
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf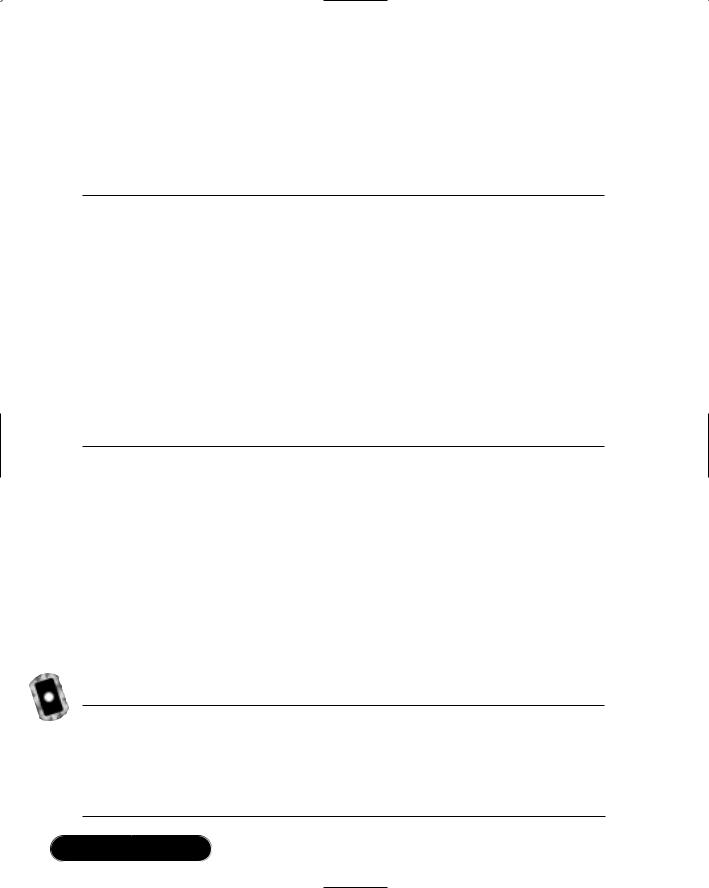
290 Chapter 6 • Optimizing Caching Methods
CacheItemRemovedCallback delegate returns three parameters that must be accepted by your event handler: the cached item’s key, the value of the cached item when it was removed, and the reason for removal.There are four valid reasons for an object to be removed from the cache, and they are outlined in Table 6.7.
Table 6.7 CacheItemRemovedReason Values
Reason |
Definition |
|
|
DependencyChanged |
When the files, directories, or keys specified in the |
|
dependency option are changed, this reason is |
|
reported upon the cached object’s removal. |
Expired |
If an expiration policy is set for a cached object, this |
|
reason is reported when the absolute expiration time |
|
has been met. |
Removed |
If a cached object is explicitly removed or replaced |
|
due to the usage of the same key, this reason is |
|
reported. |
Underused |
If ASP.NET has removed the cached object due to a |
|
lack of system resources or because the data is under- |
|
utilized, the Underused reason is reported. |
I have illustrated the use of all the caching options available for the cache.add and cache.insert methods in the code shown in Figure 6.14. In this code, we first check to see if there is any data stored in the cache under the key “MyKey.” If data is there, then note that the data was retrieved from cache, remove the data from the cache, and display the data. If no data is in the cache, then your array is loaded into the cache.When you do remove the cached data, you fire an event that adds the fact that the data was removed from cache as well as the reason code to the array, and then recache the result.When you recache it the second time, you do not add the CacheItemRemoved option; otherwise you would end up in a loop.This code is located on the CD that accompanies this book as data_cache3.aspx.
Figure 6.14 Data Caching Full Sample Code (data_cache3.aspx)
<SCRIPT language="VB" runat=server>
Private Shared OnRemove as CacheItemRemovedCallback = Nothing
Public Sub RemovedCallback(key as string, value as object, _
Continued
www.syngress.com
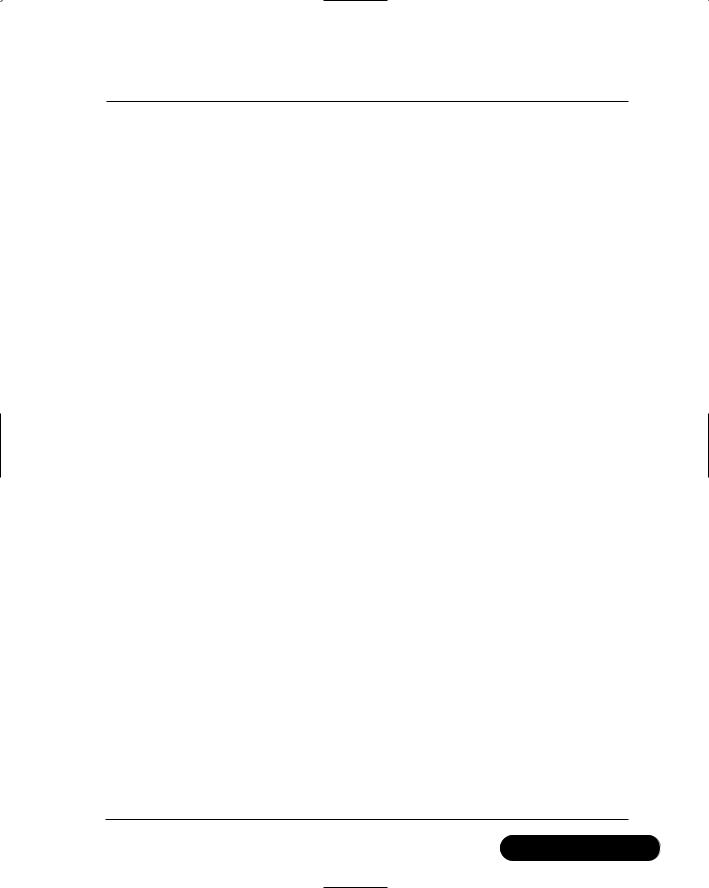
Optimizing Caching Methods • Chapter 6 |
291 |
Figure 6.14 Continued
reason as CacheItemRemovedReason)
'At this point, place any code needing to be executed upon 'an item's removal. As an example, we will now recache the 'object after making a change.
value=value & " *recached due to reason code " & reason & "*<br>" cache.insert(key, value, nothing, datetime.maxvalue, _
timespan.fromseconds(10)) End Sub 'RemovedCallback
Sub Page_Load(source As Object, e As EventArgs) onRemove = New CacheItemRemovedCallback(AddressOf _
Me.RemovedCallback)
dim MyValue as string MyValue=cache("MyKey")
if MyValue <> nothing then
output.text=MyValue & "<P>This data retrieved from cache" 'Now we'll remove the item from cache, just to trigger the
event'
Cache.Remove("MyKey") else
Dim StringArray() As string ={"Amy", "Bob", "Chris", "Damien",
_
"Eli", "Franklin", "Gerald"}
dim DependentString() as string ={server.mappath("test.txt"), _ server.mappath("test2.txt")}
dim MyString as string
for each MyString in StringArray output.Text=output.Text & MyString & "<br>"
next
cache.insert("MyKey", output.text, nothing, datetime.maxvalue, _ timespan.fromseconds(10), CacheItemPriority.Low, _
Continued
www.syngress.com
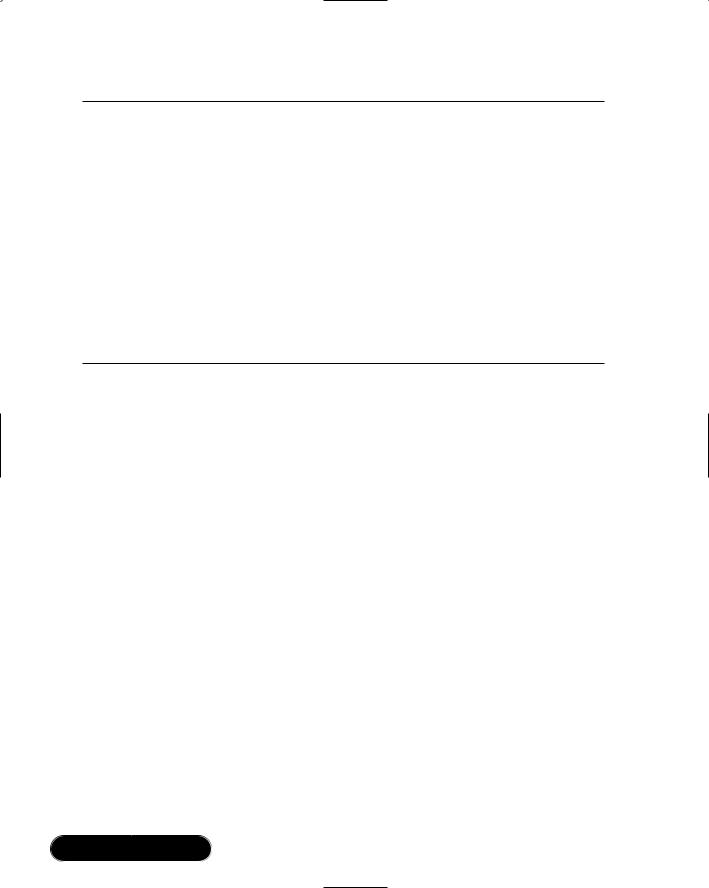
292 Chapter 6 • Optimizing Caching Methods
Figure 6.14 Continued
CacheItemPriorityDecay.Fast, onRemove)
end if End Sub </SCRIPT>
<HTML>
<HEAD>
</HEAD>
<BODY>
<P><asp:label id="output" runat="server"/> </BODY>
</HTML>Using the Cache.Remove Method
Removing data manually from the cache is a useful method of clearing up resources or giving your end user the option of verifying that the data they are viewing is up to date.The way this is done is similar to the simple dictionary cache interface. I have used the cache.remove method in Figure 6.14, which illustrates its syntax:
cache.remove("MyKey")
Advantages of Using Data Caching
We have gone over the plethora of different options available for data caching, and I believe that its many advantages are apparent.With the ability of caching data at the object level, you are given the most granular control over ASP.NET’s caching features possible. By using the dictionary interface, you have a simple manner of adding and removing data from the cache. If, however, a greater amount of control is necessary, ASP.NET provides for this with the expiration features and priority levels.
By using all of these features in the best manner possible, you can increase the overall speed of your application without the requirements of using a separate user control or a semi-static page.While data caching does take up more memory per saved object than object or fragment caching, its primary strength is that you
www.syngress.com
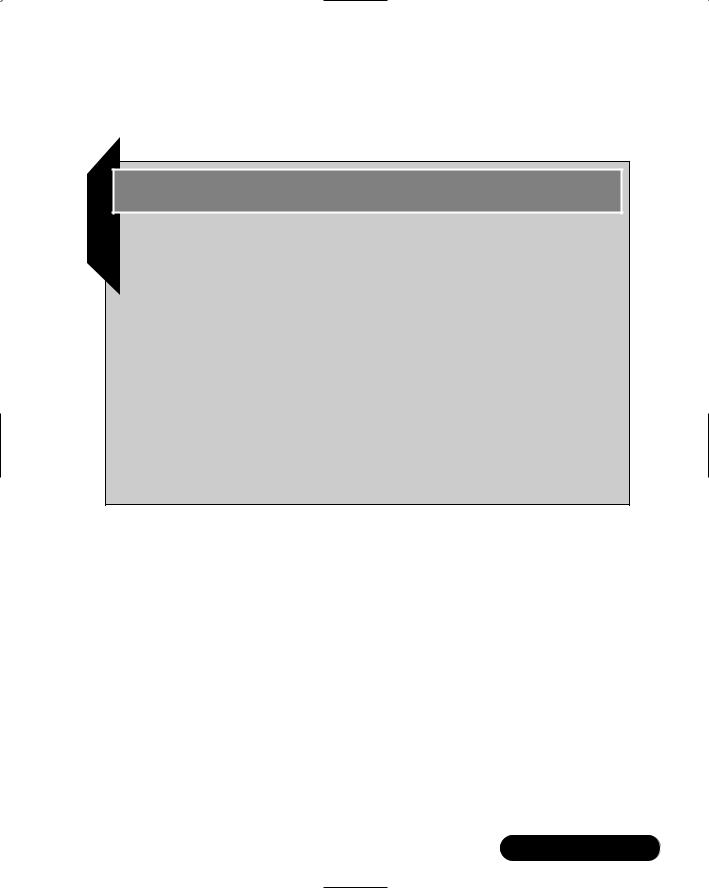
Optimizing Caching Methods • Chapter 6 |
293 |
can store smaller amounts of data in the cache, thus opening the possibility of saving memory resources overall.
Debugging…
Implementing Caching
When building your Web application, it is best to not implement caching on the initial build. There are several reasons for this, the primary being ease of debugging. You will find that it is much easier to get an application completely debugged without having to deal with the question of cached pages and data. When you have the application debugged, begin adding in the caching features of ASP.NET where they are most effective. You will see an immediate performance increase in your application, as well as make your debugging process much faster by following this process.
A second advantage of this method is that you have an opportunity to set a baseline for your application. As memory resources on the server become scarce, cached data is dumped in order to conserve resources. With this baseline performance data, you can provide a worst-case scenario for your application.
Best Uses for Caching
When should you use caching? As often as possible. In order to make your application viable in today’s fast-paced world, you have to make your data available as quickly as possible and decrease the wait-time for your end user as much as possible. By using the various types of caching in the best manner possible, you can change your application from slow yet effective to fast and amazing.When users access an application created using ASP.NET’s caching features for the first time, the first thing they will notice is the speed increase. In the overall user experience, this is one of the most important impressions that you can make. In the following section, I’ve listed several of the best uses for the different methods of caching.
www.syngress.com

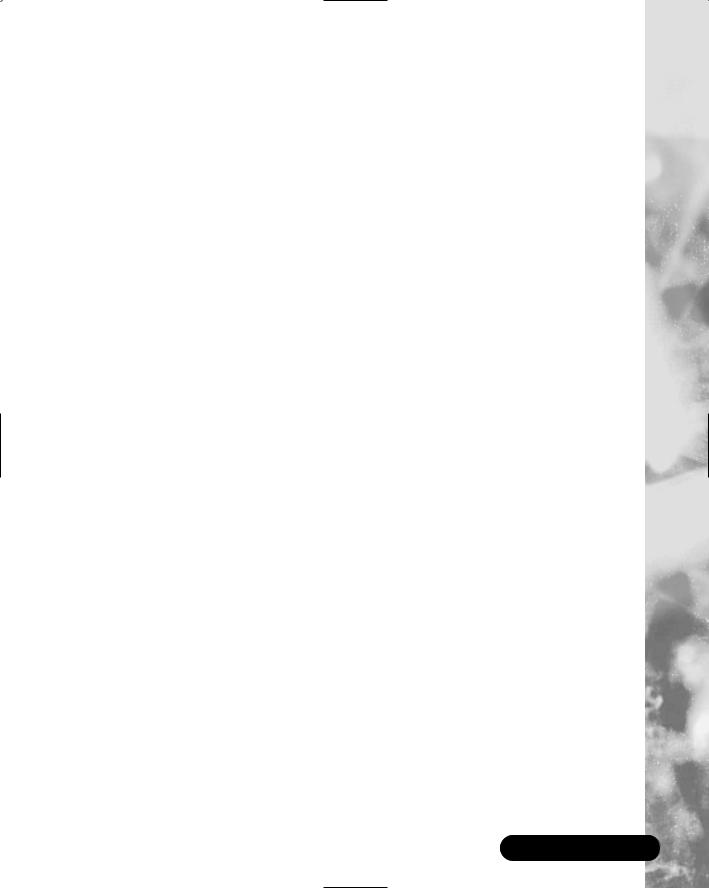
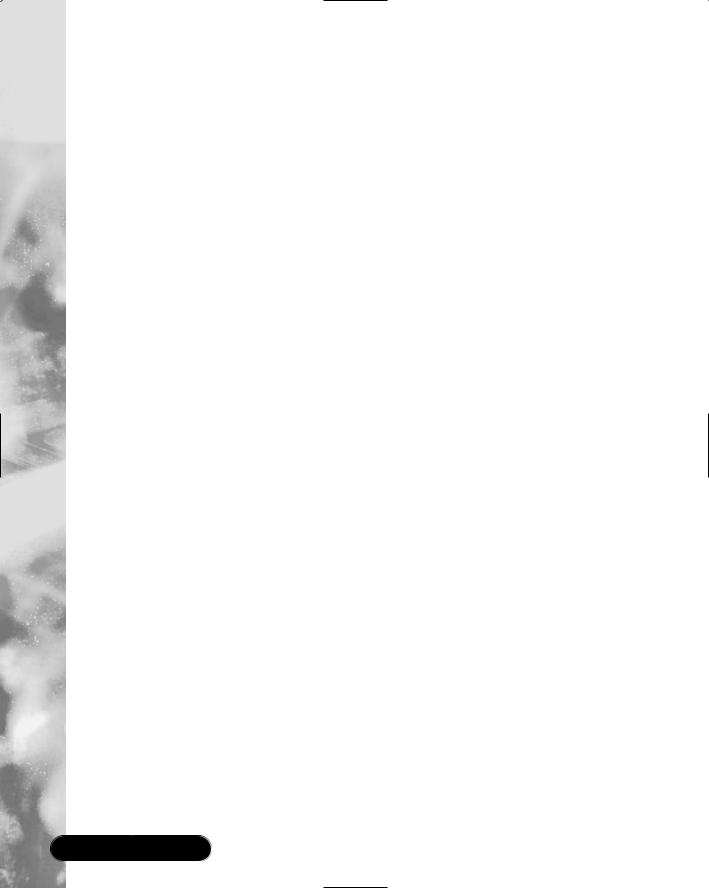

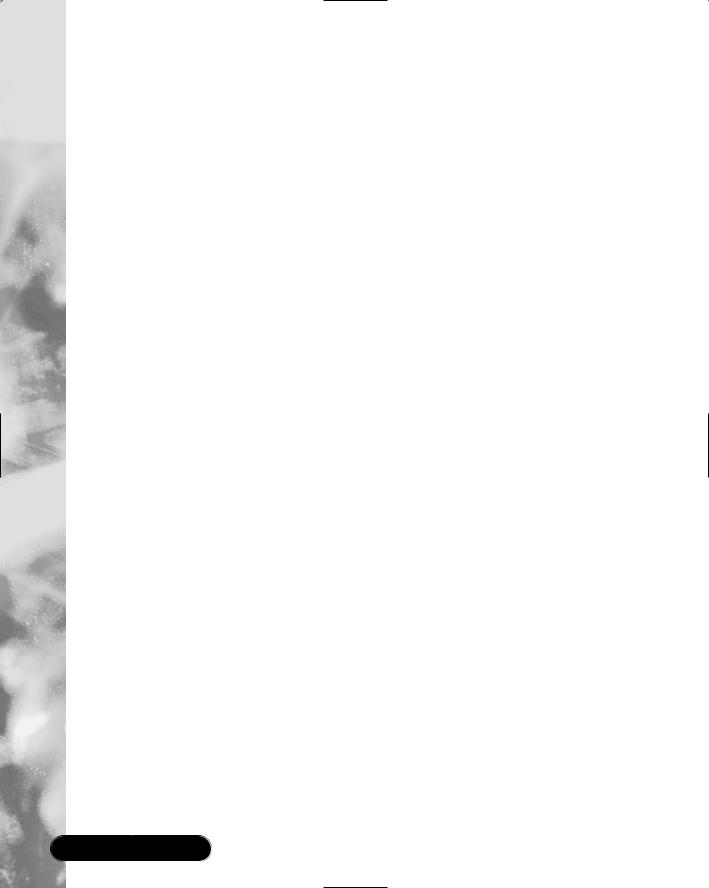
