |
||
|
|
|
|
|
|
|
|
|
Keil Software — Cx51 Compiler User’s Guide |
171 |
|
|
Compact Model Example
In the compact model, parameters passed in fixed memory locations are stored in external data memory. The parameter passing segment for variables is located in the pdata area.
The following are two assembly code examples. The first shows you how the example function is called from assembly. The second example displays the assembly code for the example function.
Calling a C function from assembly.
EXTRN CODE |
(_function) |
; Ext declarations for function names |
|
|
||
EXTRN XDATA |
(?_function?BYTE) |
; Seg for local variables |
|
|
||
EXTRN BIT |
(?_function?BIT) |
; Seg for local bit variables |
|
|
||
. |
|
|
|
|
|
|
. |
|
|
|
|
|
|
. |
|
|
|
|
|
|
MOV |
R6,#HIGH intval |
|
; int |
a |
|
|
MOV |
R7,#LOW intval |
|
; int |
a |
|
|
MOV |
R5,#charconst |
|
; char b |
|
|
|
SETB |
?_function?BIT+0 |
|
; bit |
c |
|
|
MOV |
R0,#?_function?BYTE+3 |
; Addr of 'v_d' in the passing area |
|
|
||
MOV |
A,longval+0 |
|
; long d |
|
|
|
MOVX |
@R0,A |
|
; Store parameter byte |
|
|
|
INC |
R0 |
|
; Inc parameter passing address |
|
|
|
MOV |
A,longval+1 |
|
; long d |
|
|
|
MOVX |
@R0,A |
|
; Store parameter byte |
|
|
|
INC |
R0 |
|
; Inc parameter passing address |
|
|
|
MOV |
A,longval+2 |
|
; long d |
|
|
|
MOVX |
@R0,A |
|
; Store parameter byte |
|
|
|
INC |
R0 |
|
; Inc parameter passing address |
|
|
|
MOV |
A,longval+3 |
|
; long d |
|
6 |
|
MOVX |
@R0,A |
|
; Store parameter byte |
|
||
MOV |
C,bitvalue |
|
|
|
|
|
MOV |
?_function?BIT+1,C |
|
; bit |
e |
|
|
LCALL |
_function |
|
|
|
|
|
MOV |
intresult+0,R6 |
|
; Store int |
|
|
|
MOV |
intresult+1,R7 |
|
; Retval |
|
|
|
. |
|
|
|
|
|
|
. |
|
|
|
|
|
|
. |
|
|
|
|
|
|

172 Chapter 6. Advanced Programming Techniques
Asssembly code for the example function:
|
|
NAME |
|
MODULE |
|
|
; Name of the program module |
|
|
|
?PR?FUNCTION?MODULE |
SEGMENT |
CODE |
; Seg for program code in 'function'; |
|||
|
|
?PD?FUNCTION?MODULE |
SEGMENT |
XDATA OVERLAYABLE IPAGE |
||||
|
|
|
|
|
|
|
; Seg for local vars in 'function' |
|
|
|
?BI?FUNCTION?MODULE |
SEGMENT |
BIT |
OVERLAYABLE |
|||
|
|
|
|
|
|
|
; Seg for local bit vars in |
|
|
|
'function' |
|
|
|
|
|
|
|
|
PUBLIC |
|
_function, ?_function?BYTE, ?_function?BIT |
||||
|
|
|
|
|
|
|
; Public symbols for C function call |
|
|
|
RSEG |
|
?PD?FUNCTION?MODULE |
; Segment for local variables |
|||
|
|
?_function?BYTE: |
|
|
; Start of the parameter passing seg |
|||
|
|
v_a: |
DS |
2 |
|
|
; |
int variable: v_a |
|
|
v_b: |
DS |
1 |
|
|
; |
char variable: v_b |
|
|
v_d: |
DS |
4 |
|
|
; |
long variable: v_d |
|
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
; Additional local variables |
|
|
|
. |
|
|
|
|
|
|
|
|
RSEG |
|
?BI?FUNCTION?MODULE |
; Segment for local bit variables |
|||
|
|
?_function?BIT: |
|
|
; Start of the parameter passing seg |
|||
|
|
v_c: |
DBIT |
1 |
|
|
; |
bit variable: v_c |
|
|
v_e: |
DBIT |
1 |
|
|
; |
bit variable: v_e |
|
|
. |
|
|
|
|
|
|
|
|
. |
|
|
|
|
; Additional local bit variables |
|
|
|
. |
|
|
|
|
|
|
|
|
RSEG |
|
?PR?FUNCTION?MODULE |
|
; Program segment |
||
|
|
_function: |
MOV |
R0,#?_function?BYTE+0 |
; Special function prolog |
|||
|
|
|
|
MOV |
A,R6 |
|
|
; and epilog is not |
|
|
|
|
MOVX |
@R0,A |
|
|
; necessary. All |
|
|
|
|
INC |
R0 |
|
|
; vars can immediately |
|
|
|
|
MOV |
A,R7 |
|
|
; be accessed |
|
|
|
|
MOVX |
@R0,A |
|
|
|
6 |
|
. |
|
INC |
R0 |
|
|
|
|
|
MOV |
A,R5 |
|
|
|
||
|
|
|
|
|
|
|
||
|
|
|
|
MOVX |
@R0,A |
|
|
|
|
|
. |
|
|
|
|
|
|
|
|
. |
|
MOV |
R6,#HIGH retval |
|
; Return value |
|
|
|
|
||||||
|
|
|
|
|
||||
|
|
|
|
MOV |
R7,#LOW |
retval |
|
; int constant |
|
|
|
|
RET |
|
|
|
; Return |