
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary

ENVIRONMENT OPTIONS 25
generated by the compiler, and you need not understand them at this point. If the Output window is not visible, select the View Other Windows Output command from the menu.
You can also send output to this window from within your code with the Console.WriteLine method. Actually, this is a widely used debugging technique—to print the values of certain variables before entering a problematic area of the code. As you will learn in Chapter 17, there are more elaborate tools to help you debug your application, but printing a few values to the Output window is a time-honored practice in programming with VB to test a function or display the results of intermediate calculations.
In many of the examples of this book, especially in the first few chapters, I use the Console.WriteLine statement to print something to the Output window. To demonstrate the use of the DateDiff() function, for example, I’ll use a statement like the following:
Console.WriteLine(DateDiff(DateInterval.Day, #3/9/2001#, #5/15/2001#))
When this statement is executed, the value 67 will appear in the Output Window. This statement demonstrates the syntax of the DateDiff() function, which returns the difference between the two dates in days.
The Command Window
While testing a program, you can interrupt its execution by inserting a breakpoint. When the breakpoint is reached, the program’s execution is suspended and you can execute a statement in the Command window. Any statement that can appear in your VB code can also be executed in the Command window.
The Task List Window
This window is usually populated by the compiler with error messages, if the code can’t be successfully compiled. You can double-click an error message in this window, and the IDE will take you to the line with the statement in error—which you should fix.
You can also add your own tasks to this window. Just click the first empty line and start typing. A task can be anything, from comments and reminders, to URLs to interesting sites. If you add tasks to the list, you’re responsible for removing them. Errors are removed automatically as soon as you fix the statement that caused them.
Environment Options
The Visual Studio IDE is highly customizable. I will not discuss all the customization options here, but I will show you how to change the default settings of the IDE. Open the Tools menu and select Options (the last item in the menu). The Options dialog box will appear, where you can set all the options regarding the environment. Figure 1.15 shows the options for the font of the various items of the IDE. Here you can set the font for various categories of items, like the Text Editor, the dialogs and toolboxes, and so on. Select an item in the Show Settings For list and then set the font for this item in the box below.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
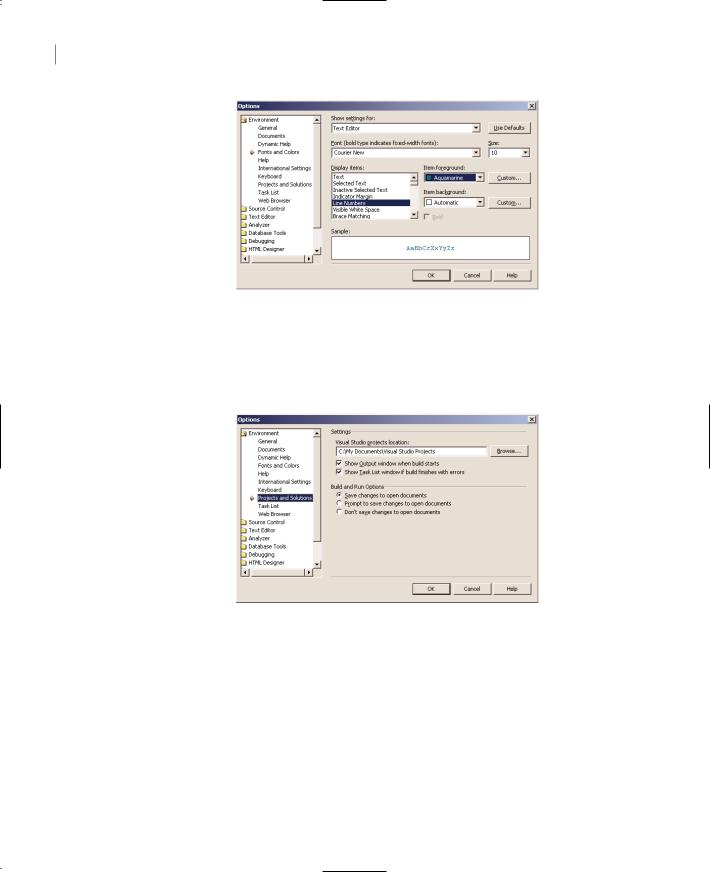
26 Chapter 1 GETTING STARTED WITH VB.NET
Figure 1.15
The Fonts and Colors options
Figure 1.16 shows the Projects and Solutions options. The top box is the default location for new projects. The three radio buttons in the lower half of the dialog box determine when the changes to the project are saved. By default, changes are saved when you run a project. If you
activate the last option, then you must save your project from time to time with the File Save All command.
Figure 1.16
The Projects and
Solutions options
Most of the tabs on the Options dialog box are straightforward, and you should take a look at them. If you don’t like some of the default aspects of the IDE, this is the place to change them.
A Few Common Properties
In the next few sections, I will go through some of the properties, methods, and events that are common to many controls, so that I will not have to repeat them with every control in the following chapters. These are very simple members you’ll be using in every application from now on.
To manipulate a control you use its properties, either on the Property Browser at design time, or though your code at runtime. To program a control, supply a handler for the appropriate events.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
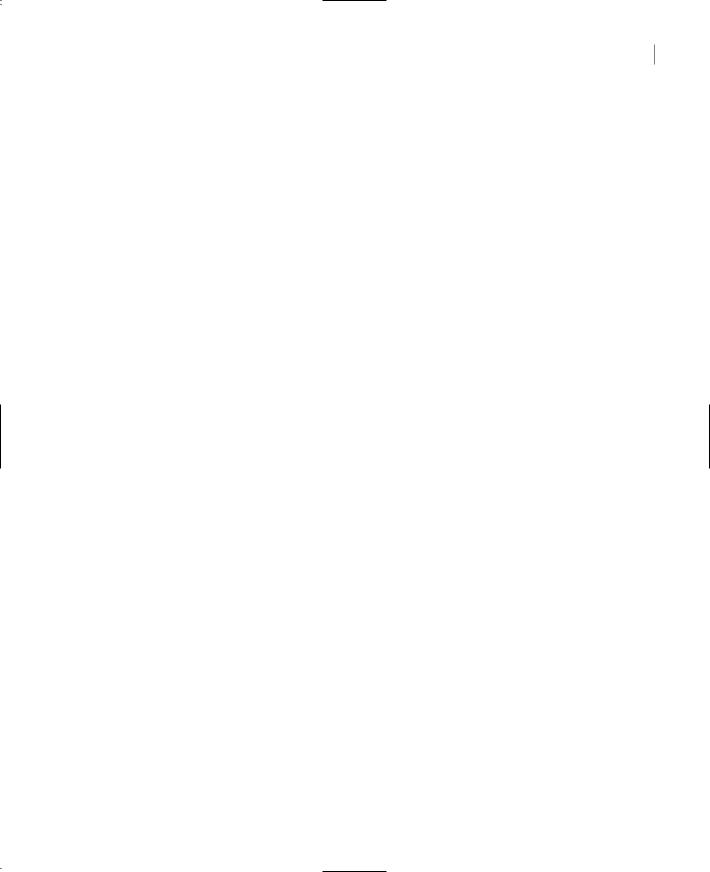
A FEW COMMON PROPERTIES 27
Controls expose methods, too, which act on the control. The Hide method, for example, makes the control invisible. Properties, methods, and events constitute the programmatic interface of the control and are collectively known as the control’s members.
All controls have a multitude of properties, which are displayed in the Properties window, and you can easily set their values. Different controls expose different properties, but here are some that are common to most:
Name The control’s name. This name appears at the top of the Properties window when a control is selected on the form and is also used in programming the control. To set the text on a TextBox control from within your code, you will use a statement like the following:
TextBox1.Text = “My TextBox Control”
You will see how to program the controls shortly.
Font A Font object that determines how the text of the control will be rendered at both design and runtime.
Enabled By default, all controls are enabled. To disable a control, set its Enabled property to False. When a control is disabled, it appears in gray color and users can’t interact with it. Disabling a control isn’t as rare as you may think, because many controls are not functional at all times. If the user hasn’t entered a value in all required fields on the form, clicking the Process button isn’t going to do anything. After all fields have been set to a valid value, you can enable the control, indicating to the user that the button can now be clicked.
Size Sets, or returns, the control’s size. The Size property is a Size object, which exposes two properties, the Width and Height properties. You can set the Size property to a string like “320, 80” or expand the Size property in the Properties window and set the Width and Height properties individually.
Tag Holds some data you want to associate with a specific control. For example, you can set the Tag property to the control’s default value, so that you can restore the control’s default value if the user supplies invalid data (a string in a TextBox control that expects a numeric value, or a date).
Text The text (a string) that appears on the control. The Label control’s caption can be set (or read) through the Text property. A control that displays multiple items, like the ListBox or the ComboBox control, returns the currently selected item in the Text property.
TabStop As you know, only one control at a time can have the focus on a form. To move the focus from one control to the other on the same form, you press the Tab key (and Shift+Tab to move the focus in reverse order). The TabStop property determines whether the control belongs to the so-called tab order. If True (which is the default value), you can move the focus to the control with the Tab key. If False, the control will be skipped in the tab order.
TabIndex A numeric value that determines the position of the control in the Tab order. The control with the smallest TabIndex value is the one that has the focus when the form is first loaded. If you press Tab once, the focus will be moved to the control with the next larger TabIndex value.
Visible Sometimes we want to make a control invisible. We do so by setting its Visible property to False (the default value of the property is True).
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
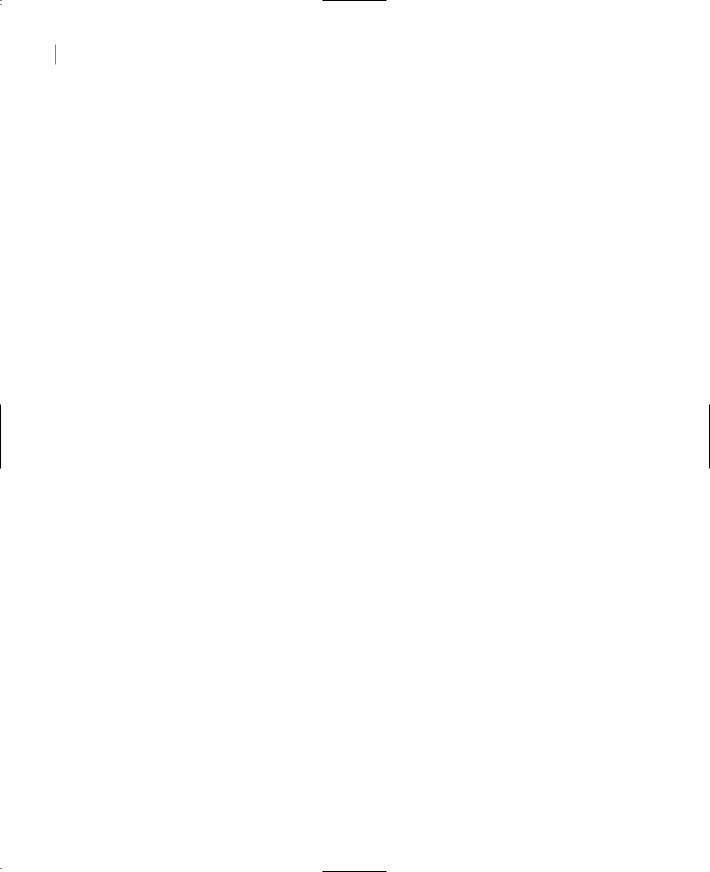
28 Chapter 1 GETTING STARTED WITH VB.NET
A Few Common Events
As you have already seen, and will also see in the coming chapters, much of the code of a Windows application manipulates the properties of the various controls on the form. The code of the application resides in selected event handlers. Each control recognizes several events, but we rarely program more than one event per control. In most cases, most of the controls on the form don’t have any code behind them. The events that are most frequently used in programming Windows applications are shown next.
Click This is the most common event in Windows programming, and it’s fired when a control is clicked.
DoubleClick Fired when the control is double-clicked.
Enter Fired when the control received the focus.
Leave Fired when the control loses the focus. We usually insert code to validate the control’s content in this event’s handler.
MouseEnter Fired when the mouse pointer enters the area of the control. This event is fired once. If you want to monitor the movement of the mouse over a control, use the MouseMove event.
MouseLeave This is the counterpart of the MouseEnter event, and it’s fired when the mouse pointer moves out of the area of the control.
XXXChanged Some events are fired when a property changes value. These events include BackColorChanged, FontChanged, VisibleChanged, and many more. The control’s properties can also change through code, and it’s very common to do so. To set the text on a TextBox control from within your code, you can execute a statement like the following:
TextBox1.Text = “a new caption”
You may wish to change the background color of a TextBox control if the numeric value displayed on it is negative:
If Val(TextBox.Text) < 0 Then
TextBox1.BackColor = Color.Red
End If
A Few Common Methods
In addition to properties, controls also expose methods. A method acts upon the control by performing a specific task. Windows controls don’t provide many methods, but the objects we’ll explore in the following chapters provide many more methods. You have already seen the ToUpper method, which converts a string to uppercase and returns it as a new string. In VB.NET, a string is more than a series of characters: it’s an object, and so is just about everything in .NET. Even a number is an object and exposes a few properties and methods of its own.
A String variable exposes the methods Length (it returns the string length), ToUpper (it converts the characters in the string to uppercase and returns a new string), and ToLower (it converts the characters in the string to lowercase and returns a new string). To see these methods in action, create
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
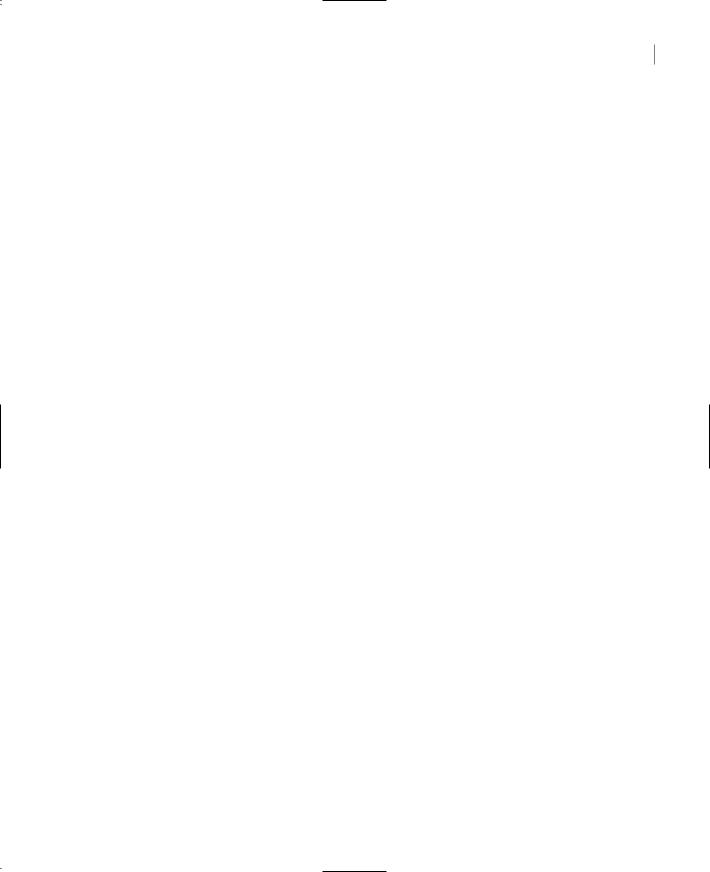
A FEW COMMON METHODS 29
a new application, place a Button control on the form and enter the following statements in its Click event handler:
Console.WriteLine(“Visual Basic”.Length)
Console.WriteLine(“Visual Basic”.ToUpper)
Console.WriteLine(“Visual Basic”.ToLower)
Then press F5 to run the application, and you will see the following in the Output window (this is where the Console.WriteLine statement sends its output):
12
VISUAL BASIC visual basic
Note If the Output window is hidden, select View Other Windows Output.
Here are a few methods that are common to most controls. In later chapters, where we’ll explore the Windows controls in detail, you’ll learn about the methods that are unique to individual controls. The following methods apply to most of the Windows controls.
Focus This method moves the focus to the control to which the method applies, regardless of the control that has the focus at the time. Your validation routine could move the focus to the control with an erroneous entry with the following statement:
TextBox1.Focus
It’s also possible to “trap” the focus to a specific TextBox control until the user enters a valid value, by calling the Focus method from within the Leave event. The following code segment doesn’t allow users to move the focus to another control while TextBox1 doesn’t contain a valid numeric value:
Private Sub TextBox1_Leave(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles TextBox1.Leave
If Not IsNumeric(TextBox1.Text) Then TextBox1.Focus()
End Sub
The function IsNumeric() returns True if its argument (the value in parentheses following the function’s name) is a numeric value, like 35 or 244.01.
Clear Many controls provide a method to clear their contents, and this is the Clear method. When you call the Clear method on a TextBox control, the control’s Text property is set to an empty string.
Hide/Show The Hide and Show methods reveal or conceal the control. The two methods are equivalent to setting the Visible property to True and False respectively.
PerformClick It’s rather common to invoke the Click event of a button from within our code. To do so, call the PerformClick method of the Button control. There are no equivalent methods for other events.
Scale This method scales the control by a value specified as argument. The following statement scales the TextBox1 control down to 75 percent of its current size:
TextBox1.Scale(0.75)
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |