
- •Table of Contents
- •C# and the .NET Platform, Second Edition
- •Introduction
- •Part One: Introducing C# and the .NET Platform
- •Part Two: The C# Programming Language
- •Part Three: Programming with .NET Assemblies
- •Part Four: Leveraging the .NET Libraries
- •Part Five: Web Applications and XML Web Services
- •Obtaining This Book's Source Code
- •The .NET Solution
- •What C# Brings to the Table
- •The Role of the Assembly Manifest
- •Summary
- •Chapter 2: Building C# Applications
- •Summary
- •Chapter 3: C# Language Fundamentals
- •Defining Program Constants
- •Defining Custom Class Methods
- •C# Enumerations
- •Summary
- •The Second Pillar: C#'s Inheritance Support
- •Summary
- •Catching Exceptions
- •Finalizing a Type
- •Garbage Collection Optimizations
- •Summary
- •Chapter 6: Interfaces and Collections
- •Building Comparable Objects (IComparable)
- •Summary
- •Summary
- •Internal Representation of Type Indexers
- •Summary
- •An Overview of .NET Assemblies
- •Understanding Delayed Signing
- •Using a Shared Assembly
- •GAC Internals
- •Summary
- •Spawning Secondary Threads
- •A More Elaborate Threading Example
- •Summary
- •Summary
- •Object Persistence in the .NET Framework
- •The .NET Remoting Namespaces
- •Understanding the .NET Remoting Framework
- •All Together Now!
- •Terms of the .NET Remoting Trade
- •Testing the Remoting Application
- •Revisiting the Activation Mode of WKO Types
- •Deploying the Server to a Remote Machine
- •Summary
- •Control Events
- •The Form Class
- •Summary
- •Regarding the Disposal of System.Drawing Types
- •Understanding the Graphics Class
- •Summary
- •The TextBox Control
- •Working with Panel Controls
- •Configuring a Control's Anchoring Behavior
- •Summary
- •Chapter 16: The System.IO Namespace
- •The Static Members of the Directory Class
- •The Abstract Stream Class
- •Summary
- •The Role of ADO.NET Data Providers
- •The Types of System.Data
- •Selecting a Data Provider
- •The Types of the System.Data.OleDb Namespace
- •Working with the OleDbDataReader
- •Summary
- •Submitting the Form Data (GET and POST)
- •Some Benefits of ASP.NET
- •Creating an ASP.NET Web Application by Hand
- •The Composition of an ASP.NET Page
- •The Derivation of an ASP.NET Page
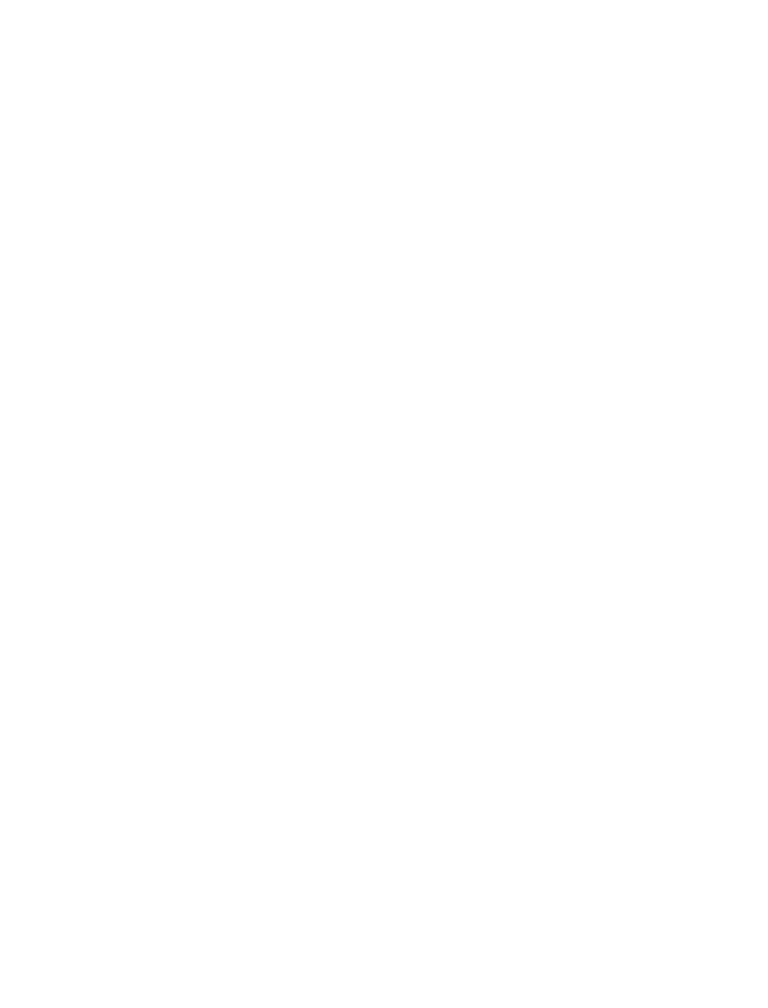
Working with the OleDbDataReader |
|
C# and the .NET Platform, S cond Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
Once you have establishedApress the active connection and SQL command, the next step is to truly submit the
© 2003 (1200 pages)
query to the data source. As you would guess, there are a number of ways to do so. The OleDbDataReader
This comprehensive text starts with a brief overview of the
type (which implementsC# languageIDataReader)and then isquicklythe simplestov toandkeyfastesttechnicalwayandto obtain information from a data store. This class representsarchitecturala read-only,issuesforwardfor .NET-onlydevelopersstream.of data returned one record at a time. Given this, it should stand to reason that data readers are useful only when submitting SQL selection statements to the underlying data store.
Table of Contents
C#TheandOleDbDataReaderthe .NET Platform,isSecondusefulEditionwhen you need to iterate over large amounts of data very quickly and have
no need to work an in-memory DataSet representation. For example, if you request 20,000 records from a
Introduction
table to store in a text file, it would be rather memory intensive to hold this information in a DataSet. A better
Part One - Introducing C# and the .NET Platform
approach would be to create a data reader that spins over each record as rapidly as possible. Be aware,
Chapter 1 - The Philosophy of .NET
however, that DataReaders (unlike data adapter types) maintain an open connection to their data source
Chapter 2 - Building C# Applications
until you explicitly close the session.
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
To illustrate, the following example issues a simple SQL query against the Cars database, using the
Chapter 4 - Object-Oriented Programming with C#
ExecuteReader() method of the OleDbCommand type. Using the Read() method of the returned
Chapter 5 - Exceptions and Object Lifetime
OleDbDataReader, you dump each member to the standard IO stream:
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
public class OleDbDR
Chapter 8 - Advanced C# Type Construction Techniques
{
Part Three - Programming with .NET Assemblies
static void Main(string[] args)
Chapter 9 - Understanding .NET Assemblies
{
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Make a connection.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
OleDbConnection cn = new OleDbConnection();
Part Four - Leveraging the .NET Libraries
cn.ConnectionString = "Provider=SQLOLEDB.1;" +
Chapter 12 - Object Serialization and the .NET Remoting Layer
"User ID=sa;Pwd=;Initial Catalog=Cars;" +
Chapter 13 - Building"DataBe terSource=(local);ConnectWindow (Introducing Wi dows Forms)Timeout=30";
Chapter 14 - A cnBetter.Open();Painting Framework (GDI+)
Chapter... 15 - Programming with Windows Forms Controls
// Create a SQL command.
Chapter 16 - The System.IO Namespace
string strSQL = "SELECT Make FROM Inventory WHERE Color='Red'";
Chapter 17 - Data Access with ADO.NET
OleDbCommand myCommand = new OleDbCommand(strSQL, cn);
Part Five - Web Applications and XML Web Services
// Obtain a data reader a la ExecuteReader().
Chapter 18 - ASP.NET Web Pages and Web Controls
OleDbDataReader myDataReader;
Chapter 19 - ASP.NET Web Applications
myDataReader = myCommand.ExecuteReader();
Chapter 20 - XML Web Services
// Loop over the results.
Index
Console.WriteLine("***** Red cars obtained from a DataReader *****");
List of Figures
while (myDataReader.Read())
List of Tables
{
Console.WriteLine("-> Red car: " + myDataReader["Make"].ToString());
}
// Don't forget this!
myDataReader.Close();
cn.Close();
}
}
The result is the listing of all red automobiles in the Cars database (Figure 17-22).
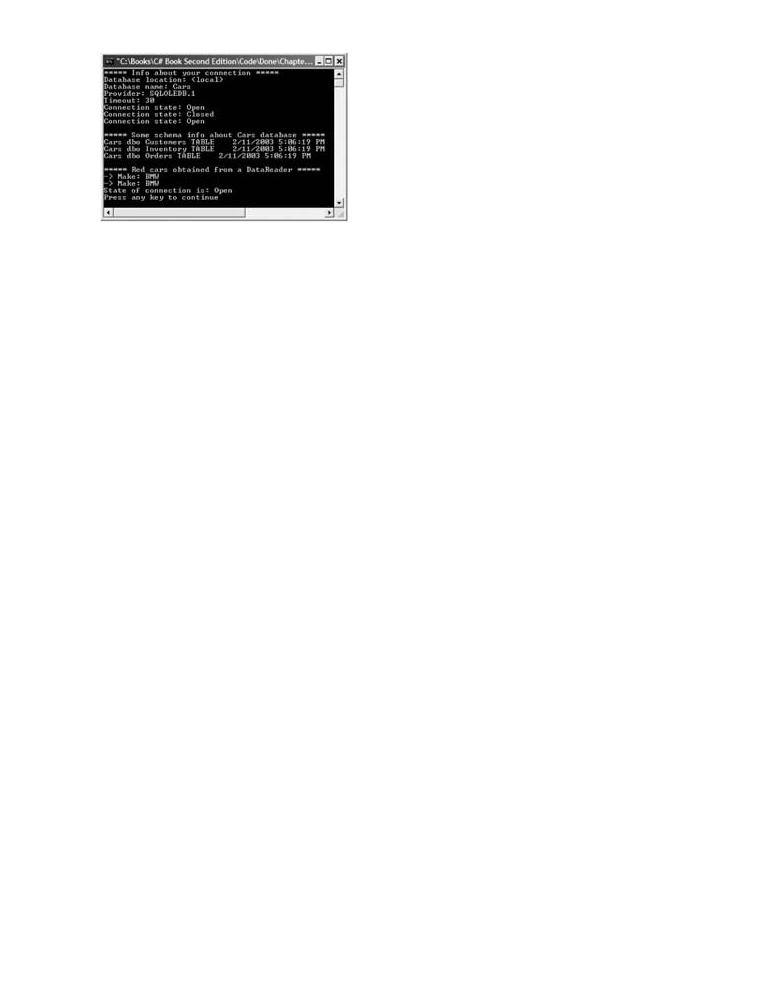
Second Edition
ISBN:1590590554
with a brief overview of the
moves to key technical and
developers.
Table
C# and
Introduction
Figure 17-22: Reading records with the OleDbDataReader
Part One - Introducing C# and the .NET Platform
ChapterRecall 1that-DataReadersThe Philosophyareof forward.NET -only, read-only streams of data. Given this, there is no way to navigate
around the contents of the OleDbDataReader. All you can do is read the current record in memory and use it
Chapter 2 - Buildi g C# Applications
in your application:
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter// Get4 the- Objectvalue-Orientedin theProgramming'Make'withcolumnC# .
ChapterConsole5 .-WriteLine("RedExceptions and Objectcar:Lifetime{0} ", myDataReader["Make"].ToString());
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
When you are finished using the DataReader, make sure to terminate the session using the appropriately
Chapter 8 - Advanced C# Type Construction Techniques named method, Close().
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
In addition to the Read() and Close() methods, there are a number of other methods that allow you to obtain
Chapter 10 - Processes, AppDomains, Contexts, and Threads
a strongly typed value from a specified column in a given format (e.g., GetBoolean(), GetByte(), and so Chapterforth). Thus,11 - Typeif youReflection,know thatLatetheBinding,column andyouAttributeare attempting-Based toProgrammingaccess has been typed as a Boolean data
Parttype,Fouryou- canLeveragingavoid antheexplicit.NET Librariescast by making a call to GetBoolean(). Finally, understand that the FieldCount
Chaptpropertyr 12returns- ObjecttheSerializationnumber ofandcolumnsthe .NETin theR motingcurrentLayerrecord.
Chapter 13 - Building a Better Window (Introducing Windows Forms)
ChaSpecifyingt r 14 - A BetterthePaintingDataFramReader'swork (GDI+)Command Behavior
Chapter 15 - Programming with Windows Forms Controls
ChapterAs you16may- ThehaveSystemnoticed,.IOtheNamespaceOleDbCommand.ExecuteReader() method has been overloaded. One version
Chapterof this member17 - DatatakesAccessa valuewith ADOfrom.NETthe CommandBehavior enumeration. This type supports the following
Partvalues:Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
public enum System.Data.CommandBehavior
Chapter{ 20 - XML Web Services
Index CloseConnection, Default,
List of FiguresKeyInfo, SchemaOnly,
SequentialAccess,
List of Tables
SingleResult, SingleRow,
}
One value of interest is CommandBehavior.CloseConnection. If you specify this value, the underlying connection maintained by the connection object will be automatically closed once you close the data reader type (check out online help for details on the remaining values):
// Auto close the connection.
OleDbDataReader myDataReader;
myDataReader =
myCommand.ExecuteReader(CommandBehavior.CloseConnection);
...
myDataReader.Close();
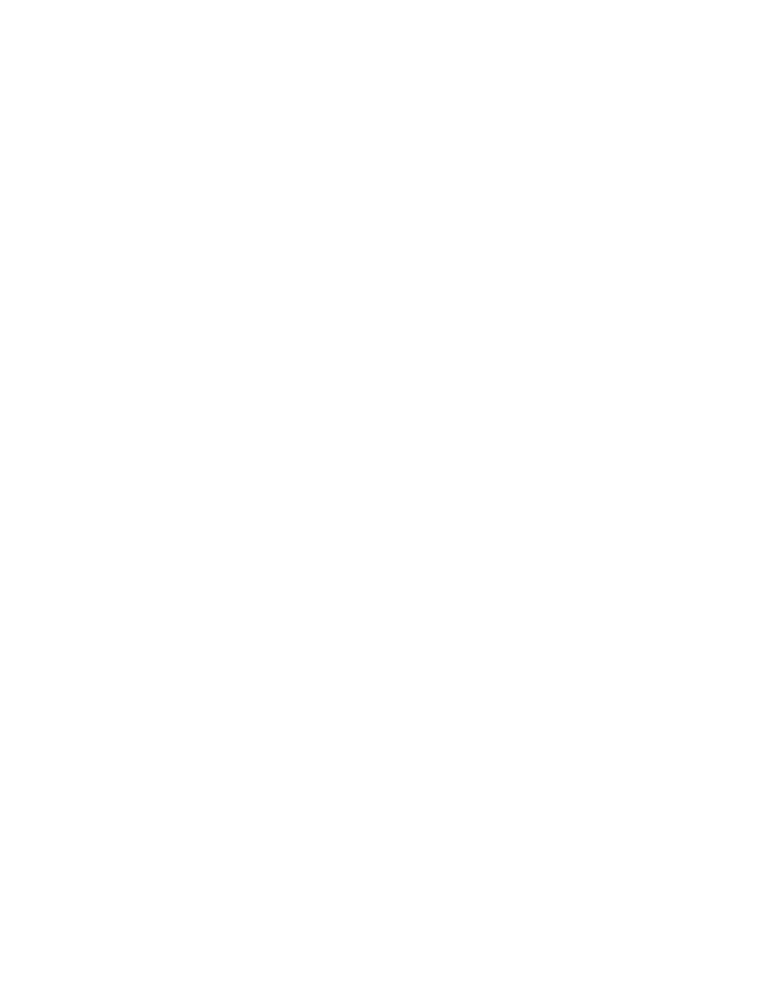
// No need to do this anymore! cn.Close();
C# and the .NET Platform, Second Edition
Console.WriteLine("State of connection is: {0}", cn.State);
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages) |
|
This comprehensive text starts with a brief overview of the
Obtaining Multiple Result Sets Using a OleDbDataReader
C# language and then quickly moves to key technical and
architectural issues for .NET developers.
Data reader types are able to obtain multiple result sets from a single command object. For example, if you are interested in obtaining all rows from the Inventory table as well as all rows from the Customers table, you
Tableare ableof Contentsto specify both SQL SELECT statements using a semicolon delimiter:
C# and the .NET Platform, Second Edition
Introductionstring theSQL = "SELECT * From Inventory;SELECT * from Customers";
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Once you obtain the data reader, you are able to iterate over each result set via the NextResult() method. Do
Chapter 2 - Building C# Applications
be aware that you are always returned the first result set automatically. Thus, if you wish to read over the
Part Two - The C# Programming Language
rows of each table, you would be able to build the following iteration construct:
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
do
Chapter 5 - Exceptions and Object Lifetime
{
Chapter 6 - Interfaces and Collections
while(myDataReader.Read())
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter{8 - Advanced C# Type Construction Techniques
// Read the info of the current result set.
Part Three - Programming with .NET Assemblies
}
Chapter 9 - Understanding .NET Assemblies
}while(myDataReader.NextResult());
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Obtaining Schema Information Using a OleDbDataReader
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
A final point to be made regarding ADO.NET data reader types is that they also provide a manner to obtain schema information. However, unlike the connection types (which allow you to obtain schema information for
the entire database), the OleDbDataReader.GetTableSchema() method returns a DataTable that describes
Chapter 16 - The System.IO Namespace
the characteristics of the table specified by the SQL SELECT statement:
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
DataTable dt = myDataReader.GetSchemaTable();
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List ofSOURCEFigures The OleDbDataReaderApp application is included under the Chapter 17 subdirectory.
List ofCODETables
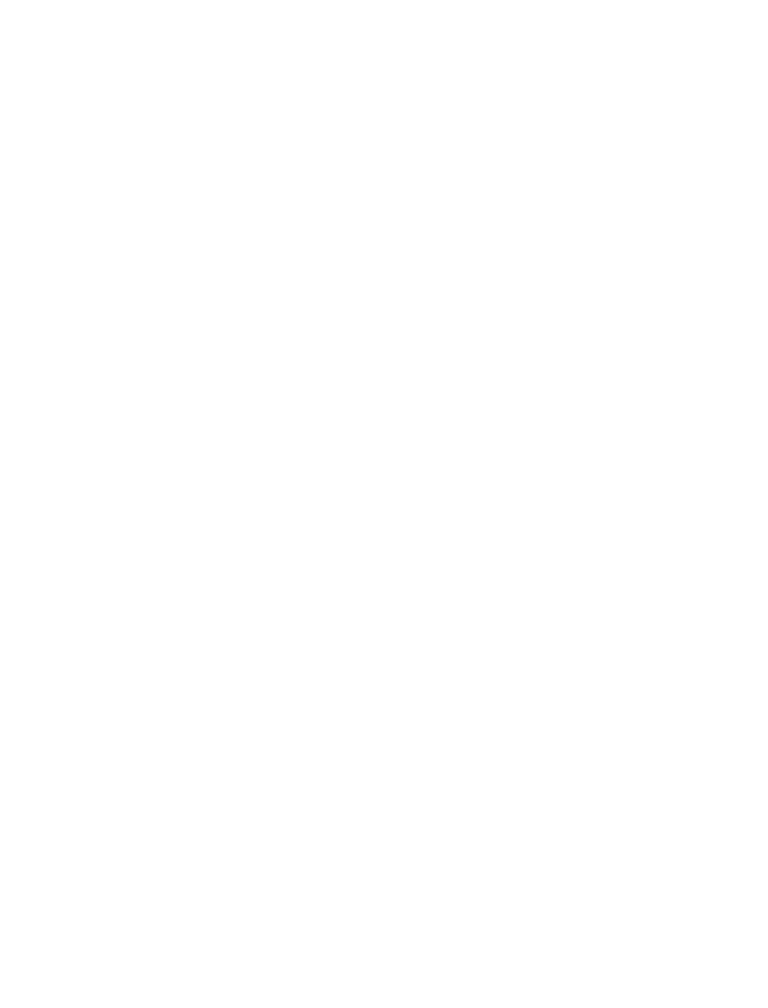
Inserting, Updating,C# and the .NETandPlatform,DeletingSecondRecordsEditi n Using OleDbCommand
by Andrew Troelsen |
ISBN:1590590554 |
As you have just seen, the ExecuteReader() method allows you to examine the results of a SQL SELECT
Apress © 2003 (1200 pages)
statement using a forward-only, read-only flow of information. However, when you wish to submit SQL
This comprehensive text starts with a brief overview of the commands that resultC# languagein the modificationand then quicklyof a givenmovestable,to keyyoutechnicalmake useand of the
OleDbCommandarchitectural.ExecuteNonQuery()iss s formethod.NET developers. This single. method will perform inserts, updates, and deletes based on the format of your command text. Be very aware that when you are making use of the OleDbCommand.ExecuteNonQuery() method, you are operating within the connected layer of ADO.NET,
Table of Contents
meaning this method has nothing to do with obtaining populated DataSet types via a data adapter.
C# and the .NET Platform, Second Edition
To illustrate modifying a data source via ExecuteNonQuery(), assume you wish to insert a new record into the
Introduction
Inventory table of the Cars database. Once you have configured your connection type, the remainder of your
Part One - Introducing C# and the .NET Platform
task is as simple as authoring the correct SQL:
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
PartclassTwo -UpdateWithCommandObjThe C# Programming Language
Chapter{ |
3 |
- C# Language Fundamentals |
Chapter static4 - Objectvoid-OrientedMain(string[]Programming withargs)C# |
||
Chapter {5 |
- Exceptions and Object Lifetime |
|
Chapter 6 |
- Interfaces// OpenandaCollectionsconnection to Cars db. |
|
Chapter 7 |
- CallbackOleDbConnectionInterfaces, Delegates,cn = andnewEventsOleDbConnection(); |
|
Chapter 8 |
- Advancedcn.ConnectionStringC# Type Construction= Techniques"Provider=SQLOLEDB.1;" + |
"User ID=sa;Pwd=;Initial Catalog=Cars;" +
Part Three - Programming with .NET Assemblies
"Data Source=(local);Connect Timeout=30";
Chapter 9 - Understanding .NET Assemblies cn.Open();
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// SQL INSERT statement.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming string sql = "INSERT INTO Inventory" +
Part Four - Leveraging the .NET Libraries
"(CarID, Make, Color, PetName) VALUES" +
Chapter 12 - Object Serialization and the .NET Remoting Layer
"('777', 'Honda', 'Silver', 'NoiseMaker')";
Chapter 13 - Building a Better Window (Introducing Windows Forms)
// Insert the record.
Chapter 14 - A Better Painting Framework (GDI+)
OleDbCommand cmd = new OleDbCommand(sql, cn);
Chapter 15 - Programming with Windows Forms Controls try
Chapter 16 - The System.IO Namespace
{ cmd.ExecuteNonQuery(); }
Chapter 17 - Datacatch(ExceptionA cess with ADO.NETex)
Part Five - Web{ApplicationsConsole.andWriteLine(exXML Web S rvices.Message); }
Chapter 18 - ASPcn.NET.Close();Web Pages and Web Controls
Chapter }19 - ASP.NET Web Applications
}
Chapter 20 - XML Web Services
Index
List of Figures
Updating or removing a record is just as easy:
List of Tables
// UPDATE existing record.
sql = "UPDATE Inventory SET Make = 'Hummer' WHERE CarID = '777'"; cmd.CommandText = sql;
try {cmd.ExecuteNonQuery();} catch(Exception ex)
{Console.WriteLine(ex.Message);}
// DELETE a record.
sql = "Delete from Inventory where CarID = '777'"; cmd.CommandText = sql;
try {cmd.ExecuteNonQuery();} catch(Exception ex)

{Console.WriteLine(ex.Message);}
C# and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Although you do notApressbother© 2003to(1200obtainpages)the value returned from the ExecuteNonQuery() method, do understand that this memberThisreturnscomprehensivea System.Int32text thatst rtsrepresentswith a brieftheoverviewnumberofoftheaffected records:
C# language and then quickly moves to key technical and
architectural issues for .NET developers.
try
{
Console.WriteLine("Number of rows effected: {0}", cmd.ExecuteNonQuery());
Table of Contents
}
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Finally, do be aware that the ExecuteNonQuery() method of a given command object is the only way to issue
Chapter 1 - The Philosophy of .NET
Data Definition Language (DDL) commands to a data source (e.g., CREATE, ALTER, DROP, and so forth).
Chapter 2 - Building C# Applications
Part TwoSOURCE- The C# ProgrammingThe UpdateDBWithCommandObjLanguage application is included under the Chapter 17
ChapterCODE3 - C# LanguagesubdirectoryFundamentals.
Chapter 4 - Object-Oriented Programming with C#
ChapterWorking5 - ExcepwithionsParameterizedand Object Lifet me Queries
Chapter 6 - Interfaces and Collections
ChapterThe previous7 - Callbackinsert, update,Interfaces,andDeldeletegates,logicandworkedEvents as expected; however, you are able to tighten things up
just a bit. As you may know, a parameterized query can be useful when you wish to treat SQL parameters as
Chapter 8 - Advanced C# Type Construction Techniques
objects, rather than as a value within a hard-coded string. Typically, parameterized queries execute much
Part Three - Programming with .NET Assemblies
faster than a literal block of text, in that they are parsed exactly once (rather than each time the SQL string is
Chapter 9 - Understanding .NET Assemblies
assigned to the CommandText property).
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Like classic ADO, ADO.NET command objects maintain a collection of discrete parameter types. While it is
Part Four - Leveraging the .NET Libraries
initially more verbose to configure the parameters used for a given SQL query, the end result is a more
Chapter 12 - Object Serialization and the .NET Remoting Layer
convenient manner to tweak SQL statements programmatically. When you wish to map a textual parameter
Chapter 13 - Building a Better Window (Introducing Windows Forms)
to a member in the command object's parameters collection, simply create a new OleDbParameter type and
Chapter 14 - A Better Painting Framework (GDI+)
add it to the OleDbCommand's internal collection using the Parameters property. In addition, make use of
Chapter 15symbol- Pr gramming with Windows Forms Controls
the "@" in the string itself. While you are free to make use of this technique whenever a SQL query is Chapterinvolved,16it-isThemostSysthelpfulm.IOwhenNamespaceyou wish to trigger a stored procedure.
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Executing aC#Storedand the .NETProcedurePlatform, SecondUsingEditionOleDbCommand
by Andrew Troelsen |
ISBN:1590590554 |
Astored procedure is a named block of SQL code stored at the database. Stored procedures can be
Apress © 2003 (1200 pages)
constructed to return a set of rows (or native data types) to the calling component and may take any
This comprehensive text starts with a brief overview of the
number of optionalC#parametersl nguage and. Thethenendquicklyresultmovesis a unitto keyof worktechnicalthat behavesand like a typical function, with the obvious differencesarchitecturalof beingissueslocatedfor .NETon adevelopersdata store. rather than a binary business object.
Let's add a simple stored procedure to the existing Cars database called GetPetName, which takes an
TableinputofparameterCont ntsof type integer. (If you ran the supplied SQL script, this stored proc is already defined.) C#Thisandisthethe.numericalNET Platform,ID ofSecondthe carEditionfor which you are interested in obtaining the pet name, which is returned
as an output parameter of type char. Here is the syntax:
Introduction
Part One - Introducing C# and the .NET Platform
ChapterCREATE1 PROCEDURE- The PhilosophyGetPetNameof .NET
Chapter@carID2 - Buildiint,g C# Applications
Part Two@petName- The C# Programmingchar(20) outputLanguage
ChapterAS 3 - C# Language Fundamentals
SELECT @petName = PetName from Inventory where CarID = @carID
Chapter 4 - Object-Oriented Programming with C# Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Now that you have a stored procedure in place, let's see the code necessary to execute it. Begin as always
Chapter 7 - Callback Interfaces, Delegates, and Events
by creating a new OleDbConnection, configure your connection string, and open the session. Next, create
Chapter 8 - Advanced C# Type Construction Techniques
a new OleDbCommand type, making sure to specify the name of the stored procedure and set the
Part Three - Programming with .NET Assemblies
CommandType property accordingly, as shown here:
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Open connection to data store.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
OleDbConnection cn = new OleDbConnection();
Part Four - Leveraging the .NET Libraries
cn.ConnectionString = "Provider=SQLOLEDB.1;" +
Chapter 12 - Object Serialization and the .NET Remoting Layer
"User ID=sa;Pwd=;Initial Catalog=Cars;" +
Chapter 13 - Building a Better Window (Introducing Windows Forms)
"Data Source=(local);Connect Timeout=30";
Chapter 14 - A Better Painting Framework (GDI+)
cn.Open();
Chapter 15 - Programming with Windows Forms Controls
// Make a command object for the stored proc.
Chapter 16 - The System.IO Namespace
OleDbCommand myCommand = new OleDbCommand("GetPetName", cn);
Chapter 17 - Data Access with ADO.NET StoredProcedure myCommand.CommandType = CommandType. ;
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Specifying Parameters Using the OleDbParameter Type
Chapter 20 - XML Web Services
Index
The next task is to establish the parameters used for the call. To illustrate the syntax of parameterized Listqueries,of Figuresyou will use the OleDbParameter type. This class maintains a number of properties that allow you
Listto configureof Tables the name, size, and data type of the parameter, as well as its direction of travel. Table 17-16 describes some key properties of the OleDbParameter type.
Table 17-16: Key Members of the OleDbParameter Type
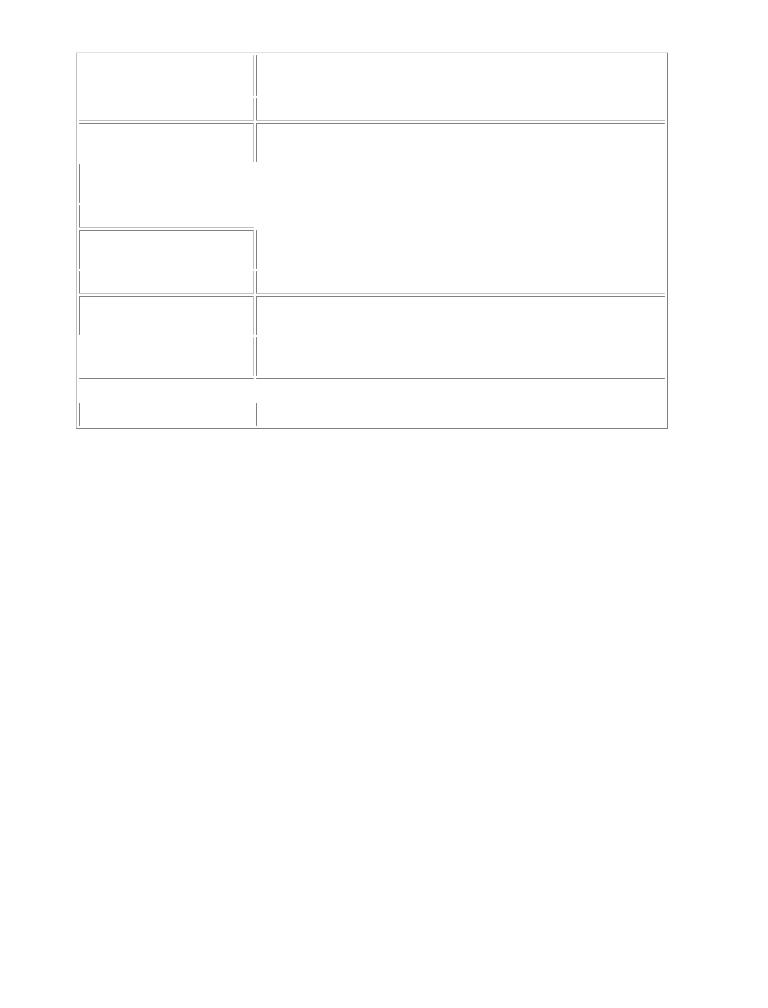
|
|
|
|
|
|
|
|
|
|
OleDbParameterC# and the .NETMeaningPlatform,inSecondLife Edition |
|
|
|||||
|
Property |
by Andrew Troelsen |
ISBN:1590590554 |
|
||||
|
|
|
|
|
|
|
|
|
|
DataType |
Apress © 2003 |
|
(1200 pages) |
|
|
||
|
|
|
|
|
Establishes the type of the parameter, in terms of .NET |
|
||
|
|
This comprehensive text starts with a brief overview of the |
|
|||||
|
|
|
||||||
|
DbType |
C# language and then quickly moves to key technical and |
|
|||||
|
|
|
|
|
Gets or sets the native data type from the data source, represented |
|
||
|
|
architectural issues for .NET developers. |
|
|
||||
|
|
|
|
|
|
as a CLR data type |
|
|
|
|
|
|
|
|
|
||
|
Direction |
|
|
|
|
Gets or sets whether the parameter is input-only, output-only, |
|
|
Table of Contents |
|
|
|
|
bidirectional, or a return value parameter |
|
||
|
|
|
|
|
|
|
||
C# and the .NET Platform, Second |
|
|
|
Edition |
|
|
||
|
IsNullable |
|
|
|
|
Gets or sets whether the parameter accepts null values |
|
|
|
Introduction |
|
|
|
|
|
|
|
|
|
|
|
|
Gets or sets the native data type from the data source represented |
|
||
|
OleDbType |
|
|
|
|
|
||
Part One - Introducing C# and the .NET Platform |
|
|
||||||
Chapter 1 - The Philosophy of .NETby the OleDbType enumeration |
|
|||||||
|
|
|
|
|
|
|
||
|
Chapter 2 - Building C# Applications |
|
|
|||||
|
ParameterName |
|
|
|
Gets or sets the name of the OleDbParameter |
|
||
Part Two - The C# Programming |
|
|
|
Language |
|
|
||
|
|
|
|
|
||||
ChapterPrecision3 - C# Language FundamentalsGets or sets the maximum number of digits used to represent the |
|
|||||||
Chapter 4 - Object-Oriented ProgrammingValue propertywith C# |
|
|
||||||
|
|
|
|
|
|
|
||
|
Chapter 5 - Exceptions and Object Lifetime |
|
|
|||||
|
Scale |
|
|
|
|
Gets or sets the number of decimal places to which the Value |
|
|
|
Chapter 6 - Interfaces and Collections |
|
|
|||||
|
|
|
|
|
|
property is resolved |
|
|
|
Chapter 7 - Callback Interfaces, |
|
|
|
Delegates, and Events |
|
|
|
|
|
|
|
|
||||
|
ChapterSize 8 - Advanced C# Type |
|
|
|
ConstructionGets or setsTechniquesthe maximum parameter size of the data |
|
||
|
|
|
|
|
|
|
||
Part Three - Programming with |
|
.NET Assemblies |
|
|
||||
|
Value |
|
|
|
|
Gets or sets the value of the parameter |
|
|
|
Chapter 9 - Understanding .NET |
|
|
Assemblies |
|
|
Chapter 10 - Processes, AppDomains, Contexts, and Threads
ChapterGiven that11 -youTypehaveR flectioone input, LateandBinding,one outputand Attributeparameter,-BasedyouProgrammingcan configure your types as follows. Note
PartthatFouryou -thenLeveragingadd theseitems.NET Librariesto the OleDbCommand type's ParametersCollection (which is, again,
Chapteraccessed12 via- Objectthe ParametersSerializationproperty):and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter// Create14 - A theBetterparametersPainting Framewfork (GDI+)the call.
ChapterOleDbParameter15 - ProgrammingtheParamwith Windows= newFormsOleDbParameter();Controls
Chapter// Input16 - TheparamSystem. .IO Namespace
theParam.ParameterName = "@carID";
Chapter 17 - Data Access with ADO.NET
theParam.OleDbType = OleDbType.Integer;
Part Five - Web Applications and XML Web Services
theParam.Direction = ParameterDirection.Input;
Chapter 18 - ASP.NET Web Pages and Web Controls
theParam.Value = 1; // Car ID = 1.
Chapter 19 - ASP.NET Web Applications
myCommand.Parameters.Add(theParam);
Chapter 20 - XML Web Services
// Output param.
Index
theParam = new OleDbParameter();
List of Figures
theParam.ParameterName = "@petName";
List of Tables
theParam.OleDbType = OleDbType.Char;
theParam.Size = 20;
theParam.Direction = ParameterDirection.Output;
myCommand.Parameters.Add(theParam);
The final step is to issue the command using (once again) OleDbCommand.ExecuteNonQuery(). Notice that the Value property of the OleDbParameter type is accessed to obtain the returned pet name, as shown here:
// Execute the stored procedure!
myCommand.ExecuteNonQuery();
// Display the result.
Console.WriteLine("Stored Proc Info:");
Console.WriteLine("Car ID: {0}", myCommand.Parameters["@carID"].Value);
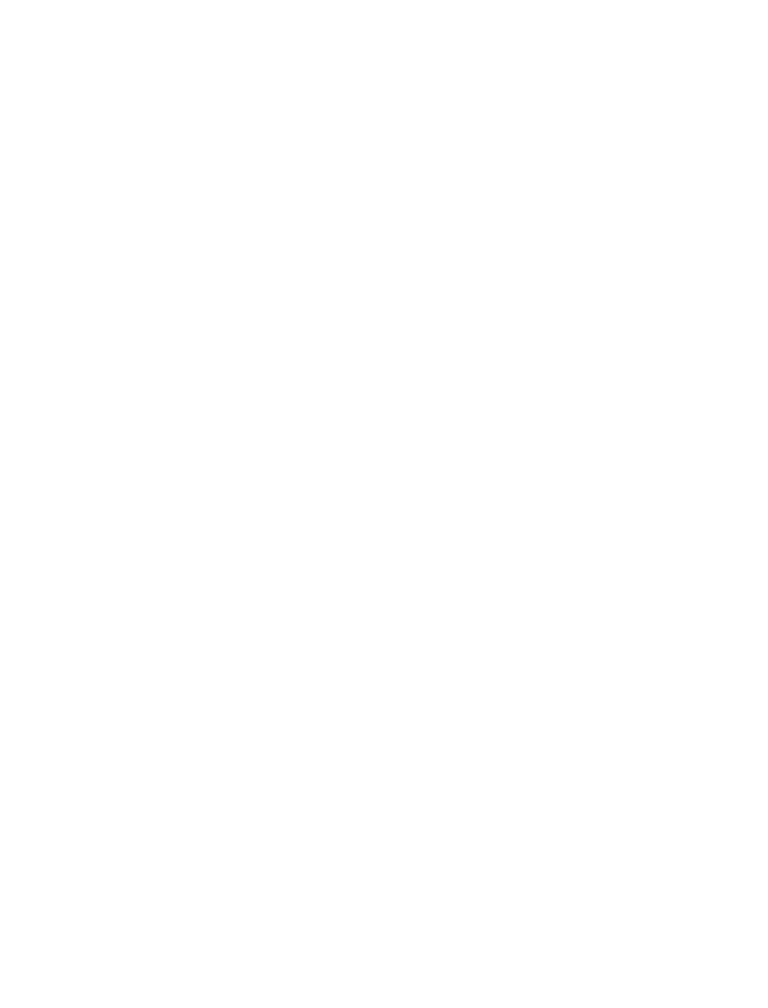
Console.WriteLine("PetName: {0}", myCommand.Parameters["@petName"].Value);
C# and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
SOURCE
CODE
Table of Contents
Apress © 2003 (1200 pages)
ThisThecomprOleDbStoredProchensive text startsprojectwith isa briefincludedoverviewunderofthetheChapter 17 subdirectory.
C# language and then quickly moves to key technical and architectural issues for .NET developers.
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

The DisconnectedC# and the Layer.NET Platform,and Secondthe OleDbDataAdapterEdition Type
by Andrew Troelsen |
ISBN:1590590554 |
At this point you should understand how to connect to a data source using the OleDbConnection type,
Apress © 2003 (1200 pages)
issue a command (using the OleDbCommand and OleDbParameter types), and iterate over a result set
This comprehensive text starts with a brief overview of the
using the OleDbDataReaderC# language. Asandyouthenhavequicklyjustmovesseen, theseo keytypestechnicalallowandyou to obtain and alter data using the connected layerarchitecturalof ADO.NETissues. However,for .NET devwhenlopersyou .are using these techniques, you are not receiving your data via DataSets, DataTables, DataRows, or DataColumns. Given that you spend a good deal of time in this chapter getting to know these types, you are right in assuming that there is more to the story of
Table of Contents
data access than the forward-only, read-only world of the data reader.
C# and the .NET Platform, Second Edition
To shift gears, we now need to examine the role of the OleDbDataAdapter type and understand how it is
Introduction
our vehicle to obtaining a populated DataSet. In a nutshell, data adapters pull information from a data
Part One - Introducing C# and the .NET Platform
store and populate a DataTable or DataSet type using the OleDbDataAdapter.Fill() method. As you can
Chapter 1 - The Philosophy of .NET
see, this method has been overloaded a number of times (FYI, the integer return type holds the number of
Chapter 2 - Building C# Applications
records returned):
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
// Fills the data set with records from a given source table.
Chapter 4 - Obj ct-Oriented Programming with C#
public int Fill(DataSet yourDS, string tableName);
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
// Fills the data set with the records located between
Chapter 7 - Callback Interfaces, Delegates, and Events
// the given bounds from a given source table.
Chapter 8 - Advanced C# Type Construction Techniques
public int Fill(DataSet yourDS, int startRecord,
Part Three - Programming with .NET Assemblies
int maxRecord, string tableName);
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
The OleDbDataAdapter.Fill() method has also been overloaded to return to you a populated DataTable
Chapter 12 |
- Object Serialization nd the .NET Remoting Layer |
type, rather than a full-blown DataSet: |
|
Chapter 13 |
- Building a Better Window (Introducing Windows Forms) |
Chapter 14 - A Better Painting Framework (GDI+)
public int Fill(System.Data.DataTable dataTable);
Chapter 15 - Programming with Windows Forms Controls Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part FiveNote- WebIt is alsoApplicationsworth pointingand XMLoutWebthatServicversionone of the Fill() method will automatically populate a
classic ADO Recordset type with the contents of a given ADO.NET DataTable.
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Before you can call this method, you obviously need a valid OleDbDataAdapter object reference. The
Chapter 20 - XML Web Services
constructor has also been overloaded a number of times, but in general you need to supply the
Index
connection information and the SQL SELECT statement used to populate the DataTable/DataSet type at
List of Figures
the time Fill() is called. Once the initial SQL Select has been established via the constructor parameter, it
List of Tables
may be obtained (and modified) via the SelectCommand property:
//Whenever you call Fill(), the SQL statement
//contained within the SelectCommand property
//will be used to populate the DataSet. myDataAdapter.Fill(myDS, "Inventory");
The OleDbDataAdapter type not only fills the tables of a DataSet on your behalf, but also is in charge of maintaining a set of core SQL statements used to push updates back to the data store. When you call the Update() method of a given data adapter, it will read the SQL contained within the DeleteCommand, InsertCommand, and UpdateCommand properties to push the changes within a given DataTable back to the data source:
//Whenever you call Update(), the SQL statements
//contained within the InsertCommand,
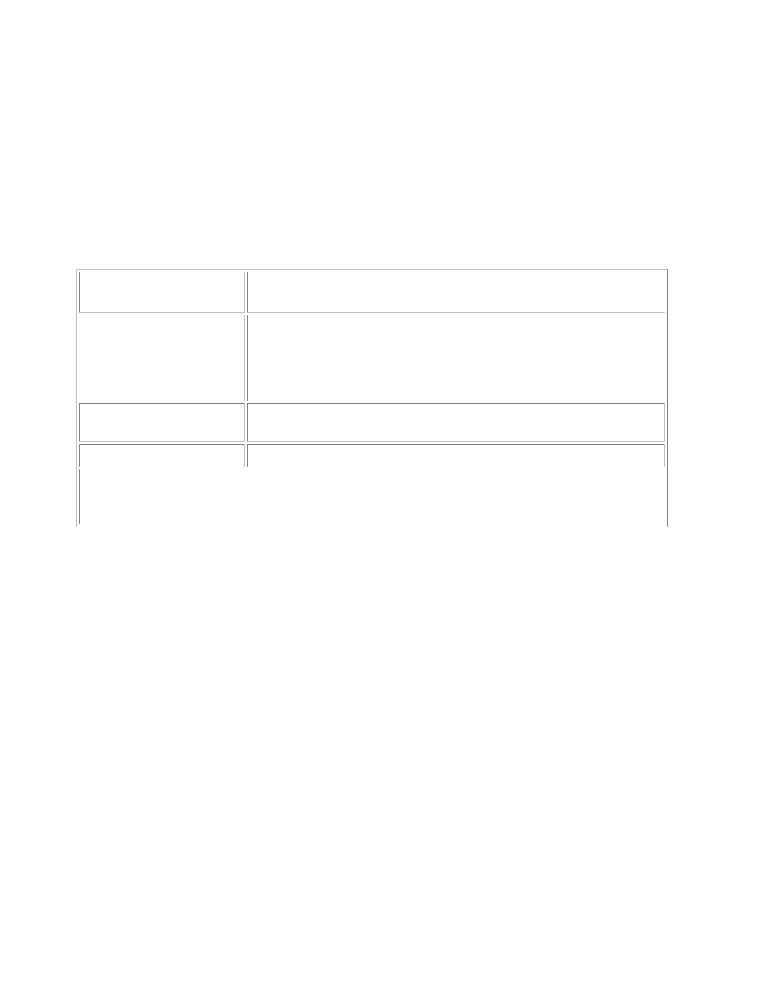
// DeleteCommand, and UpdateCommand properties
C# and the .NET Platform, Second Edition |
|
// will be used to update the data source |
ISBN:1590590554 |
by Andrew Troelsen |
|
// given a DataTable in the DataSet. |
|
Apress © 2003 (1200 pages) |
|
myDataAdapter.Update(myDS, "Inventory"); |
|
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
architectural issues for .NET developers.
Now, if you have a background in classic ADO, you should already be able to see the huge conceptual change at hand: Under ADO.NET, you are the individual in charge of specifying the SQL commands to
use during the updating of a given database.
Table of Contents
C#In additionnd the .NETto thesePlatform,four keySecondpropertiesEdition(SelectCommand, UpdateCommand, DeleteCommand, and
IntroductionInsertCommand),Table 17-17 describes some additional members of the OleDbDataAdapter type.
Part One - Introducing C# and the .NET Platform
ChTablept r 17-17:- TheCorePhilosophyMembersof .ofNETthe OleDbDataAdapter
|
|
|
|
|
|
|
Chapter 2 - Building C# Applications |
|
|||
|
OleDbDataAdapter |
|
Meaning in Life |
|
|
Part Two - The C# Programming Language |
|
||||
|
ChapterMember3 - C# Language Fundamentals |
|
|||
|
|||||
|
Chapter 4 |
- Object-Oriented |
|
Programming with C# |
|
|
DeleteCommand |
|
Used to establish SQL commands that will be issued to the data |
|
|
|
InsertCommand |
|
store when the Fill() and Update() methods are called. Each of these |
|
|
|
Chapter 5 |
- Exceptions and Object Lifetime |
|
||
|
Chapter 6 |
- Interfaces and Collectionsproperties is set using an OleDbCommand type. |
|
||
|
SelectCommand |
|
|
|
|
|
Chapter 7 |
- Callback Interfaces, Delegates, and Events |
|
||
|
ChapterUpdateCommand8 - Advanced C# Type |
|
Construction Techniques |
|
Part Three - Programming with .NET Assemblies
Fill() Fills a given table in the DataSet with some number of records
Chapter 9 - Understanding .NET Assemblies
based on the current SELECT command.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
|
|
|
GetFillParameters() |
|
Returns all parameters used when performing the select command. |
||
|
|
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming |
|
||||
|
|
|
|
|
|||
Part Four - Leveraging the .NET |
|
Libraries |
|
||||
|
|
|
Update() |
|
Calls the respective INSERT, UPDATE, or DELETE statements for |
|
|
Chapter 12 |
- Object Serialization |
|
and the .NET Remoting Layer |
|
|||
|
|
|
|
|
|
each inserted, updated, or deleted row for a given table in the |
|
Chapter 13 |
- Building a Better |
|
Window (Introducing Windows Forms) |
|
|||
|
|
|
|
|
|
DataSet. |
|
|
|
|
|
|
|
|
|
Chapter 14 |
- A Better Painting Framework (GDI+) |
||||||
Chapter 15 |
- Programming with Windows Forms Controls |
Chapter 16 - The System.IO Namespace
Filling a DataSet Using the OleDbDataAdapter Type
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
To understand the functionality of the data adapter types, let's begin by learning how to use a data adapter
Chapter 18 - ASP.programmaticallyNET Web P ges andTheW b Controls
to fill a DataSet . following code populates a DataSet (containing a single table)
Chapterusing an19OleDbDataAdapter:- ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
public static int Main(string[] args)
List of Figures
{
List of Tables
// Open a connection to Cars db.
OleDbConnection cn = new OleDbConnection(); cn.ConnectionString = "Provider=SQLOLEDB.1;" +
"User ID=sa;Pwd=;Initial Catalog=Cars;" +
"Data Source=(local);Connect Timeout=30";
cn.Open();
// Create data adapter using the following SELECT.
string sqlSELECT = "SELECT * FROM Inventory";
OleDbDataAdapter dAdapt = new OleDbDataAdapter(sqlSELECT, cn);
// Create and fill the DataSet (connection closed automatically).
DataSet myDS = new DataSet("CarsDataSet");
dAdapt.Fill(myDS, "Inventory");
// Private helper function.
PrintTable(myDS);
return 0;
}

C# and the .NET Platform, Second Edition
Notice that unlike your work during the first half of this chapter, you did not manually create a DataTable type and add it to the DataSet. Also, you did not call ExecuteReader() from a command object to stream
over the result set. Rather, you specified the Inventory table as the second parameter to the Fill() method,
This comprehensive text starts with a brief overview of the
which functions as the friendly name of the newly populated table. Do be aware that if you do not specify a
C# language and then quickly moves to key technical and
friendly name, the data adapter will simply name the table "Table": architectural issues for .NET developers.
// This DataSet has a single table called 'Table'.
Table of Contents
dAdapt.Fill(myDS);
C# and the .NET Platform, Second Edition
Introduction
Internally, Fill() builds the DataTable, given the name of the table in the data store using the SELECT
Part One - Introducing C# and the .NET Platform
command. In this iteration, the connection between the given SQL SELECT statement and the
Chapter 1 - The Philosophy of .NET
OleDbDataAdapter was established as a constructor parameter:
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter// Create3 - C#aLanguageSELECT Fundamentalscommand as string type.
string sqlSELECT = "SELECT * FROM Inventory";
Chapter 4 - Object-Oriented Programming with C#
OleDbDataAdapter dAdapt = new OleDbDataAdapter(sqlSELECT, cn);
Chapter 5 - Exceptions and Object Lifetime Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
As an alternative, you can associate the OleDbCommand to the OleDbDataAdapter, using the
Chapter 8 - Advanced C# Type Construction Techniques
SelectCommand property, as shown here:
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
// Create a SELECT command object.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
OleDbCommand selectCmd = new OleDbCommand("SELECT * FROM Inventory", cn);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
// Make a data adapter and associate commands.
Part Four - Leveraging the .NET Libraries
OleDbDataAdapter dAdapt = new OleDbDataAdapter();
Chapter 12 - Object Serialization and the .NET Remoting Layer dAdapt.SelectCommand = selectCmd;
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
ChapterThe PrintTable()15 - Programminghelper mewithodWindowsis little Formsmore thanCo trsomels formatting razzle-dazzle:
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
public static void PrintTable(DataSet ds)
Part Five - Web Applications and XML Web Services
{
Chapter
Chapter
Chapter
Index
18 - ASP.NET Web Pagestablend Web Controls
// Get Inventory from DataSet.
Console19 - ASP.NET.WriteLine("HereWeb Applications is what we have right now:\n"); DataTable20 - XML W binvTableServices = ds.Tables["Inventory"];
// Print the Column names.
for(int curCol= 0; curCol< invTable.Columns.Count; curCol++)
List of Figures
{
List of Tables
Console.Write(invTable.Columns[curCol].ColumnName.Trim() + "\t");
}
Console.WriteLine();
// Print each cell.
for(int curRow = 0; curRow < invTable.Rows.Count; curRow++)
{
for(int curCol= 0; curCol< invTable.Columns.Count; curCol++)
{
Console.Write(invTable.Rows[curRow][curCol].ToString().Trim()
+ "\t");
}
Console.WriteLine();
}
}
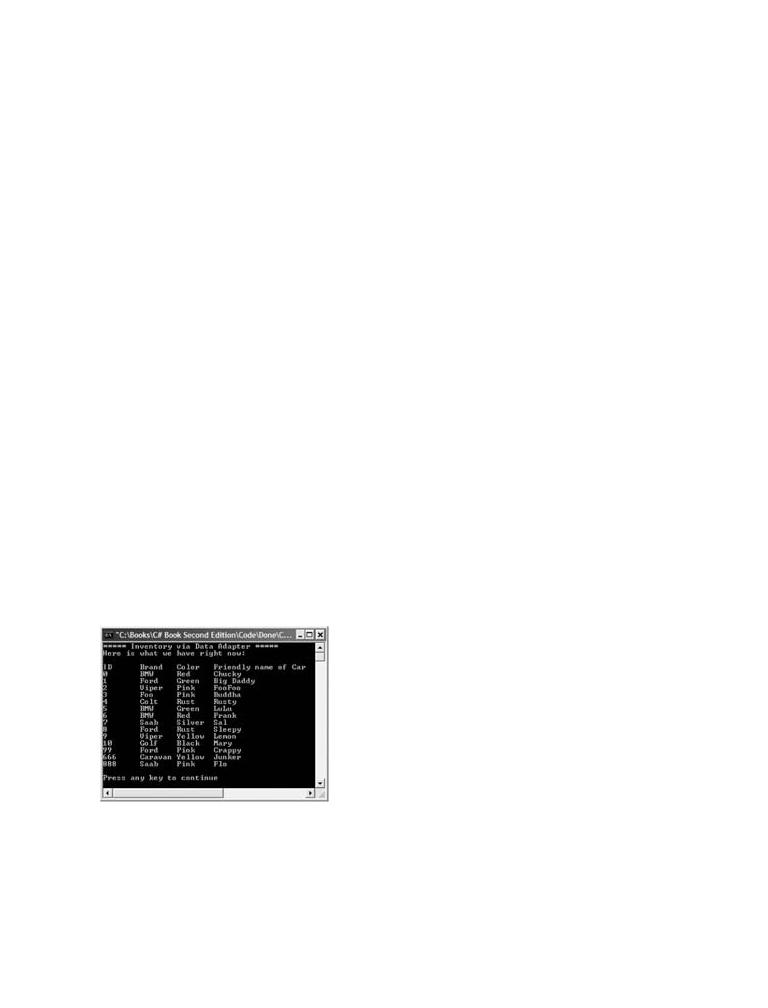
Altering ColumnC# and theNames.NET Platform,UsingSecondthe OleDbDataAdapterEdition Type
by Andrew Troelsen ISBN:1590590554
The default behavior of a data adapter type is to map column names from the data source to the
Apress © 2003 (1200 pages)
DataColumn collection without modification. However, oftentimes you wish to map the underlying column
This comprehensive text starts with a brief overview of the
name (e.g., LName) to a more friendly display name (e.g., Last Name). When you wish to do so, you will
C# language and then quickly moves to key technical and
need to establisharchitecturalspecific columnissuesmappingsfor .NET developersfor the table. you will be filling with the corresponding data adapter. To do so, you must first obtain the data adapter's underlying System.Data.Common.DataTableMapping type (and thus need to specify that you are using the
TableSystemof Con.Dataents.Common namespace). Once you have a reference to this entity, you are able to establish
C#uniqueand thecolumn.NET Plnamestform,forSeconda specificEditiontable. For example, the following code alters the display names for
Introductionthe CarID, Make, and PetName columns of the Inventory table:
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
// Must reference this namespace
Chapter 2 - Building C# Applications
// to get definitions of DataTableMapping!
PartusingTwo -SystemThe C#.ProgrDatamming.Common;Language
Chapter... 3 - C# Language Fundamentals
Chapter// Establish4 - Objectcolumn-Oriented mappingsProgrammingforwith theC# Inventory table
Chapter// by5adding- Exceptionsnew andDataColumnMappingObject Lifetime types
// to the DataTableMapping type.
Chapter 6 - Interfaces and Collections
DataTableMapping tblMapper =
Chapter 7 - Callback Interfaces, Delegates, and Events
dAdapt.TableMappings.Add("Table", "Inventory");
Chapter 8 - Advanced C# Type Construction Techniques
tblMapper.ColumnMappings.Add("CarID", "ID");
Part Three - Programming with .NET Assemblies
tblMapper.ColumnMappings.Add("Make", "Brand");
Chapter 9 - Understanding .NET Assemblies
tblMapper.ColumnMappings.Add("PetName", "Friendly name of Car");
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Create and fill the DataSet.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
dAdapt.Fill(myDS);
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Here, you explicitly alter the internal DataColumnMappingCollection type before making the call to Fill().
Chapter 14 - A Better Painting Framework (GDI+)
Furthermore, note that you specify the friendly name of the table to interact with at the time you add in your Chapterunique15table- Programmingmapping, ratherwiththanWi dowsat theFortimes youC ntrolscall the Fill() method (recall that by default, the name of
Chapterthe newly16 populated- The SystemDataTable.IO Nameispacesimply "Table").
Chapter 17 - Data Access with ADO.NET
If you run this application, you will now find the output shown in Figure 17-23.
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter
Chapter
Index
List of
List of
Figure 17-23: Mapping database column names to unique display names
SOURCE The FillSingleTableDSWithAdapter project is under the Chapter 17 subdirectory.
CODE
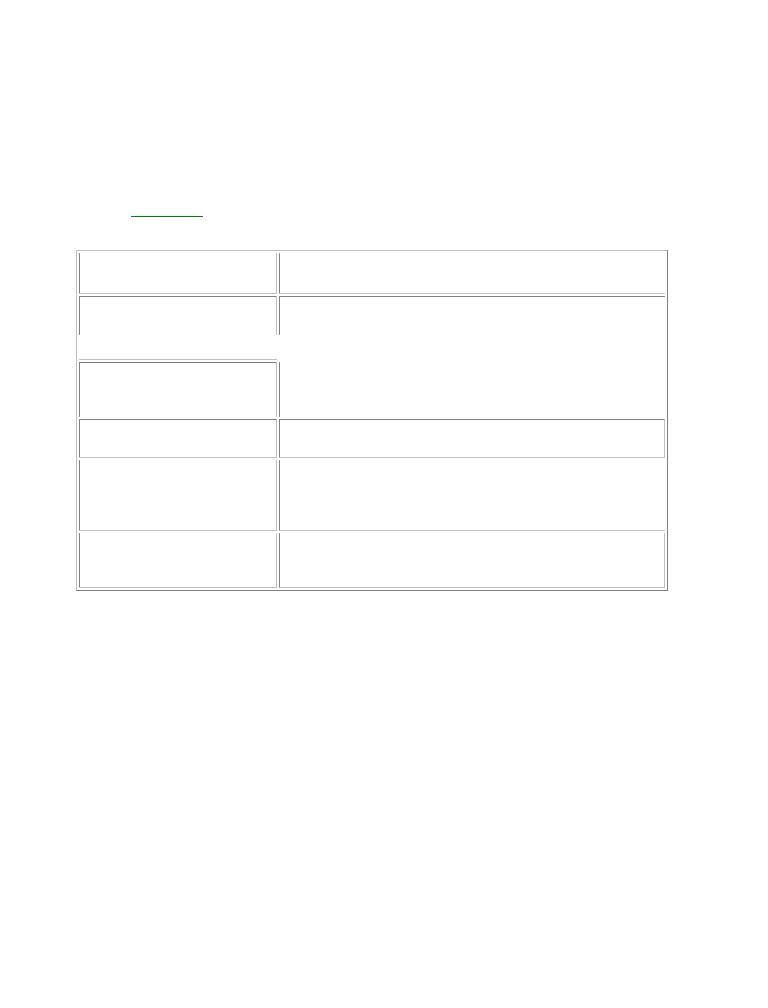
Working withC# andthetheSQL.NET Platform,Data ProviderSecond Edition
by Andrew Troelsen |
ISBN:1590590554 |
Before you see the details of inserting, updating, and removing records using a data adapter, I would like
Apress © 2003 (1200 pages)
to introduce the SQL data provider. As you recall, the OleDb provider allows you to access any OLE DB-
This comprehensive text starts with a brief overview of the
compliant data store,C# languagebut incursandoverheadthen quicklyvia themovesCOMto interoperabilitykey technical andlayer lurking in the background.
architectural issues for .NET developers.
When you know that the data source you need to manipulate is MS SQL Server, you will find performance gains if you use the System.Data.SqlClient namespace directly. Collectively, these classes constitute the
Tablefunctionalityof Contentsof the SQL data provider, which should look very familiar, given your work with the OleDb
C#providerand the(Table.NET Platform,17-18). Second Edition
Introduction
PartTableOne17- -Introducing18: Core TypesC# andofthethe.NETSystemPlatform.Data.SqlClient Namespace
|
Chapter 1 - The Philosophy of .NET |
|
Meaning in Life |
|
|
|
System.Data.SqlClient |
|
|
||
|
Chapter 2 - Building C# Applications |
|
|
|
|
|
Type |
|
|
|
|
Part Two - The C# Programming Language |
|
||||
|
|||||
ChapterSqlCommand3 - C# Language FundamentalsRepresents a Transact-SQL query to execute at a SQL Server |
|
||||
Chapter 4 - Object-Oriented Programmingdata sourcewith C#. |
|
||||
|
|
|
|
|
|
|
Chapter 5 |
- Exceptions and Object |
|
Lifetime |
|
|
SqlConnection |
|
Represents an open connection to a SQL Server data source. |
|
|
|
Chapter 6 |
- Interfaces and Collections |
|
|
|
|
|
|
|
||
|
ChapterSqlDataAdapter7 - Callback Interfaces, Delegates,Representsand Eventsa set of data commands and a database |
|
|||
|
Chapter 8 |
- Advanced C# Type ConstructionconnectionTechniquesused to fill the DataSet and update the SQL Server |
|
||
Part Three - Programming with .NET |
|
Assembliesdata source. |
|
||
|
|
|
|
|
|
Chapter 9 - Understanding .NET Assemblies
SqlDataReader Provides a way of reading a forward-only stream of data
Chapter 10 - Processes, AppDomains, Contexts, and Threads
records from a SQL Server data source.
|
|
|
|
|
|
Chapter 11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
||
|
SqlErrors |
|
SqlErrors maintains a collection of warnings or errors returned |
|
Part Four - Leveraging the .NET Libraries |
||||
|
|
|
|
by SQL Server, each of which is represented by a SQLError |
ChapterSqlError12 - Object Serialization and the .NET Remoting Layer |
||||
Chapter 13 |
|
|
type. When an error is encountered, an exception of type |
|
- Building a Better Window (Introducing Windows Forms) |
||||
|
SqlException |
|
SQLException is thrown. |
|
|
Chapter 14 |
- A Better Painting Framework (GDI+) |
ChapterSqlParameterCollection15 - Programming with WindowsSqlParametersCollectionForms Controls holds onto the parameters sent to a
Chapter 16 - The System.IO Namespacestored procedure held in the database. Each parameter is of SqlParameter
Chapter 17 - Data Access with ADO.NETtype SQLParameter.
Part Five - Web Applications and XML Web Services
ChapterGiven that18 -workingASP.NETwithWebthesePagestypesand isWebalmostC ntrolsidentical to working with the OleDb data provider, you should
already know what to do with them, as they have a similar public interface. To help you get comfortable
Chapter 19 - ASP.NET Web Applications
with this new set of types, the remainder of the examples use the SQL data provider (don't forget to specify
Chapter 20 - XML Web Services
you are using the System.Data.SqlClient namespace!)
Index
List of Figures
ListTheof TablesSystem.Data.SqlTypes Namespace
On a quick related note, when you use the SQL data provider, you also have the luxury of using a number of managed types that represent native SQL server data types. Table 17-19 gives a quick rundown.
Table 17-19: Types of the System.Data.SqlTypes Namespace

|
System.Data.C#SqlTypesand the .NETWrapperPlatform, |
|
NativeSecond EditionSQL Server |
||
|
|
|
|
|
|
|
SqlBinary |
by Andrew Troelsen |
|
|
ISBN:1590590554 |
|
Apress © 2003 (1200 pages) |
|
binary, varbinary, timestamp, image |
||
|
|
|
|
|
|
|
SqlInt64 |
This comprehensive text startsbigintwith a brief overview of the |
|||
|
|
|
|
||
|
SqlBit |
C# language and then quickly |
moves to key technical and |
||
|
architectural issues for .NET |
developersbit |
. |
||
|
|
|
|
|
|
|
SqlDateTime |
|
|
datetime, smalldatetime |
|
|
|
|
|
|
|
Table of Contents |
|
|
decimal |
|
|
|
SqlNumeric |
|
|
|
|
|
|
|
|
|
|
C# and the .NET Platform, Second Edition |
|
float |
|
||
|
SqlDouble |
|
|
|
|
|
Introduction |
|
|
|
|
|
|
|
|
||
Part One - Introducing C# and the .NET Platform |
int |
|
|||
|
SqlInt32 |
|
|
|
|
|
|
|
|
|
|
|
Chapter 1 - The Philosophy of .NET |
|
money, smallmoney |
||
|
SqlMoney |
|
|
||
|
Chapter 2 - Building C# Applications |
|
|
|
|
|
|
|
|
||
Part Two - The C# Programming Language |
|
nchar, ntext, nvarchar, sysname, text, varchar, char |
|||
|
SqlString |
|
|
||
|
|
|
|
|
|
|
Chapter 3 - C# Language Fundamentals |
|
numeric |
|
|
|
SqlNumeric |
|
|
|
|
|
Chapter 4 - Object-Oriented Programming with |
C# |
|
||
|
Chapter 5 - Exceptions and Object Lifetime |
|
real |
|
|
|
SqlSingle |
|
|
|
|
|
|
|
|
|
|
|
Chapter 6 - Interfaces and Collections |
|
smallint |
|
|
|
SqlInt16 |
|
|
|
|
|
Chapter 7 - Callback Interfaces, Delegates, and Events |
|
|||
|
System.Object |
|
|
sql variant |
|
|
Chapter 8 - Advanced C# Type Construction |
|
Techniques |
|
|
|
|
|
|
|
|
Part Three - Programming with .NET Assemblies |
|
|
|||
|
SqlByte |
|
|
tinyint |
|
Chapter 9 - Understanding .NET Assemblies
ChapterSqlGuid10 - Processes, AppDomains, Contexts,uniqueidentifiera Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Inserting Records Using the SqlDataAdapter
Chapter 13 - Building a Better Window (Introducing Windows Forms)
ChapterNow that14you- A haveBett rflippedPaintingfromFrameworkthe OleDb(GDI+)provider to the realm of the SQL provider, you can return to the
Chaptertask of15understanding- ProgrammingthewithroleWindowsof data adaptersForms Controls. Let's examine how to insert new records into a given table
Chapterusing the16SqlDataAdapter- The System.IO (whichNamespaceis nearly identical to using the OleDbDataAdapter, OdbcDataAdapter, Chapterand OracleDataAdapter17 - Access withtypes)ADO. As.NETalways, begin by creating an active connection, as shown here:
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
public class MySqlDataAdapter
Chapter{ 19 - ASP.NET Web Applications
Chapter public20 - XML WebstaticServicesvoid Main()
Index {
List of Figures
List of Tables
}
}
// Create a connection and adapter (with select command).
SqlConnection cn = new
SqlConnection("server=(local);User ID=sa;Pwd=;database=Cars");
SqlDataAdapter dAdapt = new
SqlDataAdapter("SELECT * FROM Inventory", cn);
// Kill record inserted on the last run of this app.
cn.Open();
SqlCommand killCmd = new
SqlCommand("Delete from Inventory where CarID = '1111'", cn);
killCmd.ExecuteNonQuery();
cn.Close();
You can see that the connection string has been cleaned up quite a bit. In particular, notice that you do not need to define a Provider segment (as the SQL data provider only talks to SQL server!). Next, note that you create a new SqlDataAdapter and specify the value of the SelectCommand property as a constructor
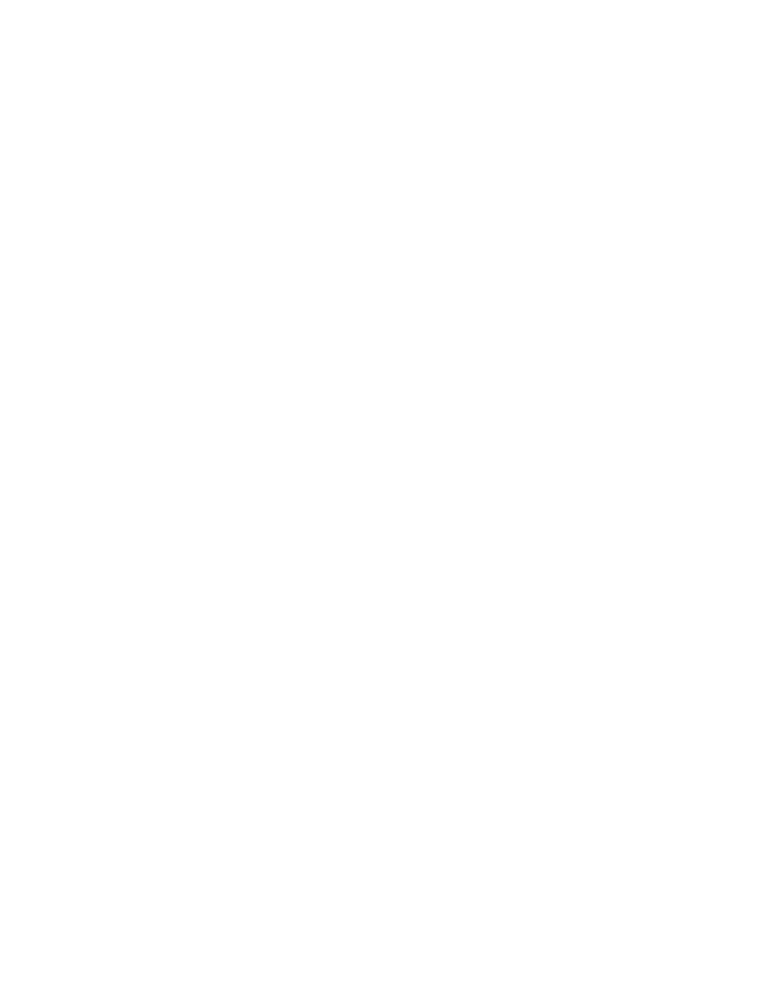
Chapter 1 - The Philosophy of .NET
{
Chapter 2 - Building C# Applications
...
Part Two - The C# Programming Language
// Build the insert command!
Chapter 3 - C# Language Fundamentals
dAdapt.InsertCommand = new SqlCommand("INSERT INTO Inventory" +
Chapter 4 - Object"(CarID,-OrientedMake,ProgrammingColor,withPetName)C# VALUES" +
Chapter 5 - Exceptions"(@CarID,and Object@Make,Lifetime@Color, @PetName)", cn)";
Chapter SqlParameter6 - Interfaces andworkParamCollections = null;
// CarID.
Chapter 7 - Callback Interfaces, Delegates, and Events
workParam = dAdapt.InsertCommand.Parameters.Add(new
Chapter 8 - Advanced C# Type Construction Techniques
SqlParameter("@CarID", SqlDbType.Int));
Part Three - Programming with .NET Assemblies
workParam.SourceColumn = "CarID";
Chapter 9 - Understanding .NET Assemblies
workParam.SourceVersion = DataRowVersion.Current;
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Make.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
workParam = dAdapt.InsertCommand.Parameters.Add(new
Part Four - Leveraging the .NET Libraries
SqlParameter("@Make", SqlDbType.VarChar));
Chapter 12 - Object Serialization and the .NET Remoting Layer workParam.SourceColumn = "Make";
Chapter 13 - Building a Better Window (Introducing Windows Forms) workParam.SourceVersion = DataRowVersion.Current;
Chapter 14 - A Better Painting Framework (GDI+)
// Color.
Chapter 15 - Programming with Windows Forms Controls
workParam = dAdapt.InsertCommand.Parameters.Add(new
Chapter 16 - The System.IO Namespace
SqlParameter("@Color", SqlDbType.VarChar));
Chapter 17 - Data Access with ADO.NET workParam.SourceColumn = "Color";
Part Five - Web Applications and XML Web Services
workParam.SourceVersion = DataRowVersion.Current;
Chapter 18 - ASP.NET Web Pages and Web Controls
// PetName.
Chapter 19 - ASP.NET Web Applications
workParam = dAdapt.InsertCommand.Parameters.Add(new
Chapter 20 - XMLSqlParameter("@PetName",Web Services SqlDbType.VarChar));
Index workParam.SourceColumn = "PetName";
List of FiguresworkParam.SourceVersion = DataRowVersion.Current;
}
List of Tables
Now that you have formatted each of the parameters, the final step is to fill the DataSet and add your new row (note that the PrintTable() helper function has carried over to this example):
public static void Main()
{
...
// Fill data set with initial data.
DataSet myDS = new DataSet();
dAdapt.Fill(myDS, "Inventory");
PrintTable(myDS);
// Add new row to the DataTable.
DataRow newRow = myDS.Tables["Inventory"].NewRow();
newRow["CarID"] = 1111;
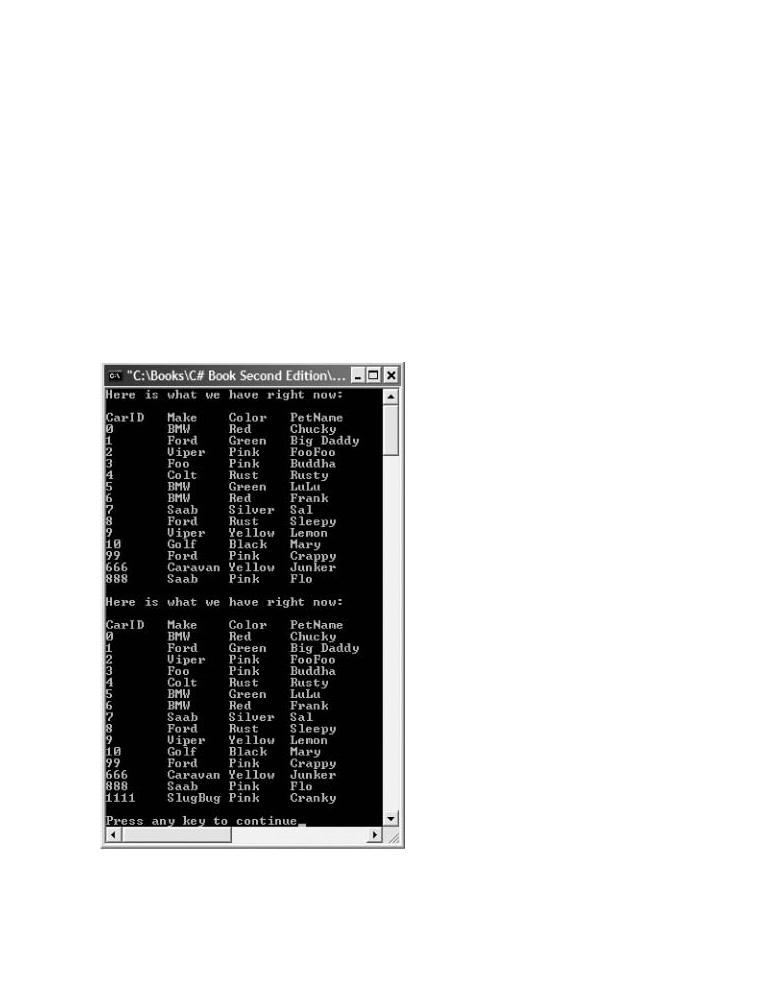
newRow["Make"] = "SlugBug";
C# and the .NET Platform, Second Edition newRow["Color"] = "Pink";
by Andrew Troelsen |
ISBN:1590590554 |
newRow["PetName"] = "Cranky"; |
|
Apress © 2003 (1200 pages)
myDS.Tables["Inventory"].Rows.Add(newRow);
This comprehensive text starts with a brief overview of the
// Send back to database and reprint.
C# language and then quickly moves to key technical and
try
architectural issues for .NET developers.
{
dAdapt.Update(myDS, "Inventory");
myDS.Dispose();
Table of Contents
myDS = new DataSet();
C# and the .NET Platform, Second Edition dAdapt.Fill(myDS, "Inventory");
Introduction
PrintTable(myDS);
Part One - Introducing C# and the .NET Platform
}
Chapter 1 - The Philosophy of .NET
catch(Exception e){ Console.Write(e.ToString()); }
Chapter 2 - Building C# Applications
}
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
ChapterWhen you4 -runObjectthe-application,Oriented Programmingyou see thewithoutputC# shown in Figure 17-24.
Chapter 5 - Exceptions and Object Lifetime
Chapter
Chapter
Chapter
Part
Chapter |
|
Chapter |
|
Chapter |
Programming |
Part |
|
Chapter |
|
Chapter |
Forms) |
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
|
Index |
|
List of |
|
List of |
|
Figure 17-24: Inserting new records using a data adapter
SOURCE The InsertRowsWithSqlAdapter project can be found under the Chapter 17
CODE subdirectory.

Updating Existing Records Using the SqlDataAdapter
C# and the .NET Platform, Second Edition
by Andrew Troelsen ISBN:1590590554
Now that you can insert new rows, let's look at how you can update existing rows, which you might guess
will look quite similar to the process of inserting new rows (as well as the process of deleting existing
This comprehensive text starts with a brief overview of the
rows). Again, start the process by obtaining a connection and creating a new SqlDataAdapter. Next, set
the value of the UpdateCommand property, using the same general approach as when setting the value of architectural issues for .NET developers.
the InsertCommand. Here is the relevant code in Main():
Table of Constaticents
public void Main()
C#{ and the .NET Platform, Second Edition
Introduction// Create a connection and adapter (same as previous code).
Part One...- Introducing C# and the .NET Platform
Chapter //1 -EstablishThe Philo ophytheof .NETUpdateCommand.
dAdapt.UpdateCommand = new SqlCommand
Chapter 2 - Building C# Applications
("UPDATE Inventory SET Make = @Make, Color = " +
Part Two - The C# Programming Language
"@Color, PetName = @PetName " +
Chapter 3 - C# Language Fundamentals
"WHERE CarID = @CarID" , cn);
Chapter 4 - Object-Oriented Programming with C#
// Build parameters for each column in Inventory table.
Chapter 5 - Exceptions and Object Lifetime
// Same as before, but now you are populating the ParameterCollection
Chapter 6 - Interfaces and Collections
// of the UpdateCommand:
Chapter 7 - Callback Interfaces, Delegates, and Events
SqlParameter workParam = null;
Chapter 8 - Advanced C# Type Construction Techniques
workParam = dAdapt.UpdateCommand.Parameters.Add(new
Part Three - Programming with .NET Assemblies
SqlParameter("@CarID", SqlDbType.Int));
Chapter 9 - Understanding .NET Assemblies workParam.SourceColumn = "CarID";
Chapter 10 - Processes, AppDomains, Contexts, and Threads workParam.SourceVersion = DataRowVersion.Current;
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
// Do the same for PetName, Make, and Color params
Part Four - Leveraging the .NET Libraries
...
Chapter 12 - Object Serialization and the .NET Remoting Layer
// Fill initial data set.
Chapter 13 - Building a Better Window (Introducing Windows Forms)
DataSet myDS = new DataSet();
Chapter dAdapt14 - Better Painting Framework (GDI+)
.Fill(myDS, "Inventory");
Chapter PrintTable(myDS);15 - Programming with Windows Forms Controls
Chapter //16 -ChangeThe Systemcolumns.IO Namespacein second row to 'FooFoo'.
Chapter DataRow17 - AchangeRowcess with ADO=.NETmyDS.Tables["Inventory"].Rows[1];
Part FivechangeRow["Make"]- Web Applications and XML= "FooFoo";Web Services
changeRow["Color"] = "FooFoo";
Chapter 18 - ASP.NET Web Pages and Web Controls
changeRow["PetName"] = "FooFoo";
Chapter 19 - ASP.NET Web Applications
// Send back to database and reprint.
Chapter 20 - XML Web Services
Index try
{
List of Figures
dAdapt.Update(myDS, "Inventory");
List of Tables
myDS.Dispose();
myDS = new DataSet(); dAdapt.Fill(myDS, "Inventory"); PrintTable(myDS);
}
catch(Exception e)
{ Console.Write(e.ToString()); }
}
Figure 17-25 shows the output.
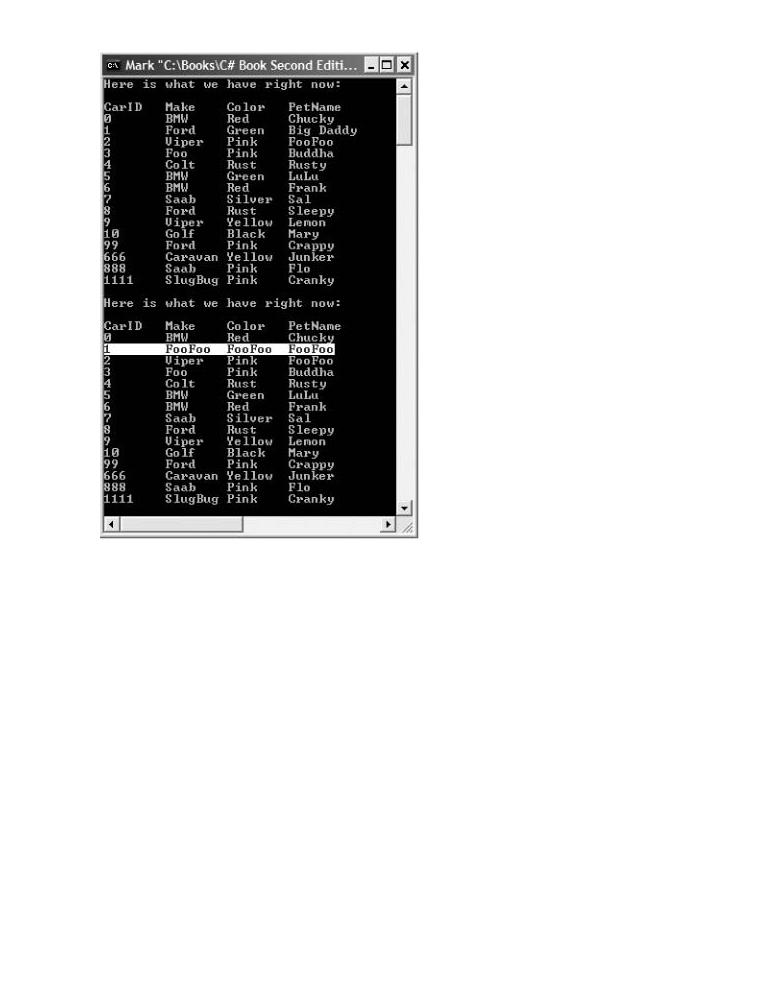
ISBN:1590590554
|
overview of the |
|
technical and |
Table |
|
C# and |
|
Part |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
Programming |
Part |
|
Chapter |
|
Chapter |
Forms) |
Chapter |
|
ChapterFigure15 - 17Programming-25: UpdatingwithexistingWindowsrecordsFormsusingControlsa data adapter
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
As you may be guessing, if you wish to inform a data adapter how it should handle rows marked as deleted, you need to build yet another command type and assign it to the data adapter's DeleteCommand
property. While having absolute control over the SQL used to insert, update, and delete records does
Chapter 19 - ASP.NET Web Applications
allow a great deal of flexibility, you may agree that it can be a bit of a bother to constantly build verbose
Chapter 20 - XML Web Services
SQL commands by hand. You are, however, provided with some helper types.
Index
List ofSOURCEFigures The UpdateRowsWithSqlAdapter project is found under the Chapter 17 subdirectory.
List ofCODETables
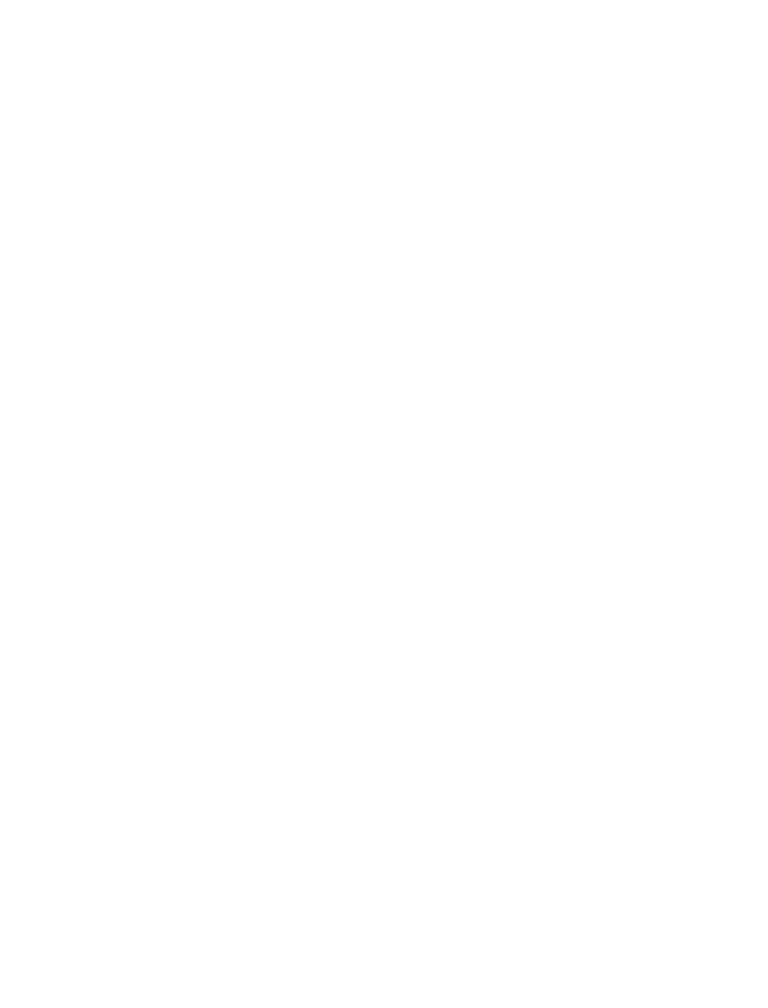
Auto-GeneratingC# a d theSQL.NETCommandsPlatfor , SecondUsingEdition CommandBuilder Types
by Andrew Troelsen |
ISBN:1590590554 |
Each ADO.NET data provider that ships with .NET 1.1 provides a command builder type. Using this type,
Apress © 2003 (1200 pages)
you are able to automatically obtain command objects that contain the correct INSERT, DELETE, and
This comprehensive text starts with a brief overview of the
UPDATE commandC# languagetypes basedandonthenthequicklyinitial SELECTmoves to statementkey tech ical. Forandexample, the SqlCommandBuilder automatically generatesarchitecturalthe valuesissues containedf r .NET eveloperswithin the. SqlDataAdapter's InsertCommand, UpdateCommand,and DeleteCommand properties based on the initial SelectCommand. The obvious benefit is that you have no need to build all the SqlCommand and SqlParameter types by hand.
Table of Contents
C#Anandobviousthe .NETquestionPlatform,at thisSecondpointEditiis hown a command builder is able to build these SQL commands on the
fly. The short answer is metadata. At runtime, when you call the Update() method of a data adapter, the
Introduction
related command builder will read the database's schema data to autogenerate the underlying INSERT,
Part One - Introducing C# and the .NET Platform
DELETE, and UPDATE logic. If you are interested in seeing these SQL statements first-hand, you may call
Chapter 1 - The Philosophy of .NET
the SqlCommandBuilder.GetInsertCommand(), GetUpdateCommand(), and GetDeleteCommand()
Chapter 2 - Building C# Applications
methods.
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Consider the following example, which deletes a row in a DataSet using the auto-generated SQL
Chapter 4 - Object-Oriented Programming with C#
statements. Furthermore, this application will print out the underlying command text of each command
Chapter 5 - Exceptions and Object Lifetime object:
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events static void Main(string[] args)
Chapter 8 - Advanced C# Type Construction Techniques
{
Part Three - Programming with .NET Assemblies
DataSet theCarsInventory = new DataSet();
Chapter 9 - Understanding .NET Assemblies
// Make connection.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
SqlConnection cn = new
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
SqlConnection("server=(local);User ID=sa;Pwd=;database=Cars");
Part Four - Leveraging the .NET Libraries
// Autogenerate INSERT, UPDATE, and DELETE commands
Chapter 12 - Object Serializaexitingion and the .NET Remoting Layer
// based on SELECT command.
ChapterSqlDataAdapter13 - Building BetterdaWindow= new(IntroducingSqlDataAdapter("SELECTWindows Forms) * FROM Inventory", cn);
ChapterSqlCommandBuilder14 - A Better Pa nting FrameworkinvBuilder(GDI+)= new SqlCommandBuilder(da);
Chapter//15Print- Programmingout valueswith Windowsof theFormsgeneratedControls command objects.
Console.WriteLine("SELECT command: {0}",
Chapter 16 - The System.IO Namespace
da.SelectCommand.CommandText);
Chapter 17 - Data Access with ADO.NET
Console.WriteLine("UPDATE command: {0}",
Part Five - Web Applications and XML Web Services
invBuilder.GetUpdateCommand().CommandText);
Chapter 18 - ASP.NET Web Pages and Web Controls
Console.WriteLine("INSERT command: {0}",
Chapter 19 - ASP.NET Web Applications
invBuilder.GetInsertCommand().CommandText);
Chapter 20 - XML Web Services
Console.WriteLine("DELETE command: {0}",
Index
invBuilder.GetDeleteCommand().CommandText);
List of Figures
// Fill data set.
List of Tables
da.Fill(theCarsInventory, "Inventory"); PrintTable(theCarsInventory);
// Delete a row and update database.
try
{
theCarsInventory.Tables["Inventory"].Rows[6].Delete(); da.Update(theCarsInventory, "Inventory");
}
catch(Exception e)
{
Console.WriteLine(e.Message);
}
// Refill and reprint Inventory table.
theCarsInventory = new DataSet();
da.Fill(theCarsInventory, "Inventory");
PrintTable(theCarsInventory);
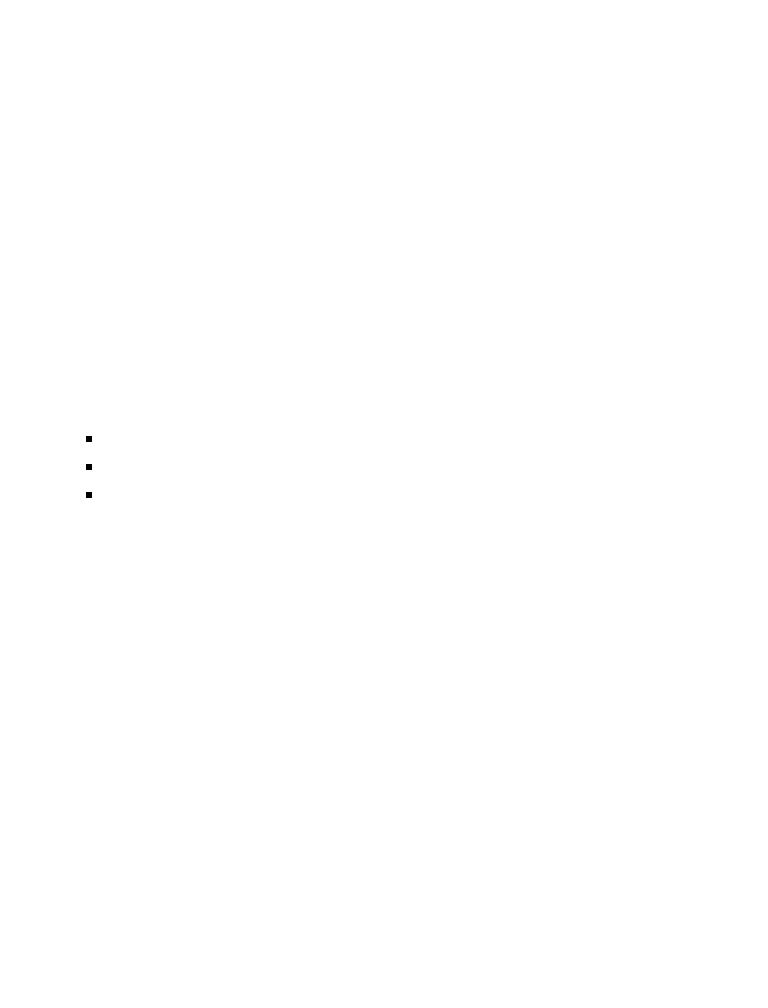
}
C# and the .NET Platform, Second Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages) |
|
Based on the initialThisSQLcomprehensiveSELECT statement,text startsthewithSqlCommandBuildera brief overview of thetype generates the following UPDATE, INSERT,C#andlanguageDELETEand parameterizedthen quickly movesqueries:to key technical and
architectural issues for .NET developers.
UPDATE Inventory SET CarID = @p1 , Make = @p2 , Color = @p3 , PetName = @p4
WHERE ( (CarID = @p5) AND
Table of Contents
((@p6 = 1 AND Make IS NULL) OR (Make = @p7)) AND
C# and the .NET Platform, Second Edition
((@p8 =1 AND Color IS NULL) OR (Color = @p9)) AND
Introduction
((@p10 = 1 AND PetName IS NULL) OR (PetName = @p11)) )
INSERT INTO Inventory( CarID , Make , Color , PetName ) VALUES
Chapter 1 - The Philosophy of .NET
( @p1 , @p2 , @p3 , @p4 )
Chapter 2 - Building C# Applications
DELETE FROM Inventory WHERE ( (CarID = @p1) AND
((@p2 = 1 AND Make IS NULL) OR (Make = @p3)) AND
Chapter 3 - C# Language Fundamentals
((@p4 = 1 AND Color IS NULL) OR (Color = @p5)) AND
Chapter 4 - Object-Oriented Programming with C#
((@p6 = 1 AND PetName IS NULL) OR (PetName = @p7)) )
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
ChapterNow, while7 - youCallbackmayInterfaces,love the ideaDelegates,of gettingandsomethingEv nts for nothing, do understand that command builders
do come with some very critical restrictions. Specifically, a command builder is only able to auto-generate
Chapter 8 - Advanced C# Type Construction Techniques
SQL commands for use by a data adapter if all of the following conditions are true:
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
The SELECT command only interacts with a single table (e.g., no joins).
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
The single table has been attributed with a primary key.
Part Four - Leveraging the .NET Libraries
The column(s) representing the primary key is accounted for in your SQL SELECT statement.
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
To wrap up our examination of the raw details of ADO.NET, allow me to provide two Windows Forms
Chapter 14 - A Better Painting Framework (GDI+)
applications that tie together the topics discussed thus far. After this point, I'll wrap up with an overview of
Chapter 15 - Programming with Windows Forms Controls
the data-centric tools provided by VS .NET and discuss strongly typed DataSets.
Chapter 16 - The System.IO Namespace
ChapterSOURCE17 - Data AccessThewithMySqlCommandBuilderADO.NET project is found under the Chapter 17 subdirectory.
Part FiveCODE- Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

A CompleteC#ADOand the.NET.NETWindowsPlatform, SecondFormsEditionExample
by Andrew Troelsen |
ISBN:1590590554 |
Now assume you have a new Windows Forms example that allows the user to edit the values in a
Apress © 2003 (1200 pages)
DataGrid (e.g., add new records, and update and delete existing records). When finished, the user may
This comprehensive text starts with a brief overview of the
submit the modifiedC# DataSetlanguagebackand thento thequicklydatabasemovesusingto keya Buttontechnicaltypeand. First, assume the following constructor logic:architectural issues for .NET developers.
public class mainForm : System.Windows.Forms.Form
Table of Contents
{
C# and the .NET Platform, Second Edition private SqlConnection cn = new
Introduction
SqlConnection("server=(local);uid=sa;pwd=;database=Cars");
Part One - Introducing C# and the .NET Platform
private SqlDataAdapter dAdapt;
Chapter 1 - The Philosophy of .NET
private SqlCommandBuilder invBuilder;
Chapter 2 - Building C# Applications
private DataSet myDS = new DataSet();
Part Two - The C# Programming Language
private System.Windows.Forms.DataGrid dataGrid1;
Chapter 3 - C# Language Fundamentals
private System.Windows.Forms.Button btnUpdateData;
Chapter 4 - Object-Oriented Programming with C#
...
Chapter public5 - Ex eptionsmainForm()and Object Lifetime
Chapter {6 - Interfaces and Collections
Chapter 7 - CallbackInitializeComponent();Interfaces, Delegates, and Events
// Create the initial SELECT SQL statement.
Chapter 8 - Advanced C# Type Construction Techniques
dAdapt = new SqlDataAdapter("SELECT * FROM Inventory", cn);
Part Three - Programming with .NET Assemblies
// Autogenerate the INSERT, UPDATE,
Chapter 9 - Understanding .NET Assemblies
// and DELETE statements.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
invBuilder = new SqlCommandBuilder(dAdapt);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
// Fill and bind.
Part Four - Leveraging the .NET Libraries
dAdapt.Fill(myDS, "Inventory");
Chapter 12 - Object Serialization and the .NET Remoting Layer
dataGrid1.DataSource = myDS.Tables["Inventory"].DefaultView;
Chapter 13 - Building a Better Window (Introducing Windows Forms)
}
Chapter 14 - A Better Painting Framework (GDI+)
...
Chapter 15 - Programming with Windows Forms Controls
}
Chapter 16 - The System.IO Namespace Chapter 17 - Data Access with ADO.NET
PartAt thisF vepoint,- WebtheApplicationsSqlDataAdapterand XMLhasWeball theServicesinformation it needs to submit changes back to the data store.
ChapterNow assume18 - ASPthat.NETyouWebhavePagesthe followingand Web Clogicntrolsbehind the Button's Click event:
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
private void btnUpdateData_Click(object sender, System.EventArgs e)
Index
{
List of Figurestry
List of Tables{
dataGrid1.Refresh(); dAdapt.Update(myDS, "Inventory");
}
catch(Exception ex)
{
MessageBox.Show(ex.Message);
}
}
As usual, you call Update() and specify the table within the DataSet you wish to update. If you take this out for a test run, you see something like Figure 17-26 (be sure you exit out of edit mode on the DataTable before you submit your results!).

Edition
ISBN:1590590554
a brief overview of the
to key technical and
.
Table
C# and
Introduction
Figure 17-26: All good things do come ...
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
SOURCE The WinFormsExample project is included under the Chapter 17 subdirectory.
Chapter 2 - Building C# Applications
CODE
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
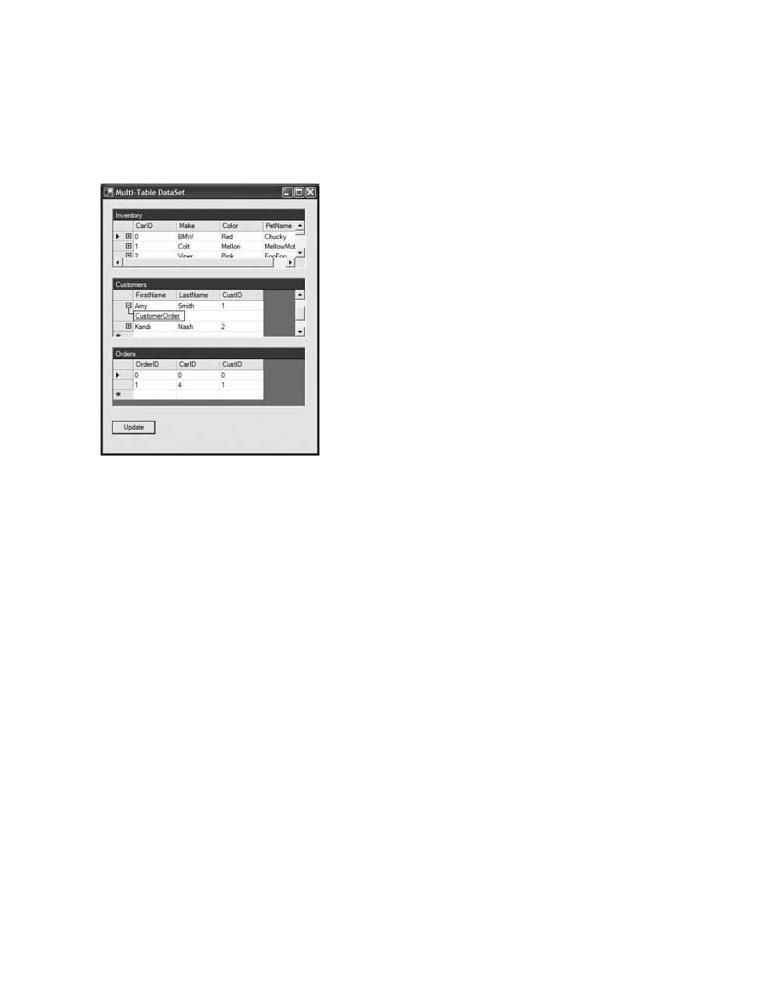
Filling a MultitabledC# and the .NETDataSetPl tform,(andSecondAddingE ition DataRelations)
by Andrew Troelsen |
ISBN:1590590554 |
Let's come full circle and build an additional Windows Forms example that mimics the application you
Apress © 2003 (1200 pages)
created during the first half of this chapter. The GUI is simple enough. In Figure 17-27 you can see three
This comprehensive text starts with a brief overview of the
DataGrid types thatC#holdlanguagethe datand retrievedthen quicklyfromovthesInventory,to key technicalOrders,andand Customers tables of the Cars database. In addition,architecturalthe singleissuesButtonf r .pushesNET developersany and. all changes back to the data store.
Table
C# and
Part
Chapter
Chapter
Part
Chapter |
|
Chapter |
with C# |
Chapter |
|
Chapter |
|
Chapter |
and Events |
Chapter |
Techniques |
Part |
|
Chapter |
|
Chapter |
and Threads |
ChapterFigure11 - 17Type-27:ReflViewingction,relatedLate Binding,DataTablesand Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
To keep things even simpler, you will use auto-generated commands for each of the three
Chapter 13 - Building a Better Window (Introducing Windows Forms)
SqlDataAdapters (one for each table). First, here is the Form's state data:
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
public class mainForm : System.Windows.Forms.Form
Chapter 16 - The System.IO Namespace
{
Chapter 17 - Data Access with ADO.NET
private System.Windows.Forms.DataGrid custGrid;
Part Five - Web Applications and XML Web Services
private System.Windows.Forms.DataGrid inventoryGrid;
Chapter 18 - ASP.NET Web Pages and Web Controls
private System.Windows.Forms.Button btnUpdate;
Chapter 19 - ASP.NET Web Applications
private System.Windows.Forms.DataGrid OrdersGrid;
Chapter 20 - XML Web Services
...
Index // Here is the connection.
List of Figures
private SqlConnection cn = new
List of Tables |
SqlConnection("server=(local);uid=sa;pwd=;database=Cars"); |
|
// Our data adapters (for each table).
private SqlDataAdapter invTableAdapter;
private SqlDataAdapter custTableAdapter;
private SqlDataAdapter ordersTableAdapter;
// Command builders (for each table).
private SqlCommandBuilder invBuilder = new SqlCommandBuilder(); private SqlCommandBuilder orderBuilder = new SqlCommandBuilder(); private SqlCommandBuilder custBuilder = new SqlCommandBuilder();
// The dataset.
DataSet carsDS = new DataSet();
...
}

The Form's constructor does the grunge work of creating your data-centric member variables and filling
C# and the .NET Platform, Second Edition
the DataSet. Also note that there is a call to a private helper function, BuildTableRelationship(), as shown
here: by Andrew Troelsen ISBN:1590590554
Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the
public mainForm()
C# language and then quickly moves to key technical and
{architectural issues for .NET developers.
InitializeComponent();
Table of //ContentsCreate adapters.
invTableAdapter = new SqlDataAdapter("Select * from Inventory", cn);
C# and the .NET Platform, Second Edition
custTableAdapter = new SqlDataAdapter("Select * from Customers", cn);
Introduction
ordersTableAdapter = new SqlDataAdapter("Select * from Orders", cn);
Part One - Introducing C# and the .NET Platform
// Autogenerate commands.
Chapter 1 - The Philosophy of .NET
invBuilder = new SqlCommandBuilder(invTableAdapter);
Chapter 2 - Building C# Applications
orderBuilder = new SqlCommandBuilder(ordersTableAdapter);
Part Two - The C# Programming Language
custBuilder = new SqlCommandBuilder(custTableAdapter);
Chapter 3 - C# Language Fundamentals
// Add tables to DS.
Chapter 4 - Object-Oriented Programming with C# invTableAdapter.Fill(carsDS, "Inventory");
Chapter 5 - Exceptions and Object Lifetime custTableAdapter.Fill(carsDS, "Customers");
Chapter 6 - Interfaces and Collections ordersTableAdapter.Fill(carsDS, "Orders");
Chapter 7 - Callback Interfaces, Delegates, and Events
// Build relations between tables.
Chapter 8 - Advanced C# Type Construction Techniques
BuildTableRelationship();
Part Three - Programming with .NET Assemblies
}
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
ChapterThe BuildTableRelationship()11 - Type Reflection, LatehelperBinding,functionand Attributedoes just-BasedwhatProgrammingyou would expect. Recall that the Cars PartdatabaseFour - Leveragingexpresses athenumber.NET Librariesof parent/child relationships. The code looks identical to the logic seen
earlier in this chapter, as shown here:
Chapt r 12 - Object Serialization and the .NET Remoting Layer Chapter 13 - Building a Better Window (Introducing Windows Forms) Chapterprivate14 -voidA BetterBuildTableRPainting Frameworklationship()(GDI+)
Chapter{ 15 - Programming with Windows Forms Controls
// Create a DR obj.
Chapter 16 - The System.IO Namespace
DataRelation dr = new DataRelation("CustomerOrder",
Chapter 17 - Data Access with ADO.NET
carsDS.Tables["Customers"].Columns["CustID"],
Part Five - Web Applications and XML Web Services
carsDS.Tables["Orders"].Columns["CustID"]);
Chapter 18 - ASP.NET Web Pages and Web Controls
// Add relation to the DataSet.
Chapter 19 - ASP.NET Web Applications
carsDS.Relations.Add(dr);
Chapter 20 - XML Web Services
// Create another DR obj.
Index
dr = new DataRelation("InventoryOrder",
List of Figures
carsDS.Tables["Inventory"].Columns["CarID"],
List of Tables
carsDS.Tables["Orders"].Columns["CarID"]);
// Add relation to the DataSet.
carsDS.Relations.Add(dr);
// Fill the grids!
inventoryGrid.SetDataBinding(carsDS, "Inventory");
custGrid.SetDataBinding(carsDS, "Customers");
OrdersGrid.SetDataBinding(carsDS, "Orders");
}
Now that the DataSet has been filled and disconnected from the data source, you can manipulate each table locally. To do so, simply insert, update, or delete values from any of the three DataGrids. When you are ready to submit the data back for processing, click the Form's Update button. The code behind the Click event should be clear at this point, as shown here:
private void btnUpdate_Click(object sender, System.EventArgs e)
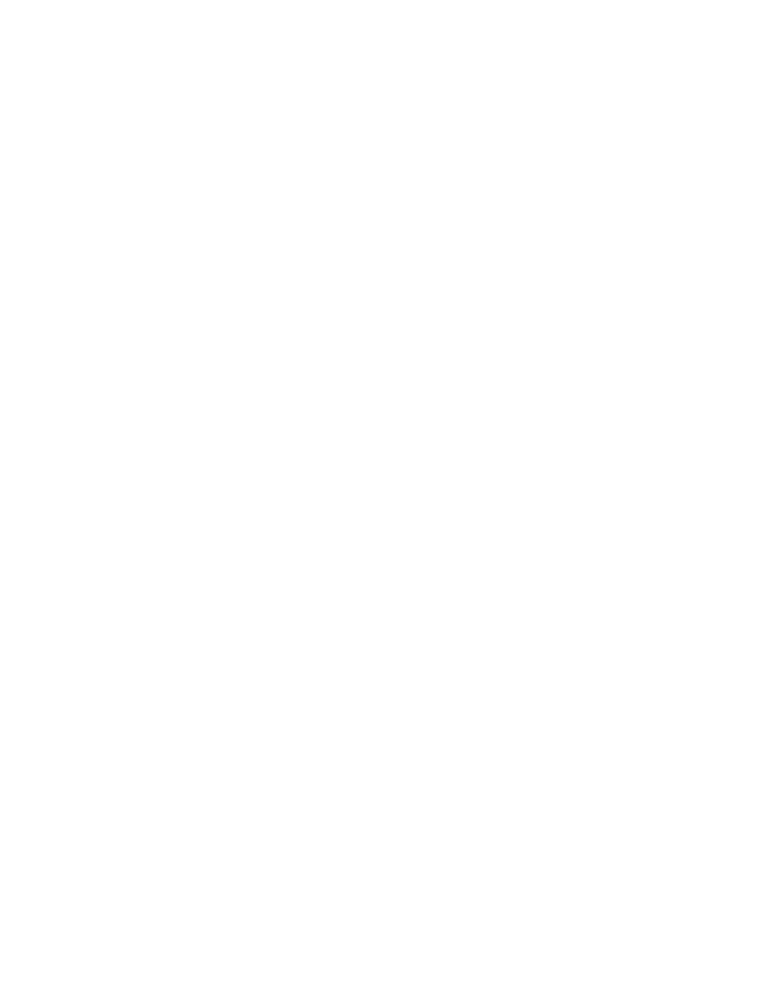
{
try |
C# and the .NET Platform, Second Edition |
|
|
by Andrew Troelsen |
ISBN:1590590554 |
||
{ |
|||
|
|
Apress © 2003 (1200 pages)
invTableAdapter.Update(carsDS, "Inventory");
This comprehensive text starts with a brief overview of the custTableAdapter.Update(carsDS, "Customers");
C# language and then quickly moves to key technical and ordersTableAdapter.Update(carsDS, "Orders");
architectural issues for .NET developers.
}
catch(Exception ex)
{
Table of Contents
MessageBox.Show(ex.Message);
C# and the .NET Platform, Second Edition
}
Introduction
}
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET |
|
|
Chapt r 2 |
- Building C# Applications |
|
Once you update, you will find each table in the Cars database correctly altered. |
||
Part Two - The C# Programming Language |
|
|
ChapterSOURCE3 - C# LanguageThe WinFormsMultiTableDataSetFundamentals |
project is included under the Chapter 17 |
|
ChapterCODE4 - Object-Orientedsubdirectory.Programming with C# |
|
|
Chapter 5 - Exceptions and Object Lifetime |
|
|
Chapter 6 |
- Interfaces and Collections |
|
Chapter 7 |
- Callback Interfaces, Delegates, and Events |
|
Chapter 8 |
- Advanced C# Type Construction Techniques |
|
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
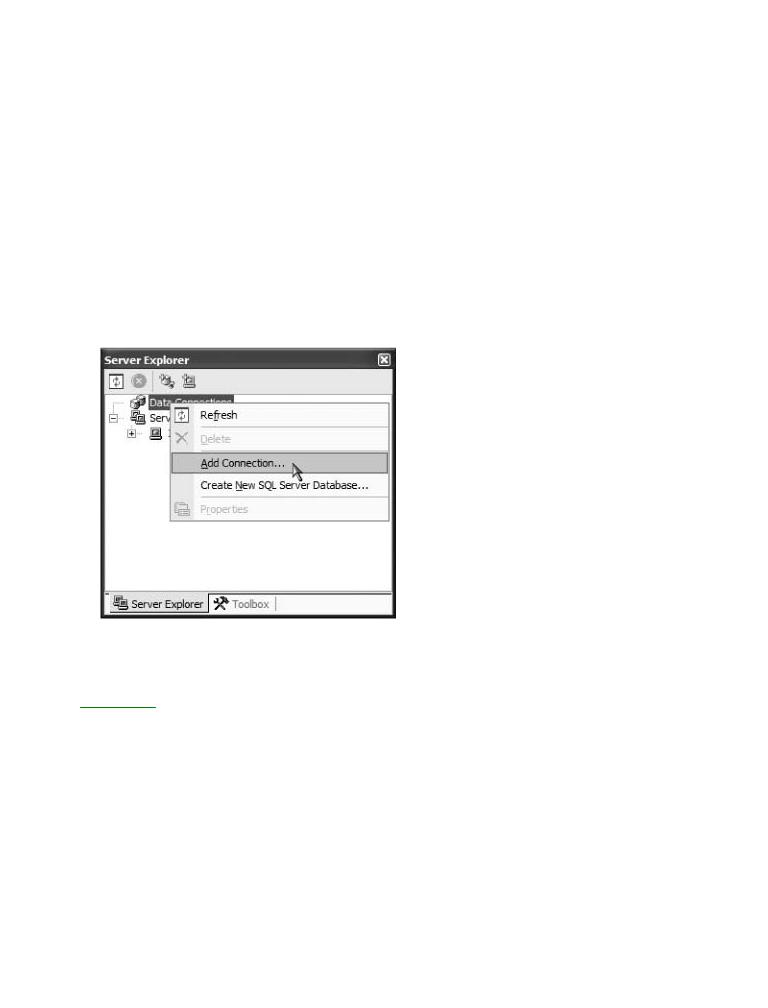
Bring In theC#Wizards!and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
To wrap up this chapter, I'd like to offer a guided tour of select integrated data-centric wizards provided by VS
Apress © 2003 (1200 pages)
.NET. Now, let me preface the following sections by saying that the goal here is not to detail each and every
This comprehensive text starts with a brief overview of the
option of each andC#everylanguagewizardand. Doingthen quicklyso wouldmovrequires to keyat leastt chnicaltwoandadditional (large) chapters. Rather, I will illustrate the use architecturalof a numberissuesof keyfortools.NETin devorderlopersto prime. the pump for further exploration on your own terms. you wish to follow along, create a brand new C# Windows Forms application named WinFormsVsNetWizardsApp.
Table of Contents
C# and the .NET Platform, Second Edition
Revisiting the Solutions Explorer
Introduction
Part One - Introducing C# and the .NET Platform
Back in Chapter 2, you were introduced to the Server Explorer utility of VS .NET. As you recall, this tool allows
Chapter 1 - The Philosophy of .NET
you to interact with a number of server-side services (such as MS SQL Server) using a familiar tree-view GUI.
Chapter 2 - Building C# Applications
This view of the world can be quite helpful when working with ADO.NET, given that you are able to add any
Part Two - The C# Programming Language
number of connections to your design time view. To do so, simply right-click the Data Connections node and
Chapter 3 - C# Language Fundamentals |
|
select Add Connection (Figure 17-28). |
|
Chapter 4 - Object-Oriented Programming with C# |
|
Chapter 5 - Exceptions and Object Lifetime |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
Based Programming |
Part |
|
Chapter |
Layer |
Chapter |
Forms) |
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
ChapterFigure19 - 17ASP.NET-28: AddingWeb Applicationsa new data connection |
|
Chapter 20 - XML Web Services |
|
Index
At this point you are provided with the Data Link Properties dialog box, which allows you to specify the name an
List of Figures
location of the database you wish to communicate with. For this example, connect to your local Cars database List(Figureof Tables17-29).
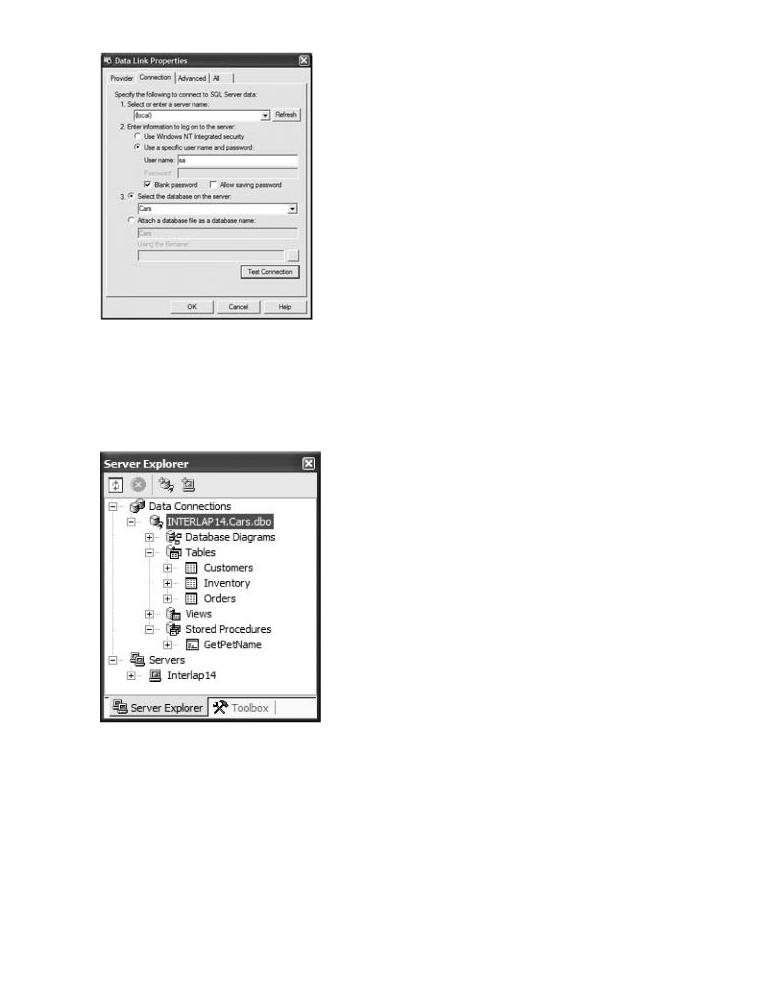
Second Edition
ISBN:1590590554
starts with a brief overview of the
quickly moves to key technical and
NET developers.
Table
C# and
Part |
Platform |
Chapter
Chapter
Part
Chapter
ChapterFigure4 - 17Object-29:-ConfiguringOriented Programmingthe new datawithconnectionC#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Once you have added a SQL connection, you are then able to view the underlying data source (Figure 17-30).
Chapter 7 - Callback Interfaces, Delegates, and Events
you would guess, each database item (tables, views, stored procedures, and whatnot) can be opened from with
Chapter 8 - Advanced C# Type Construction Techniques
VS .NET by simply double-clicking the item of interest. To take things out for a test drive, double-click the
Part Three - Programming with .NET Assemblies |
|
Inventory table and GetPetName stored procedure. |
|
Chapter 9 - Understanding .NET Assemblies |
|
Chapter 10 - Processes, AppDomains, Contexts, and Threads |
|
Chapter |
and Attribute-Based Programming |
Part |
|
Chapter |
Remoting Layer |
Chapter |
(Introducing Windows Forms) |
Chapter |
(GDI+) |
Chapter |
Controls |
Chapter |
|
Chapter |
|
Part |
Services |
Chapter |
Controls |
Chapter |
|
Chapter |
|
Index
List of
List of
Figure 17-30: Interacting with the Cars database via VS .NET
Note Many of the advanced (read: really cool and helpful) features of the Solution Explorer (such as the ability to create, edit, and delete various database objects) require Visual Studio .NET Enterprise Edition or higher.
Creating a SQL Connection at Design Time
As you have seen earlier in this chapter, the System.Data.SqlClient.SqlConnection type defines a ConnectionString property, which contains information regarding a programmatic connection to a given data source. While it is not too difficult to build a connection string by hand, the IDE provides a number of tools to assist you in this regard. To illustrate one such approach, activate the Data tab from the Toolbox window (Figur 17-31), and place a SqlConnection component onto your design time Form.
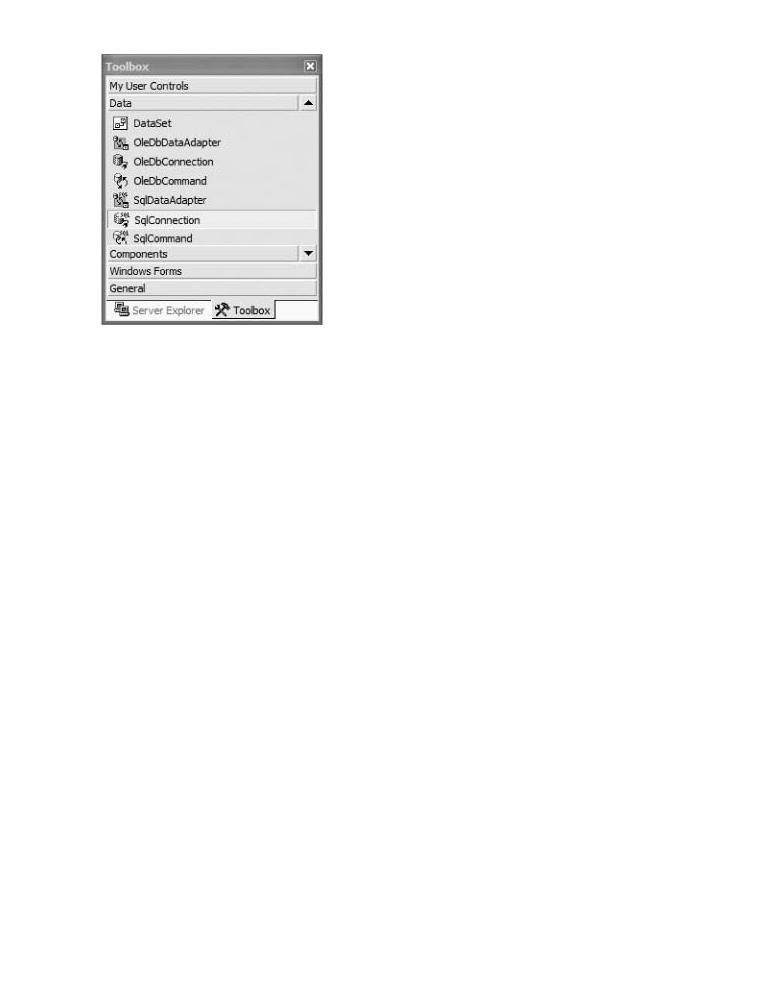
Second Edition
ISBN:1590590554
starts with a brief overview of the moves to key technical and
developers.
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter 4 - Object-Oriented Programming with C#
Figure 17-31: Design time connection configuration
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Once you have done so, select this item from the Icon Tray, and using the Properties window, select the name
Chapter 7 - Callback Interfaces, Delegates, and Events
your Cars connection from the ConnectionString property drop-down list. At this point, you should be pleased to
Chapter 8 - Advanced C# Type Construction Techniques
see that a valid connection string has been created and configured on your behalf (within the #region code
Part Three - Programming with .NET Assemblies
block):
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
public class Form1 : System.Windows.Forms.Form
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
{
Part Four - Leveraging the .NET Libraries
private System.Data.SqlClient.SqlConnection sqlConnection1;
Chapter 12 - Object Serialization and the .NET Remoting Layer
...
Chapter 13 - Building a Better Window (Introducing Windows Forms)
private void InitializeComponent()
Chapter 14 - A Better Painting Framework (GDI+)
{
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
this.sqlConnection1.ConnectionString = " <your connection string info>
Chapter 17 - Data Access with ADO.NET
...
Part Five - Web Applications and XML Web Services
}
Chapter 18 - ASP.NET Web Pages and Web Controls
}
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
IndexYou are now able to program against you new SqlConnection type using all of the techniques presented in this Listchapterof Figures. Command objects can be dragged onto the Forms designer in a similar manner. Once you do, you m
edit your SQL queries using an integrated query editor (activated via the CommandText property).
List of Tables
Building a Data Adapter at Design Time
The VS .NET IDE also provides an integrated wizard that takes care of the grunge work that is necessary to bui SELECT, UPDATE, INSERT, and DELETE commands for a given data adapter type (as you have seen, writing this code by hand can be a bit on the tedious side). To illustrate, delete your current SqlConnection component and place a SqlDataAdapter component onto your Icon Tray. This action will launch the Data Adapter Configuration Wizard. Once you click past the initial Welcome screen, you will be asked which data connection should be used to configure the data adapter (Figure 17-32). Again, pick your Cars connection.

Edition
ISBN:1590590554
brief overview of the
key technical and
.
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Figure 17-32: Specifying the connection for the new SqlDataAdapter
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
ChapterThe next6 step- Interfacesallows youandtoCollectionsconfigure how the data adapter should submit data to the data store (Figure 17-33)
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter
Part
Chapter |
|
Chapter |
|
Chapter |
Based Programming |
Part |
|
Chapter |
Layer |
Chapter |
Forms) |
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
|
IndexFigure 17-33: Specifying data store updates |
|
List of Figures |
|
List of Tables
As you can see from Figure 17-37, you have three choices. If you want to move data between the data store an the DataSet using stored procedures, you may instruct the wizard to generate new INSERT, UPDATE, and DELETE functions based on an initial SELECT statement or, as an alternative, choose prefabricated stored procedures. Your final option is to have the wizard build SQL queries. I'll assume you will check out the generated stored procedures at your leisure, so simply select Use SQL Statements for the time being.
The next step of the tool asks you to specify the SQL SELECT statement that will be used to build the set of SQ queries. Although you may type in the SQL SELECT statement by hand, you can also activate the Query Builde tool (which should look familiar to many Visual Basic developers). Figure 17-34 shows the crux of this integrate SQL editor.

ISBN:1590590554
overview of the
technical and
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Figure 17-34: Creating the initial SELECT logic
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Once you are finished with the data adapter configuration tool, open your code window and check out the
Chapter 6 - Interfaces and Collections
InitializeComponent() method. As you can see, the new SqlCommand data members are configured
Chapter 7 - Callback Interfaces, Delegates, and Events
automatically. I won't bother to list each aspect of the generated code, as you have already manually written the
Chapter 8 - Advanced C# Type Construction Techniques
same syntax by hand during the chapter. However, here is a partial snapshot:
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
public class Form1 : System.Windows.Forms.Form
Chapter 10 - Processes, AppDomains, Contexts, and Threads
{
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
private System.Data.SqlClient.SqlConnection sqlConnection1;
Part Four - Leveraging the .NET Libraries
private System.Data.SqlClient.SqlDataAdapter sqlDataAdapter1;
Chapter 12 - Object Serialization and the .NET Remoting Layer
private System.Data.SqlClient.SqlCommand sqlSelectCommand1;
Chapter 13 - Building a Better Window (Introducing Windows Forms)
private System.Data.SqlClient.SqlCommand sqlInsertCommand1;
Chapter 14 - A Better Painting Framework (GDI+)
private System.Data.SqlClient.SqlCommand sqlUpdateCommand1;
Chapter 15 - Programming with Windows Forms Controls
private System.Data.SqlClient.SqlCommand sqlDeleteCommand1;
Chapter 16 - The System.IO Namespace |
|
|
private void InitializeComponent() |
||
Chapter 17 - Data Access with ADO.NET |
|
|
{ |
|
|
Part Five - Web Applications and XML W b Servic s |
||
|
this.sqlDataAdapter1.DeleteCommand = this.sqlDeleteCommand1; |
|
Chapter 18 - ASPthis.NET.sqlDataAdapter1Web P ges and W b Controls.InsertCommand = this.sqlInsertCommand1; |
||
Chapter 19 - ASPthis.NET.sqlDataAdapter1Web Applications |
.SelectCommand = this.sqlSelectCommand1; |
|
Chapter 20 - XMLthis.sqlDataAdapter1.TableMappings.AddRangeWeb Services |
||
Index |
(new System.Data.Common.DataTableMapping[] { |
|
List of Figures |
new System.Data.Common.DataTableMapping("Table", "Inventory", |
new System.Data.Common.DataColumnMapping[] {
List of Tables
new System.Data.Common.DataColumnMapping("CarID", "CarID"), new System.Data.Common.DataColumnMapping("Make", "Make"), new System.Data.Common.DataColumnMapping("Color", "Color"),
...
//
// sqlInsertCommand1
//
this.sqlInsertCommand1.CommandText =
"INSERT INTO Inventory(CarID, Make, Color, PetName)" + "VALUES (@CarID, @Make, @Color," +
" @PetName); SELECT CarID, Make, Color, PetName FROM " + "Inventory WHERE (CarID = @CarID)"; this.sqlInsertCommand1.Connection = this.sqlConnection1; this.sqlInsertCommand1.Parameters.Add(
new System.Data.SqlClient.SqlParameter("@CarID",
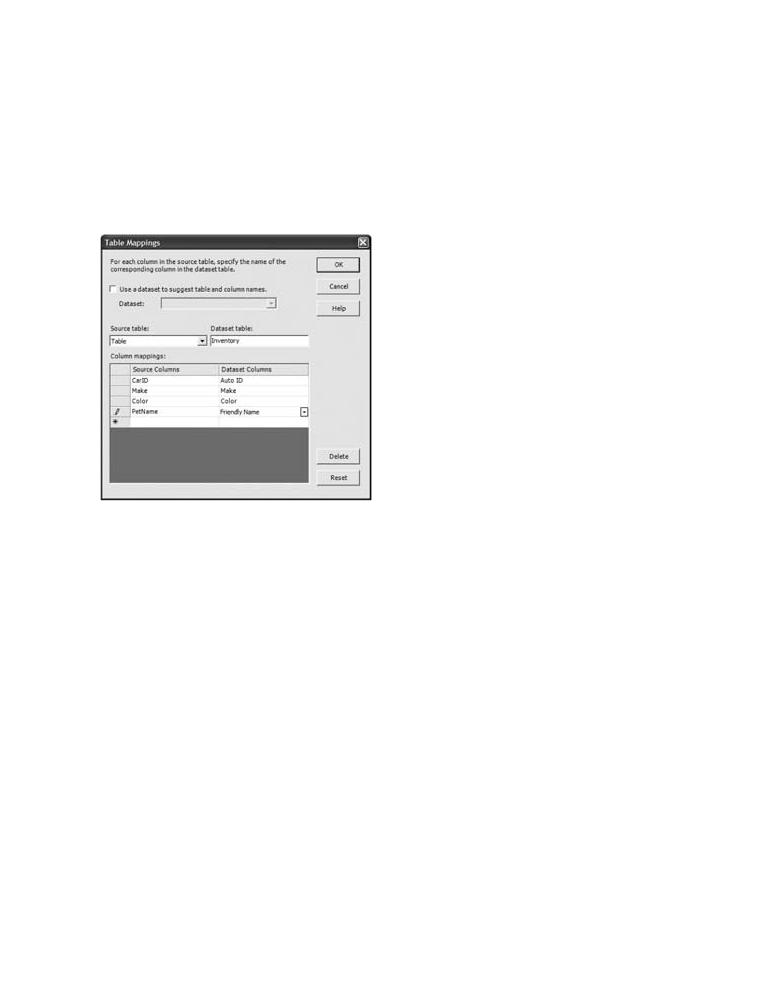
System.Data.SqlDbType.Int, 4, "CarID"));
... |
|
C# and the .NET Platform, Second Edition |
|
|
} |
by Andrew Troelsen |
ISBN:1590590554 |
||
|
||||
|
|
|
}Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and
architectural issues for .NET developers.
Hopefully, things look quite familiar. Once you have generated the basic look and feel of your data adapter, you may continue to alter its behavior using the Properties window. For example, if you were to select the
TableMappings property, you could assign display names to each column of the underlying data table (Figure
Table of Contents
17-35).
C# and the .NET Platform, Second Edition
Introduction
Part
Chapter
Chapter
Part
Chapter
Chapter
Chapter
Chapter
Chapter
Chapter
Part
Chapter |
|
Chapter |
Threads |
Chapter |
-Based Programming |
Part |
|
Chapter |
Layer |
Figure 17-35: Altering column display names at design time
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Using the Configured Data Adapter
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
At this point you are free to make use of any of the tricks you have learned about during the chapter to obtain a manipulate a DataSet. By way of a simple test, place a DataGrid widget onto the main Form, and show the
contents of the Inventory table via a Button Click event:
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
private void btnLoadInventory_Click(object sender, System.EventArgs e)
Index
{
List of Figures
DataSet myDS = new DataSet();
List of Tables sqlConnection1.Open();
sqlDataAdapter1.Fill(myDS);
dataGrid1.DataSource = myDS.Tables["Inventory"];
}
Design Time Connections and Data Adapters, Take Two
The previous example illustrated how you can gain design time assistance for connection and data adapter typ by dragging and dropping the related components from the Data tab of the Toolbox onto the forms designer. If you are looking for the optimal short cut, try the following: First, delete all items from the Form's icon tray. Next, switch to the Server Explorer view and select the Inventory icon of the Cars database from the Data Connection node. Now, drag the Inventory table icon directly onto the Form. Once you do, you will be given SqlConnection and SqlDataAdapter types that are automatically preconfigured to communicate with the Cars database!
Understand that everything shown via the Server Explorer is "drag-and-droppable." To check things out further,

select the GetPetName stored procedure and drag it onto the Form. As you can see, the result is a new
C# and the .NET Platform, Second Edition
SqlCommand type that is preconfigured to trigger the underlying stored proc:
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
this.sqlCommand1.CommandText = "dbo.[GetPetName]";
This comprehensive text starts with a brief overview of the
this.sqlCommand1.CommandType = System.Data.CommandType.StoredProcedure;
C# language and then quickly moves to key technical and
this.sqlCommand1.Connection = this.sqlConnection1; architectural issues for .NET developers.
this.sqlCommand1.Parameters.Add(
new System.Data.SqlClient.SqlParameter("@RETURN_VALUE",
Table ofSystemContents.Data.SqlDbType.Int, 4,
System.Data.ParameterDirection.ReturnValue, false,
C# and the .NET Pla form, Second Edition
((System.Byte)(0)), ((System.Byte)(0)), "",
Introduction
System.Data.DataRowVersion.Current, null));
Part One - Introducing C# and the .NET Platform
this.sqlCommand1.Parameters.Add(
Chapter 1 - The Philosophy of .NET
new System.Data.SqlClient.SqlParameter("@carID",
Chapter 2 - Building C# Applications
System.Data.SqlDbType.Int, 4));
Part Two - The C# Programming Language
this.sqlCommand1.Parameters.Add(
Chapter 3 - C# Language Fundamentals
new System.Data.SqlClient.SqlParameter("@petName",
Chapter 4 - Object-Oriented Programming with C#
System.Data.SqlDbType.VarChar, 20,
Chapter 5 - Exceptions and Object Lifetime
System.Data.ParameterDirection.Output, false,
Chapter 6 - Interfaces and Collections
((System.Byte)(0)), ((System.Byte)(0)), "",
Chapter 7 - Callback Interfaces, Delegates, and Events
System.Data.DataRowVersion.Current, null));
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
ChapterAgain, once9 - Understthe wizarndings have.NETgeneratedAssembliesthe initial code, you are free to tweak and change things to your liking.
ChapterBy way10of -example,Processeselect, AppDomains,one of theContexts,SqlCommandThreadstypes and check out the Parameters property of the ChapterProperties11 -windowType Reflecti. As youn,wouldLate Binding,guess, theand resultingAttribute-dialogB sed Programmingeditor allows you to edit parameter objects at desig
ParttimeFour. - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
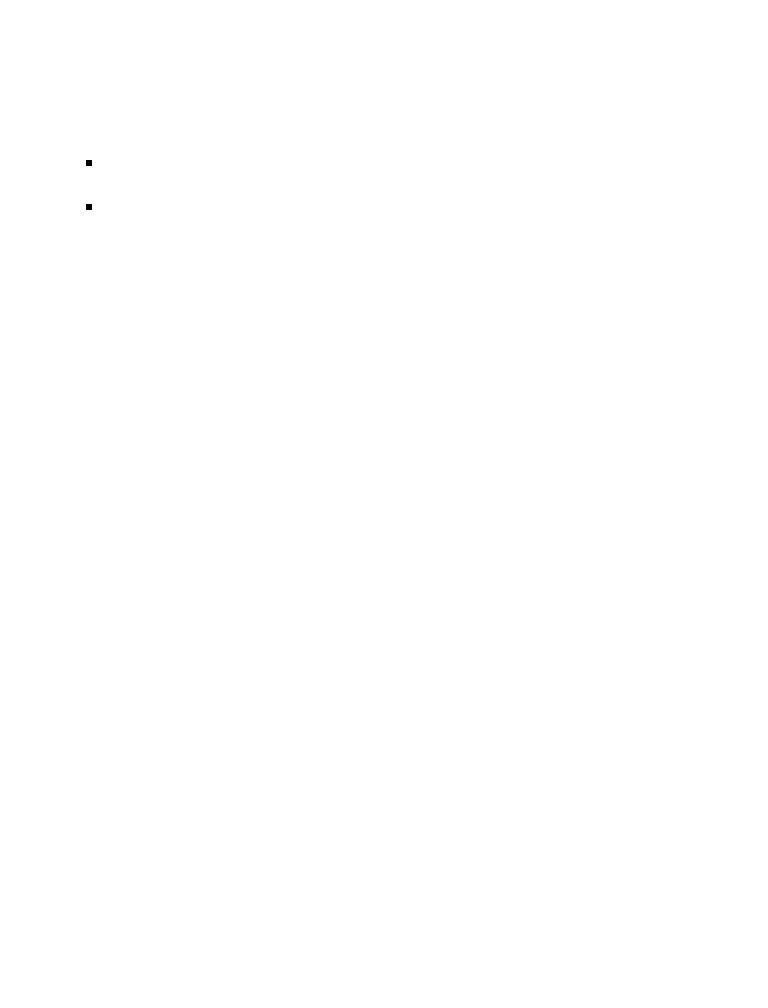
Working withC# andDataSetsthe .NET Platform,DesignSeco dTimeEdition
by Andrew Troelsen |
ISBN:1590590554 |
In the previous code samples, you directly created and allocated a DataSet type in your code base. As you
Apress © 2003 (1200 pages)
would guess, VS .NET also offers design time support for the mighty DataSet. In fact, VS .NET allows you
This comprehensive text starts with a brief overview of the to generate two typesC# languageof DataSets:and then quickly moves to key technical and
architectural issues for .NET developers.
Untyped DataSets: This corresponds to the DataSets examined thus far in the chapter. Untyped DataSets are simply variables of type System.Data.DataSet.
Table of Contents
Typed DataSets: This option allows you to generate a new .NET class type that derives from the
C# and the .NET Platform, Second Edition
DataSet class. Using this wrapper class, you can interact with the underlying data using object-
Introduction
oriented property syntax, rather than having to directly interact with the Rows and Columns collections.
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
If you wish to add an uptyped DataSet to your project, use the Data tab of the VS .NET Toolbox and place
Chapter 2 - Building C# Applications
a new DataSet onto your design time template. When you do so, you will find that a new member variable
Part Two - The C# Programming Language
of type DataSet has been added and allocated to a new instance, which can then be manipulated in your
Chapter 3 - C# Language Fundamentals
code.
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 |
- Exceptions and Object Lifetime |
|
Working with Typed DataSets at Design Time |
||
Chapter 6 |
- Interfaces and Collections |
|
Chapter 7 |
- Callback Interf ces, Delegates, and Events |
|
Now, let's wrap up this chapter by checking out the role of typed DataSets. First of all, understand the |
||
Chapter 8 |
- Advanced C# Type Constru |
Tech iques |
typed DataSets perform the same function as an untyped DataSet (they contain a client-side cache of
Partdata)Threeand-areProgrammingpopulated withand updated.NET Assembliesvia a corresponding data adapter. The key difference is, simply put,
Cheasepterof9use- .UnderstFor example,nding .whenNET Assembliyou usesuntyped DataSets, you are required to drill into the internal
Chapterstructure10of- theProcesses,DataTableAppDomaintype using, Contexts,the RowsandandThreadsColumns properties. While nothing is horribly wrong Chapterwith this11approach,- Type Reflection,it would beLateniceBinding,to buildandaAttributewrapper-Basedclass thatProgramminghides the internal complexities from view.
PartForFourexample,- Leveragingrather thanthe .NETwritingLibrariesthis:
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter// A basic13 - Buildinguntypeda BetterDataSetWindow.(Introducing Windows Forms)
ChapterlblCarID14 -.ATextBet er=PaintingmyDS.FrTameworkbles["Inventory"](GDI+) .Rows[0]["CarID"];
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
you can write the following:
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
// A typed DataSet.
Chapter 18 - ASP.NET Web Pages and Web Controls
lblCarID.Text = myDS.Inventory[0].CarID;
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
In a nutshell, strongly typed DataSets are classes that derive from DataSet, and define numerous
List of Figures
properties that interact with the underlying structure on your behalf.
List of Tables
To generate a strongly typed DataSet using VS .NET, right-click the related data adapter and select Generate DataSet (Figure 17-36).
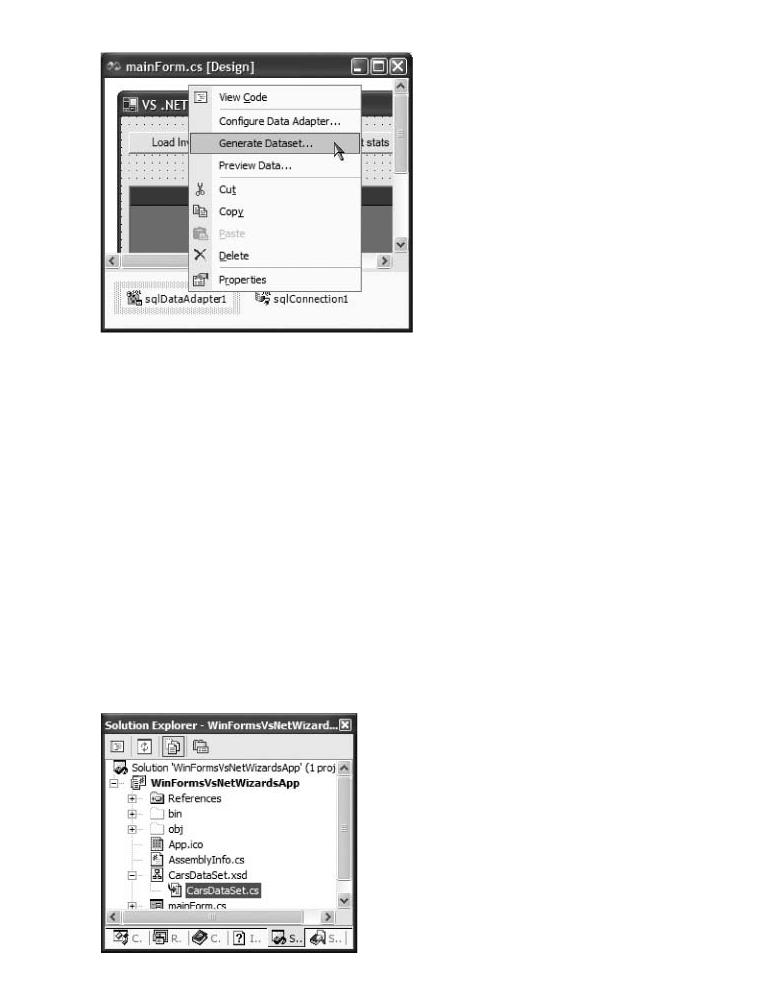
ISBN:1590590554
overview of the
technical and
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter
Figure 17-36: Creating a typed DataSet at design time
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
From the dialog box simply supply a name (such as CarsDataSet) and reconfirm the tables to wrap. At this
Chapter 7 - Callback Interfaces, Delegates, and Events
point, you can make use of your new DataSet-derived type as follows:
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
private void btnLoadInventory_Click(object sender, System.EventArgs e)
Chapter 9 - Understanding .NET Assemblies
{
Chapter 10 - Processes, AppDomains, Contexts, and Threads sqlConnection1.Open();
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming sqlDataAdapter1.Fill(carsDataSet1);
Part Four - Leveraging the .NET Libraries
dataGrid1.DataSource = carsDataSet1.Inventory;
Chapter 12 - Object Serialization and the .NET Remoting Layer
}
Chapter 13 - Building a Better Window (Introducing Windows Forms) Chapter 14 - A Better Painting Framework (GDI+)
ChapterNote that15 you- Programmingno longer needwith toWindowsmake useFormsof theControlsTables property to gain access to the contained Inventory
Chaptertable. Using16 - Thea stronglySystemtyped.IO NamespaceDataSet, you simply reference the Inventory property.
Chapter 17 - Data Access with ADO.NET
PartUnderFive - WebtheApplicationsHood ofandtheXMLTypedWeb ServicesDataSets
Chapter 18 - ASP.NET Web Pages and Web Controls
ChapterActivate19the- ASPSolution.NET WebExplorerApplicationsand click the Show all Files button. You will find that your strongly typed
ChDataSetpter 20is-representedXML Web Servicesby two related files. First you have an XML schema file (*.xsd) that represents the Indoverallx structure of the DataSet in terms of XML. Behind this schema file is a related *.cs class file (Figure
17-37).
List of Figures
List of Tables
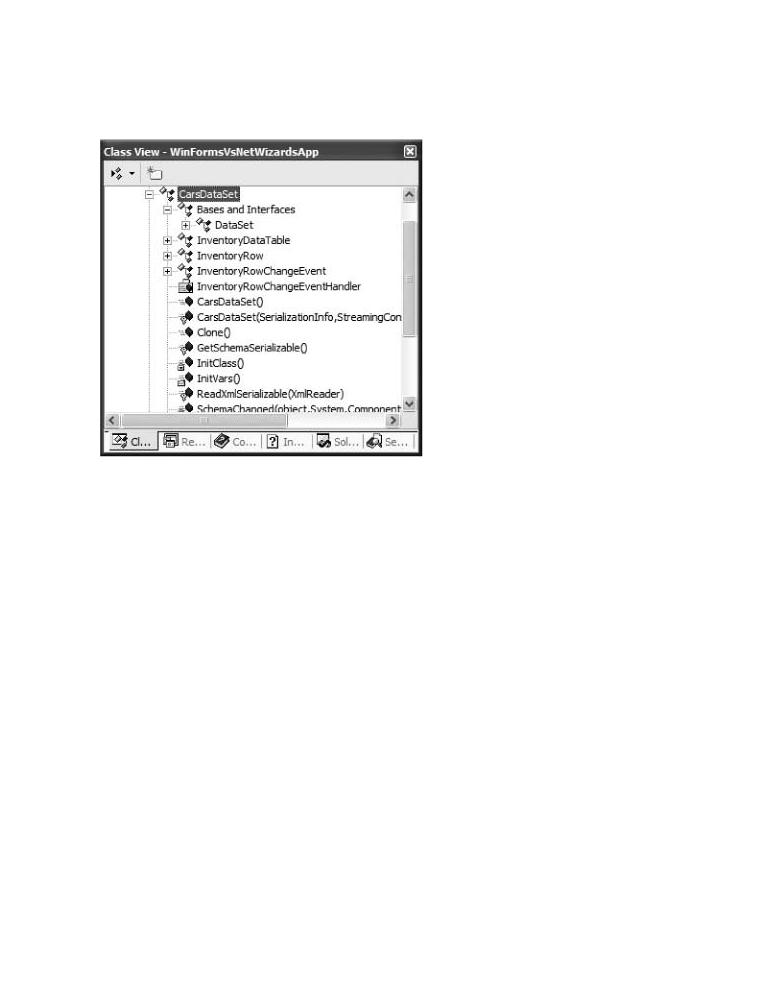
Figure 17-37: Typed DataSets have a related "code behind" file
C# and the .NET Platform, Second Edition
If you switch overbytoAndrewClass View,Troelsenyou will find that the newly generatedISBN:1590590554CarsDataSet class defines a number of nestedApreclasses© 2003to represent(1200 pages)the rows and tables it is responsible for maintaining (Figure 17-38).
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter
Chapter
Chapter
Chapter
Chapter
Part
Chapter
Chapter
Chapter 11 - Type Reflection, Late Binding, and Attrmaintainsbute-Based Programming
Figure 17-38: The DataSet-derived type a set of nested classes.
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
While I will assume you will check out the generated code at your leisure, it is worth pointing out that
Chapter 13 - Building a Better Window (Introducing Windows Forms)
strongly typed DataSets are automatically configured as serializable, which, as you recall from Chapter
Chapter 14 - A Better Painting Framework (GDI+)
12, allows you to persist the state data to some storage medium (as well as marshal the type by value):
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
[Serializable()]
Chapter 17 - Data Access with ADO.NET
...
Part Five - Web Applications and XML Web Services
public class CarsDataSet : DataSet {...}
Chapter 18 - ASP.NET Web Pages and Web Controls Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
If you were to check out the nested InventoryDataTable type, you would see that in addition to deriving
Index
from System.Data.DataTable, this new type is enumerable, and contains a number of private DataColumn Listtypesof Figuresthat are exposed via class properties (which are in turn exposed via the strongly typed DataRow-
Listderivedof Tablesclass). Thus, if you wish to access the stats of the first row in the Inventory table, you can write the following:
private void btnGetStats_Click(object sender, System.EventArgs e)
{
string info = "Color: " + carsDataSet1.Inventory[0].Color; info += "\n" + "Make: " + carsDataSet1.Inventory[0].Make; info += "\n" + "Pet Name: " +
carsDataSet1.Inventory[0].Friendly_Name_of_Car; MessageBox.Show(info, "First Car Info");
}
Again, the bonus is that the exposed properties are already mapped into CLR data types based on the underlying schema information. It is worth pointing out that while strongly typed DataSets are quite programmer friendly, the additional layers of indirection could be a potential performance drain. When you
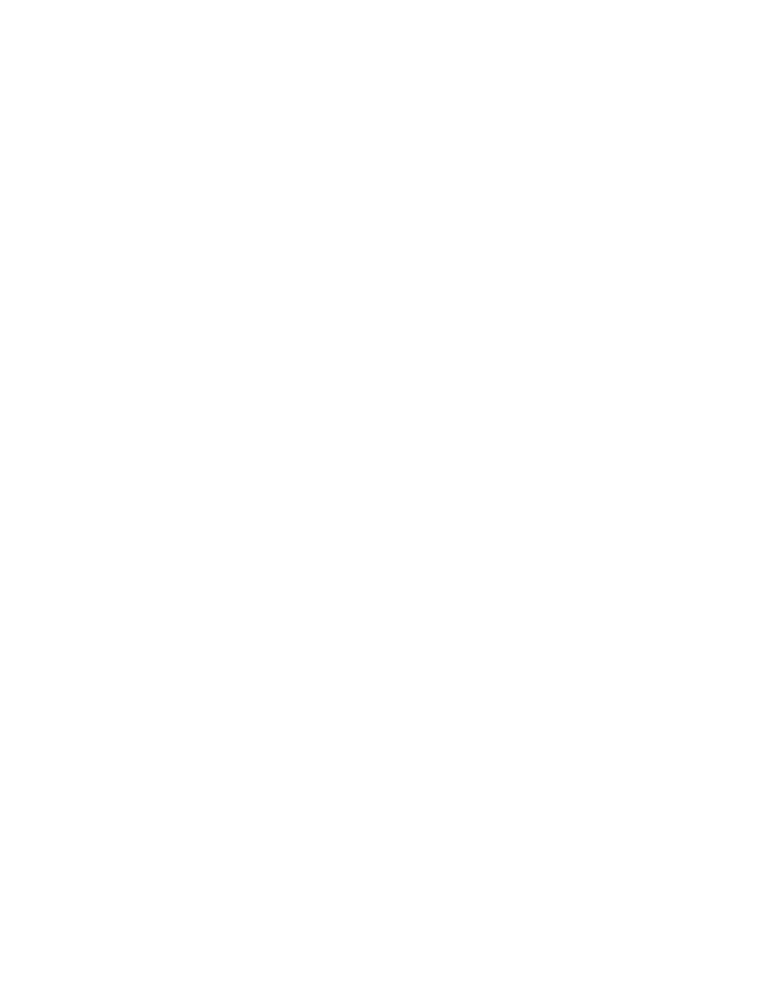
want to ensure your ADO.NET DataSets are operated on as quickly as possible, simple untyped DataSets
C# and the .NET Platform, Second Edition tend to offer better performance.
by Andrew Troelsen |
ISBN:1590590554 |
Well, that wraps thingsApre sup© 2003for (1200this chapterpages) . Obviously there is much more to say about ADO.NET than I had time to presentThisherecomprehensive. If you are lookingtext startsfor witha solida briefcomplementov rview ofto thise chapter, I recommend checking outA Programmer'sC#Guidelanguageto ADOand then.NETquicklyin C# bymovesMaheshto keyChandtechnical(Apress,and 2002).
architectural issues for .NET developers.
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables