
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary

976 Chapter 22 PROGRAMMING THE ADO.NET OBJECTS
If an error occurs, the exception handler will display the description of the error in a message box, then it will clear the edits and move to the product selected in the ListBox control. The user can select the previous row and edit it again, knowing what type of error prevented the update of the DataSet.
If you click the Delete button, the current row will be removed from the DataSet (it will also be removed from the ListBox control, just as new rows are added automatically to this control). But products are referenced in the Order Details table, so how can we delete them? Remember, the DataSet resides in the local computer and is disconnected from the database. Since the DataSet contains a single table, there are no relations to be enforced and the DataSet happily removes the row when you request it. What will happen when you attempt to update the database, however, is a different story. SQL Server will emphatically refuse to remove a row that’s being referenced by another table, and the update operation will fail. Delete a row, then click the Update button. You’ll get an error message to the effect that the row can’t be removed because this would violate the PK_Order_ Details_Products constraint.
Handling Identity Fields
I should point out the following behavior of the application. If you add a row, its ProductID field will be assigned a value automatically. The ProductID column is an Identity column, and you can’t set it; it must be assigned a value by the DBMS. The DataSet knows that the ProductID column is an Identity column and, every time you add a row, it assigns the next available value to it. If the last row’s ProductID field is 90, it will assign the value 91 to the first row you add, the value 92 to the second row, and so on. But what will happen if another user has already added a few rows to the table? Run the application again, add a couple of rows, and then switch to the Enterprise Manager. Do not update the database yet.
In the Enterprise Manager, add a couple of rows to the Products table. Then switch to the application and click the Update button. The new rows will be added to the underlying table. Write down the IDs of the new rows. Then load the DataSet again by clicking the Load button, locate the newly added rows, and examine their IDs. This time they’re different! They have the values assigned to them by the database.
This is a reasonable behavior too, as we don’t really care about the IDs of the rows. Or do we? In an application like EditProducts, no one would really care to see the actual ID of a product. There are situations, however, where you may want to use the ID of a row as a foreign key in another table. Let’s say you want to issue an invoice. First, you must add a row to the Orders table. The new order will get an ID from the database. This ID, however, must appear in all the rows of the Order Details table that refer to the specific invoice. And obviously, we must make sure that the proper ID is inserted in the Order Details table (it must be the final ID of the order assigned by the database, not a temporary ID generated by the DataSet).
Retrieving Proper IDs
There are situations where we do need to know the proper ID assigned to an identity field by the database. The best way to handle these situations is to create a stored procedure that accepts the fields of a new row, writes it to the database, and returns the ID assigned by the database. Although
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
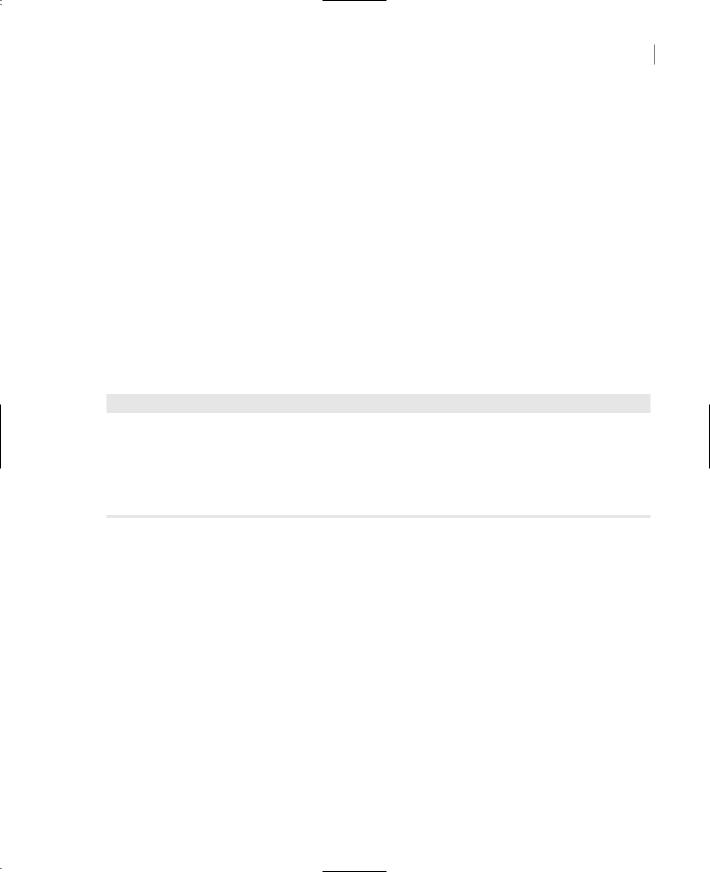
THE DATAFORM WIZARD 977
this can be done through the DataSet object, why bother with a mechanism that’s optimized for disconnected scenarios? We want to connect to the database, commit the new row, and get back its ID as soon as possible. You see, the DataSet was designed for a disconnected world, but not all dataaccess requirements fall into this category. It’s highly unlikely that ADO.NET will remain for long a disconnected data-access mechanism—Microsoft will have to add features to make ADO.NET work in connected scenarios like the one described here.
Instead of using the DataSet to update the database, we can use the Command object. I discussed the Command object in Chapter 21, but I will repeat the process here by demonstrating how to add a new row to the Orders table. First, you must create a stored procedure that inserts a new row to the table. The only required field for the Orders table is the ID of the customer that placed the order. The order’s date (OrderDate field) can be assigned the current date and time by the database. The remaining fields (the ID of the employee who made the sale, the shipping address, and so on) are optional. To keep the complexity of the sample code to the bare minimum, I will ignore these fields.
The stored procedure in Listing 22.6 accepts as argument the ID of a customer and creates a new row in the Orders table. After the execution of the INSERT statement, the stored procedure retrieves the value of the identity field of the new row (it’s given by the expression @@IDENTITY) and returns it to the calling application.
Listing 22.6: The NewOrder Stored Procedure
CREATE PROCEDURE NewOrder @custID nchar(5)
AS
INSERT INTO Orders (CustomerID, OrderDate) VALUES(@custID, GetDate()) RETURN (@@IDENTITY)
GO
The value returned by the stored procedure is known as its return value, and you’ll see shortly how you can retrieve it from within your application.
Once the stored procedure is in place, you can create a new Command object to call the stored procedure. First we establish a Connection object to the database, then we open the connection and assign the Connection object to the Connection property of the Command object.
The Command object’s CommandText property is set to the name of the stored procedure, and its CommandType property is set to the constant CommandType.StoredProcedure. At this point, we can execute the stored procedure, but we must first add some parameters to it. We create a new Parameter object for each parameter expected by the stored procedure, set its name, type, and value, and then add it to the Command object’s Parameters collection. Finally, we call the Command object’s ExecuteScalar method, which returns the stored procedure’s return value. Listing 22.7 shows how to add a new row to the Orders table and then retrieve the new row’s OrderID field. If the new row can’t be added to the table, the stored procedure will raise an error (it will also return the value zero). The ID of the new row is then stored in the orderID variable for further processing.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |