
- •Seagate Crystal Web Reports Server Overview
- •What is the Web Reports Server?
- •Who should use the Web Reports Server?
- •Web Reports Server Features
- •New Features in Version 7
- •The Web Reports Server vs. Active Server Pages
- •Sample Web Sites
- •Implementing the Web Reports Server
- •Choosing a Web Reports Server
- •System Requirements
- •Installing the Web Reports Server
- •Confirming Correct Installation
- •Virtual Directories
- •Creating a Web Site
- •For More Information
- •Crystal Web Reports Server Administration
- •The Web Reports Server Configuration Application
- •Page Server Tab
- •Image Server Tab
- •Report Exporting Tab
- •Server Mappings Tab
- •Report Viewing Tab
- •The Page Server and the Image Server
- •Smart Navigation
- •Drilling Down on Data
- •Database Location
- •Web Reports Server Commands
- •The Crystal Web Reports Server Command Expert
- •Constructing Report Requests
- •Changing Selection Formulas in Web Reports
- •SQL and ODBC Data Sources
- •SQL Stored Procedures and Parameter Fields
- •Report Exporting
- •Refreshing Web Report Data
- •Web Reports Server Architecture
- •The Web Reports Server Extension
- •The Seagate Crystal Web Image Server
- •The Seagate Crystal Web Page Server
- •Report Processing
- •Job Manager Overview
- •Seagate Crystal Report Engine Automation Server
- •Visual InterDev Design-time ActiveX Control
- •Using an Existing Report
- •Building a Report at Runtime
- •Editing Active Server Pages
- •Customizing the Crystal Smart Viewer
- •Modifying the Report
- •Session Timeout
- •Sample Web Site
- •Crystal Smart Viewer Overview
- •Features of the Crystal Smart Viewers
- •Printing from the Crystal Smart Viewers
- •Using Crystal Smart Viewers in Applications
- •Crystal Smart Viewer for HTML
- •Limitations of HTML Reports
- •Crystal Smart Viewer for Java
- •Adding the Viewer to a Web Page
- •Crystal Smart Viewer for ActiveX
- •AuthentiCode Certification
- •Adding the Viewer to a Web Page
- •Downloading the Viewer from the Server
- •ActiveX Viewer Example
- •Introduction to the Crystal Report Engine
- •Before using the Crystal Report Engine in your application
- •Using the Crystal Report Engine
- •Crystal Report Engine API
- •Declarations for the Crystal Report Engine API (REAPI)
- •Using the Crystal Report Engine API
- •The Print-Only Link
- •The Custom-Print Link
- •Working with Parameter Values and Ranges
- •Working with section codes
- •Crystal Report Engine API variable length strings
- •Crystal Report Engine API structures
- •Working with subreports
- •Changing report formats
- •Exporting reports
- •PEExportTo Overview
- •PEExportOptions Structure
- •Considerations when using the export functions
- •Handling Preview Window Events
- •Distributing Crystal Report Engine Applications
- •Additional Sources of Information
- •Using the Crystal Report Engine API in Visual Basic
- •When to Open/Close the Crystal Report Engine
- •Embedded Quotes in Visual Basic Calls to the Crystal Report Engine
- •Passing Dates/Date Ranges in Visual Basic using the Crystal Report Engine API Calls
- •Identifying String Issues in Visual Basic Links to the Crystal Report Engine
- •Hard-coded Nulls in Visual Basic User Defined Types
- •Visual Basic Wrapper DLL
- •Crystal ActiveX Controls
- •Adding the ActiveX Control to your Project
- •Using the ActiveX Controls
- •Upgrading from the Crystal Custom Control
- •Crystal Report Engine Automation Server
- •Adding the Automation Server to your Visual Basic Project
- •Using the Automation Server in Visual Basic
- •Object Name Conflicts
- •Viewing the Crystal Report Engine Object Library
- •Handling Preview Window Events
- •Distributing the Automation Server with Visual Basic Applications
- •Sample Applications
- •Active Data Driver
- •Data Definition Files
- •Using the Active Data Driver
- •Creating Data Definition Files
- •Using ActiveX Data Sources at Design Time
- •Crystal Data Object
- •CDO vs. the Crystal Data Source Type Library
- •Using the Crystal Data Object
- •Crystal Data Object Model
- •Crystal Data Source Type Library
- •Creating a new project and class
- •Adding the type library
- •Implementing the functions
- •Passing the CRDataSource object to the Active Data Driver
- •Crystal Data Source Projects
- •Grid Controls and the Crystal Report Engine
- •Bound Report Driver and Bound Report Files
- •Crystal ActiveX Control Properties
- •Creating a Bound Report using the Crystal ActiveX Control
- •Creating a Formatted Bound Report
- •Creating a Formatted Bound Report at Runtime
- •Sample Application
- •ActiveX designers
- •The Report Designer Component vs. Seagate Crystal Reports
- •Data Access
- •No drag and drop between reports – use copy and paste
- •Conditional Formatting
- •Preview Window
- •Pictures
- •Guidelines
- •Subreports
- •The dual formula environment
- •Application Distribution
- •Installing the Report Designer Component
- •System Requirements
- •Installation
- •Using the Seagate Crystal Report Designer Component
- •Adding the Report Designer Component to a Project
- •Selecting Data
- •The Report Expert
- •Adding the Smart Viewer
- •Running the Application
- •CrystalReport1 - The Report Designer Component
- •CRViewer1 - The Smart Viewer Control
- •The Code
- •Report Packages
- •Working with data
- •ADO and OLEDB
- •Connecting to data with ADO
- •Connecting to data with RDO
- •Connecting to data with DAO
- •Data Environments
- •Data Definition Files
- •Report Templates
- •ODBC, SQL, and PC data sources
- •Report Designer Overview
- •Introduction to the Report Designer Component
- •Report Designer Architecture
- •Report Designer Object Model Programming
- •Report Designer Object Model Introduction
- •Obtaining a Report object
- •Displaying the report in the Smart Viewer
- •Setting a new data source for the report
- •Using ReadRecords
- •Passing fields in the correct order
- •Working with secure data in reports
- •Handling the Format event
- •Changing the contents of a Text object
- •Changing OLE object images
- •Working with Sections
- •Working with the ReportObjects collection
- •Working with the FieldObject object
- •Working with the SubreportObject object
- •Working with the Database and DatabaseTables objects
- •Working with the CrossTabObject object
- •Exporting a report
- •The Application object
- •Report events
- •Microsoft Access Sessions
- •Programmatic ID
- •Report Distribution Considerations
- •Distributing reports as part of the application
- •Saving reports as external files
- •Saving data with reports
- •VCL Component Overview
- •Installation
- •Delphi 2
- •Delphi 3 & 4
- •C++ Builder 3
- •Programming Overview
- •Introduction to the Object Inspector
- •Changing Properties in the Object Inspector
- •Changing Properties at Runtime
- •Delphi Programmers introduction to the SCR Print Engine
- •Dealing with SubClass Objects
- •Consistent Code
- •Using the Retrieve method
- •Working with subreports
- •Other Guidelines
- •Programming Tips
- •Always Set ReportName First
- •Discard Saved Data
- •Verify Database
- •Connecting to SQL Servers
- •Changing Tables & Formulas
- •Changing Groups & Summary fields
- •Using the Send methods
- •Using the JobNumber property
- •TCrpeString
- •Introduction
- •TCrpeString VCL Properties
- •Using the TCrpeString
- •Using Variables with Formulas
- •Introduction
- •Examples
- •About Section Names
- •Introduction
- •Methodology
- •StrToSectionCode
- •C++ Builder 3
- •Introduction
- •Code Syntax
- •Additional Code Examples
- •Known Problems
- •Retrieving ParamFields from a Subreport
- •DialogParent and Temporary Forms
- •Technical Support

Using ReadRecords
When using the SetDataSource method, be aware that the method will fail if your Recordset or Resultset object goes out of scope. For example, consider the following code:
Dim Report As New CrystalReport1
Private Sub Form1_Load()
Call SetData
CRViewer1.ReportSource = rpt
CRViewer1.ViewReport
End Sub
Private Sub SetData()
Dim rs As New ADOR.Recordset
rs.Open “Select * From Customer”, “Xtreme sample data” rpt.Database.SetDataSource rs
End Sub
In this example, although the rpt object is global (for the Form), the rs object exists only for the life of the SetData procedure. Even though it is assigned to the rpt object before SetData finishes, the object, and the data, that rs represents goes out of scope and is invalid for the Load event. Thus, the code will fail.
This problem can be solved using the ReadRecords method. ReadRecords forces the report to read the records into the report so that they are internal to the Report object itself rather than remaining as a separate Recordset object referenced by the report. Normally, this process would not happen until the report is displayed in the viewer using the ViewReport method.
Private Sub SetData()
Dim rs As New ADOR.Recordset
rs.Open “Select * From Customer”, “Xtreme sample data” rpt.Database.SetDataSource rs
rpt.ReadRecords End Sub
Passing fields in the correct order
When defining a Recordset or Resultset object to pass to your report, you must pay attention to the order in which the fields are added to the recordset. Fields must appear in the recordset in the same order in which they have been added to your report. In addition, all fields that appear in a data source should be included in the recordset, whether or not they are actually used in the report. For more information on
Consider a report designed using the following fields from a Data Definition file:
●Customer ID
●Customer Name
●Last Year’s Sales
The Report Designer Component |
177 |
Now, assume the Data Definition file was originally created with all of the following fields:
●Customer ID
●Customer Name
●Contact First Name
●Contact Last Name
●Contact Title
●Contact Position
●Account Manager
●Last Year’s Sales
●Address1
●Address2
●City
●Region
●Country
●Postal Code
●Phone
●Fax
When you create a new recordset, if you only add the three fields that appear in your report, values for one or more fields may be missing. You must, instead, include the three fields in your report in the order that they appear in the Data Definition file, along with any fields that may appear between them. Thus, to correctly display Customer ID, Customer Name, and Last Year’s Sales in your report, you must design the new recordset using all of the following fields:
●Customer ID
●Customer Name
●Contact First Name
●Contact Last Name
●Contact Title
●Contact Position
●Account Manager
●Last Year’s Sales
In fact, you may want to get in the habit of designing your runtime recordsets so that they include all fields from the original Data Definition file. This can save time later if you make changes to the report.
The Report Designer Component |
178 |
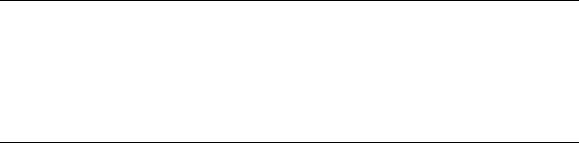
Working with secure data in reports
If your report connects to a secure data source that requires log on information, you will not be able to log off of the data source until the Report object has been closed or gone out of scope. Keep in mind that if you assign the Report object to the ReportSource property of the CRViewer object in the Seagate Crystal Reports Smart Viewer, the Report object can not be closed until you assign a new value to the Smart Viewer or close the CRViewer object. Thus, you will not be able to log off of a secure data source until the report is no longer displayed in the Smart Viewer.
Handling the Format event
The Seagate Crystal Report Designer Component provides a Format event for every section of your report. This allows you to dynamically control report formatting and output at runtime. For example, conditional formatting can be applied during the Format event, based on conditions that exist only at runtime.
The Format event is a standard Visual Basic event that can be programmed by displaying the Code View of the Report Designer Component. Assuming the Report Designer has been named CrystalReport1 in your Visual Basic project:
1In the Visual Basic Project window, select the CrystalReport1 designer from the Designers folder.
2Click the View Code button in the toolbar at the top of the Project window. A Code window will appear for the CrystalReport1 designer component.
3In the object drop-down list box at the top left of the Code window, select a Section object that you want to apply code to. Notice that Section objects are numbered in the order that they appear on your report, from the top of the Report Design window to the bottom. For instance, if you select the Section1 object, your code will apply to the Report Header section of your report. These Section objects are labeled for you at the top of each section in the Report Designer window.
Notice that when you select a Section object, the Format event is automatically selected for you in the event drop-down list box at the top right of the Code window. Format is the only event available to the Section object.
When writing code for the Format event, keep in mind that not all properties and methods for all objects are available during the Format event. Many properties are available on a read-only basis. If you are not sure about a property or method, refer to the specific property or method name in the Report Designer Object Model reference section of this Help file.
The Format event receives a single argument from the Report Designer Component. The pFormattingInfo argument is an object of type FormattingInfo. The FormattingInfo object has only three properties:
●IsEndOfGroup - This property is true if the section being formatted is a Group Footer.
●IsRepeatedGroupHeader - This property is true if the section being formatted is a repeated Group Header. Repeated Group Headers appear when a group extends to two or more pages, and the group has been formatted to repeat information that appears in the Group Header on the second, third, etc. pages. This property is only true if the Group Header is the second, third, etc. instance of the Group Header. It is false for the original first instance of the Group Header.
●IsStartOfGroup - This property is true if the section being formatted is a Group Header.
The Report Designer Component |
179 |

When designing your application, be aware that when a section is being formatted, all objects in that section are also being formatted. Also, all other sections and objects outside of the current section are not being formatted. This information can affect how data is displayed in various sections of the report, depending on your code.
Calculating Results
If you are using the Format event to calculate values, do not perform any calculations which require the values to be carried across sections. For example, if you want to display a value in a Text object for the first record in the Details section, add a value to the first value, and display the results for the next record, the result may be incorrect. (This process is known as a running total.)
It is possible for the Format event to be fired multiple times for a section, each time the report is displayed. Whether or not this happens depends on the contents of the section, groupings in the report, and various other common report features. For this reason, a calculation performed in the Format event, may be performed several times before final display, resulting in invalid results if the calculation is dependent on a value created in another record or section.
In general, the Format event should be used primarily for formatting the report. Calculations can be performed, as long as they do not depend on assigning a value to an outside variable or receiving a value from an outside variable. For example, you could evaluate the value of a field in the section, then display a flag based on its value. If Sales values drop below a minimum quota, for instance, they could be displayed in red with a warning message next to them.
Changing the contents of a Text object
Text objects are a common feature of reports designed in the Seagate Crystal Report Designer Component, and the Report Designer Object Model allows you to manipulate those objects at runtime. Use Text objects to simply display information inside a report, or use them to perform complex calculations in Visual Basic and display the results in your report.
Reports can be customized at runtime based on user input, and a Text object may be a simple way of personalizing the data based on your users’ input. Alternately, you may need to leverage the full power of Visual Basic to perform complex operations on database data. The results of such calculations can easily be assigned to a Text object at runtime to produce data that could not be displayed any other way. Only the Seagate Crystal Report Designer Component gives you all of the power of Visual Basic for your reports in a single simple straightforward object.
The following example changes the contents of a Text object during the Load event of the Form containing the Crystal Smart Viewer ActiveX control. This is especially important if the value of your text object is dependent on data in your data source. Since this is also a common place to change the data source used by a report, changing values in Text objects during the Load event often becomes convenient.
Private Sub Form_Load()
Dim Report As New CrystalReport1 ‘ More code here
Report.Text1.SetText “Here is some text from my app” CRViewer1.ReportSource = Report
CRViewer1.ViewReport End Sub
The Report Designer Component |
180 |
Notice that the SetText method is used to apply a new value. The Text property of the object model’s TextObject object is a read-only property, a convenient shortcut to get information about the existing value. However, SetText must be used to assign a new value.
The example above simply assigns a string to the Text1 object in the report. A more complicated technique may be to access data in a database and calculate a new value in Visual Basic, then display that value through a Text object on the report. In such cases, you could design your report in the Report Designer Component design window to specifically supply a Text object for such a result. The following code demonstrates this technique:
Private Sub Form_Load()
Dim maxValue As Currency
Dim maxValueString As String
Dim Report As New CrystalReport1
Dim rs As New ADODB.Recordset
rs.Open “SELECT * FROM Customer”, _ “DSN=Xtreme sample data;”, adOpenKeyset
Report.Database.SetDataSource rs
maxValue = 0
rs.MoveFirst
Do While Not rs.EOF
If rs.Fields(“Last Year’s Sales”) > maxValue Then
maxValue = rs.Fields(“Last Year’s Sales”)
End If
rs.MoveNext
Loop
maxValueString = “The maximum value is “ & _ Format(maxValue, “Currency”)
Report.Text1.SetText maxValueString
CRViewer1.ReportSource = Report
CRViewer1.ViewReport
End Sub
In this example, we are finding the maximum value of the Last Year’s Sales field of the new data source, formatting that value as Currency, then displaying a message containing the value through a Text object on the report. As you can see, Text objects provide many options for controlling the output of data in your reports.
The Report Designer Component |
181 |