
ASP .NET Web Developer s Guide - Mesbah Ahmed, Chris Garrett
.pdf
610 Chapter 13 • Creating a Message Board with ADO and XML
Figure 13.37 Continued
mPostSubject = CStr(myRow("PostSubject"))
mPostBody = CStr(myRow("PostBody"))
mCreator = New User(myRow)
mPostDate = CDate(myRow("PostDate"))
End Sub
Public ReadOnly Property ID() As Long
Get
Return mPostID
End Get
End Property
Public Property Subject() As String
Get
Return mPostSubject
End Get
Set(ByVal Value As String)
mPostSubject = Value
End Set
End Property
Public Property Body() As String
Get
Return mPostBody
End Get
Set(ByVal Value As String)
mPostBody = Value
End Set
End Property
Public ReadOnly Property Creator() As User
Get
Return mCreator
Continued
www.syngress.com

Creating a Message Board with ADO and XML • Chapter 13 |
611 |
Figure 13.37 Continued
End Get
End Property
Public ReadOnly Property PostDate() As Date
Get
Return mPostDate
End Get
End Property
End Class
As you can see, this class has five private fields with the corresponding five public properties. In addition, it has a constructor that accepts a DataRow parameter which passes the DataRow to the inflate method. Finally, it has an update method, with the rule that only the creator of the Post can actually edit the Post. Doesn’t seem too hard, does it?, Especially after all the other classes we’ve dealt with. It almost seems passé.
Designing the MessageBoard Class
We’ve finally gotten every class in our message board object library finished; now all we need is a way to get a list of every Board object from our database.This is accomplished using the MessageBoard class.We won’t bother to show you a UML diagram of the MessageBoard class, as there is only one method in it: GetBoards. Let’s take a look at the code in Figure 13.38 (which can be found on your CD under the name MessageBoard.vb).
Figure 13.38 MessageBoard.vb
Public Class MessageBoard
Public Shared Function GetBoards() As ArrayList
Dim list As New ArrayList()
Dim sql As String
Dim myData As DataSet
Dim myRow As DataRow
sql = "SELECT [BoardName] FROM [Board] ORDER BY [BoardName] Asc"
Continued
www.syngress.com
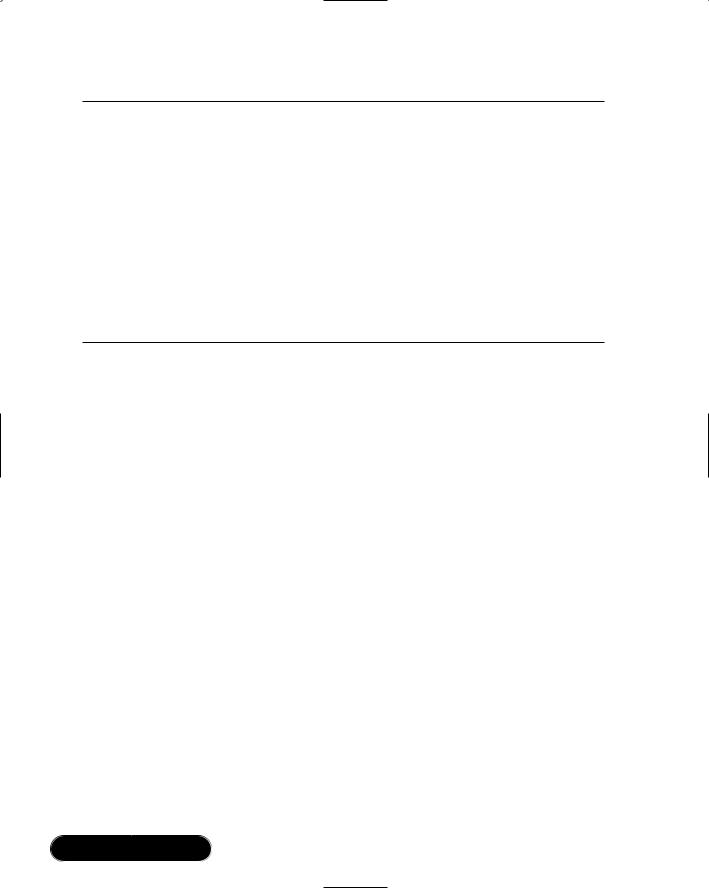
612 Chapter 13 • Creating a Message Board with ADO and XML
Figure 13.38 Continued
myData = DataControl.GetDataSet(sql)
For Each myRow In myData.Tables(0).Rows
Dim myBoard As Board
myBoard = New Board(CStr(myRow("BoardName"))) list.Add(myBoard)
Next myRow
Return list
End Function
End Class
This class is fairly easy to understand.What it does is look up each BoardName from the database, and create a new Board object based on that name. It then adds each Board to its list, and finally returns the list.
That’s it. Every single one of our objects to be used in dotBoard is completely finished.You may wonder why we did all this work ahead of time instead of just jumping into the application itself.That is a very good question, and as such, has a very good answer.We did all this work designing and setting things up so that when we actually build our application, it will go smoothly, quickly, and won’t require a lot of coding in the User Interface. Any good application splits the User Interface from the actual implementation of the application, which is exactly what we did.We are about to move on to the user interface of our message board application.You will see that using the work we’ve already done, the rest of this application is going to be very straightforward and easy.
Designing the User Interface
Finally, we’ve gotten to our User Interface. Our database is constructed. All of our message board classes are created; the final thing to do is to put a UI on top of it all. Just like when we created the classes our applications are going to use, we need to sit and think for a few minutes to determine exactly what it is our message board will do.The obvious requirements are that a user must be able to register, log in, and modify his or her profile. Anyone must be able to browse the Boards,Threads, and Posts. Registered users must be able to create threads and
www.syngress.com
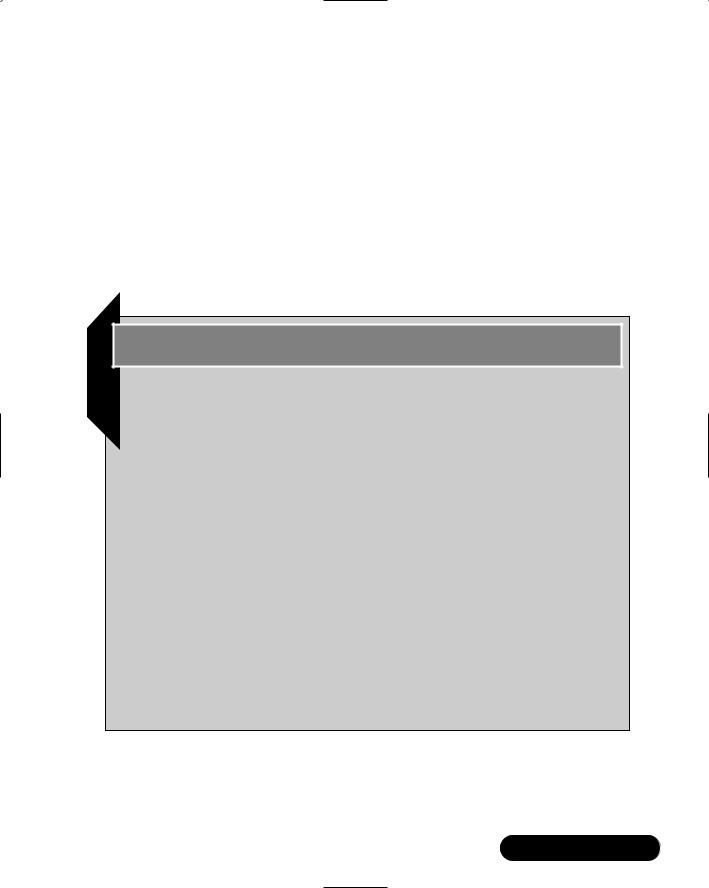
Creating a Message Board with ADO and XML • Chapter 13 |
613 |
posts, and administrators must have the ability to administer users and create and delete boards.
Sound like a lot? Well, since we have a good majority of this work already built into our numerous classes, most of our work now is to create the UI and tie events to methods our objects will handle.The only other thing our message board should be able to do is be “changed” at will.That is, colors, fonts, and any other sort of styling element should be able to be changed without needing to actually modify every single control we place on our form.This will be discussed in a moment, but for now, rest assured, it will be very exciting, and most of the work will be done for us! Let’s start by figuring out how to register and log in.
Developing & Deploying…
Copying ASP.NET Applications to Multiple Computers
If you are using the examples on your CD, please perform the following steps to get your ASP.NET message board up and running on your computer.
■Copy the files from your CD to a folder underneath your WWWRoot folder, typically located at C:\Inetpub\WWRoot. Name this folder dotBoardUI.
■Open up the Internet Services Manager from Administrative Tools in the Control Panel.
■Expand the Internet Information Services node, then your computer’s node, and finally the Default Web Site node.
■Find your dotBoardUI folder. Right-click and select
Properties to bring up the Properties pane.
■Look at the Application Settings panel, and click the Create button next to the grayed out Application Name label and text box.
■Hit OK.
www.syngress.com
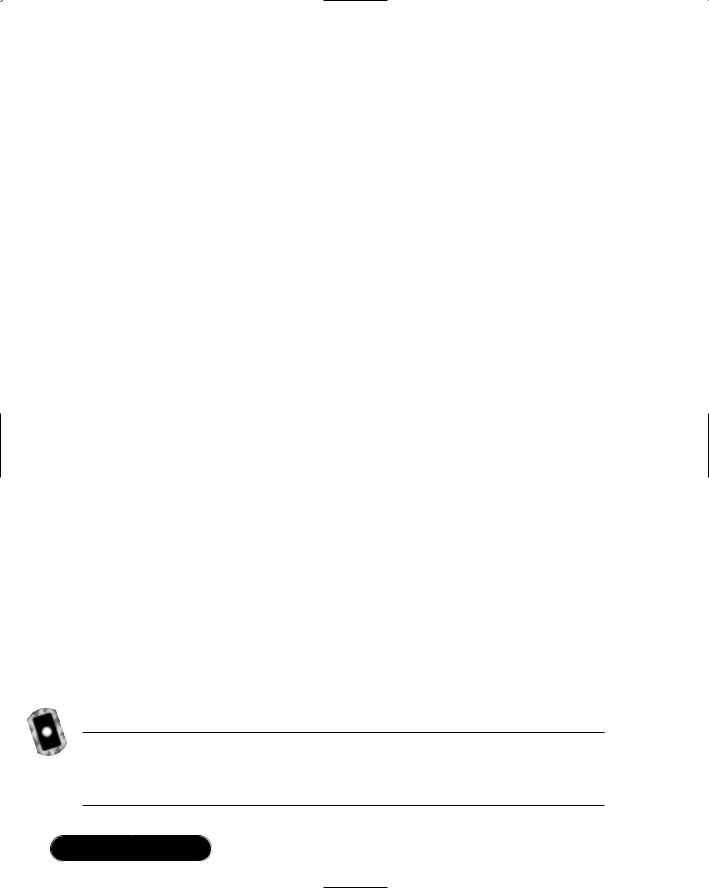
614 Chapter 13 • Creating a Message Board with ADO and XML
Setting Up General Functions
The first step in designing our application is to create the ASP.NET application. You can either get the solution from the CD, or create your own. If you get the files from the CD, they are in a folder called “dotBoardUI” in the Chapter 13 folder.The dotBoard.sln file is the main solution file, and everything else in that folder is a part of the project. Either way, your application should be named dotBoardUI, to go with your dotBoardObjects class library.After you have created your application, add your dotBoardObjects project to your solution, and add a reference to the newly added project to your ASP.NET application. Next, rename Web Form1.aspx to default.aspx.This will make it easier when it comes time to deploy your application, as default.aspx is typically one of the default documents IIS serves when the browser doesn’t request a specific file in your application.
Now that you have your project created and the appropriate references made, let’s get started on the groundwork for our application. If you think about it, every page you make will likely need access to the currently logged in user. There are many reasons for this as you’ll see later, so for now just assume that every page will need that information.There are many ways to do this. For instance, you can copy and paste the code necessary to get this information on every page. Anyone familiar with programming techniques should sense a red flag go up at that statement. Copying and pasting the code is a terrible idea, for so many reasons that we don’t have space to state them here. Another solution available is to create a public module with the common functions your pages would need.This is a good solution, but let’s do it a little differently.We are going to have one Web Form that all our Web Forms will inherit from.Why would we do this? So every Web Form you create will have direct access to the common methods, and every user control you put on these Web Forms will be able to get the information easily.
Add a new class to your project and name it FormBase.vb.We’re not adding a Web Form in this case because we don’t need any sort of UI for our FormBase; we just need access to a common set of methods.Take a look at the basic code in Figure 13.39 (which can also be found on your CD called FormBase.vb).
Figure 13.39 The Basics (FormBase.vb)
Public Class FormBase
Inherits System.Web.UI.Page
End Class
www.syngress.com

Creating a Message Board with ADO and XML • Chapter 13 |
615 |
Pretty easy, right? What we have here is a class that inherits from System.Web
.UI.Page.This allows all our Web Forms to inherit directly from this class, instead of inheriting from System.Web.UI.Page.The next thing we need is for our FormBase to be able to have a reference to the currently logged in user (if there is one). Here is the code to do just that in Figure 13.40.
Figure 13.40 Maintaining the Current User (FormBase.vb)
Private mCurrentUser As dotBoardObjects.User
Public Property CurrentUser() As dotBoardObjects.User
Get
Return mCurrentUser
End Get
Set(ByVal Value As dotBoardObjects.User) mCurrentUser = Value
'add the user's ID to the session Session.Add("userid", Value.ID.ToString())
End Set
End Property
Public ReadOnly Property IsLoggedIn() As Boolean
Get
Return Not mCurrentUser Is Nothing
End Get
End Property
All we have here is a private dotBoardObjects.User object, and a public property to retrieve it.The Set property sets the private field with the value passed in, and adds the user ID of the passed in User object to the session.We do this so a user does not have to log in multiple times while perusing your message board.You’ll see where this comes into play later.The other property we have is one that returns a Boolean value of whether or not there is a currently logged in user. This property makes it easier for someone to determine if there is a logged in user. Basically, instead of having to test for Nothing over and over, you use this Boolean property.
www.syngress.com
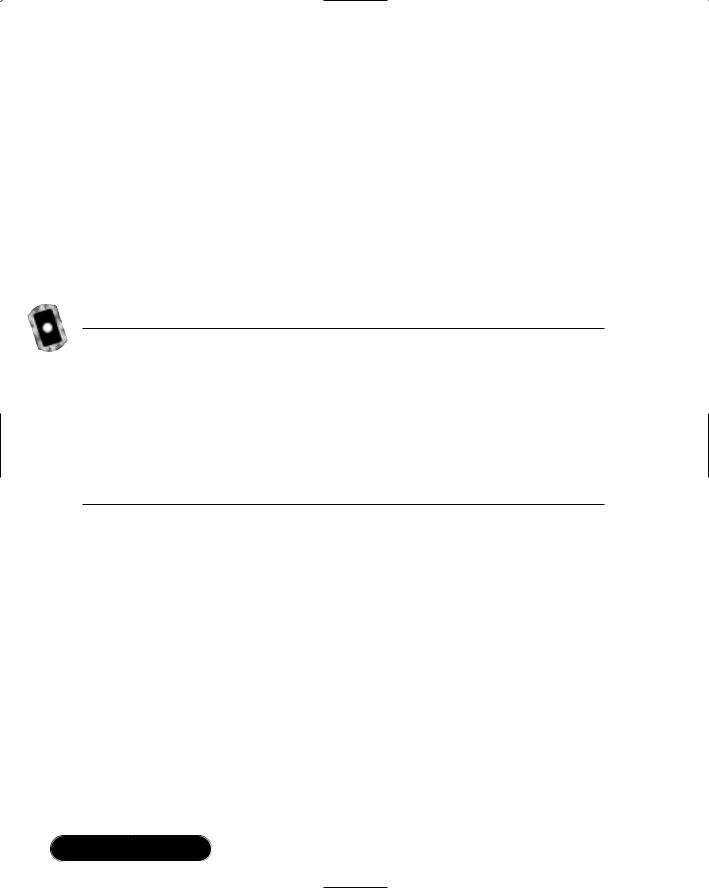
616 Chapter 13 • Creating a Message Board with ADO and XML
That is all the state maintaining we’ll need in our base class.The only other thing our FormBase class needs to do is fulfill that last requirement we talked about.That is, the ability to modify every control on every form without needing to actually rename the class names on elements.This is probably one of the most interesting techniques dotBoard will use. Basically, what we will do is create the code necessary to automate the process of restyling every control in every Web Form.This might sound like a daunting task, but actually once you take a look at it, it is rather simple.The first step we need to take is to open up our web.config file and add the following lines of XML directly beneath the <configuration> tag as shown in Figure 13.41 (which can also be found on your CD called web.config).
Figure 13.41 The web.config File
<appSettings>
<add key="ConnectionString" value="Provider=Microsoft.Jet.OLEDB.4.0;
DataSource="C:\Location\To\Your\database\dotBoard.mdb;
User ID=Admin;Password=;" />
<add key="XmlConfigFile"
value="C:\Inetpub\WWWRoot\dotBoardUI\styles.xml" />
</appSettings>
Okay, now what exactly does that mean? Your <appSettings> are custom settings you create and have access to in your application.We are creating two custom settings, which are added using the <add> tag.The key attribute is the name of the settings, and the value attribute is obviously the value. Here we are adding two keys, ConnectionString and XmlConfigFile. ConnectionString is what you use to connect to your database with. Remember the DataControl class and how it accessed System.Configuration.ConfigurationSettings? The ConnectionString key is exactly what that class will use.The other key is XmlConfigFile, which is used to hold the location to your XML file that will hold the style information we discussed earlier. Please change the values of each to represent where you actually have the files on your computer located.
We now have the ConnectionString and XmlConfigFile keys added to our appsettings. Let’s start discussing how we will accomplish the “sweeping” change of styles, without needing to manually apply any styles on your controls. First
www.syngress.com

Creating a Message Board with ADO and XML • Chapter 13 |
617 |
take a look at the following Cascading Style Sheet (CSS) file you should add to your project, as shown in Figure 13.42.
Figure 13.42 Styles.css
body
{
font-family:Tahoma, Arial, Sans-Serif; font-size:10pt;
color:#000000;
}
.errors
{
font-family:Tahoma, Arial, Sans-Serif; font-size:10pt;
color:#993300;
}
.link
{
text-decoration:underline; font-family:Tahoma, Arial, Sans-Serif; color:#FF9933;
}
.header
{
color:#003399;
font-size:16pt; font-weight:bold; font-family:Arial, Sans-Serif;
}
.panel
{
border: 1px solid #000000; padding: 10px;
}
Continued
www.syngress.com

618 Chapter 13 • Creating a Message Board with ADO and XML
Figure 13.42 Continued
.inputBox
{
border: 1px solid #000000; background-color:#e5e5e5;
}
.label
{
font-family:Tahoma; font-size:8pt;
color:#000000;
}
.button
{
border: 1px solid #000000; background-color: #FF9933; color: #000000;
font-family: Arial, Sans-Serif; font-size: 10pt;
}
You can see here that we have a number of styles we will want to apply to many different elements throughout our application. Manually setting these styles is hardly desirable, and maintaining these settings if any of your class names change would be a nightmare. So, what can be done to prevent us from having to maintain this? Enter the styles.xml file in Figure 13.43 (which can also be found on your CD under the name Styles.xml).
Figure 13.43 Styles.xml
<?xml version="1.0" encoding="utf8"?>
<styles>
<control type="System.Web.UI.WebControls.Label">label</control>
<control
type="System.Web.UI.WebControls.TextBox">inputBox</control>
<control type="System.Web.UI.WebControls.Button">button</control>
Continued
www.syngress.com
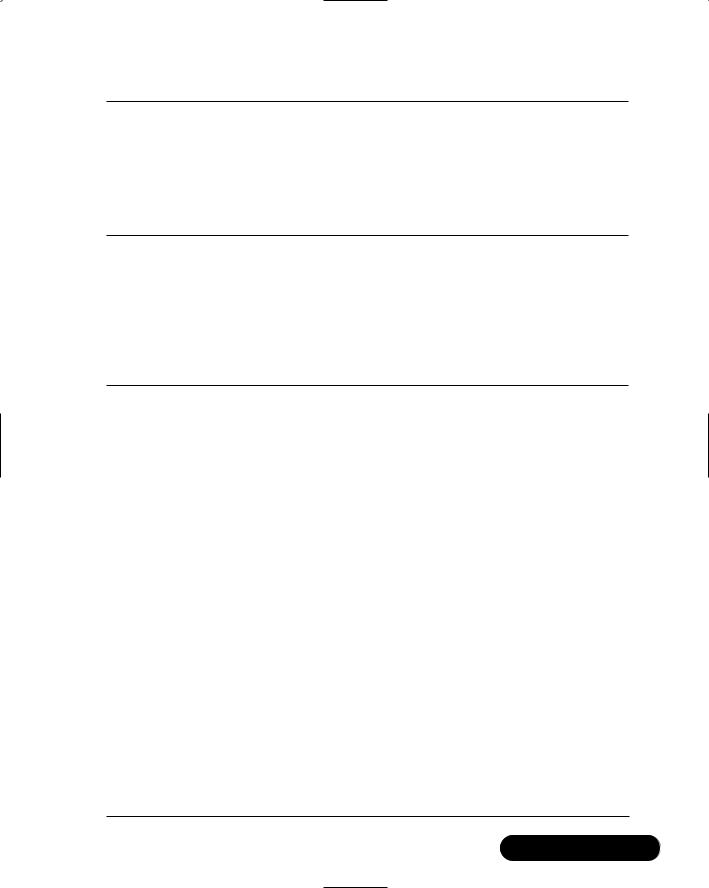
Creating a Message Board with ADO and XML • Chapter 13 |
619 |
Figure 13.43 Continued
<control type="System.Web.UI.WebControls.Panel">panel</control>
<control type="System.Web.UI.WebControls.LinkButton">link</control>
<control
type="System.Web.UI.WebControls.ValidationSummary">
errors</control>
</styles>
You should now notice that the values of these XML tags correspond to an appropriate class name in the preceding stylesheet declaration. Now all we need to do is find a way to associate these XML tags with the appropriate controls on every single page.We can accomplish this through two methods, as shown in Figure 13.44.
Figure 13.44 Two Methods to Dynamically Apply Styles to Controls (Board.vb)
Public Sub ApplyStyles(ByRef objControls As ControlCollection) If objXml Is Nothing Then
Dim xmlLoc As String
xmlLoc = ConfigurationSettings.AppSettings()("XmlConfigFile") objXml = New XmlDocument()
Try
objXml.Load(xmlLoc) Catch E As Exception
Throw New Exception("XML Style Config file not found")
End Try
End If
Dim objControl As Control
For Each objControl In objControls
Dim style As String
style = GetStyleName(objControl.GetType.ToString())
If style <> "" Then
Dim objWebControl As WebControl
objWebControl = CType(objControl, WebControl)
Continued
www.syngress.com