
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference
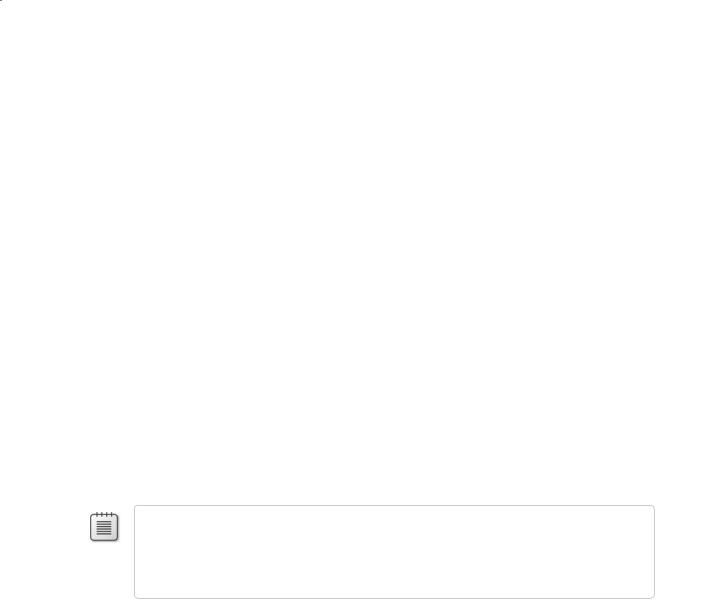
Chapter 30
Creating and Using a Web Service
After completing this chapter, you will be able to:
Create a Web service that exposes simple Web methods.
Display the description of a Web service by using Windows Internet Explorer.
Design classes that can be passed as parameters to a Web method and returned from a Web method.
Create a reference to a Web service in a client application.
Invoke a Web method.
The previous chapters showed you how to create Web forms and build Web applications by using Microsoft ASP.NET. Although this approach is appropriate for applications where the client is a Web browser, you will increasingly encounter situations where the client is some other type of application. As mentioned in previous chapters, the Internet is just a big network. By using Web services, it is possible to build distributed systems from elements that are spread across the Internet—databases, business services, and so on. The aim of this
chapter is to show you how to design, build, and test Web services that can be accessed over the Internet and integrated into distributed applications. You’ll also learn how to construct a client application that uses the methods exposed by a Web service.
Note The purpose of this chapter is to provide a very basic introduction to Web services and Microsoft Windows Communication Foundation (WCF). If you want detailed information
about how WCF works and how to build secure services by using WCF, you should consult a book such as Microsoft Windows Communication Foundation Step by Step, published by
Microsoft Press, 2007.
What Is a Web Service?
A Web service is a business component that provides some useful function to clients, or consumers. A Web service can be thought of as a component with truly global accessibility— if you have the appropriate access rights, you can make use of a Web service from anywhere in the world as long as your computer is connected to the Internet. Web services use a standard, accepted, and well-understood protocol, Hypertext Transfer Protocol (HTTP), to transmit data and a portable data format that is based on XML. HTTP and XML are both standardized technologies that can be used by other programming environments outside
623

624 |
Part VI Building Web Applications |
|
the Microsoft .NET Framework. You can build Web services by using Microsoft Visual Studio |
|
2008. Client applications running in a totally different environment, such as Java, can use |
|
them. The reverse is also true: you can build Web services by using Java and write client |
|
applications in C#. |
|
With Visual Studio 2008, you can build Web services by using Microsoft Visual C++, Microsoft |
|
Visual C#, or Microsoft Visual Basic. However, as far as a client application is concerned, the |
|
language used to create the Web service, and even how the Web service performs its tasks, is |
|
not important. The client application’s view of a Web service is of an interface that exposes a |
|
number of well-defined methods, known as Web methods. All the client application needs to |
|
do is call these Web methods by using the standard Internet protocols, passing parameters in |
|
an XML format and receiving responses also in an XML format. |
|
One of the driving forces behind the recent releases of the Windows operating system, the |
|
.NET Framework, and its associated development tools is the concept of the “programmable |
|
Web.” The idea is that you can construct systems by using the data and services supplied |
|
by multiple Web services. Web services provide the basic elements for systems, the Web |
|
provides the means to access them, and developers glue them together in meaningful ways |
|
to add functionality to their applications. Web services are a key integration technology for |
|
combining disparate systems, and they are the basis for many business-to-business (B2B) and |
|
business-to-consumer (B2C) applications. |
The Role of SOAP
Simple Object Access Protocol (SOAP) is the protocol used by client applications for sending requests to and receiving responses from Web services. SOAP is a lightweight protocol built on top of HTTP—the protocol used by the Web to send and receive HTML pages. SOAP defines an XML grammar for specifying the names of Web methods that a consumer can invoke on a Web service, for defining the parameters and return values, and for describing the types of parameters and return values. When a client calls a Web service, it must specify the method and parameters by using this XML grammar.
SOAP is an industry standard. Its function is to improve cross-platform interoperability. The strength of SOAP is its simplicity and also the fact that it is based on other industry-standard technologies, such as HTTP and XML. The SOAP specification defines a number of things. The most important are the following:
The format of a SOAP message
How data should be encoded
How to send messages (method calls)
How to process replies

Chapter 30 Creating and Using a Web Service |
625 |
Descriptions of the exact details of how SOAP works and the internal format of a SOAP message are beyond the scope of this book. It is highly unlikely that you will ever need to create and format SOAP messages manually because many development tools, including Visual Studio 2008, automate this process, presenting a programmer-friendly API to developers building Web services and client applications.
What Is the Web Services Description Language?
The body of a SOAP message is an XML document. When a client application invokes a Web method, the Web server expects the client to use a particular set of tags for encoding the parameters for the method. How does a client know which tags, or XML schema, to use? The answer is that, when asked, a Web service is expected to supply a description of itself. The Web service response is another XML document that describes the Web service. Unsurprisingly, this document is known as the Web Service Description. The XML schema used for this document has been standardized and is called Web Services Description Language (WSDL). This description provides enough information so that a client application can construct a SOAP request in a format that the Web server should understand. Again, the details of WSDL are beyond the scope of this book, but Visual Studio 2008 contains tools that parse the WSDL for a Web service in a mechanical manner. Visual Studio 2008 then uses the information to define a proxy class that a client application can use to convert ordinary method calls on this proxy class to SOAP requests that the proxy sends over the Web. This is the approach you will use in the exercises in this chapter.
Nonfunctional Requirements of Web Services
The initial efforts to define Web services and their associated standards concentrated on the functional aspects for sending and receiving SOAP messages. Not long after Web services became a mainstream technology for integrating distributed services, it became apparent that there were issues that SOAP and HTTP alone could not address. These issues concern many nonfunctional requirements that are important in any distributed environment, but much more so when using the Internet as the basis for a distributed solution. They include the following items:
Security How do you ensure that SOAP messages that flow between a Web service and a consumer have not been intercepted and changed on their way across the Internet? How can you be sure that a SOAP message has actually been sent by the consumer or Web service that claims to have sent it, and not some “spoof” site that is trying to obtain information fraudulently? How can you restrict access to a Web service to specific users? These are matters of message integrity, confidentiality, and authentication and are fundamental concerns if you are building distributed applications that make use of the Internet.
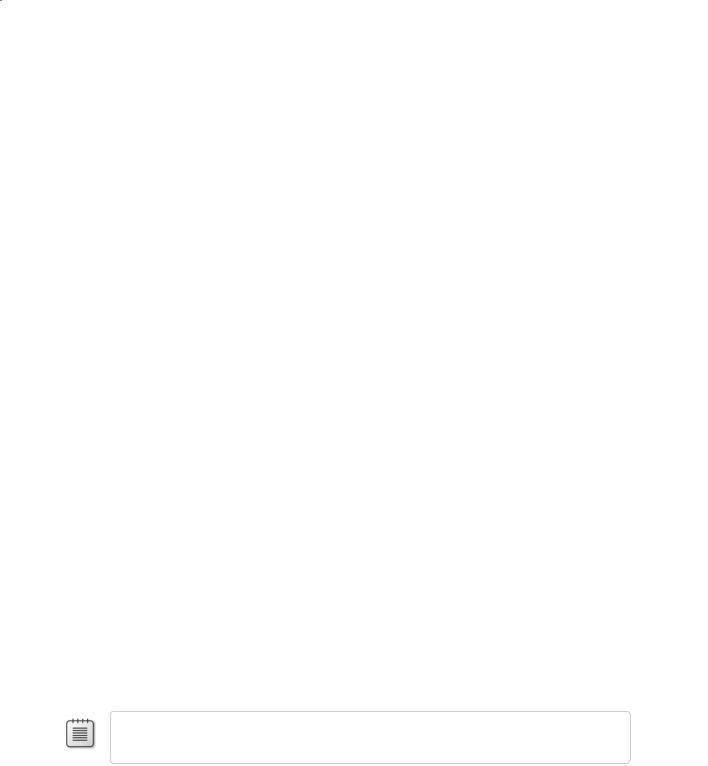
626 |
Part VI Building Web Applications |
In the early 1990s, a number of vendors supplying tools for building distributed systems formed an organization that later became known as the Organization for the Advancement of Structured Information Standards, or OASIS. As the shortcomings of the early Web services infrastructure became apparent, members of OASIS pondered these problems (and other Web services issues) and produced what became known as the WS-Security specification. The WS-Security specification describes how to protect the messages sent by Web services. Vendors that subscribe to WS-Security provide their own implementations that meet this specification, typically by using technologies such as encryption and certificates.
Policy Although the WS-Security specification defines how to provide enhanced security, developers still need to write code to implement it. Web services created by different developers can often vary in how stringent the security mechanism they have elected to implement is. For example, a Web service might use only a relatively weak form of encryption that can easily be broken. A consumer sending highly confidential information to this Web service would probably insist on a higher level of security. This is one example of policy. Other examples include the quality of service and reliability of the Web service. A Web service could implement varying degrees of security, quality of service, and reliability and charge the client application accordingly. The client application and the Web service can negotiate which level of service to use based on the requirements and cost. However, this negotiation requires that the client and the Web service have a common understanding of the policies available. The WS-Policy specification provides a general-purpose model and corresponding syntax to describe and communicate the policies that a Web service implements.
Routing and addressing It is useful for a Web server to be able to reroute a Web service request to one of a number of computers hosting instances of the service. For example, many scalable systems make use of load balancing, in which requests sent to a Web server are actually redirected by that server to other computers to spread the load across those computers. The server can use any number of algorithms to try to balance the load. The important point is that this redirection is transparent to the client making the Web service request, and the server that ultimately handles the request must know where to send any responses that it generates. Redirecting Web service requests is also useful if an administrator needs to shut down a computer to perform maintenance. Requests that would otherwise have been sent to this computer can be rerouted to one of its peers. The WS-Addressing specification describes a framework for routing Web service requests.
Note Developers refer to the WS-Security, WS-Policy, WS-Addressing, and other
WS-specifications collectively as the WS-* specifications.