
- •Table of Contents
- •Mastering UML with Rational Rose 2002
- •Chapter 1: Introduction to UML
- •Encapsulation
- •Inheritance
- •Polymorphism
- •What Is Visual Modeling?
- •Systems of Graphical Notation
- •Booch Notation
- •Object Management Technology (OMT)
- •Unified Modeling Language (UML)
- •Understanding UML Diagrams
- •Business Use Case Diagrams
- •Use Case Diagrams
- •Activity Diagrams
- •Sequence Diagrams
- •Collaboration Diagrams
- •Class Diagrams
- •Statechart Diagrams
- •Component Diagrams
- •Deployment Diagrams
- •Visual Modeling and the Software Development Process
- •Inception
- •Elaboration
- •Construction
- •Transition
- •Summary
- •Chapter 2: A Tour of Rose
- •What Is Rose?
- •Getting Around in Rose
- •Parts of the Screen
- •Exploring Four Views in a Rose Model
- •Use Case View
- •Logical View
- •Component View
- •Deployment View
- •Working with Rose
- •Creating Models
- •Saving Models
- •Exporting and Importing Models
- •Publishing Models to the Web
- •Working with Controlled Units
- •Using the Model Integrator
- •Working with Notes
- •Working with Packages
- •Adding Files and URLs to Rose Model Elements
- •Adding and Deleting Diagrams
- •Setting Global Options
- •Working with Fonts
- •Working with Colors
- •Summary
- •Chapter 3: Business Modeling
- •Introduction to Business Modeling
- •Why Model the Business?
- •Do I Need to Do Business Modeling?
- •Business Modeling in an Iterative Process
- •Business Actors
- •Business Workers
- •Business Use Cases
- •Business Use Case Diagrams
- •Activity Diagrams
- •Business Entities
- •Organization Unit
- •Where Do I Start?
- •Identifying the Business Actors
- •Identifying the Business Workers
- •Identifying the Business Use Cases
- •Showing the Interactions
- •Documenting the Details
- •Creating Business Use Case Diagrams
- •Deleting Business Use Case Diagrams
- •The Use Case Diagram Toolbar
- •Adding Business Use Cases
- •Business Use Case Specifications
- •Assigning a Priority to a Business Use Case
- •Viewing Diagrams for a Business Use Case
- •Viewing Relationships for a Business Use Case
- •Working with Business Actors
- •Adding Business Actors
- •Adding Actor Specifications
- •Assigning an Actor Stereotype
- •Setting Business Actor Multiplicity
- •Viewing Relationships for a Business Actor
- •Working with Relationships
- •Association Relationship
- •Generalization Relationship
- •Working with Organization Units
- •Adding Organization Units
- •Deleting Organization Units
- •Activity Diagrams
- •Adding an Activity Diagram
- •Adding Details to an Activity Diagram
- •Summary
- •Chapter 4: Use Cases and Actors
- •Use Case Modeling Concepts
- •Actors
- •Use Cases
- •Traceability
- •Flow of Events
- •Relationships
- •Use Case Diagrams
- •Activity Diagrams
- •Activity
- •Start and End States
- •Objects and Object Flows
- •Transitions
- •Synchronization
- •Working with Use Cases in Rational Rose
- •The Use Case Diagram Toolbar
- •Creating Use Case Diagrams
- •Deleting Use Case Diagrams
- •Adding Use Cases
- •Deleting Use Cases
- •Use Case Specifications
- •Naming a Use Case
- •Viewing Participants of a Use Case
- •Assigning a Use Case Stereotype
- •Assigning a Priority to a Use Case
- •Creating an Abstract Use Case
- •Viewing Diagrams for a Use Case
- •Viewing Relationships for a Use Case
- •Working with Actors
- •Adding Actors
- •Deleting Actors
- •Actor Specifications
- •Naming Actors
- •Assigning an Actor Stereotype
- •Setting Actor Multiplicity
- •Creating an Abstract Actor
- •Viewing Relationships for an Actor
- •Viewing an Actor's Instances
- •Working with Relationships
- •Association Relationship
- •Includes Relationship
- •Extends Relationship
- •Generalization Relationship
- •Working with Activity Diagrams
- •The Activity Diagram Toolbar
- •Creating Activity Diagrams
- •Deleting Activity Diagrams
- •Exercise
- •Problem Statement
- •Create a Use Case Diagram
- •Summary
- •Chapter 5: Object Interaction
- •Interaction Diagrams
- •What Is an Object?
- •What Is a Class?
- •Where Do I Start?
- •Finding Objects
- •Finding the Actor
- •Using Interaction Diagrams
- •Sequence Diagrams
- •The Sequence Diagram Toolbar
- •Collaboration Diagrams
- •The Collaboration Diagram Toolbar
- •Working with Actors on an Interaction Diagram
- •Working with Objects
- •Adding Objects to an Interaction Diagram
- •Deleting Objects from an Interaction Diagram
- •Setting Object Specifications
- •Naming an Object
- •Mapping an Object to a Class
- •Setting Object Persistence
- •Using Multiple Instances of an Object
- •Working with Messages
- •Adding Messages to an Interaction Diagram
- •Adding Messages to a Sequence Diagram
- •Deleting Messages from a Sequence Diagram
- •Reordering Messages in a Sequence Diagram
- •Message Numbering in a Sequence Diagram
- •Viewing the Focus of Control in a Sequence Diagram
- •Adding Messages to a Collaboration Diagram
- •Deleting Messages from a Collaboration Diagram
- •Message Numbering in a Collaboration Diagram
- •Adding Data Flows to a Collaboration Diagram
- •Setting Message Specifications
- •Naming a Message
- •Mapping a Message to an Operation
- •Setting Message Synchronization Options
- •Setting Message Frequency
- •End of a Lifeline
- •Working with Scripts
- •Switching Between Sequence and Collaboration Diagrams
- •Exercise
- •Problem Statement
- •Create Interaction Diagrams
- •Summary
- •Chapter 6: Classes and Packages
- •Logical View of a Rose Model
- •Class Diagrams
- •What Is a Class?
- •Finding Classes
- •Creating Class Diagrams
- •Deleting Class Diagrams
- •Organizing Items on a Class Diagram
- •Using the Class Diagram Toolbar
- •Working with Classes
- •Adding Classes
- •Class Stereotypes
- •Analysis Stereotypes
- •Class Types
- •Interfaces
- •Web Modeling Stereotypes
- •Other Language Stereotypes
- •Class Specifications
- •Naming a Class
- •Setting Class Visibility
- •Setting Class Multiplicity
- •Setting Storage Requirements for a Class
- •Setting Class Persistence
- •Setting Class Concurrency
- •Creating an Abstract Class
- •Viewing Class Attributes
- •Viewing Class Operations
- •Viewing Class Relationships
- •Using Nested Classes
- •Viewing the Interaction Diagrams That Contain a Class
- •Setting Java Class Specifications
- •Setting CORBA Class Specifications
- •Working with Packages
- •Adding Packages
- •Deleting Packages
- •Exercise
- •Problem Statement
- •Creating a Class Diagram
- •Summary
- •Chapter 7: Attributes and Operations
- •Working with Attributes
- •Finding Attributes
- •Adding Attributes
- •Deleting Attributes
- •Setting Attribute Specifications
- •Setting the Attribute Containment
- •Making an Attribute Static
- •Specifying a Derived Attribute
- •Working with Operations
- •Finding Operations
- •Adding Operations
- •Deleting Operations
- •Setting Operation Specifications
- •Adding Arguments to an Operation
- •Specifying the Operation Protocol
- •Specifying the Operation Qualifications
- •Specifying the Operation Exceptions
- •Specifying the Operation Size
- •Specifying the Operation Time
- •Specifying the Operation Concurrency
- •Specifying the Operation Preconditions
- •Specifying the Operation Postconditions
- •Specifying the Operation Semantics
- •Displaying Attributes and Operations on Class Diagrams
- •Showing Attributes
- •Showing Operations
- •Showing Visibility
- •Showing Stereotypes
- •Mapping Operations to Messages
- •Mapping an Operation to a Message on an Interaction Diagram
- •Exercise
- •Problem Statement
- •Add Attributes and Operations
- •Summary
- •Chapter 8: Relationships
- •Relationships
- •Types of Relationships
- •Finding Relationships
- •Associations
- •Using Web Association Stereotypes
- •Creating Associations
- •Deleting Associations
- •Dependencies
- •Creating Dependencies
- •Deleting Dependencies
- •Package Dependencies
- •Creating Package Dependencies
- •Deleting Package Dependencies
- •Aggregations
- •Creating Aggregations
- •Deleting Aggregations
- •Generalizations
- •Creating Generalizations
- •Deleting Generalizations
- •Working with Relationships
- •Setting Multiplicity
- •Using Relationship Names
- •Using Stereotypes
- •Using Roles
- •Setting Export Control
- •Using Static Relationships
- •Using Friend Relationships
- •Setting Containment
- •Using Qualifiers
- •Using Link Elements
- •Using Constraints
- •Exercise
- •Problem Statement
- •Adding Relationships
- •Summary
- •Chapter 9: Object Behavior
- •Statechart Diagrams
- •Creating a Statechart Diagram
- •Adding States
- •Adding State Details
- •Adding Transitions
- •Adding Transition Details
- •Adding Special States
- •Using Nested States and State History
- •Exercise
- •Problem Statement
- •Create a Statechart Diagram
- •Summary
- •Chapter 10: Component View
- •What Is a Component?
- •Types of Components
- •Component Diagrams
- •Creating Component Diagrams
- •Adding Components
- •Adding Component Details
- •Adding Component Dependencies
- •Exercise
- •Problem Statement
- •Summary
- •Chapter 11: Deployment View
- •Deployment Diagrams
- •Opening the Deployment Diagram
- •Adding Processors
- •Adding Processor Details
- •Adding Devices
- •Adding Device Details
- •Adding Connections
- •Adding Connection Details
- •Adding Processes
- •Exercise
- •Problem Statement
- •Create Deployment Diagram
- •Summary
- •Chapter 12: Introduction to Code Generation and Reverse Engineering Using Rational Rose
- •Preparing for Code Generation
- •Step One: Check the Model
- •Step Two: Create Components
- •Step Three: Map Classes to Components
- •Step Five: Select a Class, Component, or Package
- •Step Six: Generate Code
- •What Gets Generated?
- •Introduction to Reverse Engineering Using Rational Rose
- •Model Elements Created During Reverse Engineering
- •Summary
- •Chapter 13: ANSI C++ and Visual C++ Code Generation and Reverse Engineering
- •Generating Code in ANSI C++ and Visual C++
- •Converting a C++ Model to an ANSI C++ Model
- •Class Properties
- •Attribute Properties
- •Operation Properties
- •Package (Class Category) Properties
- •Component (Module Specification) Properties
- •Role Properties
- •Generalization Properties
- •Class Model Assistant
- •Component Properties
- •Project Properties
- •Visual C++ and ATL Objects
- •Generated Code
- •Code Generated for Classes
- •Code Generated for Attributes
- •Code Generated for Operations
- •Visual C++ Code Generation
- •Reverse Engineering ANSI C++
- •Reverse Engineering Visual C++
- •Summary
- •Overview
- •Introduction to Rose J
- •Beginning a Java Project
- •Selecting a Java Framework
- •Linking to IBM VisualAge for Java
- •Linking to Microsoft Visual J++
- •Project Properties
- •Class Properties
- •Attribute Properties
- •Operation Properties
- •Module Properties
- •Role Properties
- •Generating Code
- •Generated Code
- •Classes
- •Attributes
- •Operations
- •Bidirectional Associations
- •Unidirectional Associations
- •Associations with a Multiplicity of One to Many
- •Associations with a Multiplicity of Many to Many
- •Reflexive Associations
- •Aggregations
- •Dependency Relationships
- •Generalization Relationships
- •Interfaces
- •Java Beans
- •Support for J2EE
- •EJBs
- •Servlets
- •JAR and WAR Files
- •Automated J2EE Deployment
- •Reverse Engineering
- •Summary
- •Starting a Visual Basic Project
- •Class Properties
- •Attribute Properties
- •Operation Properties
- •Module Specification Properties
- •Role Properties
- •Generalization Properties
- •Generated Code
- •Classes
- •Attributes
- •Operations
- •Bidirectional Associations
- •Unidirectional Associations
- •Associations with a Multiplicity of One to Many
- •Associations with a Multiplicity of Many to Many
- •Reflexive Associations
- •Aggregations
- •Dependency Relationships
- •Generalization Relationships
- •Reverse Engineering
- •Summary
- •Overview
- •Introduction to XML DTD
- •Elements
- •Attributes
- •Entities and Notations
- •Project Properties
- •Class Properties
- •Attribute Properties
- •Role Properties
- •Component Properties
- •Generating Code
- •Generated Code
- •Classes
- •Attributes
- •Reverse Engineering DTD
- •Summary
- •Project Properties
- •Class Properties
- •Attribute Properties
- •Operation Properties
- •Module Properties
- •Association (Role) Properties
- •Dependency Properties
- •Generated Code
- •Classes
- •Attributes
- •Operations
- •Bidirectional Associations
- •Unidirectional Associations
- •Associations with a Multiplicity of One to Many
- •Associations with a Multiplicity of Many to Many
- •Associations with Bounded Multiplicity
- •Reflexive Associations
- •Aggregations
- •Dependency Relationships
- •Generalization Relationships
- •Reverse Engineering CORBA Source Code
- •Summary
- •Chapter 18: Rose Data Modeler
- •Object Models and Data Models
- •Creating a Data Model
- •Logic in a Data Model
- •Adding a Database
- •Adding Tablespaces
- •Adding a Schema
- •Creating a Data Model Diagram
- •Creating Domain Packages and Domains
- •Adding Tables
- •Adding Columns
- •Setting a Primary Key
- •Adding Constraints
- •Adding Triggers
- •Adding Indexes
- •Adding Stored Procedures
- •Adding Relationships
- •Adding Referential Integrity Rules
- •Working with Views
- •Generating an Object Model from a Data Model
- •Generating a Data Model from an Object Model
- •Generating a Database from a Data Model
- •Updating an Existing Database
- •Reverse Engineering a Database
- •Summary
- •Chapter 19: Web Modeling
- •Modeling a Web Application
- •Web Class Stereotypes
- •Relationships
- •Reverse Engineering a Web Application
- •Generating Code for a Web Application
- •Summary
- •Appendix: Getting Started with UML
- •Building a Business Use Case Diagram
- •Building a Workflow (Activity) Diagram
- •Building a Use Case Diagram
- •Building an Interaction Diagram
- •Building a Class Diagram
- •Web Modeling
- •Adding Class Relationships
- •Building a Statechart Diagram
- •Building a Component Diagram
- •Building a Deployment Diagram
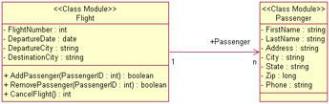
Chapter 15: Visual Basic Code Generation and Reverse Engineering
Again we have the Passenger and FrequentFlyerAccount classes, but this time the relationship is unidirectional. An attribute will be created inside Passenger, but not inside FrequentFlyerAccount. The following lines of code are from Passenger:
'##ModelId=39614F990350
Private Account As FrequentFlyerAccount
As you can see, Rose will generate a private attribute for the relationship at only one end of the association. Specifically, it will generate an attribute in the client class, but not in the supplier class.
The code generated in the supplier class includes all of the code lines discussed in the previous section about bidirectional associations. With a bidirectional association, each class is given a new attribute, and the code discussed in the previous section is included in both classes. With a unidirectional association, the code is included only in the client class.
Again, note that the multiplicity here is one to one. Let's take a look at how code is affected when the multiplicity settings are changed.
Associations with a Multiplicity of One to Many
In a one−to−one relationship, Rose can simply create the appropriate attributes to support the association. With a one−to−many relationship, however, one class must contain a set of the other class.
To begin, let's look at an example.
In this case, we have a one−to−many relationship. As we saw in the previous section, Flight can simply generate an attribute that is a reference to Passenger. However, a simple attribute in the Flight class won't be enough. Instead, the attribute generated in Flight must use a sort of container class or an array as its data type. (Rose will use a collection as the default.) The following code is generated in the Flight class:
'##ModelId=396154B0001F Public Passenger As Collection
Rose provides you with the Collection type as a container class. If you would rather, you can use an array by specifying the Subscript role property. To do so, open the specification window for the relationship and select the Visual Basic A or Visual Basic B tab. Then specify a value for the Subscript code−generation property. Here, we use a subscript of 3:
'##ModelId=396154B0001F
Public Passenger(3) As Passenger
Associations with a Multiplicity of Many to Many
The code generated here is similar to that created for a one−to−many relationship. In this type of relationship, however, Rose will generate container classes on both ends of the relationship.
534
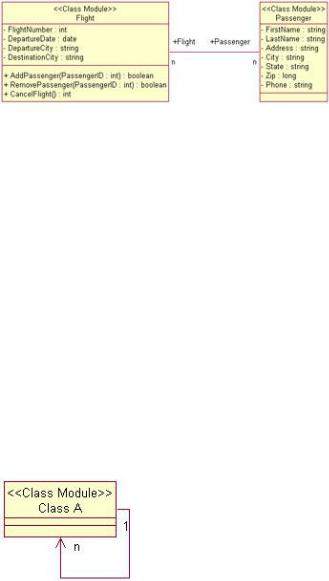
Chapter 15: Visual Basic Code Generation and Reverse Engineering
Let's look at the code generated for the following relationship:
In this situation, container classes are used at both ends of the relationship. The code that is generated will look something like the following two classes.
First, the Flight class will contain:
'##ModelId=396154B0001F Public Passenger As Collection
Next, the Passenger class will contain:
'##ModelId=396154B00021 Public Flight As Collection
Again, Rose uses a collection class as the default container, but you can change this to an array by modifying the Subscript code−generation property in the relationship specification window.
Reflexive Associations
A reflexive association is treated much the same as an association between two classes. For the following situation,
this code is generated:
'##ModelId=3961578B0369
Public NewProperty As Collection
As with a regular association, an attribute is created inside the class to support the relationship. If the multiplicity is one, a simple attribute is created. If the multiplicity is more than one, a container class is used.
As you can see, the code generated here is very similar to the code generated in a typical one−to−many relationship. In this situation, Class A contains an attribute of type Collection.
Aggregations
There are two types of aggregation relationships: by value and by reference. With a by−value relationship, one class contains another. With a by−reference relationship, one class contains a reference to another. Both
535

Chapter 15: Visual Basic Code Generation and Reverse Engineering
of these types of relationships are generated identically in Rose Visual Basic.
The code generated for an aggregation relationship is the same as the code generated for an association relationship. Let's look at an example:
The aggregation relationship tells us that, conceptually, a fleet is made up of many aircraft. When we generate code, however, Rose doesn't care whether there is an association or an aggregation between the classes. The Fleet class will contain a collection of Airplanes:
'##ModelId=396159720233
Public NewProperty As Collection
Dependency Relationships
With a dependency relationship, no attributes are created. If there is a dependency between ClassA and ClassB, no attributes will be created in either ClassA or ClassB.
Generalization Relationships
A generalization relationship in UML becomes an inheritance relationship in object−oriented languages. However, Visual Basic does not support inheritance. Instead, a generalization can become an implements delegation in Visual Basic. In your Rose model, a generalization relationship is shown as follows:
No special code will be inserted into the parent class. This allows it to be reusable and independent of the child class.
The child class contains three special areas of code. First, an Implements Parent statement is added. Second, an instance of the parent class is created inside the child class. Finally, copies of the parent's public methods are inserted into the child class.
Option Explicit
536