
книги2 / Ilyin-Fundamentals-and-methodology-of-programming
.pdfy = -- x ; cout << y << x << endl ; // output y = 5, x = 5, because x decreases by 1, then y = x .
y = x ++; cout << y << x << endl ; // output y = 5, x = 6, because the value y = x is assigned , then x is increased by 1.
Real numbers
In most languages, the integer and fractional parts of a real number are separated by a dot.
Example:
float f = 987.654;
cout . width (10); // width 10 characters
cout . precision (5); // significant 5 characters cout << f << endl ; // output: _ _ _ _ 987.65
In scientific calculations, real numbers are often represented in exponential format. Example:
float x = 987.654; cout.width(10);
cout.precision(2); // in the fractional part cout << scientific << x; // _9.87e+002
Standard Math Functions
To connect the mathematical library of the C language, you need to write the following line:
#include <math.h> // or < with math>
...
abs ( x ) — modulus of an integer; fabs ( x ) — module of a real number; sqrt ( x ) — square root;
sin ( x ) — sine of the angle specified in radians; cos ( x ) — cosine of the angle specified in radians; exp ( x ) — exponent ex;
ln ( x ) — natural logarithm;
30

pow ( x , y ) — raising the number x to the power y ; floor ( x ) — rounding down;
ceil ( x ) — rounding up
Pseudorandom numbers
In a number of tasks (simulation modeling, modeling of complex processes, etc.) it is necessary to obtain a random value in a certain range (random number sensor (RNS)). For these purposes , the library function rand () is provided , returning a “pseudorandom” number in the range from 0 to RAND_MAX (for the MS compiler Visual Studio constant value is 32767).
Listing. Random Number Sensor (RNS)
#include <iostream> #include <ctime> using namespace std; void main()
{
system("cls"); // clear screen srand(time(0)); cout<<RAND_MAX<< endl;
int a = rand()%1000; cout << a; system("pause");
}
Test questions and general tasks:
1.Define an algorithm. List the properties of the algorithm.
2.Name ways to write algorithms.
3.Name the basic algorithmic structures and give them a brief description.
4.List the main programming paradigms.
5.Provide basic C ++ data types.
6.How do you stream data input and output in C++?
7.Describe the input and output format in C language.
8.Describe the rules for evaluating an algebraic expression.
9.Explain basic logical operations and give examples of their use in programs.
31

10. General task. Organize the calculation of the area of a rectangle (data input, calculation, data output).
Conditional operator
In some cases, it is necessary to implement the choice of one of two possible paths of program execution depending on some condition. To check the condition, a logical statement (expression) is formulated, which can be true (True) or false (False) (Fig. 1.8).
Fig. 1.8. Full form of branching organization
The syntax for the full form of branching is:
if <logical expression> { exptession 1; }
else
{ exptession 2; }
The shorthand syntax for branching is:
if <logical expression> { statement block; }
To organize a logical expression, relation signs are used:
•Equality ==
•Inequality !=
•Greater than or equal to >=
•Less than or equal to <=
•More >
•Less <
32

Listing. Maximum of two numbers
void main ()
{
int a , b , max ;
printf ("Entering numbers\ n "); scanf("%d%d", &a, &b );
if (a > b) max = a;
else
max = b;
printf ("Maximum number = % d ", max );
}
It is possible to use nested conditions:
if (< boolean expression 1>)
{
operator block 1;
}
else if (< Boolean expression 2>)
{
operator block 2;
}
else
{
operator block 3;
}
The logical expression in a conditional statement can be as complex as desired. A complex condition is a condition consisting of several simple conditions (relations) connected using basic logical operations:
! – NOT (not, negation, inversion);
&& – AND (and, logical multiplication, conjunction, simultaneous fulfillment of conditions);
|| – OR (or, logical addition, disjunction, fulfillment of at least one of the conditions).
33

The programming language defines the order in which complex conditions are executed:
•expressions in brackets
•! (NOT, negation)
•<, <=, >, >=
•==, !=
•&& (AND)
•|| (OR)
Example: condition for hiring employees aged 16–65 years (inclusive).
if (v >= 16 && v <= 65) cout << "fits";
else cout << "not suitable";
A shortened way of writing the conditional operator ("? :") is also possible . Syntax operator :
"condition" ? "expression 1" : "expression 2" .
The conditional operator (?:) takes three operands. The first operand is evaluated and implicitly converted to type bool . If the result of the first operand is true (1), then "expression 1" is executed . If the result of the first operand is false (0), then "expression 2" is executed .
Listing. Maximum of two numbers
int i |
= |
1, j = 2; |
|
int |
max |
= 0; |
|
i > |
j |
? |
max = i : max = j; |
|
cout |
<< max << " is max"; |
The above code can be replaced with the following:
cout << ( i > j ? i : j ) << " is max";
34

Example: Finding a Positive Number
int i=3;
int j=(i>0) ? eleven;
The above code can be replaced with the following:
if (i>0) j=1;
else j=-1;
Multiple selection operator (switch)
switch selection statement is a replacement for the multiple use of if statements
. The operator is designed to organize a choice from many different options. The key variable in parentheses is compared with the values described after the case statement
. If none of the keys is equal to the expression, then control is transferred to the default operator . The break operator ensures that the execution of the innermost of the switch, do, for, and while statements that unite it is terminated .
Listing. Select one of 4 options
#include <iostream> using namespace std; void main()
{
int key; int a,b;
cout << "First number: "; cin >> a ;
cout << "Second number: "; cin >> b ;
cout << "1-addition; 2-subtraction; 3-multiplication; 4-divi- sion: ";
cin >> key; switch (key)
35
{
case 1:
{
cout << a << " + " << b << " = " << a + b << endl; break;
}
case 2:
{
cout << a << " – " << b << " = " << a – b << endl; break;
}
case 3:
{
cout << a << " * " << b << " = " << a * b << endl; break;
}
case 4:
{
cout << a << " / " << b << " = " << float (a) / b << endl; break;
}
default:
cout << "Invalid input" << endl;
}
system (" pause ");
}
Test questions and general tasks:
1.Draw a block diagram of a fork (complete).
2.Draw a block diagram of the fork (incomplete).
3.What type of expression can act as a condition when organizing branching?
4.What is a complex condition?
5.When is it appropriate to use the switch multiple choice operator ?
6.General task. Find the sum of the maximum and minimum values of the three entered numbers.
Cycles (Loops)
Cyclic algorithms are designed for repeated execution of program fragments. From a theoretical point of view, there are two types of cycles:
• cycles with an unknown number of steps (with precondition and postcondition);
36

•cycles with a known number of steps.
1.Loop with precondition
The block diagram of a cycle with a precondition is shown in Fig. 1.9. In the diagram, the “loop body” is one or more operators, in the general case a compound operator. A condition (logical expression) is an expression of the condition for completing a loop. The body of the loop is executed as long as the boolean expression evaluates to true. When the boolean expression is false (equal to 0), control is transferred to the statement behind the loop.
Fig. 1.9. Block diagram of a loop with a precondition
Syntax:
while (<Condition: while the condition is true, execute>)
{
Loop Body
}
Listing. Converting units of measurement (loop with precondition)
#include <iostream> using namespace std; int main()
{
int sm(0);
37
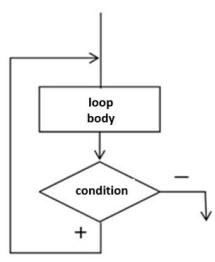
double d; while (sm<100)
{
sm++;
d=sm/2.54;
cout << sm <<" centimeters equals "<< d <<" inches\ n ";
}
return 0;
}
2. Loop with postcondition
The block diagram of a cycle with a postcondition is shown in Fig. 1.10. The diagram shows that when entering the loop, the condition is not checked and the body of the loop is always executed at least once. As soon as the condition (logical expression) becomes false (i.e. equal to 0), the loop ends and control is transferred to the next operator in order.
Fig. 1.10. Block diagram of loop with postcondition (C/C ++)
Syntax
do
{
Loop body
}
while (<Condition: while the condition is true, execute>)
38

Listing. Converting units of measurement (loop with postcondition)
#include <iostream> using namespace std; int main()
{
int sm(0); double d; do
{
sm++;
d=sm/2.54;
cout << sm <<" centimeters equals "<< d <<" inches \n";
}
while (sm<100); return 0;
}
3. Cycle with parameter
If the number of repetitions (iterations) of a loop is known in advance, then it is more convenient to use a loop with a parameter. Its block diagram is shown in Fig. 1.11.
Fig. 1.11. Block diagram of a loop with a parameter
Syntax:
for (expression_1; expression_2; expression_3)
{
Loop body;
}
39