
Professional Java.JDK.5.Edition (Wrox)
.pdf

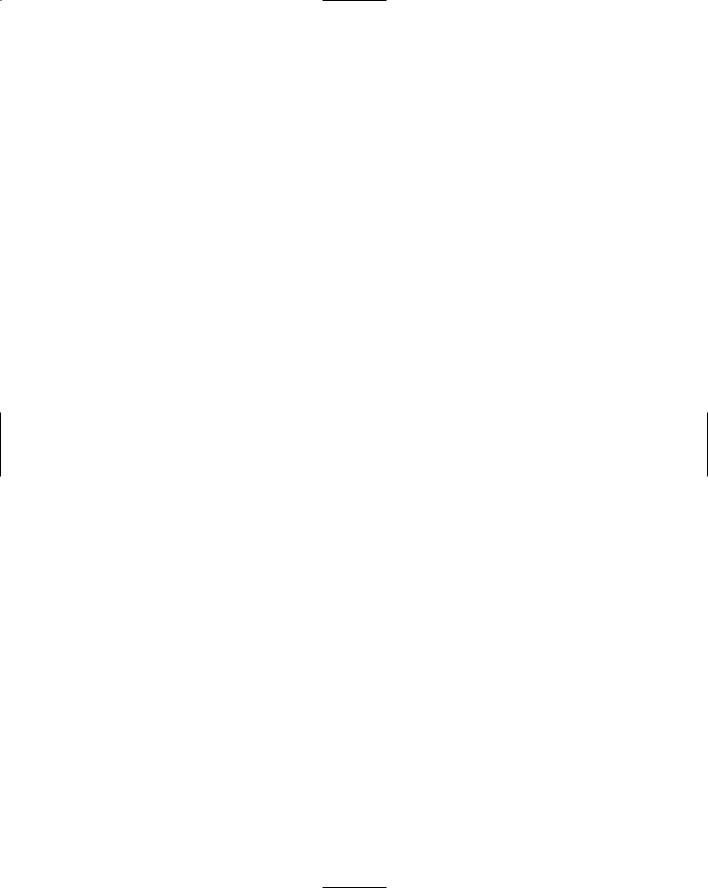
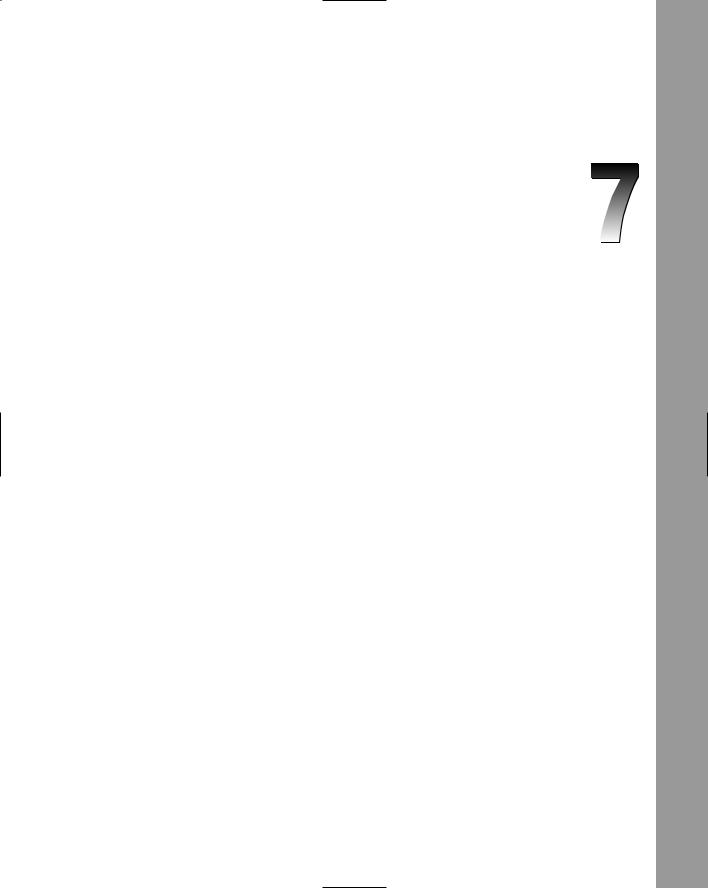
Developing Web
Applications Using the
Model 1 Architecture
Software development activities generally involve domain-driven speculations that attempt to tackle complexity by aggregating knowledge of a subject matter so that it can be handled for your own purposes. This reflection generally involves experimentation by software and domain experts to organize knowledge for use by development teams. Ultimately, these modeling tasks involve the abstraction and filtering of nonessential data and the attainment of purposeful knowledge so that developer needs are served and proper deployments are made to customers.
This chapter will demonstrate how you can overcome speculation over how to construct a Web application using the Model 1 Architecture by constructing a hands-on Contact Management Tool. Two different types of Java syntax, JSTL 1.1 and JSP 2.0, will be utilized to craft the sample GUI component that will allow users to manage contact information through upload and query activities. The sample application’s use of Model 1 was chosen to suit design and implementation needs for a quick prototype that can be implemented by novice Java Web developers in an easy fashion, and to demonstrate some of the new Java language enhancements that were delivered with the JSTL 1.1 and JSP 2.0 specifications.
What Is Model 1? Why Use It?
The Model 1 Architecture is a page-centric approach where page flows are handled by individual Web components. This means that request and response processing are hard-coded into pages to accommodate user navigations in a Web application. With Model 2 Architecture, navigation flows are generally handled by a servlet controller that works in conjunction with configuration files to dictate page renderings during application operations.





