
3D Game Programming All In One (2004)
.pdf
268Chapter 7 ■ Common Scripts
actual chat message in a tagged string. The rest of the parameters are not actually acted on
so can be safely ignored for now. The parameters are passed on to the pseudo-handler OnChatMessage. It's called a pseudo-handler because the function that calls OnChatMessage is not really calling out from the engine. However, it is useful to treat this operation as if a callback message and handler were involved for conceptual reasons.
The next function, ClientCmdServerMessage, is used to deal with game event descriptions, which may or may not include text messages. These can be sent using the message functions in the server-side Message module. Those functions use CommandToClient with the type ServerMessage, which invokes the function described next.
For ServerMessage messages, the client can install callbacks that will be run according to the type of the message.
Obviously, ClientCmdServerMessage is more involved. After it uses the GetWord function to extract the message type as the first text word from the string %msgType, it iterates through the message callback array ($MSGCB) looking for any untyped callback functions and executes them all. It then goes through the array again, looking for registered callback functions with the same message type as the incoming message, executing any that it finds.
The next function, addMessageCallback, is used to register callback functions in the $MSGCB message callback array. This is not complex; addMessageCallback merely steps through the array looking for the function to be registered. If it isn't there, addMessageCallback stores a handle to the function in the next available slot.
The last function, DefaultMessageCallback, is supplied in order to provide an untyped message to be registered. The registration takes place with the line after the function definition.
A Final Word
The common code base includes a ton of functions and methods. We have only touched on about half of them here. I aimed to show you the most important modules and their contents, and I think that's been accomplished nicely. For your browsing pleasure, Table 7.2 contains a reference to find all the functions in all common code modules.
Team LRN
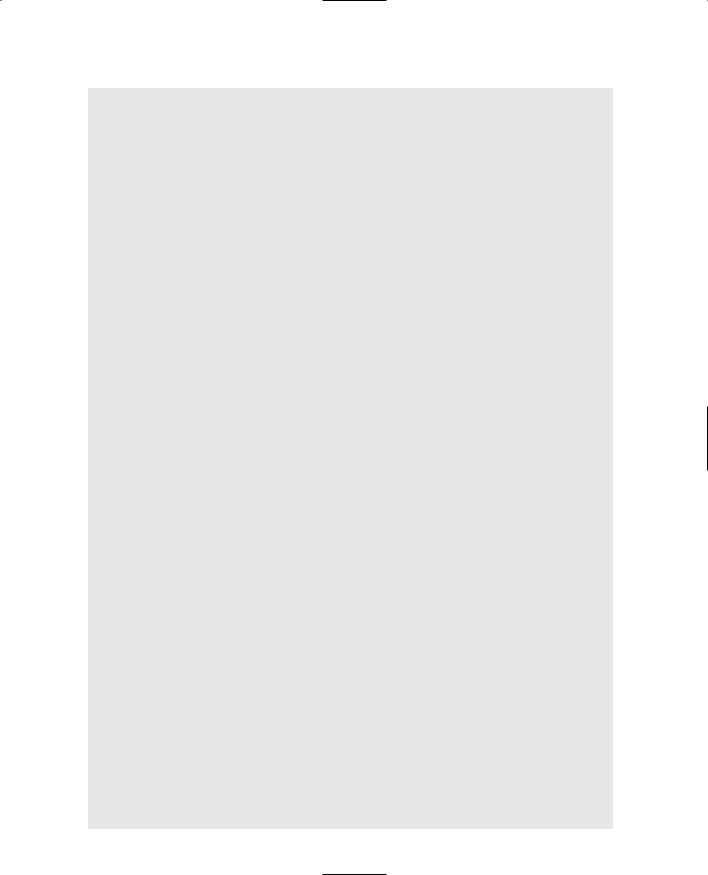
A Final Word 269
Table 7.2 Common Code Functions
Module |
Function |
common/main.cs |
InitCommon |
|
InitBaseClient |
|
InitBaseServer |
|
DisplayHelp |
|
ParseArgs |
|
OnStart |
|
OnExit |
common/client/actionMap.cs |
ActionMap::copyBind |
|
ActionMap::blockBind |
common/client/audio.cs |
OpenALInit |
|
OpenALShutdown |
common/client/canvas.cs |
InitCanvas |
|
ResetCanvas |
common/client/cursor.cs |
CursorOff |
|
CursorOn |
|
GuiCanvas::checkCursor |
|
GuiCanvas::setContent |
|
GuiCanvas::pushDialog |
|
GuiCanvas::popDialog |
|
GuiCanvas::popLayer |
common/client/help.cs |
HelpDlg::onWake |
|
HelpFileList::onSelect |
|
GetHelp |
|
ContextHelp |
|
GuiControl::getHelpPage |
|
GuiMLTextCtrl::onURL |
common/client/message.cs |
ClientCmdChatMessage |
|
ClientCmdServerMessage |
|
AddMessageCallback |
|
DefaultMessageCallback |
common/client/messageBox.cs |
MessageCallback |
|
MBSetText |
|
MessageBoxOK |
|
MessageBoxOKDlg::onSleep |
|
MessageBoxOKCancel |
|
MessageBoxOKCancelDlg::onSleep |
|
MessageBoxYesNo |
|
MessageBoxYesNoDlg::onSleep |
|
MessagePopup |
|
CloseMessagePopup |
continued
Team LRN
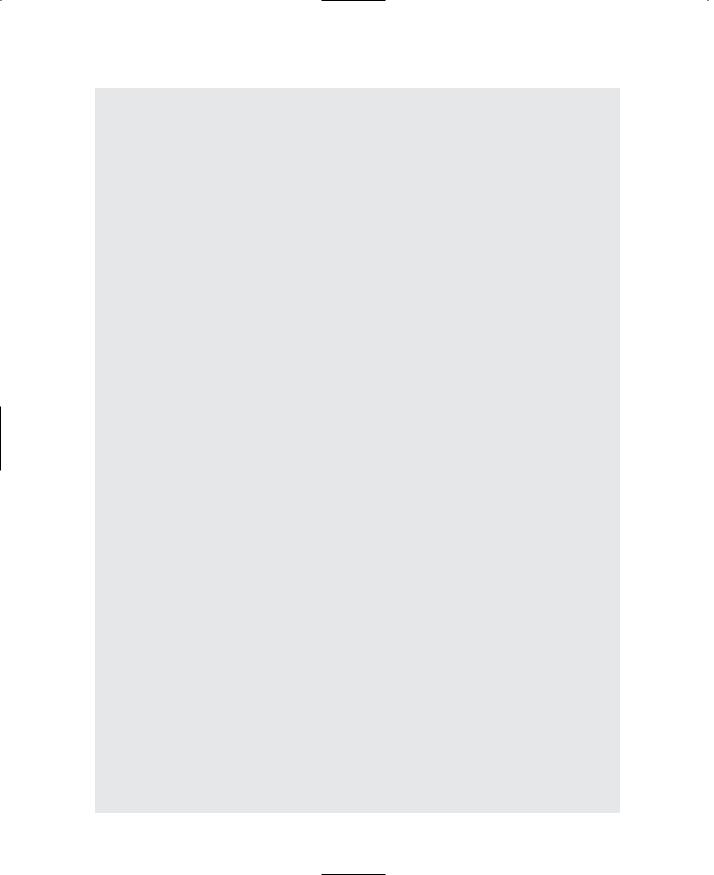
270 Chapter 7 ■ Common Scripts
common/client/metrics.cs FpsMetricsCallback TerrainMetricsCallback VideoMetricsCallback InteriorMetricsCallback TextureMetricsCallback WaterMetricsCallback TimeMetricsCallback VehicleMetricsCallback AudioMetricsCallback DebugMetricsCallback Metrics
common/client/mission.cs ClientCmdMissionStart ClientCmdMissionEnd
common/client/missionDownload.cs ClientCmdMissionStartPhase1 OnDataBlockObjectReceived ClientCmdMissionStartPhase2 OnGhostAlwaysStarted OnGhostAlwaysObjectReceived ClientCmdMissionStartPhase3 UpdateLightingProgress SceneLightingComplete
common/client/recordings.cs RecordingsDlg::onWake StartSelectedDemo StartDemoRecord StopDemoRecord DemoPlaybackComplete
common/client/screenshot.cs FormatImageNumber RecordMovie MovieGrabScreen StopMovie DoScreenShot
common/server/audio.cs ServerPlay2D ServerPlay3D
common/server/clientConnection.cs GameConnection::onConnectRequest GameConnection::onConnect GameConnection::setPlayerName IsNameUnique GameConnection::onDrop GameConnection::startMission GameConnection::endMission GameConnection::syncClock
GameConnection::incScore
continued
Team LRN

|
|
A Final Word |
271 |
|
|
|
|
common/server/commands.cs |
ServerCmdSAD |
|
|
|
ServerCmdSADSetPassword |
|
|
|
ServerCmdTeamMessageSent |
|
|
|
ServerCmdMessageSent |
|
|
common/server/game.cs |
OnServerCreated |
|
|
|
OnServerDestroyed |
|
|
|
OnMissionLoaded |
|
|
|
OnMissionEnded |
|
|
|
OnMissionReset |
|
|
|
GameConnection::onClientEnterGame |
|
|
|
GameConnection::onClientLeaveGame |
|
|
|
CreateGame |
|
|
|
DestroyGame |
|
|
|
StartGame |
|
|
|
EndGame |
|
|
common/server/kickban.cs |
Kick |
|
|
|
Ban |
|
|
common/server/message.cs |
MessageClient |
|
|
|
MessageTeam |
|
|
|
MessageTeamExcept |
|
|
|
MessageAll |
|
|
|
MessageAllExcept |
|
|
|
GameConnection::spamMessageTimeout |
|
|
|
GameConnection::spamReset |
|
|
|
SpamAlert |
|
|
|
ChatMessageClient |
|
|
|
ChatMessageTeam |
|
|
|
ChatMessageAll |
|
|
common/server/missionDownload.cs |
GameConnection::loadMission |
|
|
|
ServerCmdMissionStartPhase1Ack |
|
|
|
GameConnection::onDataBlocksDone |
|
|
|
ServerCmdMissionStartPhase2Ack |
|
|
|
GameConnection::clientWantsGhostAlwaysRetry |
|
|
|
GameConnection::onGhostAlwaysFailed |
|
|
|
GameConnection::onGhostAlwaysObjectsReceived |
|
|
|
ServerCmdMissionStartPhase3Ack |
|
|
common/server/missionInfo.cs |
ClearLoadInfo |
|
|
|
BuildLoadInfo |
|
|
|
DumpLoadInfo |
|
|
|
SendLoadInfoToClient |
|
|
|
LoadMission |
|
|
|
LoadMissionStage2 |
|
|
|
EndMission |
|
|
|
ResetMission |
continued |
|
|
|
|
|
Team LRN

272 Chapter 7 ■ Common Scripts
common/server/missionLoad.cs LoadMission LoadMissionStage2 EndMission ResetMission
common/server/server.cs PortInit CreateServer DestroyServer ResetServerDefaults AddToServerGuidList
RemoveFromServerGuidList OnServerInfoQuery
common/ui/ConsoleDlg.gui ConsoleEntry::eval ToggleConsole
common/ui/GuiEditorGui.gui GuiEditorStartCreate GuiEditorCreate GuiEditorSaveGui GuiEditorSaveGuiCallback GuiEdit
GuiEditorOpen
GuiEditorContentList::onSelect
GuiEditorClassPopup::onSelect
GuiEditorTreeView::onSelect
GuiEditorInspectApply
GuiEditor::onSelect
GuiEditorDeleteSelected
Inspect
InspectApply
InspectTreeView::onSelect
Tree
GuiInspector::toggleDynamicGroupScript
GuiInspector::toggleGroupScript
GuiInspector::setAllGroupStateScript
GuiInspector::addDynamicField
InspectAddFieldDlg::doAction common/ui/LoadFileDlg.gui FillFileList
GetLoadFilename common/ui/SaveFileDlg.gui GetSaveFilename
DoSACallback
SA_directoryList::onSelect
SA_filelist::onSelect
Team LRN

Moving Right Along |
273 |
One last thing to remember about the common code: As chock-full of useful and important functionality as it is, you don't need to use it to create a game with Torque. You'd be nuts to throw it away, in my humble opinion. Nonetheless, you could create your own script code base from the bottom up. One thing I hope this chapter has shown you is that a huge pile of work has already been done for you. You just need to build on it.
Moving Right Along
In this chapter, we took a look at the capabilities available in the common code base so that you will gain familiarity with how Torque scripts generally work. For the most part, it is probably best to leave the common code alone. There may be times, however, when you will want to tweak or adjust something in the common code, or add your own set of features, and that's certainly reasonable. You will find that the features you want to reuse are best added to the common code.
As you saw, much of the critical server-side common code is related to issues that deal with loading mission files, datablocks, and other resources from the server to each client as it connects.
In a complementary fashion, the client-side common code accepts the resources being sent by the server, and uses them to prepare to display the new game environment to the user.
So, that's enough programming and code for a while. In the next few chapters, we get more artistic, dealing with visual things. In the next chapter, we will take a look at textures, how to make them and how to use them. We'll also learn a new tool we can use to create them.p
Team LRN
This page intentionally left blank
Team LRN
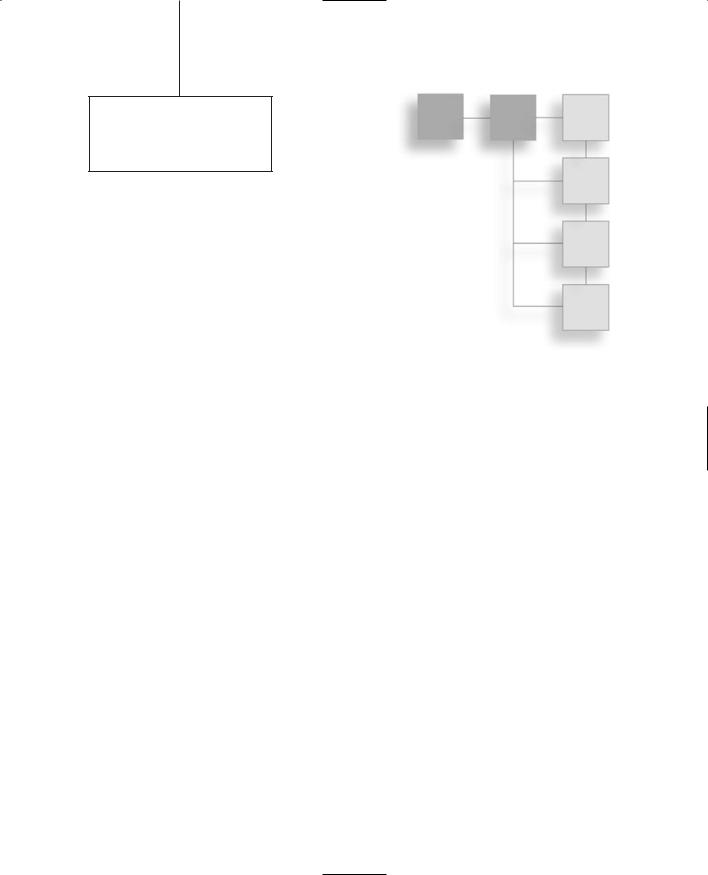
chapter 8
Introduction to
Textures
3D computer games are intensely visual. In this chapter we begin to explore the creative process behind the textures that give 3D objects their pizzazz.
Using Textures
Textures are probably the unsung heroes of 3D gaming. It is difficult to overstate the importance of textures. One of the most important uses of textures in a game is in creating and sustaining the ambience, or the look and feel of a game.
Textures also can be used to create apparent properties of objects, properties that the object shape doesn't have—it just looks like it does. For example, blocky shapes with jutting corners can appear to be smoothed by the careful application of an appropriate texture using a process called texture mapping.
Another way textures can be used is to create the illusion of substructure and detail. Figure 8.1 shows a castle with towers and walls that appear to be made of blocks of stone. The stone blocks are merely components of the textures applied to the tower and wall objects. There are no stone blocks actually modeled in that scene. The same goes for the appearance of the wooden boards in the steps and other structures. The texture gives not only the appearance of wood but the structure of individually nailed planks and boards. This is a powerful tool, using textures to define substructures and detail.
This ability to create the illusion of structure can be refined and used in other ways. Figure 8.2 shows a mountainside scene with bare granite rock and icefalls. Again, textures were created and applied with this appearance in mind. This technique greatly reduces the need to create 3D models for the myriad of tiny objects, nooks, and crannies you're going to
275
Team LRN

276 Chapter 8 ■ Introduction to Textures
Figure 8.1 Structure definition through using textures.
Figure 8.2 Rock and icefalls appearance on a mountainside.
Figure 8.3 Shoreline foam and deepwater textures.
encounter on an isolated and barren mountain crag.
Textures appear in many guises in a game. In Figure 8.3 two different textures are used to define the water near the shoreline. A foamy texture is used for the areas that splash against rock and sand, and a more wavelike texture is used for the deep water. In this application the water block is a dynamic object that has moving waves. It ebbs and flows and splashes against the shore. The water textures are distorted and twisted in real time to match the motion of the waves.
Another area in a game where textures are used to enhance the ambience of a game is when they are used to define the appearance of the sky. Figure 8.4 shows cloud textures being used in a skybox. The skybox is basically the inside of a big six-sided box that surrounds your scene. By applying specially distorted and treated textures to the skybox, we can create the appearance of an all-enveloping 360-degree sky above the horizon.
We can use textures to enhance the appearance of other objects in a scene. For example, in Figure 8.5 we see a number of coniferous trees on a hillside. By designing the ground texture that occupies the terrain location of the trees appropriately, we can achieve the forest look we want without needing to completely cover every inch of ground with the tree objects. This is helpful because the fewer objects we need to use for such a purpose—
Team LRN

basically decoration—the more objects that will be available for us to use in other ways.
One of the most amazing uses of textures is when defining technological items. Take the Tommy gun in Figure 8.6, for instance. There are only about a dozen objects in that model, and most of them are cubes, with a couple of cylinders tossed in, as well as two or three irregular shapes. Yet by using an appropriately designed texture, we can convey much greater detail. The weapon is easily identifiable as a Thompson Submachine Gun, circa 1944.
Following the theme of technological detail, Figure 8.7 is another example. This model of a Bell 47 Helicopter (think M*A*S*H) shows two trick uses of textures in one model. The engine detail and the instrument panel dials were created using textures we've already seen. Now take a look at the tail boom and the cockpit canopy. The tail boom looks like it is made of several dozen intersecting and overlapping metal bars; after all, you can see right through it to the buildings and ground in the background. But it is actually a single elongated and pinched box or cube with a single texture applied! The texture utilizes the alpha channel to convey the transparency information to the Torque renderer. Cool, huh? Then there is the canopy. It is semitransparent or mildly translucent. You can obviously see right through it, as you should when looking through Perspex, but you can
Using Textures |
277 |
Figure 8.4 Clouds in a skybox using textures.
Figure 8.5 Terrain accents.
Figure 8.6 Weapon detail using textures.
Team LRN