
- •Table of Contents
- •C# and the .NET Platform, Second Edition
- •Introduction
- •Part One: Introducing C# and the .NET Platform
- •Part Two: The C# Programming Language
- •Part Three: Programming with .NET Assemblies
- •Part Four: Leveraging the .NET Libraries
- •Part Five: Web Applications and XML Web Services
- •Obtaining This Book's Source Code
- •The .NET Solution
- •What C# Brings to the Table
- •The Role of the Assembly Manifest
- •Summary
- •Chapter 2: Building C# Applications
- •Summary
- •Chapter 3: C# Language Fundamentals
- •Defining Program Constants
- •Defining Custom Class Methods
- •C# Enumerations
- •Summary
- •The Second Pillar: C#'s Inheritance Support
- •Summary
- •Catching Exceptions
- •Finalizing a Type
- •Garbage Collection Optimizations
- •Summary
- •Chapter 6: Interfaces and Collections
- •Building Comparable Objects (IComparable)
- •Summary
- •Summary
- •Internal Representation of Type Indexers
- •Summary
- •An Overview of .NET Assemblies
- •Understanding Delayed Signing
- •Using a Shared Assembly
- •GAC Internals
- •Summary
- •Spawning Secondary Threads
- •A More Elaborate Threading Example
- •Summary
- •Summary
- •Object Persistence in the .NET Framework
- •The .NET Remoting Namespaces
- •Understanding the .NET Remoting Framework
- •All Together Now!
- •Terms of the .NET Remoting Trade
- •Testing the Remoting Application
- •Revisiting the Activation Mode of WKO Types
- •Deploying the Server to a Remote Machine
- •Summary
- •Control Events
- •The Form Class
- •Summary
- •Regarding the Disposal of System.Drawing Types
- •Understanding the Graphics Class
- •Summary
- •The TextBox Control
- •Working with Panel Controls
- •Configuring a Control's Anchoring Behavior
- •Summary
- •Chapter 16: The System.IO Namespace
- •The Static Members of the Directory Class
- •The Abstract Stream Class
- •Summary
- •The Role of ADO.NET Data Providers
- •The Types of System.Data
- •Selecting a Data Provider
- •The Types of the System.Data.OleDb Namespace
- •Working with the OleDbDataReader
- •Summary
- •Submitting the Form Data (GET and POST)
- •Some Benefits of ASP.NET
- •Creating an ASP.NET Web Application by Hand
- •The Composition of an ASP.NET Page
- •The Derivation of an ASP.NET Page
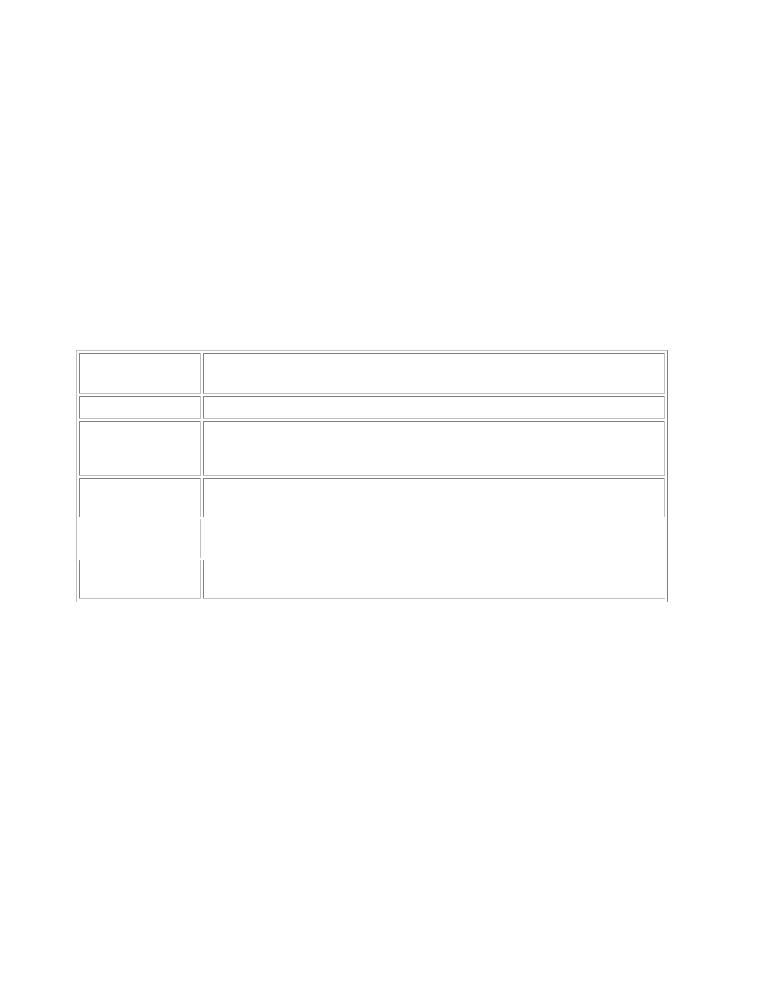
Defining Custom Class Methods |
|
C# and the .NET Platform, Second Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
Now that we have concluded our mid-chapter reprieve, let's move on to more interesting topics and
Apress © 2003 (1200 pages)
examine the details of defining custom methods for a C# class. First understand that, like points of data,
This comprehensive text starts with a brief overview of the
every method youC#implementlanguage mustand thenbe aquicklymembermovesof atoclasskey technicalor struct and(global methods are not allowed in
C#). architectural issues for .NET developers.
As you know, a method exists to allow the type to perform a unit of work. Like Main(), your custom
Tablemethodsof Contentsmay or may not take parameters, may or may not return values (of any intrinsic or user defined C#types)and.theAlso.NETcustomPlatform,methodsSecondmayEditionbe declared nonstatic (instance-level) or static (class-level) entities.
Introduction
PartMethodOne - IntroduAccessing C# andModifiersthe .NET Platform
Chapter 1 - The Philosophy of .NET
ChaptA methodr 2 -mustBuildingspecifyC# itsApplicatilevel ofnsaccessibility (see Table 3-8). C# offers the following method access
PartmodifiersTwo - The(youC#examineProgrammingthe useLanguageof protected and internal methods in the next chapter during the
Chapterdiscussion3 -ofC#classLanguagehierarchies):Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Table 3-8: C# Accessibility Keywords
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections |
|
C# Access |
Meaning in Life |
Chapter 7 - Callback Interfaces, Delegates, and Events
Modifier
Chapter 8 - Advanced C# Type Construction Techniques
PartpublicThree - ProgrammingMarkswith a.NETmethodAssembliesas accessible from an object instance, or any subclass.
Chapter 9 - Understanding .NET Assemblies
private Marks a method as accessible only by the class that has defined the
Chapter 10 - Processes, AppDomains, Contexts, and Threads
method. If you don't say otherwise, private is assumed (it is the default
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming visibility level).
Part Four - Leveraging the .NET Libraries
Chapterprotected12 - Object SerializationMarks aandmethodthe .NETas usableRemotingby theLayerdefining class, as well as any child class, |
||||
|
Chapter 13 - Building a |
|
but is private as far as the outside world is concerned. |
|
|
|
Better Window (Introducing Windows Forms) |
|
|
|
|
|
|
|
|
Chapter 14 - A Better |
|
Painting Framework (GDI+) |
|
|
internal |
|
Defines a method that is publicly accessible by all types in an assembly (but |
|
|
Chapter 15 - Programming |
with Windows Forms Controls |
|
|
|
|
|
not from outside the assembly). |
|
|
|
|
|
|
|
Chapter 16 - The System.IO Namespace |
|
||
|
protected internal |
|
Defines a method whose access is limited to the current assembly or types |
|
|
Chapter 17 - Data Access with ADO.NET |
|
||
|
|
|
derived from the defining class in the current assembly. |
|
Part Five - Web Applications and XML Web Services |
|
|||
|
|
|
|
|
Chapter 18 - ASP.NET Web Pages and Web Controls
Here are the implications of each accessibility keyword:
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
// Visibility options.
Index
class SomeClass
List of Figures
{
List of Tables
// Accessible anywhere.
public void MethodA(){}
// Accessible only from SomeClass types.
private void MethodB(){}
// Accessible from SomeClass and any descendent.
protected void MethodC(){}
// Accessible from within the same assembly.
internal void MethodD(){}
// Assembly-protected access.
protected internal void MethodE(){}
// Unmarked members are private by default in C#.
void MethodF(){}
}
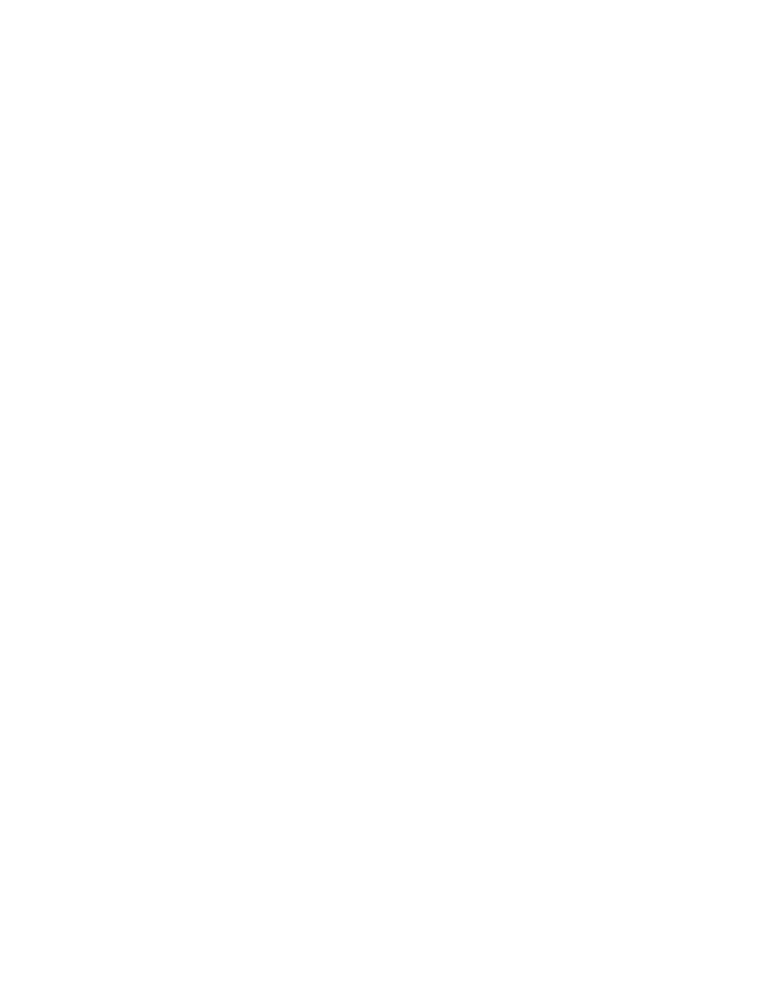
As you may already know, methods that are declared public are directly accessible from an object
C# and the .NET Platform, Second Edition |
|
instance via the dot operator. Private methods cannot be accessed by an object reference, but instead are |
|
by Andrew Troelsen |
ISBN:1590590554 |
called internally by the object to help the instance get its work done (that is, private helper functions). To
Apress © 2003 (1200 pages)
illustrate, the Teenager class shown next defines two public methods, Complain() and BeAgreeable(),
This comprehensivethe text starts with a brief overview of the
each of which returns a string to object user. Internally, both methods make use of a private helper
C# language and then quickly moves to key technical and
method named GetRandomNumber(), which manipulates a private member variable of type architectural issues for .NET developers.
System.Random:
Table// Twoof Contentspublic methods, each using an internal helper function.
using System;
C# and the .NET Platform, Second Edition
class Teenager
Introduction
{
Part One - Introducing C# and the .NET Platform
// The System.Random type generates random numbers.
Chapter 1 - The Philosophy of .NET
private Random r = new Random();
Chapter 2 - Building C# Applications
public string Complain()
Part Two - The C# Programming Language
{
Chapter 3 - C# Language Fundamentals
string[] messages = new string[5]{ "Do I have to?",
Chapter 4 - Object-Oriented Programming with C#
"He started it!", "I'm too tired...",
Chapter 5 - Exceptions and Object Lifetime
"I hate school!", "You are sooo wrong." } ;
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
}
Chapter 8 - Advanced C# Type Construction Techniques
public string BeAgreeable()
Part Three - Programming with .NET Assemblies
{
Chapter 9 - Understanding .NET Assemblies
string[] messages = new string[3]{ "Sure! No problem!",
Chapter 10 - Processes, AppDomains, Contexts, and Threads
"Uh uh.", "I guess so." } ;
Chapter 11 - TypereturnReflection,messages[GetRandomNumber(3)];Late Binding, Attribute-Based Programming
Part Four}- Leveraging the .NET Libraries
Chapter private12 - Obj ctintSerializaGetRandomNumber(shortion the .NET Remoting LayerupperLimit)
Chapter {13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A //BetterRandomPainting.Next()Frameworeturnsk (GDI+) a random integer
// between 0 and upperLimit.
Chapter 15 - Programming with Windows Forms Controls
return r.Next(upperLimit);
Chapter 16 - The System.IO Namespace
}
Chapter 17 - Data Access with ADO.NET
public static void Main(string[] args)
Part Five - Web Applications and XML Web Services
Chapter {18 |
- ASP.NET Web Pages and Web Controls |
|
Chapter 19 |
- ASP.NET// LetWebmikeApplicationsdo his thing. |
Mike says:"); |
Chapter 20 |
- XMLConsole.WriteLine("GrumpyWeb Services |
Index |
Teenager mike = new Teenager(); |
|
List of Figures |
for(int i |
= 0; i < 10; i++) |
List of Tables |
Console.WriteLine("-> {0}", mike.Complain()); |
|
// Now be |
agreeable. |
Console.WriteLine("\nHappy Mike says:");
Console.WriteLine("-> {0}", mike.BeAgreeable());
}
}
Obviously the benefit of defining GetRandomNumber() as a private helper method is that various parts of the Teenager class can make use of its functionality. The only alternative would be to duplicate the random number logic within the Complain() and BeAgreeable() methods (which in this case would not be too traumatic, but assume GetRandomNumber() contains 20 or 30 lines of code). Figure 3-14 shows a possible test run.
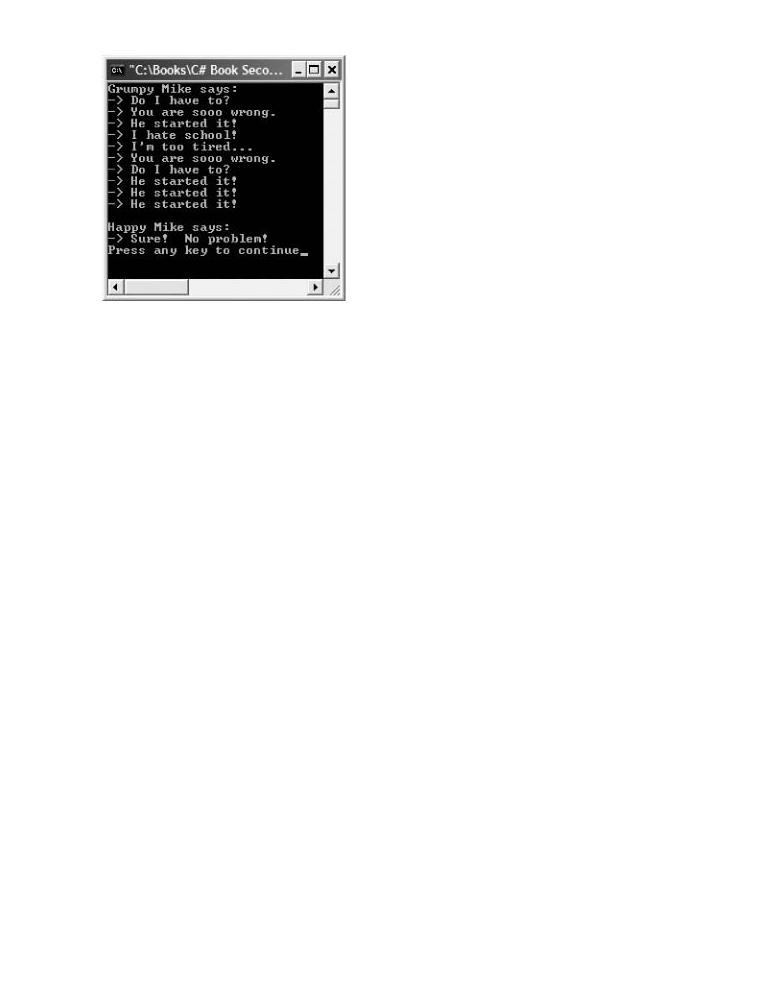
Second Edition
ISBN:1590590554
with a brief overview of the moves to key technical and
developers.
Table
C# and
Part
Chapter
Chapter
Part
ChapterFigure3 - 3C#-14:LanguageRandomFundamentalsteenager chatter
Chapter 4 - Object-Oriented Programming with C#
Note The System.Random type is used to generate and manipulate random numbers.
Chapter 5 - Exceptions and Object Lifetime
Random.Next() method returns a number between 0 and the specified upper limit. As you would
Chapter 6 - Interfaces and Collections
guess, the Random type provides additional members, all of which are documented within
Chapter 7 - Callback Interfaces, Delegates, and Events
MSDN.
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
SOURCE The Teenager application is located under the Chapter 3 subdirectory.
Chapter 9 - Understanding .NET Assemblies
CODE
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

UnderstandingC# andStaticthe .NETMethodsPla form, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
As you have seen, methods can be declared "static." But, what exactly does it mean to be a static method?
Apress © 2003 (1200 pages)
When a method is marked with the "static" keyword, it may be called directly from the class level, and does
This comprehensive text starts with a brief overview of the
not require an objectC# languagevariable.andForthenthis veryquicklyreason,m vesMain()to keyistechnicaldeclaredandstatic to allow the runtime to invoke this function without needingarchitecturalto allocateissues fora new.NETinstancedevelopersof the. defining class. This is a good thing of course, or else you would need to create an object to create an object to create an object to (...).
TableYouofshouldContentsalso consider our good friend System.Console. As you have seen, you do not invoke the
C#WriteLine()and the .NETmethodPlatfofromm, Secondan objectEditioninstance:
Introduction
Part// OneNo!- IntroducingWriteLine()C# andnottheinstance.NET Platformlevel...
ChapterConsole1 -cThe= Philosophynew Console();of .NET
Chapterc.WriteLine("I2 - Building C#can'tApplicationsbe printed...");
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
but instead simply prefix the type name to the static WriteLine() member.
Chapter 4 - Object-Oriented Programming with C# Chapter 5 - Exceptions and Object Lifetime
// Yes! WriteLine() is static...
Chapter 6 - Interfaces and Collections
Console.WriteLine("Thanks...");
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Simply put, static members are methods that are deemed (by the class designer) to be so commonplace that
Chapter 9 - Understanding .NET Assemblies
there is no need to create an instance of the type. When you are designing your custom class types, you are
Chapter 10 - Processes, AppDomains, Contexts, and Threads
also able to define any number of static and/or instance level members. To illustrate custom static methods,
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
assume I have reconfigured the Complain() and BeAgreeable() methods of the Teenager class as follows:
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
// Teenagers complain so often, there is no need to create an initial object...
Chapter 13 - Building a Better Window (Introducing Windows Forms) publicstatic string Complain()
Chapter 14 - A Better Painting Framework (GDI+)
{
Chapter 15 - Programming with Windows Forms Controls
string[] messages = new string[5]{ "Do I have to?",
Chapter 16 - The System.IO Namespace
"He started it!", "I'm too tired...",
Chapter 17 - Data Access with ADO.NET
"I hate school!", "You are sooo wrong." } ;
Part Five - Web Applications and XML Web Services
return messages[GetRandomNumber(5)];
Chapter 18 - ASP.NET Web Pages and Web Controls
}
Chapterpublic19static- ASP.NETstringWeb ApplicationsBeAgreeable()
Chapter{ 20 - XML Web Services
Index string[] messages = new string[3]{ "Sure! No problem!",
"Uh uh.", "I guess so." };
List of Figures
return messages[GetRandomNumber(3)];
List of Tables
}
This update also assumes that the System.Random and GetRandomNumber() helper function method have also been declared as static members of the Teenager class, given the rule that static members can only operate on other static members:
class Teenager
{
private static Random r = new Random();
private static int GetRandomNumber(short upperLimit)
{ return r.Next(upperLimit); }
...
}
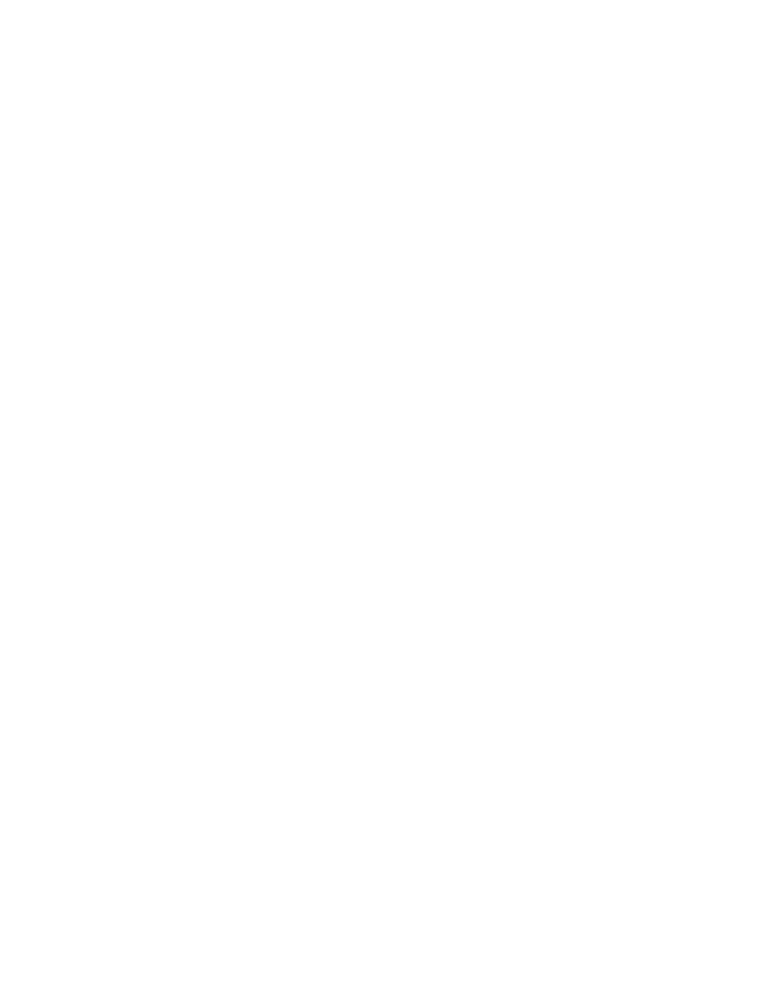
These assumptions aside, the process of calling a static method is simple. Just append the member to the
C# and the .NET Platform, Second Edition name of the defining class:
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
// Call the static Complain method of the Teenager class.
public static void Main(string[] args)
C# language and then quickly moves to key technical and
{architectural issues for .NET developers.
for(int i = 0; i < 40; i++)
Console.WriteLine("-> {0}", Teenager.Complain());
Table} of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Again, by contrast, nonstatic (instance) methods are scoped at the object level. Thus, if Complain() was not
Chapter 1 - The Philosophy of .NET
marked static, you would need to create an instance of the Teenager class before you could hear about the
Chapter 2 - Building C# Applications gripe of the day:
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
// Must make an instance of Teenager class to call instance methods.
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
joe.Complain();
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part ThreeSOURCE- ProgrammingThewithStaticTeenager.NET Assembliesapplication is located under the Chapter 3 subdirectory.
ChapterCODE9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Defining Static Data
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
In addition to static methods, a C# class may also define static data (such as the previous Random member Chvariablepter 13from- Buildingthe TeenagerBett rexample)Window (Introducing. Typically, aWindowsclass definesForm )a set of nonstatic state data. This simply
Chaptermeans14that- eachA BetterobjectPaintinginstanceFrameworkmaintains(GDI+)a private copy of the underlying values. Thus, if you have a class
Chapterdefined15as-follows:Pr gramming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
// We all love Foo.
PartclassFive -FooWeb Applications and XML Web Services
Chapter{ 18 - ASP.NET Web Pages and Web Controls
Chapter public19 - ASP.NETintWebintFoo;Applications
Chapter} 20 - XML Web Services
Index
List of Figures
you can create any number of objects of type Foo and assign the intFoo field to a value to each instance:
List of Tables
// Each Foo reference maintains a copy of the intFoo field. Foo f1 = new Foo();
f1.intFoo = 100; Foo f2 = new Foo(); f2.intFoo = 993; Foo f3 = new Foo(); f3.intFoo = 6;
Static data, on the other hand, is shared among all object instances of the same type. Rather than each object holding a copy of a given field, a point of static data is allocated exactly once for all instances of the type. Assume you have a class named Airplane that contains a single point of static data. In the constructor of the Airplane class you increment this data point. Here is the initial definition:
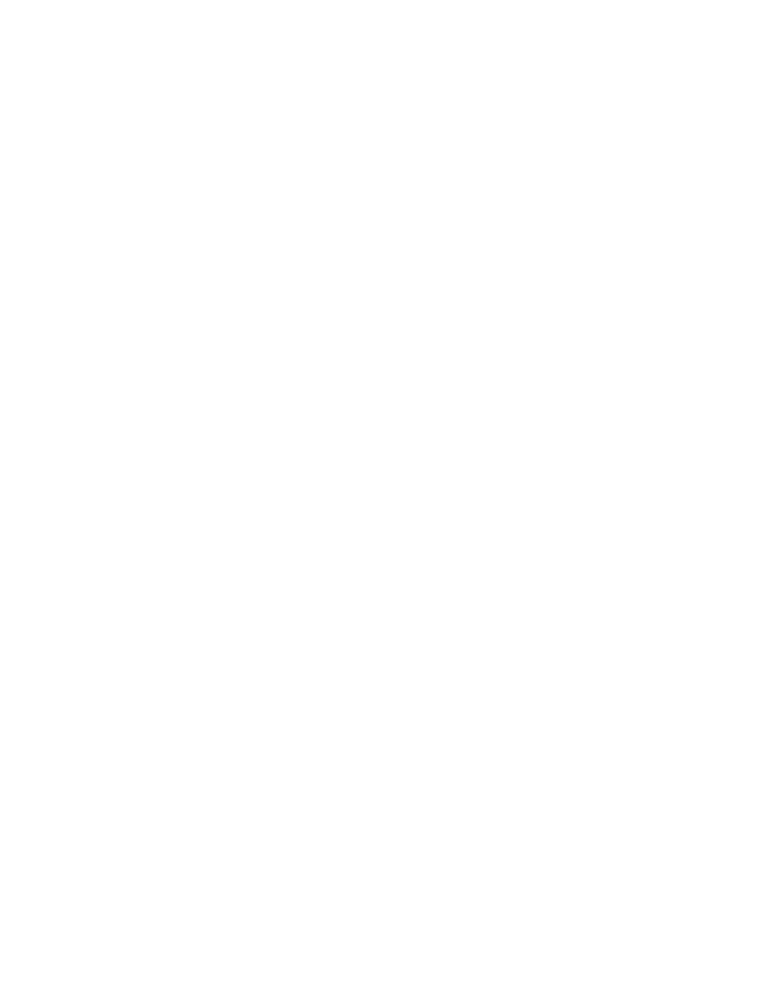
// Note the use of static keyword.
C# and the .NET Platform, Second Edition class Airplane
{ by Andrew Troelsen ISBN:1590590554
Apress © 2003 (1200 pages)
// This static data member is shared by all Airplane objects.
This comprehensive text starts with a brief overview of the private static int NumberInTheAir = 0;
C# language and then quickly moves to key technical and public Airplane()
architectural issues for .NET developers.
{
NumberInTheAir++;
}
Table of Contents
// Get value from an object instance.
C# and the .NET Platform, Second Edition
public int GetNumberFromObject() { return NumberInTheAir; }
Introduction
// Get value from class level.
Part One - Introducing C# and the .NET Platform
public static int GetNumber() { returnNumberInTheAir; }
Chapter 1 - The Philosophy of .NET
}
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
ChapterNotice 3that-theC# AirplaneLanguageclassFundamentalsdefines two methods. The static GetNumber() returns the current number of
Chaairplaneter 4 objects- Objectthat-OrienthavedbeenProgrammingallocatedwithby theC#application. GetNumberFromObject() also returns the value
Chaptof therstatic5 - ExceptionsNumberInTheAirand Objectinteger,Lifetimehowever given that this method has not been defined as static, the Chapterobject user6 - mustInterfacescall thisandmethodCollectionsfrom an instance of Airplane. To illustrate, observe the following usage:
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter// Make8 -someAdvancedairplanesC# Type Constructionare examineTechniquesthe static members.
PartclassThreeStaticApp- Programming with .NET Assemblies
Chapter{ 9 - Understanding .NET Assemblies
Chapter public10 - Procestaticses, AppDomains,int Main(string[]Contexts, and Threadsargs)
{
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging// Makethe some.NET Librariesplanes. |
|
Chapter 12 |
- ObjectConsole.WriteLine("CreatedSerialization and the .NET RemotingtwoLayerAirplane types"); |
Chapter 13 |
- BuildingAirplanea Bettera1Window= new(IntroducingAirplane();Windows Forms) |
Chapter 14 |
- A AirplaneBetter Paintinga2Framework= new Airplane();(GDI+) |
Chapter 15 |
- Programming// How manywith Windowsare in Formsflight?Controls |
Chapter 16 - TheConsole.WriteLine("NumberSystem.IO Namespace |
of planes: {0} ", |
||
|
a1.GetNumberFromObject()); |
||
Chapter 17 - Data Access with ADO.NET |
|
||
Part Five - WebConsole.WriteLine("NumberApplications and XML Web Services of planes: {0} ", |
|||
|
Airplane.GetNumber()); |
||
Chapter 18 - ASP.NET Web Pages and Web Controls |
|
||
|
// More planes! |
|
|
Chapter 19 - ASP.NET Web Applications |
|
||
|
Console.WriteLine("\nCreated two more Airplane types"); |
||
Chapter 20 - XML Web Services |
|
||
Index |
Airplane a3 = new Airplane(); |
||
Airplane a4 = new Airplane(); |
|||
|
|||
List of Figures |
// Now how many? |
|
|
List of Tables |
Console.WriteLine("Number of planes: {0} ", |
a3.GetNumberFromObject()); Console.WriteLine("Number of planes: {0} ",
Airplane.GetNumber()); return 0;
}
}
Figure 3-15 shows the output.

Edition
ISBN:1590590554
brief overview of the
to key technical and
.
Table Figureof Contents3-15: Static data is shared among like objects.
C# and the .NET Platform, Second Edition
Introduction
As you can see, all instances of the Airplane class are sharing (i.e., viewing) the same integer. If one object changes the value (using either static or instance level members) all types 'see' the change. That's the point
of static data: To allow all objects to share a given value at the class (rather than at the object) level.
Chapter 2 - Building C# Applications
Part TwoSOURCE- The C# ProgrammingThe StaticDataLanguageproject is located under the Chapter 3 subdirectory.
ChapterCODE3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Method ParameterC# and the .ModifiersNET Platfo m, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Methods tend to take parameters. If you have a COM background, you are certainly familiar with the use of
Apress © 2003 (1200 pages)
the [in], [out], and [in, out] IDL attributes. Classic COM objects use these attributes to clearly identify the
This comprehensive text starts with a brief overview of the
direction of travelC#(andlanguagememoryandallocationthen quicklyrules)movesfor atogivenk y technicalinterfaceandmethod parameter. While IDL is not used in the .NETarchitecturaluniverse, thereissuesis analogousfor .NET devbehaviorlopers.with the set of C# parameter modifiers shown in Table 3-9.
Table of Contents |
|
|
|
|
|||
|
Table 3-9: C# Parameter Modifiers |
|
|||||
C# and the .NET Platform, Second Edition |
|
|
|
||||
IntroductionParameter |
Meaning in Life |
|
|
|
|||
PartModifierOne - Introducing C# and the .NET Platform |
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
Chapter 1 |
- The Philosophy of .NET |
|
|
|
|
|
|
(none) |
|
If a parameter is not marked with a parameter modifier, it is assumed to be |
|
|
|
|
Chapter 2 |
- Building C# Applications |
|
|
|
||
|
|
|
|
an input parameter passed by value. This is analogous to the IDL [in] |
|
|
|
Part Two - The C# Programming Language |
|
|
|
||||
|
|
|
|
attribute. |
|
|
|
|
Chapter 3 |
- C# Language Fundamentals |
|
|
|
||
|
|
|
|
||||
|
|
out |
|
This is analogous to an IDL [out] parameter. Output parameters are assigned |
|
|
|
|
Chapter 4 |
- Object-Oriented Programming with C# |
|
|
|
||
|
|
|
|
by the called member. |
|
|
|
|
Chapter 5 |
- Exceptions |
and Object Lifetime |
|
|
|
|
|
|
|
|
||||
|
|
Chapter 6 |
- Interfaces |
and Collections |
|
|
|
|
|
ref |
|
Analogous to the IDL [in, out] attribute. The value is assigned by the caller, |
|
|
|
|
Chapter 7 |
- Callback |
Interfaces, Del gates, and Eve ts |
|
|
|
|
|
|
|
|
but may be reassigned within the scope of the method call. |
|
|
|
|
|
|
|
|
|
|
|
|
|
Chapter 8 |
- Advanced |
C# Type Construction Techniques |
|
|
|
|
|
params |
|
This parameter modifier allows you to send in a variable number of |
|
|
|
Part Three - Programming |
with .NET Assemblies |
|
|
|
|||
Chapter 9 |
|
parameters as a single parameter. A given method can only have a single |
|
|
|
||
- Understanding .NET Assemblies |
|
|
|
||||
|
|
|
|
params modifier, and must be the final parameter of the method. |
|
|
|
|
|
Chapter 10 |
- Processes, |
AppDomains, Contexts, and Threads |
|
|
|
Chapter 11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
|
|||||
Part Four - Leveraging the .NET Libraries |
|
||||||
ChaptTherDefault12 - Object SerializationParameterand thePassing.NET RemotingBehaviorLayer |
|
||||||
Chapter 13 |
- Building a Better Window (Introducing Windows Forms) |
|
The default manner in which a parameter is sent into a function is by value. Simply put, if you do not mark an argument with a parameter-centric keyword, a copy of the data is passed into the function (the story
changes slightly when passing reference types by value, as you will see in just a bit). You have already
Chapter 16 - The System.IO Namespace
been making use of by-value parameter passing, however, here is a simple example (with implications):
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
// Default param passing is by value.
Chapter 18 - ASP.NET Web Pages and Web Controls public static int Add(int x, int y)
Chapter 19 - ASP.NET Web Applications
{
Chapter 20 - XML Web Services int ans = x + y;
Index // Caller will not see these changes.
List of Figures
x = 99999;
List of Tables
y = 88888;
return ans;
}
Here, the two value type parameters have been sent by value into the Add() method. Therefore, if we change the values of the parameters within the scope of the member, the caller is blissfully unaware, given that we are changing the values of copies of the caller's System.Int32 data types:
// Call Add() using input params.
Console.WriteLine("***** Adding 2 ints *****");
int x = 9, y = 10;
Console.WriteLine("X: {0}, Y: {1}", x, y);
Console.WriteLine("Answer is: {0}", Add(x, y));
Console.WriteLine("X: {0}, Y: {1}", x, y);
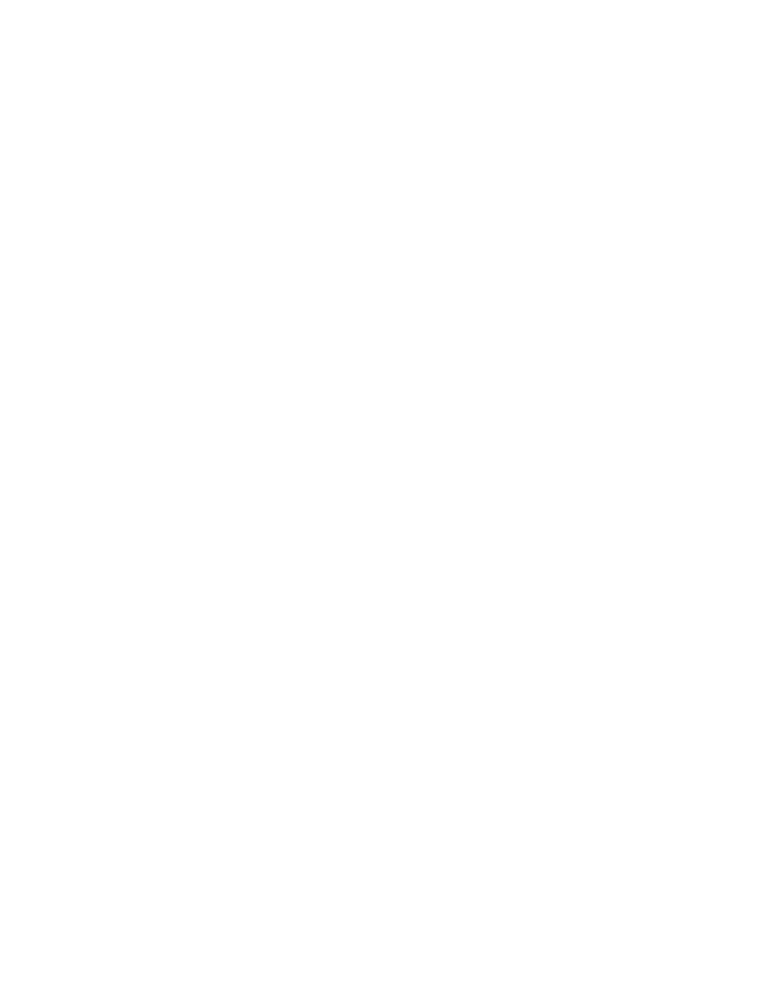
As you would expect, the values of x and y remain identical before and after the call to Add().
C# and the .NET Platform, Second Edition
by Andrew Troelsen ISBN:1590590554
The C# "out"ApressKeyword© 2003 (1200 pages)
This comprehensive text starts with a brief overview of the
Next, we have theC#uselanguageof outputandparametersthen quickly. Heremovesis anto keyalternativetechnicalversionand of the Add() method that
architectural issues for .NET developers.
returns the sum of two integers using the C# "out" keyword (note the physical return value of this method is now void):
Table of Contents
// Output parameters are allocated by the callee.
C# and the .NET Platform, Second Edition
public static void Add(int x, int y, out int ans)
Introduction
{
Part One - Introducing C# and the .NET Platform
ans = x + y;
Chapter 1 - The Philosophy of .NET
}
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
ChapterCalling3a method- C# Languagewith outputFundamentalsparameters also requires the use of the "out" keyword. Recall that when you
Chapterare local,4 output- Objectvariables-OrientedareProgrammingnot requiredwitoh beC#assigned before use (if you do so, the value is forgotten
Chapterafter the5 call)- Exceptions. For example:and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
// No need to assign before use when a variable is used ala out.
Chapterint ans;8 - Advanced C# Type Construction Techniques
Part// ThreeNote- Programminguse of outwithkeyword.NET Assembliesin calling syntax.
ChapterAdd(90,9 -90,Understandiout ans);g .NET Assemblies
ChapterConsole10.-WriteLine("90P ocesses, AppDomains,+ 90Contexts,= {0} and", Threadsans);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Now in this case, we really have no good reason to return the value of our summation using an output
Chapter 12 - Object Serialization and the .NET Remoting Layer
parameter. However, the C# "out" keyword does serve a very useful purpose: It allows the caller to obtain
Chapter 13 - Building a Better Window (Introducing Windows Forms)
multiple return values from a single method invocation. For example, assume we have the following
Chapter 14 - A Better Painting Framework (GDI+) member:
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
// Returning multiple output parameters.
Chapter 17 - Data Access with ADO.NET
public static void FillTheseValues(out int a, out string b, out bool c)
Part Five - Web Applications and XML Web Services
{
Chapter 18 - ASP.NET Web Pages and Web Controls a = 9;
Chapter 19 - ASP.NET Web Applications
b = "Enjoy your string...";
Chapter 20 - XML Web Services c = true;
Index
}
List of Figures
List of Tables
The caller would be able to invoke the following method:
// Method with multiple output params.
Console.WriteLine("Calling method with multiple output params"); int i;
string str; bool b;
FillTheseValues(out i, out str, out b);
Console.WriteLine("Int is: {0}", i);
Console.WriteLine("String is: {0}", str);
Console.WriteLine("Boolean is: {0}", b);
The C# "ref" Keyword

Now consider the use of the C# ref parameter modifier. Reference parameters are necessary when you
C# and the .NET Platform, Second Edition
wish to allow a method to operate on (and usually change the values of) various data points declared in
by Andrew Troelsen ISBN:1590590554
the caller's scope (such as a sort routine). Note the distinction between output and reference parameters:
Apress © 2003 (1200 pages)
Output parametersThis comprehedo not needsive textto bestarinitializeds with abeforebrief overviewthey areofsenttheto the callee. Reason? It is assumed theC#methodlanguagefillsandthe thenvaluequicklyon yourmovesbehalfto. key technical and
architectural issues for .NET developers.
Reference parameters must be initialized before being sent to the callee. Reason? You are passing a reference to an existing type. If you don't assign it to an initial value, that would be the equivalent to
Table operatingf Contents
on a NULL pointer!
C# and the .NET Platform, Second Edition
Let's check out the use of the "ref" keyword:
Introduction
Part One - Introducing C# and the .NET Platform
Chapter// Reference1 - The Philosophyparameterof .NET.
Chapterpublic2 static- BuildingvoidC# ApplicationsUpperCaseThisString(ref string s)
Part{ Two - The C# Programming Language
// Return the uppercase version of the string.
Chapter 3 - C# Language F ndamentals
Chapter s4 |
=- Objects.ToUpper();-Oriented Programming with C# |
|
Chapter} |
5 |
- Exceptions and Object Lifetime |
Chapterpublic6 |
static- Interfacesvoidand Main()Collections |
|
Chapter{ |
7 |
- Callback Interfaces, Delegates, and Events |
Chapter |
...8 - Advanced C# Type Construction Techniques |
// Use 'ref'.
Part Three - Programming with .NET Assemblies
string s = "Can you really have sonic hearing for $19.00?";
Chapter 9 - Understanding .NET Assemblies
Console.WriteLine("Before: {0} ", s);
Chapter 10 - Processes, AppDomains, Contexts, and Threads
UpperCaseThisString(ref s);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Console.WriteLine("After: {0} ", s);
Part Four - Leveraging the .NET Libraries
}
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
ChapterHere, the14 caller- A Betterhas PaintiassignedFranmeworkinitial value(GDI+)to a local string named "s." Once the call to ChaptUpperCaseThisString()15 - Programmingreturns,with Windowsthe callerFormswill findControls"s" has been converted into uppercase, given the use of
the "ref" keyword.
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
PartTheFiveC#- Web"params"Applications andKeywordXML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
ChapterThe final19parameter- ASP.NET modifierWeb Applicationsis the "params" keyword, which is somewhat odd (but convenient) given that
it allows you to send a varied number of arguments as a singleparameter. Yes, this can be confusing. To
Chapter 20 - XML Web Services
clear the air, assume I have written a simple method defined as follows:
Index
List of Figures
List//ofThisTablesmethod has two physical parameters.
public static void DisplayArrayOfInts(string msg, params int[] list)
{
Console.WriteLine(msg);
for ( int i = 0 ; i < list.Length ; i++) Console.WriteLine(list[i]);
}
This method has been defined to take two physical parameters: one of type string, and one as a parameterized array of integers. What this method is in fact saying is, "Send me a string as the first parameter and any number of integers as the second." Given this, you can call ArrayOfInts() in any of the following ways:
// Use 'params' keyword.
int[] intArray = new int[3] {10,11,12} ;

DisplayArrayOfInts ("Here is an array of ints", intArray);
C# and the .NET Platform, Second Edition
DisplayArrayOfInts ("Enjoy these 3 ints", 1, 2, 3);
by Andrew Troelsen |
ISBN:1590590554 |
DisplayArrayOfInts ("Take some more!", |
55, 4, 983, 10432, 98, 33); |
Apress © 2003 (1200 pages) |
|
This comprehensive text starts with a brief overview of the
Looking at the previousC# languagecode,andyouthencan seequicklythatmovesthe boldedto keyitemstechnicalin aandgiven invocation correspond to the
architectural issues for .NET developers. second parameter (the array of integers).
Of course, you do not have to make use of simple numeric value types when using the params keyword.
Table of Contents
Assume we have yet another variation of the Person class, now defined as follows:
C# and the .NET Platform, Second Edition
Introduction
// Yet another person class.
Part One - Introducing C# and the .NET Platform
class Person
Chapter 1 - The Philosophy of .NET
{
Chapter 2 - Building C# Applications
private string fullName;
Part Two - The C# Programming Language
private byte age;
Chapter 3 - C# Language Fundamentals
public Person(string n, byte a)
Chapter {4 - Object-Oriented Programming with C#
Chapter 5 - ExceptionsfullNameand=Objectn; Lifetime
Chapter 6 - Interfacesage = a;nd Collections
}
Chapter 7 - Callback Interfaces, Delegates, and Events
public void PrintInfo()
Chapter 8 - Advanced C# Type Construction Techniques
{ Console.WriteLine("{0} is {1} years old", fullName, age); }
Part Three - Programming with .NET Assemblies
}
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Now assume a new method that leverages the "params" keyword. This time however, you specify an array
Part Four - Leveraging the .NET Libraries
of objects, which boils down to any number of anything. With the method implementation, you can test for
Chapter 12 - Object Serialization and the .NET Remoting Layer
an incoming Person type using the C# "is" keyword. If your current item is of type Person, call the
Chapter 13 - Building a Better Window (Introducing Windows Forms)
PrintInfo() method. If you do not have a Person type, just dump the textual information of the type to the
Chapter 14 - A Better Painting Framework (GDI+) console:
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
// This method takes any number of any type.
Chapter 17 - Data Access with ADO.NET
public static void DisplayArrayOfObjects(params object[] list)
Part Five - Web Applications and XML Web Services
{
Chapter 18 - ASP.NET Web Pages and Web Controls |
||
for ( int i = 0 ; i < list.Length ; i++) |
||
Chapter 19 - ASP.NET Web Applications |
|
|
{ |
|
// Is the current item a Person type? |
Chapter 20 - XML Web Serv ces |
||
|
if(list[i] is Person) |
|
Index |
{ |
|
List of Figures |
((Person)list[i]).PrintInfo(); // If so, call some methods. |
|
List of Tables |
} |
|
|
else |
|
Console.WriteLine(list[i]);
}
Console.WriteLine();
}
The calling logic is as follows:
// Pass a System.Int32, Person and System.String into our method.
Person p = new Person("Fred", 93);
DisplayArrayOfObjects(777, p, "I really am an instance of System.String");
As you can see, C# allows you to work with parameters on many different levels. For the C++
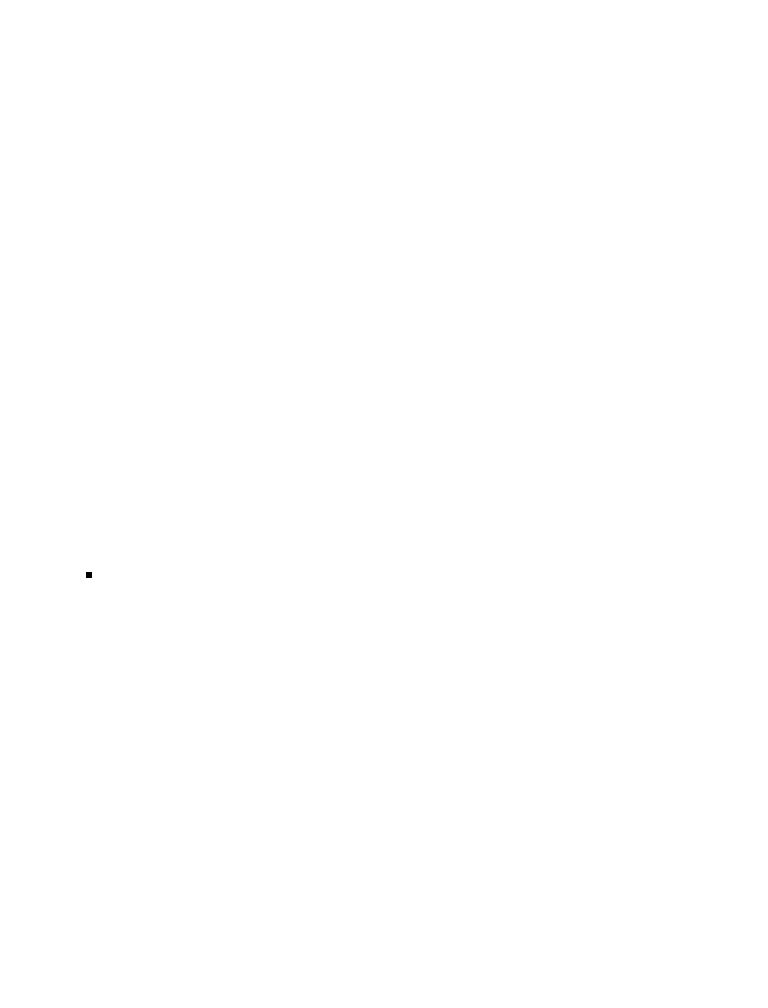
programmers of the world, you should be able to map C# output and reference parameters to pointer (or
C# and the .NET Platform, Second Edition
C++ reference) primitives without the ugly * and & operators.
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages) |
|
Passing Reference Types By Reference (and By Value)
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
A majority of the previous static methods illustrated how value types may be sent into a function by value architectural issues for .NET developers.
(no parameter keyword) or by reference (the "ref" keyword). However, what if we had two additional methods that allow the user to send in Person types by reference as well as by value? First, consider the
Tablefollowingof Contentsmethods:
C# and the .NET Platform, Second Edition
Introductionpublic static void SendAPersonByValue(Person p)
Part{ One - Introducing C# and the .NET Platform
Chapter //1 -ChangeThe Philosomeophy ofdata.NET of 'p'.
Chapter p2.age- Building= 666;C# Applications
Part Two//- TheThisC# Programmingwill be forgottenLanguage after the call!
p = new Person("Nikki", 999);
Chapter 3 - C# Language Fundamentals
}
Chapter 4 - Object-Oriented Programming with C#
public static void SendAPersonByReference(ref Person p)
Chapter 5 - Exceptions and Object Lifetime
{
Chapter 6 - Interfaces and Collections
// Change some data of 'p'.
Chapter 7 - Callback Interfaces, Delegates, and Events
p.age = 555;
Chapter 8 - Advanced C# Type Construction Techniques
// 'p' is now pointing to a new object on the heap!
Part Three - Programming with .NET Assemblies
p = new Person("Nikki", 999);
Chapter 9 - Understanding .NET Assemblies
}
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
PartNoticeFourhow- Leveragithe SendAPersonByValue()g the .NET Libr ries method attempts to reassign the incoming Person reference to a Chapternew object12 -. ObjectHowever,Serializationgiven thatandthisthemethod.NET Remotingis makingLayeruse of the default parameter passing behavior
Chapter(pass by13value),- Buildingthe aobjectB ttertoWindowwhich the(IntroduciincomingWindowsreferenceForms)is pointing to is preserved. However, if you
Chapterwere to14check- A Betterthe agePaintingof theFrameworkperson after(GDI+)the call, you might be shocked to see s/he is now 666 years old! ChapterHow can15this- Programmingbe? The goldenwith ruleWindowsto keepFormsin mindControlswhen passing reference types by value is the following:
Chapter 16 - The System.IO Namespace
If a reference type is passed by value, the callee may change the values of the object's state data, but
Chapter 17 - Data Access with ADO.NET
may not change the object it is referencing.
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Strange, huh? To clarify, here is some calling code:
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
// Passing ref-types by value.
Index
Console.WriteLine("***** Passing person by value *****");
List of Figures
Person fred = new Person("Fred", 12);
List of Tables
Console.WriteLine("Before by value call, Person is:");
fred.PrintInfo();
SendAPersonByValue(fred);
Console.WriteLine("After by value call, Person is:");
fred.PrintInfo();
Figure 3-16 shows the output of this call.
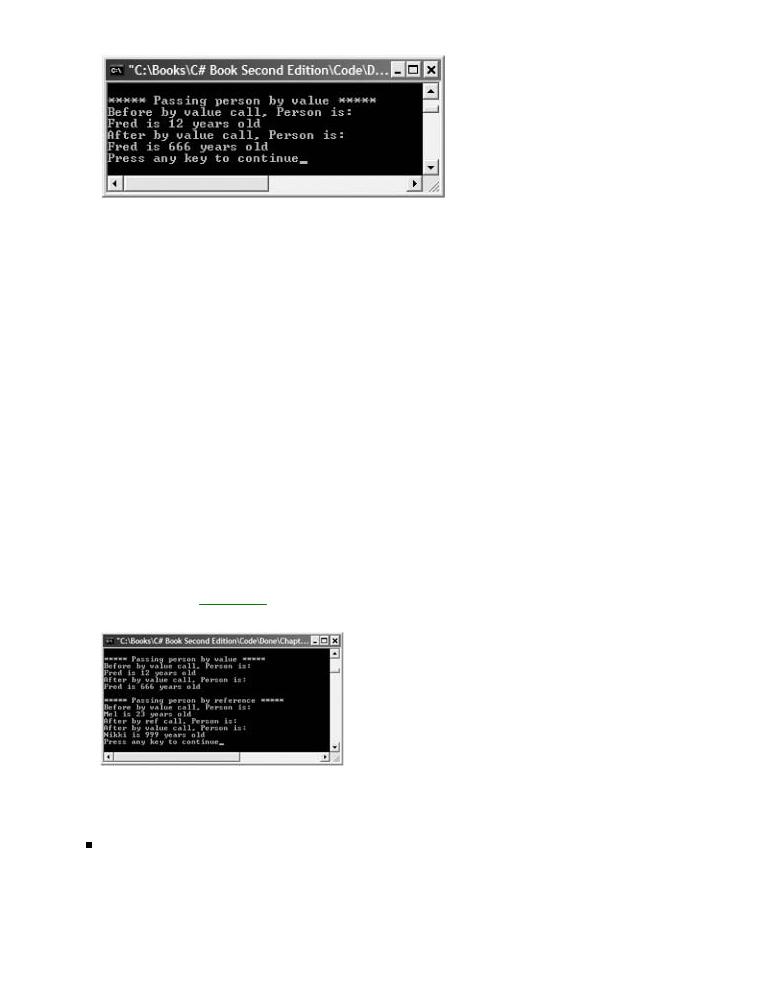
ISBN:1590590554
of the and
Table
Figure 3-16: Passing reference types by value locks the reference in place.
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
This behavior seems to fly in the face of what it means to pass a parameter "by value." Given that we were
Chapter 1 - The Philosophy of .NET
able to change the state of the incoming Person, what was copied? The answer: a copy of the reference to
Chapter 2 - Building C# Applications
the caller's object! Therefore, as the SendAPersonByValue() method is pointing to the same object as the Partcaller,Twoit-isThepossibleC# Programmingto alter the Languageobject's state data. What is not permissible is to reassign what the Chapterreference3 -isC#pointingLa uageto (slightlyFundamentalsakin to a constant pointer in C++).
Chapter 4 - Object-Oriented Programming with C#
In contrast, we have the SendAPersonByReference() method, which passes a reference type by
Chapter 5 - Exceptions and Object Lifetime
reference. As you may expect, this allows complete flexibility of how the callee is able to manipulate the
Chapter 6 - Interfaces and Collections
incoming parameter. Not only can the callee change the state of the object, but if it so chooses, it may
Chapter 7 - Callback Interfaces, Delegates, and Events
reassign the pointer! Consider the following calling logic:
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
// Passing ref-types by ref.
Chapter 9 - Understanding .NET Assemblies
Console.WriteLine("\n***** Passing person by reference *****");
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Person mel = new Person("Mel", 23);
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Console.WriteLine("Before by value call, Person is:");
Part Four - Leveraging the .NET Libraries
mel.PrintInfo();
Chapter 12 - Object Serialization and the .NET Remoting Layer
Console.WriteLine("After by ref call, Person is:");
Chapter 13 - Building a Better Window (Introducing Windows Forms)
SendAPersonByReference(ref mel);
Chapter 14 - A Better Painting Framework (GDI+)
Console.WriteLine("After by value call, Person is:");
Chapter 15 - Programming with Windows Forms Controls mel.PrintInfo();
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
PartAs youFivecan- WebseeApplicationsfrom Figureand3-17XML, theWebtypeServicesnamed Fred returns after the call as a type named Nikki:
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter
Chapter
Index
List of
List of
Figure 3-17: Passing reference types by reference allows the reference to be redirected.
The golden rule to keep in mind when passing reference types by reference is the following:
If a class type is passed by reference, the callee may change the values of the object's state data as well as the object it is referencing.
That wraps up our examination of parameter passing behavior under C#. Next up: the role of System.Array.
SOURCE The MethodsAndParams project is located under the Chapter 3 subdirectory.
CODE

C# and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and architectural issues for .NET developers.
Table of Contents
C# and the .NET Platform, Second Edition
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Array ManipulationC# and the .NETin C#Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Mechanically, C# arrays look and feel much like their C, C++ counterparts. However, as you'll soon see, all C#
Apress © 2003 (1200 pages)
arrays actually derive from the System.Array base class, and therefore share a common set of members (in
This comprehensive text starts with a brief overview of the the same vein asC#Java)l nguage. and then quickly moves to key technical and
architectural issues for .NET developers.
Formally speaking, an array is a collection of data points (of the same underlying type) that are accessed using a numerical index. Understand that CLS-compliant arrays always have a lower bound of zero (although
Tableit is possibleof Contentsto create a non-CLS compliant array with arbitrary lower bounds using the static CreateInstance() C#methodand theof.SystemNET Platform,.Array)Second. As youEditmighton assume, arrays can contain any intrinsic type defined by C#, including
arrays of objects, interfaces, or structures. In C#, arrays can be single or multidimensional, and must be
Introduction
declared with the square brackets ([]) placed after the data type of the array. For example:
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter// A string2 - BuildingarrayC# Applicationscontaining 10 elements {0, 1, ..., 9}
Partstring[]Two - ThebooksOnCOM;C# Programming Language
ChapterbooksOnCOM3 - C# =Languagenew string[10];Fundamentals
// A 2 item string array, numbered {0, 1}
Chapter 4 - Object-Or ented Programming with C#
string[] booksOnPL1 = new string[2];
Chapter 5 - Exceptions and Object Lifetime
// 100 item string array, numbered {0, 1, ..., 99}
Chapter 6 - Interfaces and Collections
string[] booksOnDotNet = new string[100];
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
As you can see, the first example declares the type and size of the array on two separate lines. The final two
Chapter 9 - Understanding .NET Assemblies
examples illustrate that you are also able to declare and construct your array on a single line (just like any
Chapter 10 - Processes, AppDomains, Contexts, and Threads
object). In either case, notice that you are required to make use of the "new" keyword when you are
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
constructing an array of an initial fixed size. Thus, the following array declaration is illegal:
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
// Need 'new' keyword when you define a fixed size array.
Chapter 13 |
- Building a Better Window (Introducing Windows Forms) |
|
int[4] ages = {30, 54, 4, 10} ; |
// Error! |
|
Chapter 14 |
- A Better Painting Framework (GDI+) |
|
Chapter 15 |
- Programming with Windows Forms Controls |
ChaptUnderstand16 - Tthate Systemthe previous.IO Namespacethree valid array declarations have simply allocated a type on the managed Cheap,apterwhich17 - Datahas someAccessinitialwith ADOstorage.NETspace. At this point, you have not literally filled with array with data. To do Partso, Fiveyou would- Web needApplicationsto makeanduseXMLof theW bindexServicesoperator as follows:
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter// A 219item- ASP.NETstringWeb Applicationsarray, numbered {0, 1}
Chapterstring[]20 - XMLbooksOnPL1Web Services= new string[2];
IndexbooksOnPL1[0] = "PL1 for Dummies";
ListbooksOnPL1[1]f Figures = "What have we done?! Essays on the PL1 programming language";
List of Tables
The same holds true if you create an array of custom objects:
// A 2 item Person array, numbered {0, 1}
Person[] theFolks = new Person[2]; theFolks[0] = new Person("Alex", 23); theFolks[1] = new Person("Liz", 32);
//This creates the System.Array type.
//This creates a new Person in slot 0.
//This creates a new Person in slot 1.
Allocating and filling arrays using this longhand notation can become a bother, especially if the array in question contains numerous elements. Therefore, if you would rather let the compiler determine the size of the array, you are free to use the following shorthand notation:
//The size of this array will automatically be set to 4.
//Note the lack of the 'new' keyword and empty [].
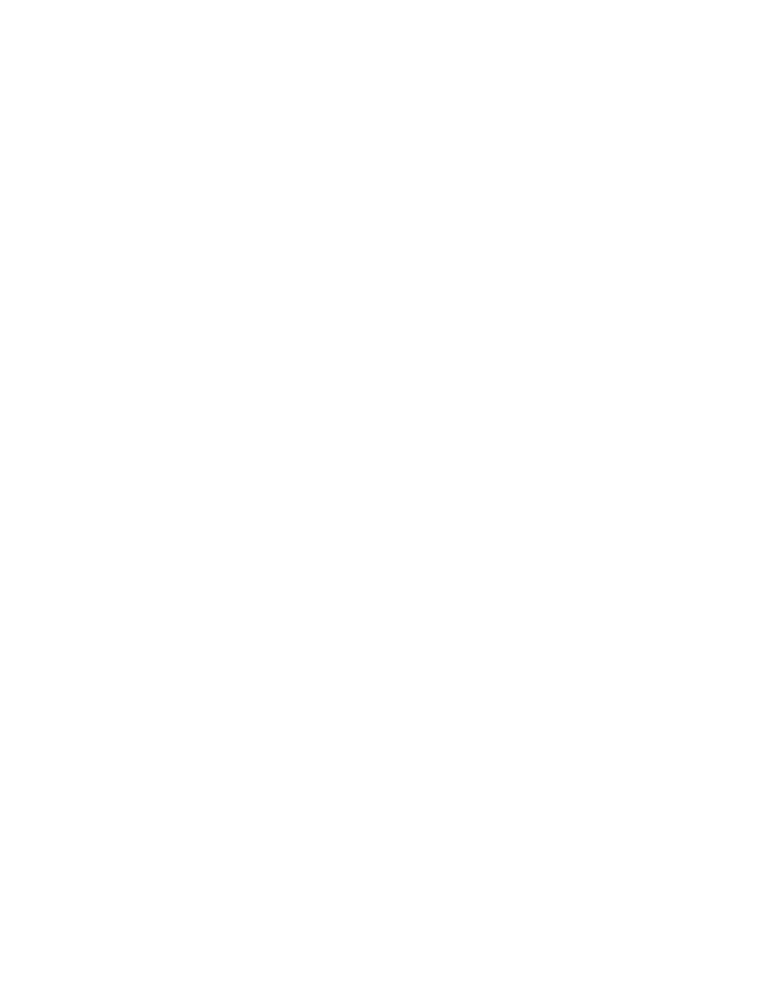
int[] ages = {20, 22, 23, 0} ;
C# and the .NET Platform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
Again, the curly bracketApress ©notation2003 (1200({pages)}) is simply a time saver. Therefore, the following two arrays are identical:
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
// Initialize each member at declaration...
architectural issues for .NET developers.
string[] firstNames = new string[5]{ "Steve", "Gina",
"Swallow", "Baldy", "Gunner" } ;
Table of Contents
// ...OR assign values member by member.
C# and the .NET Platform, Second Edition
string[] firstNames = new string[5];
Introduction
firstNames[0] = "Steve";
Part One - Introducing C# and the .NET Platform
firstNames[1] = "Gina";
Chapter 1 - The Philosophy of .NET firstNames[2] = "Swallow";
Chapter 2 - Building C# Applications firstNames[3] = "Baldy";
Part Two - The C# Programming Language
firstNames[4] = "Gunner";
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
ChapterFinally5be -awareExc ptionsthat theandmembersObject Lifetimein a .NET array are automatically set to their respective default value.
ChapterThus, if6you- Interfhaveancesarrayand Collectionsof numerical types, each member is set to 0 (or 0.0 in the case of floating point Chaptnumerics),r 7 -arraysCallbackof Interfaces,objects beginDelegates,life set toandnull,Eventsand Boolean types are set to false.
Chapter 8 - Advanced C# Type Construction Techniques
PartArraysThree - AsProgrammingParameterswith .NET Assemblies(and Return Values)
Chapter 9 - Understanding .NET Assemblies
ChapterDeclaring10 -andProcesses,filling localAppDomains,arrays mayContexts,be helpfulandfromThreadstime to time. However, as you would hope, arrays can
Chapteralso be11passed- TypeintoReflection,and returnedLate Binding,from customand Attribufunctionse-Based. ForProgrammingexample:
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer public static void PrintArray(int[] myInts)
Chapter{ 13 - Building a Better Window (Introducing Windows Forms)
Chapterfor(int14 - A Betteri =Painting0; Fra< myIntsework (GDI+).Length; i++)
Chapter 15 -ConsoleProgramming.WriteLine("Itemwith Windows Forms{0}Controlsis {1}", i, myInts[i]);
}
Chapter 16 - The System.IO Namespace
public static string[] GetStringArray()
Chapter 17 - Data Access with ADO.NET
{
Part Five - Web Applications and XML Web Services
string[] theStrings = { "Hello", "from", "GetStringArray" };
Chapter 18 - ASP.NET Web Pages and Web Controls
return theStrings;
Chapter 19 - ASP.NET Web Applications
}
Chapter 20 - XML Web Services
Index
List of Figures
Calling these static members is equally as straightforward:
List of Tables
int[] ages = {20, 22, 23, 0} ;
PrintArray(ages);
string[] strs = GetStringArray();
foreach(string s in strs)
Console.WriteLine(s);
Working with Multidimensional Arrays
In addition to the single dimension arrays you have seen thus far, C# also supports two varieties of multidimensional arrays. The first of these is termed a rectangular array. This type is simply an array of multiple dimensions, where each row is of the same length. To declare and fill a multidimensional rectangular array, proceed as follows:
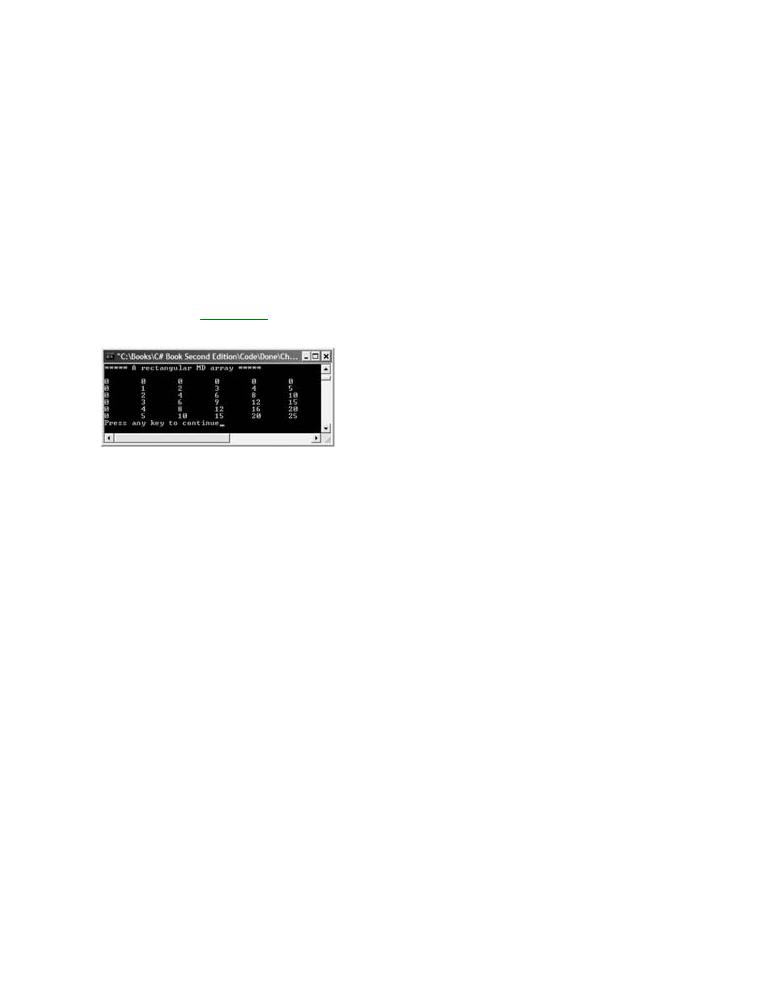
// A rectangular MD array.
C# and the .NET Platform, Second Edition int[,] myMatrix;
by Andrew Troelsen |
ISBN:1590590554 |
|
myMatrix = new |
int[6,6]; |
|
Apress © 2003 (1200 pages) |
|
|
// Populate (6 |
* 6) array. |
|
This comprehensive text starts with a brief overview of the for(int i = 0; i < 6; i++)
C# language and then quickly moves to key technical and for(int j = 0; j < 6; j++)
architectural issues for .NET developers. myMatrix[i, j] = i * j;
// Show (6 * 6) array.
for(int i = 0; i < 6; i++)
Table of Contents
{
C# and the .NET Platform, Second Edition for(int j = 0; j < 6; j++)
Introduction
{ Console.Write(myMatrix[i, j] + "\t"); }
Part One - Introducing C# and the .NET Platform
Console.WriteLine();
Chapter 1 - The Philosophy of .NET
}
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
ChapterThe output3 - isC#seenLa guagein FigureFundamentals3-18 (note the rectangular nature of the array).
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter
Chapter
Chapter
Part
Chapter
Chapter and Threads
Figure 3-18: A multidimensional array
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
ChapterThe second12 - ObjtypectofSerializationmultidimensionaland thearray.NETisRemotingtermed aLayerjagged array. As the name implies, jagged arrays
Chapcontainer 13some- Buildingnumbera Betterof innerWindowarrays,(Introducingeach of whichWindowsmay haveForms)a unique upper limit. For example:
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
// A jagged MD array (i.e., an array of arrays).
Chapter// Here16 -weThehaveSysteman.IOarrayNamespaceof 5 different arrays.
Chapter int[][]17 - Data AccessmyJagArraywith ADO.=NETnew int[5][];
Part// FiveCreate- W btheApplicjaggedtions andarrayXML Web. Services
Chapterfor (int18 - ASPi =.NET0;Webi <PagesmyJagArraynd Web Controls.Length; i++)
{
Chapter 19 - ASP.NET Web Applications
myJagArray[i] = new int[i + 7];
Chapter 20 - XML Web Services
}
Index
// Print each row (remember, each element is defaulted to zero!)
List of Figures
for(int i = 0; i < 5; i++)
List of Tables
{
Console.Write("Length of row {0} is {1} :\t", i, myJagArray[i].Length); for(int j = 0; j < myJagArray[i].Length; j++)
{
Console.Write(myJagArray[i][j] + " ");
}
Console.WriteLine();
}
The output is seen in Figure 3-19 (note the jaggedness of the array).
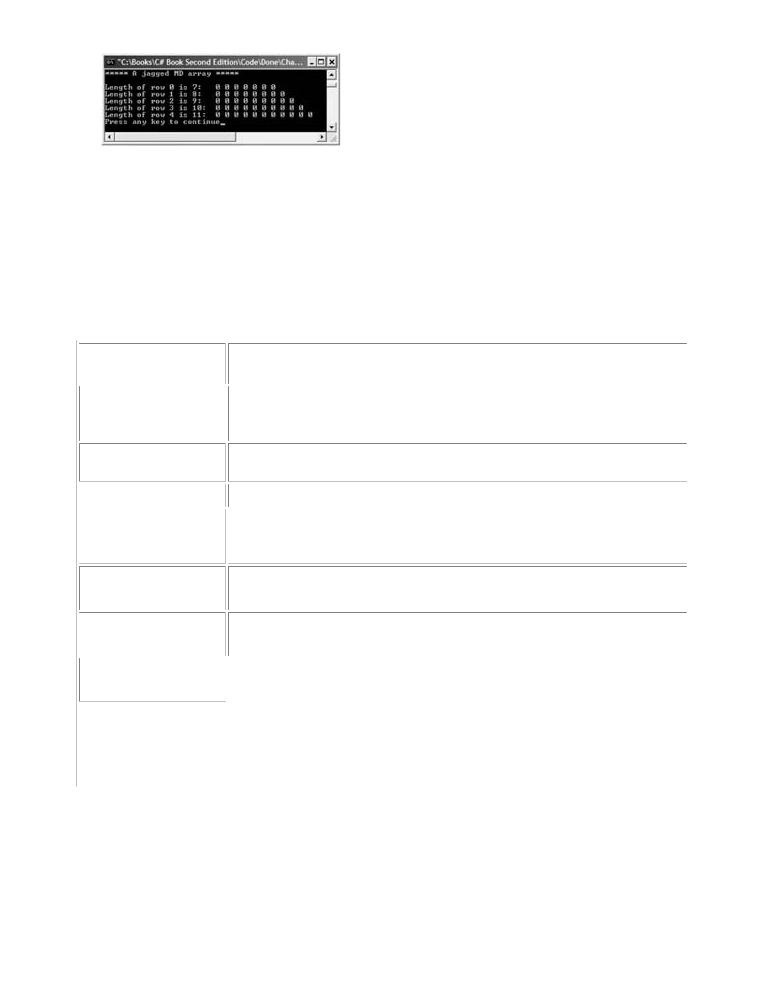
Second Edition
ISBN:1590590554
with a brief overview of the
moves to key technical and
architectural issues for .NET developers.
Figure 3-19: Jagged arrays
Now that you understand how to build and populate C# arrays, you can turn your attention to the ultimate base
Table of Contents
class of any array, System.Array.
C# and the .NET Platform, Second Edition
Introduction
The System.Array Base Class
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
The most striking difference between C and C++ arrays is the fact that every array you create is automatically
Chapter 2 - Building C# Applications
derived from System.Array. This class defines a number of helpful methods that make working with arrays
Part Two - The C# Programming Language |
|
|
|
|||||
|
much more palatable. Table 3-10 gives a rundown of some (but not all) of the more interesting members. |
|||||||
Chapter 3 |
- C# Language Fundamentals |
|
|
|
||||
Chapter 4 |
- Object-Oriented Programming with C# |
|||||||
|
Table 3-10: Select Members of System.Array |
|||||||
|
|
Chapter 5 |
- Exceptions and |
|
Object Lifetime |
|
|
|
|
|
ChapterMember6 |
-ofInterfacesArrayand |
|
CollectionsMeaning in Life |
|
|
|
|
|
ChapterClass7 |
- Callback Interfaces, Delegates, and Events |
|
|
|||
|
|
|
|
|
|
|
||
|
|
Chapter 8 |
- Advanced C# |
|
Type Construction Techniques |
|
|
|
|
|
BinarySearch() |
|
This static method is applicable only if the items in the (previously sorted) |
|
|
||
Part Three - Programming |
with .NET Assemblies |
|
|
|||||
|
|
|
|
|
array implement the IComparer interface (see Chapter 6). If so, |
|
|
|
Chapter 9 |
- Understanding |
.NET Assemblies |
|
|
|
|||
|
|
|
|
|
BinarySearch() finds a given item. |
|
|
|
|
|
Chapter 10 |
- Processes, |
AppDomains, Contexts, and Threads |
|
|
||
|
|
|
||||||
|
|
Clear() |
- Type Reflection, |
|
This static method sets a range of elements in the array to empty values (0 |
|
|
|
|
Chapter 11 |
Late Binding, and Attribute-Based Programming |
|
|
||||
|
|
|
|
|
for value items, null for object references). |
|
|
|
Part Four - Leveraging the |
.NET Libraries |
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
Chapter 12 |
- Object Serialization and the .NET Remoting Layer |
|
|
|||
|
|
CopyTo() |
|
Used to copy elements from the source array into the destination array. |
|
|
||
|
|
|
|
|
|
|||
|
|
Chapter 13 |
- Building a Better |
Window (Introducing Windows Forms) |
|
|
||
|
|
GetEnumerator() |
|
Returns the IEnumerator interface for a given array. I address interfaces in |
|
|
||
|
Chapter 14 |
- A Better Painting |
Framework (GDI+) |
|
|
|||
|
|
Chapter 15 |
- Programming |
Chapter 5, but for the time being, keep in mind that this interface is required |
|
|
||
|
with Windows Forms Controls |
|
|
|||||
|
|
|
|
|
by the "foreach" keyword. |
|
|
|
|
|
Chapter 16 |
- The System.IO |
|
Namespace |
|
|
|
|
|
|
|
|
|
|||
|
ChapterGetLength()17 - Data Access withTheADOGetLength().NET |
method is used to determine the number of elements in a |
|
|
||||
Part Five - Web Applications andgivenXMLdimensionWeb Servicesof the array. Length is a read-only property. |
|
|
||||||
|
|
Length |
|
|
|
|
|
|
|
Chapter 18 |
- ASP.NET Web |
|
Pages and Web Controls |
|
|
||
|
|
|
||||||
|
|
GetLowerBound() |
|
As you can guess, these two methods can be used to determine the bounds |
|
|
||
|
Chapter 19 |
- ASP.NET Web |
|
Applications |
|
|
|
|
|
|
|
|
|
of a given dimension. |
|
|
|
|
ChapterGetUpperBound()20 - XML Web Services |
|
|
|
||||
|
|
|
|
|
|
|
|
|
Index |
|
|
Retrieves or sets the value for a given index in the array. These methods |
|
|
|||
|
|
GetValue() |
|
|
|
|||
List of Figures |
|
have been overloaded to work with single and multidimensional arrays. |
|
|
||||
|
|
SetValue() |
|
|
|
|||
List of Tables |
|
|
|
|
|
|||
|
|
|
|
|
||||
|
|
Reverse() |
|
This static method reverses the contents of a one-dimensional array. |
|
|
||
|
|
|
|
|
|
|||
|
|
Sort() |
|
|
Sorts a one-dimensional array of intrinsic types. If the elements in the array |
|
|
|
|
|
|
|
|
implement the IComparer interface, you can also sort your custom types |
|
|
|
|
|
|
|
|
(again, see Chapter 5). |
|
|
|
|
|
|
|
|
|
|
|
|
Let's see some of these members in action. The following code makes use of the static Reverse() and Clear() methods (and the Length property) to pump out some information about the firstName string array to the console:
// Create some string arrays and exercise some System.Array members.
class Arrays
{
public static int Main(string[] args)
{
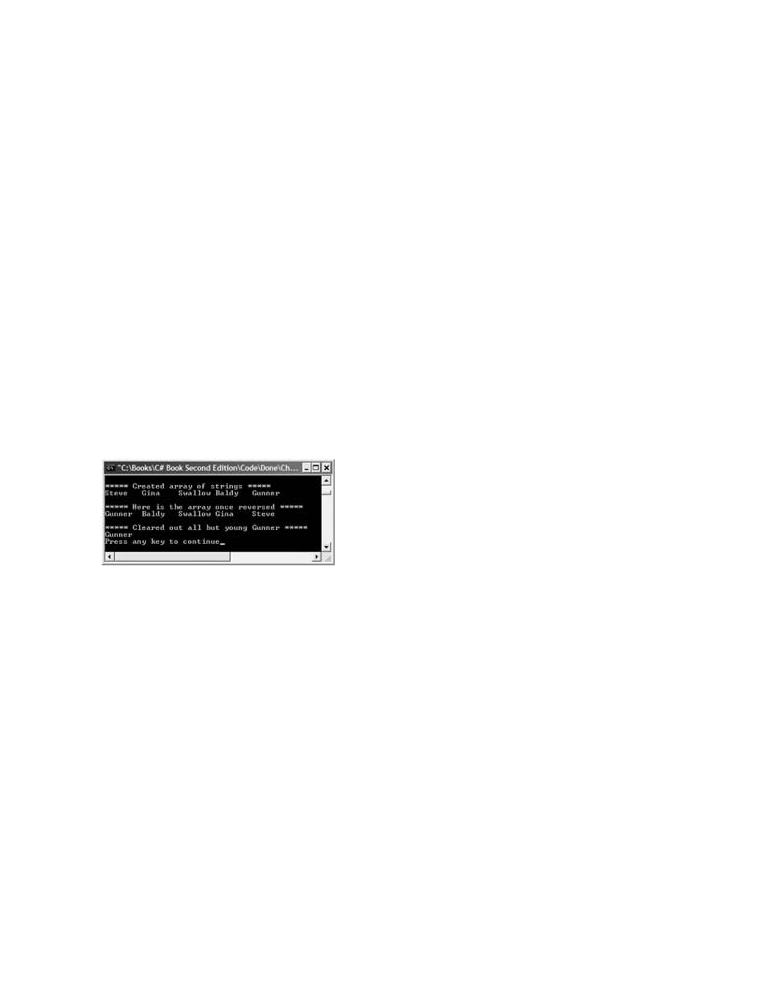
// Array of strings.
C# and the .NET Platform, Second Edition
string[] firstNames = new string[5]{ "Steve", "Gina", "Swallow",
by Andrew Troelsen |
ISBN:1590590554 |
"Baldy", "Gunner" } ; |
|
Apress © 2003 (1200 pages)
// Print out names in declared order.
This compWriteLine("Hereehensive text starts with a brief overview of the
Console. is the array:");
C# language and then quickly moves to key technical and for(int i = 0; i < firstNames.Length; i++)
architectural issues for .NET developers.
Console.Write(firstNames[i] + "\t");
// Flip things around using the static Reverse() method...
Array.Reverse(firstNames);
Table of Contents
// ... and print them.
C# and the .NET Platform, Second Edition
Console.WriteLine("Here is the array once reversed:");
Introduction
for(int i = 0; i < firstNames.Length; i++)
Console.Write(firstNames[i] + "\t");
Chapter 1 - The Philosophy of .NET
// Clear out all but young gunner.
Chapter 2 - Building C# Applications
Console.WriteLine("Cleared out all but young Gunner...");
Part Two - The C# Programming Language
Array.Clear(firstNames, 1, 4);
Chapter 3 - C# Language Fundamentals
for(int i = 0; i < firstNames.Length; i++)
Chapter 4 - Object-Oriented Programming with C#
Console.Write(firstNames[i] + "\t\n");
Chapter 5 - Exceptions and Object Lifetime
return 0;
Chapter 6 - Interfaces and Collections
}
Chapter} 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
The complete output of our array example can be seen in Figure 3-20. |
|
Chapter 9 - Understanding .NET Assemblies |
|
Chapter 10 - Processes, AppDomains, Contexts, and Threads |
|
Chapter |
Attribute-Based Programming |
Part |
|
Chapter |
Remoting Layer |
Chapter |
Windows Forms) |
Chapter |
|
Chapter |
Controls |
Chapter
Figure 3-20: Fun with System.Array
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
SOURCE The Arrays application is located under the Chapter 3 subdirectory.
Chapter 18 - ASP.NET Web Pages and Web Controls
CODE
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
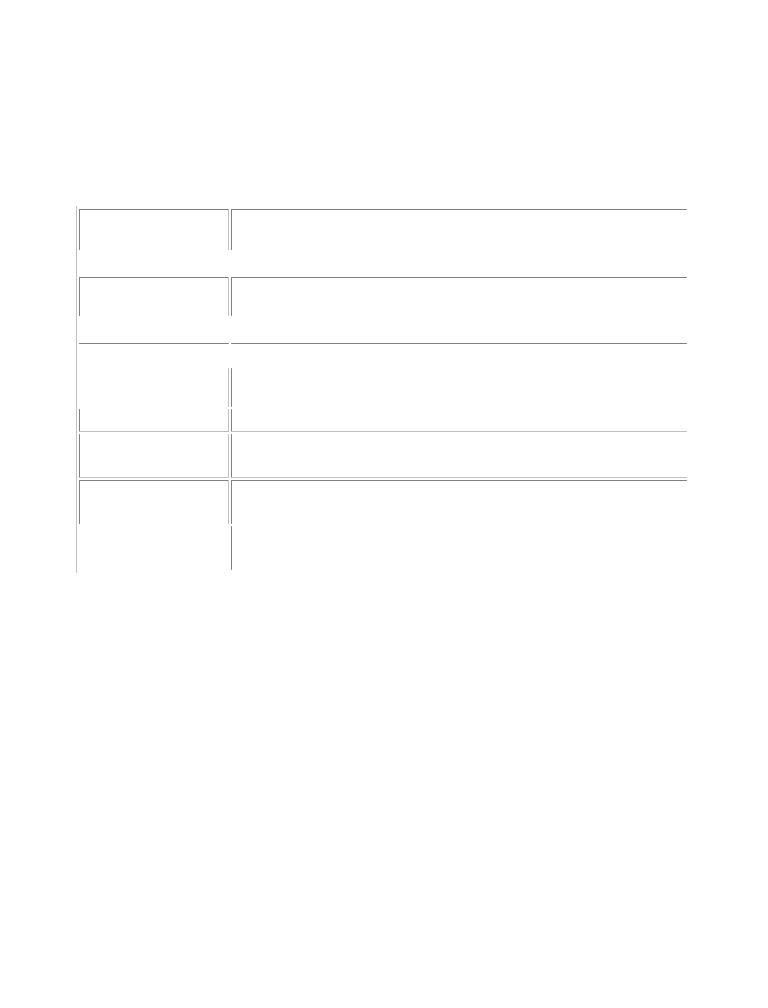
String ManipulationC# and the .NETin Platform,C# Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
As you have already seen, string is a keyword type in C#. However, like all intrinsic types, string actually
Apress © 2003 (1200 pages)
aliases a type in the .NET library, which in this case is a class named System.String. System.String provides a
This comprehensive text starts with a brief overview of the
number of methodsC# youlanguagewouldandexpectthenfromquicklysuchmovesa utilityto keyclass,techincludingical a d methods that return the length, find substrings, convertarchitecturalto and fromissuppercase/lowercase,es for .NET developersand. so forth. Table 3-11 lists some (but by no means all) of the interesting members.
Table of Contents |
|
|
|
|
|
||||
|
|
Table 3-11: Select Members of System.String |
|||||||
C# and the .NET Platform, |
Second Edition |
|
|
||||||
IntroductionMember of String |
|
|
Meaning in Life |
|
|
||||
PartClassOne - Introducing C# and the .NET Platform |
|
|
|||||||
|
|
|
|
|
|
|
|
||
|
|
|
Chapter 1 |
- The Philosophy |
|
|
of .NET |
|
|
|
|
|
Length |
|
|
|
This property returns the length of the current string. |
|
|
|
|
|
Chapter 2 |
- Building C# |
|
Applications |
|
|
|
|
|
|
|
|
|
||||
PartConcat()Two - The C# ProgrammingThisLanguagestatic method of the String class returns a new string that is composed |
|
|
|||||||
|
Chapter 3 |
- C# Language |
|
Fundamentalsof two discrete strings. |
|
|
|||
|
|
|
|
|
|
|
|
||
|
|
|
Chapter 4 |
- Object-Oriented |
|
|
Programming with C# |
|
|
|
|
|
CompareTo() |
|
|
Compares two strings. |
|
|
|
|
|
|
Chapter 5 |
- Exceptions and |
|
|
Object Lifetime |
|
|
|
|
|
|
|
|
|
|||
|
|
|
Copy() |
- Interfaces and |
|
|
This static method returns a fresh new copy of an existing string. |
|
|
|
Chapter 6 |
|
|
Collections |
|
|
|||
|
|
|
|
|
|
|
|||
|
|
|
Chapter 7 |
- Callback Interfaces, Delegates, and Events |
|
|
|||
|
|
|
Format() |
|
|
Used to format a string using other primitives (i.e., numerical data, other |
|
|
|
|
Chapter 8 |
- Advanced C# Type Construction Techniques |
|
|
|||||
|
|
|
|
|
|
|
strings) and the {0} notation examined earlier in this chapter. |
|
|
|
|
|
|
|
|
||||
Part Three - Programming with .NET Assemblies |
|
|
|||||||
|
|
|
Insert() |
|
|
|
Used to insert a string within a given string. |
|
|
|
Chapter 9 |
- Understanding |
|
|
.NET Assemblies |
|
|
||
|
|||||||||
|
|
|
Chapter 10 |
- Processes, AppDomains, Contexts, and Threads |
|
|
|||
|
|
|
PadLeft() |
|
|
These methods are used to pad a string with some character. |
|
|
|
|
Chapter 11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
|
|
|||||
|
|
|
PadRight() |
|
|
|
|
|
|
Part Four - Leveraging the .NET Libraries |
|
|
|||||||
|
|
||||||||
ChapterRemove()12 - Object SerializationUseandthesemethods.NET Remotingto receiveLayera copy of a string, with modifications |
|
|
|||||||
Chapter 13 |
- Building a Better(charactersWindow (Introducingremoved Windowsor replaced)Forms). |
|
|
||||||
|
|
|
Replace() |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
Chapter 14 |
- A Better Painting |
|
Framework (GDI+) |
|
||
|
|
|
ToUpper() |
|
|
Creates a copy of a given sting in uppercase or lowercase. |
|
|
|
|
Chapter 15 |
- Programming |
|
with Windows Forms Controls |
|
|
|||
|
ChapterToLower()16 - The System.IO |
|
Namespace |
|
|
||||
|
|
|
|
|
|
|
|
|
|
Chapter 17 |
- Data Access with ADO.NET |
You should be aware of a few aspects of C# string manipulation. First, although string is a reference type, the
Part Five - Web Applications and XML Web Services
equality operators (== and !=) are defined to compare the values of string objects, not the memory to which
Chapter 18 - ASP.NET Web Pages and Web Controls
they refer. On a related note, understand that the addition operator (+) has been overloaded as a shorthand
Chapter 19 - ASP.NET Web Applications
alternative to calling Concat():
Chapter 20 - XML Web Services
Index
// == and != are used to compare the values within strings.
List of Figures
// + is used for concatenation.
List of Tables
public static int Main(string[] args)
{
// Alternative ways to declare a string.
System.String strObj = "This is a TEST";
string s = "This is another TEST";
// Test for equality between the stings.
if(s == strObj)
Console.WriteLine("Same info...");
else
Console.WriteLine("Not the same info...");
// Concatenation.
string newString = s + strObj; Console.WriteLine("s + strObj = {0} ", newString);
//System.String also defines a custom indexer to access each
//character in the string.
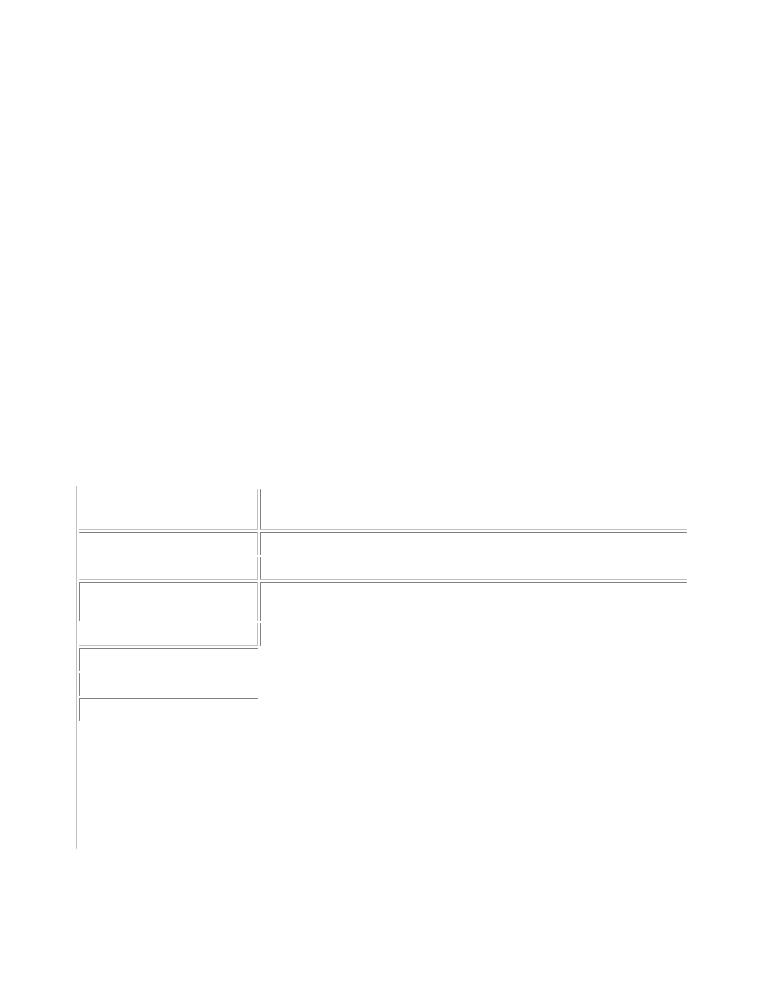
for(int k = 0; k < s.Length; k++)
C# and the .NET Platform, Second Edition
Console.WriteLine("Char {0} is {1} ", k, s[k]);
by Andrew Troelsen |
ISBN:1590590554 |
return 0; |
|
}Apress © 2003 (1200 pages)
This comprehensive text starts with a brief overview of the C# language and then quickly moves to key technical and
architectural issues for .NET developers.
When you run this program, you are able to verify that the two string objects (s and strObj) do not contain the same values, and therefore, the test for equality fails. When you examine the contents of newString, you will
see it is indeed "This is another TESTThis is a TEST." Finally, notice that you can access the individual
Table of Contents
characters of a string using the index operator ([]).
C# and the .NET Platform, Second Edition
Introduction
Escape Characters and "Verbatim Strings"
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Like C(++) and Java, C# strings can contain any number of escape characters:
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
// Escape characters (\t, \\ , \n, et al.)
Chapter 3 - C# Language Fundamentals
string anotherString;
Chapter 4 - Object-Oriented Programming with C#
anotherString = "Every programming book needs \"Hello World\"";
Chapter 5 - Exceptions and Object Lifetime
Console.WriteLine("\t" + anotherString);
Chapter 6 - Interfaces and Collections
anotherString = "c:\\ CSharpProjects\\ Strings\\ string.cs";
Chapter 7 - Callback Interfaces, Delegates, and Events
Console.WriteLine("\t" + anotherString);
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
ChapterIn case9you- Understandingare bit rusty with.NETtheAssembliesmeaning behind these escape characters, Table 3-12 should refresh your
Chaptermemory10. - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
PartTableFour3--12:LeveragingString Escapethe .NETCharactersLib ries
|
|
|
|
|
|
|
|
|
|
Chapter |
12 |
- Object Serialization |
|
and the .NET Remoting Layer |
|
|
|
String Escape |
|
Meaning in Life |
|
||
|
|
Chapter |
13 |
- Building a Better Window (Introducing Windows Forms) |
|
||
|
|
Character |
|
|
|
||
|
|
Chapter |
14 |
- A Better Painting Framework (GDI+) |
|
||
|
|
|
|||||
|
|
Chapter\' |
15 |
- Programming with WindowsInsertsFormsa singleContrquotels into a string literal. |
|
||
|
|
|
|
|
|
|
|
|
|
Chapter |
16 |
- The System.IO Namespace |
|
||
|
|
\" |
|
|
|
Inserts a double quote into a string literal. |
|
|
|
Chapter |
17 |
- Data Access with ADO.NET |
|
||
|
|
|
|||||
Part\\ Five - Web Applications and XMLInsertsWebaServicesbackslash into a string literal. This can be quite helpful when |
|
||||||
Chapter |
18 |
- ASP.NET Web Pages anddefiningWeb Controlsfile paths. |
|
||||
|
|
|
|
|
|
|
|
|
|
Chapter |
19 |
- ASP.NET Web Applications |
|
||
|
|
\a |
|
|
|
Triggers a system alert. |
|
|
|
Chapter |
20 |
- XML Web Services |
|
|
|
|
|
|
|
|
|||
Index\b |
|
|
|
Triggers a backspace. |
|
||
|
|
|
|
|
|||
List\fof Figures |
|
Triggers a form feed. |
|
||||
|
|
|
|
|
|||
List of Tables |
|
|
|
||||
|
|
|
|||||
|
|
\n |
|
|
|
Inserts a new line. |
|
|
|
|
|
|
|
|
|
|
|
\r |
|
|
|
Inserts a carriage return. |
|
|
|
|
|
|
|
|
|
|
|
\t |
|
|
|
Inserts a horizontal tab into the string literal. |
|
|
|
|
|
|
|
|
|
|
|
\u |
|
|
|
Inserts a Unicode character into the string literal. |
|
|
|
|
|
|
|
|
|
|
|
\v |
|
|
|
Inserts a vertical tab into the string literal. |
|
|
|
|
|
|
|||
|
|
\0 |
|
|
|
Represents a NULL character. |
|
|
|
|
|
|
|
|
|
In addition to traditional escape characters, C# introduces the @-quoted string literal notation (termed a verbatim string). Using verbatim strings, you are able to bypass the use of cryptic escape characters and define your literals as follows:
// The following string is printed verbatim (e.g., all \markers are displayed!).

string finalString = @"\n\tString file: 'C:\CSharpProjects\Strings\string.cs'";
C# and the .NET Platform, Second Edition
Console.WriteLine(finalString);
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages) |
|
The output has beenThis prefixedcomprehensivewith "\n\t",text asstartsthesewithescapea bri fcharactersoverview ofarethenot processed in @-quoted strings. Also note that verbatimC# languagestringsandcanthenbe usedquicklytomovesignoretowhitekeyspacetechnicalthatandflows over multiple lines. Assume you
architectural issues for .NET developers.
have a mighty long string literal that would require the reader to scroll right for a rather long time. If you were to make use of @-prefixed strings, you would be able to author the following (more readable) code:
Table of Contents
// White Space is preserved!
C# and the .NET Platform, Second Edition
string myLongString = @"Stuff Stuff Stuff Stuff Stuff
Introduction
Stuff Stuff Stuff Stuff Stuff Stuff Stuff
Part One - Introducing C# and the .NET Platform
Stuff Stuff Stuff Stuff Stuff";
Chapter 1 - The Philosophy of .NET
Console.WriteLine(myLongString);
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Using System.Text.StringBuilder
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
One thing to be very aware of with regard to C# strings: The value of a string cannot be modified once
Chapter 6 - Interfaces and Collections
established. Like Java, C# strings are immutable. In fact, if you examine the methods of System.String, you
Chapter 7 - Callback Interfaces, Delegates, and Events
notice that the methods that seem to internally modify a string, in fact return a modified copy of the string. For
Chapter 8 - Advanced C# Type Construction Techniques
example, when you send the ToUpper() message to a string object, you are not modifying the underlying
Part Three - Programming with .NET Assemblies
buffer of the existing string object, but are returned a fresh copy of the buffer in uppercase form:
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Make changes to this string? Not really...
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
System.String strFixed = "This is how I began life";
Part Four - Leveraging the .NET Libraries
Console.WriteLine(strFixed);
Chapter 12 - Object Serialization and the .NET Remoting Layer
// Returns an uppercase copy of strFixed.
Chapter 13 - Building a Better Window (Introducing Windows Forms) string upperVersion = strFixed.ToUpper();
Chapter 14 - A Better Painting Framework (GDI+)
Console.WriteLine(strFixed);
Chapter 15 - Programming with Windows Forms Controls
Console.WriteLine(upperVersion);
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
It can become slightly annoying (and inefficient) to work with copies of copies of strings. To help ease the pain,
Part Five - Web Applications and XML Web Services
the System.Text namespace defines a class named StringBuilder. This class operates much more like an
Chapter 18 - ASP.NET Web Pages and Web Controls
MFC CString or ATL CComBSTR, in that any modifications you make to the StringBuilder instance affect the |
|
Chapter 19 - ASP.NET Web Applications |
|
underlying buffer (and are thus more efficient): |
|
Chapter 20 - XML Web Services |
|
Index |
|
Listusingof FiguresSystem; |
// Recall, StringBuilder lives here! |
Listusingof TablesSystem.Text; |
|
class StringApp |
|
{ |
|
public static int Main(string[] args)
{
// Create a StringBuilder and change the underlying buffer.
StringBuilder myBuffer = new StringBuilder("I am a buffer");
myBuffer.Append(" that just got longer...");
Console.WriteLine(myBuffer);
return 0;
}
}
Beyond appending to your internal buffer, the StringBuilder class allows you to replace and remove characters at will. Once you have established the state of your buffer, call ToString() to store the final result into a System.String data type:
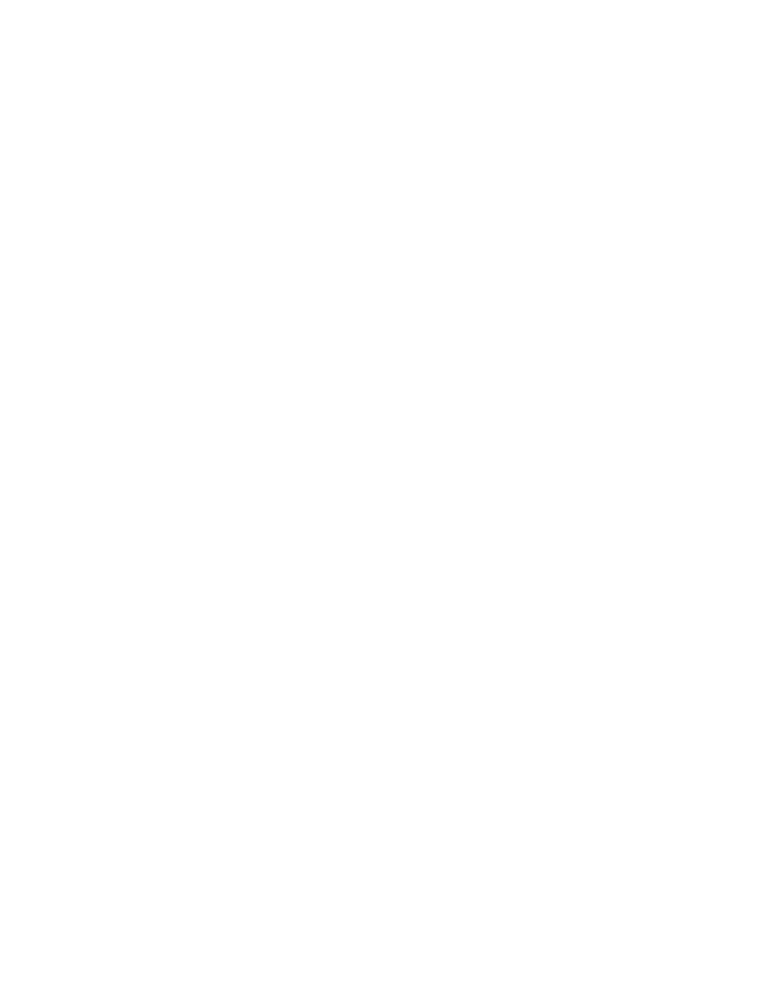
C# and the .NET Platform, Second Edition using System;
|
by Andrew Troelsen |
ISBN:1590590554 |
using System.Text; |
|
|
|
Apress © 2003 (1200 pages) |
|
class StringApp |
|
|
{ |
This comprehensive text starts with a brief overview of the |
|
C# language and then quickly moves to key technical and |
public static int Main(string[] args) architectural issues for .NET developers.
{
StringBuilder myBuffer = new StringBuilder("I am a buffer");
myBuffer.Append(" that just got longer...");
Table of Contents
Console.WriteLine(myBuffer);
C# and the .NET Platform, Second Edition
myBuffer.Append("and even longer.");
Introduction
Console.WriteLine(myBuffer);
Part One - Introducing C# and the .NET Platform
// Transfer the buffer to an uppercase fixed string.
Chapter 1 - The Philosophy of .NET
string theReallyFinalString = myBuffer.ToString().ToUpper();
Chapter 2 - Building C# Applications
Console.WriteLine(theReallyFinalString);
Part Two - The C# Programming Language
return 0;
Chapter 3 - C# Language Fundamentals
}
Chapter 4 - Object-Oriented Programming with C#
}
Chapter 5 - Exceptions and Object Lifetime Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
ChapterNow, do8 understand- AdvancedthatC# inTypemanyConstructioncases, SystemTechniques.String will be your textual object of choice. For most
Papplications,rt Three - Programmingthe overheadwithassociated.NET Assembliwith returnings modified copies of character data will be negligible. ChaptHowever,r 9 if- youUnderstandingare building.NETa textAssemblies-intensive application (such as a word processor application) you will most
likely find that using System.Text.StringBuilder will improve performance. As you might assume, StringBuilder
Chapter 10 - Processes, AppDomains, Contexts, and Threads
contains additional methods and properties beyond those examined here. I leave it to you to drill into more
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
specifics at your leisure.
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
SOURCE The Strings project is located under the Chapter 3 subdirectory.
Chapter 13 - Building a Better Window (Introducing Windows Forms)
CODE
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables