
PICmicro MCU C - An itroduction to programming The Microchip PIC in CCS C (N.Gardner, 2002)
.pdf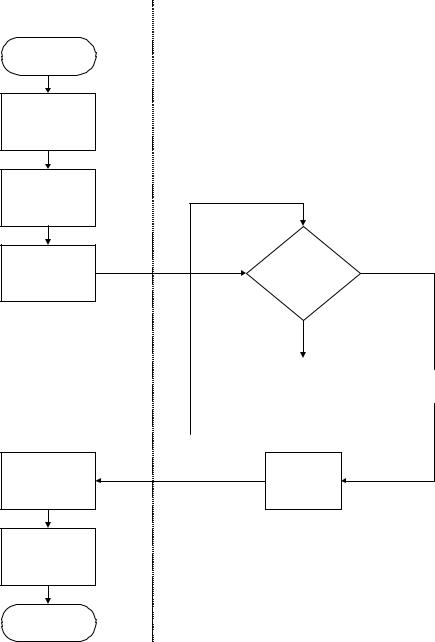
Interrupts can come from a wide range of sources within the PICmicro®MCU and also from external events. When an interrupt occurs, the PIC hardware follows a fixed pattern as shown below.
HARDWARE FLOW |
SOFTWARE FLOW |
INTERRUPT
GIE = 0
RETURN
ADDRESS TO
STACK
PC LOADED WITH 04H
PC LOADED WITH STACK
GIE = 1
PROGRAM CONTINUES
TEST
INTERRUPT
FLAGS
|
|
DO ROUTINES IN |
|
|
|
|
|
|
|
|
ALL FLAGS |
||
|
|
INTERRUPT & |
|
|
||
|
|
|
|
CLEARED |
||
|
|
CLEAR FLAGS |
|
|
||
|
|
|
|
|
||
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
RETFIE
The hardware is completely under PICmicro®MCU control, the software is all your responsibility. When an interrupt occurs, the first step is to test and determine if the source is the desired one or, in the case of multiple interrupts, which one to handle first, etc.
Depending on which PIC is used in a design, the type and number of interrupts may vary. The PIC16C5X series have no interrupts, and software written for these products will have to perform a software poll. Some of the interrupt sources are shown below, but refer to the data sheet for latest information.
131
#priority sets up the order of the interrupt priority:
#priority rtcc, rb, tmr0, portb
#int_globe - use with care to create your own interrupt handler. The main save and restore of registers and startup code is not generated.
#int_default is used to capture unsolicited interrupts form sources not setup for interrupt action. Examine the interrupt flags to determine which false interrupt has been triggered.
#int_xxx – where xxx is the desired interrupt:
#int_rda |
//enable usart receive interrupt |
|
rs232_handler() |
//interrupt driven data read and store |
|
{ |
|
|
b=getch(); |
//load character |
|
Buffer[Buff+1]=b; |
//store character |
|
Buff++; |
|
//increment pointer |
}
enable_interrupts(level); disable_interrupts(level);
This functions set or clear the respective interrupt enable flags so interrupts can be turned on and off during the program execution.
ext_int_edge (edge);
This is used to select the incoming polarity on PORTB bit0 when used as an external interrupt. The edge can be 1_to_h or h_to_1.
This example forces an interrupt on receipt of a character received via the USART. The character is placed in a buffer and the buffer incremented, ready for the next character. This function is extracted from an LCD display program, as the characters are received faster than the display can handle them.
#int_rda |
//enable usart receive interrupt |
|
rs232_handler() |
//interrupt driven data read and store |
|
{ |
|
|
b=getch(); |
//load character |
|
Buffer[Buff+1]=b; |
//store character |
|
Buff++; |
|
//increment pointer |
} |
|
|
main() |
|
|
{ |
|
|
enable_interrupts(INT_RDA); enable_interrupts(GLOBAL); do { while(True); }
132
}
Include Libraries
These libraries add the ‘icing on the cake’ for C programmer. They contain all the string handling and math functions that will be used in a program. The various libraries are included as, and when, the user requires them.
CTYPE.H contains several traditional macros as follows:
Returns a TRUE if:
isalnum(x) |
x is an alphanumeric value i.e. 0-9, ’A’ to ‘Z’, or ‘a’ to ‘z’ |
isalpha(x) |
x is an alpha value i.e. ’A’ to ‘Z’, or ‘a’ to ‘z’ |
isdigit(x) |
x is an numeric value i.e. 0-9 |
islower(x) |
x is an lower case value i.e. ‘a’ to ‘z’ |
isupper(x) |
x is an upper case value i.e. ’A’ to ‘Z’ |
isspace(x) |
x is an space |
isxdigit(x) |
x is a hexadecimal digit i.e. 0-9, ’A’ to ‘F’, or ‘a’ to ‘f’ |
STDLIB.H contains
f = abs(x)
i = atoi(char *ptr) i = atol(char *ptr) i = labs(i)
returns the absolute value of x
returns the ASCII representation of the character as int returns the ASCII representation of the character as long returns the absolute value of a long integer x
MATH.H holds all the complicated math functions. Examination of this file gives an insight into how the mathematical functions operate. In all cases, the value returned is a floating-point number.
l = sqrt(x) |
returns the non-negative square root of the float value x |
l = sin(x) |
returns the sine value of float x |
l = asin(x) |
returns the arc sine value of float x |
l = tan(x) |
returns the tan value of float x |
l = atan(x) |
returns the arc tan value of float x |
l = cos(x) |
returns the cos value of float x |
l = acos(x) |
returns the arc cos value of float x |
l = floor(x) |
returns the largest value not greater than the value of float x |
l = ceil(x) |
returns the smallest value not greater than the value of float x |
l = exp(x) |
returns the exponential value of float x |
l = log(x) |
returns the log to base e value of float x |
l = log10(x) |
returns the log to base 10 value of float x |
Where Next
What do I need to start development?
The minimum items required to start PICmicro®MCU development work are:
An IBM compatible PC
133
Windows 95, 98, NT, 2000, Me, XP or Linux
C Compiler
If you then wish to take the development from paper to a hardware design, you will need:
A programmer – for reliability and support get the PIC®START PLUS Which covers all the PICmicro®MCU devices and is upgradable
A development board or hardware starter kit – to save time trying to debug software and hardware
Some EEPROM or Flash part, you will not need an eraser, as the device is electrically erasable (i.e. no window)
Development Path
Zero Cost |
Demo versions of the C compiler |
Starter |
PICSTART PLUS programmer, C compiler, and PIC MCU sample |
Intermediate |
Microchip ICD for 16F87x family or ICD2 for most Flash PIC MCU |
Serious |
In Circuit Emulator (ICE). ICEPIC, PIC MASTER, MPLAB ICE2000 or |
|
ICE4000 allows debugging of hardware and software at the |
|
same time. You will need a programmer to go with the ICE, see |
|
a catalog for part numbers. |
Pointers to get started
Start off with a simple program – don’t try to debug 2000 lines of code in one go.
Use known working hardware.
Have a few flash version of PIC MCU chip on hand when developing to save time for waiting.
If using PIC®START PLUS (programmer only) you will need to use the program-test-modify process – so allow extra development time.
Use some form of I/O map when starting your design to speed up port identification and function.
Draw a software functional block diagram to enable modular code writing.
Comment on the software as it’s written. Otherwise, it is meaningless the following day or if read by another.
Write, test, and debug each module stage by stage.
Update documentation at the end of the process.
►Attend a Microchip approved training workshop.
What happens when my program won’t run?
Has the oscillator configuration been set correctly when you programmed the PIC?
Was the watchdog enabled when not catered for in the software?
Have all the ports been initialized correctly?
134
On 16C7X devices, check if the ADCON1 register is configured as analog or digital.
Ensure the data registers are set to a known condition.
Make sure no duplication of names given to variables, registers, and lables.
Is the reset vector correct, especially if code has been moved from one PICmicro®MCU family to another?
Reference Literature
Microchip data sheets and CDROM for latest product information.
CCS Reference Manual
Microchip MPLAB Documentation and Tutorial
Some good reference books on C (in general)
Turbo C – Kelly & Pohl
An Introduction to Programming in C - Kelly & Pohl
C Programming Guide - Purdum
The C Programming Language – Kernighan & Ritchie
Internet Resource List
http://www.pic-c.com
http://www.piclist.com
Contact Information
CCS |
http://www.ccsinfo.com |
Microchip |
http://www.microchip.com |
Authors Information
Nigel Gardner is an Electronic Engineer of over 20 years industrial experience in various field. He owns Bluebird Electronics which specializes in LCD display products, custom design work, PIC support products and Microchip training workshops. Nigel is a member of the Microchip Consultants Group.
Tel: |
01380 827080 |
Fax: |
01380 827082 |
Email: |
info@bluebird-electronics.co.uk |
Web: |
www.bluebird-electronics.co.uk |
135