
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary
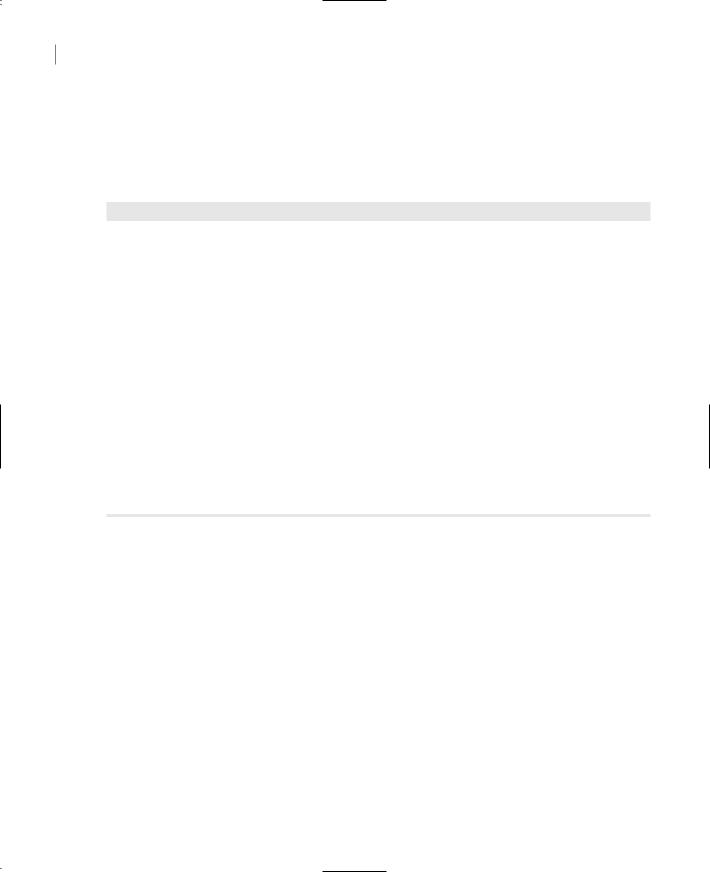
472 Chapter 10 AUTOMATING MICROSOFT OFFICE APPLICATIONS
The rest of the code is straightforward. When an item on the ListView control is clicked, the program recalls the selected item and displays its basic entries in the corresponding Label controls at the bottom of the screen and its body in the TextBox control (whose ReadOnly property must be set to True to prevent editing of the message). Listing 10.17 is the ListView control’s SelectedIndexChanged event handler.
Listing 10.17: Viewing a Message Item
Private Sub ListView1_SelectedIndexChanged(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles ListView1.SelectedIndexChanged
Dim selID As String
If ListView1.SelectedItems.Count() = 0 Then Exit Sub txtMessage.Text = “”
selID = ListView1.SelectedItems(0).SubItems(2).Text Dim mssg As Outlook.MailItem
mssg = OLObjects.GetItemFromID(selID) txtMessage.Text = mssg.Body lblSubject.Text = mssg.Subject lblSender.Text = mssg.SenderName
lblSentOn.Text = mssg.SentOn.ToShortDateString lblRecvdOn.Text = mssg.ReceivedTime.ToShortDateString Dim i As Integer
lstAttachments.Items.Clear()
For i = 1 To mssg.Attachments.Count lstAttachments.Items.Add(mssg.Attachments.Item(i).FileName)
Next End Sub
Open the Messages project in the Visual Basic IDE to examine its code and see how it combines the items of the Contacts folder and uses them to retrieve mail items from the Inbox folder. You can modify the code to add more selection criteria or to work with different folders (for example, the Outbox folder or a subfolder under the Inbox folder).
Warning The Messages project uses the FullName property of the contacts to display the names of possible message senders. If the names you’ve used in the Contacts folder are not the same as the sender names in the incoming messages, then the program won’t select all the messages as you’d expect. There are many methods for matching contacts and messages, but they require additional effort. For example, you can use each contact’s e-mail address, which is the same in both the Contacts and Inbox folders. However, contacts may have multiple e-mail addresses, so you must make sure you search the mail items for all e-mail addresses (aliases) of the selected contact.
Recursive Scanning of the Contacts Folder
The Contacts project of the previous section assumes that all contacts are stored in the Contacts folder (likewise, the other projects assume that all messages reside in a single folder). This may be
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
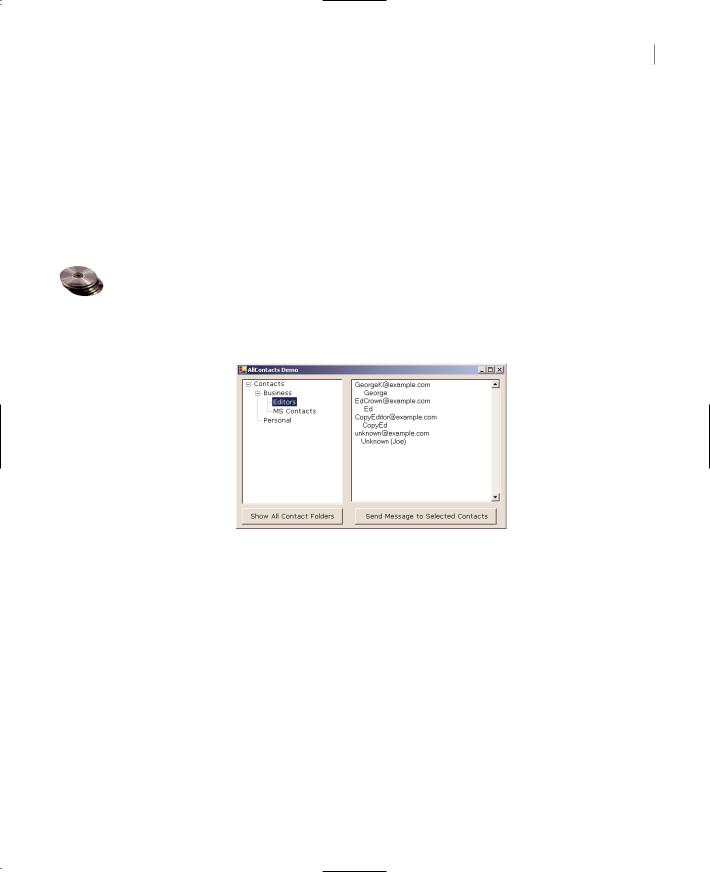
PROGRAMMING OUTLOOK 473
the case for users with a small number of contacts (or totally unorganized users), but it’s not common. Most users organize their contacts in subfolders to better classify them and simplify searching. Scanning a Contacts folder with subfolders is not as simple. This operation calls for recursive programming. If you thought that the chapter on recursive programming was uncalled for in an introductory book, this is another attestation to its usefulness. The topic of recursive programming is discussed in detail in Chapter 18. In this chapter, I’ll explain the code as we go along, but if you’re totally unfamiliar with this programming technique, you should read the material on recursion first and you’ll find it easier to understand the code of this section.
VB.NET at Work: The AllContacts Project
The application that demonstrates how to recursively scan the Contacts folder is called AllContacts and can be found in this chapter’s folder on the CD. The TreeView control with the names of all subfolders under the Contacts folder is populated when the form is loaded (see Figure 10.9). Expand the various folders on the TreeView control, and click a folder’s name to see its contact items in the ListBox control on the right.
Figure 10.9
Populating the TreeView control with the names of the subfolders
The Project’s Code
Let’s start with the trivial code. First, declare the following object variables, which are used by most procedures:
Dim OutlookApp As New Outlook.Application()
Dim OlObjects As Outlook.Namespace
Dim OlContacts As Outlook.MAPIFolder
Then, in the Show All Contact Folders button’s Click event handler, enter the statements of Listing 10.18 to instantiate the OlObjects and OlContacts variables, needed to access the folders of Outlook. This code adds the root node to the TreeView control—the root node being the Contacts folder—and all the first-level subfolders under it. Each time a new subfolder is added to the TreeView control, the code calls the ScanSubFolders() subroutine, passing the current folder as argument. The ScanSubFolders() subroutine iterates through the subfolders of the folder passed as argument and adds them to the TreeView control, under the appropriate node.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
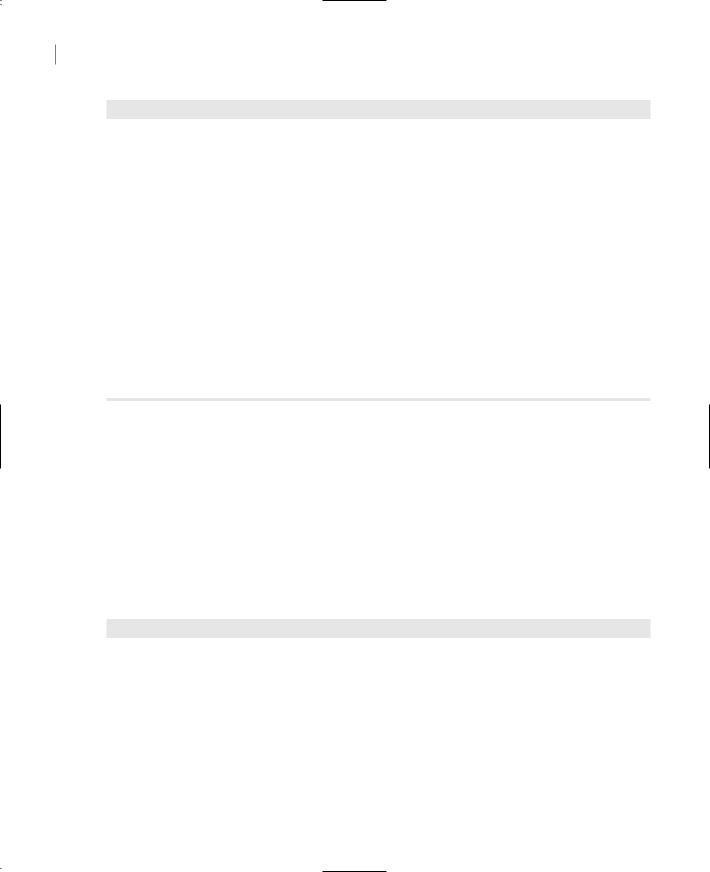
474 Chapter 10 AUTOMATING MICROSOFT OFFICE APPLICATIONS
Listing 10.18: Scanning the Subfolders of the Contact Folder
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnShowContacts.Click OlObjects = OutlookApp.GetNamespace(“MAPI”)
OlContacts = _ OlObjects.GetDefaultFolder(Outlook.OlDefaultFolders.olFolderContacts)
Dim rootNode, newNode As TreeNode rootNode = TreeView1.Nodes.Add(“Contacts”) rootNode.Tag = OlContacts.EntryID
Dim allFolders As Outlook.Folders Dim folder As Outlook.MAPIFolder allFolders = OlContacts.Folders folder = allFolders.GetFirst While Not folder Is Nothing
newNode = rootNode.Nodes.Add(folder.Name) ScanSubFolders(folder, newNode)
folder = allFolders.GetNext End While
End Sub
Later in our application, we want to be able to retrieve the contacts in any folder, when the user selects the folder. The folder’s name is not enough, because it doesn’t uniquely identify a folder. All folders have a unique ID, which you can retrieve with the EntryID property. To have this information handy later in code, we store the ID of each folder to the corresponding node’s Tag property.
The ScanSubFolders() subroutine (Listing 10.19) iterates through all the subfolders of the folder passed as argument. If any of these subfolders have subfolders of their own, the program calls the ScanSubFolders() subroutine again to scan them. This process is repeated recursively, until the initial folder has been scanned to any depth necessary. At each iteration, the code adds a new folder to the TreeView control and sets the node’s Tag property to the ID of the folder. This ID will be extracted later from the Tag property of the selected node and used to retrieve the corresponding folder.
Listing 10.19: The ScanSubFolders() Subroutine
Private Sub ScanSubFolders(ByVal currentFolder As outlook.MAPIFolder, _ ByVal currentNode As TreeNode)
Dim subfolders As Outlook.Folders subfolders = currentFolder.Folders
Dim parentNode As TreeNode = currentNode Dim newNode As TreeNode
If subfolders.Count > 0 Then Dim strFolderKey As String
Dim subFolder As Outlook.MAPIFolder subFolder = subfolders.GetFirst While Not subFolder Is Nothing
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
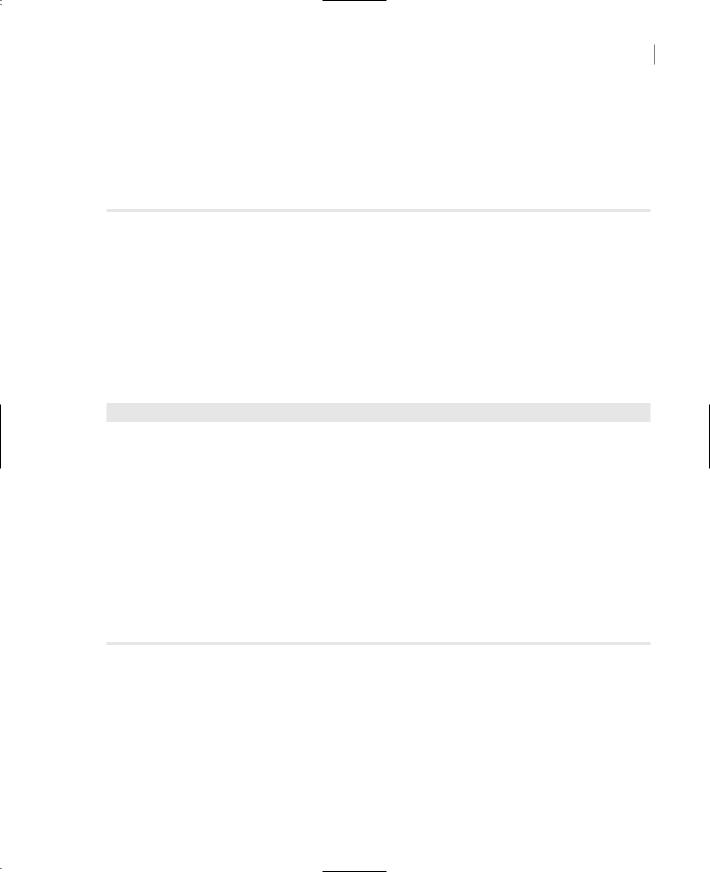
PROGRAMMING OUTLOOK 475
newNode = parentNode.Nodes.Add(subFolder.Name) newNode.Tag = subFolder.EntryID ScanSubFolders(subFolder, newNode)
subFolder = subfolders.GetNext End While
End If End Sub
Viewing a Folder’s Contacts
After populating the TreeView control with the structure of the subfolders under the Contacts folder, you can select a folder in the TreeView control with the mouse to display its contacts on the ListBox control at the right side of the form. When an item in the TreeView control is clicked, the AfterSelect event is triggered; the code for this is presented in Listing 10.20. This event reports the node clicked, and you can use the event’s argument to retrieve the node’s tag, which is the ID of the selected folder. Once you know the ID of the selected folder, you can create a reference to this folder (variable selFolder) and use it to scan the contact items in the actual folder.
Listing 10.20: Listing the Items of the Selected Folder
Private Sub TreeView1_AfterSelect(ByVal sender As System.Object, _ ByVal e As System.Windows.Forms.TreeViewEventArgs) _ Handles TreeView1.AfterSelect
If e.Node.Tag Is Nothing Then Exit Sub Dim folderid As String = e.Node.Tag Dim selFolder As Outlook.MAPIFolder
selFolder = OlObjects.GetFolderFromID(folderid) Dim itm As Integer
ListBox1.Items.Clear()
For itm = 1 To selFolder.Items.Count ListBox1.Items.Add(selFolder.Items.Item(itm).Email1Address) ListBox1.Items.Add(vbTab & selFolder.Items.Item(itm).FullName)
Next End Sub
The code displays only the contact’s name and e-mail address. You can modify the code to display any fields. For example, you can retrieve the contact’s e-mail address and send a message to all the contacts in a specific folder, as we’ll do in the last example of the chapter.
Automated Messages
The Send Message to Selected Contacts button demonstrates how to send a message once the user has picked one or more recipients. The message’s subject and body are hard-coded in this project, but you can easily modify the application so that it reads the message’s body from a text file. You
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |