
- •Chapter 1. Introduction
- •Support for all 8051 Variants
- •Books About the C Language
- •Chapter 2. Compiling with the Cx51 Compiler
- •Environment Variables
- •Running Cx51 from the Command Prompt
- •ERRORLEVEL
- •Cx51 Output Files
- •Control Directives
- •Directive Categories
- •Reference
- •Chapter 3. Language Extensions
- •Keywords
- •Memory Areas
- •Program Memory
- •Internal Data Memory
- •External Data Memory
- •Far Memory
- •Special Function Register Memory
- •Memory Models
- •Small Model
- •Compact Model
- •Large Model
- •Memory Types
- •Explicitly Declared Memory Types
- •Implicit Memory Types
- •Data Types
- •Bit Types
- •Special Function Registers
- •sbit
- •Absolute Variable Location
- •Pointers
- •Generic Pointers
- •Pointer Conversions
- •Abstract Pointers
- •Function Declarations
- •Function Parameters and the Stack
- •Passing Parameters in Registers
- •Function Return Values
- •Specifying the Memory Model for a Function
- •Specifying the Register Bank for a Function
- •Register Bank Access
- •Interrupt Functions
- •Reentrant Functions
- •Chapter 4. Preprocessor
- •Directives
- •Stringize Operator
- •Predefined Macro Constants
- •Chapter 5. 8051 Derivatives
- •Analog Devices MicroConverter B2 Series
- •Atmel 89x8252 and Variants
- •Dallas 80C320, 420, 520, and 530
- •Arithmetic Accelerator
- •Data Pointers
- •Library Routines
- •Philips 8xC750, 8xC751, and 8xC752
- •Philips 80C51MX Architecture
- •Philips and Atmel WM Dual DPTR
- •Customization Files
- •STARTUP.A51
- •INIT.A51
- •XBANKING.A51
- •Basic I/O Functions
- •Memory Allocation Functions
- •Optimizer
- •General Optimizations
- •Options for Code Generation
- •Segment Naming Conventions
- •Data Objects
- •Program Objects
- •Interfacing C Programs to Assembler
- •Function Parameters
- •Parameter Passing in Registers
- •Parameter Passing in Fixed Memory Locations
- •Function Return Values
- •Using the SRC Directive
- •Register Usage
- •Overlaying Segments
- •Example Routines
- •Small Model Example
- •Compact Model Example
- •Large Model Example
- •Data Storage Formats
- •Bit Variables
- •Signed and Unsigned Long Integers
- •Generic and Far Pointers
- •Floating-point Numbers
- •Accessing Absolute Memory Locations
- •Absolute Memory Access Macros
- •Linker Location Controls
- •The _at_ Keyword
- •Debugging
- •Chapter 7. Error Messages
- •Fatal Errors
- •Actions
- •Errors
- •Syntax and Semantic Errors
- •Warnings
- •Chapter 8. Library Reference
- •Intrinsic Routines
- •Library Files
- •Standard Types
- •va_list
- •Absolute Memory Access Macros
- •CBYTE
- •CWORD
- •DBYTE
- •DWORD
- •FARRAY, FCARRAY
- •FVAR, FCVAR,
- •PBYTE
- •PWORD
- •XBYTE
- •XWORD
- •Routines by Category
- •Buffer Manipulation
- •Character Conversion and Classification
- •Data Conversion
- •Math Routines
- •Memory Allocation Routines
- •Stream Input and Output Routines
- •String Manipulation Routines
- •Miscellaneous Routines
- •Include Files
- •8051 Special Function Register Include Files
- •ABSACC.H
- •ASSERT.H
- •CTYPE.H
- •INTRINS.H
- •MATH.H
- •SETJMP.H
- •STDARG.H
- •STDDEF.H
- •STDIO.H
- •STDLIB.H
- •STRING.H
- •Reference
- •Compiler-related Differences
- •Library-related Differences
- •Appendix B. Version Differences
- •Version 6.0 Differences
- •Version 5 Differences
- •Version 4 Differences
- •Version 3.4 Differences
- •Version 3.2 Differences
- •Version 3.0 Differences
- •Version 2 Differences
- •Appendix C. Writing Optimum Code
- •Memory Model
- •Variable Location
- •Variable Size
- •Unsigned Types
- •Local Variables
- •Other Sources
- •Appendix D. Compiler Limits
- •Appendix E. Byte Ordering
- •Recursive Code Reference Error
- •Problems Using the printf Routines
- •Uncalled Functions
- •Using Monitor-51
- •Trouble with the bdata Memory Type
- •Function Pointers
- •Glossary
- •Index
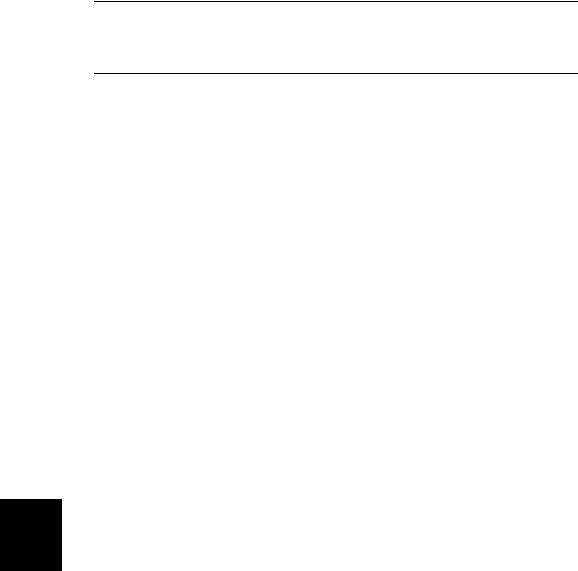
218 |
Chapter 8. Library Reference |
|
|
Routines by Category
This sections gives a brief overview of the major categories of routines available in the Cx51 standard library. Refer to “Reference” on page 232 for a complete description of routine syntax and usage.
NOTE
Many of the routines in the Cx51 standard library are reentrant, intrinsic, or both. These specifications are listed under attributes in the following tables. Unless otherwise noted, routines are non-reentrant and non-intrinsic.
Buffer Manipulation
Routine |
Attributes |
Description |
memccpy |
|
Copies data bytes from one buffer to another until a |
|
|
specified character or specified number of characters has |
|
|
been copied. |
memchr |
reentrant |
Returns a pointer to the first occurrence of a specified |
|
|
character in a buffer. |
memcmp |
reentrant |
Compares a given number of characters from two different |
|
|
buffers. |
memcpy |
reentrant |
Copies a specified number of data bytes from one buffer |
|
|
to another. |
memmove |
reentrant |
Copies a specified number of data bytes from one buffer |
|
|
to another. |
memset |
reentrant |
Initializes a specified number of data bytes in a buffer to a |
|
|
specified character value. |
The buffer manipulation routines are used to work on memory buffers on a character-by-character basis. A buffer is an array of characters like a string, however, a buffer is usually not terminated with a null character (‘\0’). For this reason, these routines require a buffer length or count argument.
All of these routines are implemented as functions. Function prototypes are included in the STRING.H include file.
8

C51 Compiler |
219 |
|
|
Character Conversion and Classification
Routine |
Attributes |
Description |
isalnum |
reentrant |
Tests for an alphanumeric character. |
isalpha |
reentrant |
Tests for an alphabetic character. |
iscntrl |
reentrant |
Tests for a Control character. |
isdigit |
reentrant |
Tests for a decimal digit. |
isgraph |
reentrant |
Tests for a printable character with the exception of space. |
islower |
reentrant |
Tests for a lowercase alphabetic character. |
isprint |
reentrant |
Tests for a printable character. |
ispunct |
reentrant |
Tests for a punctuation character. |
isspace |
reentrant |
Tests for a whitespace character. |
isupper |
reentrant |
Tests for an uppercase alphabetic character. |
isxdigit |
reentrant |
Tests for a hexadecimal digit. |
toascii |
reentrant |
Converts a character to an ASCII code. |
toint |
reentrant |
Converts a hexadecimal digit to a decimal value. |
tolower |
reentrant |
Tests a character and converts it to lowercase if it is |
|
|
uppercase. |
_tolower |
reentrant |
Unconditionally converts a character to lowercase. |
toupper |
reentrant |
Tests a character and converts it to uppercase if it is |
|
|
lowercase. |
_toupper |
reentrant |
Unconditionally converts a character to uppercase. |
The character conversion and classification routines allow you to test individual characters for a variety of attributes and convert characters to different formats.
The _tolower, _toupper, and toascii routines are implemented as macros. All other routines are implemented as functions. All macro definitions and function prototypes are found in the CTYPE.H include file.
8
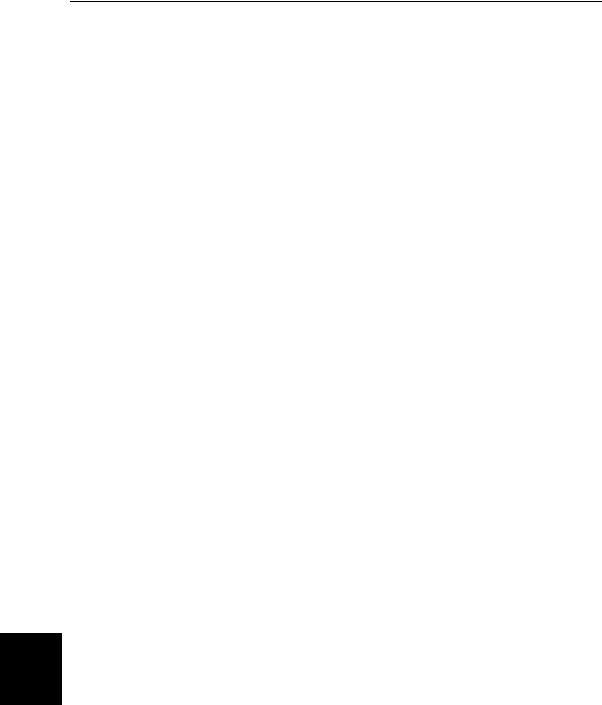
220 Chapter 8. Library Reference
Data Conversion
Routine |
Attributes |
Description |
abs |
reentrant |
Generates the absolute value of an integer type. |
atof / atof517 |
|
Converts a string to a float. |
atoi |
|
Converts a string to an int. |
atol |
|
Converts a string to a long. |
cabs |
reentrant |
Generates the absolute value of a character type. |
labs |
reentrant |
Generates the absolute value of a long type. |
strtod / |
|
Converts a string to a float. |
strtod517 |
|
|
strtol |
|
Converts a string to a long. |
strtoul |
|
Converts a string to an unsigned long. |
The data conversion routines convert strings of ASCII characters to numbers. All of these routines are implemented as functions and most are prototyped in the include file STDLIB.H. The abs, cabs, and labs functions are prototyped in the MATH.H include file. The atof517, and strtod517 function are prototyped in the include file 80C517.H.
8
C51 Compiler |
221 |
|
|
Math Routines
Routine |
Attributes |
Description |
|
|
|
acos / acos517 |
|
Calculates the arc cosine of a specified number. |
|
|
|
asin / asin517 |
|
Calculates the arc sine of a specified number. |
|
|
|
atan / atan517 |
|
Calculates the arc tangent of a specified number. |
|
|
|
atan2 |
|
Calculates the arc tangent of a fraction. |
|
|
|
ceil |
|
Finds the integer ceiling of a specified number. |
|
|
|
cos / cos517 |
|
Calculates the cosine of a specified number. |
|
|
|
cosh |
|
Calculates the hyperbolic cosine of a specified number. |
|
|
|
exp / exp517 |
|
Calculates the exponential function of a specified number. |
|
|
|
fabs |
reentrant |
Finds the absolute value of a specified number. |
|
|
|
floor |
|
Finds the largest integer less than or equal to a specified |
|
|
|
|
|
number. |
|
|
|
fmod |
|
Calculates the floating-point remainder. |
|
|
|
log / log517 |
|
Calculates the natural logarithm of a specified number. |
|
|
|
log10 / log10517 |
|
Calculates the common logarithm of a specified number. |
|
|
|
modf |
|
Generates integer and fractional components of a |
|
|
|
|
|
specified number. |
|
|
|
pow |
|
Calculates a value raised to a power. |
|
|
|
rand |
reentrant |
Generates a pseudo random number. |
|
|
|
sin / sin517 |
|
Calculates the sine of a specified number. |
|
|
|
sinh |
|
Calculates the hyperbolic sine of a specified number. |
|
|
|
srand |
|
Initializes the pseudo random number generator. |
|
|
|
sqrt / sqrt517 |
|
Calculates the square root of a specified number. |
|
|
|
tan / tan517 |
|
Calculates the tangent of a specified number. |
|
|
|
tanh |
|
Calculates the hyperbolic tangent of a specified number. |
|
|
|
_chkfloat_ |
intrinsic, |
Checks the status of float numbers. |
|
|
|
|
reentrant |
|
|
|
|
_crol_ |
intrinsic, |
Rotates an unsigned char left a specified number of bits. |
|
|
|
|
reentrant |
|
|
|
|
_cror_ |
intrinsic, |
Rotates an unsigned char right a specified number of bits. |
|
|
|
|
reentrant |
|
|
|
|
_irol_ |
intrinsic, |
Rotates an unsigned int left a specified number of bits. |
|
|
|
|
reentrant |
|
|
|
|
_iror_ |
intrinsic, |
Rotates an unsigned int right a specified number of bits. |
|
|
|
8 |
|||||
|
reentrant |
|
|
||
_lrol_ |
intrinsic, |
Rotates an unsigned long left a specified number of bits. |
|
||
|
reentrant |
|
|
||
_lror_ |
intrinsic, |
Rotates an unsigned long right a specified number of bits. |
|
|
|
|
reentrant |
|
|
|

222 |
Chapter 8. Library Reference |
|
|
The math routines perform common mathematical calculations. Most of these routines work with floating-point values and therefore include the floating-point libraries and support routines.
All of these routines are implemented as functions. Most are prototyped in the include file MATH.H. Functions which end in 517 (acos517, asin517, atan517, cos517, exp517, log517, log10517, sin517, sqrt517, and tan517) are prototyped in the 80C517.H include file. The rand and srand functions are prototyped in the STDLIB.H include file.
The _chkfloat_, _crol_, _cror_, _irol_, _iror_, _lrol_, and _lror_ functions are prototyped in the INTRINS.H include file.
8