
книги2 / Ilyin-Fundamentals-and-methodology-of-programming
.pdfAre common
<algorithm> module – implements definitions of many algorithms for working with containers.
<iterator> module – implements classes and templates for working with iterators.
String
<string> module – implements standard string classes and templates.
The Standard Template Library (STL) is a subset of the C++ Standard Library. STL objects are declared within the namespace STD (Standard Template Definition).
Connecting library functions
In any language there is a way to connect to the program various structures used in another module.
For example,
#include <iostream>
#include directive is used to include other files in the code. The <iostream> module is in the "standard library" and is responsible for standard input streams and console output.
Header file , or included file, is a file “inserted” by the compiler into the source text in the place where the #include <file.h> directive is located (Extension.h ; sometimes
.hpp.).
20
TOPIC 3. BASIC STRUCTURES OF ALGORITHMS
Variables and data types
A variable is a value that has a name, type, and value. The value of a variable can be changed while the program is running. A variable (C/C++) stores data of a certain type. The variable characterizes:
•size of the allocated memory area and data storage format;
•the range of values it can store;
•a set of operations with this variable.
Data types:
• int // integer
• long int // long integer
•float // real
•double // double precision real
•bool // logical values
•char // character
•and etc.
Declaring a variable is defining a name, type, and allocating space in memory. The initial value can be specified directly in the variable definition statement (initialization).
Declaring a variable in C / C ++: Specifies the data type for that variable, then the name of that variable. If we declare, for example, a variable of type int , then at the machine level it is described by two parameters – its address and the size of the stored data.
int a; // declaration of a variable a of integer type
float b; // declaration of variable b of floating point data type double c = 9999.99; // initialization of a double type variable char d = 's'; // initialization of a variable of type char
bool k = true; // initialization of the logical variable k
Note that when memory is allocated, global variables are filled with zeros, and local variables contain “garbage” (remaining data in memory from other programs).
The most important operator is the assignment operator.
21
An assignment operator is a command to write a new value to a variable.
int i = 1024; or int i ( 1024 );
string p = "Hello"; // or string p("Hello");
Built-in data types have a special syntax for specifying a null value:
int i = int(); double d = double();
Variable i gets the value 0, and variable d gets the value 0.0
There are also special variables for storing memory addresses – these are pointers . Pointer declaration syntax:
<type> *<name>;
An example of a pointer declaration is shown below:
int a = 5; // variable of integer type
int * p ; // declaration of a pointer (from English Pointer )
p = & a ; // the address of an existing static variable is written to the pointer
Data recording formats
The octal format is described by the prefix "0" . For example:
int b = 010; // 8th SS
cout << b +1 << endl ; // 9
The hexadecimal format is described by the prefix "0x" . For example: int a = 0xFFFFFF; // hexadecimal
cout << a + 1 << endl; // 16777216
Description of constants
The const keyword can be added to an object's declaration to make that object a constant rather than a variable.
const int m = 1;
22

Input and output (C library functions)
Format is a character string that shows what types of data the input/output is organized for. A distinctive feature of the format is the presence of the "%" symbol in front of it:
printf ("%d", var); // output on display
Formats:
•%d – signed integer in decimal notation;
•%x – signed integer in hexadecimal notation;
•%o – signed integer in the octal number system;
•%f – real number;
•%c – symbol;
•%s – character string;
•and etc.
Printing data in a specified format:
int a = 1234;
int b = 1234.567;
printf ("%8d", a); // total 8 positions
printf ("%9.4f", b); // 9 positions in total, 4 fractional digits
scanf () function implements formatted input by passing the input format string and memory cell addresses.
scanf("%d%d", &a, &b);
Listing. Finding the sum and difference of two numbers
#include <stdio.h> #include <conio.h> void main()
{
int a, b, c;
23
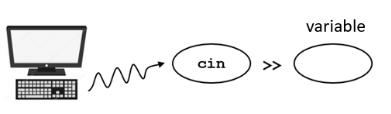
printf ("Enter two integers\ n "); scanf("%d%d", &a, &b);
c = a + b;
printf("Amount: %d \n ", c); printf("Difference: %d-%d=%d", a, b, a-b); getch ();
}
Input and output streams ( C ++ language library functions)
Data stream in programming is an abstraction used for read and write operations (i.e. moving data from source to destination).
The module <iostream> supports file input/output of built-in data types. I/O operations are performed using: istream class (streaming input), ostream class (streaming output), iostream class supports bidirectional I/O.
The library defines standard stream objects:
1. Data entry. cin object belongs to the istream class and corresponds to the standard input stream, which in general allows you to read (extract) data from the terminal (keyboard). Input is done using the overloaded bit shift operator >>. In C ++ language, operations can be overloaded, i.e. can perform different actions depending on the context in which they occur.
Fig. 1.6. Reading (extracting) data (>>) from standard input using c in object
Example: data entry
cin >> a;
cin >> a >> b;
2. Data output. An object cout belongs to the ostream class and corresponds to the standard output stream, which in general allows you to output (put) data to the
24
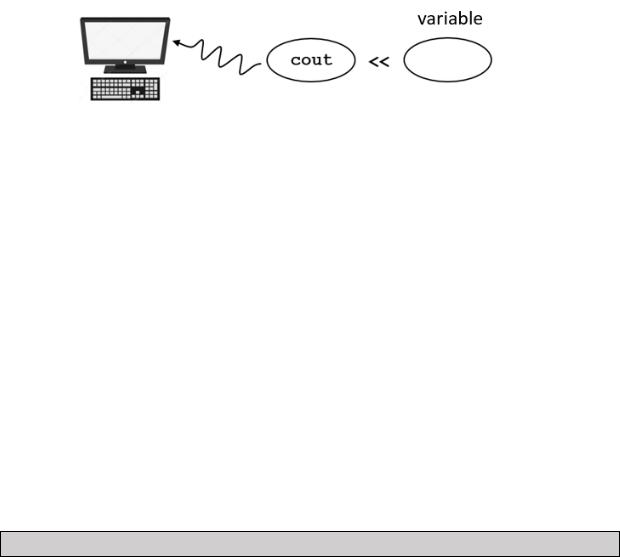
terminal. The output is done using the overloaded left shift operator << . In this case, this is a write operation to a stream (put data into a stream).
Fig. 1.7. Writing data ( << ) to standard output using cout
Example: Data Output
cout << a;
cout << " Answer : " << c;
There are also additional objects, for example, cerr – an object of the ostream class , corresponding to the output of program error messages.
In practice, the names of user variables may coincide with the names of standard programming language library objects. Therefore, a namespace is introduced – an additional identifier to any names declared in it to prevent name collisions. Used when using libraries developed by various independent vendors. If, for example, you do not include the namespace of the standard template library “using namespace std” , then you will have to separately register std::“object”.
For example, std::cout << "Programming";
Listing. Finding the sum and difference of two numbers (version 2)
// without using namespace std #include <iostream> #include <iomanip>
void main()
{
int a, b, c; std::cin >> a >> b; c = a + b;
std::cout << std::setw(10) << c; // set width – set width std::cout << a << "-" << b << "=" << a – b;
system (" pause ");
}
25
Namespaces can be at the level of a specific programming language library, for example:
using namespace System;
...
Console::WriteLine("C++/CLI language (Visual C++)");
There may also be custom ones, for example:
...
namespace X{int a = 2, b = 8;}; namespace Y{int b = 3;};
using namespace X; using namespace Y; int main()
{
Y::b = X::b / X::a;
printf("%d", Y::b); // Print : 4
}
Cyrillic in the console
Text written in Latin in the Windows command line will be displayed correctly. And if the text message is written in Cyrillic, then instead of the transmitted message, an “incomprehensible” sequence of letters and symbols will be displayed. Reason: use of different character encoding tables (cp866, cp1251, utf-8, etc.). SetConsoleCP() function, setting the locale ( setlocale() function performs character conversion in accordance with the required language).
Option 1:
# include < Windows.h >
...
SetConsoleCP (1251); // set the win code page – cp 1251 to the input stream
SetConsoleOutputCP (1251); // set the win code page – cp 1251 to the output stream
26

Option 2:
#include <iostream>
...
system("chcp 1251");
...
Option 3:
#include <locale.h>
...
setlocale(LC_ALL,"RUS");
// setting the locale
...
Command Line Options
When running a program from the command line, it is possible to pass parameters to it in text form. Parameters are described as arguments to the main () function. The first argument argc is the number of parameters passed to the program. The second argument * argv [] is a pointer to an array storing the remaining parameters (see section 3 for more information on pointers). In fact, argc is the array counter * argv [].
Listing. Passing parameters on the command line
#include <iostream> using namespace std;
void main(int argc, char *argv[])
{
for (int i = 0; i < argc; i++) { // Output arguments in a loop
cout << "Argument " << i << " : " << argv[i] << endl;
}
}
Next, I launch the compiled program file from the command line, passing parameters, for example:
C :\> test \ test 1. exe 5 0 1 2 3 4
27
The output will be as follows (with argv [0] containing the name of the program
file):
Argument 0 : test\test1.exe
Argument 1:5
Argument 2: 0
Argument 3: 1
Argument 4:2
Argument 5:3
Argument 6:4
Evaluating Expressions
C/C++ uses linear notation of expressions with the following priority (precedence) of operations :
•brackets;
•multiplication and division;
•addition and subtraction.
The division operation ( C / C ++) has a special feature: the result of dividing an
“integer by an integer” is an integer (the data type is determined by the operands).
Example :
int |
x |
= |
3, |
y |
= |
4; |
|
float |
z; |
|
|
|
|
||
z = |
3 |
/ 4; // = |
0 |
||||
z |
= |
3.0 |
/ 4; // |
= 0.75 |
|||
z |
= |
float( |
x ) |
/ 4; // = 0.75 |
If you need to get the remainder of a division, use the % operation. Example:
17 |
= 8 |
* 2 |
+ 1 |
||
17 |
/ |
2 |
= |
8 |
(whole part) |
17 |
% |
2 |
= |
1 |
(remainder) |
Example:
int a, b, d;
28
d = |
75; |
|
|
|
|
b = |
d |
/ |
10; // |
output |
7 |
a = |
d |
% |
10; // |
output |
5 |
In C/C ++, abbreviated notations of operations are used (primarily this is justified by the convenience of the computational process, and not by the brevity of the notation):
int x, y ;
...
x ++; // full form x = x + 1; x --; // full form x = x – 1;
x += y ; // full form x = x + y ; x -= y ; // full form x = x – y ; x *= y ; // full form x = x * y ; and etc.
The abbreviated notation of the operation can be located to the left or to the right of the object, according to which a prefix-increment and a post-increment are distinguished.
The post-increment operation (i++) returns the value of variable i before the increment was performed, and the prefix-increment operation (++i) returns the value of the variable that has already been modified.
Example :
int a = 10; int b = 10;
cout << a++ << endl; // output 10 cout << ++b << endl; // output 11
In the above example, the result of the program will be the output of the numbers 10 and 11, since the post-increment operation returns the value of the incremented variable before the increment is performed.
Example :
int x = 5; int y;
y = ++x; cout << y << x << endl; // output y = 6, x = 6, t . to . first x increases by 1, then y = x .
29