
- •Preface
- •1.1 Machine Language
- •1.3 The Java Virtual Machine
- •1.4 Building Blocks of Programs
- •1.5 Object-oriented Programming
- •1.6 The Modern User Interface
- •Quiz on Chapter 1
- •2 Names and Things
- •2.1 The Basic Java Application
- •2.2.1 Variables
- •2.2.2 Types and Literals
- •2.2.3 Variables in Programs
- •2.3.2 Operations on Strings
- •2.3.3 Introduction to Enums
- •2.4 Text Input and Output
- •2.4.1 A First Text Input Example
- •2.4.2 Text Output
- •2.4.3 TextIO Input Functions
- •2.4.4 Formatted Output
- •2.4.5 Introduction to File I/O
- •2.5 Details of Expressions
- •2.5.1 Arithmetic Operators
- •2.5.2 Increment and Decrement
- •2.5.3 Relational Operators
- •2.5.4 Boolean Operators
- •2.5.5 Conditional Operator
- •2.5.7 Type Conversion of Strings
- •2.5.8 Precedence Rules
- •2.6 Programming Environments
- •2.6.1 Java Development Kit
- •2.6.2 Command Line Environment
- •2.6.3 IDEs and Eclipse
- •2.6.4 The Problem of Packages
- •Exercises for Chapter 2
- •Quiz on Chapter 2
- •3 Control
- •3.1 Blocks, Loops, and Branches
- •3.1.1 Blocks
- •3.1.2 The Basic While Loop
- •3.1.3 The Basic If Statement
- •3.2 Algorithm Development
- •3.2.2 The 3N+1 Problem
- •3.2.3 Coding, Testing, Debugging
- •3.3.1 The while Statement
- •3.3.2 The do..while Statement
- •3.3.3 break and continue
- •3.4 The for Statement
- •3.4.1 For Loops
- •3.4.2 Example: Counting Divisors
- •3.4.3 Nested for Loops
- •3.5 The if Statement
- •3.5.1 The Dangling else Problem
- •3.5.2 The if...else if Construction
- •3.5.3 If Statement Examples
- •3.5.4 The Empty Statement
- •3.6 The switch Statement
- •3.6.1 The Basic switch Statement
- •3.6.2 Menus and switch Statements
- •3.6.3 Enums in switch Statements
- •3.7.1 Exceptions
- •3.7.2 try..catch
- •3.7.3 Exceptions in TextIO
- •Exercises for Chapter 3
- •Quiz on Chapter 3
- •4 Subroutines
- •4.1 Black Boxes
- •4.2.2 Calling Subroutines
- •4.2.3 Subroutines in Programs
- •4.2.4 Member Variables
- •4.3 Parameters
- •4.3.1 Using Parameters
- •4.3.2 Formal and Actual Parameters
- •4.3.3 Overloading
- •4.3.4 Subroutine Examples
- •4.3.5 Throwing Exceptions
- •4.3.6 Global and Local Variables
- •4.4 Return Values
- •4.4.1 The return statement
- •4.4.2 Function Examples
- •4.4.3 3N+1 Revisited
- •4.5 APIs, Packages, and Javadoc
- •4.5.1 Toolboxes
- •4.5.3 Using Classes from Packages
- •4.5.4 Javadoc
- •4.6 More on Program Design
- •4.6.1 Preconditions and Postconditions
- •4.6.2 A Design Example
- •4.6.3 The Program
- •4.7 The Truth About Declarations
- •4.7.1 Initialization in Declarations
- •4.7.2 Named Constants
- •4.7.3 Naming and Scope Rules
- •Exercises for Chapter 4
- •Quiz on Chapter 4
- •5 Objects and Classes
- •5.1.1 Objects, Classes, and Instances
- •5.1.2 Fundamentals of Objects
- •5.1.3 Getters and Setters
- •5.2 Constructors and Object Initialization
- •5.2.1 Initializing Instance Variables
- •5.2.2 Constructors
- •5.2.3 Garbage Collection
- •5.3 Programming with Objects
- •5.3.2 Wrapper Classes and Autoboxing
- •5.4 Programming Example: Card, Hand, Deck
- •5.4.1 Designing the classes
- •5.4.2 The Card Class
- •5.4.3 Example: A Simple Card Game
- •5.5.1 Extending Existing Classes
- •5.5.2 Inheritance and Class Hierarchy
- •5.5.3 Example: Vehicles
- •5.5.4 Polymorphism
- •5.5.5 Abstract Classes
- •5.6 this and super
- •5.6.1 The Special Variable this
- •5.6.2 The Special Variable super
- •5.6.3 Constructors in Subclasses
- •5.7 Interfaces, Nested Classes, and Other Details
- •5.7.1 Interfaces
- •5.7.2 Nested Classes
- •5.7.3 Anonymous Inner Classes
- •5.7.5 Static Import
- •5.7.6 Enums as Classes
- •Exercises for Chapter 5
- •Quiz on Chapter 5
- •6 Introduction to GUI Programming
- •6.1 The Basic GUI Application
- •6.1.1 JFrame and JPanel
- •6.1.2 Components and Layout
- •6.1.3 Events and Listeners
- •6.2 Applets and HTML
- •6.2.1 JApplet
- •6.2.2 Reusing Your JPanels
- •6.2.3 Basic HTML
- •6.2.4 Applets on Web Pages
- •6.3 Graphics and Painting
- •6.3.1 Coordinates
- •6.3.2 Colors
- •6.3.3 Fonts
- •6.3.4 Shapes
- •6.3.5 Graphics2D
- •6.3.6 An Example
- •6.4 Mouse Events
- •6.4.1 Event Handling
- •6.4.2 MouseEvent and MouseListener
- •6.4.3 Mouse Coordinates
- •6.4.4 MouseMotionListeners and Dragging
- •6.4.5 Anonymous Event Handlers
- •6.5 Timer and Keyboard Events
- •6.5.1 Timers and Animation
- •6.5.2 Keyboard Events
- •6.5.3 Focus Events
- •6.5.4 State Machines
- •6.6 Basic Components
- •6.6.1 JButton
- •6.6.2 JLabel
- •6.6.3 JCheckBox
- •6.6.4 JTextField and JTextArea
- •6.6.5 JComboBox
- •6.6.6 JSlider
- •6.7 Basic Layout
- •6.7.1 Basic Layout Managers
- •6.7.2 Borders
- •6.7.3 SliderAndComboBoxDemo
- •6.7.4 A Simple Calculator
- •6.7.5 Using a null Layout
- •6.7.6 A Little Card Game
- •6.8 Menus and Dialogs
- •6.8.1 Menus and Menubars
- •6.8.2 Dialogs
- •6.8.3 Fine Points of Frames
- •6.8.4 Creating Jar Files
- •Exercises for Chapter 6
- •Quiz on Chapter 6
- •7 Arrays
- •7.1 Creating and Using Arrays
- •7.1.1 Arrays
- •7.1.2 Using Arrays
- •7.1.3 Array Initialization
- •7.2 Programming With Arrays
- •7.2.1 Arrays and for Loops
- •7.2.3 Array Types in Subroutines
- •7.2.4 Random Access
- •7.2.5 Arrays of Objects
- •7.2.6 Variable Arity Methods
- •7.3 Dynamic Arrays and ArrayLists
- •7.3.1 Partially Full Arrays
- •7.3.2 Dynamic Arrays
- •7.3.3 ArrrayLists
- •7.3.4 Parameterized Types
- •7.3.5 Vectors
- •7.4 Searching and Sorting
- •7.4.1 Searching
- •7.4.2 Association Lists
- •7.4.3 Insertion Sort
- •7.4.4 Selection Sort
- •7.4.5 Unsorting
- •7.5.3 Example: Checkers
- •Exercises for Chapter 7
- •Quiz on Chapter 7
- •8 Correctness and Robustness
- •8.1 Introduction to Correctness and Robustness
- •8.1.1 Horror Stories
- •8.1.2 Java to the Rescue
- •8.1.3 Problems Remain in Java
- •8.2 Writing Correct Programs
- •8.2.1 Provably Correct Programs
- •8.2.2 Robust Handling of Input
- •8.3 Exceptions and try..catch
- •8.3.1 Exceptions and Exception Classes
- •8.3.2 The try Statement
- •8.3.3 Throwing Exceptions
- •8.3.4 Mandatory Exception Handling
- •8.3.5 Programming with Exceptions
- •8.4 Assertions
- •8.5 Introduction to Threads
- •8.5.1 Creating and Running Threads
- •8.5.2 Operations on Threads
- •8.5.4 Wait and Notify
- •8.5.5 Volatile Variables
- •8.6 Analysis of Algorithms
- •Exercises for Chapter 8
- •Quiz on Chapter 8
- •9.1 Recursion
- •9.1.1 Recursive Binary Search
- •9.1.2 Towers of Hanoi
- •9.1.3 A Recursive Sorting Algorithm
- •9.1.4 Blob Counting
- •9.2 Linked Data Structures
- •9.2.1 Recursive Linking
- •9.2.2 Linked Lists
- •9.2.3 Basic Linked List Processing
- •9.2.4 Inserting into a Linked List
- •9.2.5 Deleting from a Linked List
- •9.3 Stacks, Queues, and ADTs
- •9.3.1 Stacks
- •9.3.2 Queues
- •9.4 Binary Trees
- •9.4.1 Tree Traversal
- •9.4.2 Binary Sort Trees
- •9.4.3 Expression Trees
- •9.5 A Simple Recursive Descent Parser
- •9.5.1 Backus-Naur Form
- •9.5.2 Recursive Descent Parsing
- •9.5.3 Building an Expression Tree
- •Exercises for Chapter 9
- •Quiz on Chapter 9
- •10.1 Generic Programming
- •10.1.1 Generic Programming in Smalltalk
- •10.1.2 Generic Programming in C++
- •10.1.3 Generic Programming in Java
- •10.1.4 The Java Collection Framework
- •10.1.6 Equality and Comparison
- •10.1.7 Generics and Wrapper Classes
- •10.2 Lists and Sets
- •10.2.1 ArrayList and LinkedList
- •10.2.2 Sorting
- •10.2.3 TreeSet and HashSet
- •10.2.4 EnumSet
- •10.3 Maps
- •10.3.1 The Map Interface
- •10.3.2 Views, SubSets, and SubMaps
- •10.3.3 Hash Tables and Hash Codes
- •10.4 Programming with the Collection Framework
- •10.4.1 Symbol Tables
- •10.4.2 Sets Inside a Map
- •10.4.3 Using a Comparator
- •10.4.4 Word Counting
- •10.5 Writing Generic Classes and Methods
- •10.5.1 Simple Generic Classes
- •10.5.2 Simple Generic Methods
- •10.5.3 Type Wildcards
- •10.5.4 Bounded Types
- •Exercises for Chapter 10
- •Quiz on Chapter 10
- •11 Files and Networking
- •11.1 Streams, Readers, and Writers
- •11.1.1 Character and Byte Streams
- •11.1.2 PrintWriter
- •11.1.3 Data Streams
- •11.1.4 Reading Text
- •11.1.5 The Scanner Class
- •11.1.6 Serialized Object I/O
- •11.2 Files
- •11.2.1 Reading and Writing Files
- •11.2.2 Files and Directories
- •11.2.3 File Dialog Boxes
- •11.3 Programming With Files
- •11.3.1 Copying a File
- •11.3.2 Persistent Data
- •11.3.3 Files in GUI Programs
- •11.3.4 Storing Objects in Files
- •11.4 Networking
- •11.4.1 URLs and URLConnections
- •11.4.2 TCP/IP and Client/Server
- •11.4.3 Sockets
- •11.4.4 A Trivial Client/Server
- •11.4.5 A Simple Network Chat
- •11.5 Network Programming and Threads
- •11.5.1 A Threaded GUI Chat Program.
- •11.5.2 A Multithreaded Server
- •11.5.3 Distributed Computing
- •11.6 A Brief Introduction to XML
- •11.6.1 Basic XML Syntax
- •11.6.2 XMLEncoder and XMLDecoder
- •11.6.3 Working With the DOM
- •Exercises for Chapter 11
- •Quiz on Chapter 11
- •12 Advanced GUI Programming
- •12.1 Images and Resources
- •12.1.2 Working With Pixels
- •12.1.3 Resources
- •12.1.4 Cursors and Icons
- •12.1.5 Image File I/O
- •12.2 Fancier Graphics
- •12.2.1 Measuring Text
- •12.2.2 Transparency
- •12.2.3 Antialiasing
- •12.2.4 Strokes and Paints
- •12.2.5 Transforms
- •12.3 Actions and Buttons
- •12.3.1 Action and AbstractAction
- •12.3.2 Icons on Buttons
- •12.3.3 Radio Buttons
- •12.3.4 Toolbars
- •12.3.5 Keyboard Accelerators
- •12.3.6 HTML on Buttons
- •12.4 Complex Components and MVC
- •12.4.1 Model-View-Controller
- •12.4.2 Lists and ListModels
- •12.4.3 Tables and TableModels
- •12.4.4 Documents and Editors
- •12.4.5 Custom Components
- •12.5 Finishing Touches
- •12.5.1 The Mandelbrot Set
- •12.5.2 Design of the Program
- •12.5.3 Internationalization
- •12.5.4 Events, Events, Events
- •12.5.5 Custom Dialogs
- •12.5.6 Preferences
- •Exercises for Chapter 12
- •Quiz on Chapter 12
- •Appendix: Source Files

CHAPTER 12. ADVANCED GUI PROGRAMMING |
635 |
g.setColor(Color.DARK GRAY); g.fillRect(0,0,30,30); g.setColor(c);
g.fill3DRect(3, 3, 24, 24, false); g.dispose();
Icon selectedIcon = new ImageIcon(image);
/* Create and configure the button. */
JRadioButton button = new JRadioButton(unselectedIcon); button.setSelectedIcon(selectedIcon); button.addActionListener( new ActionListener() {
public void actionPerformed(ActionEvent e) {
//The action for this button sets the current drawing color
//in the display to c.
display.setCurrentColor(c);
}
});
grp.add(button); if (selected)
button.setSelected(true);
return button;
} // end makeColorRadioButton
It is possible to create radio buttons and check boxes from Actions. The button takes its name, main icon, tooltip text, and enabled/disabled state from the action. However, in Java 5.0, an action has no property corresponding to the selected/unselected state. This means that you can’t check or set the selection state through the action. In Java 6.0, the action API is considerably improved, and among the changes is support for selection state.
12.3.4Toolbars
It has become increasingly common for programs to have a row of small buttons along the top or side of the program window that o er access to some of the commonly used features of the program. The row of buttons is known as a tool bar . Typically, the buttons in a tool bar are presented as small icons, with no text. Tool bars can also contain other components, such as
JTextFields and JLabels.
In Swing, tool bars are represented by the class JToolBar. A JToolBar is a container that can hold other components. It is also itself a component, and so can be added to other containers. In general, the parent component of the tool bar should use a BorderLayout. The tool bar should occupy one of the edge positions—NORTH, SOUTH, EAST, or WEST—in the BorderLayout. Furthermore, the other three edge positions should be empty. The reason for this is that it might be possible (depending on the platform and configuration) for the user to drag the tool bar from one edge position in the parent container to another. It might even be possible for the user to drag the toolbar o its parent entirely, so that it becomes a separate window.
Here is a picture of a tool bar from the sample program ToolBarDemo.java.
CHAPTER 12. ADVANCED GUI PROGRAMMING |
636 |
In this program, the user can draw colored curves in a large drawing area. The first three buttons in the tool bar are a set of radio buttons that control the drawing color. The fourth button is a push button that the user can click to clear the drawing.
Tool bars are easy to use. You just have to create the JToolBar object, add it to a container, and add some buttons and possibly other components to the tool bar. One fine point is adding space to a tool bar, such as the gap between the radio buttons and the push button in the sample program. You can leave a gap by adding a separator to the tool bar. For example:
toolbar.addSeparator(new Dimension(20,20));
This adds an invisible 20-by-20 pixel block to the tool bar. This will appear as a 20 pixel gap between components.
Here is the constructor from the ToolBarDemo program. It shows how to create the tool bar and place it in a container. Note that class ToolBarDemo is a subclass of JPanel, and the tool bar and display are added to the panel object that is being constructed:
public ToolBarDemo() {
setLayout(new BorderLayout(2,2)); setBackground(Color.GRAY); setBorder(BorderFactory.createLineBorder(Color.GRAY,2));
display = new Display(); add(display, BorderLayout.CENTER);
JToolBar toolbar = new JToolBar(); add(toolbar, BorderLayout.NORTH);
ButtonGroup group = new ButtonGroup();
toolbar.add( makeColorRadioButton(Color.RED,group,true) ); toolbar.add( makeColorRadioButton(Color.GREEN,group,false) ); toolbar.add( makeColorRadioButton(Color.BLUE,group,false) ); toolbar.addSeparator(new Dimension(20,20));
toolbar.add( makeClearButton() );
}
Note that the gray outline of the tool bar comes from two sources: The line at the bottom shows the background color of the main panel, which is visible because the BorderLayout that is used on that panel has vertical and horizontal gaps of 2 pixels. The other three sides are part of the border of the main panel.
If you want a vertical tool bar that can be placed in the EAST or WEST position of a BorderLayout, you should specify the orientation in the tool bar’s constructor:
JToolBar toolbar = new JToolBar( JToolBar.VERTICAL );
The default orientation is JToolBar.HORIZONTAL. The orientation is adjusted automatically when the user drags the toolbar into a new position. If you want to prevent the user from dragging the toolbar, just say toolbar.setFloatable(false).
12.3.5Keyboard Accelerators
In most programs, commonly used menu commands have keyboard equivalents. The user can type the keyboard equivalent instead of selecting the command from the menu, and the result will be exactly the same. Typically, for example, the “Save” command has keyboard

CHAPTER 12. ADVANCED GUI PROGRAMMING |
637 |
equivalent CONTROL-S, and the “Undo” command corresponds to CONTROL-Z. (Under Mac OS, the keyboard equivalents for these commands would probably be META-C and META-Z, where META refers to holding down the “apple” key.) The keyboard equivalents for menu commands are referred to as accelerators.
The class javax.swing.KeyStroke is used to represent key strokes that the user can type on the keyboard. A key stroke consists of pressing a key, possibly while holding down one or more of the modifier keys control, shift, alt, and meta. The KeyStroke class has a static method, getKeyStroke(String), that makes it easy to create key stroke objects. For example,
KeyStroke.getKeyStroke( "ctrl S" )
returns a KeyStroke that represents the action of pressing the “S” key while holding down the control key. In addition to “ctrl”, you can use the modifiers “shift”, “alt”, and “meta” in the string that describes the key stroke. You can even combine several modifiers, so that
KeyStroke.getKeyStroke( "ctrl shift Z" )
represents the action of pressing the “Z” key while holding down both the control and the shift keys. When the key stroke involves pressing a character key, the character must appear in the string in upper case form. You can also have key strokes that correspond to non-character keys. The number keys can be referred to as “1”, “2”, etc., while certain special keys have names such as “F1”, “ENTER”, and “LEFT” (for the left arrow key). The class KeyEvent defines many constants such as VK ENTER, VK LEFT, and VK S. The names that are used for keys in the keystroke description are just these constants with the leading “VK ” removed.
There are at least two ways to associate a keyboard accelerator with a menu item. One is to use the setAccelerator() method of the menu item object:
JMenuItem saveCommand = new JMenuItem( "Save..." ); saveCommand.setAccelerator( KeyStroke.getKeyStroke("ctrl S") );
The other technique can be used if the menu item is created from an Action. The action property Action.ACCELERATOR KEY can be used to associate a KeyStroke with an Action. When a menu item is created from the action, the keyboard accelerator for the menu item is taken from the value of this property. For example, if redoAction is an Action representing a “Redo” action, then you might say:
redoAction.putValue( Action.ACCELERATOR KEY, KeyStroke.getKeyStroke("ctrl shift Z") );
JMenuItem redoCommand = new JMenuItem( redoAction );
or, alternatively, you could simply add the action to a JMenu, editMenu, with editMenu.add(redoAction). (Note, by the way, that accelerators apply only to menu items, not to push buttons. When you create a JButton from an action, the ACCELERATOR KEY property of the action is ignored.)
Note that you can use accelerators for JCheckBoxMenuItems and JRadioButtonMenuItems, as well as for simple JMenuItems.
For an example of using keyboard accelerators, see the solution to Exercise 12.2.
By the way, as noted above, in the Macintosh operating system, the meta (or apple) key is usually used for keyboard accelerators instead of the control key. If you would like to make your program more Mac-friendly, you can test whether your program is running under Mac OS and, if so, adapt your accelerators to the Mac OS style. The recommended way to detect Mac
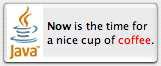
CHAPTER 12. ADVANCED GUI PROGRAMMING |
638 |
OS is to test the value of System.getProperty("mrj.version"). This function call happens to return a non-null value under Mac OS but returns null under other operating systems. For example, here is a simple utility routine for making Mac-friendly accelerators:
/**
*Create a KeyStroke that uses the meta key on Mac OS and
*the control key on other operating systems.
*@param description a string that describes the keystroke,
*without the "meta" or "ctrl"; for example, "S" or
*"shift Z" or "alt F1"
*@return a keystroke created from the description string
*with either "ctrl " or "meta " prepended
*/
private static KeyStroke makeAccelerator(String description) { String commandKey;
if ( System.getProperty("mrj.version") == null ) commandKey = "ctrl";
else
commandKey = "meta";
return KeyStroke.getKeyStroke( commandKey + " " + description );
}
12.3.6HTML on Buttons
As a final stop in this brief tour of ways to spi up your buttons, I’ll mention the fact that the text that is displayed on a button can be specified in HTML format. HTML is the markup language that is used to write web pages. A brief introduction to HTML can be found in Subsection 6.2.3. HTML allows you to apply color or italics or other styles to just part of the text on your buttons. It also makes it possible to have buttons that display multiple lines of text. (You can also use HTML on JLabels, which can be even more useful.) Here’s a picture of a button with HTML text (along with a “Java” icon):
If the string of text that is applied to a button starts with “<html>”, then the string is interpreted as HTML. The string does not have to use strict HTML format; for example, you don’t need a closing </html> at the end of the string. To get multi-line text, use <br> in the string to represent line breaks. If you would like the lines of text to be center justified, include the entire text (except for the <html>) between <center> and </center>. For example,
JButton button = new JButton(
"<html><center>This button has<br>two lines of text</center>" );
creates a button that displays two centered lines of text. You can apply italics to part of the string by enclosing that part between <i> and </i>. Similarly, use <b>...</b> for bold text and <u>...</u> for underlined text. For green text, enclose the text between <font color=green> and </font >. You can, of course, use other colors in place of “green.” The “Java” button that is shown above was created using: