
- •Chapter 1. Introduction
- •Support for all 8051 Variants
- •Books About the C Language
- •Chapter 2. Compiling with the Cx51 Compiler
- •Environment Variables
- •Running Cx51 from the Command Prompt
- •ERRORLEVEL
- •Cx51 Output Files
- •Control Directives
- •Directive Categories
- •Reference
- •Chapter 3. Language Extensions
- •Keywords
- •Memory Areas
- •Program Memory
- •Internal Data Memory
- •External Data Memory
- •Far Memory
- •Special Function Register Memory
- •Memory Models
- •Small Model
- •Compact Model
- •Large Model
- •Memory Types
- •Explicitly Declared Memory Types
- •Implicit Memory Types
- •Data Types
- •Bit Types
- •Special Function Registers
- •sbit
- •Absolute Variable Location
- •Pointers
- •Generic Pointers
- •Pointer Conversions
- •Abstract Pointers
- •Function Declarations
- •Function Parameters and the Stack
- •Passing Parameters in Registers
- •Function Return Values
- •Specifying the Memory Model for a Function
- •Specifying the Register Bank for a Function
- •Register Bank Access
- •Interrupt Functions
- •Reentrant Functions
- •Chapter 4. Preprocessor
- •Directives
- •Stringize Operator
- •Predefined Macro Constants
- •Chapter 5. 8051 Derivatives
- •Analog Devices MicroConverter B2 Series
- •Atmel 89x8252 and Variants
- •Dallas 80C320, 420, 520, and 530
- •Arithmetic Accelerator
- •Data Pointers
- •Library Routines
- •Philips 8xC750, 8xC751, and 8xC752
- •Philips 80C51MX Architecture
- •Philips and Atmel WM Dual DPTR
- •Customization Files
- •STARTUP.A51
- •INIT.A51
- •XBANKING.A51
- •Basic I/O Functions
- •Memory Allocation Functions
- •Optimizer
- •General Optimizations
- •Options for Code Generation
- •Segment Naming Conventions
- •Data Objects
- •Program Objects
- •Interfacing C Programs to Assembler
- •Function Parameters
- •Parameter Passing in Registers
- •Parameter Passing in Fixed Memory Locations
- •Function Return Values
- •Using the SRC Directive
- •Register Usage
- •Overlaying Segments
- •Example Routines
- •Small Model Example
- •Compact Model Example
- •Large Model Example
- •Data Storage Formats
- •Bit Variables
- •Signed and Unsigned Long Integers
- •Generic and Far Pointers
- •Floating-point Numbers
- •Accessing Absolute Memory Locations
- •Absolute Memory Access Macros
- •Linker Location Controls
- •The _at_ Keyword
- •Debugging
- •Chapter 7. Error Messages
- •Fatal Errors
- •Actions
- •Errors
- •Syntax and Semantic Errors
- •Warnings
- •Chapter 8. Library Reference
- •Intrinsic Routines
- •Library Files
- •Standard Types
- •va_list
- •Absolute Memory Access Macros
- •CBYTE
- •CWORD
- •DBYTE
- •DWORD
- •FARRAY, FCARRAY
- •FVAR, FCVAR,
- •PBYTE
- •PWORD
- •XBYTE
- •XWORD
- •Routines by Category
- •Buffer Manipulation
- •Character Conversion and Classification
- •Data Conversion
- •Math Routines
- •Memory Allocation Routines
- •Stream Input and Output Routines
- •String Manipulation Routines
- •Miscellaneous Routines
- •Include Files
- •8051 Special Function Register Include Files
- •ABSACC.H
- •ASSERT.H
- •CTYPE.H
- •INTRINS.H
- •MATH.H
- •SETJMP.H
- •STDARG.H
- •STDDEF.H
- •STDIO.H
- •STDLIB.H
- •STRING.H
- •Reference
- •Compiler-related Differences
- •Library-related Differences
- •Appendix B. Version Differences
- •Version 6.0 Differences
- •Version 5 Differences
- •Version 4 Differences
- •Version 3.4 Differences
- •Version 3.2 Differences
- •Version 3.0 Differences
- •Version 2 Differences
- •Appendix C. Writing Optimum Code
- •Memory Model
- •Variable Location
- •Variable Size
- •Unsigned Types
- •Local Variables
- •Other Sources
- •Appendix D. Compiler Limits
- •Appendix E. Byte Ordering
- •Recursive Code Reference Error
- •Problems Using the printf Routines
- •Uncalled Functions
- •Using Monitor-51
- •Trouble with the bdata Memory Type
- •Function Pointers
- •Glossary
- •Index
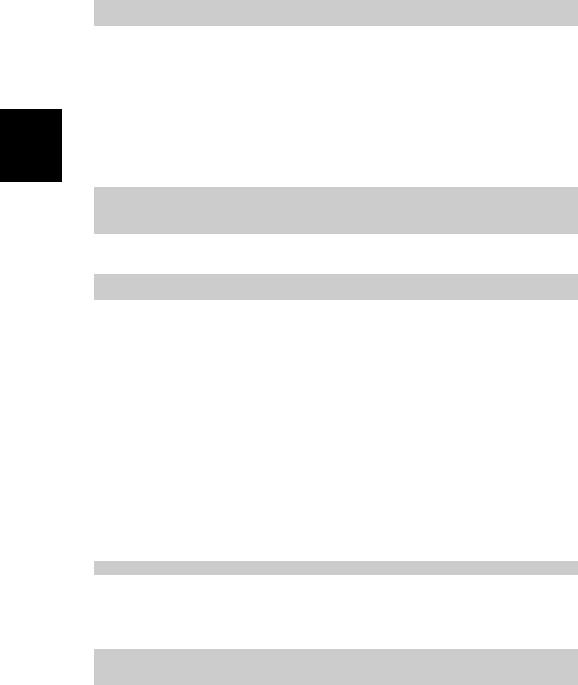
18 |
Chapter 2. Compiling with the Cx51 Compiler |
|
|
SET C51INC=C:\KEIL\C51\INC
SET C51LIB=C:\KEIL\C51\LIB
Running Cx51 from the Command Prompt
To invoke the C51 or CX51 compiler, enter C51 or CX51 at the command prompt. On this command line, you must include the name of the C source file
2 to be compiled, as well as any other necessary control directives required to compile your source file. The format for the Cx51 command line is:
C51 sourcefile directives…
CX51 sourcefile directives…
or:
C51 @commandfile
CX51 @commandfile
where:
sourcefile is the name of the source program you want to compile.
directives are the directives you want to use to control the function of the compiler. Refer to “Control Directives” on page 20 for a detailed list of the available directives.
commandfile is the name of a command input file that may contain sourcefile and directives. A commandfile is used, when the Cx51 invocation line gets complex and exceeds the limits of the Windows command prompt.
The following command line example invokes C51, specifies the source file SAMPLE.C, and uses the controls DEBUG, CODE, and PREPRINT.
C51 SAMPLE.C DEBUG CODE PREPRINT
The Cx51 compiler displays the following information upon successful compilation.
C51 COMPILER V6.10
C51 COMPILATION COMPLETE. 0 WARNING(S), 0 ERROR(S)
Keil Software — Cx51 Compiler User’s Guide |
19 |
|
|
ERRORLEVEL
After compilation, the number of errors and warnings detected is output to the screen. The Cx51 compiler then sets the ERRORLEVEL to indicate the status of the compilation. Values are listed in the following table:
|
ERRORLEVEL |
Meaning |
|
2 |
|
0 |
No errors or warnings |
|
|
|
1 |
Warnings only |
|
|
|
2 |
Errors and possibly warnings |
|
|
|
|
|||
|
3 |
Fatal errors |
|
|
You can access the ERRORLEVEL variable in batch files. Refer to the Windows command index or to batch commands in the Windows on-line help for more information on ERRORLEVEL or batch files.
Cx51 Output Files
The Cx51 compiler generates a number of output files during compilation. By default, each of these output files shares the same filename as the source file. However, each has a different file extension. The following table lists the files and gives a brief description of each.
File Extension |
Description |
filename.LST |
Files with this extension are listing files that contain the formatted source |
|
text along with any errors detected by the compiler. Listing files may |
|
optionally contain the symbols used and the assembly code generated. |
|
For more information, refer to the PRINT directive in the following sections. |
filename.OBJ |
Files with this extension are object modules that contain relocatable object |
|
code. Object modules may be linked to an absolute object module by the |
|
Lx51 Linker/Locator. |
filename.I |
Files with this extension contain the source text as expanded by the |
|
preprocessor. All macros are expanded and all comments are deleted in |
|
this listing. For more information, refer to the PREPRINT directive in the |
|
following sections. |
filename.SRC |
Files with this extension are assembly source files generated from your C |
|
source code. These files can be assembled with the A51 assembler. For |
|
more information, refer to the SRC directive in the following sections. |