
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf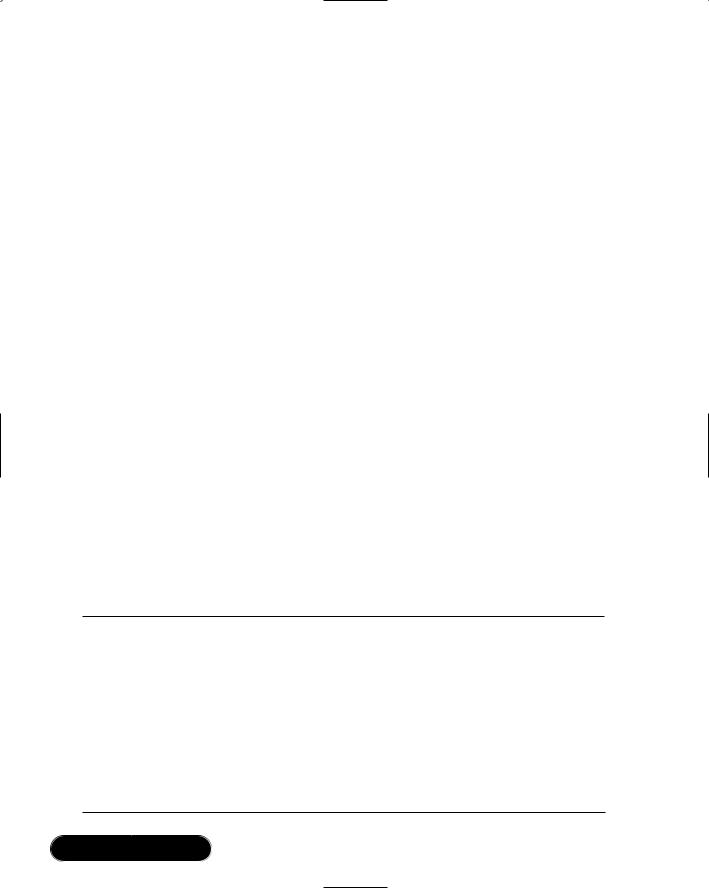
240 Chapter 5 • An ASP.NET Application
Understanding Session State
When a visitor first logs on to your Web application, they are said to have started a new session with your application, and it generates a unique Session ID.This session ID is usually stored in a cookie, but the server can be instructed to use an alternative method of passing the session ID around as part of the URL. ASP sessions enable the developer to store information for that user’s session in Session variables. Session variables are a lot like dictionary values in that they are a keyword and value pair; in an astrology application, a keyword such as “starsign” could have the value “Leo.”
You have the option of storing session values in the Web server’s memory, specifying one server in a Web farm to maintain the values or specifying the location of a database. Many developers will be glad for the many improvements this brings; the new ASP.NET solutions are broadly how many programmers overcame the limitations of ASP state management in the past.
Despite the solution provided in ASP.NET being greatly improved over the situation we have had in the past, we are still not in a position where everybody is happy. Using server memory has long been criticized, as this hogs a lot of memory resource per user, so does not scale well.Writing to a database moves the load out of memory, but adds a burden to your database management system and relies on connections being made and broken over your network.Think very carefully about what you want to achieve and what effect this will have on your system now and in the future, and, above all, do whatever works for you. If you have a few hundreds of thousands of visitors a month or less, you can probably handle using a few session variables without much concern. Our session state example is shown in Figures 5.3 and 5.4.
Figure 5.3 Session State
<html>
<head>
<title>Chapter 5</title> </head>
<body bgcolor="white">
<%
Continued
www.syngress.com
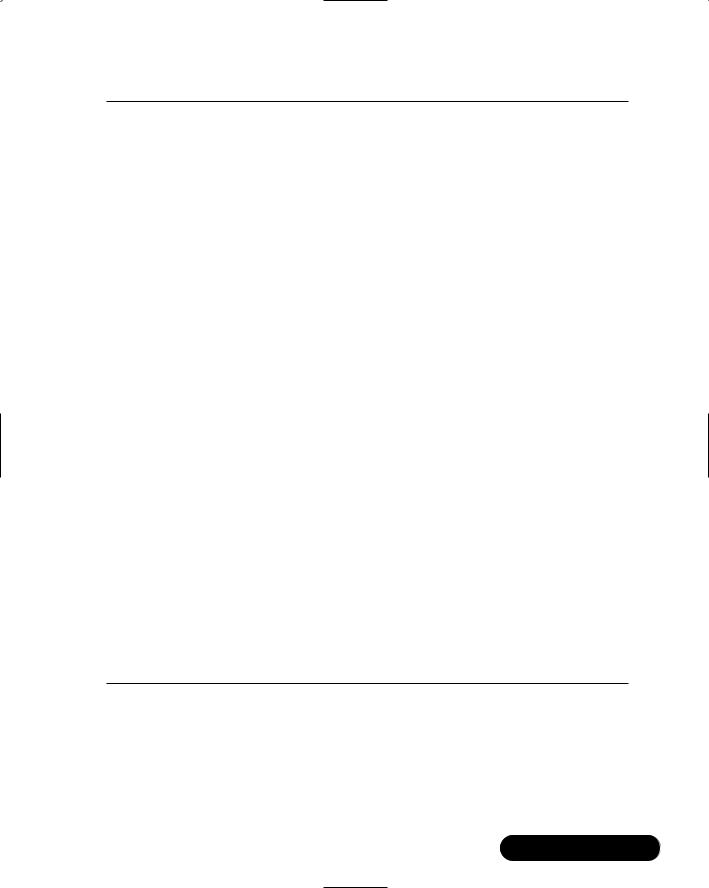
An ASP.NET Application • Chapter 5 |
241 |
Figure 5.3 Continued
'# lock the application to prevent clashes Application.Lock()
'# increment application counter Application(page.ToString) += 1
'# unlock application Application.UnLock()
'# increment session counter Session(page.ToString) += 1
%>
<p>
This page has been visited <b><%=application(page.toString)%></b> times since the application started<br>
You have visited this page <b><%=session(page.toString)%></b> times in this session<br>
</body>
</html>
Configuring Sessions
Each user session in ASP.NET is allocated a unique random 120-bit session ID that is communicated across server requests in a cookie or a modified URL, depending on how you choose to work it. If you want the application to not rely
www.syngress.com

242 Chapter 5 • An ASP.NET Application
on cookies, then you can set the application to put the ID in the URL using the following web.config setting:
<sessionstate cookieless="true" />
Figure 5.4 Application and Session State
By default, the session will expire due to the user’s inactivity after a period of 20 minutes.This is usually a reasonable figure, but there are cases where you would want to change this behavior to another duration, either to a lower amount of time because you require higher security, in case the user walks away from the browser, or change to a longer period.You can set the figure with the following web.config setting:
<sessionstate timeout="5" />
It is possible to switch off session state management.You may wonder why you would want to do this after reading how useful and easy it can be to use session state management. Session state demands additional processing by your server that may not be necessary, and the default setting sets a cookie on the clients browser that may not be ever used.
You can turn off session state in a single page with the following directive:
<%@ Page EnableSessionState="false" %>
www.syngress.com

An ASP.NET Application • Chapter 5 |
243 |
To turn off session state for the whole application, put the following in the web.config file:
<sessionstate mode="off">
Using Session Events
Just as applications have events, the session has related events also: session_OnStart and session_OnEnd. Figures 5.5, 5.6, and 5.7 demonstrate how to implement session and application events within your Global.asax files.
Figure 5.5 Session Events in Global.asax
<script runat=server>
Sub Session_onStart(ByVal sender As Object, ByVal e As EventArgs)
'# lock the application to prevent clashes Application.Lock()
'# increment application counter Application("cntApplication") += 1
'# unlock application Application.UnLock()
End Sub
</script>
Figure 5.6 Using Session Events
<html>
<head>
<title>Chapter 5</title> </head>
Continued
www.syngress.com
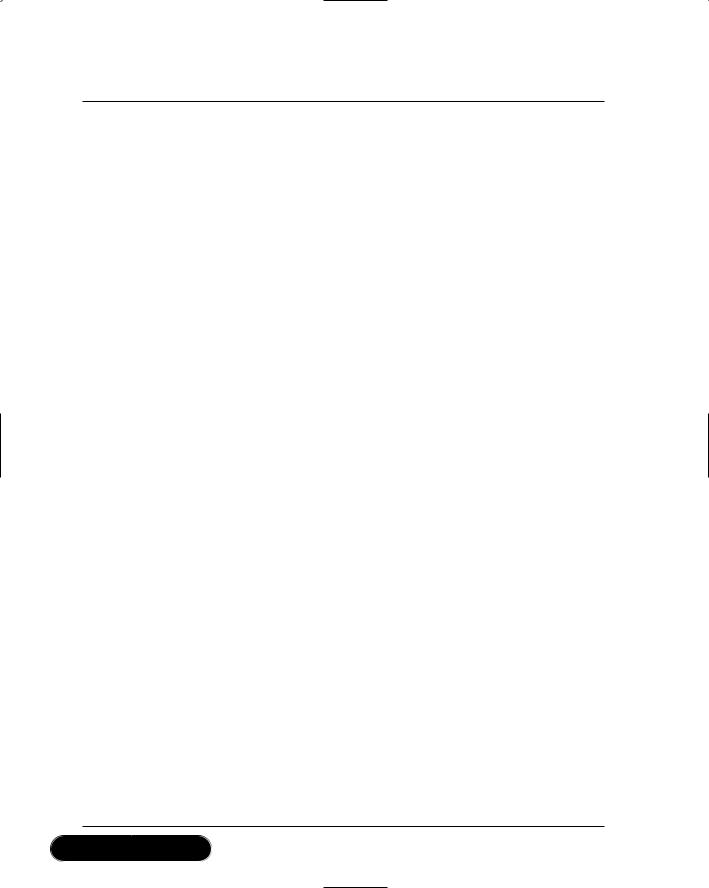
244 Chapter 5 • An ASP.NET Application
Figure 5.6 Continued
<body bgcolor="white">
<%
'# lock the application to prevent clashes Application.Lock()
'# increment application counter Application(page.ToString) += 1
'# unlock application Application.UnLock()
'# increment session counter Session(page.ToString) += 1
%>
<p>
The application has been visited <b><%=application("cntApplication")%></b> times since the application started<br>
This page has been visited <b><%=application(page.toString)%></b> times since the application started<br>
You have visited this page <b><%=session(page.toString)%></b> times in this session<br>
</body>
</html>
www.syngress.com

An ASP.NET Application • Chapter 5 |
245 |
Figure 5.7 Application Variable Updated Using Session Events
Working with Session Events
As an example of how you might implement Session events in your projects, if you wanted to use the Session_OnStart event, then you could create a Global.asax like the following:
<script language="VB" runat="server"> Sub Session_OnStart()
session("sessionStart")=DateTime.Now End Sub
Sub Session_OnEnd()
End Sub </script>
In this example, we have added code for the OnStart, but we do not need to process anything at session end, so we have left that subroutine blank.
www.syngress.com
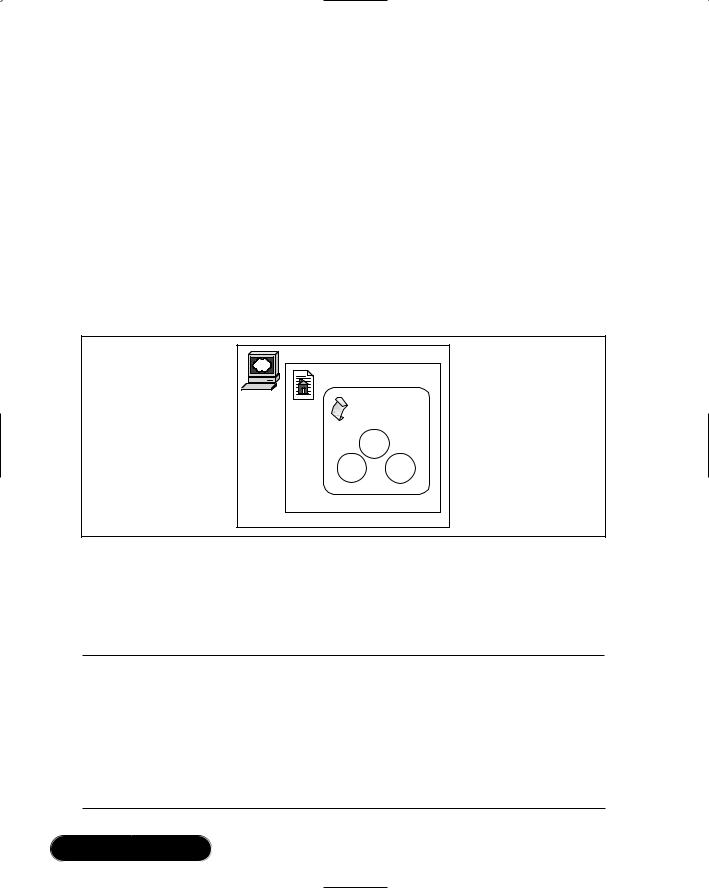
246 Chapter 5 • An ASP.NET Application
Comparing Application
and Session States
Application State deals with application-wide issues. Application state impacts every page for every user currently live in the Web application. Session State is only relevant to one user, for the duration of the particular session they are currently taking part in. One user’s session does not have any impact on any other, whereas one user can affect values that another user can see and interact with.
As you can see from the code examples and in Figure 5.8, both states complement each other, and you will probably have used many Web applications that demonstrate examples of both.
Figure 5.8 Relationship between Sessions and Applications
Web Server |
|
Web Site |
|
Application |
|
Session |
|
Session |
Session |
So far in this chapter, all the example code has been in Visual Basic .NET, so in Figures 5.9 and 5.10 we have reworked the existing application example in C# so that you can see the differences in syntax.
Figure 5.9 C# Global.asax
<script language="c#" runat=server>
public void Session_onStart()
{
// lock the application to prevent clashes Application.Lock();
Continued
www.syngress.com

An ASP.NET Application • Chapter 5 |
247 |
Figure 5.9 Continued
// increment application counter if(Application["cntApplication"] == null)
{
Application["cntApplication"] = 0;
}
Application["cntApplication"]=((int)Application["cntApplication"])+1;
// unlock application Application.UnLock();
}
</script>
Much of the change in code is due to the fact that C# is much more conscious about variable types and conversion of one type to another than in Visual Basic .NET.
In theVB example, we did not have to worry what the application variable contained; we simply incremented whatever was there.Visual Basic would worry about if it was empty, and would simply convert the empty object to be a number. C# would have an error in this scenario, both because we were trying to
increment an object instead of an integer, and also because the object at that point would contain Null.
To get around this, we have had to add a check to see what the variable contains.We know if the variable contains Null, then this is the first run through the procedure since the application was started. If this is the case, then we initialize the variable to be a number—zero, to be exact.
We now know that by the time we get to incrementing the counter, there will always be a valid number there.This is where we encounter our next difficulty.
Where in the Visual Basic .NET example we simply incremented whatever was there, using the “+=” facility, in C# it’s not that simple.The application variable would not be allowed to simply increment by one; we have to make the variable equal to one plus whatever is currently there, converted to an integer.We do this by preceding the application variable with “(int),” which means to the compiler:“Treat the following as an integer.”
www.syngress.com

248 Chapter 5 • An ASP.NET Application
Other notable differences are that C# syntax, for arrays and collections, uses square brackets rather than the curved brackets used in Visual Basic .NET, and has curly brackets around functions.
Figure 5.10 Example in C#
<%@page language="c#" %>
<html>
<head>
<title>Chapter 5</title> </head>
<body bgcolor="white">
<%
//lock the application to prevent clashes Application.Lock();
//increment application counter if(Application[Page.ToString()] == null)
{
Application[Page.ToString()] = 0;
}
Application[Page.ToString()]=((int)Application[Page.ToString()])+1;
//unlock application Application.UnLock();
//increment session counter
if(Session[Page.ToString()]==null) Session[Page.ToString()] = 0; Session[Page.ToString()]=((int)Session[Page.ToString()]) + 1;
Continued
www.syngress.com
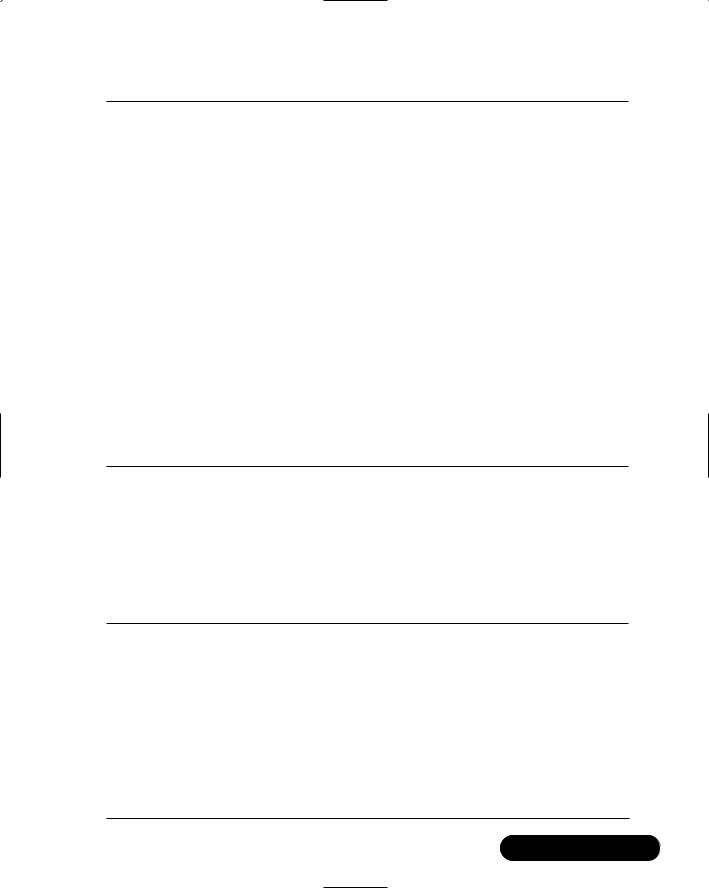
An ASP.NET Application • Chapter 5 |
249 |
Figure 5.10 Continued
%>
<p>
The application has been visited <b><%=Application["cntApplication"]%></b> times since the application started<br>
This page has been visited <b><%=Application[Page.ToString()]%></b> times since the application started<br>
You have visited this page <b><%=Session[Page.ToString()]%></b> times in this session<br>
</body>
</html>
Static Values
To return to the subject of the alternatives to application, which is caching and static variables, let us look first at an example of how static variables can make our code cleaner and make it perform better (Figure 5.11).
Figure 5.11 A C# Global.asax Class
<%@ Application Classname="Chapter5" %>
<script language="c#" runat=server>
public static int cntApplication = 0;
public void Session_onStart()
{
Continued
www.syngress.com