
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index
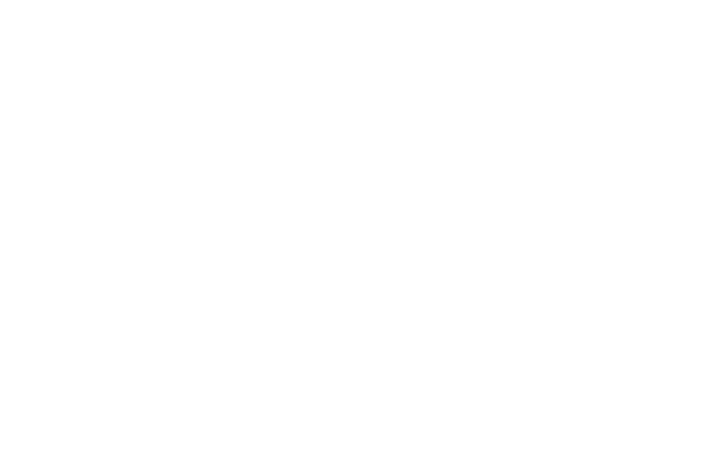
IMPLEMENTING THE BUSINESS LOGIC |
Chapter 21 |
495 |
|
|
|
|
|
sqlConnection1.Close();
foreach (DataRow myRow in dataSet11.Tables[“UserList”].Rows)
{
if (myRow[0].ToString().Trim().ToLower()==username.ToLower())
{
userexists=true;
}
}
if (userexists==false)
{
lblMessage.Text=”The user does not exist”; return;
}
sqlDataAdapter1.DeleteCommand.Parameters[0].Value=username; sqlConnection1.Open(); sqlDataAdapter1.DeleteCommand.ExecuteNonQuery(); sqlConnection1.Close();
lblMessage.Text=”User disabled successfully”; txtDelUserName.Text=””;
}
}
The ManageDatabases.aspx Form
The ManageDatabases.aspx form is used for moving data between the dtReser-
vations and dtDepartedFlights tables. It is also used to update the dtPassen-
gerDetails table for the frequent fliers program.
For updating the dtDepartedFlights and dtPassengerDetails tables,I have created stored procedures in SQL Server. These procedures are called from the SkyShark Airlines application so that the data can be updated directly at the back end. There are several advantages of using a stored procedure in this scenario:
Since the data is not required in the application, it does not need to be retrieved from the application and then posted back again.This saves a lot of unnecessary network congestion and improves the performance of the application and the database.
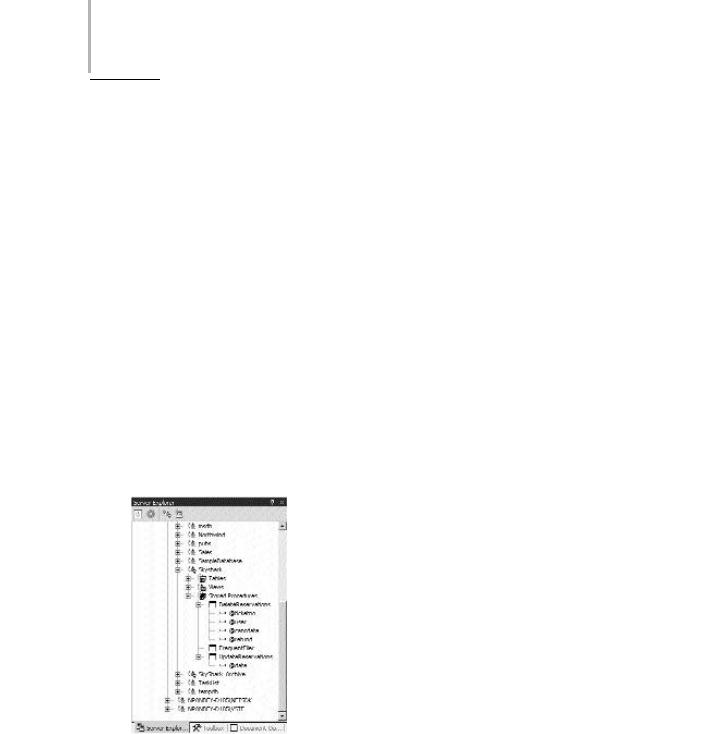
496 Project 4 CREATING AN AIRLINE RESERVATION PORTAL
The developer does not need to write unnecessary code for the application. SQL queries that are used in stored procedures can be easily tested by using Query Analyzer.
To move data from the dtReservations table to the dtDepartedFlights table, you need to write the following stored procedure:
CREATE PROCEDURE UpdateReservations
@date datetime
AS
INSERT INTO dtDepartedFlights
SELECT * from dtReservations
WHERE (DateOfJourney < @date) AND (TicketConfirmed=1)
DELETE from dtReservations
WHERE (DateOfJourney < @date)
GO
To execute a stored procedure, you need to associate it with an SqlCommand object. Stored procedures can be associated with SqlCommand objects in the same way as you associate SQL Server tables with your application. The stored procedures in the SkyShark Airlines database are shown in Figure 21-2.
FIGURE 21-2 Stored procedures can be accessed from Server Explorer
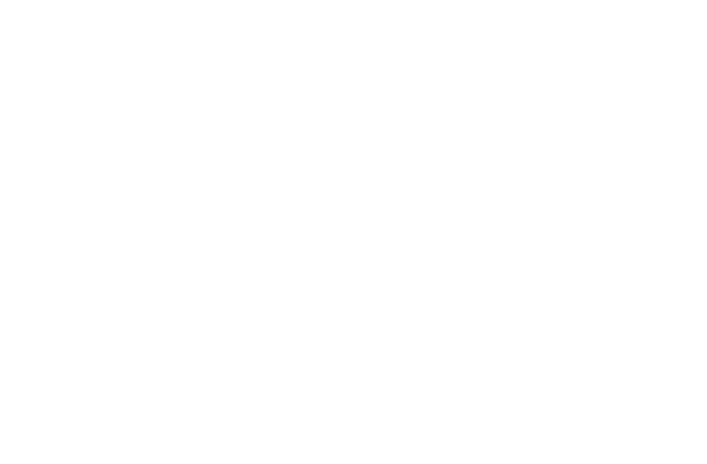
IMPLEMENTING THE BUSINESS LOGIC |
Chapter 21 |
497 |
|
|
|
|
|
To write the code for executing the UpdateReservations stored procedure from the SkyShark Airlines application,drag the Update Reservations stored procedure form Ser ver Explorer to the design view of the form. Visual Studio .NET automatically creates the sqlDataAdapter1 and sqlCommand1 controls. To run the stored procedure when a user clicks on the Archive button, write the following code for the Click event of the Archive button:
private void BtnArchive_Click(object sender, System.EventArgs e)
{
lblMessage.Text=””; sqlConnection1.Open();
sqlCommand1.Parameters[1].Value=DateTime.Today.Date.ToShortDateString(); sqlCommand1.ExecuteNonQuery();
sqlConnection1.Close(); lblMessage.Text=”Done.”;
}
To move data between the dtDepartedFlights and dtPassengerDetails tables, I
have created the FrequentFlier stored procedure.The definition of this procedure is given as follows:
CREATE PROCEDURE FrequentFlier
AS
DELETE dtFrequentFliers
INSERT INTO dtPassengerDetails
SELECT EMail, Sum(Fare), Count(EMail) from dtDepartedFlights where EMAIL!=’NotSpecified’ group by EMail
GO
To run this procedure, specify the following code in the Click event of the Update button:
private void btnUpdate_Click(object sender, System.EventArgs e)
{
lblMessage.Text=””; sqlConnection1.Open(); sqlCommand2.ExecuteNonQuery(); sqlConnection1.Close(); lblMessage.Text=”Done.”;
}
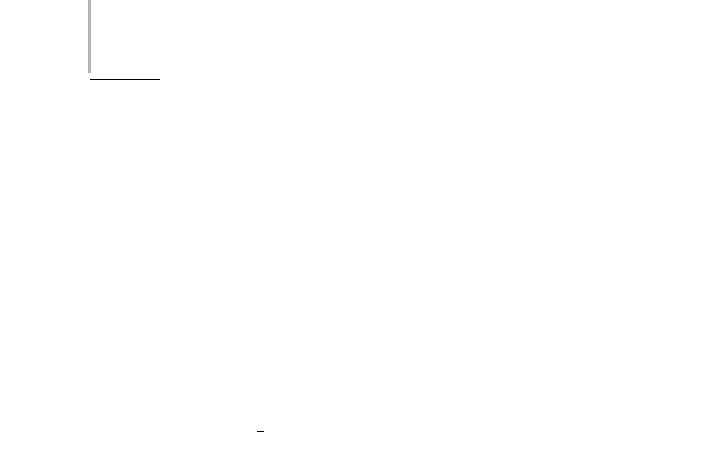
498 Project 4 CREATING AN AIRLINE RESERVATION PORTAL
The ChangePassword.aspx Form
The ChangePassword.aspx form is included in the folders for network administrators, business managers, and LOB (line-of-business) executives. However, I will discuss the coding and functionality of this form in this section only. The functionality remains same across the forms for all the roles.
To add functionality to the ChangePassword.aspx page, drag the dtUsers table from Server Explorer to Component Designer. In the resulting sqlDataAdapter1 control that is added to the form, change the UpdateCommand property as mentioned here:
|
|
Y |
UPDATE dtUsers SET Password = @Password, PasswordChanged = ‘1’ WHERE (Username = |
||
@Original_Username) |
L |
|
|
F |
|
After specifying the preceding query, double-click on the Submit button to code |
||
|
M |
|
|
A |
|
|
E |
|
the functionality for its Click event. Write the following code for the Click event
private void btnSubmitTClick(object sender, System.EventArgs e)
{
sqlConnection1.Open(); sqlDataAdapter1.UpdateCommand.Parameters[0].Value=txtPassword.Text.Trim(); sqlDataAdapter1.UpdateCommand.Parameters[1].Value=Session[“usrName”]; sqlDataAdapter1.UpdateCommand.ExecuteNonQuery();
sqlConnection1.Close(); Response.Redirect(“ManageUsers.aspx”);
}
The preceding code accepts the new password specified by the user as the first parameter and the username, which is retrieved from the Session state variables, as the second parameter to update the password of the user in the dtUsers table.
Restricting Access to Web Forms
One aspect that is common across all Web pages of the application is that the users should be able to access Web forms pertaining to a role only if they are in that role. For example, the ManageUsers.aspx form should be accessible to network administrators only.
Team-Fly®
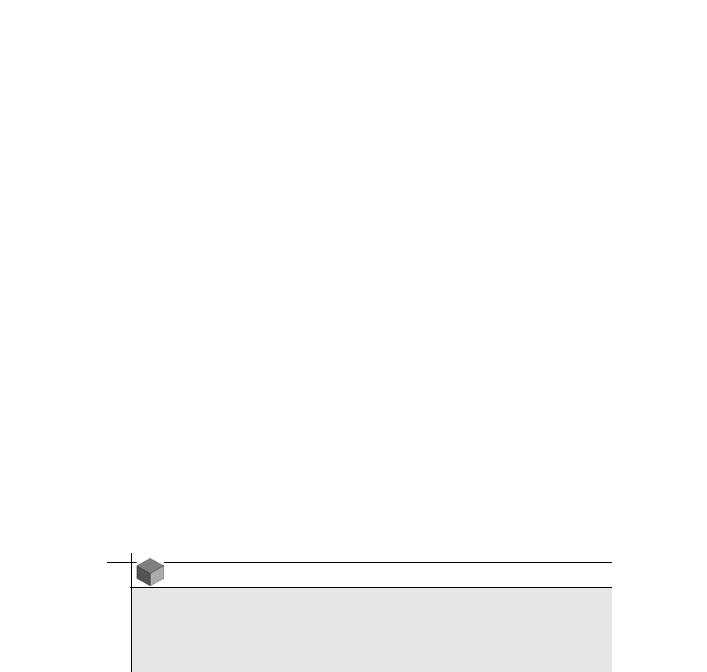
IMPLEMENTING THE BUSINESS LOGIC |
Chapter 21 |
499 |
|
|
|
|
|
The SkyShark Airlines application enforces this constraint by using Session variables. The role of the user is queried from these variables in the Load event of all forms. When the role of the user matches with the intended audience of the form, the user is allowed to load the Web form. If the user should not be allowed to access the page, the user is redirected to the default.aspx page.The code that controls access to Web pages in the Load event of forms for network administrators is given as follows:
private void Page_Load(object sender, System.EventArgs e)
{
if (Session[“usrRole”]==null)
{
Response.Redirect(“..\\default.aspx”);
}
if (!(Session[“usrRole”].ToString()==”Admin”))
{
Response.Redirect(“..\\default.aspx”);
}
else
{
txtUser.Text=”Changing password for “+ Session[“usrName”].ToString();
}
}
NOTE
You need to add the code given here in the Load event of all forms. The only precaution you need to take is that you should change the value to check in the if clause ((!(Session[“usrRole”].ToString()==”Admin”))) to “BA” for business managers and “LOB” for LOB executives.