
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference

Chapter 12 Working with Inheritance |
233 |
The Drive method is virtual, so the runtime (not the compiler) works out which version of the Drive method to call when invoking it through a Vehicle variable based on the real type of the object referenced by this variable. In the first case, the Vehicle object refers to a Car, so the application calls the Car.Drive method. In the second case, the Vehicle object refers to an Airplane, so the application calls the Airplane.Drive method.
28. Press Enter to close the application and return to Visual Studio 2008.
Understanding Extension Methods
Inheritance is a very powerful feature, enabling you to extend the functionality of a class by creating a new class that derives from it. However, sometimes using inheritance is not the most appropriate mechanism for adding new behaviors, especially if you need to quickly extend a type without affecting existing code.
For example, suppose you want to add a new feature to the int type—a method named Negate that returns the negative equivalent value that an integer currently contains. (I know
that you could simply use the unary minus operator [-] to perform the same task, but bear with me.) One way to achieve this would be to define a new type named NegInt32 that inherits from System.Int32 (int is an alias for System.Int32) and that adds the Negate method:
class NegInt32 : System.Int32 // don’t try this!
{
public int Negate()
{
...
}
}
The theory is that NegInt32 will inherit all the functionality associated with the System.Int32 type in addition to the Negate method. There are two reasons why you might not want to
follow this approach:
This method will apply only to the NegInt32 type, and if you want to use it with existing int variables in your code, you would have to change the definition of every int variable to the NegInt32 type.
The System.Int32 type is actually a structure, not a class, and you cannot use inheritance with structures.
This is where extension methods become very useful.
An extension method enables you to extend an existing type (a class or a structure) with additional static methods. These static methods become immediately available to your code in any statements that reference data of the type being extended.
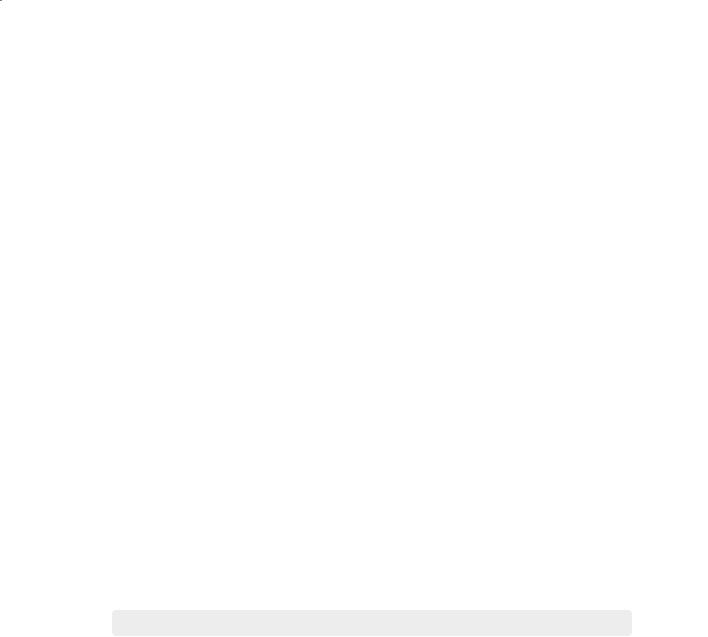
234 Part II Understanding the C# Language
You define an extension method in a static class and specify the type that the method applies
to as the first parameter to the method, along with the this keyword. Here’s an example showing how you can implement the Negate extension method for the int type:
static class Util
{
public static int Negate(this int i)
{
return –i;
}
}
The syntax looks a little odd, but it is the this keyword prefixing the parameter to Negate that identifies it as an extension method, and the fact that the parameter that this prefixes is an int means that you are extending the int type.
To use the extension method, bring the Util class into scope (if necessary, add a using statement specifying the namespace to which the Util class belongs), and then you can simply use “.” notation to reference the method, like this:
int x = 591;
Console.WriteLine(“x.Negate {0}”, x.Negate());
Notice that you do not need to reference the Util class anywhere in the statement that calls
the Negate method. The C# compiler automatically detects all extension methods for a given type from all the static classes that are in scope. You can also invoke the Utils.Negate method
passing an int as the parameter, using the regular syntax you have seen before, although this use obviates the purpose of defining the method as an extension method:
int x = 591;
Console.WriteLine(“x.Negate {0}”, Util.Negate(x));
In the following exercise, you will add an extension method to the int type. This extension method enables you to convert the value an int variable contains from base 10 to a representation of that value in a different number base.
Create an extension method
1.In Visual Studio 2008, open the ExtensionMethod project, located in the \Microsoft Press\Visual CSharp Step by Step\Chapter 12\ExtensionMethod folder in your Documents folder.
2.Display the Util.cs file in the Code and Text Editor window.
This file contains a static class named Util in a namespace named Extensions. The class is empty apart from the // to do comment. Remember that you must define extension methods inside a static class.

Chapter 12 Working with Inheritance |
235 |
3.Add a public static method to the Util class, named ConvertToBase. The method should take two parameters: an int parameter named i, prefixed with the this keyword to indicate that the method is an extension method for the int type, and another ordinary int parameter named baseToConvertTo. The method will convert the value in i to the base indicated by baseToConvertTo. The method should return an int containing the converted value.
The ConvertToBase method should look like this:
static class Util
{
public static int ConvertToBase(this int i, int baseToConvertTo)
{
}
}
4.Add an if statement to the ConvertToBase method that checks that the value of the baseToConvertTo parameter is between 2 and 10. The algorithm used by this exercise does not work reliably outside this range of values. Throw an ArgumentException with a suitable message if the value of baseToConvertTo is outside this range.
The ConvertToBase method should look like this:
public static int ConvertToBase(this int i, int baseToConvertTo)
{
if (baseToConvertTo < 2 || baseToConvertTo > 10)
throw new ArgumentException(“Value cannot be converted to base “ + baseToConvertTo.ToString());
}
5.Add the following statements shown in bold to the ConvertToBase method, after the statement that throws the ArgumentException. This code implements a well-known algorithm that converts a number from base 10 to a different number base:
public static int ConvertToBase(this int i, int baseToConvertTo)
{
...
int result = 0; int iterations = 0; do
{
int nextDigit = i % baseToConvertTo;
result += nextDigit * (int)Math.Pow(10, iterations); iterations++;
i /= baseToConvertTo;
}
while (i != 0); return result;
}
6. Display the Program.cs file in the Code and Text Editor window.
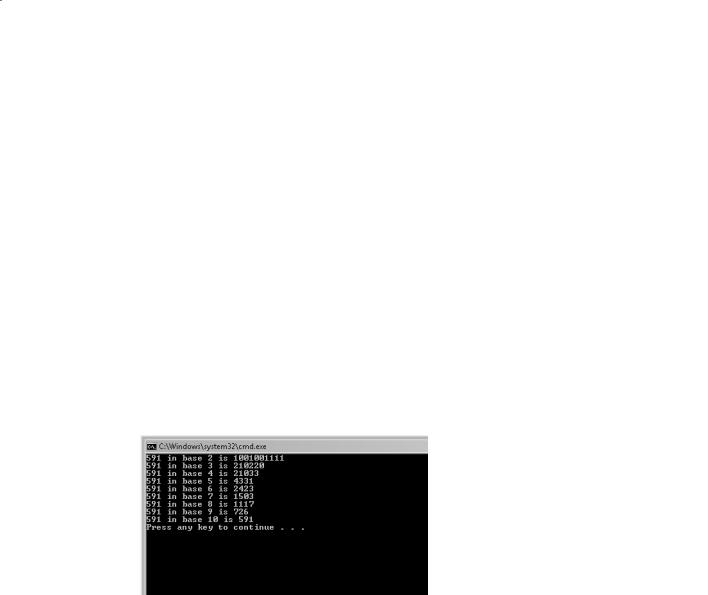
236Part II Understanding the C# Language
7.Add the following using statement after the using System; statement at the top of the file:
using Extensions;
This statement brings the namespace containing the Util class into scope. The ConvertToBase extension method will not be visible in the Program.cs file if you do not
perform this task.
8. Add the following statements to the Entrance method of the Program class:
int x = 591;
for (int i = 2; i <= 10; i++)
{
Console.WriteLine(“{0} in base {1} is {2}”, x, i, x.ConvertToBase(i));
}
This code creates an int named x and sets it to the value 591. (You could pick any
integer value you want.) The code then uses a loop to print out the value 591 in all number bases between 2 and 10. Notice that ConvertToBase appears as an extension method in IntelliSense when you type the period (.) after x in the Console.WriteLine
statement.
9.On the Debug menu, click Start Without Debugging. Confirm that the program displays messages showing the value 591 in the different number bases to the console, like this:
10. Press Enter to close the program.
Congratulations. You have successfully used inheritance to define a hierarchy of classes, and you should now understand how to override inherited methods and implement virtual methods. You have also seen how to add an extension method to an existing type.