
- •Using UserForms
- •Creating UserForms
- •Adding and Manipulating Controls
- •Setting Properties
- •Types of Controls
- •The Label Control
- •The TextBox Control
- •The ComboBox Control
- •The ListBox Control
- •The CheckBox Control
- •The OptionButton Control
- •The ToggleButton Control
- •The Frame Control
- •The CommandButton Control
- •The TabStrip Control
- •The MultiPage Control
- •The ScrollBar Control
- •The SpinButton Control
- •The Image Control
- •Controls in the Sample Application
- •Naming Controls
- •UserForm Events
- •Your First Event Procedure
- •Useful Events
- •Events in the Sample Application
- •Working with Forms in AutoCAD
- •Launching a Form
- •Filling a ListBox
- •Selecting a Line
- •The Call Stack
- •The Locals Window
- •Finishing Touches
- •Automatically Loading a VBA Project
- •Automatically Running a VBA Procedure
- •Protecting Your VBA Code
- •Importing and Exporting Components
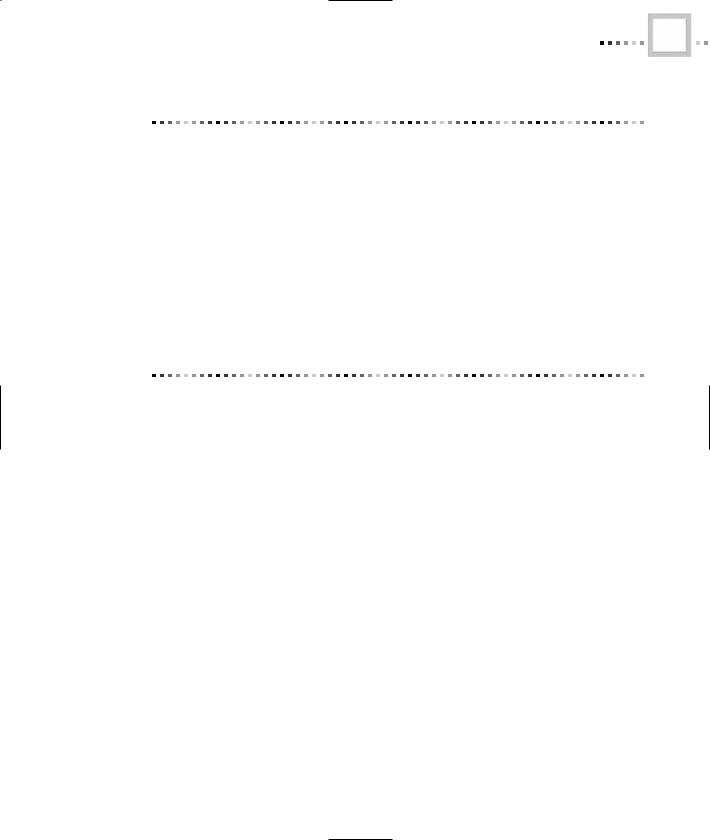
USERFORM EVENTS |
21 |
Table 99.1 (Continued) Common Control Prefixes
CONTROL TYPE |
PREFIX |
CheckBox |
chk |
OptionButton |
opt |
ToggleButton |
tgl |
Frame |
fra |
CommandButton |
cmd |
TabStrip |
tbs |
MultiPage |
mpg |
ScrollBar |
scr |
SpinButton |
spn |
Image |
img |
UserForm Events
Events provide the glue between your application and the user’s actions. Event-driven programming is one of the basic paradigms of the Windows environment, and VBA is designed to work with this paradigm. The basic idea is simple. As the user works with the application, events are passed back to the code. For example, clicking a command button, typing in a text box, or selecting an item in a list box, all these actions generate events. It’s up to you, the developer, to decide which events to respond to and how to respond to them.
In this section, you’ll learn how to write event procedures, which are the bits of code that respond to events.
Your First Event Procedure
To understand event procedures, let’s build a very simple one by following these steps:
1.Create a new UserForm.
2.Add a single command button to the UserForm.
3.Double-click the command button. This puts you into the UserForm’s module.
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |
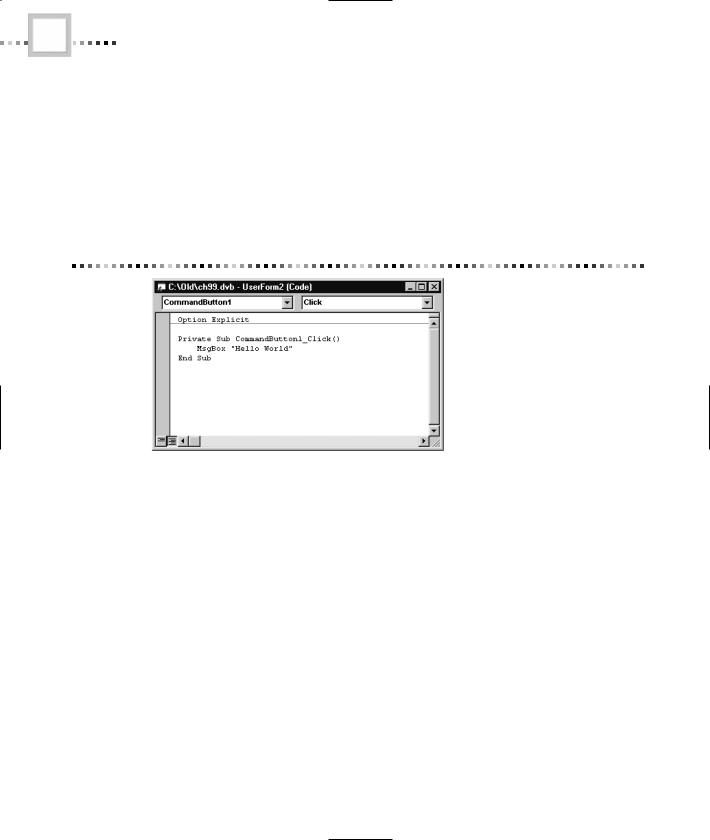
22 |
CHAPTER NINETY-NINE • USING VBA TO CREATE AUTOCAD APPLICATIONS |
4.Type the line of code MsgBox “Hello World” between the Sub and End Sub lines that were generated for you in the module. Figure 99.20 shows how the UserForm’s module will look at this point.
5.Click the Run Sub/UserForm button on the VBA standard toolbar. This launches your UserForm over your AutoCAD drawing.
6.Click the CommandButton1 button on the UserForm. You’ll see a message box with Hello World in it.
Figure 99.20 Creating an event procedure
Let’s take a closer look at the event procedure you just created. Here’s the code:
Private Sub CommandButton1_Click()
MsgBox “Hello World”
End Sub
Note the following characteristics of this code:
•The event procedure is a Sub, not a Function. This is a general rule of event procedures, because there’s no place a return value could go.
•The name of the procedure, CommandButton1_Click, is constructed by VBA out of the control name and the event name. If you change this name, the event stops working.
•Whenever the specified event happens, the code in the event procedure runs. If there’s no event procedure with the correct name, then VBA simply discards the event.
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |

USERFORM EVENTS |
23 |
Be sure to assign names to your controls before you write any event procedures for them. If you rename a control after creating an event procedure for it, the procedure will no longer be “hooked up” to the control.
Useful Events
VBA supports many, many events. Each control type, in fact, has its own set of events to which it can respond. The two combo boxes at the top of the UserForm’s modules window let you select a control (in the left-hand box) and see all of its events (in the right-hand box). As Figure 99.21 shows, a command button has an extensive list of events. Each of these events is a potential place for you to create code.
Figure 99.21 CommandButton events
Not all events are of equal importance. You’re unlikely, for example, to need the MouseMove event of the CommandButton control in most applications. Rather than bury you under an exhaustive list of events, Table 99.2 lists a few of the most useful events for AutoCAD applications. By using these events, you can handle most user interface problems.
Table 99.2 Useful Control Events
CONTROL TYPE |
EVENT |
HAPPENS WHEN... |
CheckBox |
Click |
The user clicks the check box. |
ComboBox |
Change |
A new value is selected in the combo box. |
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |
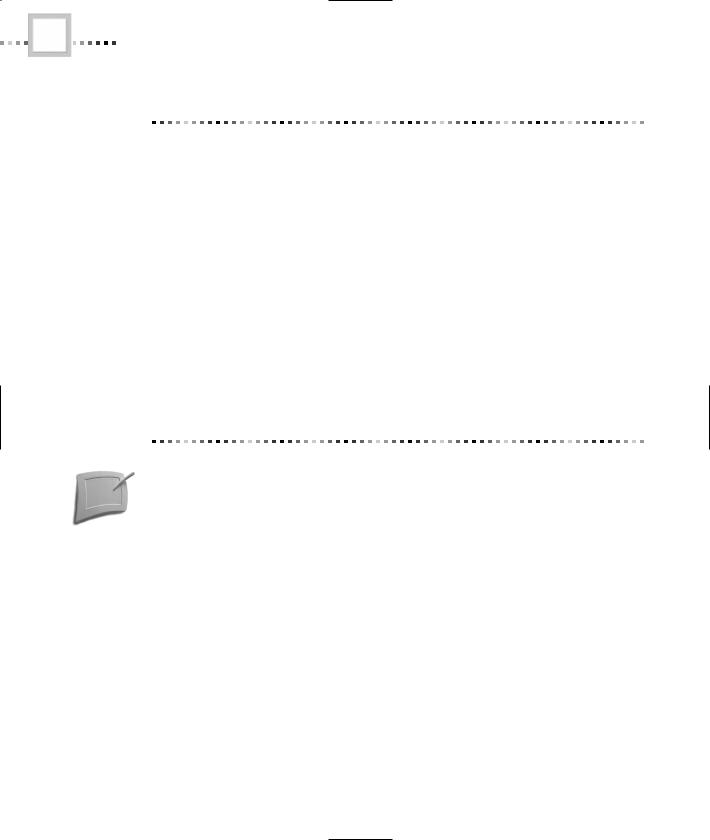
24 |
CHAPTER NINETY-NINE • USING VBA TO CREATE AUTOCAD APPLICATIONS |
Table 99.2 (Continued) Useful Control Events
CONTROL TYPE |
EVENT |
HAPPENS WHEN... |
ComboBox |
DblClick |
The user double-clicks the combo box. |
CommandButton |
Click |
The user clicks the command button. |
Image |
Click |
The user clicks anywhere on the image. |
ListBox |
Change |
A new value is selected in the list box. |
ListBox |
DblClick |
The user double-clicks the list box. |
MultiPage |
Change |
The user selects a new page in the MultiPage control. |
OptionButton |
Click |
The user clicks the option button. |
ScrollBar |
Change |
The user moves the thumb in the scroll bar. |
SpinButton |
Change |
The user presses either button in the spin button. |
SpinButton |
SpinUp |
The user presses the up button in the spin button. |
SpinButton |
SpinDown |
The user presses the down button in the spin button. |
TextBox |
Change |
The user changes the value in the text box. |
ToggleButton |
Click |
The user clicks the toggle button. |
In addition to control events, there are also UserForm events. For example, the UserForm KeyPress event can be used to sense keystrokes anywhere on the UserForm.
Using the Object Browser to Learn about
Events
The events listed in Table 99.2 are just a sample of the events available from controls on UserForms. VBA provides a tool called the Object Browser that allows you to learn more about objects, including controls. Let’s look at the Object Browser and see how you can use it to learn more about available events.
To launch the Object Browser, choose View Object Browser or press the shortcut key F2. Figure 99.22 shows the Object Browser displaying the events of a particular control type.
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |
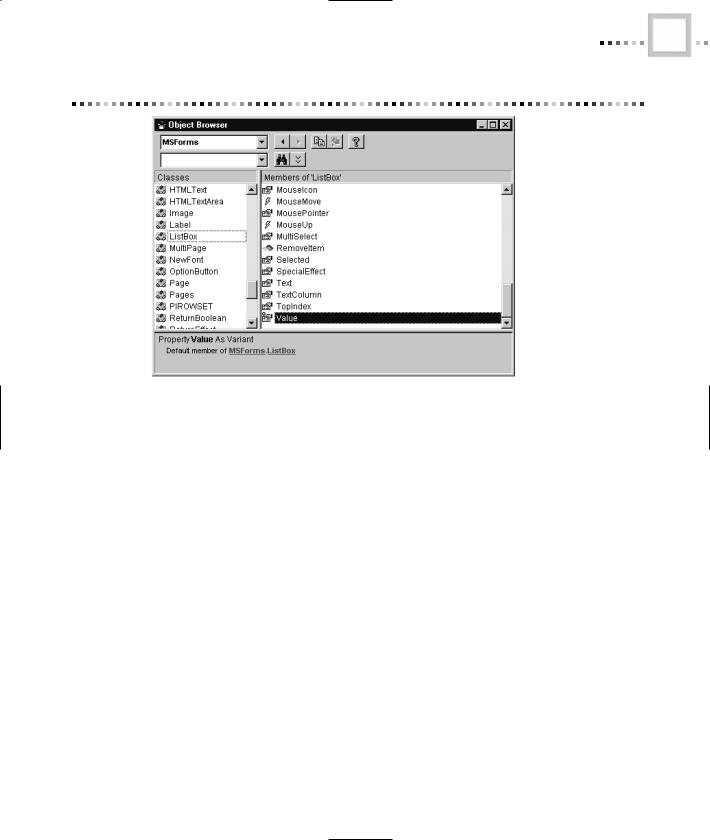
USERFORM EVENTS |
25 |
Figure 99.22 The Object Browser
As its name suggests, the Object Browser displays information about the objects available to your application. This includes AutoCAD objects, VBA objects, and UserForm objects. The combo box at the top of the Object Browser allows you to select an individual library of objects to investigate. In this case, the MSForms library is chosen. MSForms is the internal name of the Microsoft Forms 2 library.
The Classes section on the left side of the Object Browser window shows all of the objects contained in this particular library. In this case, the ListBox object is chosen and information is displayed about the ListBox control implemented by this particular library.
The Members section on the right side of the Object Browser window shows detailed information about the object selected in the Classes section. There are three types of information shown in this section, distinguished by unique icons:
•The Property Sheet icon indicates a property: Text, TextColumn, Value, and so on, are properties. The little blue ball next to the icon for the Value property indicates that it’s the default property for this object.
•The green “flying eraser” icon indicates a method: AddItem, Clear, and RemoveItem are all methods of the ListBox control.
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |
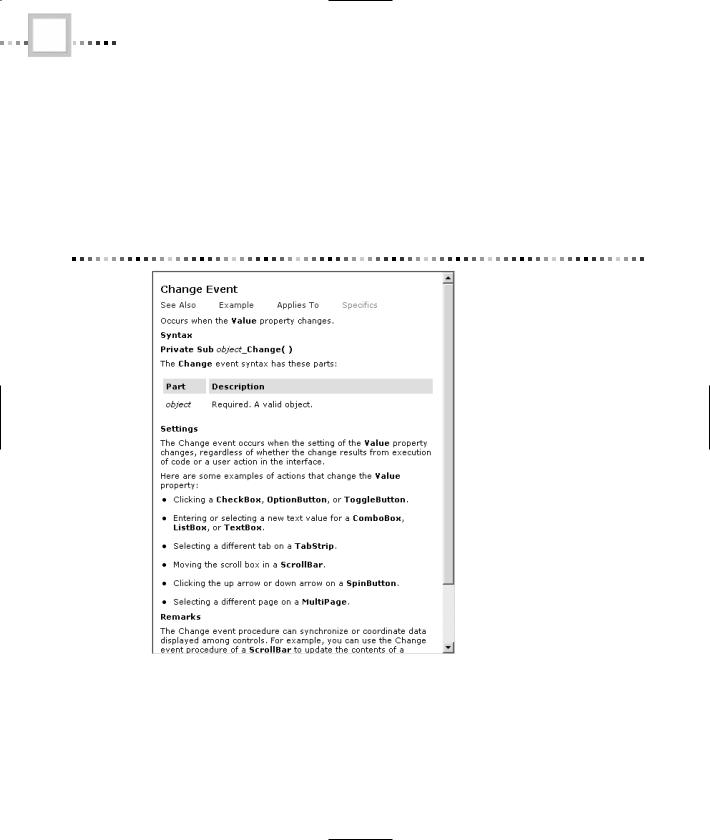
26 |
CHAPTER NINETY-NINE • USING VBA TO CREATE AUTOCAD APPLICATIONS |
•The lightning bolt icon indicates an event. The events of the object in this figure start with BeforeDragOver and continue.
The Help button at the top of the Object Browser window will jump directly to a help topic for the selected item. So, for example, if you’d like to learn more about the Change event of the ListBox, just bring up the Object Browser, select the appropriate library, class, and member, and press the Help button. You’ll get the help topic shown in Figure 99.23.
Figure 99.23 Help launched from the Object Browser
Copyright ©2001 SYBEX, Inc., Alameda, CA |
www.sybex.com |