
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf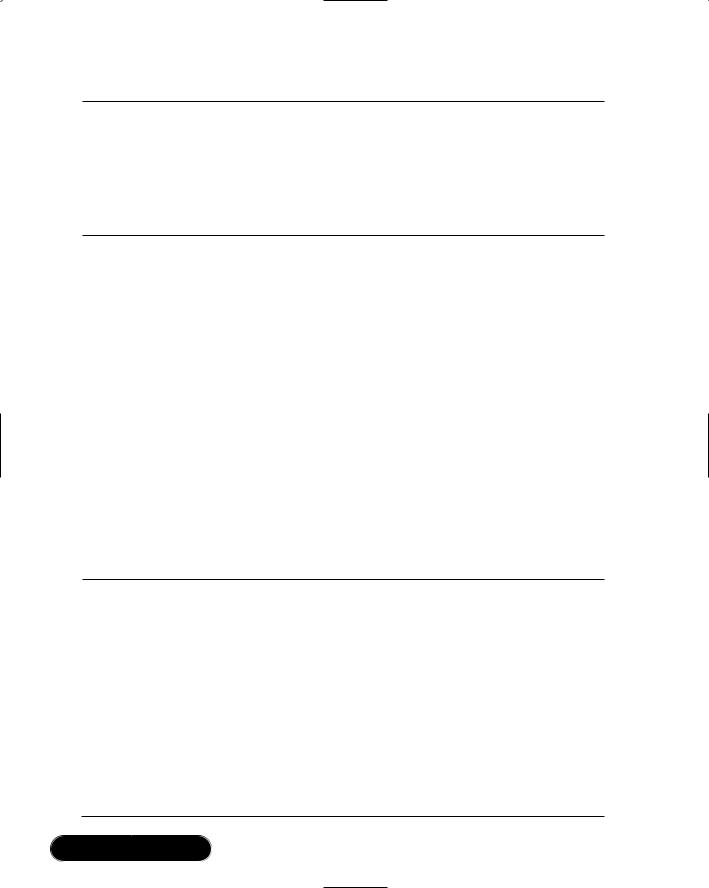
250 Chapter 5 • An ASP.NET Application
Figure 5.11 Continued
// increment application counter
cntApplication++;
}
</script>
Compare this code to the previous Global.asax. Isn’t it much cleaner? The best thing is that it performs faster in many cases, too!
To use our static variable, “cntApplication,” we must first name our class.We do this with the very first line.We have called this class “Chapter5” in honor of this very chapter you are reading.
Once the class is named, we then declare our static variable. For the variable to be used outside the class, we must make it public. Static means that it retains its value from one access to another, and we have declared this variable as an integer number.
All that remains is to increment the value whenever a new session starts, using the C-like shorthand “++” increment operation.Whereas in the other examples, when we first locked the application before doing our increment and then unlocked the application, here you will see that we do not need to worry about locking at all, as the application class does this for us. Figure 5.12 shows the example C# static variable page.
Figure 5.12 C# Static Variable Page
<%@page language="c#" %>
<html>
<head>
<title>Chapter 5</title> </head>
<body bgcolor="white">
<%
Continued
www.syngress.com
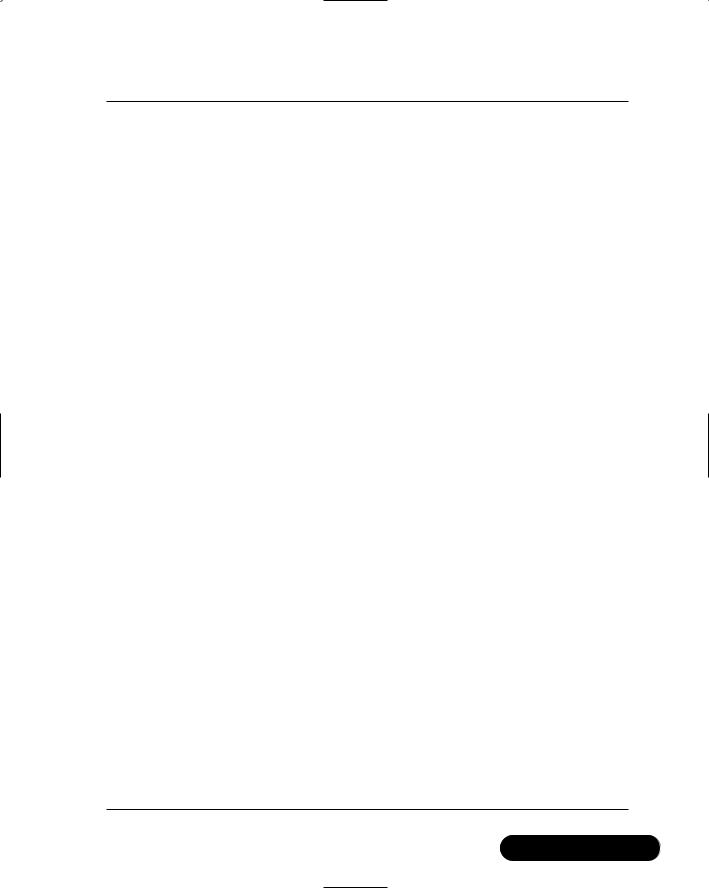
An ASP.NET Application • Chapter 5 |
251 |
Figure 5.12 Continued
//lock the application to prevent clashes Application.Lock();
//increment application counter if(Application[Page.ToString()]==null) Application[Page.ToString()]=0; Application[Page.ToString()]=((int) Application[Page.ToString()])+1;
//unlock application
Application.UnLock();
// increment session counter
if(Session[Page.ToString()] == null) Session[Page.ToString()] = 0; Session[Page.ToString()] = ((int) Session[Page.ToString()]) + 1;
%>
<p>
The application has been visited <b><%=Chapter5.cntApplication%></b> times since the application started<br>
This page has been visited <b><%=Application[Page.ToString()]%></b> times since the application started<br>
You have visited this page <b><%=Session[Page.ToString()]%></b> times in this session<br>
</body>
</html>
www.syngress.com
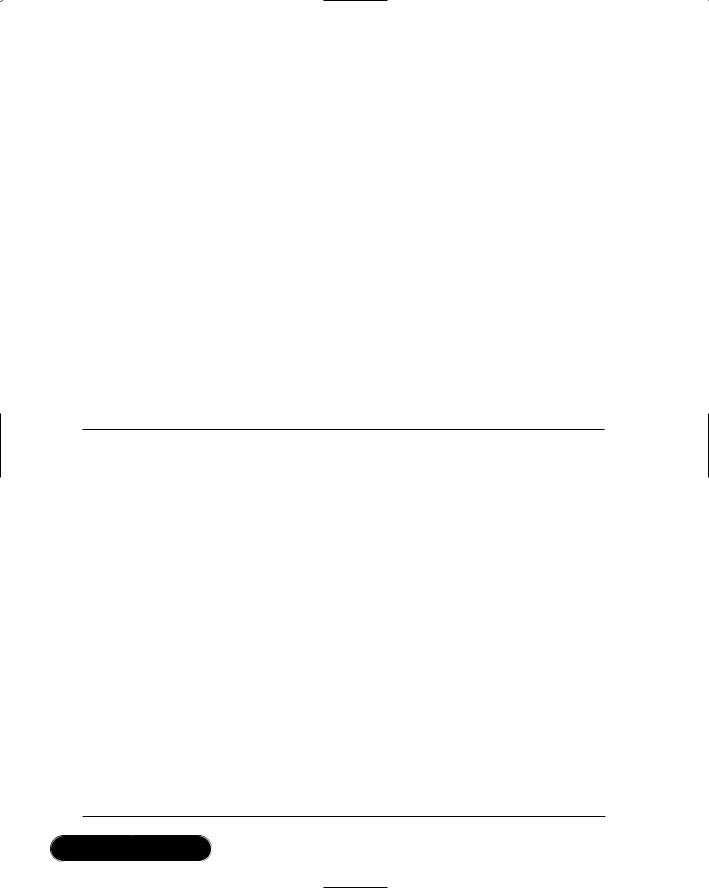
252 Chapter 5 • An ASP.NET Application
For the other counters we still use application variables, as we do not know what the names will be beforehand, or how many there will be, as we could use this script for many pages and the code picks up the name of the page as the application key.To display the value of our static variable counter, we use the name of our class, “Chapter5” and the name of the variable, “cntApplication.” As we are outputting the value using response.write (or the shorthand version at least), the variable knows to convert the integer to a string before passing out the value.
Caching Data
Storing frequently used data in memory can give you immediate and large performance gains, and can reduce load on your network and servers immensely. In this example, we will demonstrate how the new ASP.NET caching facility can be implemented in your scripts to improve performance by storing data in a cache object on the first user page request, and then using this cached copy of the data for future requests.The code for this example is shown in Figure 5.13, and the outputs are shown in Figures 5.14, 5.15, and 5.16.
Figure 5.13 Caching Example
<%@ Import Namespace="System.Data" %>
<%@ Import Namespace="System.Data.SqlClient" %> <%@ page debug=true %>
<html>
<head>
<title>
Chapter 5: Caching </title>
</head>
<body>
<form runat="server">
<p>
<!— a label to display status info —> <asp:label id="oLabel" runat="server"/>
Continued
www.syngress.com
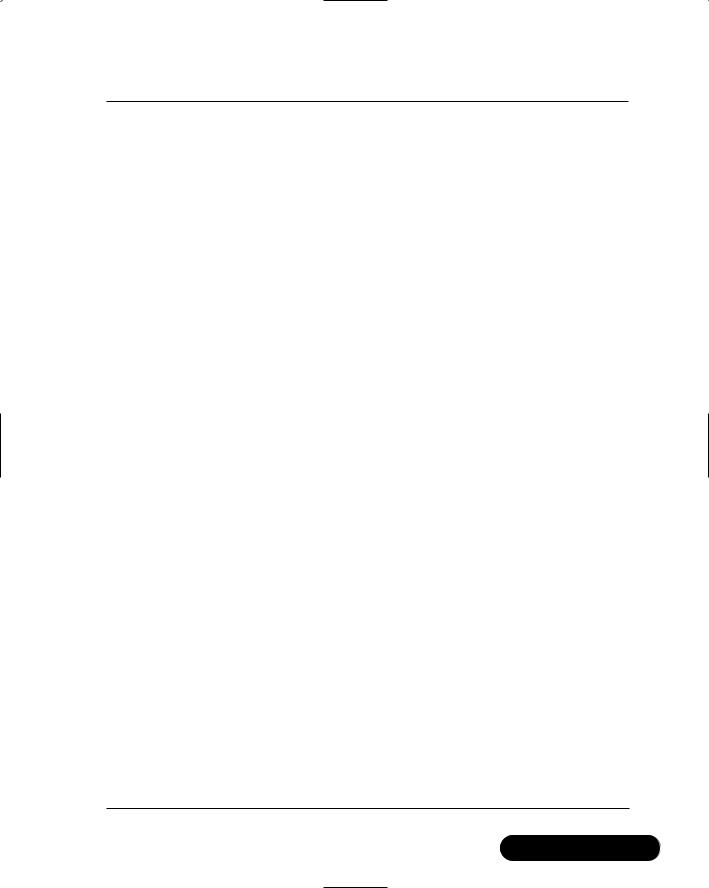
An ASP.NET Application • Chapter 5 |
253 |
Figure 5.13 Continued
<p>
<!— a button to force a cache refresh —> <asp:Button
text="Refresh Cache" id="oRefresh" OnClick="ReCache" runat="server"
/>
<p>
<!— a link to reload the page —>
<a href="cache.aspx">Reload Page</a>
<p>
<!— our data grid to display data —> <ASP:DataGrid
id="oDataGrid"
runat="server"
BorderColor="silver"
CellPadding=2
CellSpacing=2
Font-Name="Verdana"
Font-Size="10pt"
HeaderStyle-BackColor="gray"
/>
</form>
</body>
</html>
<script runat="server">
Continued
www.syngress.com

254 Chapter 5 • An ASP.NET Application
Figure 5.13 Continued
Sub Page_Load(Src As Object, E As EventArgs)
'# show the data grid with data call ShowGrid()
End Sub
Sub ReCache(Src As Object, E As EventArgs)
'# force the cache to refresh from db call RefreshCache() response.redirect("cache.aspx")
end sub
Sub ShowGrid()
'# check to see if we have cache data If Cache("gridData") Is Nothing
'# cache is empty so read from db oLabel.Text = "Reading data from Database" call RefreshCache()
Else
'# ok, we have cached data
oLabel.Text = "Reading data from Cache"
End If
'# set the data grid contents to '# whatever is in the cache
oDataGrid.DataSource=Cache("gridData")
Continued
www.syngress.com
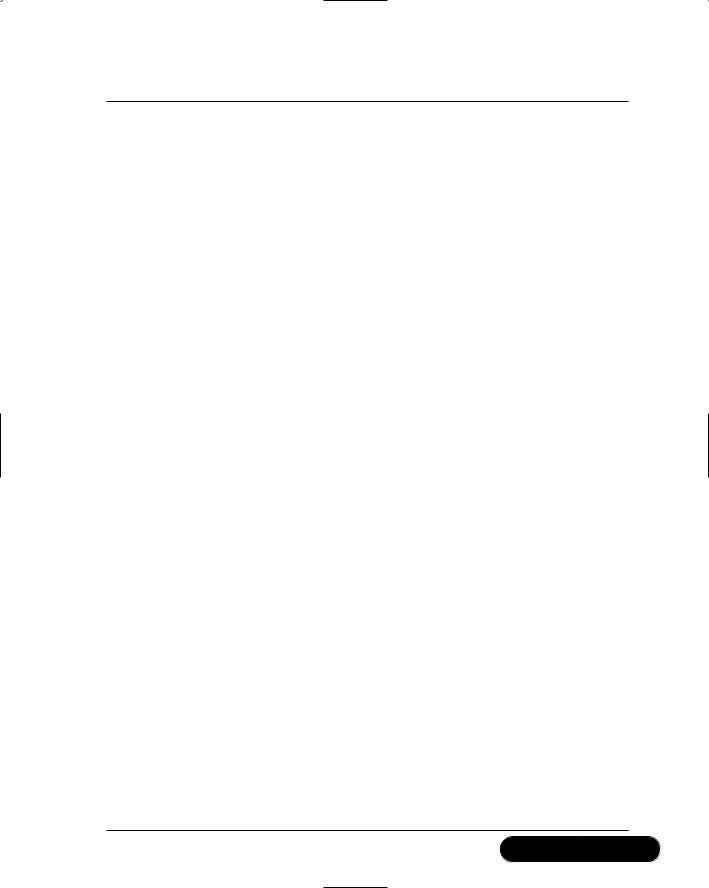
An ASP.NET Application • Chapter 5 |
255 |
Figure 5.13 Continued
oDataGrid.DataBind()
End Sub
sub RefreshCache()
'# load data from the db into '# our cache
Dim cn As SqlConnection
Dim da As SqlDataAdapter
'# set up db connection Dim dsn as String
dsn="server=(local)\NetSDK;"
dsn+="database=pubs;" dsn+="Trusted_Connection=yes" cn = New SqlConnection(dsn)
'# load sql query Dim sql as String
sql="select getDate() as Refreshed,"
sql+=" au_fname + ' ' + au_lname as Name from Authors" da = New SqlDataAdapter(sql, cn)
'# fill dataset
Dim ds As New DataSet da.Fill(ds, "Authors")
'# cache the data
Cache("gridData") = New DataView(ds.Tables("Authors"))
end sub
</script>
www.syngress.com

256 Chapter 5 • An ASP.NET Application
Figure 5.14 Screenshot Showing First Request
Figure 5.15 Screenshot Showing Subsequent Requests
www.syngress.com
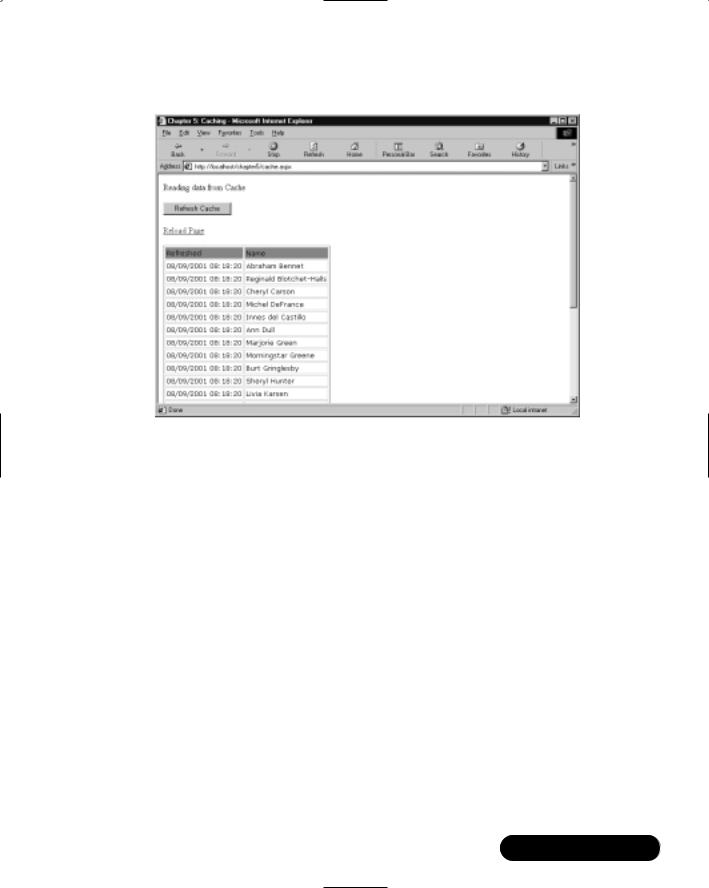
An ASP.NET Application • Chapter 5 |
257 |
Figure 5.16 Screenshot Showing Forcing Cache Refresh
As you can see, using the cache is really easy to implement; the majority of this page deals with loading data from the database or displaying results.
First the page is set up, and we display the usual HTML head and body information, plus some server-side Web form components. After this, we check to see if a copy of the data has been previously cached. If we are on our first request of the page, then the cache will be empty, so we must extract and store the data in the cache.We fill a datagrid with the data that the cache contains.We know for testing purposes whether the data has been refreshed from the database by setting a label caption.We can force the data to refresh by pushing the button that calls the same routine used when the page is first loaded, to retrieve the database data.
This is fine for circumstances where the data is sure not to change, or, like in our example, we can trust the user to push a button to force a refresh. But what if this is not acceptable?
The cache functionality includes the facility to enable you to expire content based on a time delay, another value, or a dependency on an external file.The next example shows how you can use the timed expiry to make the application refresh the data periodically, and enforce up-to-date values.
www.syngress.com

258 Chapter 5 • An ASP.NET Application
Expiring the Cache
The cache has two methods of time expiring a cache value, absoluteExpiration and slidingExpiration. Absolute expiration is when the cache is deleted at a certain date or time, and sliding expiration is a time after the cache entry was last accessed.
Syntax for the Cache Insert method is as follows, with the parameters shown in Table 5.3:
Cache.insert(key, value, dependencies, absoluteExpiration,
slidingExpiration)
Table 5.3 Parameters of the Cache Insert Method
Parameter |
Description |
|
|
key |
Identifying key for cache item |
value |
The data to store |
dependencies |
Check for changes in this value or file to expire content |
absoluteExpiration |
Remove the cache value at a specific plan |
slidingExpiration |
Delete so long after the cache value was last accessed |
To change our example so that the content is expired at a specific time interval of 10 minutes, we replace the cache-filling statements with the following:
'# cache the data
Dim MyData = New DataView(ds.Tables("Authors"))
Cache.insert("gridData", myData, nothing, _
DateTime.Now.AddMinutes(10),TimeSpan.Zero)
www.syngress.com
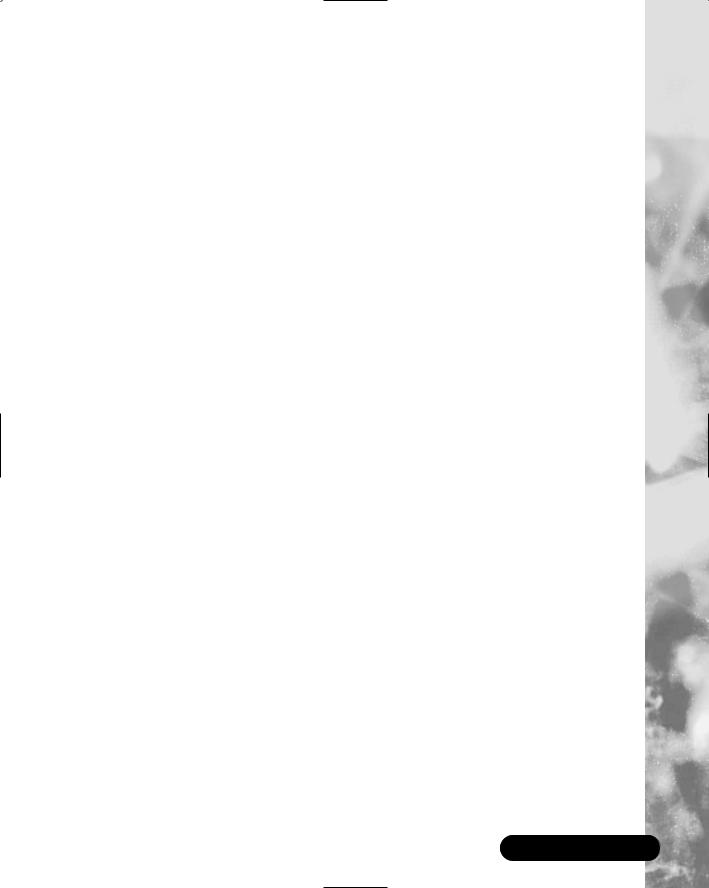
An ASP.NET Application • Chapter 5 |
259 |
Summary
ASP.NET provides an excellent framework for building Web applications. Now even more than in the past, ASP equips the programmer with excellent tools for dealing with application events and maintaining state. State is a very important subject when dealing with Web applications, and Microsoft has clearly looked carefully at how they can help the programmer out in this area.
All through this book you will see references to how Microsoft has built ASP.NET and the .NET framework as a fully object-oriented technology; ASP.NET applications carry this through to great effect, making the application Global.asax a class definition in its own right and allowing its member contents to be accessible to the rest of the application—its static variables especially. As well as application variables and the static variables, there is the new functionality of the cache object, enabling us to avoid lengthy delays over and over again for often-used data, by preloading and caching or caching on first hit.The cache also has the powerful ability to expire at a certain time and detect changes in the source it is dependant upon so that data can be refreshed.
Solutions Fast Track
Understanding ASP.NET Applications
;ASP.NET applications collect Web site resources into manageable organizational units.
;An application is made up of the files and directories within its parent folder.
;Applications can store values that are accessible to the whole application and all the users of that application.
;Events can be used to run procedures at certain points in the applications lifecycle.
Managing State
;State management allows the application to “remember” values from one transaction to the next.
www.syngress.com