
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf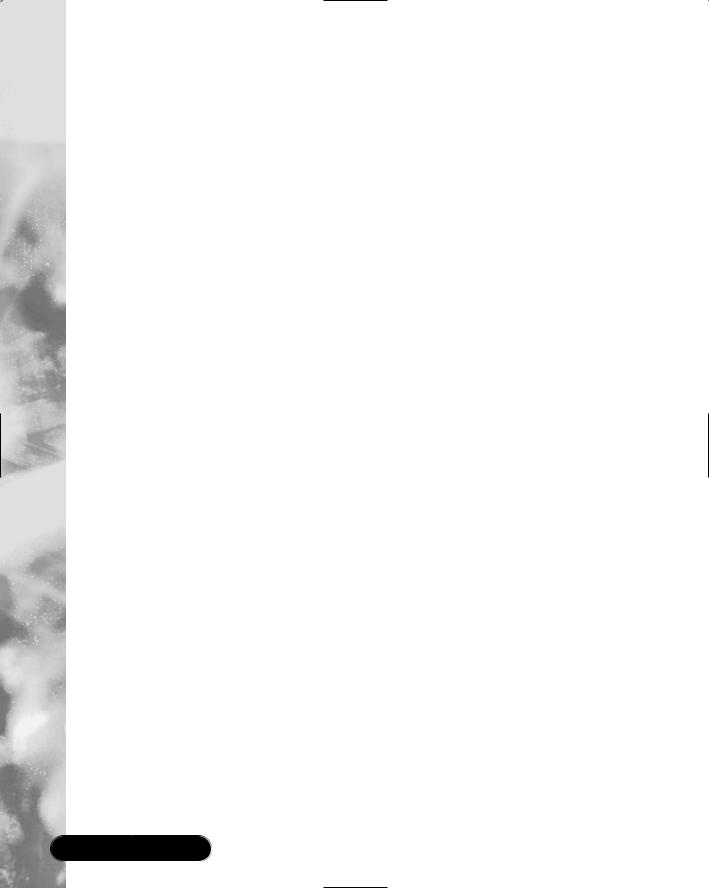
260Chapter 5 • An ASP.NET Application
;ASP.NET state management is improved over previous versions by not solely relying on cookies.
;Data can now be persisted using a database, and it offers more flexibility in load balancing situations.
Analyzing Global.asax
;Each application has its own Global.asax.
;Global.asax sets the event code and values for an application using script blocks and directives.
;When executed, Global.asax is a .NET component with events, values, and inheritance abilities.
;What is set in the Global.asax affects the whole of the application and scripts contained within it.
;To use application, session, and cache values, you do not need to write your own Global.asax, but you must if you wish to use static variables.
Understanding Application State
;The whole of the application code and all application users currently active can access application variables.
;ASP.NET introduces two new facilities to extend the application functionality, the Cache object and static variables.
;Cache values enable the programmer to preload content and data, with automatic expiry and dependencies.
;Static variables are the Global.asax application class member variables and are accessible to the whole application; and as they are not object collections, they do not have the overhead of application variables and so they have, in many cases, higher performance.
Using Application Events
;Application event code is written into the Global.asax as subroutines.
;Most commonly used events are those that deal with the application starting and ending, errors, or processing a request.
www.syngress.com
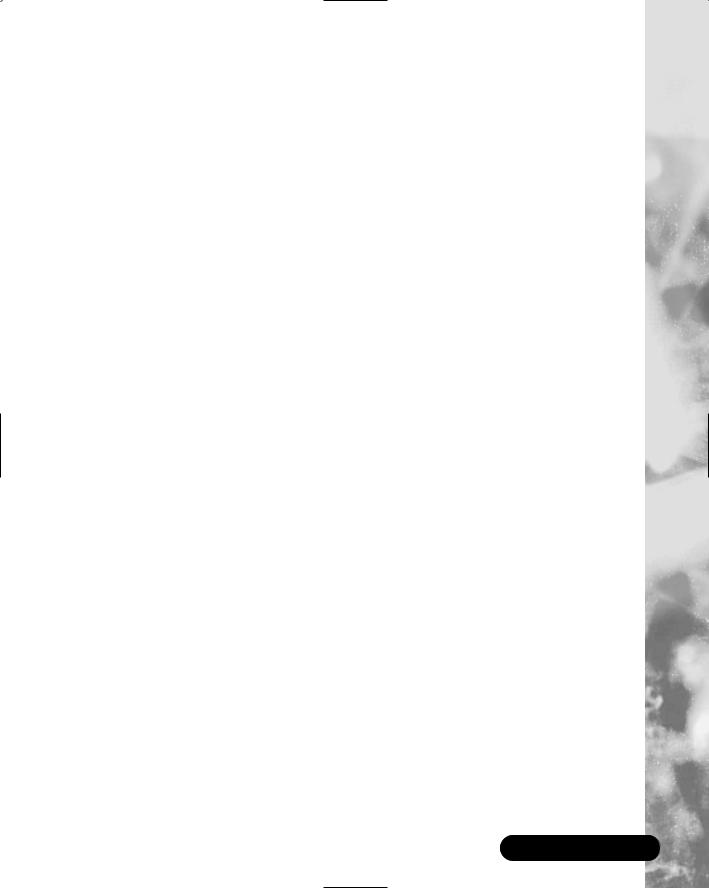
An ASP.NET Application • Chapter 5 |
261 |
; Other events deal with authentication and cache issues.
Understanding Session State
;Session state deals with the information relating to one user for the duration of his or her active session, and any script within the application the user visits has access to all of the user’s values.
;ASP.NET improves on previous versions of ASP session state solutions by allowing centralized state storage and on the ability to allow state management for users without cookies.
;A server will usually expire a session after 20 minutes of inactivity, but this can be set to a different value by the programmer.
Configuring Sessions
;Sessions are configured using web.config.
;Cookies are now no longer a requirement, and sessions can be set to be “cookieless.”
;Using the timeout attribute, you can reduce or increase the session timeout value from the default.
;Pages and applications can turn off session state completely by setting the session-state mode to “off.”
Using Session Events
;Session events deal with the processing of one user’s session.
;Using session_OnStart and session_OnEnd, you can add code to run when the user enters and leaves a site.
;The session may end when the user closes his or her browser or the session has timed out.
www.syngress.com
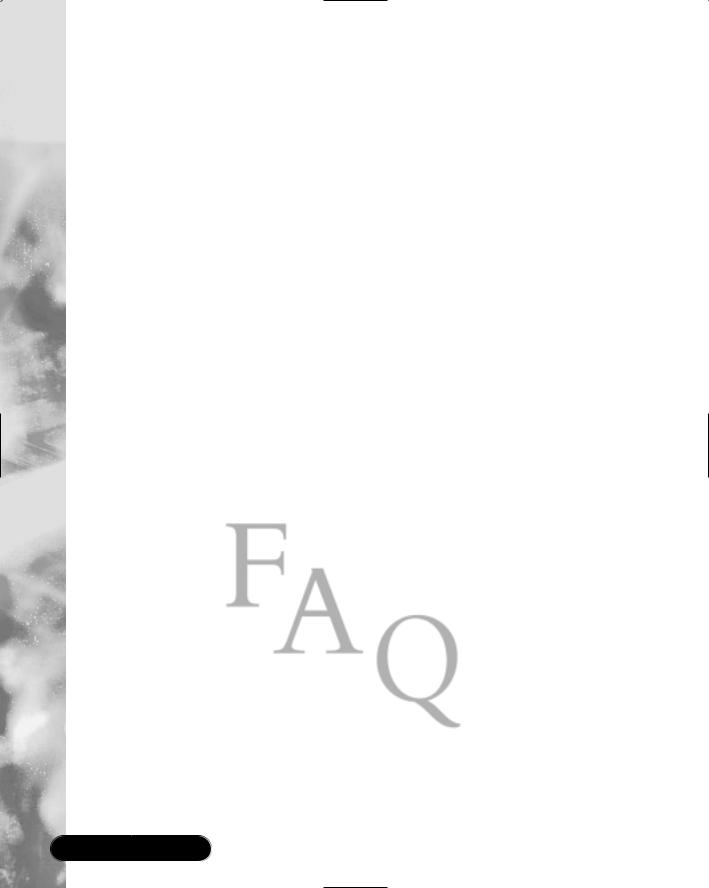
262 Chapter 5 • An ASP.NET Application
Comparing Application and Session States
;Application state is the management of information relating to the whole application and all of the currently live users.
;Session state relates to each user separately.
;All users and scripts have access to the same application values, whereas one user can only access his or her own session information and can not see another’s session variables.
Frequently Asked Questions
The following Frequently Asked Questions, answered by the authors of this book, are designed to both measure your understanding of the concepts presented in this chapter and to assist you with real-life implementation of these concepts. To have your questions about this chapter answered by the author, browse to www.syngress.com/solutions and click on the “Ask the Author” form.
Q: Must I have a Global.asax?
A:A Global.asax is not required; only use one if you need to.You will not need a Global.asax unless you want to use events or static variables, for example. Application, session, and cache values do not depend on your writing a Global.asax.
Q: Are there any security risks associated with session and application variables?
A:As the state information is stored in storage (server memory or a database) that the user has no direct access to, providing databases are secured, there is no direct security risk. Having said that, ensure that application variables do not contain sensitive information, as they are accessible to the whole application and to all users within it.
Q: Should I use application, cache, or static variables?
A:Use whichever is appropriate for your situation; if in doubt, use application variables. For simple values where the names are known beforehand and do not change, you may find static variables give you cleaner code and faster processing. Cached values are excellent for situations where users will frequently need to read the same data or where the application occasionally
www.syngress.com

An ASP.NET Application • Chapter 5 |
263 |
needs to refresh this data while the application is running. Application variables are probably best in cases where users might need to both read and write values to the variables often, and the variable names are not necessarily known beforehand.
Q: Can I use application state in a Web farm?
A:Yes, but your application data will only be visible to the process in which it is running. If you want this data to be shared, then you should store it in an external store instead, such as a database. For this reason, application state should not be used in a Web farm or a load-balanced environment when critical values are required.
Q: Can I use session state in a Web farm?
A: Yes, but carefully consider all the implications. Storing session state either puts a load on your servers, or network, or both.
www.syngress.com


Chapter 6
Optimizing Caching
Methods
Solutions in this chapter:
■Caching Overview
■Output Caching
■Fragment Caching
■Data Caching
■Best Uses for Caching
;Summary
;Solutions Fast Track
;Frequently Asked Questions
265

266 Chapter 6 • Optimizing Caching Methods
Introduction
Data caching was introduced with Internet Information Server (IIS) in order to optimize the transfer of Web pages and speed up the user’s access to these pages. ASP 2.x did not have any native caching ability and simply made use of the caching provided by IIS.Third-party utilities could also be used to increase the caching abilities of ASP 2.x and provide a greater level of control over the IIS cache.
Caching is now available natively within ASP.NET and has three new faces: output, data, and fragment caching. Each of these methods provides new and powerful methods of optimizing the utilization of system resources and increasing application performance.
Output caching is more like the old method of caching provided by IIS; a single page is stored within memory for a small period of time, for any reason that the programmer sees fit.While this model is troublesome in some instances, it can be helpful to the end-user at times.This allows for faster access to pages that contain some dynamic content without having to regenerate the entire page.
Fragment caching is an innovation to output caching; it enables the programmer to determine which parts of a page should be cached for future reference.This is done by breaking the code into separate user controls and then caching the control.This new feature greatly expands on our caching options.
Data caching enables the programmer to have full control over the caching at the object level.You can define which objects and which areas are to be cached and for what length of time, as you see fit.This detailed level of control enables you to save any object to memory that you wish, in order to speed up access to that object.
In this chapter, we are going to go over the three methods of caching that are available in ASP.NET.We will also discuss how and why to use each method and in what situations each method should be used.The options and parameters for each method will be discussed and illustrated. By using this information, you can greatly increase the performance of your application.This objective is key in creating an application that fits well with the needs of your users.
Caching Overview
Caching is a technique used in various aspects of computing to increase performance by decreasing access times for obtaining data.This is accomplished by retaining frequently accessed data within memory.This technique is used by
www.syngress.com
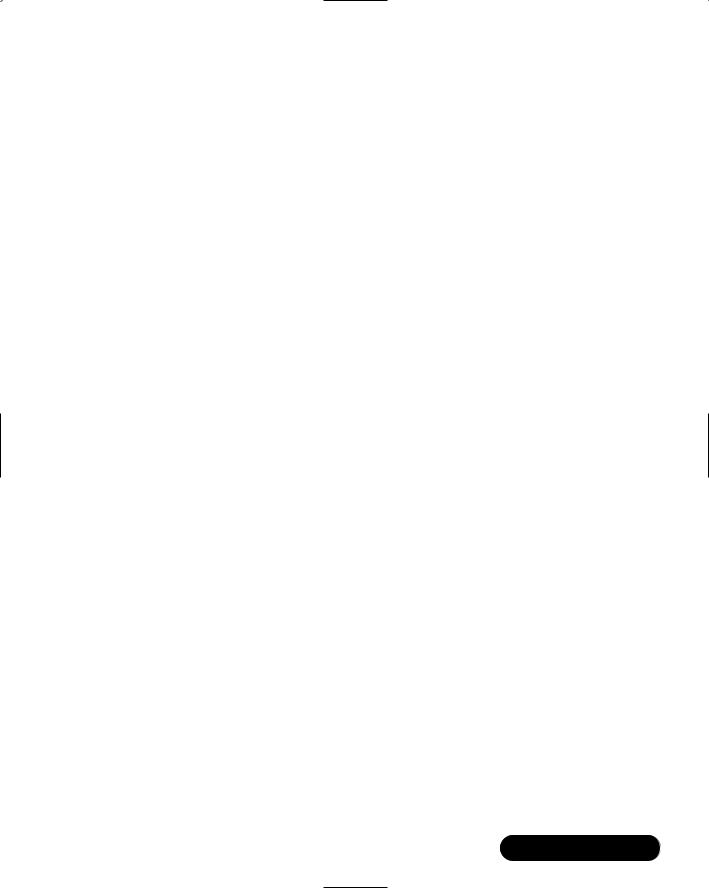
Optimizing Caching Methods • Chapter 6 |
267 |
many operating systems to cut down on the number of times that a hard drive must be accessed or a network connection utilized, by storing the needed data in the system’s memory. It is also used by some databases to store data retrieved from queries that may be needed again later. As it pertains to a Web application, data is retained from across multiple HTTP requests, and can then be reused without incurring additional access times that would normally be necessary to recreate the data.
ASP.NET makes available three different types of caching, which, when used properly, can greatly increase the overall performance of your application.These types are as follows:
■Output Caching
■Fragment Caching
■Data Caching
We will go into detail in this chapter on each of these caching types, but they all are based off of the basic concept of saving all or a portion of the data generated by your application, with the purpose of presenting the same data again at a later time.
Output caching basically caches the entire content of an output Web page. This can be very useful when the content of your pages changes very little. Programmers familiar with ASP 2.x should be familiar with this concept, as it was the only available caching method for ASP.This method provides the greatest performance increase, but can only be used when nothing on the output page is expected to change within the valid timeframe of the cache.
Fragment caching, which is new in ASP.NET, allows for the caching of portions of your output page.This is an excellent improvement in caching technique, and is best used when your application’s output page has content that changes constantly in addition to content that changes very little.While this method does not provide as much of a performance increase as output caching, it does increase performance for applications that would formerly have been unable to use any caching at all due to the strict requirements of output caching.
Data caching, also new in ASP.NET, provides the ability to cache individual objects. Placing objects into the cache in this manner is similar to adding items to a dictionary. By using a simple dictionary-style interface, this method makes for an easy-to-use temporary data storage area while conserving server resources by releasing memory as cached objects expire.
www.syngress.com
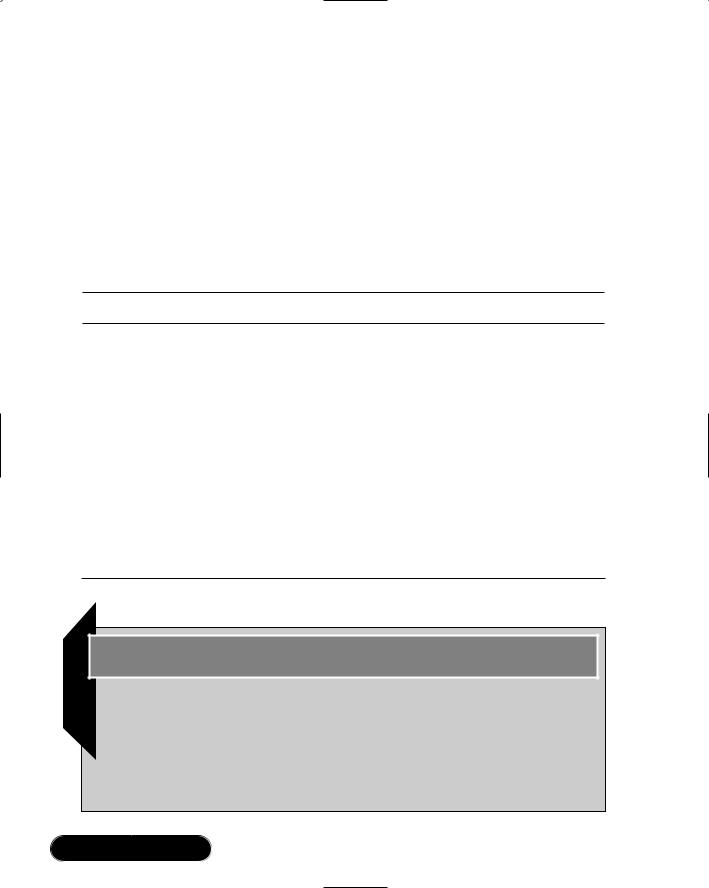
268 Chapter 6 • Optimizing Caching Methods
A major consideration in planning your caching strategy is the appropriate utilization of server resources.There is a trade-off when it comes to the use of any kind of caching, in that for every item cached, less memory is available for other uses.While output caching provides the greatest performance increase, it also utilizes more memory than caching a few objects using data caching. On the other hand, due to the overhead required to store multiple objects by using data caching, it may be more logical to cache a portion of the output page by using fragment caching. Suggested uses are listed in Table 6.1; however, the best caching method for your specific application is dependant upon your output.
Table 6.1 Suggested Uses of Caching Types
Situation |
Suggested Caching Type |
The generated page generally stays the same, but there are several tables shown within the output that change regularly.
The generated page constantly changes, but there are a few objects that don’t change very often.
The generated page changes every few hours as information is loaded into a database through automated processes.
Use Fragment Caching in this situation. Cache the portions of the page that remain somewhat static, while dynamically generating the table contents. Also consider using Data Caching for storing some individual objects.
Use Data Caching for the objects.
Use Output Caching and set the duration to match the frequency of the data changes.
Developing & Deploying…
Optimizing Cache versus
Optimizing Server Resources
When it comes to optimizing your Web application’s performance, there are two factors that you must keep in mind. The first is the caching method(s) that you choose, and the second is server resources. It is not possible to implement a good performance plan without keeping both
Continued
www.syngress.com
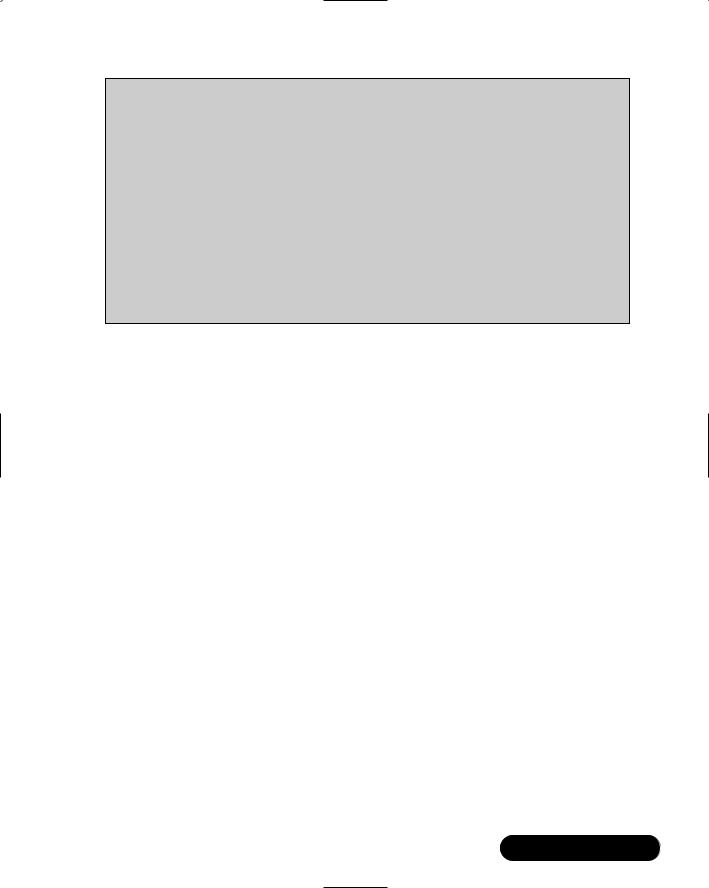
Optimizing Caching Methods • Chapter 6 |
269 |
factors in mind. The choice of caching method will depend on the output of your page more than any other factor. For example, it doesn’t matter if you have enough server resources to implement Output Caching, if your output changes constantly.
When data is cached on the server, additional memory resources are used for storage of the cached data. The cached data includes not only the page output or the objects that you cache, but also header information necessary to obtain the correct cached data again later. As you cache more data, less memory is available for other uses. You may need to add more memory to your server to compensate for this in order to provide the highest performance increase. By testing your Web application extensively, you will be able to find the right mix of caching and hardware to provide the best performance at the right price.
Output Caching
Output caching provides the capability to cache response content generated from dynamic pages for the purpose of increasing application performance.This form of caching should be applied when the content of your page is somewhat static. Various options can be set for output caching including the duration.
In order for a page to be cached using output caching, it must have a valid expiration or validation policy.These options can be set either through the
@ OutputCache directive or through the HttpCachePolicy class.
Using the @ OutputCache Directive
When the @ OutputCache directive is used at the top of the page, ASP.NET basically uses the Page.InitOutputCache method to translate the directive parameters into HttpCachePolicy class methods.These methods and properties can also be accessed through the HttpResponse.Cache property, which will be discussed later in this section.To set the expiration of a page you intend to cache, you can use the following code at the top of the page:
<% @ OutputCache Duration="60" VaryByParam="None" %>
This sets the cache duration for this page to 60 seconds as well as setting the VaryByParam attribute to not provide additional functions.The VaryByParam attribute is required when using the @ OutputCache directive.The VaryByParam attribute is one of three attributes used to control caching of multiple pages by the @ OutputCache directive.These attributes are as follows:
www.syngress.com