
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf
220 Chapter 4 • Configuring ASP.NET
Retrieving Settings
Many of the settings used within the web.config and machine.config files simply modify the way that ASP.NET works. Others enable us to dynamically customize our applications based on many factors. In order to customize our applications, we have to be able to retrieve settings from the configuration.
When we retrieve data, we are not retrieving it from any specific configuration file, but from the cached configuration.This cached configuration includes all inherited configuration and any location specific configuration information.
For the examples used in this section, we will be using the “TestConfig” application that we designed the preceding web.config file for.
ASP.NET exposes intrinsic static methods for some configuration options. An example of this is the Session method.When you have set session state configuration by using the <sessionState> tag, you can read this in by using the Session method.This process is shown in the following code sample:
Dim nocookies As Boolean = Session.Cookieless
The second method of retrieving settings is only applicable to settings configured using the <appSettings> tag.To retrieve these settings, you simply use the ConfigurationSettings.AppSettings method and supply the key.This method will return the value stored under that keyname.This method is shown in the following code sample:
Dim myvalue As String = ConfigurationSettings.AppSettings("mykey")
You can use the final retrieval method to obtain any value within the configuration.This is the ConfigurationSettings.GetConfig method. In order to use this, you must know the exact path to the configuration setting that you wish to retrieve.The syntax for this method is shown in Figure 4.3.This code is available on the included CD as testconfig.aspx.
Figure 4.3 Application (TestConfig.aspx)
<html>
<script language="VB" runat="server">
Public tblBack As String = ""
Public tblFore As String = ""
Continued
www.syngress.com

Configuring ASP.NET • Chapter 4 |
221 |
Figure 4.3 Continued
Sub Page_Load(source As Object, E As EventArgs) dim config As NameValueCollection=
ConfigurationSettings.GetConfig
("testConfig.group/mainAppSettings")
dim strTblBack as string = config("tableBackgroundColor") dim strTblFore as string = config("tableForegroundColor")
if strTblBack <> nothing then tblBack=strTblBack
else tblBack="lightgreen"
end if
if strTblFore <> nothing then tblFore=strTblFore
else tblFore="purple"
end if
End Sub
</script>
<head>
<title>Test Configuration</title> </head>
<body>
<table border=1 bgcolor=<%=tblBack%> bordercolor=<%=tblFore%>> <tr>
<td>Some</td>
<td>Important</td>
<td>Data</td>
Continued
www.syngress.com
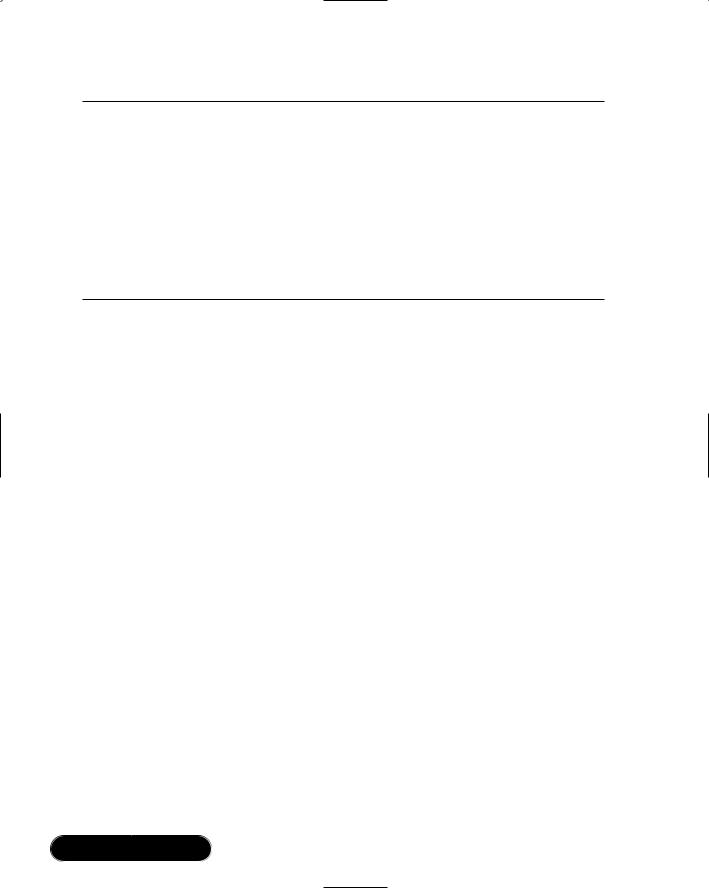
222 Chapter 4 • Configuring ASP.NET
Figure 4.3 Continued
</tr>
<tr>
<td>Some</td>
<td>More</td>
<td>Data</td>
</tr>
</table>
</body>
</html>
This code uses configuration sections that we defined within our web.config file in the previous section.The full possibilities of this configuration can be realized by first running the code in its own virtual directory, and then running the same code within an “execreports” subdirectory.This will show you how the configuration options change based on the <location> tag.
www.syngress.com

Configuring ASP.NET • Chapter 4 |
223 |
Summary
The configuration capabilities provided by ASP.NET enable you to configure almost every aspect of ASP.NET and the way that your applications are processed. It provides this ability through the use of the machine.config file and web.config files.These files are processed in a hierarchical manner with each higher-level file overriding previous settings. All settings are cached, and when a change is detected in the configuration files, the configuration is then recached.
When using the configuration files to configure ASP.NET, various tags, subtags, attributes, and options are used. Each of these enables you to control built-in configuration options or create new configuration options as you see fit. By using the available options, you can control everything from application variables down to compilation options.
The configuration files used by ASP.NET are formatted in XML and are case-sensitive. Using the correct formatting for these files is critical if you want your configuration to work correctly. All values within your configuration are accessible by using one of the three methods listed in the “Retrieving Settings” section.
Solutions Fast Track
Overview of an ASP.NET Configuration
;ASP.NET configuration settings are stored in the machine.config and the web.config files.
;ASP.NET processes the configuration settings in a hierarchical manner. It does this by processing the machine.config first, and then processing all web.config files.
;You can override the hierarchal method of configuration file processing by the use of the allowOverride attribute and the <location> tag.
Uses for a Configuration File
;By using the machine.config file as well as web.config files, you can configure ASP.NET at a very granular level.
;You can use configuration files to control most aspects of ASP.NET including application, security, and system-related options.
www.syngress.com

224Chapter 4 • Configuring ASP.NET
;You can configure additional configuration tags by creating new configuration section handlers.
Anatomy of a Configuration File
;The ASP.NET configuration files are configured using XML formatting.
;Tags and subtags contain attributes that control the various configuration options available in ASP.NET.
;You can retrieve all configuration settings within ASP.NET at any time from within your application.
Frequently Asked Questions
The following Frequently Asked Questions, answered by the authors of this book, are designed to both measure your understanding of the concepts presented in this chapter and to assist you with real-life implementation of these concepts. To have your questions about this chapter answered by the author, browse to www.syngress.com/solutions and click on the “Ask the Author” form.
Q:Should I modify the machine.config file or create a web.config file for my application?
A:That depends on the situation. If you have multiple applications running on a server, and several configuration options need to be shared between them, then place the shared configuration settings in the machine.config and any application specific settings in individual web.config files. If you only have one application on your server, just create a web.config file with all your configuration settings.
Q:Why should I use configuration files at all? Can’t I just define everything in my application?
A:Some options available within the configuration files are not available within an application. One good example of this is the use of compilation options. If you aren’t working at this level of configuration, then there are still several advantages to using the configuration files:They provide a single reference point for configuration, configuration options are cached and load quickly,
www.syngress.com

Configuring ASP.NET • Chapter 4 |
225 |
and they enable you to distribute changes to static variables within your application easily.
Q:I don’t understand what some of the configuration options do. How can I find out more about them?
A:There are two resources that I highly recommend.The first is Microsoft’s MSDN site, which contains all of the ASP.NET documentation.The second is hands-on practice. If want to learn everything about a configuration option, try it yourself in as many ways as possible.
Q: Are the configuration files case-sensitive?
A:Yes! Make sure that you follow the case guidelines for working with your configuration files. If your configuration isn’t working correctly, a good thing to look at is the case formatting of your configuration files.
www.syngress.com
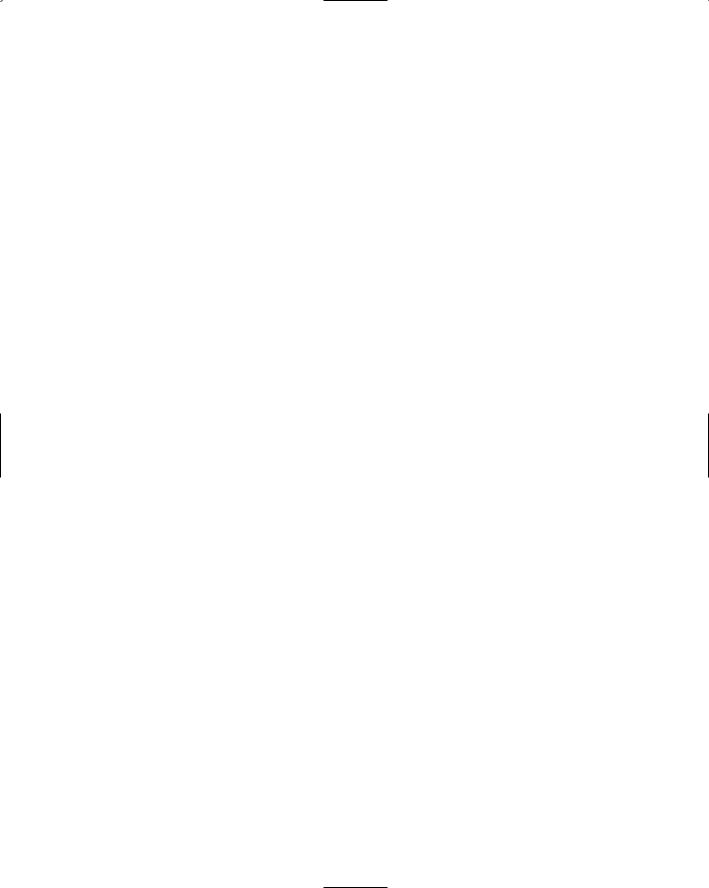
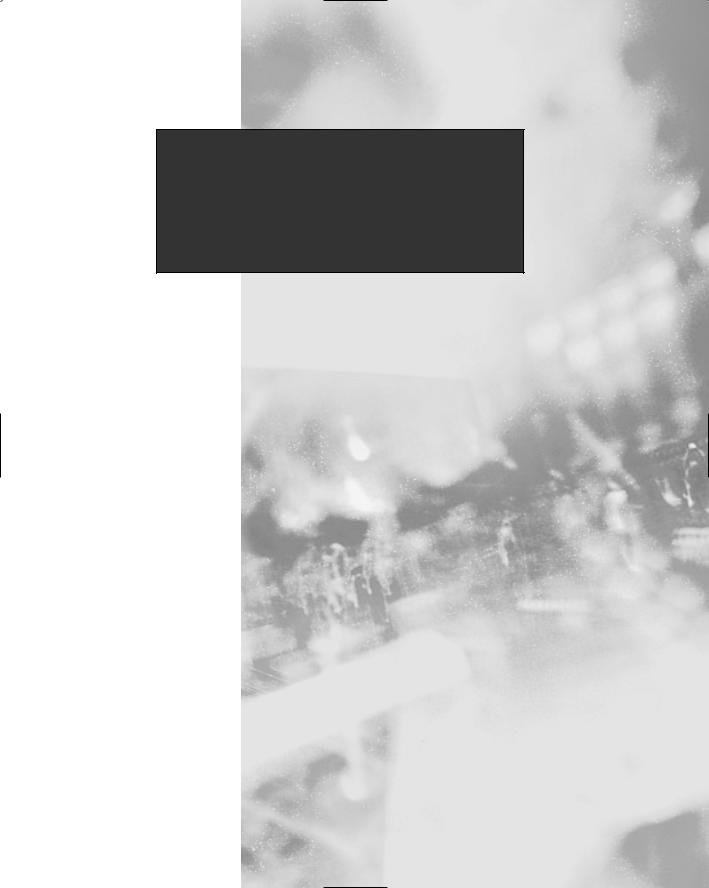
Chapter 5
An ASP.NET
Application
Solutions in this chapter:
■Understanding ASP.NET Applications
■Managing State
■Analyzing Global.asax
■Understanding Application State
■Using Application Events
■Understanding Session State
■Configuring Sessions
■Using Session Events
■Comparing Application and Session States
;Summary
;Solutions Fast Track
;Frequently Asked Questions
227
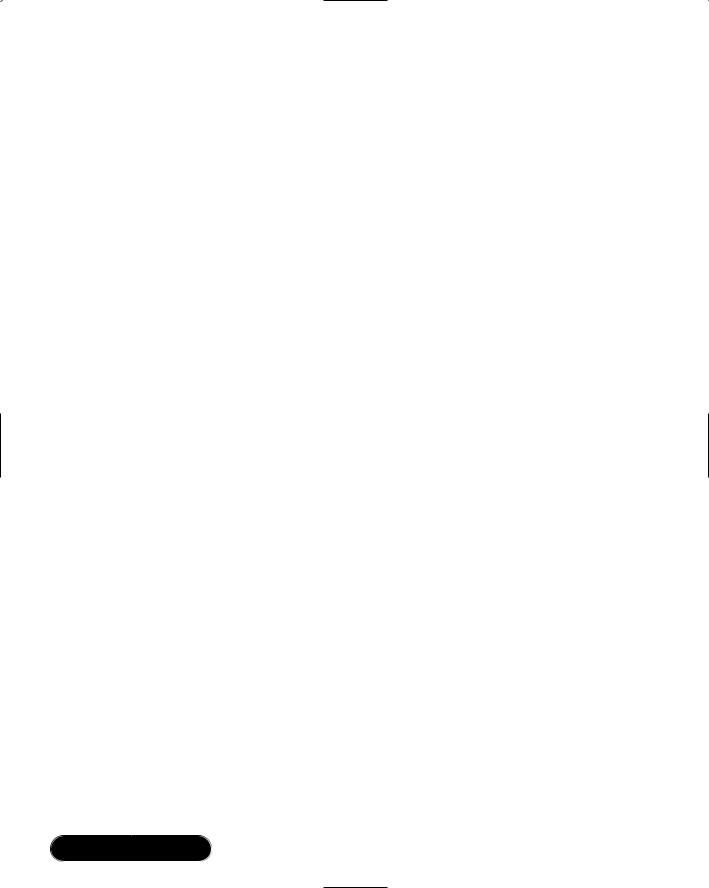
228 Chapter 5 • An ASP.NET Application
Introduction
ASP.NET applications have not changed a great deal through ASP’s development. Granted, the actual inner workings have definitely changed dramatically, but the application concept itself has not; an ASP.NET application is still defined as the developer-created files and directories that can be requested, invoked, and processed through ASP.NET within its local directory structures.
Each application can have within its own local subdirectory a file named Global.asax that defines application parameters to Internet Information Server (IIS), and to the ASP.NET Web application scripts local to it. Global.asax tells IIS what to do when the application is started and how to handle processing, depending on the state of the application.
An application can have both an application state and a session state.They are both very useful and versatile facilities when used knowledgably, reflecting the functionality that .NET has provided them. Each of these states can be further customized to contain the information needed to refine the application to your purposes.
In this chapter we will look at how you can implement application and session functionality, and will work through examples of using state in ASP.NET projects.
Understanding ASP.NET Applications
As previously outlined, ASP.NET applications collect Web site resources into manageable organizational units within the Web server’s file system hierarchy. But what exactly does this mean to the programmer?
A user expects a Web site to simply serve up the pages the visitor has asked for. He or she may be pleasantly surprised that the Web site knows who they are and personalizes their experience, but they will not necessarily expect it. If they choose to use a Web application, on the other hand, their expectations will be very different.
Examples of a Web application could be a multi-page bank account sign-up form.The user would expect to be able to navigate to the next and previous pages without reentering information. Another example may be an intranet. Once logged in, the user would expect the intranet to remember who they are and what they have access to without repeatedly entering the same information.
As well as user-centric information, it is also useful to remember that Web applications are multi-user. It can be handy for the application to be aware of its
www.syngress.com
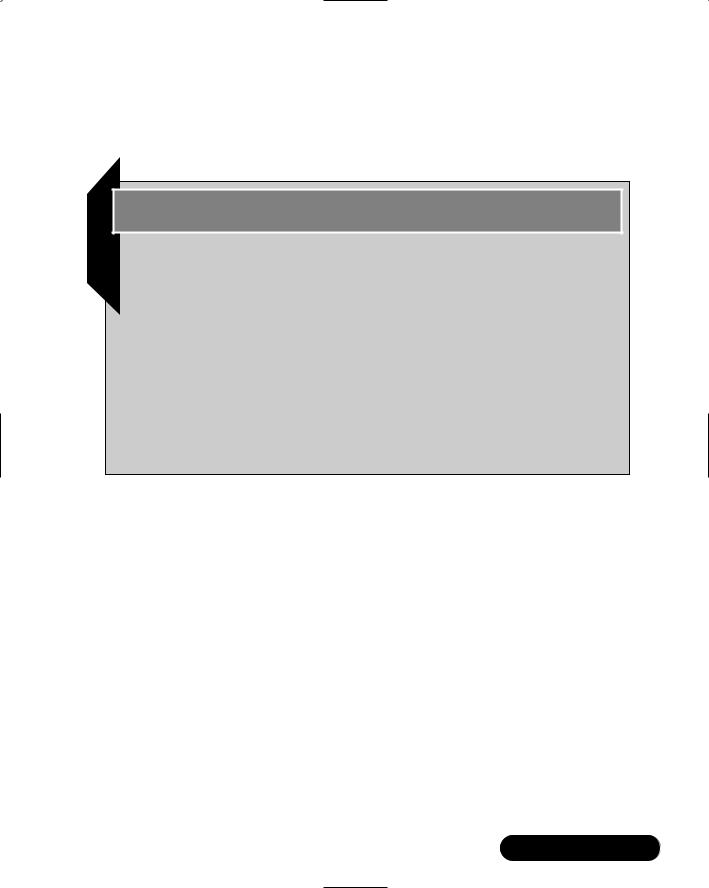
An ASP.NET Application • Chapter 5 |
229 |
own application settings and values and all of its logged-in users, especially in some sort of multi-user environment, such as a live chat application or multi-user adventure game.
Developing & Deploying…
Creating Your Application
Unfortunately, applications are one of the few areas that must still be managed initially by your Web server administrator through the Microsoft Management Console (MMC). By default, the Web root folder is considered the only application (the default application), and all subdirectories of that can access the application variables of their parent. If you require a new application, you must go into the MMC and either create a new virtual directory, or get the properties of the folder in which you wish to make an application by right-clicking and selecting Properties and then clicking the Create button. Any scripts in this new application will now access application variables for this application only and will not have access to those of its parent.
Managing State
State management has always been a subject of much debate since Web development began. For smaller projects, state is a little easier to manage and you can pretty much do whatever works best for you, but Web architects still disagree on the subject when discussing multi-million-dollar mega-projects.What is state, and why is the subject so difficult?
One of the first things that strike an experienced programmer coming to the Web for the first time is the odd fact that from one transaction to the next, the Web server, and therefore application, forgets who you are. On a desktop application, clicking buttons affects only the action you wish to achieve; it is very easy for the programmer to track what you have done before and what you are likely to do next.With a Web application, each request appears to the server as if it came from a new user, as the connection between the client and server is closed once the request has been processed. A desktop application maintains state by default, whereas the Web is stateless.
www.syngress.com