
- •Table of Contents
- •C# and the .NET Platform, Second Edition
- •Introduction
- •Part One: Introducing C# and the .NET Platform
- •Part Two: The C# Programming Language
- •Part Three: Programming with .NET Assemblies
- •Part Four: Leveraging the .NET Libraries
- •Part Five: Web Applications and XML Web Services
- •Obtaining This Book's Source Code
- •The .NET Solution
- •What C# Brings to the Table
- •The Role of the Assembly Manifest
- •Summary
- •Chapter 2: Building C# Applications
- •Summary
- •Chapter 3: C# Language Fundamentals
- •Defining Program Constants
- •Defining Custom Class Methods
- •C# Enumerations
- •Summary
- •The Second Pillar: C#'s Inheritance Support
- •Summary
- •Catching Exceptions
- •Finalizing a Type
- •Garbage Collection Optimizations
- •Summary
- •Chapter 6: Interfaces and Collections
- •Building Comparable Objects (IComparable)
- •Summary
- •Summary
- •Internal Representation of Type Indexers
- •Summary
- •An Overview of .NET Assemblies
- •Understanding Delayed Signing
- •Using a Shared Assembly
- •GAC Internals
- •Summary
- •Spawning Secondary Threads
- •A More Elaborate Threading Example
- •Summary
- •Summary
- •Object Persistence in the .NET Framework
- •The .NET Remoting Namespaces
- •Understanding the .NET Remoting Framework
- •All Together Now!
- •Terms of the .NET Remoting Trade
- •Testing the Remoting Application
- •Revisiting the Activation Mode of WKO Types
- •Deploying the Server to a Remote Machine
- •Summary
- •Control Events
- •The Form Class
- •Summary
- •Regarding the Disposal of System.Drawing Types
- •Understanding the Graphics Class
- •Summary
- •The TextBox Control
- •Working with Panel Controls
- •Configuring a Control's Anchoring Behavior
- •Summary
- •Chapter 16: The System.IO Namespace
- •The Static Members of the Directory Class
- •The Abstract Stream Class
- •Summary
- •The Role of ADO.NET Data Providers
- •The Types of System.Data
- •Selecting a Data Provider
- •The Types of the System.Data.OleDb Namespace
- •Working with the OleDbDataReader
- •Summary
- •Submitting the Form Data (GET and POST)
- •Some Benefits of ASP.NET
- •Creating an ASP.NET Web Application by Hand
- •The Composition of an ASP.NET Page
- •The Derivation of an ASP.NET Page
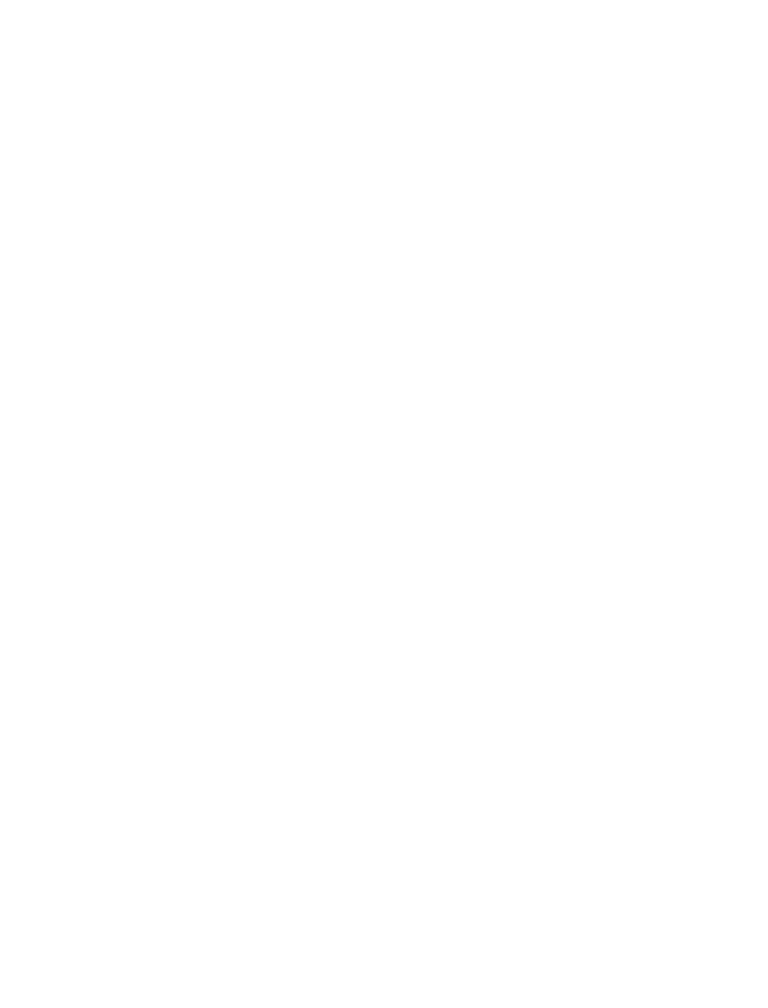
Summary C# and the .NET Platform, Second Edition
by Andrew Troelsen ISBN:1590590554
If you already come to the universe of .NET from another object-oriented language, this chapter may have
Apress © 2003 (1200 pages)
been more of a quick compare and contrast between your current language of choice and C#. On the
This comprehensive text starts with a brief overview of the
other hand, thoseC#oflanguageyou who arend exploringthen quicklyOOPmovesfor theto keyfirsttechnicaltime mayandhave found many of the concepts presented here aarchitecturalbit confoundingissues. Regardlessfor .NET developeof yoursbackground,. rest assured that the information presented here is the foundation for any .NET application, and will be reiterated over the course of this text.
Table of Contents
C#Thisandchapterthe .NETbeganPl tform,with aSecondreviewEditionof the pillars of OOP: encapsulation, inheritance, and polymorphism. As
you have seen, C# provides full support for each aspect of object orientation. Encapsulation services can
Introduction
be accounted for using traditional accessor/ mutator methods, type properties, or read-only public fields.
Part One - Introducing C# and the .NET Platform
Inheritance under C# could not be any simpler, given that the language does not provide a specific
Chapter 1 - The Philosophy of .NET
keyword, but rather makes use of the simple colon operator. Last but not least, you have polymorphism,
Chapter 2 - Building C# Applications
which is supported via the "abstract", "virtual", and "new" keywords (as you recall, "new" can be applied to
Part Two - The C# Programming Language
members as well!).
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
The chapter closed by illustrating some built-in tools of VS .NET that can be used to lessen reliance on the
Chapter 5 - Exceptions and Object Lifetime
"keyboard wizard." Again, the mission of this text is not to examine every aspect of VS .NET; however, you
Chapter 6 - Interfaces and Collections
will find numerous examples throughout the remainder of the book.
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Chapter C#5:andExceptionsthe .NET Platform, andSecond EditionObject Lifetime
by Andrew Troelsen ISBN:1590590554
Apress © 2003 (1200 pages)
In the previous chapter, you dug into the details of how C# supports encapsulation services, reuse of code
This comprehensive text starts with a brief overview of the
(inheritance), and polymorphic activity among related classes. The point of this chapter is twofold. First,
C# language and then quickly moves to key technical and
you will come to understand how to handle runtime anomalies in your code base through the use of architectural issues for .NET developers.
structured exception handling. Not only will you learn about the C# keywords that allow you to handle such problems ("try", "catch", "throw", "finally"), but you will also come to understand the distinction between
application-level and system-level exceptions. This discussion will also provide a lead-in to the topic of
Table of Contents
building custom exception types, as well as how to leverage the built-in exception handling functionality of
C# and the .NET Platform, Second Edition
Visual Studio .NET.
Introduction
Part One - Introducing C# and the .NET Platform
The second half of the chapter will examine the process of object lifetime management as handled by the
Chapter 1 - The Philosophy of .NET
CLR. As you will see, the .NET runtime destroys objects in a rather nondeterministic nature. Thus, you
Chapter 2 - Building C# Applications
typically do not know when a given object will be deallocated from the managed heap, only that it will Part(eventually)Two - ThecomeC# Prtogrpassmming. On Languagethis note, you will come to understand how the System.Object.Finalize() Chaptermethod3and- C#IDisposableLanguage interfaceFund m ntcanlsbe used to interact with an object's lifetime management. Finally,
Chapterwe wrap4 up- Objectwith an-OrientedexaminationPr grammingof the Systemwith C#.GC type, and illustrate a number of ways you are able to
Chapter"get involved"5 - Exceptionswith the garbageand Obj collectionLifetime process.
Chapter 6 - Interfaces and Collections
ChaptOder 7to-Errors,Callback InterfaceBugs,, Delegates,and Exceptionsand Events
Chapter 8 - Advanced C# Type Construction Techniques
PartDespiteThr ewhat- Programmiour (oftenginflated)with .NETegosAs emblimay tells us, no programmer is perfect. Writing software is a complex Chapterundertaking,9 - Understandinggiven this.NETcomplexity,Assembliesit is quite common for even the best software to ship with various
"problems." Sometimes the problem is caused by "bad code" (such as overflowing the bounds of an
Chapter 10 - Processes, AppDomains, Context , and Threads
array). Other times, a problem is caused by bogus user input that has not been accounted for in the
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
application's code base (e.g., a phone number field assigned "Chucky"). Now, regardless of the cause of
Part Four - Leveraging the .NET Libraries
said problem, the end result is that your application does not work as expected. To help frame the
Chapter 12 - Object Serialization and the .NET Remoting Layer
upcoming discussion of structured exception handling, allow me to provide definitions for three common
Chapter 13 - Building a Better Window (Introducing Windows Forms)
anomaly-centric terms:
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Bugs: This is, simply put, an error on the part of the programmer. For example, assume you are
Chapter 16 - The System.IO Namespace
programming with unmanaged C++. If you make calls on a NULL pointer, overflow the bounds of an
Chapter 17 - Data Access with ADO.NET
array, or fail to delete allocated memory (resulting in a memory leak), you have a bug.
Part Five - Web Applications and XML Web Services
Errors: Unlike bugs, errors are typically caused by the end user of the application, rather than by those
Chapter 18 - ASP.NET Web Pages and Web Controls
who created said application. For example, an end user who enters a malformed string into a text box
Chapter 19 - ASP.NET Web Applications
that requires a Social Security number could very well generate an error if you fail to trap this faulty
Chapter 20 - XML Web Services Indexinput in your code base.
List of Figures
Exceptions: Exceptions are typically regarded as runtime anomalies that are difficult, if not impossible,
List of Tables
to prevent. Possible exceptions include attempting to connect to a database that no longer exists, opening a corrupted file, or contacting a machine that is currently offline. In each of these cases, the programmer (and end user) has little control over these "exceptional" circumstances.
Given the previous definitions, it should be clear that .NET structured exception handling is a technique best suited to deal with runtime exceptions. However, as for the bugs and errors that have escaped your view, do be aware that the CLR will generate a corresponding exception that identifies the problem at hand. As you will see, the .NET base class libraries define exceptions such as OutOfMemoryException, IndexOutOfRangeException, FileNotFoundException, ArgumentOutOfRangeException, and so forth.
Before we get too far ahead of ourselves, let's formalize the role of structured exception handling and check out how it differs from traditional error handling techniques.
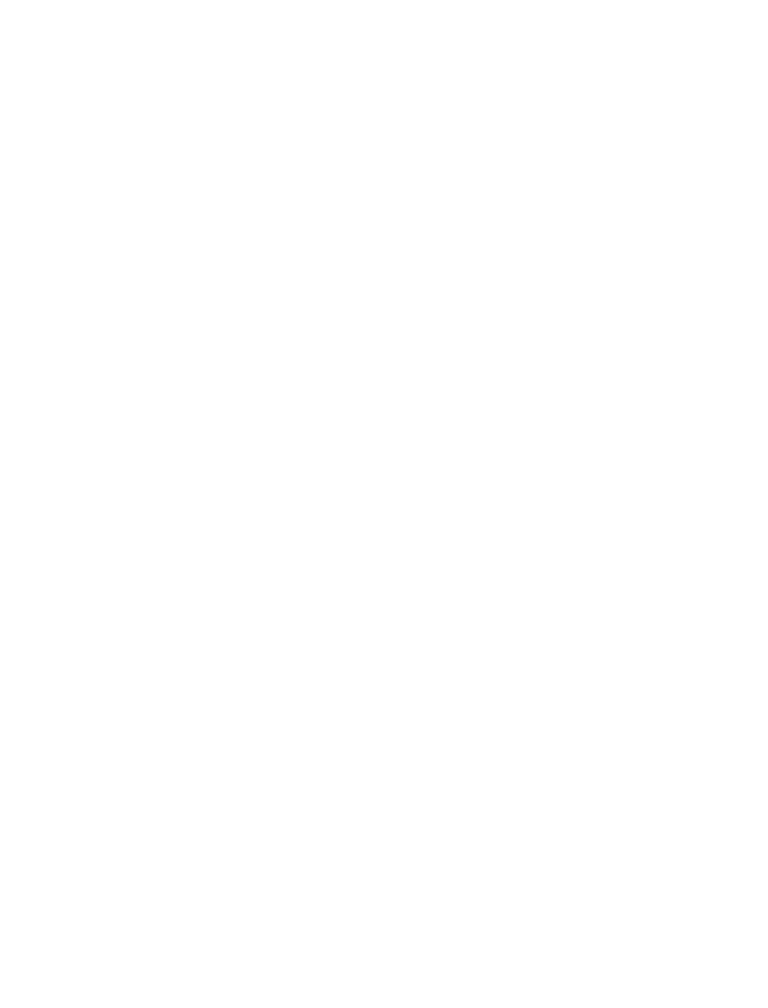
The Role ofC#.NETand theException.NET Platform,HandlingSecond Edition
by Andrew Troelsen |
ISBN:1590590554 |
Error handling among Windows developers has grown into a confused mishmash of techniques over the
Apress © 2003 (1200 pages)
years. Many programmers roll their own error handling logic within the context of a given application. For
This comprehensive text starts with a brief overview of the
example, a developmentC# languageteamandmaythendefinequicklya setmovesof numericalto key technicalconstantsandthat represent known error conditions, and makearchitecturaluse of themissuesasformethod.NET evelopersreturn values. . For example, ponder the following C code:
/* A very C-style error trapping mechanism. */
Table of Contents
#defineE_FILENOTFOUND 1000
C# and the .NET Platform, Second Edition
Introduction
int SomeFunction()
Part One - Introducing C# and the .NET Platform
{
Chapter 1 - The Philosophy of .NET
// Assume something happens in this f(x)
Chapter 2 - Building C# Applications
// which causes the following return value.
Part Two - The C# Programming Language
return E_FILENOTFOUND;
Chapter 3 - C# Language Fundamentals
}
Chapter 4 - Object-Oriented Programming with C#
Chaptervoid Main()5 - Exceptions and Object Lifetime
Chapter{ 6 - Interfaces and Collections
Chapterint7 -retValCallb ck Interfac= SomeFunction();s, Delegates, and Events
if(retVal == E_FILENOTFOUND)
Chapter 8 - Adv nced C# Type Construction Techniques
{ printf("Can not find file...");}
Part Three - Programming with .NET Assemblies
}
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
This approach is less than ideal, given the fact that the constant E_FILENOTFOUND is little more than a
Part Four - Leveraging the .NET Libraries
raw numerical value, and is far from being a self-describing agent. Ideally, you would like to encapsulate
Chapter 12 - Object Serialization and the .NET Remoting Layer
the name, message, and other helpful information regarding this error condition into a single, well-defined
Chapter 13 - Building a Better Window (Introducing Windows Forms)
package (which is exactly what happens under structured exception handling).
Chapter 14 - A Better Painting Framework (GDI+)
ChapterIn addition15 -toProgrthisadmminghoc technique,with WindowstheFormsWindowsControlsAPI defines hundreds of error codes that come by way of Chapter#defines,16 HRESULTs,- The Systemand.IO Nfarmespacetoo many variations on the simple Boolean (bool, BOOL, VARIANT_BOOL,
and so on). Also, many C++ COM developers (and indirectly, many VB 6.0 COM developers) have made
Chapter 17 - Data Access with ADO.NET
use of a small set of standard COM interfaces (e.g., ISupportErrorInfo, IErrorInfo, ICreateErrorInfo) to
Part Five - Web Applications and XML Web Services
return meaningful error information to a COM client using a COM error object.
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
The obvious problem with these previous techniques is the tremendous lack of symmetry. Each approach
Chapter 20 - XML Web Services
is more or less tailored to a given technology, a given language, and perhaps even a given project. In
Index
order to put an end to this madness, the .NET platform provides exactly one technique to send and trap
List of Figures
runtime errors: structured exception handling (SEH).
List of Tables
The beauty of this approach is that developers now have a well-defined approach to error handling, which is common to all languages targeting the .NET universe. Therefore, the way in which a C# programmer handles errors is conceptually similar to that of a VB .NET programmer, and a C++ programmer using managed extensions (MC++). As an added bonus, the syntax used to throw and catch exceptions across assemblies, AppDomains (defined in Chapter 10), and machine boundaries is identical.
Another bonus of .NET exceptions is the fact that rather than receiving a cryptic numerical value that identifies the problem at hand, exceptions are objects that contain a human-readable description of the problem, as well as a detailed snapshot of the call stack that eventually triggered the exception in the first place. Furthermore, you are able to provide the end user with help link information that points the user to a URL that provides detailed information regarding the error at hand.
The Atoms of .NET Exception Handling
Programming with structured exception handling involves the use of four key (and interrelated) elements:
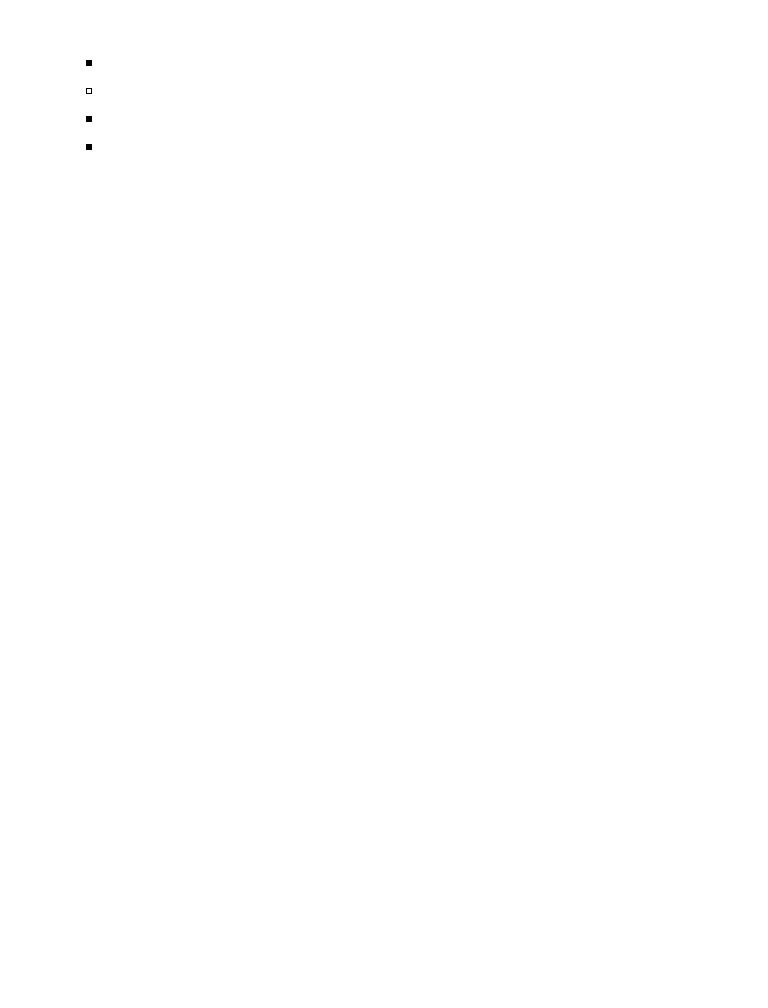
A type that represents the details of the exceptional circumstance
C# and the .NET Platform, Second Edition
|
by Andrew Troels |
ISBN:1590590554 |
|
||
|
A method that throws the exception to the caller |
|
|
Apress © 2003 (1200 pages) |
|
A block of code that will invoke the exception-ready method
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
A block of code that will process (or catch) the exception (should it occur) architectural issu s for .NET developers.
As you will see, the C# programming language offers four keywords ("try", "catch", "throw", and "finally")
that allow you to throw and handle exceptions. The type that represents the problem at hand is a class
Table of Contents
derived from System.Exception (or a descendent thereof). Given this fact, let's check out the role of this
C# and the .NET Platform, Second Edition
exception-centric base class.
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
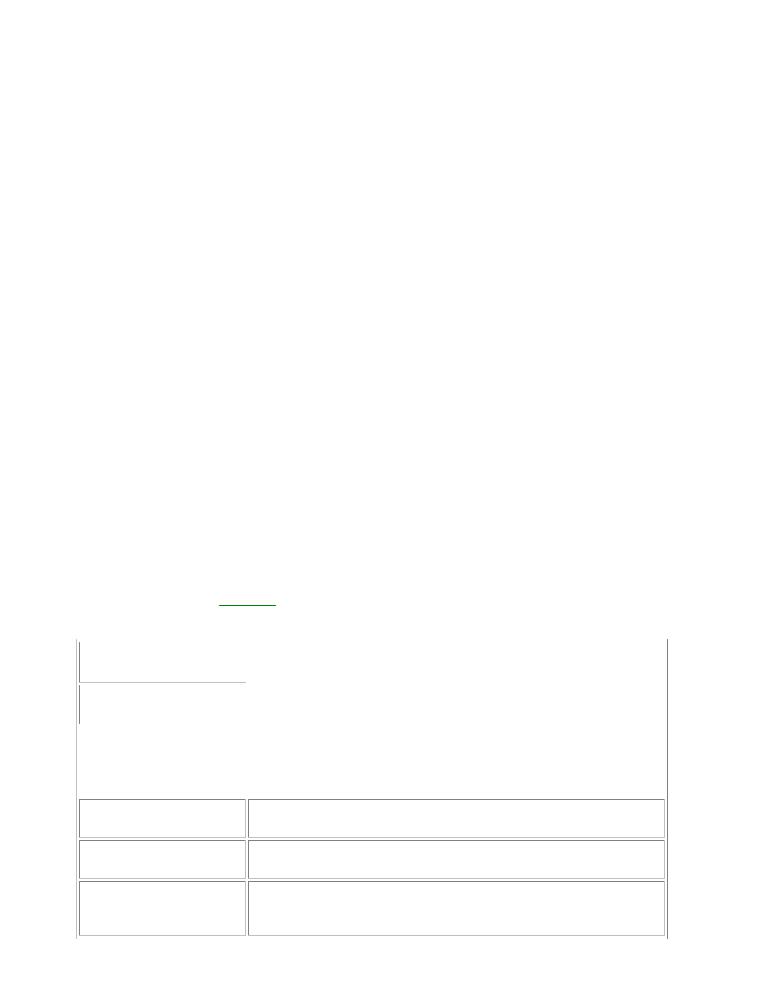
The SystemC#.Exceptionand the .NET Platform,Base ClassSecond Edition
by Andrew Troelsen |
ISBN:1590590554 |
All system-supplied and custom exceptions ultimately derive from the System.Exception base class (which
Apress © 2003 (1200 pages)
in turn derives from System.Object). As you can see from the following C# definition of this type,
This comprehensive text starts with a brief overview of the
System.ExceptionC#alsolanguageimplementsand thena standardquickly movesinterfaceto keynamedtechnicalISerializable;nd however, ignore this fact for the time being (givenarchitecturalthat thisissuesinterfacefor .hasNETmoredev lopersto do.with .NET Remoting than SEH).
public class Exception : object,
Table of Contents
ISerializable
C# and the .NET Platform, Second Edition
{
Introduction
public Exception();
Part One - Introducing C# and the .NET Platform
public Exception(string message);
Chapter 1 - The Philosophy of .NET
public Exception(string message, Exception innerException);
Chapter 2 - Building C# Applications
public string HelpLink { virtual get; virtual set; }
Part Two - The C# Programming Language |
|
public Exception InnerException { get; } |
|
Chapter 3 |
- C# Language Fundamentals |
public string Message { virtual get; } |
|
public string Source { virtual get; virtual set; } |
|
Chapter 4 |
- Object-Oriented Programm ng with C# |
Chapter 5 |
- Except ons and Object Lif time |
public string StackTrace { virtual get; }
Chapterpublic6 - InterfacesMethodBaseand CollectionsTargetSite { get; }
Chapterpublic7 - CallbackvirtualInterfaces,bool Delegates,Equals(objectand Eventsobj);
public virtual Exception GetBaseException();
Chapter 8 - Advanced C# Type Construction Techniques
public virtual int GetHashCode();
Part Three - Programming with .NET Assemblies
public virtual void
Chapter 9 - Understanding .NET Assemblies
GetObjectData(SerializationInfo info, StreamingContext context);
Chapter 10 - Processes, AppDomains, Contexts, and Threads
public Type GetType();
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
public virtual string ToString();
Part Four - Leveraging the .NET Libraries
}
Chapter 12 - Object Serialization and the .NET Remoting Layer Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
As you can see, many of the members defined by System.Exception are read-only in nature. This is due to
Chapter 15 - Programming with Windows Forms Controls
the simple fact that derived types will typically supply default values for each property (for example, the
Chapter 16 - The System.IO Namespace
default message of the IndexOutOfRangeException type is "Index was outside the bounds of the array"). ChapterAs you17would- Dataexpect,Ac essthesewithdefaultADO.NETvalues can be altered and extended with custom information via
PartconstructorFive - WebparametersApplications. Tableand XML5-1 describesWeb Servicesthe core members of System.Exception.
Chapter 18 - ASP.NET Web Pages and Web Controls
|
Table 5-1: Core Members of the System.Exception Type |
||||
Ch pt r 19 - ASP.NET Web Applications |
|||||
|
|
|
|
|
|
|
|
Chapter 20 - XML Web Services |
|
Meaning in Life |
|
IndexSystem.Exception |
|
|
|||
|
|
Property |
|
|
|
List of Figures |
|
|
|
||
|
|
HelpLink |
|
This property returns a URL to a help file describing the error in gory |
|
List of Tables |
|
|
|
||
|
|
|
|
detail. |
|
|
|
|
|
|
|
|
|
InnerException |
|
This read-only property can be used to obtain information about the |
|
|
|
|
|
previous exceptions that caused the current exception to occur. The |
|
|
|
|
|
previous exceptions are documented by passing them into the |
|
|
|
|
|
constructor of the most current exception. |
|
Message
Source
StackTrace
This read-only property returns the textual description of a given error. The error message itself is set as a constructor parameter.
This property returns the name of the assembly that threw the exception.
This read-only property contains a string that identifies the sequence of calls that triggered the exception. As you might guess, this property is very useful during debugging.
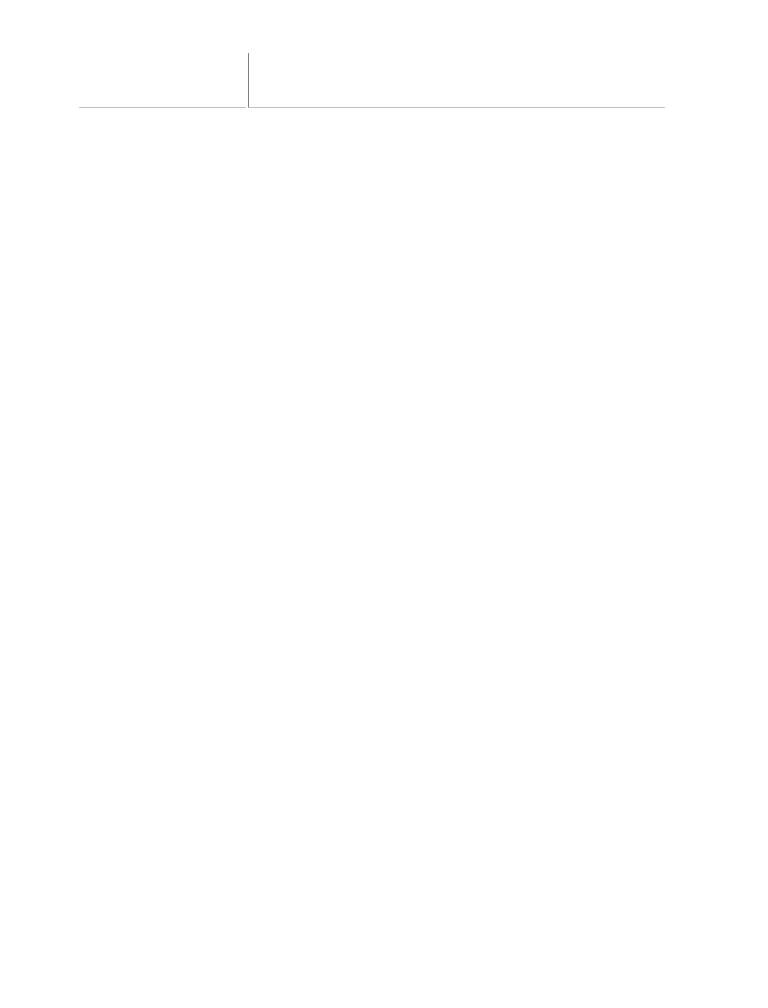
|
TargetSite |
C# and the |
|
.NETThisPlatform,read-onlySecondpropertyEditionreturns a MethodBase type, which describes |
|
|
|
|
by Andrew |
|
numerous details about the method that threw the exception |
|
|
|
|
|
Troelsen |
ISBN:1590590554 |
|
|
|
|
Apress © 2003 |
|
(ToString() will identify the method by name). |
|
|
|
|
|
(1200 pages) |
|
|
|
|
|
This comprehensive text starts with a brief overview of the |
||||
|
|
C# language and then quickly moves to key technical and |
||||
|
|
architectural issues for .NET developers. |
|
|
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Throwing aC#Genericand the .NExceptionT Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
To illustrate the simplest use of System.Exception, let's revisit the Car class defined in Chapter 4, in
Apress © 2003 (1200 pages)
particular, the SpeedUp() method. Here is current implementation:
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and
architectural issues for .NET developers.
// Currently, SpeedUp() reports errors using console IO. public void SpeedUp(int delta)
{
Table of Contents
// If the car is dead, just say so...
C# and the .NET Platform, Second Edition if(carIsDead)
Introduction |
Console.WriteLine("{0} is out of order....", petName); |
|||
Part One - Introducing C# and the .NET Platform |
||||
|
else |
// Not dead, speed up. |
||
Chapter |
1 |
- The Philosophy of .NET |
||
|
{ |
|
|
|
Chapter |
2 |
- Building C# Applications |
||
|
|
|
currSpeed += delta; |
|
Part Two - The C# Programming Language |
||||
|
|
|
if(currSpeed >= maxSpeed) |
|
Chapter |
3 |
- C# Language Fundamentals |
||
|
|
|
{ |
|
Chapter |
4 |
- Object-Oriented Programming with C# |
||
|
|
|
Console.WriteLine("{0} has overheated...", petName); |
|
Chapter |
5 |
- Exceptions and Object Lifetime |
||
|
|
|
carIsDead = true; |
|
Chapter |
6 |
- Interfaces} |
and Collections |
|
Chapter |
7 |
- Callbackelse Interfaces, Delegates, and Events |
||
Chapter |
8 |
- AdvancedConsole.WriteLine("=>C# Type Construction TechniquesCurrSpeed = {0}", currSpeed); |
||
Part Three} - Programming with .NET Assemblies |
||||
Chapter} |
9 |
- Understanding .NET Assemblies |
||
Chapter |
10 |
- Processes, AppDomains, Contexts, and Threads |
||
Chapter |
11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
Now, let's retrofit SpeedUp() to throw an exception if the user attempts to speed up the automobile after it
Part Four - Leveraging the .NET Libraries
has met its maker (carIsDead == true). First, you want to create and configure a new instance of the
Chapter 12 - Object Serialization and the .NET Remoting Layer
System.Exception class, setting the value of the read-only Message property via the class constructor.
Chapter 13 - Building a Better Window (Introducing Windows Forms)
When you wish to send the error object back to the caller, make use of the C# "throw" keyword. Here is
Chapter 14 - A Better Painting Framework (GDI+) the relevant code update:
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
// This time, throw an exception if the user speeds up a trashed automobile.
Chapter 17 - Data Access with ADO.NET public void SpeedUp(int delta)
Part Five - Web Applications and XML Web Services
{
Chapter 18 - ASP.NET Web Pages and Web Controls
if(carIsDead)
Chapter 19 - ASP.NET Web Applications
throw new Exception("This car is already dead...");
Chapter 20 - XML Web Services
else
Index { ... }
List} of Figures
List of Tables
Before examining how to catch this exception, a few points of interest. First of all, when you are building a custom type, it is always up to you to decide exactly what constitutes an exception, and when it should be thrown. Here, you are making the assumption that if the program attempts to increase the speed of a car that has expired, a System.Exception type should be thrown to indicate the SpeedUp() method cannot continue (which may or may not be a valid assumption).
Alternatively, you could implement SpeedUp() to recover automatically without needing to throw an exception in the first place. By and large, exceptions should be thrown only when a more terminal condition has been met (for example, the inability to allocate a block of unmanaged memory, not finding a necessary file, failing to connect to a database, and whatnot). Deciding exactly what constitutes throwing an exception is a design issue you must always contend with. For our current purposes, assume that asking a doomed automobile to increase its speed justifies a cause for an exception.