
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference

|
|
Chapter 4 Using Decision Statements |
71 |
|
Category |
Operators |
Description |
Associativity |
|
Primary |
( ) |
Precedence override |
Left |
|
++Post-increment
--Post-decrement
Unary |
! |
Logical NOT |
Left |
|
+ |
Addition |
|
|
- |
Subtraction |
|
|
++ |
Pre-increment |
|
|
-- |
Pre-decrement |
|
|
|
|
|
Multiplicative |
* |
Multiply |
Left |
|
/ |
Divide |
|
|
% |
Division remainder |
|
|
|
(modulus) |
|
|
|
|
|
Additive |
+ |
Addition |
Left |
|
- |
Subtraction |
|
|
|
|
|
Relational |
< |
Less than |
Left |
|
<= |
Less than or equal to |
|
|
> |
Greater than |
|
|
>= |
Greater than or equal to |
|
|
|
|
|
Equality |
== |
Equal to |
Left |
|
!= |
Not equal to |
|
|
|
|
|
Conditional AND |
&& |
Logical AND |
Left |
Conditional OR |
|| |
Logical OR |
Left |
|
|
|
|
Assignment |
= |
|
Right |
|
|
|
|
Using if Statements to Make Decisions
When you want to choose between executing two different blocks of code depending on the result of a Boolean expression, you can use an if statement.
Understanding if Statement Syntax
The syntax of an if statement is as follows (if and else are C# keywords):
if ( booleanExpression ) statement-1;
else
statement-2;
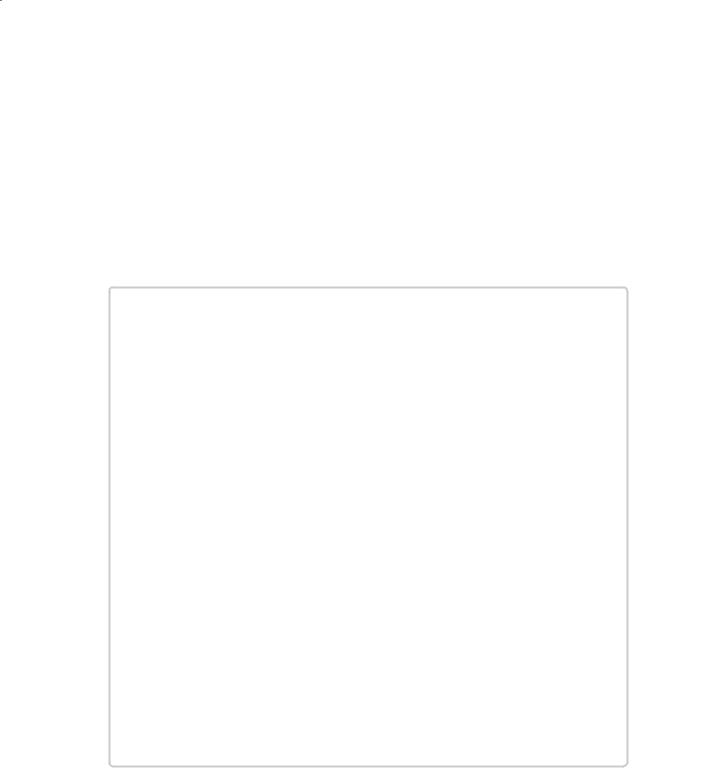
72 |
Part I Introducing Microsoft Visual C# and Microsoft Visual Studio 2008 |
|
If booleanExpression evaluates to true, statement-1 runs; otherwise, statement-2 runs. The |
|
else keyword and the subsequent statement-2 are optional. If there is no else clause and the |
|
booleanExpression is false, execution continues with whatever code follows the if statement. |
|
For example, here’s an if statement that increments a variable representing the second hand |
|
of a stopwatch (minutes are ignored for now). If the value of the seconds variable is 59, it is |
|
reset to 0; otherwise, it is incremented using the ++ operator: |
|
int seconds; |
|
... |
|
if (seconds == 59) |
|
seconds = 0; |
|
else |
|
seconds++; |
Boolean Expressions Only, Please!
The expression in an if statement must be enclosed in parentheses. Additionally, the expression must be a Boolean expression. In some other languages (notably C and
C++), you can write an integer expression, and the compiler will silently convert the integer value to true (nonzero) or false (0). C# does not support this behavior, and the
compiler reports an error if you write such an expression.
If you accidentally specify the assignment operator, =, instead of the equality test operator, ==, in an if statement, the C# compiler recognizes your mistake and refuses to compile your code. For example:
int seconds;
...
if (seconds = 59) // compile-time error
...
if (seconds == 59) // ok
Accidental assignments were another common source of bugs in C and C++ programs, which would silently convert the value assigned (59) to a Boolean expression (anything nonzero was considered to be true), with the result that the code following the if statement would be performed every time.
Incidentally, you can use a Boolean variable as the expression for an if statement, although it must still be enclosed in parentheses, as shown in this example:
bool inWord;
...
if (inWord == true) // ok, but not commonly used
...
if (inWord) // better
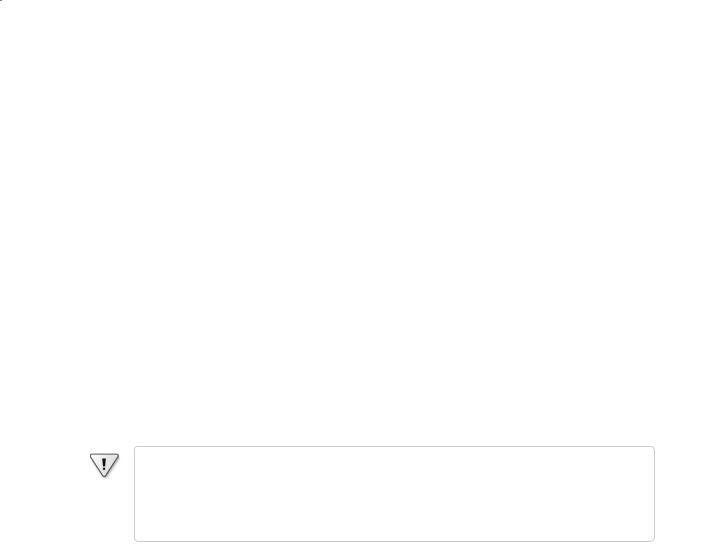
Chapter 4 Using Decision Statements |
73 |
Using Blocks to Group Statements
Notice that the syntax of the if statement shown earlier specifies a single statement after the if (booleanExpression) and a single statement after the else keyword. Sometimes you’ll want
to perform more than one statement when a Boolean expression is true. You could group the statements inside a new method and then call the new method, but a simpler solution is to group the statements inside a block. A block is simply a sequence of statements grouped between an opening and a closing brace. A block also starts a new scope. You can define variables inside a block, but they will disappear at the end of the block.
In the following example, two statements that reset the seconds variable to 0 and increment the minutes variable are grouped inside a block, and the whole block executes if the value of seconds is equal to 59:
int seconds = 0; int minutes = 0;
...
if (seconds == 59)
{
seconds = 0; minutes++;
}
else
seconds++;
Important If you omit the braces, the C# compiler associates only the first statement (seconds = 0;) with the if statement. The subsequent statement (minutes++;) will not be recognized by the compiler as part of the if statement when the program is compiled. Furthermore, when the compiler reaches the else keyword, it will not associate it with the previous if statement, and it will report a syntax error instead.
Cascading if Statements
You can nest if statements inside other if statements. In this way, you can chain together a
sequence of Boolean expressions, which are tested one after the other until one of them evaluates to true. In the following example, if the value of day is 0, the first test evaluates to true and dayName is assigned the string “Sunday”. If the value of day is not 0, the first test
fails and control passes to the else clause, which runs the second if statement and compares the value of day with 1. The second if statement is reached only if the first test is false. Similarly, the third if statement is reached only if the first and second tests are false.
if (day == 0)
dayName = “Sunday”; else if (day == 1)
dayName = “Monday”;
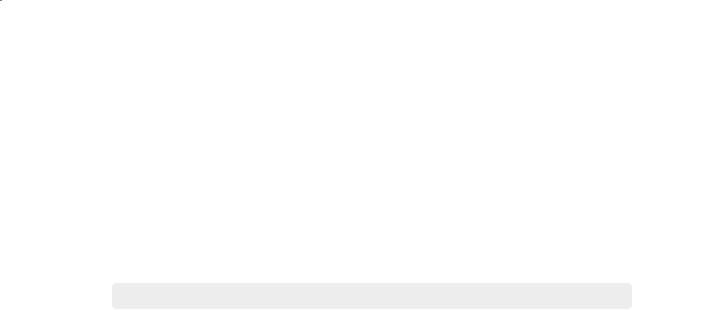
74Part I Introducing Microsoft Visual C# and Microsoft Visual Studio 2008
else if (day == 2) dayName = “Tuesday”;
else if (day == 3) dayName = “Wednesday”;
else if (day == 4) dayName = “Thursday”;
else if (day == 5) dayName = “Friday”;
else if (day == 6) dayName = “Saturday”;
else
dayName = “unknown”;
In the following exercise, you’ll write a method that uses a cascading if statement to compare two dates.
Write if statements
1.Start Microsoft Visual Studio 2008 if it is not already running.
2.Open the Selection project, located in the \Microsoft Press\Visual CSharp Step by Step\Chapter 4\Selection folder in your Documents folder.
3.On the Debug menu, click Start Without Debugging.
Visual Studio 2008 builds and runs the application. The form contains two DateTimePicker controls called first and second. (These controls display a calendar allowing you to select a date when you click the drop-down arrow.) Both controls are initially set to the current date.
4.Click Compare.
The following text appears in the text box:
first == second : False first != second : True first < second : False first <= second : False first > second : True first >= second : True
The Boolean expression first == second should be true because both first and second are set to the current date. In fact, only the less than operator and the greater than or equal to operator seem to be working correctly.
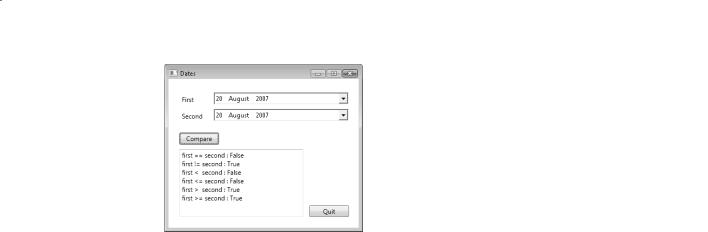
Chapter 4 Using Decision Statements |
75 |
5.Click Quit to return to the Visual Studio 2008 programming environment.
6.Display the code for Window1.xaml.cs in the Code and Text Editor window.
7.Locate the compareClick method, which looks like this:
private int compareClick(object sender, RoutedEventArgs e)
{
int diff = dateCompare(first.Value, second.Value); info.Text = “”;
show(“first == second”, diff == 0); show(“first != second”, diff != 0); show(“first < second”, diff < 0); show(“first <= second”, diff <= 0); show(“first > second”, diff > 0); show(“first >= second”, diff >= 0);
}
This method runs whenever the user clicks the Compare button on the form. It retrieves the values of the dates displayed in the first and second DateTimePicker controls on the form and calls another method called dateCompare to compare them. You will examine the dateCompare method in the next step.
The show method summarizes the results of the comparison in the info text box control on the form.
8. Locate the dateCompare method, which looks like this:
private int dateCompare(DateTime leftHandSide, DateTime rightHandSide)
{
// TO DO return 42;
}
This method currently returns the same value whenever it is called, rather than 0, -1, or +1 depending on the values of its parameters. This explains why the application is not working as expected!

76 |
Part I Introducing Microsoft Visual C# and Microsoft Visual Studio 2008 |
The purpose of this method is to examine its arguments and return an integer value based on their relative values; it should return 0 if they have the same value, -1 if the value of the first argument is less than the value of the second argument, and +1 if the value of the first argument is greater than the value of the second argument. (A date is considered greater than another date if it comes after it chronologically.) You need to implement the logic in this method to compare two dates correctly.
9.Remove the // TO DO comment and the return statement from the dateCompare method.
10.Add the following statements shown in bold type to the body of the dateCompare method:
private int dateCompare(DateTime leftHandSide, DateTime rightHandSide)
{
int result;
if (leftHandSide.Year < rightHandSide.Year) result = -1;
else if (leftHandSide.Year > rightHandSide.Year) result = +1;
}
If the expression leftHandSide.Year < rightHandSide.Year is true, the date in leftHandSide must be earlier than the date in rightHandSide, so the program
sets the result variable to -1. Otherwise, if the expression leftHandSide.Year > rightHandSide.Year is true, the date in leftHandSide must be later than the date in rightHandSide, and the program sets the result variable to +1.
If the expression leftHandSide.Year < rightHandSide.Year is false and the expression leftHandSide.Year > rightHandSide.Year is also false, the Year property of both dates must be the same, so the program needs to compare the months in each date.
11.Add the following statements shown in bold type to the body of the dateCompare method, after the code you entered in the preceding step:
private int dateCompare(DateTime leftHandSide, DateTime rightHandSide)
{
...
else if (leftHandSide.Month < rightHandSide.Month) result = -1;
else if (leftHandSide.Month > rightHandSide.Month) result = +1;
}
These statements follow a similar logic for comparing months to that used to compare years in the preceding step.

Chapter 4 Using Decision Statements |
77 |
If the expression leftHandSide.Month < rightHandSide.Month is false and the expression leftHandSide.Month > rightHandSide.Month is also false, the Month property of both dates must be the same, so the program finally needs to compare the days in each date.
12.Add the following statements to the body of the dateCompare method, after the code you entered in the preceding two steps:
private int dateCompare(DateTime leftHandSide, DateTime rightHandSide)
{
...
else if (leftHandSide.Day < rightHandSide.Day) result = -1;
else if (leftHandSide.Day > rightHandSide.Day) result = +1;
else
result = 0; return result;
}
You should recognize the pattern in this logic by now.
If leftHandSide.Day < rightHandSide.Day and leftHandSide.Day >
rightHandSide.Day both are false, the value in the Day properties in both variables must be the same. The Month values and the Year values must also be identical,
respectively, for the program logic to have reached this far, so the two dates must be the same, and the program sets the value of result to 0.
The final statement returns the value stored in the result variable.
13.On the Debug menu, click Start Without Debugging.
The application is rebuilt and restarted. Once again, the two DateTimePicker controls, first and second, are set to the current date.
14.Click Compare.
The following text appears in the text box:
first == second : True first != second : False first < second : False first <= second : True first > second : False first >= second : True
These are the correct results for identical dates.
15.Click the drop-down arrow for the second DateTimePicker control, and then click tomorrow’s date.