
Professional Java.JDK.5.Edition (Wrox)
.pdf
Chapter 7
Figure 7-6
The code below performs Expression Language checks to ensure that the user-specified filename is not null and its length is greater than zero. The code also performs checks with the JavaBean FileDir to determine if all of the input fields (Name, Telephone, Marking, Comments) have been properly entered. If that action has been performed properly, then the application will use that bean to upload the image file designated for upload and insert the meta data associated with that image into the picture database for future retrieval. The string validation checks are redundant for the filename attribute, but were shown to demonstrate how user form inputs might be implemented to ensure that proper data is propagated to back-end components:
<c:if test=”${param.filename != null && fn:length(param.filename) > 0}”> <%
if (fd.isValid()) {
if (fd.fileUpload(fd.getFilename())) { fd.addMetadata(fd.getName(), fd.getTelephone(), fd.getMarking(),
fd.getComments());
}
}else { System.out.println(“INVALID...”);
356

Developing Web Applications Using the Model 1 Architecture
}
%></c:if>
<form method=”post” action=”home.jsp?taxonomyIndex=102”>
<table border=”0” cellpadding=”0” cellspacing=”0” bgcolor=”#336699”><tbody> <tr valign=”bottom”><td nowrap=”nowrap”>
The following code represents the form values for input. Notice the JSP 2.0 syntax without scriplets, like ${fd.filename}. The Servlet 2.4 container, along with the JSP 2.0 container, now can handle EL expressions as native JSP syntax:
<table cellspacing=”2” cellpadding=”2”> <tbody>
<tr>
<td nowrap=”nowrap” class=”mandatory” colspan=”2”> Add Contact [ NOTE: All field inputs required ] </td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>File:</td> <td class=”value”>
<input type=”file” size=”60” name=”filename” value=”${fd.filename}”> </td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>Name:</td> <td class=”value”>
<input name=”name” value=”${fd.name}” size=”40” maxlength=”50”> </td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>Telephone:</td> <td class=”value”>
<input name=”telephone” value=”${fd.telephone}” size=”40” maxlength=”50”> </td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>Marking:</td> <td class=”value”>
<input name=”marking” value=”${fd.marking}” size=”40” maxlength=”50”> </td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>Comments:</td> <td class=”value”>
<input name=”comments” value=”${fd.comments}” size=”40” maxlength=”50”> </td>
</tr>
<tr>
<td align=”middle” class=”mandatory” colspan=”2”>
357

Chapter 7
<input type=”submit” name=”UploadFile” value=”Upload”> </td>
</tr>
</tbody>
</table>
</td></tr>
</tbody>
</table>
</form>
The Java Bean FileDir below accepts the inputs from the addContact.jsp file and performs checks to ensure that all of the proper fields have been entered prior to uploading the user-specified image and its related meta data:
// FileDir.java
package com.model1;
import java.io.*; import java.net.*; import java.sql.*; import java.util.*;
import java.util.logging.*; import java.nio.channels.*;
// needed for marking import java.awt.*;
import java.awt.image.BufferedImage; import javax.imageio.*;
import javax.imageio.stream.*; import javax.imageio.ImageIO.*;
import com.sun.media.imageio.plugins.tiff.TIFFImageWriteParam; import javax.imageio.plugins.jpeg.JPEGImageWriteParam;
public class FileDir {
private static Logger log = Logger.getLogger(“FileDir”);
private String txtPath = “C:\\tmp”; // image repository directory private Connection connection;
private Statement statement; private String driverName = “”; private String className = “”; private String user = “”; private String pass = “”;
// form entries
private String filename; private String name; private String telephone; private String marking; private String comments;
358

Developing Web Applications Using the Model 1 Architecture
The FileDir constructor uses a class loader object to discover the file.properties file that outlines the JDBC parameters needed to connect to the picture database. If all goes well, a connection object will be instantiated to perform operations on that database:
public FileDir() throws ClassNotFoundException, SQLException {
Properties resource = new Properties(); try {
URL url = this.getClass().getClassLoader().getResource(“file.properties”);
resource.load( new FileInputStream(url.getFile()) ); // Get properties
driverName = resource.getProperty(“driverName”); className = resource.getProperty(“className”); user = resource.getProperty(“user”);
pass = resource.getProperty(“pass”);
log.info(“Using parameters from the file.properties file for Contact Information.”);
}catch (Exception e) {
log.info(“[FileDir()] EXCEPTION. “ + e.toString());
}
Class.forName(className);
connection = DriverManager.getConnection(driverName,user, pass); statement = connection.createStatement();
}
The fileUpload method is passed the filename specified by the user in the Add Contact form above so that it can be uploaded to the filesystem for rendering when queried by the View Contact page. The method uses the NIO (New IO) libraries to stream the data from the local filesystem. A local file copy routine was introduced here for demonstration purposes only; normally, an enterprise Web upload application would handle remote file upload, too:
public boolean fileUpload(String srcFilename) {
String dstFilename = “”; log.info(“srcFilename= “ + srcFilename);
if ((srcFilename != null) && (!srcFilename.equals(“”))) {
int x = srcFilename.lastIndexOf(File.separatorChar)+1; if (x > 0) {
dstFilename = txtPath + File.separatorChar + srcFilename.substring(x);
log.info(“dstFilename= “ + dstFilename);
try {
File f = new File(dstFilename).getParentFile(); if (f.mkdirs() || f.isDirectory()) {
FileChannel srcChannel = new FileInputStream(srcFilename).getChannel();
FileChannel dstChannel =
new FileOutputStream(dstFilename).getChannel(); dstChannel.transferFrom(srcChannel, 0, srcChannel.size());
359
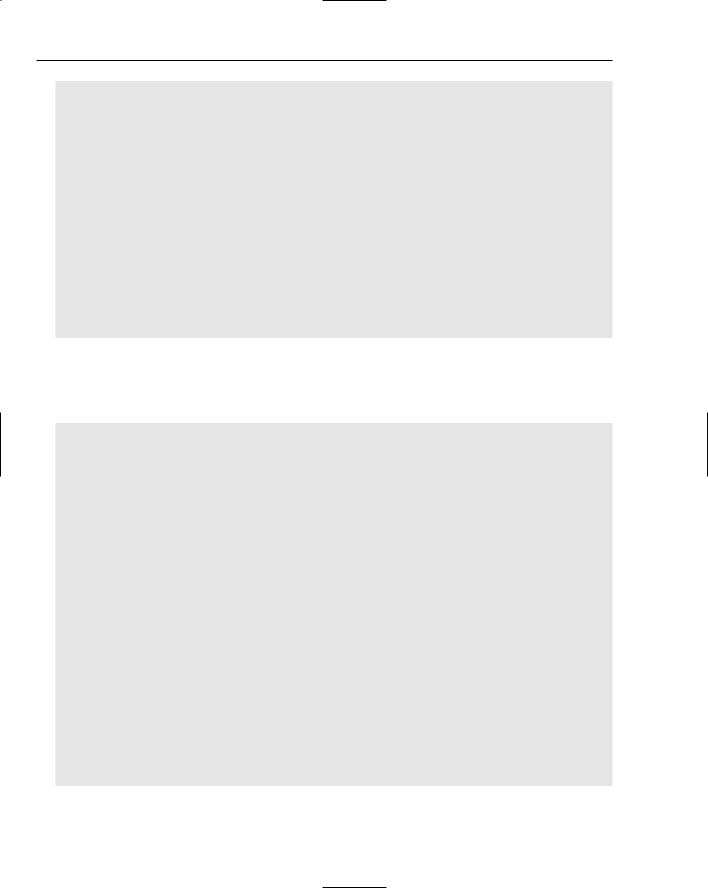
Chapter 7
srcChannel.close();
dstChannel.close();
log.info(“uploaded to “ + txtPath + “ file -> “ + dstFilename); } else {
log.info(“FAILURE with file [f]”); return false;
}
}catch (IOException e) { log.info(“IOException= “ + e.toString()); return false;
}
} else {
log.info(“could not parse: “ + srcFilename); return false;
}
}
return true;
}
The markImage method allows users to pass an image file with a marking value so that the marking can be overlayed on to that image file. The Java Image IO libraries are used to perform these actions. The sample application is set up so that *.jpeg images can be uploaded for tag marking. Logic has also been added to accommodate *.tif files, which would need to be uncommented in order to work:
public void markImage(String inFile, String outFile, String marking) {
try {
BufferedImage image = ImageIO.read(new File(inFile));
Graphics graphics = image.getGraphics(); graphics.setColor(Color.black);
graphics.setFont(new Font(“Arial”, Font.BOLD | Font.ITALIC, 20));
graphics.drawString(marking, (image.getWidth()*4/10), (image.getHeight() - (image.getHeight()/10)));
graphics.drawString(marking, (image.getWidth()*4/10) , image.getHeight()/10);
// Create Image
IIOImage iioImage = new javax.imageio.IIOImage(image, null, null);
//If TIFF document used, uncomment this and comment JPEG
//Iterator writers = ImageIO.getImageWritersByFormatName(“tiff”); Iterator writers = ImageIO.getImageWritersByFormatName(“jpeg”); ImageWriter writer = (ImageWriter)writers.next();
//Set WriteParam’s
//TIFFImageWriteParam writeParam = (TIFFImageWriteParam)writer.getDefaultWriteParam();
360

Developing Web Applications Using the Model 1 Architecture
// writeParam.setCompressionMode(ImageWriteParam.MODE_EXPLICIT); JPEGImageWriteParam writeParam =
(JPEGImageWriteParam)writer.getDefaultWriteParam();
// writeParam.setCompressionType(“CCITT T.6”); // for TIF compression writeParam.setCompressionType(“JPEG”);
//Create File to save the image File f = new File(outFile);
if (!f.exists()) f.createNewFile(); ImageOutputStream ios = createImageOutputStream(f); writer.setOutput(ios);
//Save the image
writer.write(null, iioImage, writeParam); ios.close();
} catch (IOException e) { log.info(“FILE FAILED:” + inFile);
}
}
The addMetadata method adds the meta data associated with the image file uploaded to the picture database. Additional checks on the meta data passed for database insertion could also be added to this routine to ensure that properly formatted data is passed to the database, but such checks were omitted for the sake of brevity. The string value passed in for marking text is passed along to the markImage method, which inserts that marking into the image file using the Java IO image libraries:
public void addMetadata(String name, String telephone, String marking, String comments) {
StringBuffer sqlString = new StringBuffer();
sqlString.append(“INSERT INTO picture (name, telephone_num, marking, comments) VALUES (“);
sqlString.append(“‘“ + name + “‘,”); sqlString.append(“‘“ + telephone + “‘,”); sqlString.append(“‘“ + marking + “‘,”); sqlString.append(“‘“ + comments + “‘);”);
try { statement.executeUpdate(sqlString.toString()); // mark images.
markImage(dstFilename, dstFilename, marking);
}catch (SQLException sqle) { log.info(“SQLException: “ + sqle.toString());
}
}
The getFiles method can be used to check the file system for files loaded to the c:\\tmp directory, which is the designated repository for image file uploads. Checks are performed on the filesystem to ensure that only *.gif and *.jpg files are loaded to the Map object that is returned to the routine that invoked it:
361

Chapter 7
public Map getFiles() {
Map map = new HashMap(); map = new TreeMap();
File dir = new File(“C:\\tmp”);
String[] children = dir.list();
if (children == null) {
log.info(“Directory does not exist, or perhaps is NOT a directory.”); } else {
for (int i=0; i < children.length; i++) {
//Get filename of file or directory String filename = children[i];
if ((filename.endsWith(“.gif”)) || (filename.endsWith(“.jpg”))) map.put(filename, filename);
}
//Iterate over the keys in the map
Iterator it = map.keySet().iterator(); while (it.hasNext()) {
Object key = it.next(); log.info(“[FileDir] key= “ + key);
}
}
return map;
}
The next section of the FileDir code represents the setter methods that are used by the Add Contact form to persist the form parameters that have been entered by the form user:
// setters
public void setFilename(String filename) { this.filename = filename;
}
public void setName(String name) { this.name = name;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public void setMarking(String marking) { this.marking = marking;
}
public void setComments(String comments) { this.comments = comments;
}
362

Developing Web Applications Using the Model 1 Architecture
The getter methods below retrieve the form values so that the user form can ensure that all the fields have been properly entered by the user, prior to having the FileDir JavaBean application upload and save the meta data:
// getters
public String getFilename() { return filename;
}
public String getName() { return name;
}
public String getTelephone() { return telephone;
}
public String getMarking() { return marking;
}
public String getComments() { return comments;
}
public boolean isValid() {
return ( (filename != null) && (!filename.equals(“”)) && (name != null) && (!name.equals(“”)) && (telephone != null) && (!telephone.equals(“”)) && (marking != null) && (!marking.equals(“”)) && (comments != null) && (!comments.equals(“”)) );
}
public static void main(String[] args) {
try {
FileDir fd = new FileDir(); log.info(“getting files...”); fd.getFiles();
String s = “c:\\tmp\\test\\screenshot1.gif”; fd.fileUpload(s);
}catch(Exception e) { log.info(“EXCEPTION: “ + e.toString());
}
}
}
The FileDir Bean application demonstrates how Java components can be constructed with robust libraries to facilitate form processing and data persistence activities. The key to good bean development is to migrate common methods with one another for easy maintenance and to provide simple interfaces to data so that users will be more likely to incorporate them into their presentation code.
363

Chapter 7
Summar y
This chapter has demonstrated two different implementations of database transactions using both tag libraries and JavaBean components within the Contact Management Tool incorporating the Model 1 Architecture approach. The page flows were hard-coded to accommodate user navigations from a taxonomy drill-down component, with the assistance of core tag library logic operators and JavaBean validation methods. Naturally, not all of the features of the JSP 2.0 and JSTL 1.1 specifications and API libraries could be discussed in this chapter alone, but the sample Web application that was constructed should provide ample knowledge on how to craft J2EE Web components for the needs of the presentation tier.
Hopefully, you will find that this chapter eliminates some of your problems with your development operations and enhances your ability to make knowledgeable design decisions concerning Model 1 Architecture deployments.
364

Developing Web
Applications Using the
Model 2 Architecture
In the last chapter, you learned about building Web applications using the Model 1 Architecture, which is heavily dependent on a page-centric development focus. In this chapter, you will review and apply a prominent pattern in software development known as Model-View-Controller (MVC) to build Web applications in a more modular and componentized manner. You will learn a little about the Model 2 Architecture, particularly a framework known as WebWork, and its use of a concept known as Inversion of Control. You will see an example of how componentized development with WebWork provides a tremendous advantage to you as a Web developer, as it saves you time in having to rebuild the same components over and over in your application.
The Problem
Imagine your office needed a centralized contact manager for referencing people that could be used for given projects. You know that such functionality would be useful, but are worried about trying to do something too ad hoc and inflexible, leading to it being quickly thrown away.
You need something quick, but flexible. You need something where you can reuse a lot of components to build your solution. You need to look at a Model 2 Architecture framework.
What Is Model 2?
In order to understand Model 2, you should review the Model-View-Controller paradigm, which you examined in depth in Chapter 3, “Exploiting Patterns in Java.” As you saw in Chapter 3, MVC