
- •Contents at a Glance
- •Table of Contents
- •Acknowledgments
- •Introduction
- •Who This Book Is For
- •Finding Your Best Starting Point in This Book
- •Conventions and Features in This Book
- •Conventions
- •Other Features
- •System Requirements
- •Code Samples
- •Installing the Code Samples
- •Using the Code Samples
- •Support for This Book
- •Questions and Comments
- •Beginning Programming with the Visual Studio 2008 Environment
- •Writing Your First Program
- •Using Namespaces
- •Creating a Graphical Application
- •Chapter 1 Quick Reference
- •Understanding Statements
- •Identifying Keywords
- •Using Variables
- •Naming Variables
- •Declaring Variables
- •Working with Primitive Data Types
- •Displaying Primitive Data Type Values
- •Using Arithmetic Operators
- •Operators and Types
- •Examining Arithmetic Operators
- •Controlling Precedence
- •Using Associativity to Evaluate Expressions
- •Associativity and the Assignment Operator
- •Incrementing and Decrementing Variables
- •Declaring Implicitly Typed Local Variables
- •Chapter 2 Quick Reference
- •Declaring Methods
- •Specifying the Method Declaration Syntax
- •Writing return Statements
- •Calling Methods
- •Specifying the Method Call Syntax
- •Applying Scope
- •Overloading Methods
- •Writing Methods
- •Chapter 3 Quick Reference
- •Declaring Boolean Variables
- •Using Boolean Operators
- •Understanding Equality and Relational Operators
- •Understanding Conditional Logical Operators
- •Summarizing Operator Precedence and Associativity
- •Using if Statements to Make Decisions
- •Understanding if Statement Syntax
- •Using Blocks to Group Statements
- •Cascading if Statements
- •Using switch Statements
- •Understanding switch Statement Syntax
- •Following the switch Statement Rules
- •Chapter 4 Quick Reference
- •Using Compound Assignment Operators
- •Writing while Statements
- •Writing for Statements
- •Understanding for Statement Scope
- •Writing do Statements
- •Chapter 5 Quick Reference
- •Coping with Errors
- •Trying Code and Catching Exceptions
- •Handling an Exception
- •Using Multiple catch Handlers
- •Catching Multiple Exceptions
- •Using Checked and Unchecked Integer Arithmetic
- •Writing Checked Statements
- •Writing Checked Expressions
- •Throwing Exceptions
- •Chapter 6 Quick Reference
- •The Purpose of Encapsulation
- •Controlling Accessibility
- •Working with Constructors
- •Overloading Constructors
- •Understanding static Methods and Data
- •Creating a Shared Field
- •Creating a static Field by Using the const Keyword
- •Chapter 7 Quick Reference
- •Copying Value Type Variables and Classes
- •Understanding Null Values and Nullable Types
- •Using Nullable Types
- •Understanding the Properties of Nullable Types
- •Using ref and out Parameters
- •Creating ref Parameters
- •Creating out Parameters
- •How Computer Memory Is Organized
- •Using the Stack and the Heap
- •The System.Object Class
- •Boxing
- •Unboxing
- •Casting Data Safely
- •The is Operator
- •The as Operator
- •Chapter 8 Quick Reference
- •Working with Enumerations
- •Declaring an Enumeration
- •Using an Enumeration
- •Choosing Enumeration Literal Values
- •Choosing an Enumeration’s Underlying Type
- •Working with Structures
- •Declaring a Structure
- •Understanding Structure and Class Differences
- •Declaring Structure Variables
- •Understanding Structure Initialization
- •Copying Structure Variables
- •Chapter 9 Quick Reference
- •What Is an Array?
- •Declaring Array Variables
- •Creating an Array Instance
- •Initializing Array Variables
- •Creating an Implicitly Typed Array
- •Accessing an Individual Array Element
- •Iterating Through an Array
- •Copying Arrays
- •What Are Collection Classes?
- •The ArrayList Collection Class
- •The Queue Collection Class
- •The Stack Collection Class
- •The Hashtable Collection Class
- •The SortedList Collection Class
- •Using Collection Initializers
- •Comparing Arrays and Collections
- •Using Collection Classes to Play Cards
- •Chapter 10 Quick Reference
- •Using Array Arguments
- •Declaring a params Array
- •Using params object[ ]
- •Using a params Array
- •Chapter 11 Quick Reference
- •What Is Inheritance?
- •Using Inheritance
- •Base Classes and Derived Classes
- •Calling Base Class Constructors
- •Assigning Classes
- •Declaring new Methods
- •Declaring Virtual Methods
- •Declaring override Methods
- •Understanding protected Access
- •Understanding Extension Methods
- •Chapter 12 Quick Reference
- •Understanding Interfaces
- •Interface Syntax
- •Interface Restrictions
- •Implementing an Interface
- •Referencing a Class Through Its Interface
- •Working with Multiple Interfaces
- •Abstract Classes
- •Abstract Methods
- •Sealed Classes
- •Sealed Methods
- •Implementing an Extensible Framework
- •Summarizing Keyword Combinations
- •Chapter 13 Quick Reference
- •The Life and Times of an Object
- •Writing Destructors
- •Why Use the Garbage Collector?
- •How Does the Garbage Collector Work?
- •Recommendations
- •Resource Management
- •Disposal Methods
- •Exception-Safe Disposal
- •The using Statement
- •Calling the Dispose Method from a Destructor
- •Making Code Exception-Safe
- •Chapter 14 Quick Reference
- •Implementing Encapsulation by Using Methods
- •What Are Properties?
- •Using Properties
- •Read-Only Properties
- •Write-Only Properties
- •Property Accessibility
- •Understanding the Property Restrictions
- •Declaring Interface Properties
- •Using Properties in a Windows Application
- •Generating Automatic Properties
- •Initializing Objects by Using Properties
- •Chapter 15 Quick Reference
- •What Is an Indexer?
- •An Example That Doesn’t Use Indexers
- •The Same Example Using Indexers
- •Understanding Indexer Accessors
- •Comparing Indexers and Arrays
- •Indexers in Interfaces
- •Using Indexers in a Windows Application
- •Chapter 16 Quick Reference
- •Declaring and Using Delegates
- •The Automated Factory Scenario
- •Implementing the Factory Without Using Delegates
- •Implementing the Factory by Using a Delegate
- •Using Delegates
- •Lambda Expressions and Delegates
- •Creating a Method Adapter
- •Using a Lambda Expression as an Adapter
- •The Form of Lambda Expressions
- •Declaring an Event
- •Subscribing to an Event
- •Unsubscribing from an Event
- •Raising an Event
- •Understanding WPF User Interface Events
- •Using Events
- •Chapter 17 Quick Reference
- •The Problem with objects
- •The Generics Solution
- •Generics vs. Generalized Classes
- •Generics and Constraints
- •Creating a Generic Class
- •The Theory of Binary Trees
- •Building a Binary Tree Class by Using Generics
- •Creating a Generic Method
- •Chapter 18 Quick Reference
- •Enumerating the Elements in a Collection
- •Manually Implementing an Enumerator
- •Implementing the IEnumerable Interface
- •Implementing an Enumerator by Using an Iterator
- •A Simple Iterator
- •Chapter 19 Quick Reference
- •What Is Language Integrated Query (LINQ)?
- •Using LINQ in a C# Application
- •Selecting Data
- •Filtering Data
- •Ordering, Grouping, and Aggregating Data
- •Joining Data
- •Using Query Operators
- •Querying Data in Tree<TItem> Objects
- •LINQ and Deferred Evaluation
- •Chapter 20 Quick Reference
- •Understanding Operators
- •Operator Constraints
- •Overloaded Operators
- •Creating Symmetric Operators
- •Understanding Compound Assignment
- •Declaring Increment and Decrement Operators
- •Implementing an Operator
- •Understanding Conversion Operators
- •Providing Built-In Conversions
- •Creating Symmetric Operators, Revisited
- •Adding an Implicit Conversion Operator
- •Chapter 21 Quick Reference
- •Creating a WPF Application
- •Creating a Windows Presentation Foundation Application
- •Adding Controls to the Form
- •Using WPF Controls
- •Changing Properties Dynamically
- •Handling Events in a WPF Form
- •Processing Events in Windows Forms
- •Chapter 22 Quick Reference
- •Menu Guidelines and Style
- •Menus and Menu Events
- •Creating a Menu
- •Handling Menu Events
- •Shortcut Menus
- •Creating Shortcut Menus
- •Windows Common Dialog Boxes
- •Using the SaveFileDialog Class
- •Chapter 23 Quick Reference
- •Validating Data
- •Strategies for Validating User Input
- •An Example—Customer Information Maintenance
- •Performing Validation by Using Data Binding
- •Changing the Point at Which Validation Occurs
- •Chapter 24 Quick Reference
- •Querying a Database by Using ADO.NET
- •The Northwind Database
- •Creating the Database
- •Using ADO.NET to Query Order Information
- •Querying a Database by Using DLINQ
- •Creating and Running a DLINQ Query
- •Deferred and Immediate Fetching
- •Joining Tables and Creating Relationships
- •Deferred and Immediate Fetching Revisited
- •Using DLINQ to Query Order Information
- •Chapter 25 Quick Reference
- •Using Data Binding with DLINQ
- •Using DLINQ to Modify Data
- •Updating Existing Data
- •Adding and Deleting Data
- •Chapter 26 Quick Reference
- •Understanding the Internet as an Infrastructure
- •Understanding Web Server Requests and Responses
- •Managing State
- •Understanding ASP.NET
- •Creating Web Applications with ASP.NET
- •Building an ASP.NET Application
- •Understanding Server Controls
- •Creating and Using a Theme
- •Chapter 27 Quick Reference
- •Comparing Server and Client Validations
- •Validating Data at the Web Server
- •Validating Data in the Web Browser
- •Implementing Client Validation
- •Chapter 28 Quick Reference
- •Managing Security
- •Understanding Forms-Based Security
- •Implementing Forms-Based Security
- •Querying and Displaying Data
- •Understanding the Web Forms GridView Control
- •Displaying Customer and Order History Information
- •Paging Data
- •Editing Data
- •Updating Rows Through a GridView Control
- •Navigating Between Forms
- •Chapter 29 Quick Reference
- •What Is a Web Service?
- •The Role of SOAP
- •What Is the Web Services Description Language?
- •Nonfunctional Requirements of Web Services
- •The Role of Windows Communication Foundation
- •Building a Web Service
- •Creating the ProductsService Web Service
- •Web Services, Clients, and Proxies
- •Talking SOAP: The Easy Way
- •Consuming the ProductsService Web Service
- •Chapter 30 Quick Reference
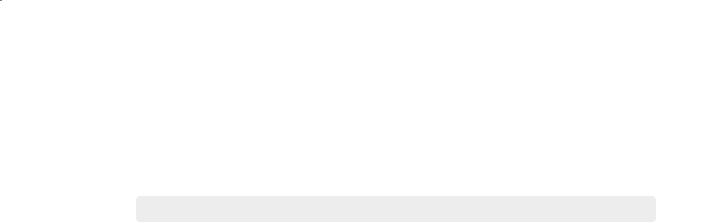
Chapter 22 Introducing Windows Presentation Foundation |
439 |
Changing Properties Dynamically
You have been using the Design View window, the Properties window, and the XAML pane to set properties statically. When the form runs, it would be useful to reset the value of each
control to an initial default value. To do this, you will need to write some code (at last). In the following exercises, you will create a private method called Reset. Later, you will invoke the Reset method when the form first starts as well as when the user clicks the Clear button.
Create the Reset method
1.In the Design View window, right-click the form, and then click View Code. The Code and Text Editor window opens and displays the Window1.xaml.cs file so that you can add C# code to the form.
2.Add the following Reset method, shown in bold type, to the Window1 class:
public partial class Window1 : Window
{
...
public void Reset()
{
firstName.Text = String.Empty; lastName.Text = String.Empty;
}
}
The two statements in this method ensure that the firstName and lastName text boxes are blank by assigning an empty string to their Text property.
You also need to initialize the properties for the remaining controls on the form and populate the towerNames combo box and the methods list box.
If you recall, the towerName combo box will contain a list of all the bell towers in the
Middleshire district. This information would usually be held in a database, and you would write code to retrieve the list of towers and populate the ComboBox. For this example, the application will use a hard-coded collection. A ComboBox has a property called Items that contains a list of the data to be displayed.
3.Add the following string array called towers, shown in bold type, which contains a hard-coded list of tower names, to the Window1 class:
public partial class Window1 : Window
{
private string[] towers = { “Great Shevington”, “Little Mudford”, “Upper Gumtree”, “Downley Hatch” };
...
}

440 |
Part IV Working with Windows Applications |
4.In the Reset method, after the code you have already written, add the following statements shown in bold type to clear the towerNames combo box (this is important
because otherwise you could end up with many duplicate values in the list) and add the towers found in the towers array. The statement after the foreach loop causes the first tower to be displayed as the default value:
public void Reset()
{
...
towerNames.Items.Clear();
foreach (string towerName in towers)
{
towerNames.Items.Add(towerName);
}
towerNames.Text = towerNames.Items[0] as string;
}
Note You can also specify hard-coded values at design time in the XAML description of a combo box, like this:
<ComboBox Text=”towerNames”> <ComboBox.Items>
<ComboBoxItem> Great Shevington
</ComboBoxItem>
<ComboBoxItem> Little Mudford
</ComboBoxItem>
<ComboBoxItem> Upper Gumtree
</ComboBoxItem>
<ComboBoxItem> Downley Hatch
</ComboBoxItem>
</ComboBox.Items>
</ComboBox>
5.You must populate the methods list box with a list of bell-ringing methods. Like a combo box, a list box has a property called Items that contains a collection of values to be displayed. Also, like the ComboBox, it could be populated from a database. However, as before, you will simply supply some hard-coded values for this example. Add the
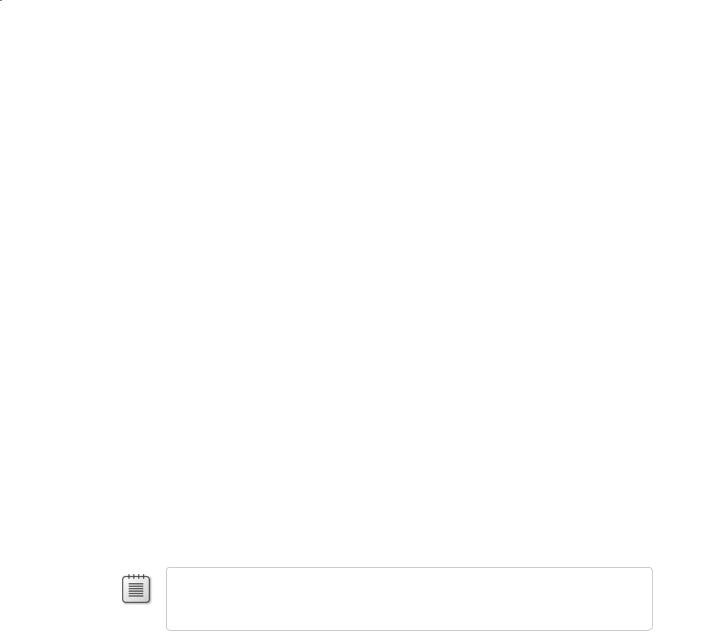
Chapter 22 Introducing Windows Presentation Foundation |
441 |
following string array shown in bold type, which contains the list of methods, to the
Window1 class:
public partial class Window1 : Window
{
...
private string[] ringingMethods = { “Plain Bob”, “Reverse Canterbury”, “Grandsire”, “Stedman”, “Kent Treble Bob”, “Old Oxford Delight”, “Winchendon Place”, “Norwich Surprise”, “Crayford Little Court” };
...
}
6.The methods list box should display a list of check boxes rather than ordinary text strings. With the flexibility of the WPF model, you can specify a variety of different
types of content for controls such as list boxes and combo boxes. Add the following code shown in bold type to the Reset method to fill the methods list box with the methods in the ringingMethods array. Notice that this time each item is a check box. You can specify the text displayed by the check box by setting its Content property, and you can specify the spacing between items in the list by setting the Margin property; this code inserts a spacing of 10 units after each item:
public void Reset()
{
...
methods.Items.Clear(); CheckBox method;
foreach (string methodName in ringingMethods)
{
method = new CheckBox();
method.Margin = new Thickness(0, 0, 0, 10); method.Content = methodName; methods.Items.Add(method);
}
}
Note Most WPF controls have a Content property that you can use to set and read the value displayed by that control. This property is actually an object, so you can set it to
almost any type, as long as it makes sense to display it!
7.The isCaptain check box should default to false. To do this, you need to set the IsChecked property. Add the following statement shown in bold type to the Reset method:
public void Reset()
{
...
isCaptain.IsChecked = false;
}

442 |
Part IV Working with Windows Applications |
8.The form contains four radio buttons that indicate the number of years of bell-ringing experience the member has. A radio button is similar to a CheckBox in that it can contain a true or false value. However, the power of radio buttons increases when you put them together in a GroupBox. In this case, the radio buttons form a mutually exclusive collection—at most, only one radio button in a group can be selected (set to true), and all the others will automatically be cleared (set to false). By default, none of the buttons will be selected. You should rectify this by setting the IsChecked property of the novice radio button. Add the following statement shown in bold type to the Reset method:
public void Reset()
{
...
novice.IsChecked = true;
}
9.You should ensure that the Member Since DateTimePicker control defaults to the current date. You can do this by setting the Value property of the control. You can obtain the current date from the static Today method of the DateTime class.
Add the following code shown in bold type to the Reset method to initialize the
DateTimePicker control.
public void Reset()
{
...
System.Windows.Forms.DateTimePicker memberDate = hostMemberSince.Child as System.Windows.Forms.DateTimePicker;
memberDate.Value = DateTime.Today;
}
Notice that to access an object in a WindowsFormsHost container, you reference the Child property of the container and then cast it to the appropriate type.
Additionally, notice that the DateTimePicker class is defined in the System.Windows. Forms namespace. Typically, you add a using statement for the file defining a class to
bring the namespace of the class into scope, but you should not do this when integrating Windows Forms controls into a WPF application. The reason is that the System. Windows.Forms namespace contains many controls that use the same names as those
in the WPF library, so adding a using statement would make all references to these controls ambiguous!
10.Finally, you need to arrange for the Reset method to be called when the form is first displayed. A good place to do this is in the Window1 constructor. Insert a call to the Reset method after the statement that calls the InitializeComponent method, as shown in bold type here:
public Window1()
{
InitializeComponent();
this.Reset();
}