
- •Table of Contents
- •C# and the .NET Platform, Second Edition
- •Introduction
- •Part One: Introducing C# and the .NET Platform
- •Part Two: The C# Programming Language
- •Part Three: Programming with .NET Assemblies
- •Part Four: Leveraging the .NET Libraries
- •Part Five: Web Applications and XML Web Services
- •Obtaining This Book's Source Code
- •The .NET Solution
- •What C# Brings to the Table
- •The Role of the Assembly Manifest
- •Summary
- •Chapter 2: Building C# Applications
- •Summary
- •Chapter 3: C# Language Fundamentals
- •Defining Program Constants
- •Defining Custom Class Methods
- •C# Enumerations
- •Summary
- •The Second Pillar: C#'s Inheritance Support
- •Summary
- •Catching Exceptions
- •Finalizing a Type
- •Garbage Collection Optimizations
- •Summary
- •Chapter 6: Interfaces and Collections
- •Building Comparable Objects (IComparable)
- •Summary
- •Summary
- •Internal Representation of Type Indexers
- •Summary
- •An Overview of .NET Assemblies
- •Understanding Delayed Signing
- •Using a Shared Assembly
- •GAC Internals
- •Summary
- •Spawning Secondary Threads
- •A More Elaborate Threading Example
- •Summary
- •Summary
- •Object Persistence in the .NET Framework
- •The .NET Remoting Namespaces
- •Understanding the .NET Remoting Framework
- •All Together Now!
- •Terms of the .NET Remoting Trade
- •Testing the Remoting Application
- •Revisiting the Activation Mode of WKO Types
- •Deploying the Server to a Remote Machine
- •Summary
- •Control Events
- •The Form Class
- •Summary
- •Regarding the Disposal of System.Drawing Types
- •Understanding the Graphics Class
- •Summary
- •The TextBox Control
- •Working with Panel Controls
- •Configuring a Control's Anchoring Behavior
- •Summary
- •Chapter 16: The System.IO Namespace
- •The Static Members of the Directory Class
- •The Abstract Stream Class
- •Summary
- •The Role of ADO.NET Data Providers
- •The Types of System.Data
- •Selecting a Data Provider
- •The Types of the System.Data.OleDb Namespace
- •Working with the OleDbDataReader
- •Summary
- •Submitting the Form Data (GET and POST)
- •Some Benefits of ASP.NET
- •Creating an ASP.NET Web Application by Hand
- •The Composition of an ASP.NET Page
- •The Derivation of an ASP.NET Page
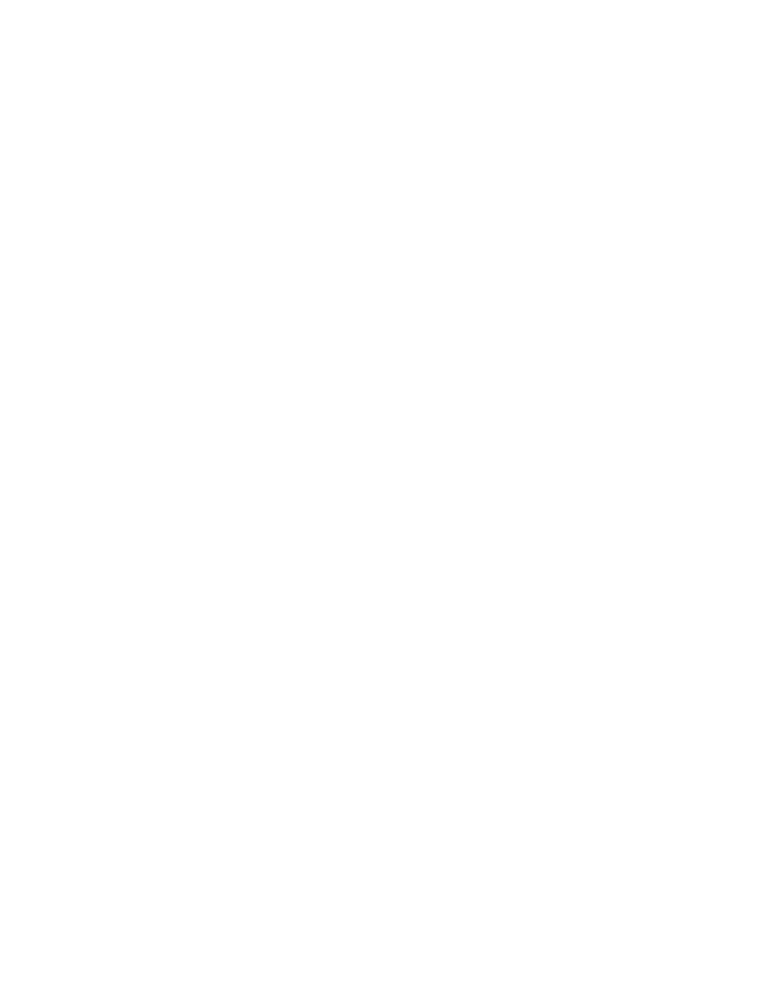
Internal Representation of Type Indexers |
|
C# and the .NET Platform, Second Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
Now that you have seen a number of variations on the C# indexer method, you may be wondering how this
Apr ss © 2003 (1200 pages)
member is represented in terms of raw CIL. If you were to open up the numerical indexer of the Cars type,
This comprehensive text starts with a brief overview of the you would find thatC#thelanguageC# compilerand thenhasquicklycreatedmovesa propertyto key namedtechnicalItem:and
architectural issues for .NET developers.
.property instance class Indexer.Car Item(int32)
{
Table of Contents
.get instance class Indexer.Car Indexer.Cars::get_Item(int32)
C# and the .NET Platform, Second Edition
.set instance void Indexer.Cars::set_Item(int32,
Introduction
class Indexer.Car)
Part One - Introducing C# and the .NET Platform
} // end of property Cars::Item
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
ChapterNow, if3you- haveC# LanguagebackgroundFundamentalsin the Visual Basic programming language, you may be happy to see that Chapterthe internal4 - representationObject-OrientedofProgrammingC# indexerwithis indeedC# a property named Item. The reason (for those who
are not aware of various VB-isms) is that VB types that provide access to subtypes typically define a
Chapter 5 - Exceptions and Object Lifetime
default method named Item. In the world of VB, a default item identifies the method to be called on a type
Chapter 6 - Interfaces and Collections
if the caller does not explicitly specify it by name, but rather makes use of the index notation (which under
Chapter 7 - Callback Interfaces, Delegates, and Events
VB is denoted as () rather than []). Given this factoid, understand that C# indexers are CLS compliant, and
Chapter 8 - Advanced C# Type Construction Techniques
can be called from any .NET-aware programming language.
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Note FYI, unlike VB 6.0, VB .NET will only allow you to define a default property that has at least one
Chapter 10 - Processes, AppDomains, Contexts, and Threads
parameter. Given this, the VB 6.0 "Set" keyword is now obsolete.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
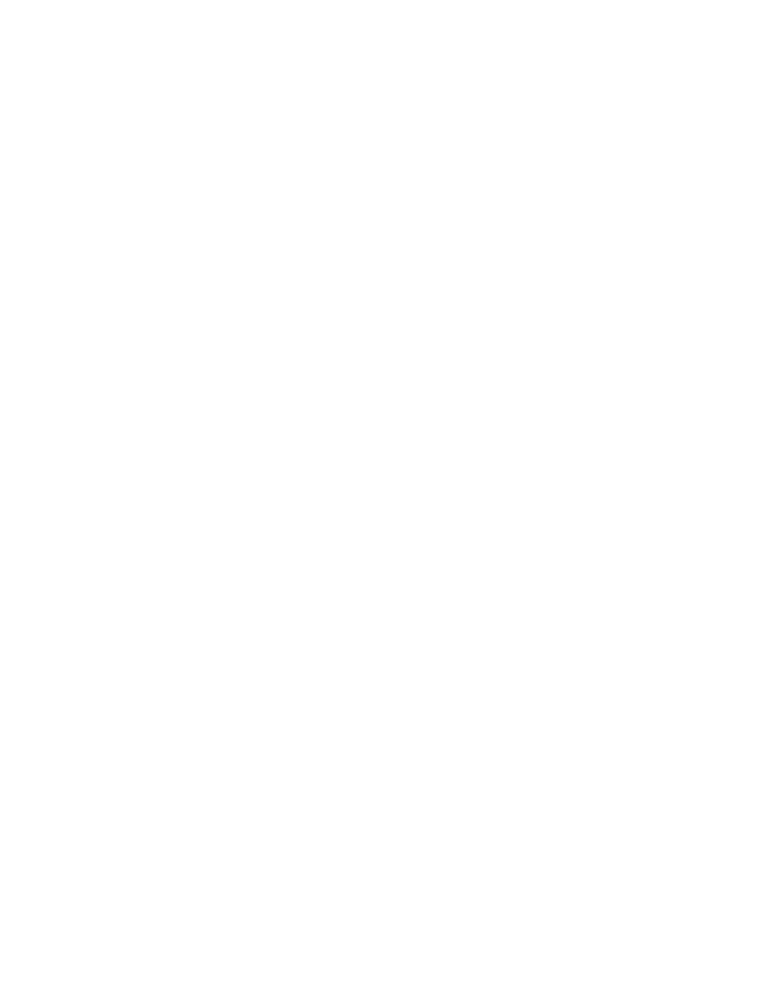
Using the C# aIndexerd the .NETfromPlatform,VBSecond.NETEdition
by Andrew Troelsen |
ISBN:1590590554 |
To illustrate the language-agnostic nature of C# indexers requires an understanding of .NET code libraries
Apress © 2003 (1200 pages)
(e.g., custom *.dll assemblies). The next chapter will examine this process in great detail, so for the time
This comprehensive text starts with a brief overview of the
being simply assumeC# languageyou haveandcreatedthen quicklya C# codemoveslibraryto keynamedtechnicalIndexerCodeLibraryand that contains the Cars, Radio, andarchitecturalCar types. Ifissuesa VB .forNET.NETapplicationdeveloperswere. to set a reference to this external assembly, it would be able to interact with the contained types as follows:
Table of Contents
' The VB .NET 'Imports' keyword is
C# and the .NET Platform, Second Edition
' the same as the C# 'using' keyword.
Introduction
Imports IndexerCodeLibrary
Part One - Introducing C# and the .NET Platform |
|
||||
' A VB .NET 'Module' is simple a class containing nothing |
|||||
Chapter 1 |
|
- The Philosophy of .NET |
|
||
' but |
static members. |
|
|||
Chapter 2 |
|
- Building C# Applications |
|
||
Module CarApp |
|
||||
Part Two - The C# Programming Language |
|
||||
Sub Main() |
|
||||
Chapter 3 |
|
- C# Language Fundamentals |
|
||
|
|
Dim carLot As New Cars() |
|
||
Chapter 4 |
|
- Object-Oriented Programming with C# |
|
||
|
|
' Item is mapped to the default property in VB .NET, |
|||
Chapter 5 |
|
- Exceptions and Obj ct Li etime |
|
||
|
|
' and can therefore be omitted. Thus, either of these calls |
|||
Chapter 6 |
|
- Interfac' ares perfectlyand Collectionsfine and legal. |
|||
Chapter 7 |
|
- CallbackcarLotInterfaces,.Item(0)Delegates,= New andCar("MB",Events |
150, 40) |
||
Chapter 8 |
|
- AdvcarLot(1)nced C# Type= NewConstructionCar("Daisy",Techniques130, 40) |
|||
Part Three - ProgrammingDim c AswithCar.NET Assemblies |
|
||||
Chapter 9 |
|
- UnderstandingFor Each c.NETInAssembliescarLot |
|
||
Chapter 10 |
- Processes,Console.WriteLine("Name:AppDomains, Contexts, and Threads{0}, Max Speed: {1}", _ |
||||
Chapter 11 |
- Type Reflection,c.PetName,Late Binding,c.MaxSpeed)and Attribute-Based Programming |
||||
Part Four - LeveragingNext the .NET Libraries |
|
||||
Chapter End12 - ObjectSub Serialization and the .NET Remoting Layer |
|||||
ChapterEnd Module13 - Building a Better Window (Introducing Windows Forms) |
|||||
Chapter 14 |
- A Better Painting Framework (GDI+) |
|
|||
Chapter 15 |
- Programming with Windows Forms Controls |
|
|||
Chapter 16 |
- T System.IO Namespace |
|
|||
Although we have not yet drilled into the details of code libraries or cross-language programming (more |
|||||
Chapter 17 |
- Data Access with ADO.NET |
|
|||
details to come in Chapter 9), the code shown here should be quite easy on the eyes. Do note that VB |
|||||
|
|
|
|||
.NET client applications have the option of specifying the Item property by name, given that VB .NET |
|||||
Part Five - Web Applications and XML Web Services |
|
interprets any C# indexers as the default property of the type (e.g., it will be invoked even if the code base |
|
Chapter 18 - ASP.NET W b P ges and Web Controls |
|
Chapterdoes not19explicitly- ASP.NETreferenceW b Applicationsit by name). |
|
Chapter 20 - XML Web Services |
|
IndexSOURCE |
The IndexerCodeLibrary and VbNetIndexerClient projects are located under the |
CODE |
Chapter 8 subdirectory. |
List of Figures |
|
List of Tables

OverloadingC#Operatorsand the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
So much for the topic of C# indexers. Next up, we will examine a technique that is not supported by all
Apress © 2003 (1200 pages)
.NET-aware programming languages, but is useful nonetheless. C#, like any programming language, has
This comprehensive text starts with a brief overview of the
a canned set of tokensC# languthatgeareandusedthentoquicklyperformovesbasictooperationskey technicalon andintrinsic types. For example, everyone knows that the + architecturoperator canl issuesbe appliedfor .NETto twodevelopersintegers. in order to yield a new integer:
// The + operator in action.
Table of Contents int a = 100;
C# and the .NET Platform, Second Edition int b = 240;
Introduction
int c = a+b; // c is now 340
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
ChapterThis is 2no -majorBuildingnewsC#flash,Applicationsbut have you ever stopped and noticed how the same + operator can be
Pappliedrt Two to- TheanyC#intrinsicProgrammingC# dataLanguagetype? For example:
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
// + operator with strings.
Chapter 5 - Exceptions and Object Lifetime
string s1 = "Hello";
Chapterstring6 s2- Interfaces= " world!";and Collections
Chapterstring7 s3- Callback= s1 Interfaces,+ s2; //Delegates,s3 is nowand Events"Hello world!"
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
In essence, the + operator has been overloaded to function correctly on various individual data types.
Chapter 9 - Understanding .NET Assemblies
When the + operator is applied to numerical types, the result is the summation of the operands. However,
Chapter 10 - Processes, AppDomains, Contexts, and Threads
when applied to string types, the result is string concatenation.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Note Understand that unlike numerous types in the .NET base class libraries, the intrinsic data types
Chapter 12 - Object Serialization and the .NET Remoting Layer
of the System namespace have not literally overloaded the set of C# operators, as CIL provides
Chapter 13 - Building a Better Windowmanipulation(Introduci g Windows Forms) specific opcodes for the of numeric data types.
Chapter 14 - A Better Painting Framework (GDI+)
The C# language (like C++ and unlike Java) provides the capability for you to build custom classes and
Chapter 15 - Programming with Windows Forms Controls
structures that also respond uniquely to the same set of basic tokens (such as the + operator). To
Chapter 16 - The System.IO Namespace
illustrate, assume the following simple Point structure (and yes, you are able to overload operators on
Chapter 17 - Data Access with ADO.NET
class types as well):
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
// Just a simple everyday C# struct.
Chapter 19 - ASP.NET Web Applications
public struct Point
Chapter 20 - XML Web Services
{
Index
private int x, y;
List of Figures
public Point(int xPos, int yPos)
List of Tables
{
x = xPos; y = yPos;
}
public override string ToString()
{
return string.Format("X pos: {0} Y pos: {1}", this.x, this.y);
}
}
Now, logically speaking, it makes sense to add Points together. On a related note, it would be helpful to subtract one Point from another. For example, if you created two Point objects with some initial startup values, you may like to do something like this:
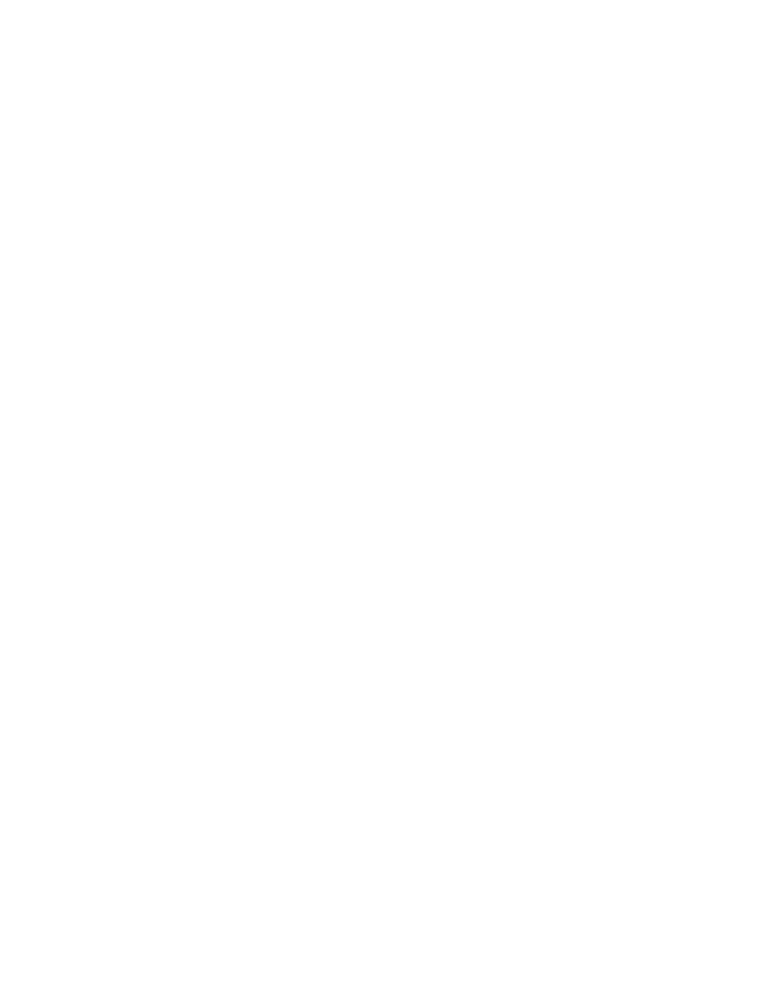
// Adding and subtracting two points.
C# and the .NET Platform, Second Edition public static int Main(string[] args)
{ |
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages) |
|
|
|
// Make two points |
|
This comprehensive text starts with a brief overview of the
Point ptOne = new Point(100, 100);
C# language and then quickly moves to key technical and
Point ptTwo = new Point(40, 40); architectural issues for .NET developers.
// Add the points to make a new point.
Point bigPoint = ptOne + ptTwo;
Console.WriteLine("Here is the big point: {0} ", bigPoint.ToString());
Table of Contents
// Subtract the points to make a new point.
C# and the .NET Platform, Second Edition
Point minorPoint = bigPoint - ptOne;
Introduction
Console.WriteLine("Just a minor point: {0} ", minorPoint.ToString());
Part One - Introducing C# and the .NET Platform
return 0;
Chapter 1 - The Philosophy of .NET
}
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
ChapterClearly,3your- C#goalLanguageis to somehowFundamentalsmake your Point type react uniquely to the + and – operators. To allow a
Chaptercustom4type- Objectto respond-OrientoedtheseProgrammingintrinsic tokens,with C#C# provides the "operator" keyword, which can only be
Chapterused in5conjunction- ExceptionswithandstaticObjectmethodsLifetime. To illustrate:
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
// A more intelligent Point type.
Chapterpublic8 struct- AdvancedPointC# Type Construction Techniques
Part{ Three - Programming with .NET Assemblies
Chapter private9 - Und rstandingint x,.NETy; Assemblies
Chapter public10 - Processes,Point(intAppDomains,xPos,Contexts,int yPos){and Threadsx = xPos; y = yPos; }
// The Point type can be added...
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
public static Point operator + (Point p1, Point p2)
Part Four - Leveraging the .NET Libraries
{
Chapter 12 - Object Serialization and the .NET Remoting Layer
Point newPoint = new Point(p1.x + p2.x, p1.y + p2.y);
Chapter 13 - Building a Better Window (Introducing Windows Forms)
return newPoint;
Chapter 14 - A Better Painting Framework (GDI+)
}
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
public static Point operator - (Point p1, Point p2)
Chapter 17 - Data Access with ADO.NET
{
Part Five - Web Applications and XML Web Services
// Figure new X (assume [0,0] base).
Chapter 18 |
- ASP.NET Web Pages and Web Controls |
||
Chapter 19 |
|
int newX = p1.x - p2.x; |
|
- ASP.NET Web Applications |
|||
|
|
if(newX < 0) |
|
Chapter 20 |
- XML Web Services |
||
|
|
throw new ArgumentOutOfRangeException(); |
|
Index |
|
// Figure new Y (also assume [0,0] base). |
|
List of Figures |
|||
int newY = p1.y - p2.y; |
|||
List of Tables |
if(newY < 0) |
||
|
|
throw new ArgumentOutOfRangeException(); |
|
|
|
return new Point(newX, newY); |
}
public override string ToString()
{
return string.Format("X pos: {0} Y pos: {1}", this.x, this.y);
}
}
Notice that the class now contains two strange looking methods called operator + and operator –.The logic behind operator + is simply to return a brand new Point based on the summation of the incoming Point arguments. Thus, when you write pt1 + pt2, under the hood you can envision the following hidden call to the static operator + method:

// |
p3 = |
Point.operator+C# and the .NET(p1,Platform,p2) Second Edition |
|
p3 |
= p1 |
+ p2;by Andrew Troelsen |
ISBN:1590590554 |
|
|
Apress © 2003 (1200 pages) |
|
This comprehensive text starts with a brief overview of the
Likewise, p1 – p2 maps to the following:
C# language and then quickly moves to key technical and architectural issues for .NET developers.
// p3 = Point.operator- (p1, p2)
p3 = p1 - p2;
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Overloading the Equality Operators
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
As you may recall, System.Object.Equals() can be overridden in order to perform valuebased (rather than
Part Two - The C# Programming Language
referenced-based) comparisons between objects. In addition to overriding Equals() and GetHashCode(),
Chapter 3 - C# Language Fundamentals
an object may choose to override the equality operators (= = and !=). To illustrate, here is the updated
Chapter 4 - Object-Oriented Programming with C#
Point type:
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
// This incarnation of Point also overloads the == and != operators.
Chapter 7 - Callback Interfaces, Delegates, and Events public struct Point
Chapter 8 - Advanced C# Type Construction Techniques
{
Part Three - Programming with .NET Assemblies
public int x, y;
Chapter 9 - Understanding .NET Assemblesint
public Point(int xPos, yPos){ x = xPos; y = yPos;}
Chapter... 10 - Processes, AppDomains, Contexts, and Threads
Chapter public11 - TypeoverrideReflect on, LateboolBinding,Equals(objectand Attribute-Basedo) Programming
Part Four{- Leveraging the .NET Libraries
Chapter 12 - Objectif((Serialization(Point)o)and.xthe=.NET= thisRemoting.x &&Layer
((Point)o).y = = this.y)
Chapter 13 - Building a Better Window (Introducing Windows Forms)
return true;
Chapter 14 - A Better Painting Framework (GDI+)
else
Chapter 15 - Programming with Windows Forms Controls
return false;
Chapter 16 - The System.IO Namespace
}
Chapter 17 - Data Access with ADO.NET
public override int GetHashCode()
Part Five - Web Applications and XML Web Services
{ return this.ToString().GetHashCode(); }
Chapter 18 - ASP.NET Web Pages and Web Controls
// Now let's overload the == and != operators.
Chapter 19 - ASP.NET Web Applications
public static bool operator ==(Point p1, Point p2)
Chapter 20 - XML Web Services
{ return p1.Equals(p2); }
Index public static bool operator !=(Point p1, Point p2)
List of Figures
{ return !p1.Equals(p2); }
List of Tables
}
Notice how the implementation of operator == and operator != simply makes a call to the overridden Equals() method to get the bulk of the work done. Given this, you can now exercise your Point class as follows:
// Make use of the overloaded equality operators. public static int Main(string[] args)
{
...
if(ptOne == ptTwo) |
// |
Are they |
the same? |
|
Console.WriteLine("Same values!"); |
||||
else |
|
|
different values."); |
|
Console.WriteLine("Nope, |
||||
if(ptOne != ptTwo) |
// |
Are |
they |
different? |
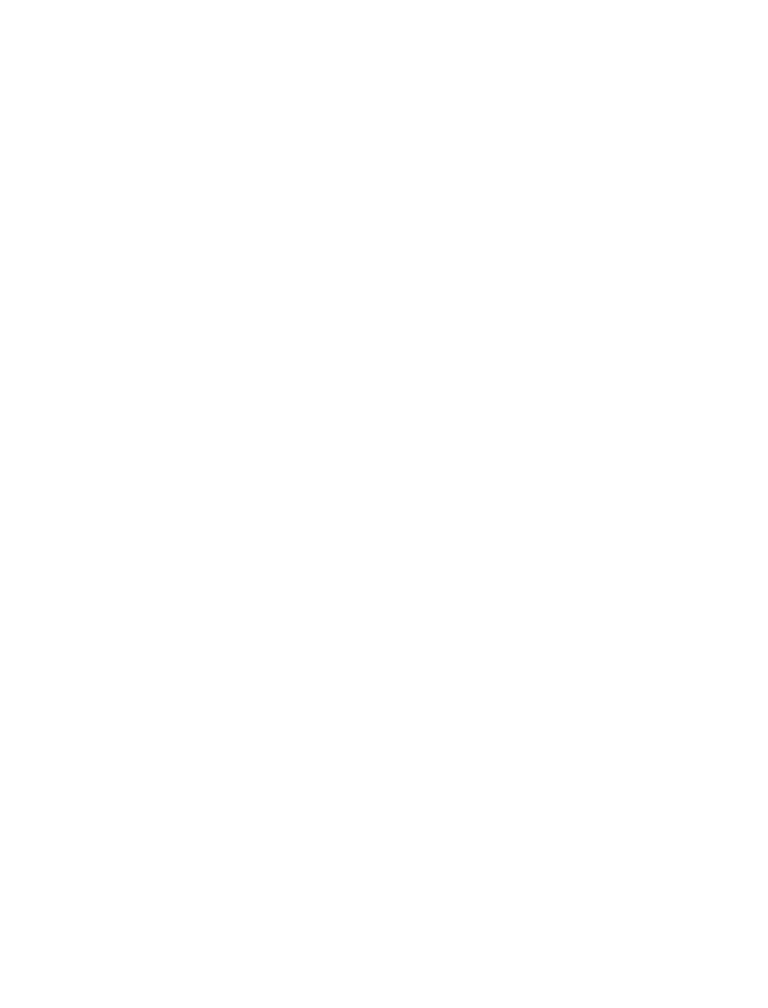
Console.WriteLine("These are not equal.");
else |
C# and the .NET Platform, Second Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
|
|
Console.WriteLine("Same values!"); |
|
}Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and
architectural issues for .NET developers.
As you can see, it is quite intuitive to compare two objects using the well-known == and != operators rather than making a call to Object.Equals(). As a rule of thumb, classes that override
Object.Equals()/Object.GetHashCode() should always overload the == and != operators.
Table of Contents
C#If youanddotheoverload.NET Platform,the equalitySecondoperatorsEdition for a given class, keep in mind that C# demands that if you
Introductionoverride operator ==, you must also override operator !=, just as when you override Equals() you will need
Partto overrideOne - IntroducingGetHashCode()C# and. Thist e .NETensuresPlatformthat an object behaves in a uniform manner during comparisons
Chapterand functions1 - ThecorrectlyPhilosophyif placedof .NETinto a hash table (if you forget, the compiler will let you know).
Chapter 2 - Building C# Applications
SOURCE The OverLoadOps project is located under the Chapter 8 subdirectory.
Part Two - The C# Programming Language
CODE
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
ChaptOverloadingr 5 - ExceptionstheandComparisonObject Lifetime Operators
Chapter 6 - Interfaces and Collections
InChapter 6, you learned how to implement the IComparable interface in order to compare the relative
Chapter 7 - Callback Interfaces, Delegates, and Events
relationship between two like objects. Additionally, you may also overload the comparison operators (<, >, <=, and >=) for the same class. Like the equality operators, C# demands that < and > are overloaded as a set. The same holds true for the <= and >= operators. If the Car type you developed in Chapter 6
overloaded these comparison operators, the object user could now compare types as follows:
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
// Exercise the overloaded < operator for the Car class.
Part Four - Leveraging the .NET Libraries
public class CarApp
Chapter 12 - Object Serialization and the .NET Remoting Layer
{
Chapter 13 - Building a Better Window (Introducing Windows Forms) public static int Main(string[] args)
Chapter 14 - A Better Painting Framework (GDI+)
{
Chapter 15 - Programming with Windows Forms Controls |
|||
|
// Make an array |
of Car types. |
|
Chapter 16 - The System.IO Namespace |
|||
|
Car[] myAutos = new Car[5]; |
||
Chapter 17 - Data Access with ADO.NET |
Car(123, "Rusty"); |
||
|
myAutos[0] = new |
||
Part Five - Web Applications and XML Web Services |
|||
|
myAutos[1] = new |
Car(6, "Mary"); |
|
Chapter 18 - ASP.NET Web Pages and Web Controls |
|||
|
myAutos[2] = new Car(6, "Viper"); |
||
Chapter 19 - ASP.NET Web Applications |
Car(13, "NoName"); |
||
|
myAutos[3] = new |
||
Chapter 20 - XML Web Services |
Car(6, "Chucky"); |
||
Index |
myAutos[4] = new |
||
// Is Rusty less |
than Chucky? |
||
List of Figures |
|||
List of Tables |
if(myAutos[0] < myAutos[4]) |
Console.WriteLine("Rusty is less than Chucky!");
else
Console.WriteLine("Chucky is less than Rusty!"); return 0;
}
}
Assuming you have a Car type that implements IComparable, overloading the comparison operators is trivial. Here is the updated class definition:
// This class is also comparable using the comparison operators.
public class Car : IComparable
{
...
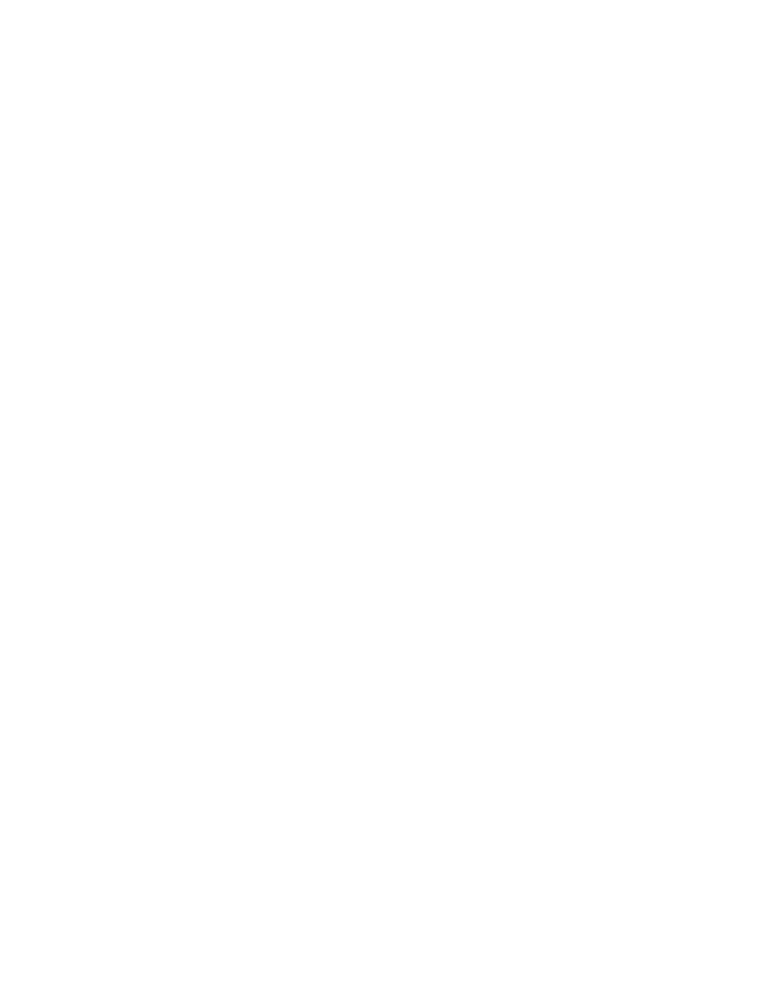
public int CompareTo(object o)
C# and the .NET Platform, Second Edition
{
by Andrew Troelsen |
ISBN:1590590554 |
Car temp = (Car)o; |
|
Apress © 2003 (1200 pages) |
|
if(this.CarID > temp.CarID) |
|
Thisreturncomp ehensive text starts with a brief overview of the
1;
C# language and then quickly moves to key technical and if(this.CarID < temp.CarID)
architectural issues for .NET developers. return -1;
else
return 0;
Table of Contents
}
C# and the .NET Platform, Second Edition
public static bool operator < (Car c1, Car c2)
Introduction
{
Part One - Introducing C# and the .NET Platform
IComparable itfComp = (IComparable)c1;
Chapter 1 - The Philosophy of .NET
return (itfComp.CompareTo(c2) < 0);
Chapter 2 - Building C# Applications
}
Part Two - The C# Programming Language
public static bool operator > (Car c1, Car c2)
Chapter 3 - C# Language Fundamentals
{
Chapter 4 - Object-Oriented Programming with C#
IComparable itfComp = (IComparable)c1;
Chapter 5 - Exceptions and Object Lifetime
return (itfComp.CompareTo(c2) > 0);
Chapter 6 - Interfaces and Collections
}
Chapter public7 - CallbackstaticInterfaces,boolDelegates,operatorand<=Events(Car c1, Car c2)
Chapter {8 - Advanced C# Type Construction Techniques
Part Three - PrograIComparableming with .NETitfCompAssemblies= (IComparable)c1;
Chapter 9 - Understandingreturn (itfComp.NET Assemblies.CompareTo(c2) <= 0);
}
Chapter 10 - Processes, AppDomains, Contexts, and Threads
public static bool operator >= (Car c1, Car c2)
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
{
Part Four - Leveraging the .NET Libraries
IComparable itfComp = (IComparable)c1;
Chapter 12 - Object Serialization and the .NET Remoting Layer
return (itfComp.CompareTo(c2) >= 0);
Chapter 13 - Building a Better Window (Introducing Windows Forms)
}
Chapter 14 - A Better Painting Framework (GDI+)
}
Chapter 15 - Programming with Windows Forms Controls Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
SOURCE The ObjCompWithOps project is located under the Chapter 8 subdirectory.
Chapter 18 - ASP.NET Web Pages and Web Controls
CODE
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

The InternalC#Representationand the .NET Platf rm,ofSecondOverloadedEdition Operators
by Andrew Troelsen |
ISBN:1590590554 |
Like any C# programming element, overloaded operators are represented using specific CIL instructions. To
Apress © 2003 (1200 pages)
begin examining what takes place behind the scenes, open up your OverLoadOps.exe assembly (created
This comprehensive text starts with a brief overview of the
previously) usingC#ildasmlanguage.exe. Asandyouthencanquicklysee frommovesFigureto key8-technical3, the +, and-, = =, and != operators are internally
expressed via hiddenarchitecturalmethods,issueswhichforin.NETthis developcase arersnamed. op_Addition(), op_Subtraction(), op_Equality(), and op_Inequality().
Table of Contents
C# and
Part
Chapter
Chapter
Part
Chapter |
|
Chapter |
C# |
Chapter |
|
Chapter |
|
Chapter |
Events |
Chapter |
Techniques |
Part |
|
Chapter |
|
Chapter |
and Threads |
Chapter 11 - Type Reflection, Late Binding, |
d Attribute-Based Programming |
Figure 8-3: Internal CIL representation of overloaded operators
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Now, if you were to examine the specific CIL instructions for the op_Addition method, you would find that the
Chapter 13 - Building a Better Window (Introducing Windows Forms) specialname flag has also been inserted by csc.exe:
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
.method public hidebysig specialname static
Chapter 16 - The System.IO Namespace
valuetype OverLoadOps.Point op_Addition(valuetype OverLoadOps.Point p1,
Chapter 17 - Data Access with ADO.NET
valuetype OverLoadOps.Point p2) cil managed
Part Five - Web Applications and XML Web Services
{
Chapter 18 - ASP.NET Web Pages and Web Controls
...
Chapter 19 - ASP.NET Web Applications
}
Chapter 20 - XML Web Services
Index
ListTheoftruthFiguresof the matter is that any operator that you may overload equates to a specially named method in
Listtermsof Tablesof CIL. Table 8-3 documents the C#-operator-to-CIL mapping for the key C# operators.
Table 8-3: C#-Operator-to-CIL Special Name Roadmap
|
Intrinsic C# Operator |
|
CIL Representation |
|
|
|
|
|
- - |
|
op_Decrement() |
|
|
|
|
|
++ |
|
op_Increment() |
|
|
|
|
|
Unary- |
|
op_UnaryNegation() |
|
|
|
|
|
Unary + |
|
op_UnaryPlus() |
|
|
|
|
|
! |
|
op_LogicalNot() |
|
|
|
|
|
True |
|
op_True() |
|
|
|
|
|
False |
|
op_False() |
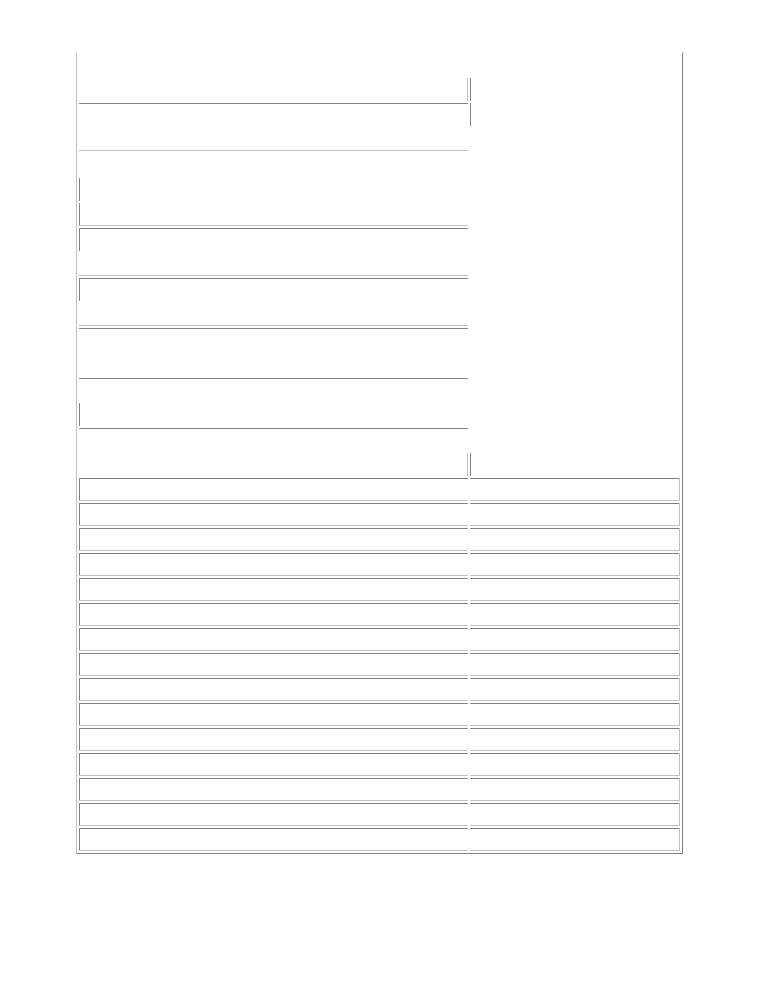
|
~ |
|
C# and the .NET Platform, Second Edition |
|
op_OnesComplement() |
|
|
|
|
|
|
|
Binary + |
by Andrew Troelsen |
ISBN:1590590554 |
||
|
|
|
op_Addition() |
||
|
|
|
Apress © 2003 (1200 pages) |
|
|
|
Binary - |
|
op_Subtraction() |
||
|
This comprehensive text starts with a brief overview |
||||
|
|
|
of the |
||
|
|
|
|
||
|
Binary * |
C# language and then quickly moves to key technical |
and |
||
|
architectural issues for .NET developers. |
|
op_Multiply() |
||
|
|
|
|
||
|
/ |
|
|
|
op_Division() |
|
|
|
|
||
Table% of Contents |
|
op_Modulus() |
|||
|
|
|
|
||
C#^and the .NET Platform, Second Edition |
|
op_ExclusiveOr() |
|||
|
Introduction |
|
|
||
|
|
|
|||
PartBinaryOne -&Introducing C# and the .NET Platform |
|
op_BitwiseAnd() |
|||
|
|
|
|
|
|
|
Chapter |
1 |
- The Philosophy of .NET |
|
op_BitwiseOr() |
|
| |
|
|
|
|
|
Chapter |
2 |
- Building C# Applications |
|
|
|
|
|
|||
Part&&Two - The C# Programming Language |
|
op_LogicalAnd() |
|||
|
|
|
|
|
|
|
Chapter |
3 |
- C# Language Fundamentals |
|
op_LogicalOr() |
|
|| |
|
|
|
|
|
Chapter |
4 |
- Object-Oriented Programming with C# |
|
|
|
|
|
|||
|
Chapter= |
5 |
- Exceptions and Object Lifetime |
|
op_Assign() |
|
|
|
|
|
|
|
Chapter |
6 |
- Interfaces and Collections |
|
op_LeftShift() |
|
<< |
|
|
|
|
|
Chapter |
7 |
- Callback Interfaces, Delegates, and Events |
|
|
|
|
|
|||
|
Chapter>> |
8 |
- Advanced C# Type Construction Techniques |
|
op_RightShift() |
|
|
|
|
||
Part Three - Programming with .NET Assemblies |
|
op_Equality() |
|||
= = |
|
|
|
||
|
Chapter |
9 |
- Understanding .NET Assemblies |
|
|
|
|
|
|||
|
Chapter> |
10 |
- Processes, AppDomains, Contexts, and Threads |
|
op_GreaterThan() |
|
|
|
|
|
|
|
Chapter |
11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
||
|
< |
|
|
|
op_LessThan() |
Part Four - Leveraging the .NET Libraries
!=
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
>=
Chapter 14 - A Better Painting Framework (GDI+)
<=
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
->
Chapter 17 - Data Access with ADO.NET
>>=
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
*=
Chapter 19 - ASP.NET Web Applications
->*
Chapter 20 - XML Web Services
Index
-=
List of Figures
^=
List of Tables
<<=
%=
+=
&=
|=
/=
op_Inequality()
op_GreaterThanOrEqual()
op_LessThanOrEqual()
op_MemberSelection()
op_RightShiftAssignment()
op_MultiplicationAssignment()
op_PointerToMemberSelection()
op_SubtractionAssignment()
op_ExclusiveOrAssignment()
op_LeftShiftAssignment()
op_ModulusAssignment()
op_AdditionAssignment()
op_BitwiseAndAssignment()
op_BitwiseOrAssignment()
op_DivisionAssignment()
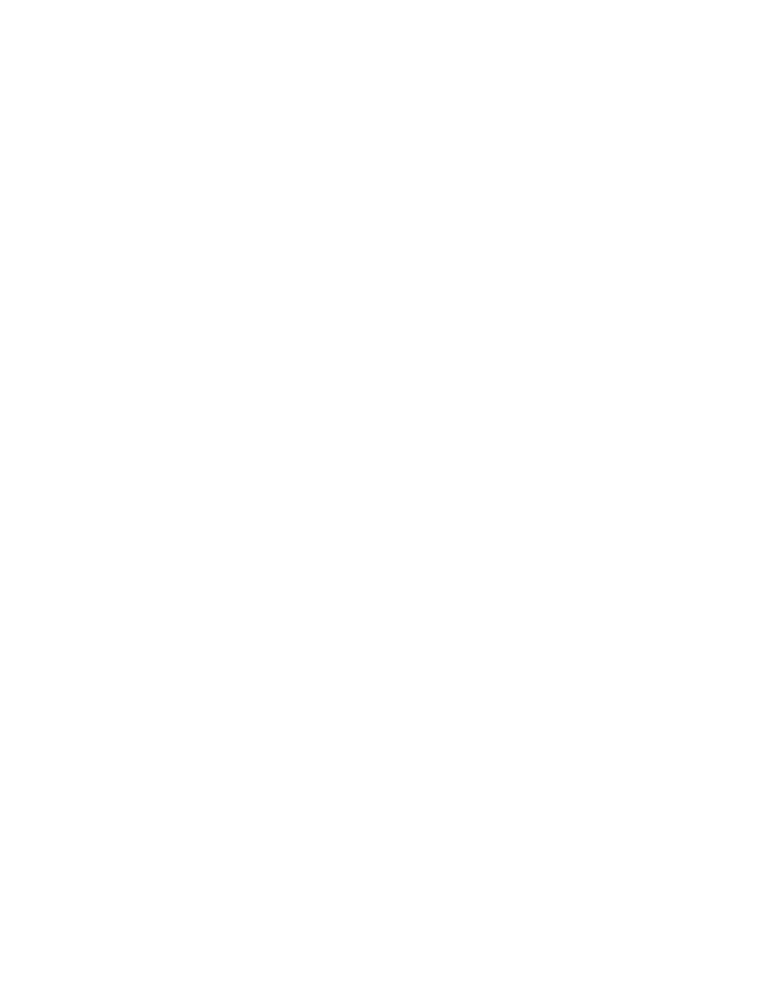
InteractingC#withand Overloadedthe .NET Platform,OperatorsSecond Editionfrom Overloaded-Operator-
by Andrew Troelsen |
ISBN:1590590554 |
Challenged Languages |
|
Apress © 2003 (1200 pages) |
|
This comprehensive text starts with a brief overview of the
The capability to overload operators is useful in that it enables the object user to work with your types
C# language and then quickly moves to key technical and
(more or less) like any intrinsic data point. Now, understand that the capability to overload operators is not architectural issues for .NET developers.
a requirement of the Common Language Specification; thus, not all .NET-aware languages support the construction of types that overload operators.
Table of Contents
What then would happen if an overloaded-operator-challenged language wished to add two points
C# and the .NET Platform, Second Edition
together? To make it as simple as possible for such languages to trigger the same functionality as an
Introduction
overloaded operator, you will do well to provide a publicly named member for the same purpose. To
Part One - Introducing C# and the .NET Platform
illustrate, assume you have updated the Point type as follows:
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
// Exposing overloaded operator semantics using simple
Part Two - The C# Programming Language
// member functions.
Chapter 3 - C# Language Fundamentals
public struct Point
Chapter 4 - Object-Oriented Programming with C#
{
Chapter 5 - Exceptions and Object Lifetime
...
Chapter 6 - Interfaces and Collections
// Operator + via AddPoints()
Chapter 7 |
- Callback Interfaces, Delegates, a Events |
p1, Point p2) |
public static Point AddPoints (Point |
||
Chapter 8 |
- Adva ced C# Type Construction Techniques |
+ p2.y); } |
{ |
return new Point(p1.x + p2.x, p1.y |
Part Three//- ProgOperatormming-withvia.NETSubtractPoints()Assemblies
Chapter public9 - Undersstandticng Point.NET AssemSubtractPointslies (Point p1, Point p2)
Chapter {10 - Processes, AppDomains, Contexts, and Threads
// Figure new X.
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
int newX = p1.x - p2.x;
Part Four - Leveraging the .NET Libraries
if(newX < 0)
Chapter 12 - Object Serialization and the .NET Remoting Layer
throw new ArgumentOutOfRangeException();
Chapter 13 - Building a Better Window (Introducing Windows Forms)
// Figure new Y.
Chapter 14 - A Better Painting Framework (GDI+)
int newY = p1.y - p2.y;
Chapter 15 - Programming with Windows Forms Controls Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
return new Point(newX, newY);
Part Five - Web Applications and XML Web Services
}
Chapter 18 - ASP.NET Web Pages and Web Controls
}
Chapter 19 - ASP.NET Web Applications Chapter 20 - XML Web Services
Index
ListRatherof Figuresthan having duplicate code within your overloaded operators and public members, to maximize Listyourof codingTables efforts, simply implement the overloaded operators to call the member function alternative (or vice versa). For example:
public struct Point
{
...
// For overload-operator-aware languages.
public static Point operator + (Point p1, Point p2)
{return AddPoints(p1, p2); }
//For overload-challenged languages.
public static Point AddPoints (Point p1, Point p2) { return new Point(p1.x + p2.x, p1.y + p2.y); }
}
With this, the Point type is able to expose the same functionality using whichever technique a given

language demands. C# users can apply the + and – operators and/or call AddPoints()/SubtractPoints().
C# and the .NET Platform, Second Edition
Languages that cannot use overloaded operators (such as VB .NET) can make due with the public
by Andrew Troelsen ISBN:1590590554 member functions.
Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the
Triggering the "Special Names" from VB .NET
C# language and then quickly moves to key technical and
architectural issues for .NET developers.
On a related note, understand that it is also possible to directly call the specially named methods from operator-overload-lacking languages, given that they appear as public members on the type. Thus, if you
TablewereoftoContentsbundle the logic of the previous OverLoadOps project into a C# code library (named
OverLoadOpsCodeLibrary), a VB .NET client could interact with the + operator as follows:
C# and the .NET Platform, Second Edition
Introduction
PartImportsOne - IntroducingOverLoadOpsCodeLibraryC# and the .NET Platform
ChapterModule1 OverLoadedOpClient- The Philosophy of .NET
ChapterSub2 -Main()Bu lding C# Applications
Part Two - TheDimC#p1ProgrammingAs PointLanguage
p1.x = 200
Chapter 3 - C# Language Fundamentals
p1.y = 9
Chapter 4 - Object-Oriented Programming with C#
Dim p2 As Point
Chapter 5 - Exceptions and Object Lifetime
p2.x = 9
Chapter 6 - Interfaces and Collections
p2.y = 983
Chapter 7 - Callback Interfaces, Delegates, and Events
' Not as clean as calling AddPoints(),
Chapter 8 - Advanced C# Type Construction Techniques
' but it gets the job done.
Part Three - Programming with .NET Assemblies
Dim bigPoint = Point.op_Addition(p1, p2)
Chapter 9 - Understanding .NET Assemblies
Console.WriteLine("Big point is {0}", bigPoint)
Chapter 10 - Processes, AppDomains, Contexts, and Threads
End Sub
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
End Module
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
ChapterAs you13can- see,BuildingoverloadedBett r-Windowoperator(Introducing-challengedWindows.NET programmingForms) languages are able to directly invoke
Chapterthe internal14 - CILA Bettmethodsr Paintingas ifFrametheywereork (GDI+)"normal" methods. While it is not pretty, it works. Therefore, even Chapterif you (or15your- Programmingteammates)withdo Windowsnot take theFormstimeControlsto create a more friendly named method (such as
AddPoints()), the same functionality can be obtained using the underlying "special" methods.
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
SOURCE The OverLoadOpsCodeLibrary and VbNetOverLoadOpsClient projects are located
Part Five - Web Applications and XML Web Services
CODE under the Chapter 8 subdirectory.
Chapter 18 - ASP.NET Web Pages and Web Controls Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
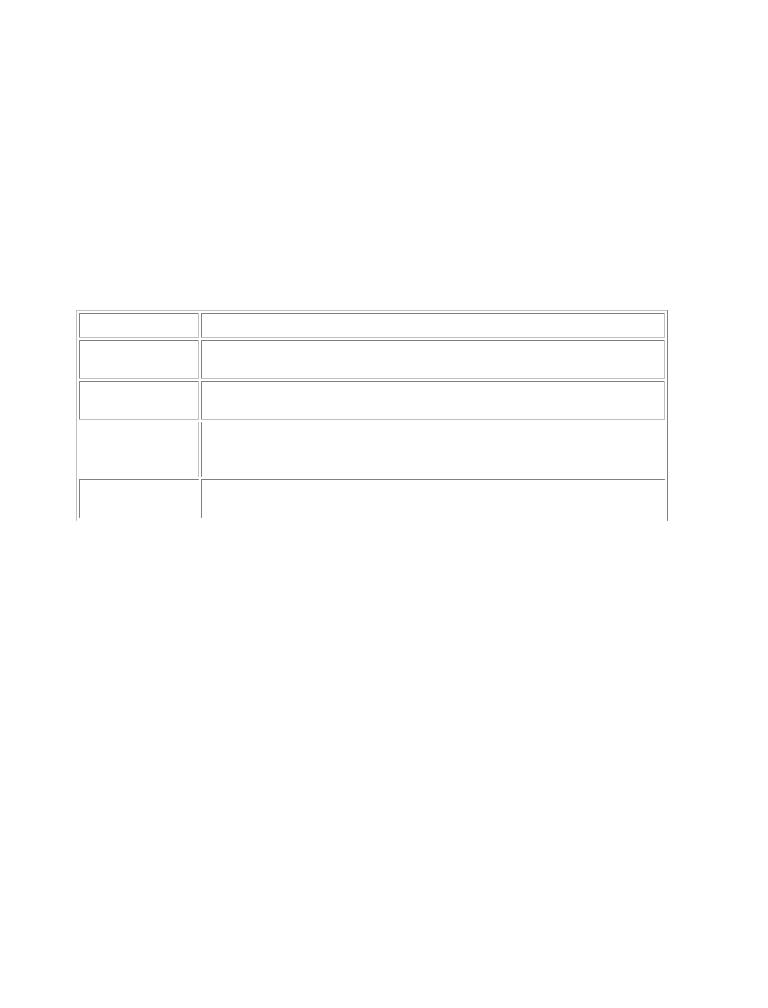
Final ThoughtsC# andRegardingthe .NET Platform,OperatorS cond EditionOverloading
by Andrew Troelsen |
ISBN:1590590554 |
C# provides the capability to build types that can respond uniquely to various intrinsic, well-known
Apress © 2003 (1200 pages)
operators. Now, before you go and retrofit all your classes to support such behavior, you must be sure that
This comprehensive text starts with a brief overview of the
the operator(s) youC#arelanguageabout andto overloadthen quicklymakemovessometosortkeyoftechniclogical senseand in the world at large.
architectural issues for .NET developers.
For example, let's say you overloaded the multiplication operator for the Engine class. What exactly would it mean to multiply two Engine objects? Not much. Overloading operators is generally only useful when
Tablebuildingof Contentsutility types. Strings, points, rectangles, fractions, and hexagons make good candidates for C#operatorand theoverloading.NET Platform,. People,Secondmanagers,Edition cars, headphones, and baseball hats do not. Use this feature
wisely.
Introduction
Part One - Introducing C# and the .NET Platform
Finally, be aware that you cannot overload each and every intrinsic C# operator. Table 8-4 outlines the
Chapter 1 - The Philosophy of .NET
"overloadability" of each item.
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Table 8-4: Valid Overloadable Operators
Chapter 3 - C# Language Fundamentals
ChapterC# Operator4 - Object-OrientedMeaningPro rammingin Lifewith(Can# This Operator Be Overloaded?)
Chapter 5 - Exceptions and Object Lifetime
+, -, !, ~, ++, - -, This set of unary operators can be overloaded.
Chapter 6 - Interfaces and Collections true, false
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter+, -, *,8/, %,- Advanced&, |, C#TheseTypebinaryConstructionoperatorsTechniqucan besoverloaded.
Part^, Three<<, >>- Programming with .NET Assemblies
|
|
|
|
|
|
|
|
|
|
|
|
Chapter |
9 |
- Understanding .NET Assemblies |
|
|
|
||||
|
= =, !=, <, >, <=, |
|
|
The comparison operators can be overloaded. Recall, however, that C# will |
|
|
|
|||
|
Chapter |
10 |
- Processes, AppDomains, Contexts, and Threads |
|
|
|
||||
|
>= |
11 |
|
|
|
demand that "like" operators (i.e., < and >, <= and >=, = = and !=) are |
|
|
|
|
|
Chapter |
- Type Reflection, Late Binding, and Attribute-Based Programming |
|
|
|
|||||
|
|
|
|
|
|
|
overloaded together. |
|
|
|
Part Four - Leveraging |
|
|
the .NET Libraries |
|
|
|
||||
|
|
|
||||||||
Chapter[] |
12 |
- Object |
|
|
SerializationThe [] operatorand thecannot.NET RemotingtechnicallyLayerbe overloaded. As you have seen earlier in |
|
|
|
||
|
Chapter |
13 |
- Building a |
|
|
Betterthis chapter,Windowhowever,(Introducingthe indexerWindowsconstructForms) provides the same functionality. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Chapter |
14 |
- A Better Painting Framework (GDI+) |
|
|||||||
Chapter |
15 |
- Programming with Windows Forms Controls |
|
|||||||
|
And What of the += and -+ Operators? |
|
||||||||
|
Chapter |
16 |
- The System.IO Namespace |
|
||||||
Chapter |
17 |
- Data Access with ADO.NET |
|
If you are coming to C# from a C++ background, you may lament the loss of overloading the shorthand
Part Five - Web Applications and XML Web Services
assignment operators (+=, -=, and so forth) as well as the explicit cast operator (()). Fear not. In terms of
Chapter 18 - ASP.NET Web Pages and Web Controls
C#, the shorthand assignment operators are automatically simulated if a type overloads a given unary
Chapter 19 - ASP.NET Web Applications
operator and the assignment operator. Thus, given that the Point structure has already overloaded the +, -,
Chapter 20 - XML Web Services
and == operators, you are able to write the following:
Index
List of Figures
// Freebie!!
List of Tables
Point ptThree = new Point(90, 5);
ptThree += ptTwo;
Console.WriteLine("ptThree is now {0}", ptThree);
In a similar manner, given that the Point type has overloaded the - and assignment operators, you are also able to exercise a Point type as follows:
// Another freebie!!
Point ptFour = new Point(0, 500);
ptFour += ptThree;
Console.WriteLine("ptFour is now {0}", ptFour);
As for the apparent loss of overloading the explicit cast operator, C# offers an appealing alternative. ...
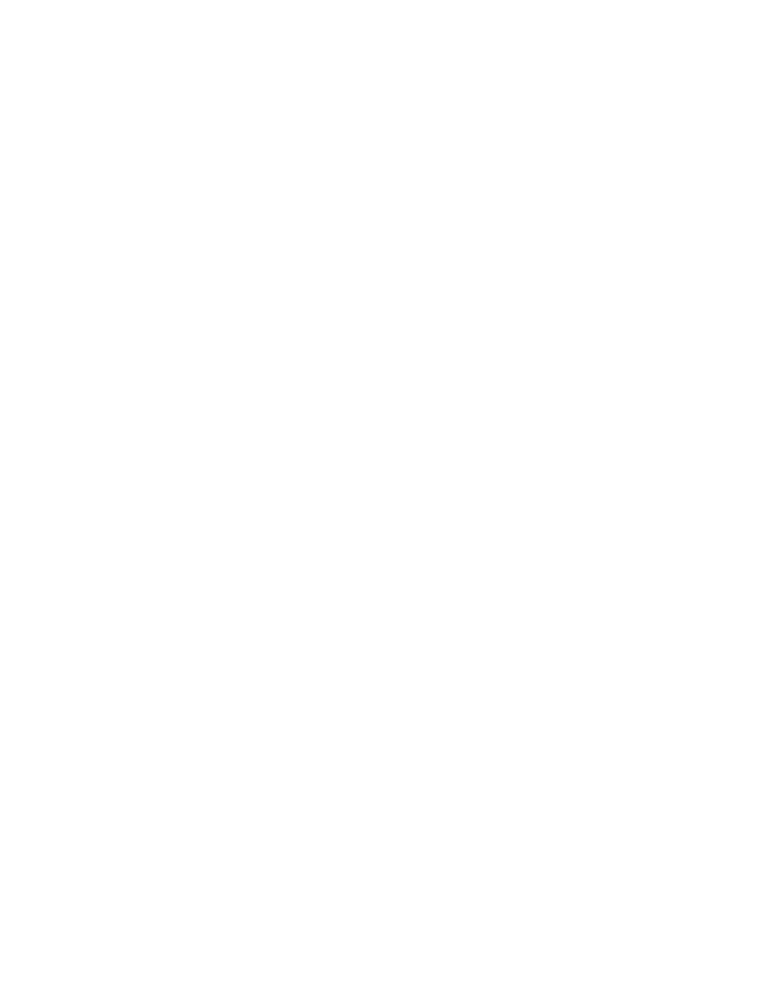
C# and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and architectural issues for .NET developers.
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

UnderstandingC# andCustomthe .NET Platform,Type SecoConversionsd Edition
by Andrew Troelsen |
ISBN:1590590554 |
To wrap up this chapter, we will examine a topic closely related to operator overloading: defining custom
Apress © 2003 (1200 pages)
type conversion functions (as mentioned, this technique is functionally similar to overloading the ()
This comprehensive text starts with a brief overview of the
operator using C++)C# .languageBefore weanddivethenintoquicklythe details,moves let'sto keyquicklytechnicalreviewandthe notion of explicit and implicit conversions betweenarchitecturalnumericalissuesdataforas.NETwelldevelopersas related.class types.
Recall: Numerical Conversions
Table of Contents
C# and the .NET Platform, Second Edition
In terms of the intrinsic numerical types (sbyte, int, float, etc.), an explicit conversion is required when you
Introduction
attempt to store a larger value into a smaller container, as this may result in a loss of data. Basically, this is
Part One - Introducing C# and the .NET Platform
your way to tell the compiler, "Leave me alone, I know what I am trying to do." As you recall, in an explicit
Chapter 1 - The Philosophy of .NET
conversion in C#, you make use of an explicit cast (via the () operator). Conversely, implicit conversions
Chapter 2 - Building C# Applications
happen automatically when you attempt to place a smaller type into a destination type that will not result in
Part Two - The C# Programming Language
a loss of data:
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C# |
||
int a = 123; |
|
|
Chapter 5 - Exceptions and Object Lifetime |
||
long b = a; |
// Implicit conversion from int to long |
|
Chapter 6 |
- Interfaces and Collections |
|
int c |
= (int) b; |
// Explicit conversion from long to int |
Chapter 7 |
- Callback Interfaces, Delegates, and Events |
|
Chapter 8 |
- Advanced C# Type Construction Techniques |
Part Three - Programming with .NET Assemblies
Note Also recall that the "checked"/"unchecked" keywords can be used to generate (or disable)
Chapter 9 - Und rstanding .NET Assemblies
runtime errors should a loss of data occur (as you have seen at the opening of this chapter).
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Now, assume for a moment that C# did not honor the use of the () operator. This would be nasty, as you
Part Four - Leveraging the .NET Libraries
would be required to call various member functions in order to convert between types. For example:
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
int a = 123;
Chapter 14 - A Better Painting Framework (GDI+) long b = a;
Chapter 15 - Programming with Windows Forms Controls
int c = ((IConvertible)b).ToInt32(CultureInfo.CurrentCulture); // Yuck...
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Recall: Conversions Among Related Class Types
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
As seen in Chapter 4, class types may be related by classical inheritance (the "is-a" relationship). In this
Chapter 20 - XML Web Services
case, the C# conversion process allows you to cast up and down the class hierarchy. For example, a
Index
derived class can always be implicitly cast into a given base type. However, if you wish to store a base
List of Figures
class type in a derived variable, you must perform an explicit cast:
List of Tables
// Implicit cast between derived to base.
Base myBaseType;
myBaseType = new Derived();
//Must explicitly cast to store base reference
//in derived type.
Derived myDerivedType = (Derived)myBaseType;
This explicit cast works due to the fact that the Base and Derived classes are related by classical inheritance. However, what if you had two class types in different class hierarchies that require conversions? On a related note, consider structure types. Assume you have two .NET structures named Square and Rectangle. Given that structures cannot leverage classic inheritance, you have no natural way to cast between these seemingly related types.
While you could build custom methods (such as Square.ToRectangle() or Rectangle.ToSquare()), C#

allows you to build custom conversion routines that allow your types to respond to the () operator.
C# and the .NET Platform, Second Edition
Therefore, if you configured the Square type correctly, you would be able to use the following syntax to
by Andrew Troelsen ISBN:1590590554 explicitly convert between types:
|
Apress © 2003 (1200 pages) |
// Convert |
This comprehensive text starts with a brief overview of the |
a C#Squarelanguagetoanda thenRectangle.quickly moves to key technical and |
|
Square sq; |
architectural issues for .NET developers. |
sq.sideLength = 3;
Rectangle rect = (Rectangle)sq;
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Creating CustomC# and theConversion.NET Platform, SecRoutinesnd Edition
by Andrew Troelsen |
ISBN:1590590554 |
Now that you have a better feel about how it would look to interact with a type using a custom conversion
Apress © 2003 (1200 pages)
routine, let's see how to equip the type itself. C# provides two keywords ("explicit" and "implicit") that are
This comprehensive text starts with a brief overview of the
used to control howC# yourlanguagetypesandrespondthen quicklyduringmovesan attemptedto key techconversionical and. To begin, assume you have the following structurearchitecdefinitions:ural issues for .NET developers.
public struct Rectangle
Table of Contents
{
C# and the .NET Platform, Second Edition public int width, height;
Introduction
public void Draw()
Part One - Introducing C# and the .NET Platform
{ Console.WriteLine("Drawing a rect.");}
Chapter 1 - The Philosophy of .NET
// Rectangles can be explicitly converted
Chapter 2 - Building C# Applications
// into squares using the () syntax.
Part Two - The C# Programming Language
public static explicit operator Rectangle(Square s)
Chapter{3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Rectangle r;
Chapter 5 -rExc.heightptions and= sObject.sideLength;Lif time
Chapter 6 -rInterfaces.width and= s.CollectionssideLength;
Chapter 7 -returnCallback Interfaces,r; Delegates, and Events
Chapter}8 - Advanced C# Type Construction Techniques
public override string ToString()
Part Three - Programming with .NET Assemblies
Chapter{9 - Understanding .NET Assemblies
return string.Format("[Width = {0}; Height = {1}]",
Chapter 10 - Processes, AppDomains, Contexts, and Threads
width, height);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
}
Part Four - Leveraging the .NET Libraries
}
Chapter 12 - Object Serialization and the .NET Remoting Layer
public struct Square
Chapter 13 - Building a Better Window (Introducing Windows Forms)
{
Chapter 14 - A Better Painting Framework (GDI+) public int sideLength;
Chapter 15 - Programming with Windows Forms Controls public void Draw()
Chapter 16 - The System.IO Namespace
{ Console.WriteLine("Drawing a square.");}
Chapter 17 - Data Access with ADO.NET
public override string ToString()
Part Five - Web Applications and XML Web Services
{
Chapter 18 - ASP.NET Web Pages and Web Controls
return string.Format("[SideLength = {0}]", sideLength);
Chapter}19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
}
Index
List of Figures
Notice that the Rectangle type defines an explicit conversion operator. Like the process of overloading an
List of Tables
operator, conversion routines make use of the C# operator keyword (in conjunction with the "explicit" or "implicit" keyword), and must be defined as static. The incoming parameter is the entity you are converting from while the return value is the entity you are converting to:
public static explicit operator Rectangle(Square s)
{...}
In any case, the assumption is that a square (being a geometric pattern in which all sides are of equal length) can be transformed into a rectangle, provided that the dimensions remain identical. Thus, you are free to convert a Square into a Rectangle in the following ways:
// Create a 10 * 10 square.
Square sq;
sq.sideLength = 3;

Console.WriteLine("sq = {0}", sq);
C# and the .NET Platform, Second Edition
// Convert Square to a new Rectangle.
by Andrew Troelsen |
ISBN:1590590554 |
Rectangle rect = (Rectangle)sq; |
|
Apress © 2003 (1200 pages) |
|
Console.WriteLine("rect = {0}", rect); |
|
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and architectural issues for .NET developers.
While it may not be all that helpful to convert your Square into a Rectangle within the same scope,
assume you have a function that has been prototyped to take Rectangle types. Using your explicit
Table of Contents
conversion operation, you can safely pass in Square types for processing:
C# and the .NET Platform, Second Edition
Introduction
private static void DrawThisRect(Rectangle r)
Part One - Introducing C# and the .NET Platform
{r.Draw();}
Chapter 1 - The Philosophy of .NET
...
Chapter 2 - Building C# Applications
// Meanwhile, back in Main()...
Part Two - The C# Programming Language
DrawThisRect((Rectangle)sq);
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Explicit Conversion Operators for the Square Type
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Now that you can explicitly convert squares into rectangles, let's update the Square structure to support a
Chapter 8 - Advanced C# Type Construction Techniques
few conversions of its own. Given that a square is symmetrical on each side, it might be helpful to provide
Part Three - Programming with .NET Assemblies
an explicit conversion routine that allows the caller to cast from a System.Int32 type into a Square (which,
Chapter 9 - Understanding .NET Assemblies
of course, will have a side length equal to the incoming integer). Likewise, what if you were to update
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Square such that the caller can cast from a Square into a System.Int32? Here is the calling logic:
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
// Converting System.Int32 to Square.
Chapter 12 - Object Serialization and the .NET Remoting Layer
Square sq2 = (Square)90;
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Console.WriteLine("sq2 = {0}", sq2);
Chapter 14 - A Better Painting Framework (GDI+)
// Convert a Square to a System.Int32.
Chapter 15 - Programming with Windows Forms Controls int side = (int)sq2;
Chapter 16 - The System.IO Namespace
Console.WriteLine("Side of sq2 = {0}", side);
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
And here is the update to the Square type:
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapterpublic20struct- XML WebSquareServices
{
Index
public int sideLength;
List of Figures
public void Draw()
List of Tables
{ Console.WriteLine("Drawing a square.");} public override string ToString()
{
return string.Format("[SideLength = {0}]", sideLength);
}
public static explicit operator Square(int sideLength)
{
Square newSq;
newSq.sideLength = sideLength; return newSq;
}
public static explicit operator int (Square s) {return s.sideLength; }
}
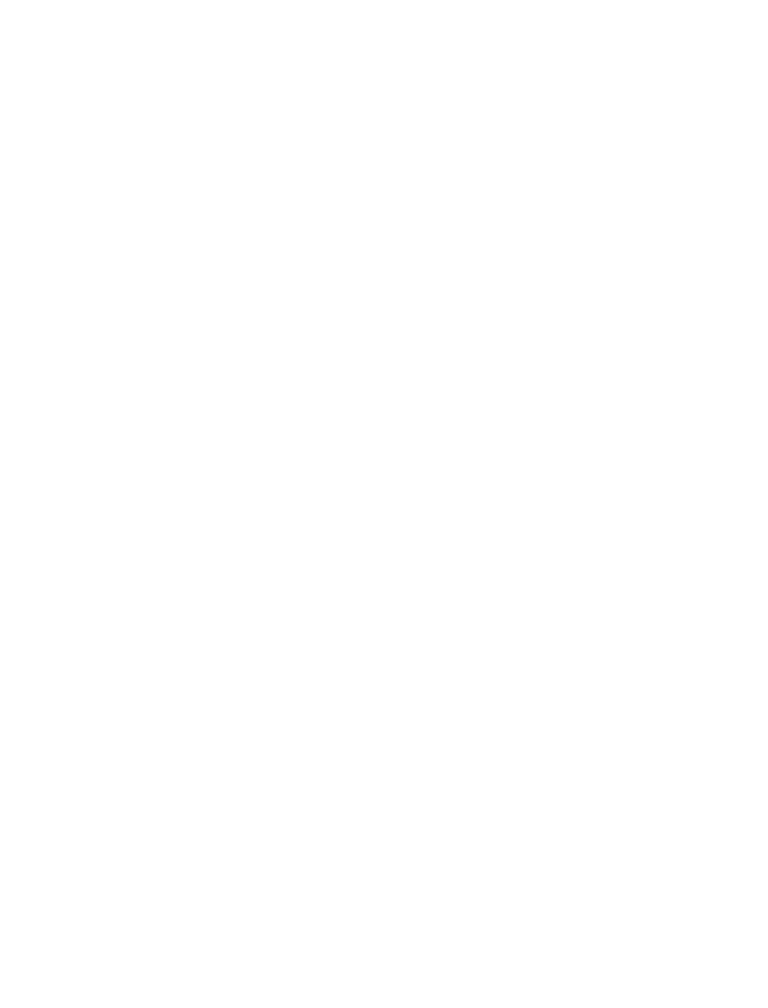
Wild, huh? To be honest, the need to convert from a Square into a System.Int32 may not be the most
C# and the .NET Platform, Second Edition |
|
intuitive (or useful) operation. However, this does point out a very important fact regarding custom |
|
by Andrew Troelsen |
ISBN:1590590554 |
conversion routines: The compiler does not care what you convert to or from, as long as you have written
Apress © 2003 (1200 pages)
syntactically correct code. Thus, as with overloading operators, just because you can create an explicit
This comprehensive text starts with a brief overview of the
cast operation for a given type does not mean you should. Typically this technique will be most helpful
C# language and then quickly moves to key technical and
when creating .NET structure types, given that they are unable to participate in classical inheritance architectural issues for .NET developers.
(where casting comes for free).
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
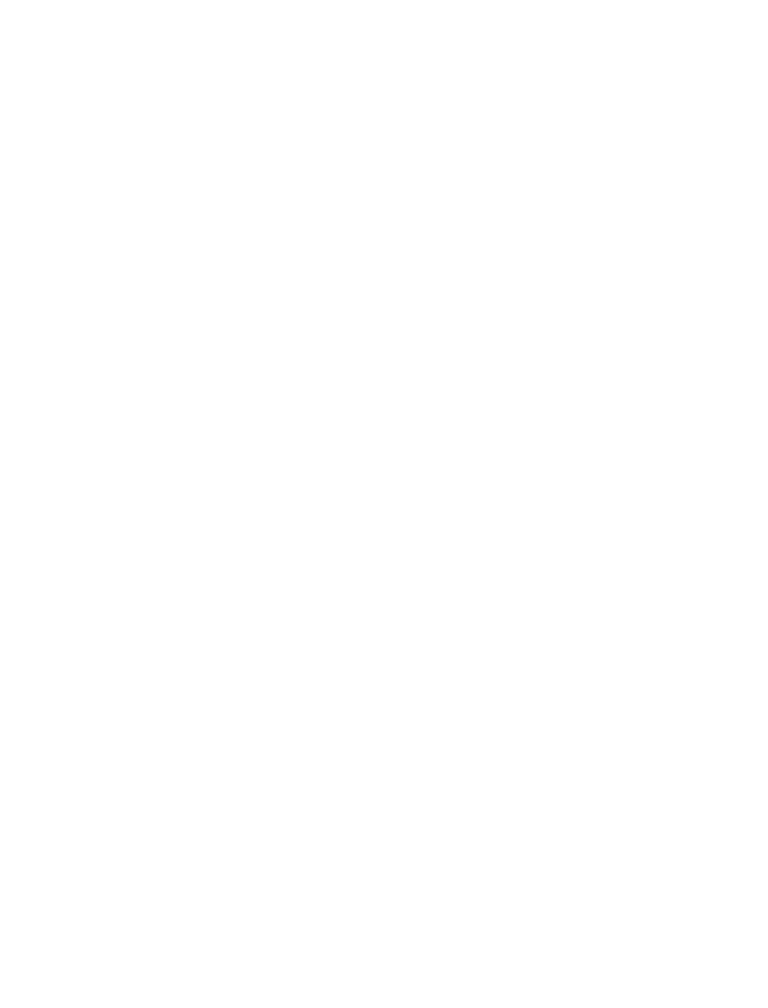
Defining ImplicitC# and theConversion.NET Platf rm,RoutinesSecond Edition
by Andrew Troelsen |
ISBN:1590590554 |
Thus far, you have equipped your Rectangle type to honor explicit conversions between Square types.
Apress © 2003 (1200 pages)
However, what about the following implicit conversion?
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
architectural issues for .NET developers.
// Attempt to make an implicit cast.
Square s3;
s3.sideLength= 83;
Table of Contents
Rectangle rect2 = s3;
C# and the .NET Platform, Second Edition
Introduction
PartAs youOnemight- Introducingexpect, C#thisandcodethewill.NETnotPlatformcompile, given that you have not provided an implicit conversion Chapterroutine1for-theTheRectanglePhilosophytypeof ..NETNow here is the catch: It is illegal to define explicit and implicit conversion
Chapterfunctions2 on- Buildingthe sameC#type,A plicationsif they do not differ by their return type or parameter set. This might seem like
Partlimitation;Two - Thehowever,C# Programmingthe secondLanguagecatch is that when a type defines an implicit conversion routine, it is legal
Chapterfor the 3caller- C#toLanguagemake useFundamof the explicitntals cast syntax!
Chapter 4 - Object-Oriented Programming with C#
Confused? To clear things up, let's begin by modifying the existing conversion routine for the Rectangle to
Chapter 5 - Exceptions and Object Lifetime
make use of the C# "implicit" keyword:
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
public static implicit operator Rectangle(Square s)
Chapter 8 - Advanced C# Type Construction Techniques
{
Part Three - Programming with .NET Assemblies
Rectangle r;
Chapter 9 - Understanding .NET Assemblies
r.height = s.sideLength;
Chapter 10 - Processes, AppDomains, Contexts, and Threads r.width = s.sideLength;
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming return r;
Part Four - Leveraging the .NET Libraries
}
Chapter 12 - Object Serialization and the .NET Remoting Layer Chapter 13 - Building a Better Window (Introducing Windows Forms)
ChapterWith this14update,- A B tteryouPareintinowg Frablem wtorkconvert(GDI+)between types as follows:
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
// Implicit cast OK!
Chapter 17 - Data Access with ADO.NET
Square s3;
Part Five - Web Applications and XML Web Services
s3.sideLength= 83;
Chapter 18 - ASP.NET Web Pages and Web Controls
Rectangle rect2 = s3;
ChapterConsole19.-WriteLine("rect2ASP.NET b Applications= {0}", rect2);
ChapterDrawThisRect(s3);20 - XML Web Services
Index// Explicit cast syntax still OK!
Square s4;
List of Figures
s4.sideLength = 3;
List of Tables
Rectangle rect3 = (Rectangle)s4;
Console.WriteLine("rect3 = {0}", rect3);
Do be aware that it is permissible to define explicit and implicit conversion routines for the same type as long as their signatures differ. Thus, you would update the Square as follows:
public struct Square
{
//Can call as:
//Square sq2 = (Square)90;
//or as:
//Square sq2 = 90;
public static implicit operator Square(int sideLength)
{

Square newSq;
C# and the .NET Platform, Second Edition newSq.sideLength = sideLength;
by Andrew Troelsen |
ISBN:1590590554 |
return newSq; |
|
}Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the
//Must call as:
C# language and then quickly moves to key technical and
// int side = (Square)mySquare; architectural issues for .NET developers.
public static explicit operator int (Square s)
{ return s.sideLength; }
}
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
SOURCE The UserDefinedConversions project is located under the Chapter 8 subdirectory.
Chapter 1 - The Philosophy of .NET
CODE
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

The InternalC#Representationand the .NET Platf rm,ofSecondCustomEditionConversion Routines
by Andrew Troelsen |
ISBN:1590590554 |
Before moving on, you have one more conversion detail to contend with. Like overloaded operators,
Apress © 2003 (1200 pages)
methods that are qualified with the "implicit" or "explicit" keywords have "special" names in terms of CIL:
This comprehensive text starts with a brief overview of the op_implicit and opC#_explicit,languagerespectivelyand then quickly(Figuremoves8-4). to key technical and
architectural issues for .NET developers.
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter |
C# |
Chapter
Chapter
Figure 8-4: CIL representation of user-defined conversion routines
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
PartAs youThreemight- Programmingimagine, youwithcannot.NET guaranteeAssembli sthat a given .NET language will be able to interact with a Chaptertype's set9 of- Understandicustom conversiong .NET Assembliesfunctions, given that the () cast operator is a C#-ism. However, you would
Chapterstill be 10able- toProcesses,interact withAppDomains,these membersContexts,usinga d theirThreadsunderlying CIL representation:
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Module UserConvApp
Chapter 12 - Object Serialization and the .NET Remoting Layer
Sub Main()
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Dim s As Square
Chapter 14 - A sBetter.sideLengthPainting Framework= 83 (GDI+)
Chapter 15 - ProgrammingDim rect withAs WindowsRectangleForms=ControlsRectangle.op_Implicit(s)
Chapter 16 - TheConsoleSy tem..IOWriteLine("rectNamespace = {0}", rect)
End Sub
Chapter 17 - Data Access with ADO.NET
End Module
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
That wraps up our examination of defining custom conversion routines (and this chapter). Remember that
Chapter 20 - XML Web Services
this bit of syntax is simply a shorthand notation for "normal" member functions, and in this light is always
Index optional.
List of Figures
List ofSOURCETables The UserConvLib and VbNetUserConvClient projects are located under the Chapter 8
CODE subdirectory.