
Professional Java.JDK.5.Edition (Wrox)
.pdf
Chapter 7
</tr>
</table>
</td>
</tr>
</table>
</td>
</table>
</td>
</tr>
</c:forEach>
</td></tr></table>
</td></tr></table>
<br>
<br>
The resulting display is demonstrated in the Contact Management Tool screenshot (see Figure 7-3 following). The JSP script culls the picture database for the image and meta data associated with that image for rendering. The person that is marked in the file text is hyperlinked so that users can click it and obtain more information about the selected contact.
Figure 7-3
346

Developing Web Applications Using the Model 1 Architecture
The next application, addProfile.jsp, uses both the core tag libraries for logic operations and the SQL actions to perform form processing actions on the Add Profile page. Once the form has been properly filled out, checks will be done to ensure that required fields have been entered. Once those checks have been performed, and the application has determined that the form entries can be pushed to the back-end database, the application will send the data to the registration database for storage and subsequent retrievals:
<%@ taglib prefix=”c” uri=”http://java.sun.com/jstl/core” %> <%@ taglib prefix=”fmt” uri=”http://java.sun.com/jstl/fmt” %> <%@ taglib uri=”http://java.sun.com/jstl/sql_rt” prefix=”sql” %>
<script language=”JavaScript”>
function textCounter(field, countfield, maxlimit) { if (field.value.length > maxlimit) {
field.value = field.value.substring(0, maxlimit); } else {
countfield.value = maxlimit - field.value.length;
}
}
</script>
The JSTL 1.1 core library tags are used below to perform logic operations on the form entries specified by the user. If either firstName, lastName, or email is empty, the application will not allow the form to pass the data to the back-end registration database:
<c:if test=”${param.submitted}”>
<c:if test=”${empty param.firstName}” var=”noFirstName” /> <c:if test=”${empty param.lastName}” var=”noLastName” /> <c:if test=”${empty param.email}” var=”noEmail” />
<c:if test=”${not (noFirstName or noLastName or noEmail)}”>
<c:set value=”${param.firstName}” var=”firstName” scope=”request”/> <c:set value=”${param.lastName}” var=”lastName” scope=”request”/> <c:set value=”${param.email}” var=”email” scope=”request”/>
Once the proper form entries have been entered by the user, the data source will be established with the SQL action tags by passing familiar JDBC driver, URL, username, and password parameters to the library to create a connection. After the connection has been created, then the SQL update tag can be used to perform an insert operation on the registration database using a prepared statement construct:
<sql:setDataSource
var=”datasource”
driver=”org.gjt.mm.mysql.Driver”
url=”jdbc:mysql://localhost/registration”
user=””
password=””
scope=”page”/>
<sql:update dataSource=”${datasource}”>
INSERT INTO registration (registration_id, first_name, last_name, email) VALUES(?, ?, ?, ?)
347

Chapter 7
<sql:param value=”${param.firstName}” /> <sql:param value=”${param.lastName}” /> <sql:param value=”${param.email}” />
</sql:update>
</c:if>
</c:if>
The following code represents the registration form and its components that will be used to register contacts in the Contact Management Tool. Expression Language constructs, like ${param.lastName}, are used to represent and persist data items entered by the form user:
<form method=”post”>
<table border=”0” cellpadding=”0” cellspacing=”0”><tbody>
<tr valign=”bottom”> <td nowrap=”nowrap”>
<table cellspacing=”2” cellpadding=”2” bgcolor=”#336699”> <tbody>
<tr>
<td nowrap=”nowrap” colspan=”2”>Registration</td> </tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>First Name: (required)</td> <td class=”value”>
<input name=”firstName” value=”${param.firstName}” size=”25” maxlength=”50”>
<c:if test=”${noFirstName}”> <small><font color=”red”> Please enter a First Name </font></small>
</c:if>
</td>
</tr>
<tr>
<td nowrap=”nowrap” class=”mandatory”>Last Name: (required)</td> <td class=”value”>
<input name=”lastName” value=”${param.lastName}” size=”25” maxlength=”50”> <c:if test=”${noLastName}”>
<small><font color=”red”> Please enter a Last Name </font></small>
</c:if>
</td>
348

Developing Web Applications Using the Model 1 Architecture
</tr>
<!--- Email, Gender, Marital Status, Date of Birth, Country, Zip Code, Age, Place of Birth, Occupation and Interests components were omitted for the sake of brevity -- >
<tr>
<td align=”left” nowrap=”nowrap” class=”field” colspan=”2”> Characters remaining:
<input readonly=”readonly” type=”text” name=”inputcount” size=”5” maxlength=”4” value=”” class=”text”>
<br>
<script language=”JavaScript”> document.form1.inputcount.value = (200 -
document.form1.interests.value.length);
</script>
</td>
</tr>
<tr>
<td nowrap=”nowrap” class=”field” align=”middle” colspan=”2”> <input type=”hidden” name=”submitted” value=”true” />
<input type=”submit” value=”Register” /> </td>
</tr>
</tbody>
</table>
</form>
The form visualization (see Figure 7-4 following) is the result of the code fragments in the addProfile.jsp script described above. Some JavaScript code was used for the comments section to provide client-side validation, which ensures that the user does not enter more than 200 characters.
349
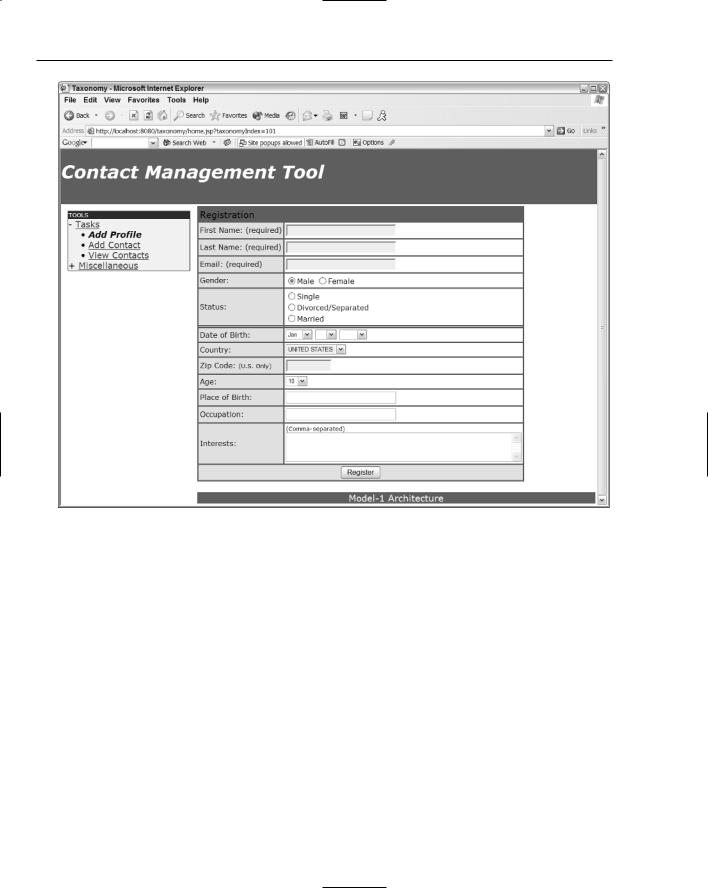
Chapter 7
Figure 7-4
Developing Your Web Application Visualizations with JSP 2.0
Java Server Pages (JSPs) are generally implemented in distributed systems to aggregate content with back-end components for user visualizations. When application servers first receive a request from a JSP component, the JSP engine compiles that page into a servlet. Additionally, when changes to a JSP occur, that same component will be recompiled into a servlet again where it will be processed by a class loader so that it can restart its life cycle in the Web container.
A general best practice for developing Web components is to use JSPs for display generation and servlets for processing requests. The idea is to encapsulate complicated business logic in JavaBean components written in Java that are entirely devoid of scriplet syntax so that display scripts are not obfuscated with complicated logic that might make your code hard to decipher for maintenance purposes. Naturally, your JavaBean code artifacts will transfer across platforms because they are written in Java, which accommodates reuse in your overall development operations.
350

Developing Web Applications Using the Model 1 Architecture
The benefits of JSP technology include the following points:
Code reuse across disparate platforms. Components and tag libraries can be shared in development operations and among different tools.
Separation of roles. Web designers can work presentation scripts and developers can work back-end data transaction activities.
Separation of content. Both static and dynamic content can be “template-tized”, which inevitably facilitates coding operations.
A JSP page has two distinct phases during operations: translation and execution. During translation, the Web container validates the syntax of a JSP script. The Web container manages the class instances of a JSP during the execution phase as user requests are made for it.
Figure 7-5 conceptualizes how a Web page can be constructed using the Model 1 Architecture.
home.jsp
header.jsp
View #1
View #2
View #3
NOTE:
As a user clicks down the taxonomy in the leftNav.jsp,, a different parameter will be generated and passed to the content.jsp page. Logic inside the content.jsp component will be used to determine which view to present to the user display.
leftNav.jsp |
content.jsp |
footer.jsp
Figure 7-5
The following code is used by the leftNav.jsp page to read an XML file so that a drill-down navigation component can be used by a user to select different views for presentation. This application uses an open source product called dom4j to extract and navigate links from a hierarchical tree in an XML file. The dom4j library is a great tool for parsing and manipulating content because it offers full support for JAXP, SAX, DOM, and XSLT. It also has Xpath support for navigating XML artifacts, and is based on Java interfaces for flexible support:
351
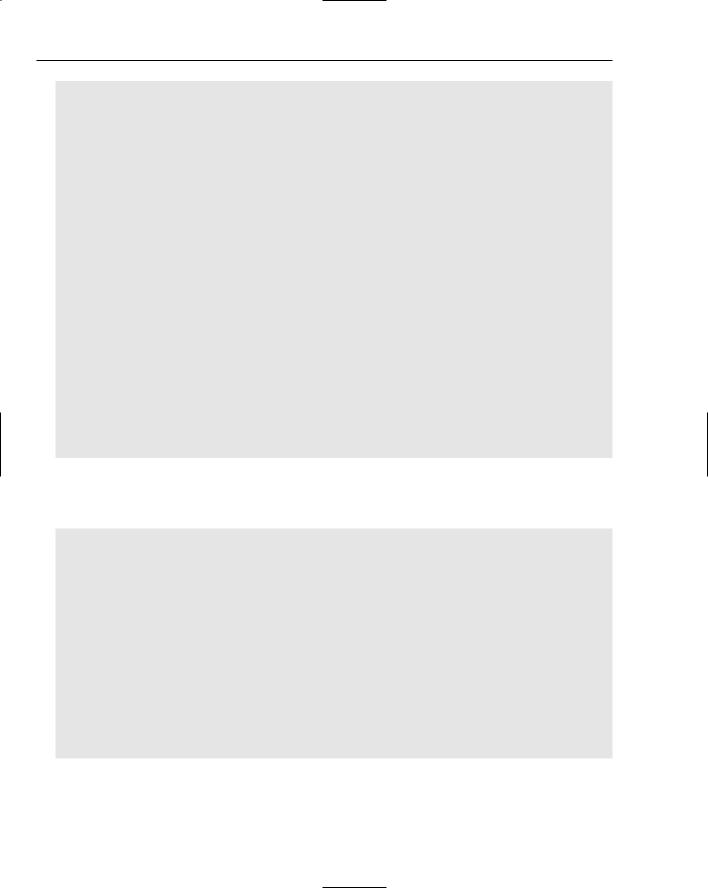
Chapter 7
package taxonomy;
/**
*@author MMitchell
*To change this generated comment edit the template variable “typecomment”:
*Window>Preferences>Java>Templates.
*To enable and disable the creation of type comments go to
*Window>Preferences>Java>Code Generation.
*/
import java.net.*; import java.util.*; import javax.naming.*;
import java.util.logging.*; import org.dom4j.*;
import org.dom4j.io.*;
public class TopicGenerator {
protected static String EXPANDED_SYMBOL = “- ”; protected static String COLLAPSED_SYMBOL = “+ ”; protected static String LEAF = “ ”; protected static String SPACER = “ ”;
protected static String TOPIC_CSS_CLASS = “SelectedTopic”;
private Document document;
private static Logger log = Logger.getLogger(“TopicGenerator”);
The following code represents the constructor methods for the TopicGenerator application that reads the Topic.xml file into memory so that it can be navigated by users in the Contact Management Tool, which in turn will render a different view in the content.jsp page:
public TopicGenerator() throws NamingException { Context initCtx = new InitialContext();
Context ctx = (Context) initCtx.lookup(“java:comp/env”); String topicsDirectory = (String) ctx.lookup(“topicsDirectory”);
setFilename(topicsDirectory + java.io.File.separator + “Topic.xml”); log.info(“topicsDirectory= “ + topicsDirectory);
}
public TopicGenerator(String fileName) throws Exception { parseDocument(fileName);
if (this.document == null)
throw new Exception(“Problem initializing TopicGenerator”);
}
public void setFilename(String fileName) { parseDocument(fileName);
}
352

Developing Web Applications Using the Model 1 Architecture
The parseDocument method initiates the application by reading the filename Topic.xml into memory for manipulation:
private void parseDocument(String fileName) { try {
SAXReader reader = new SAXReader(); document = reader.read(fileName);
} catch (DocumentException de) {
log.info(“Problem initializing TopicGenerator.”);
de.printStackTrace();
}catch (MalformedURLException me) { log.info(“Malformed URL.”); me.printStackTrace();
}
}
The addElement method adds hyperlinked elements to the navigation tree for visualization. The implementation of the StringBuffer class is preferred over String class concatenation because of significant performance differences:
private void addElement(StringBuffer sb, Set set, Element element, String topicId,
String topicParamName, String href, String extraParams, int level) {
if (level != -1) {
// write current node
for (int i = 0; i < level*4; i++) sb.append(TopicGenerator.SPACER);
if (element.elements().isEmpty()) sb.append(TopicGenerator.LEAF);
else if (set.contains(element)) sb.append(TopicGenerator.EXPANDED_SYMBOL);
else sb.append(TopicGenerator.COLLAPSED_SYMBOL);
String thisTopicId = element.attributeValue(“value”);
if (topicId.equals(thisTopicId))
sb.append(“<a class=\”” + TopicGenerator.TOPIC_CSS_CLASS + “\” “); else
sb.append(“<a “);
sb.append(“href=\””);
sb.append(href);
sb.append(‘?’);
sb.append(topicParamName);
sb.append(‘=’);
sb.append(thisTopicId);
sb.append(extraParams);
sb.append(“\”>” + element.attributeValue(“text”) + “</a><br>”);
353
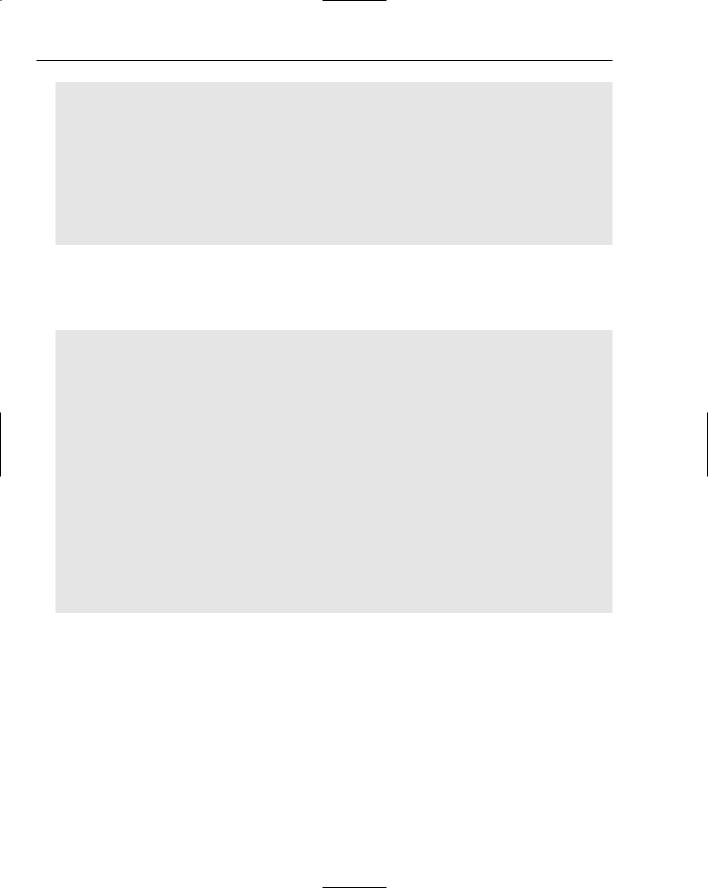
Chapter 7
}
if (set.contains(element) || level == -1) { Iterator it = element.elementIterator(); while (it.hasNext()) {
Element currElement = (Element) it.next();
addElement(sb, set, currElement, topicId, topicParamName, href,
extraParams,
level + 1);
}
}
}
The generateParams method receives a HashMap object from the getTopics method so that it can generate the parameters needed for traversal. Please note that it is always a good practice to check the input parameters that are passed into a method prior to performing operations on them as has been done in the following example code:
private String generateParams(HashMap params) { if (params == null || params.isEmpty())
return “”;
StringBuffer toReturn = new StringBuffer();
Iterator keys = params.keySet().iterator(); while (keys.hasNext()) {
String currParam = (String) keys.next();
String currParamValue = (String) params.get(currParam);
if (currParamValue != null) { toReturn.append(‘&’); toReturn.append(currParam); toReturn.append(‘=’); toReturn.append(currParamValue);
}
}
return toReturn.toString();
}
The getTopics method establishes a tree structure based on the taxonomies residing in the Topic.xml file, which is discovered by a context lookup at the onset of the application. The overloaded getTopics methods return the topic values that are read from the taxonomy for user navigation:
354
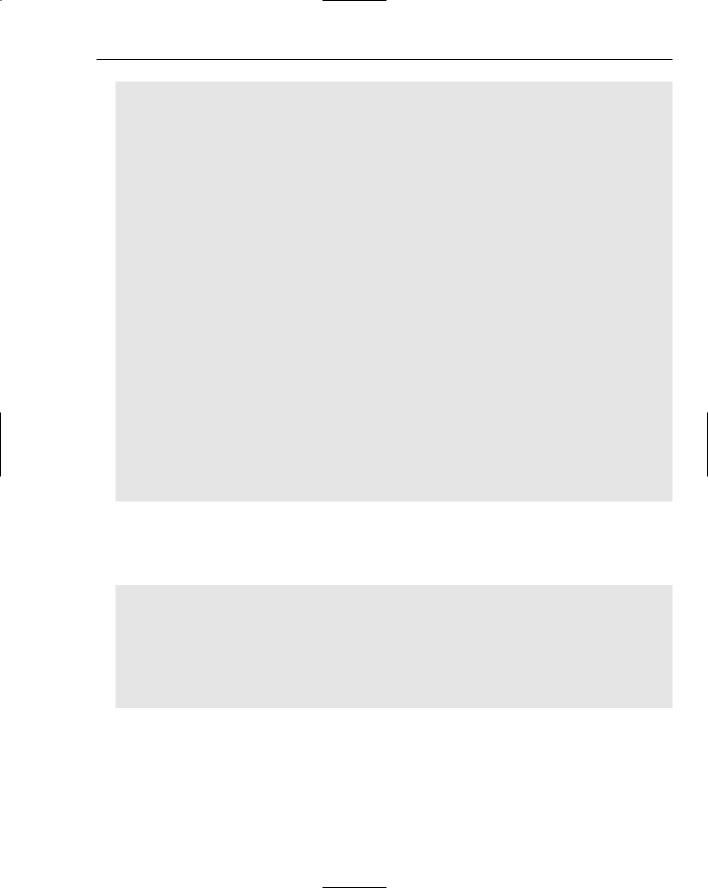
Developing Web Applications Using the Model 1 Architecture
public String getTopics(String topicId, String topicParamName, String href, HashMap params) {
if (topicId == null) topicId = “”;
Element taxonomy = (Element) document.selectSingleNode(“/navigation/taxonomy”);
List list = taxonomy.selectNodes(“//topic[@value=’” + topicId + “‘]/ ancestor-or-self::*”);
Set expandedNodeSet = new HashSet(list);
StringBuffer toReturn = new StringBuffer();
addElement(toReturn, expandedNodeSet, taxonomy, topicId, topicParamName,
href,
generateParams(params), -1);
return toReturn.toString();
}
public String getTopics(String topicId, String topicParamName, String href) { HashMap params = new HashMap();
return getTopics(topicId, topicParamName, href, params);
}
public static void main(String[] args) throws Exception {
String s = “c:\\tomcat5019\\webapps\\mojo\\WEB-INF\\classes\\Topic.xml”);” TopicGenerator tg = new TopicGenerator(s);
}
}
In our GUI presentation shown in Figure 7-6, when a user attempts to add a new contact to the Contact Management Tool, a form will be presented to the user for a picture and meta data that will be associated with that picture. The Web application uses JSP 2.0 Expression Language (EL) features to present data, and JavaBean components to persist and manipulate contact data for retrieval and storage.
<%@ page language=”java” contentType=”text/html”
import=”java.util.*,java.nio.channels.*,java.lang.*,java.io.*,com.model1.*” %>
<jsp:useBean id=”fd” class=”com.model1.FileDir” scope=”request”> <jsp:setProperty name=”fd” property=”*”/>
</jsp:useBean>
<title>Insert Contact</title>
355