
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary
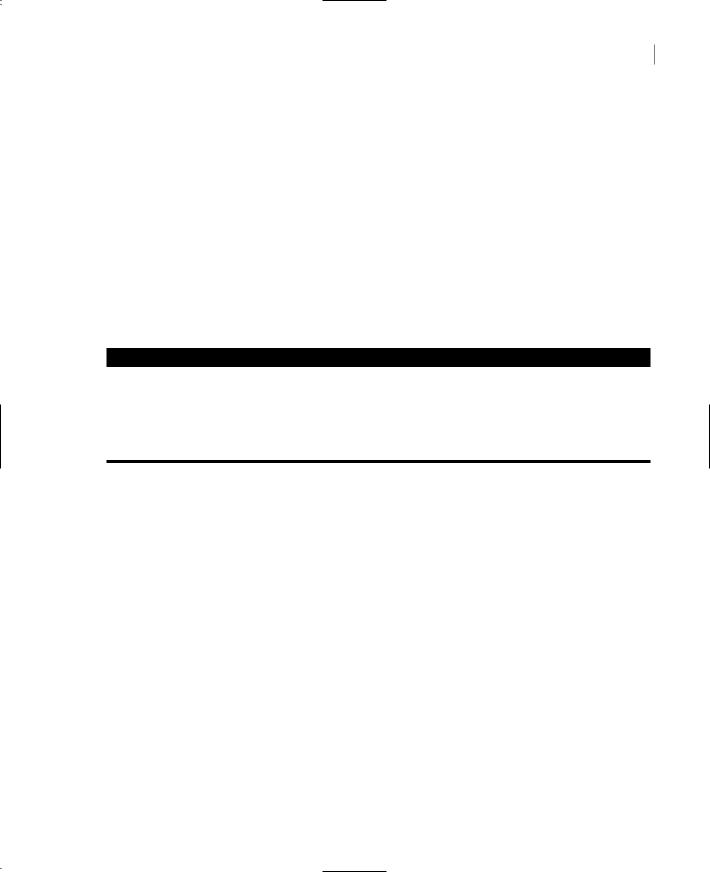
THE SCROLLBAR AND TRACKBAR CONTROLS 279
You can also add new items at runtime by adding the same code in the control’s LostFocus event handler:
Private Sub ComboBox1_LostFocus(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles ComboBox1.LostFocus Dim newItem As String = ComboBox1.Text
AddElement(newItem) End Sub
The ScrollBar and TrackBar Controls
The ScrollBar and TrackBar controls let the user specify a magnitude by scrolling a selector between its minimum and maximum values. In some situations, the user doesn’t know in advance the exact value of the quantity to specify (in which case, a text box would suffice), so your application must provide a more flexible mechanism for specifying a value, along with some type of visual feedback.
VB6 VB.NET
The ScrollBar control is the same as in VB6 with no substantial improvements. You will notice that there is only one ScrollBar control on the Toolbox, instead of the horizontal and vertical ones of VB6. In VB.NET, you can set the orientation of the control through the Orientation property. The main event of the ScrollBar control, Change, has a new name: it’s now called ValueChanged.
The TrackBar control is the old Slider control; other than its name, nothing else has changed.
The vertical scroll bar that lets a user move up and down a long document is a typical example of the use of a ScrollBar control. In the past, users had to supply line numbers to locate the section of the document they wanted to view. With a highly visual operating system, however, this is no longer even an option.
The scroll bar and visual feedback are the prime mechanisms for repositioning the view in a long document or in a large picture that won’t fit entirely in its window. When scrolling through a document or image to locate the area of interest, the user doesn’t know or care about line numbers or pixel coordinates. Rather, the user uses the scroll bar to navigate through the document, and the visible part of the document provides the required feedback.
The TrackBar control is similar to the ScrollBar control, but it doesn’t cover a continuous range of values. The TrackBar control has a fixed number of tick marks, which the developer can label (e.g., Off, Slow, and Speedy, as shown in Figure 6.12). The user can place the slider’s indicator to the desired value. While the ScrollBar control relies on some visual feedback outside the control to help the user position the indicator to the desired value, the TrackBar control forces the user to select from a range of valid values.
In short, the ScrollBar control should be used when the exact value isn’t as important as the value’s effect on another object or data element. The TrackBar control should be used when the user can type a numeric value and the value your application expects is a number in a specific range; for example, integers between 0 and 100, or a value between 0 and 5 inches in steps of 0.1 inches (0.0, 0.1, 0.2 … 5.0).
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
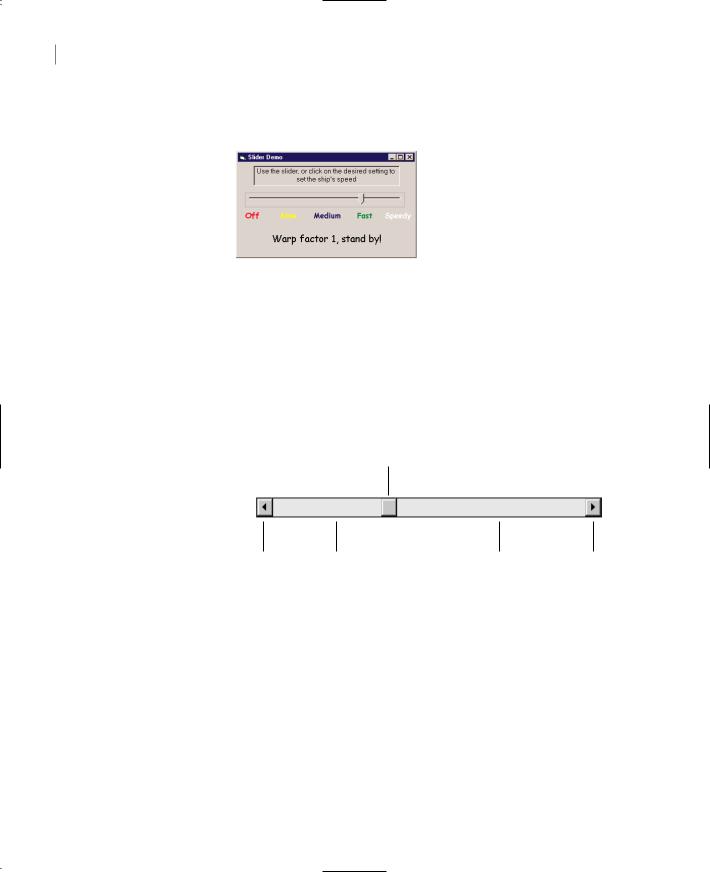
280 Chapter 6 BASIC WINDOWS CONTROLS
The TrackBar control is preferred to the TextBox control in similar situations because there’s no need for data validation on your part. The user can only specify valid numeric values with the mouse.
Figure 6.12
The TrackBar control lets the user select one of several discrete values.
The ScrollBar Control
The ScrollBar control is a long stripe with an indicator that lets the user select a value between the two ends of the control, and it can be positioned either vertically or horizontally. Use the Orientation property to make the control vertical or horizontal. The left (or bottom) end of the control corresponds to its minimum value; the other end is the control’s maximum value. The current value of the control is determined by the position of the indicator, which can be scrolled between the minimum and maximum values. The basic properties of the ScrollBar control, therefore, are properly named Minimum, Maximum, and Value (see Figure 6.13).
Figure 6.13 |
Value |
The basic properties of the ScrollBar control
SmallChange |
LargeChange |
LargeChange |
SmallChange |
Minimum The control’s minimum value. The default value is 0, but because this is an Integer value you can set it to negatives values as well.
Maximum The control’s maximum value. The default value is 100, but you can set it to any value you can represent with the Integer data type.
Value The control’s current value, specified by the indicator’s position.
The Minimum and Maximum properties are positive Integer values. To cover a range of negative numbers or non-integers, you must supply the code to map the actual values to Integer values. For example, to cover a range from 2.5 to 8.5, set the Minimum property to 25, set the Maximum property to 85, and divide the control’s value by 10. If the range you need is from –2.5 to 8.5, do the same but set the Minimum property to 0, set the Maximum property to 110, and subtract 25 from the Value property every time you read it.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
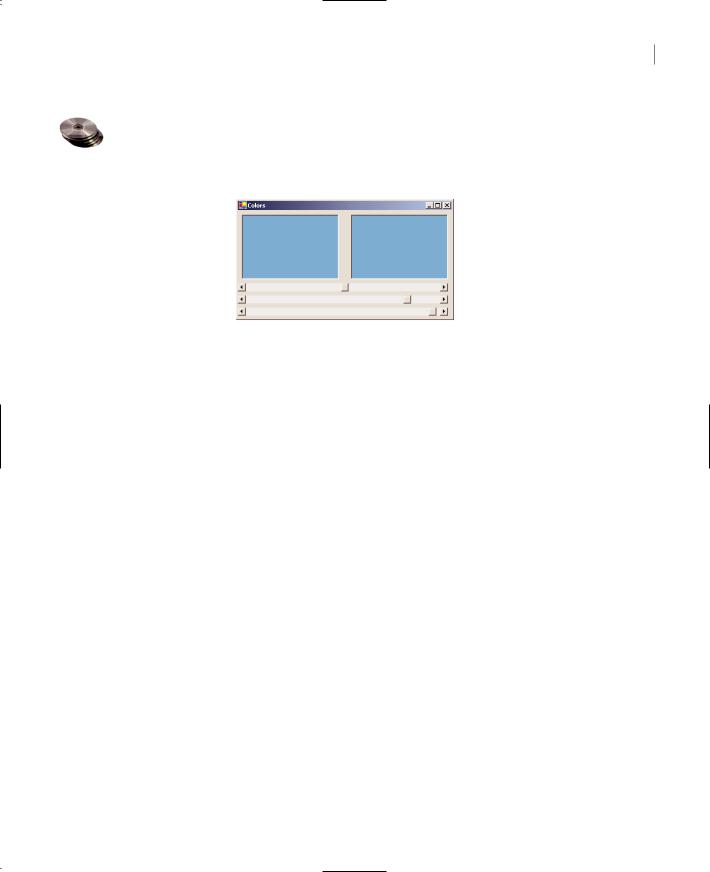
THE SCROLLBAR AND TRACKBAR CONTROLS 281
VB.NET at Work: The Colors Project
Figure 6.14 shows another example that demonstrates how the ScrollBar control works. The Colors application lets the user specify a color by manipulating the value of its basic colors (red, green, and blue) through scroll bars. Each basic color is controlled by a scroll bar and has a minimum value of 0 and a maximum value of 255.
Figure 6.14
The Colors application demonstrates the use of the ScrollBar control.
Note If you aren’t familiar with color definition in the Windows environment, see the section “Specifying Colors” in Chapter 14.
As the scroll bar is moved, the corresponding color is displayed, and the user can easily specify a color without knowing the exact values of its primary components. All the user needs to know is whether the desired color contains, for example, too much red or too little green. With the help of the scroll bars and the immediate feedback from the application, the user can easily pinpoint the exact value. Notice that this “exact value” is of no practical interest; only the final color counts.
Scroll bars and slider bars have minimum and maximum values that can be set with the Minimum and Maximum properties. The indicator’s position in the control determines its value, which is set or read with the Value property. In the Colors application, the initial value of the control is set to 128 (the middle of the range). Before looking at the code for the Colors application, let’s examine the control’s events.
The ScrollBar Control’s Events
The user can change the ScrollBar control’s value in three ways:
By clicking the two arrows at its ends. The value of the control changes by the amount specified with the SmallChange property.
By clicking the area between the indicator and the arrows. The value of the control changes by the amount specified with the LargeChange property.
By dragging the indicator with the mouse.
You can monitor the changes on the ScrollBar’s value from within your code with two events: ValueChanged and Scroll. Both events are fired every time the indicator’s position is changed. If you change the control’s value from within your code, then only the ValueChanged event will be fired.
The Scroll event can be fired in response to many different actions, such as the scrolling of the indicator with the mouse or a click on one of the two buttons at the ends of the scrollbars. If you
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
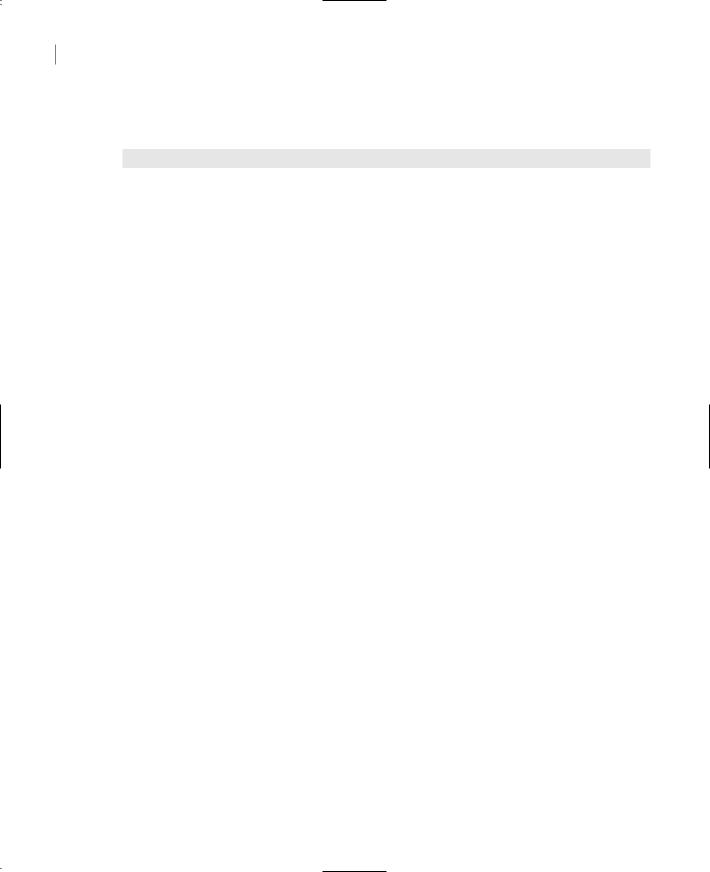
282 Chapter 6 BASIC WINDOWS CONTROLS
want to know the action that caused this event, you can examine the Type property of the second argument of the event handler. The settings of the e.Type property are shown in Table 6.4.
Table 6.4: The Actions That Can Cause the Scroll Event
Member |
Description |
EndScroll |
The user has stopped scrolling the control. |
First |
The control was scrolled to the Minimum position. |
LargeDecrement |
The control was scrolled by a large decrement (user clicked the bar between |
|
the button and the left arrow). |
LargeIncrement |
The control was scrolled by a large increment (user clicked the bar between |
|
the button and the right arrow). |
Last |
The control was scrolled to the Maximum position. |
SmallDecrement |
The control was scrolled by a small decrement (user clicked the left arrow). |
SmallIncrement |
The control was scrolled by a small increment (user clicked the right arrow). |
ThumbPosition |
The button was moved. |
ThumbTrack |
The button is being moved. |
|
|
Events in the Colors Application
The Colors application demonstrates how to program the two events. The two PictureBox controls display the color designed with the three scroll bars. The left PictureBox is colored from within the Scroll event, while the other one is colored from within the ValueChanged event.
As the user moves the indicator with the mouse, different colors are shown in the second PictureBox, which is colored from within the ValueChanged event. This event is every time a scrollbar changes value.The other PictureBox doesn’t follow the changes as they occur. In the Scroll event handler of the three scroll bars, the code examines the value of the e.Type property and reacts to it only if the event was fired because the scrolling of the indicator has ended. For all other actions, the event handler doesn’t update the color of the left PictureBox.
If the user attempts to change the Color value by clicking the two arrows of the scroll bars or by clicking in the area to the left or to the right of the indicator, both PictureBox controls are updated. While the user slides the indicator, or keeps pressing one of the end arrows, only the PictureBox to the right is updated.
The conclusion from this experiment is that you can program either event to provide continuous feedback to the user. If this feedback requires too many calculations, which would slow down the reaction of the corresponding event handler, you can postpone the reaction until the user has stopped scrolling the indicator. You can detect this condition by examining the value of the e.Type property. When it’s ScrollEventType.EndScroll, you can execute the appropriate statements. Listing 6.17 shows the code behind the Scroll and ValoueChanged events of the Srollbar that controls the red component of the color. The code of the corresponding events of the other two controls is identical.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |