
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary
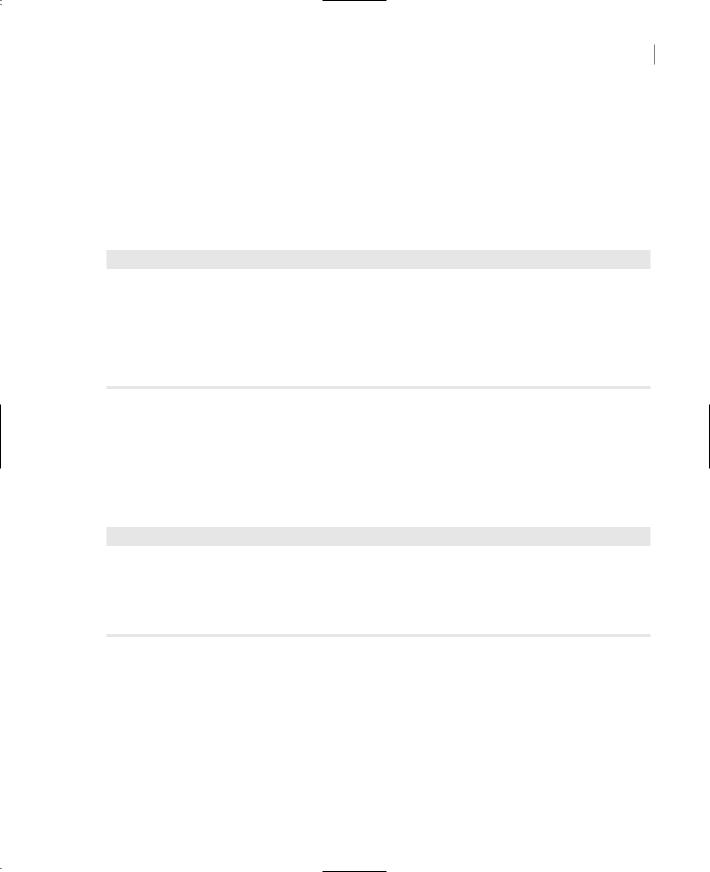
USING ACTIVEX CONTROLS 429
To test the enhanced ListBox control, place two buttons on the form, as shown in Figure 9.11. The Add New Item button prompts the user for a new item (a string) and adds it to the items ArrayList. Then it calls the Add method of the ListBox.Items collection to add the new item to the ListBox control. The reason you must add the new item to the ArrayList collection is that you can’t directly add items to the control. As you recall, the MeasureItem event adds one element of the ArrayList to the control at a time. Since both the MeasureItem and DrawItem methods pick up the item to be added from the ArrayList collection, you need not specify any argument to the ListBox1.Items.Add method. Listing 9.23 provides the code that adds a new item to the list.
Listing 9.23: Adding an Item to the List at Runtime
Private Sub Button2_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button2.Click Dim newItem As String
newItem = InputBox(“Enter item to add to the list”) items.Add(newItem)
ListBox1.Items.Add(“”) End Sub
The last feature in the test application is the reporting of the selected item when the user doubleclicks an item. I’ve included this code to demonstrate that, other than some custom drawing, the owner-drawn ListBox control carries with it all the functionality of the original ListBox control. It fires the same events, reports the same properties, and can be manipulated with the same methods.
When the user double-clicks the ListBox control, the code shown in Listing 9.24 is executed. This code retrieves SelectedIndex and SelectedItem properties and reports them to the application.
Listing 9.24: Retrieving the Selected Item from the Owner-Drawn ListBox Control
Private Sub ListBox1_DoubleClick(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles ListBox1.DoubleClick MsgBox(“Item at location “ & ListBox1.SelectedIndex & _
“ is “ & vbCrLf & ListBox1.SelectedItem)
End Sub
Using ActiveX Controls
Before ending this chapter, I would like to show you how to use ActiveX control with .NET. If you’re new to VB, ActiveX controls were the old Windows controls used with previous versions of VB. There are tons of ActiveX controls out there, and many of you are already using them in your projects. You can continue using them with your .NET projects as well. At this point, there’s a
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
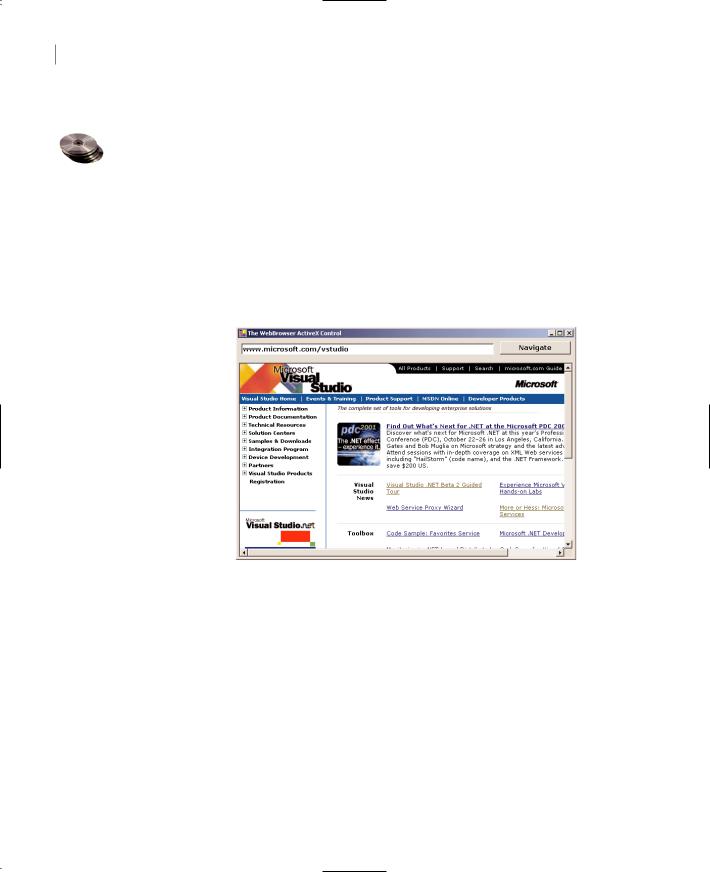
430 Chapter 9 BUILDING CUSTOM WINDOWS CONTROLS
relatively small number of .NET controls, so for a while we will have to use the same controls we used in our VB6 projects.
One of my favorite ActiveX controls is the WebBrowser control. I happen to have this control because I’ve installed VB6. If you’re a VB6 programmer, you will have access to this control. If not, hopefully Microsoft will make these controls available with the release version of Visual Studio, or allow developers to download them. The WebBrowser control is nothing less than Internet Explorer in a control. This control can render any HTML document and Web page you can view with Internet Explorer, but it will do so in the context of a form. You can create forms that allow users to navigate the Web (or at least connect to your company’s Web site) from within your application. Figure 9.12 shows a Windows form with an instance of the WebBrowser control on it. The document displayed on the control is Visual Studio’s home page. You can navigate to any URL by typing an address in the TextBox control at the top of the form and clicking the Navigate button.
Figure 9.12
Navigating the Web with the WebBrowser control
By default, .NET doesn’t know how to handle ActiveX controls. To use an ActiveX control in a
.NET application, you must create a “wrapper” around the ActiveX control. The wrapper is a layer of code that allows .NET to communicate with the ActiveX control. .NET thinks it’s talking to a
.NET control, and the ActiveX control thinks its talking to a COM component. In effect, the wrapper is a translator that allows the two parties to communicate with one another, even though they don’t understand each other’s language. This is called a runtime callable wrapper (RCW), and it’s created for you by the CLR as soon as you add an ActiveX control to your Toolbox.
To add an ActiveX control, such as the WebBrowser control, to your Toolbox, open the Customize Toolbox dialog box (right-click the Toolbox and select Customize). In the COM Components tab, locate the ActiveX control you want to add, as shown in Figure 9.13.
Now design a form like the one shown in Figure 9.12 and enter the code from Listing 9.25 in the button’s Click event handler:
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
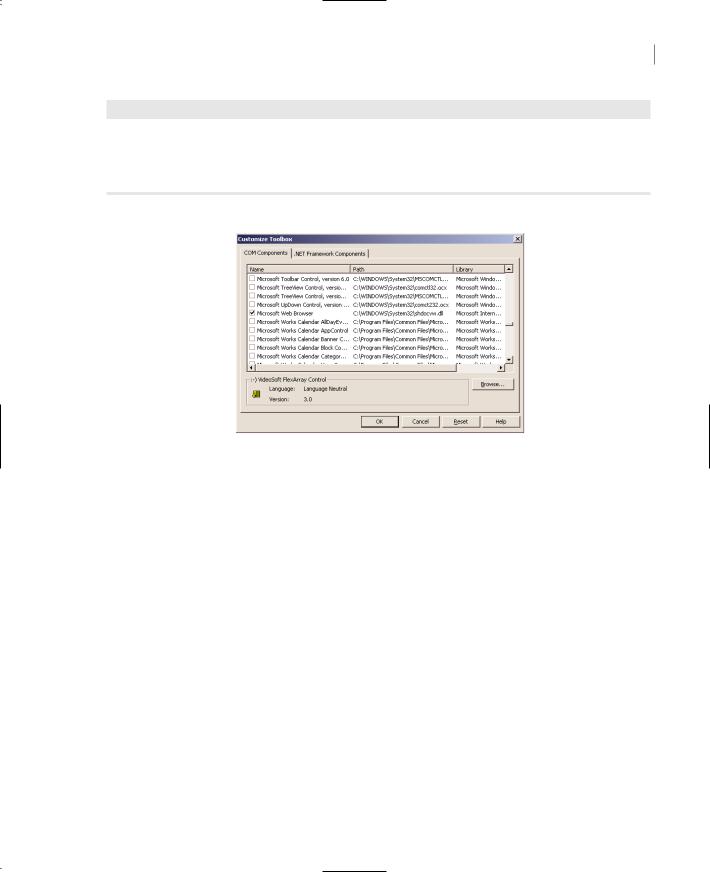
SUMMARY 431
Listing 9.25: Navigating to a URL with the WebBrowser Control
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
AxWebBrowser1.Navigate2(TextBox1.Text)
End Sub
Figure 9.13
Adding an ActiveX control to the Toolbox
That’s all it takes! You can also use this control to display local files to the users, such as help documents. Or allow them to connect to your site from within your application. I have found this control extremely useful in a large number of projects.
In Chapter 14, you’ll see how to use another ActiveX control, the Script control. The Script control allows you to execute short programs written in VBScript (a subset of Visual Basic). As you will see, it’s an extremely useful control that allows you to add scripting capabilities to your applications.
It is also possible to build your .NET controls so that they can be used in a COM environment, like Visual Studio 6. The process isn’t complicated, but you must first understand how ActiveX controls are registered in the system Registry. I won’t get into the details here, because this topic is of interest mostly to programmers who write .NET controls and want to expand their market by making their .NET controls compatible with earlier versions of Visual Studio. Please look up the documentation for specific information and step-by-step instructions on making your .NET control usable in the COM world.
Summary
One of the primary reasons for the phenomenal success of Visual Basic was that it could host custom controls. If you’ve been programming with VB for several years, you’ll remember the VBX controls. If you started using VB the last few years, you’ll remember the OCX controls. Now, it’s the
.NET controls. The controls keep getting better and so do the tools for creating custom controls.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
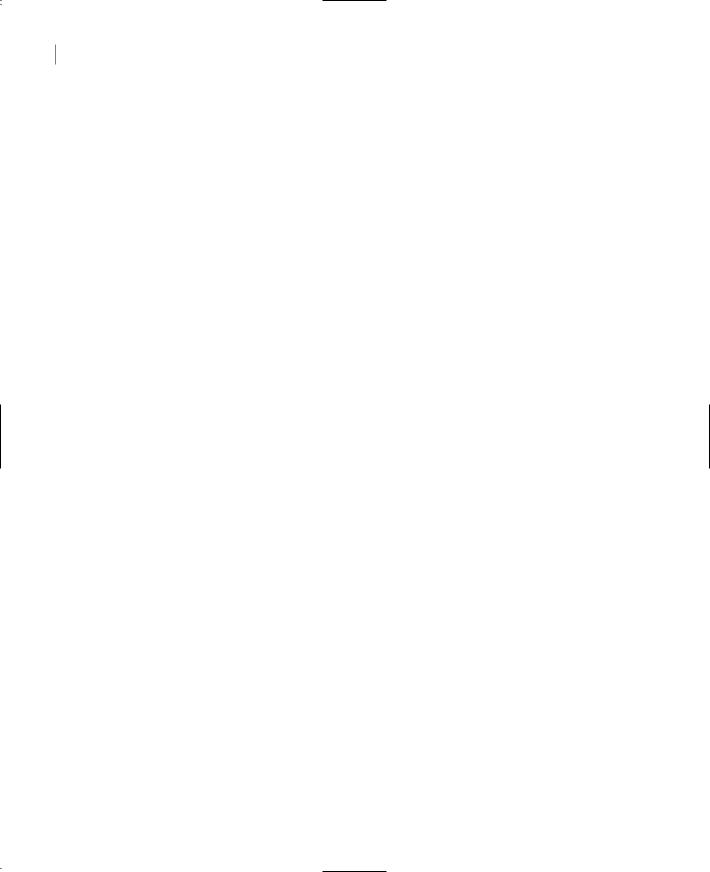
432 Chapter 9 BUILDING CUSTOM WINDOWS CONTROLS
The idea behind custom controls is that you can build a component with a visible and a programmatic interface and make it part of any development environment in the Windows world. Building custom controls isn’t just a task for the few companies that sell them. Even modest applications may call for custom controls. When you build a control, you’re actually encapsulating a lot of functionality in a black box, similar to a class. Unlike classes, however, controls have a visible interface too. Now that you know how to build your own classes and controls, you’re ready to distribute your code not only to end users, but developers as well. Even if you won’t make money by selling your components, there will always be a need for custom components in any programming environment. If you think that your code will be used repeatedly, either in multiple projects or by multiple programmers, consider packaging it as a custom class or control. The difference between the two is that controls expose the same functionality as classes but add a visible interface.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
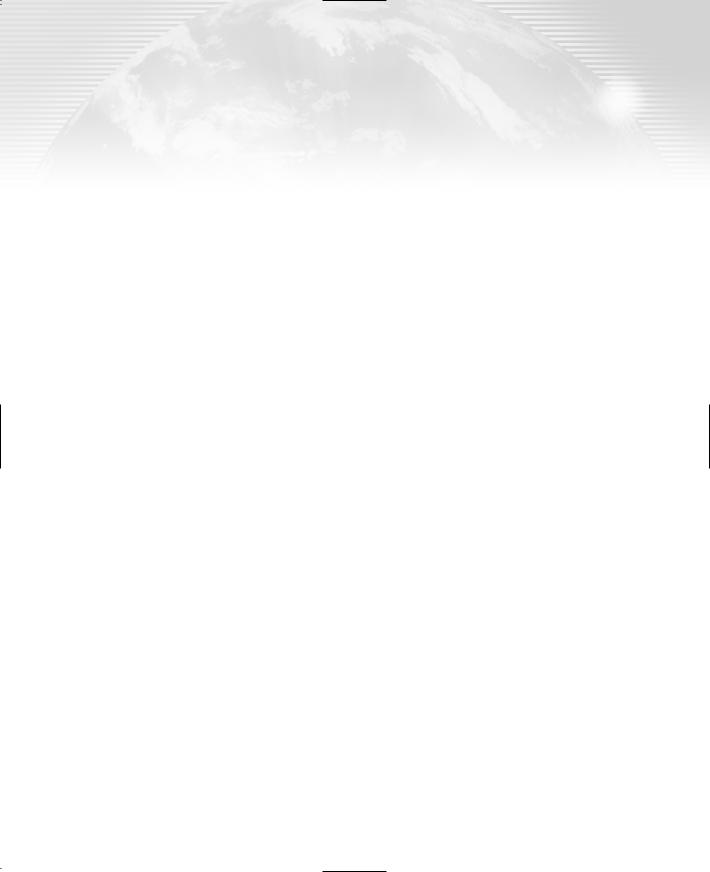
Chapter 10
Automating Microsoft Office
Applications
Another way to extend Visual Basic is to program the various objects exposed by the Office applications, or any other application that exposes an object model. Word, Excel, and Outlook expose rich object models that can be programmed, either from within the Office applications themselves, or in external applications, written in any .NET language. You can program any Office application with VBA or use VB.NET to program against the object model of each application. In this chapter, I’ll present the basic objects of the Office applications (Office 2000 and Office XP), because they are extremely popular and expose a whole lot of functionality.
I will limit the discussion to the three basic Office applications, Word, Excel, and Outlook, but once you understand how to manipulate their objects it shouldn’t be hard to look into the object models of PowerPoint, Project, and VISIO. Practically speaking, the most useful object model for VB programmers is that of Outlook, which allows you to read incoming messages or to create and send new ones from within applications written in VB.NET. Excel and Word also expose quite a bit of functionality, and you will see how you can tap into this functionality from within your VB applications.
An object model is a collection of classes that represents the objects, or entities, each application can handle, the properties that determine the characteristics of these entities, and the methods that act on them. The object model exposes all the functionality of its application, and you can program the exposed objects with any language. Word, for example, manipulates documents, which are made up of text, graphics, and tables (among other things). Word’s object model exposes the Document object, which represents a Word document. The Document object has a Range property, which represents a segment of the document (or all of it). The Range object, in turn, provides three collections—Paragraphs, Words, and Characters—which contain the corresponding entities of the document. All three collections provide the Font property, which lets you format the corresponding item by setting the font attributes. You can also apply a style to a paragraph or a word with the Style property.
As you will see, it is possible (and fairly straightforward) to start Word, create a new document, print it, and then close the application without ever displaying Word’s window. You can use this functionality to generate elaborate printouts from within your VB applications. You can
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |